If you are a developer who works with APIs, you might have heard of gRPC, a modern, high-performance framework for building and consuming services. But what is gRPC, and how does it compare to the traditional HTTP-based approach? In this blog post, I will explain the differences between gRPC and HTTP, and why you should consider using gRPC for your next API project.
What is gRPC?
gRPC is an open-source framework that enables efficient, reliable, and scalable communication between services. It is based on the Remote Procedure Call (RPC) model, which means that you can define the operations and data structures of your service in a language-neutral and platform-independent way, and then generate code for both the server and the client in your preferred programming language. gRPC uses HTTP/2 as the underlying transport protocol, which offers many benefits over HTTP/1.1, such as multiplexing, binary framing, header compression, and server push. gRPC also uses Protocol Buffers, a compact and fast binary format for serializing data, which reduces the network overhead and improves the performance of your service.
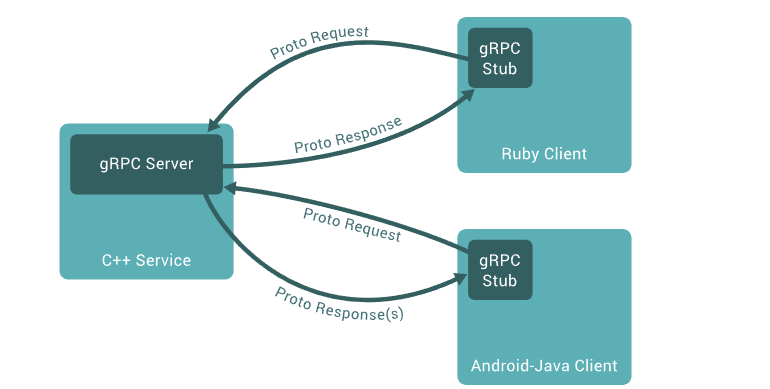
What is HTTP?
HTTP, or Hypertext Transfer Protocol, is the most widely used protocol for transferring data over the web. It is based on the Representational State Transfer (REST) model, which means that you can expose the resources of your service as URLs, and use different HTTP methods (such as GET, POST, PUT, and DELETE) to manipulate them. HTTP uses a human-readable text format for sending and receiving data, such as JSON or XML, which makes it easy to debug and understand. HTTP/1.1 is the most common version of HTTP, but it has some limitations, such as the need for multiple TCP connections, the overhead of textual headers, and the lack of support for streaming and bidirectional communication.
Why gRPC is Better than HTTP
There are many reasons why gRPC is a better choice than HTTP for building and consuming APIs. Here are some of the main advantages of gRPC over HTTP:
Performance
gRPC is faster and more efficient than HTTP in terms of request-response time and resource consumption. This is because gRPC uses HTTP/2, which allows multiple requests and responses to be sent over a single TCP connection, eliminating the latency and overhead of opening and closing connections. gRPC also uses Protocol Buffers, which are smaller and faster to serialize and deserialize than JSON or XML, reducing the network bandwidth and CPU usage. gRPC also supports compression, which further reduces the data size and improves the speed of your service.
Reliability
gRPC is more reliable than HTTP in terms of error handling and fault tolerance. This is because gRPC has built-in features for detecting and recovering from failures, such as timeouts, retries, load balancing, and health checks. gRPC also supports streaming, which allows you to send and receive data in chunks, rather than as a single message, which makes it easier to handle large or complex data and avoid timeouts or memory issues. gRPC also supports bidirectional communication, which enables you to send and receive data at the same time, and implement push notifications or real-time updates.
Flexibility
gRPC is more flexible than HTTP in terms of compatibility and extensibility. This is because gRPC is language-neutral and platform-independent, which means that you can use any programming language and any operating system to build and consume your service, and easily integrate with existing or new systems. gRPC also supports custom metadata, which allows you to attach additional information to your requests and responses, such as authentication tokens, tracing IDs, or user preferences. gRPC also supports interceptors, which allow you to modify or enhance the behavior of your service, such as logging, monitoring, or validation.
Comparison table between gRPC and HTTP
Feature | gRPC | HTTP |
---|---|---|
Protocol | gRPC uses HTTP/2 as the underlying protocol | HTTP uses HTTP/1.1 or HTTP/2 |
Data Serialization | Uses Protocol Buffers (protobuf) by default, which is a binary serialization format. Supports other formats like JSON | Typically uses JSON, XML, or other text-based formats |
Performance | Generally faster due to binary format and multiplexing | Slower compared to gRPC due to text-based format and lack of multiplexing |
Streaming | Supports both unary (request-response) and bidirectional streaming | Supports streaming but typically not as efficient as gRPC |
Error Handling | Uses gRPC status codes for errors, which are more detailed and structured | Uses HTTP status codes, which are less detailed |
Security | Built-in support for Transport Layer Security (TLS) | Requires additional configuration for security |
Language Support | Supports multiple languages through auto-generated client and server code | Widely supported in almost all programming languages |
Tooling | Provides a rich set of tools for debugging and monitoring | Limited tooling compared to gRPC |
Use Cases | Best suited for microservices and inter-service communication | Suitable for web applications and APIs |
Adoption | Growing adoption, especially in microservices architectures | Widely adopted and established |
How to Get Started with gRPC
If you are interested in using gRPC for your next API project, you can get started by following these steps:
- Install the gRPC tools and libraries for your preferred programming language. You can find the official documentation and tutorials for each language on the gRPC website.
- Define your service and data structures using the Protocol Buffers syntax in a .proto file. You can find the reference and examples for the Protocol Buffers syntax on the Protocol Buffers website.
- Generate the server and client code from the .proto file using the gRPC tools. You can customize the code generation options and plugins according to your needs and preferences.
- Implement the server logic and the client logic using the generated code and the gRPC libraries. You can use the gRPC APIs and features to create, send, and receive requests and responses, and handle errors and failures.
- Run and test your service and client using the gRPC tools and libraries. You can use the gRPC command-line tools, such as grpcurl or grpc_cli, to interact with your service, or use the gRPC testing frameworks, such as grpc-java-testing or grpc-go-testing, to write and run unit tests and integration tests.
How to Use gRPC with apidog?
Apidog is a tool that helps you design, document, and test your APIs. You can use apidog to create interactive documentation for your gRPC APIs and share it with your team or clients. You can also use apidog to generate mock servers and clients for your gRPC APIs and test them in various scenarios.
Server Streaming
Server Streaming, as the name implies, involves sending multiple response data in a single request. For instance, it could involve subscribing to all the transaction price data of stocks within a one-minute timeframe.
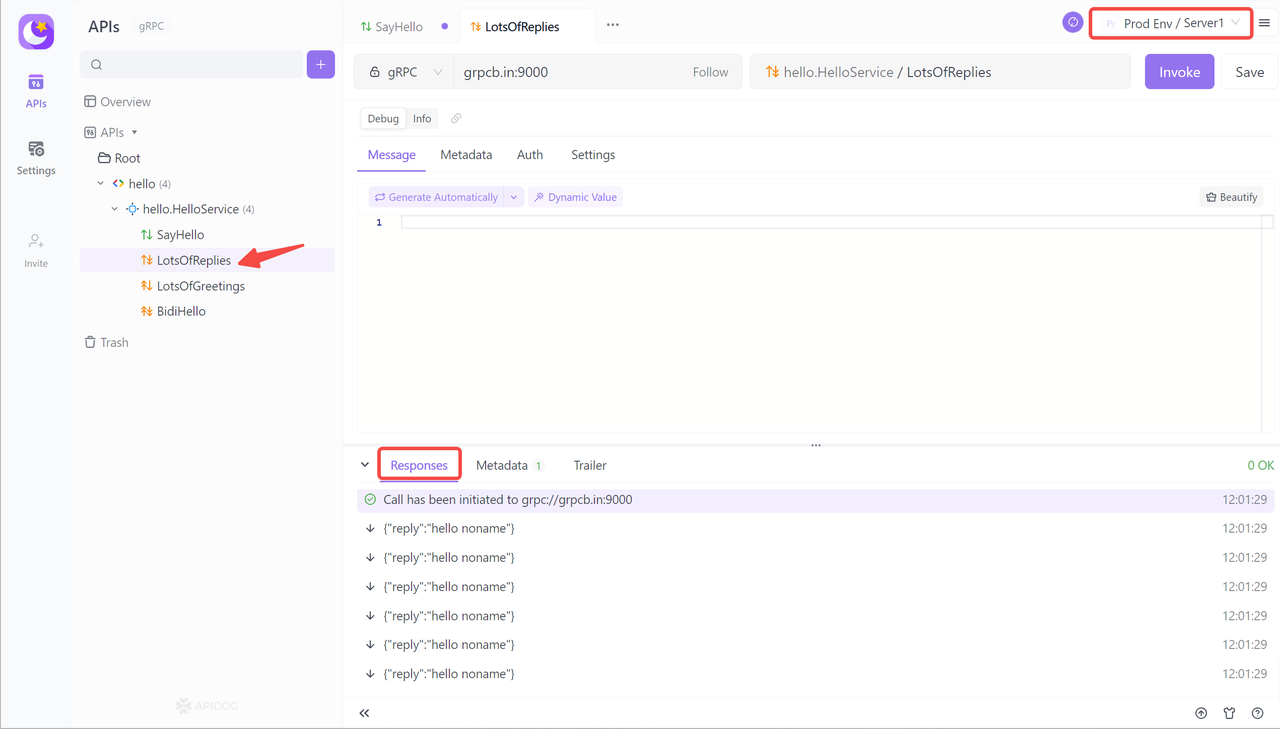
Client Streaming
In this mode, the client can continuously send multiple request messages to the server without waiting for immediate responses. After processing all the requests, the server sends a single response message back to the client. This approach is well-suited for efficiently transmitting large amounts of data in a streaming manner, which helps reduce latency and optimize data exchange.
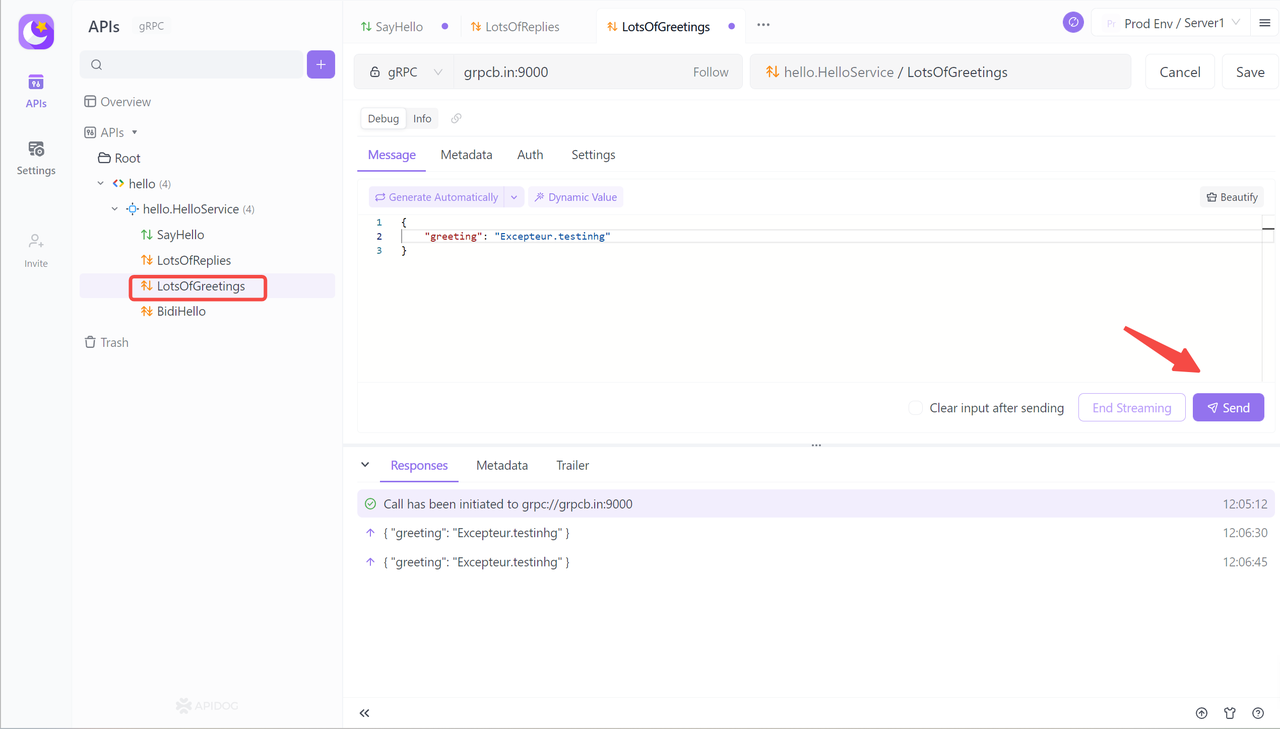
Bidirectional Streaming
Bidirectional Streaming enables clients and servers to establish persistent bidirectional communication and transmit multiple messages simultaneously. It is commonly employed in online games and real-time video call software, and is well-suited for real-time communication and large-scale data transmission scenarios. After initiating the call, the client and the server maintain a session between them and receive real-time responses after sending different request contents.
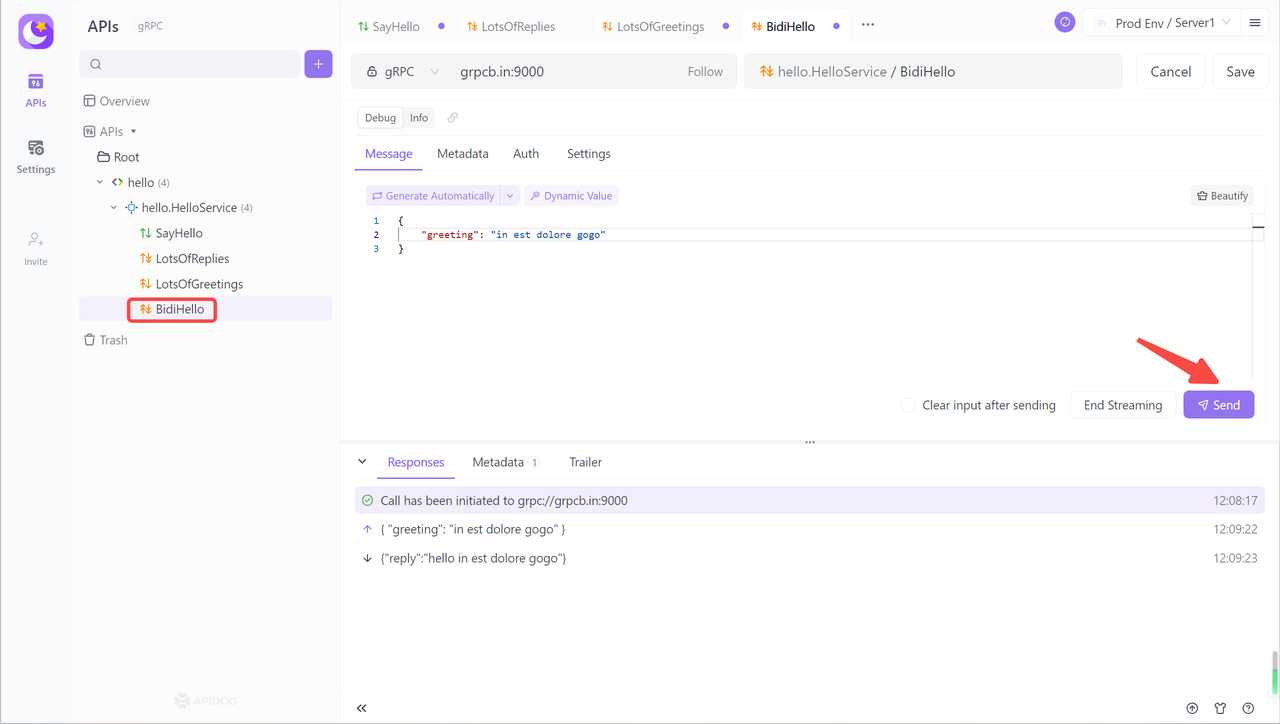
Collaborating on gRPC APIs
Apidog can generate human-readable gRPC interface documents from .proto files, facilitating team collaboration on interfaces. Click the menu button on the right side of the interface to obtain the collaboration link and share it with other team members to align the interface debugging approach.
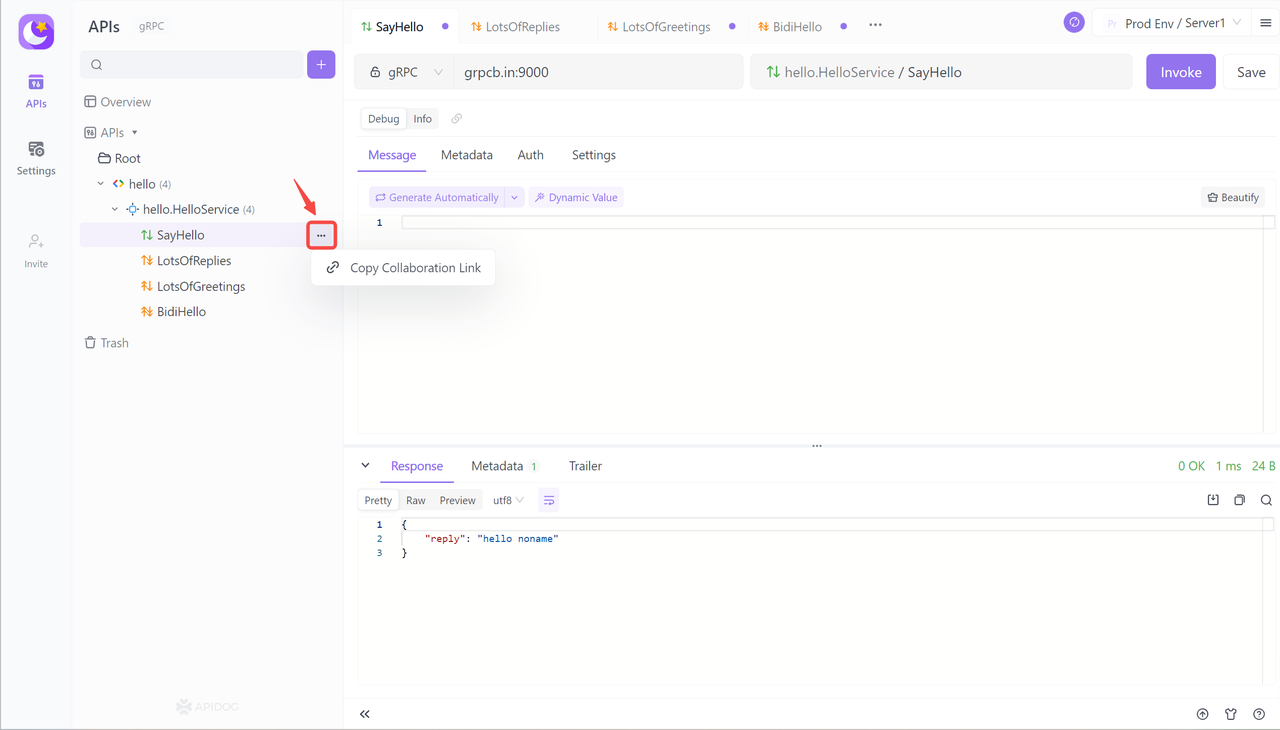
Go to the test tab and create test cases and scenarios for your gRPC API. You can use the mock server and client or the real server and client to test your API. You can also use assertions, variables, hooks, etc. to enhance your tests. You can run your tests and see the results and reports in the test panel.
Conclusion
gRPC is a modern, high-performance framework for building and consuming services. It offers many benefits over HTTP, such as performance, reliability, and flexibility. If you are looking for a fast, reliable, and flexible way to create and consume APIs, you should consider using gRPC for your next project. You can learn more about gRPC and how to use it on the gRPC website or on the Apidog website, where you can find more articles, tutorials, and resources about gRPC and other API technologies.