When it comes to building APIs, speed and efficiency are everything. Developers are always on the lookout for tools that can deliver results faster, with minimal overhead. Enter FastAPI, a modern, fast (hence the name) web framework for building APIs with Python 3.7+ based on standard Python-type hints. But today, we’re not just talking about FastAPI in general—we’re diving into something more specific: FastAPI Streaming Response. If you’re looking to handle large datasets or real-time data transmission, streaming responses are your new best friend.
What is FastAPI?
Before we dive into the nitty-gritty of streaming responses, let's briefly revisit what FastAPI is all about. FastAPI is a web framework built on top of Starlette for the web parts and Pydantic for the data parts. The beauty of FastAPI lies in its speed—it's one of the fastest Python frameworks available, nearly as fast as NodeJS and Go. It's designed to help developers build APIs quickly and efficiently, with a minimal amount of code.
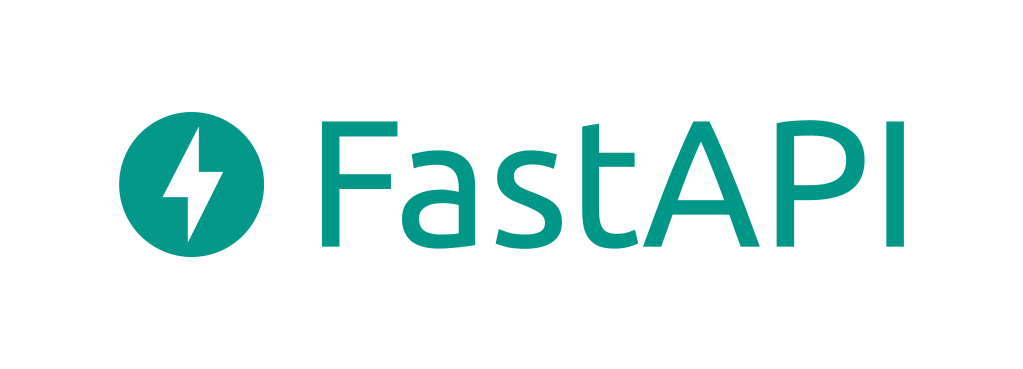
Why FastAPI?
If you’ve worked with frameworks like Django or Flask, you know they’re powerful, but they can be slow and cumbersome when dealing with complex APIs. FastAPI, on the other hand, is optimized for performance, allowing you to write clean, type-annotated Python code that is both efficient and easy to understand. With its asynchronous capabilities, it’s perfectly suited for modern applications that require real-time data processing.
But let’s cut to the chase—what exactly is a streaming response, and why should you care?
Understanding Streaming Responses
What is a Streaming Response?
A streaming response allows you to send parts of your response back to the client while the rest of your data is still being processed. This is incredibly useful when dealing with large datasets or real-time data that needs to be delivered to the client as soon as it becomes available.
Think of it like watching a live sports event online. Instead of waiting for the entire event to finish before you can watch it, the video streams to your device in real-time, allowing you to watch as the action unfolds. Similarly, with streaming responses in FastAPI, your API can start sending data to the client as soon as it’s ready, without waiting for the entire dataset to be processed.
Why Use Streaming Responses?
There are several scenarios where streaming responses are not just useful but essential:
- Handling Large Files: When you need to send large files (like videos or datasets) to a client, streaming them reduces the memory load on your server and speeds up delivery.
- Real-Time Data: For applications like chat systems, live sports updates, or financial tickers, real-time data is crucial. Streaming allows data to be sent as soon as it’s available, ensuring the client receives the most up-to-date information.
- Improved User Experience: By sending data in chunks, the user can start processing or viewing data immediately, without waiting for the entire response. This improves the perceived speed and responsiveness of your application.
Now that we’ve covered the basics, let’s look at how you can implement streaming responses in FastAPI.
Implementing Streaming Responses in FastAPI
Basic Setup
First things first, make sure you have FastAPI installed. You can do this by running:
pip install fastapi
pip install uvicorn
You’ll also need Uvicorn, an ASGI server for serving your FastAPI app. Once that’s set up, let’s dive into some code.
A Simple Example
Here’s a basic example of how to implement a streaming response in FastAPI:
from fastapi import FastAPI
from fastapi.responses import StreamingResponse
import time
app = FastAPI()
def fake_video_streamer():
for i in range(10):
yield f"frame {i}\n"
time.sleep(1)
@app.get("/video")
async def video():
return StreamingResponse(fake_video_streamer(), media_type="text/plain")
In this example, the fake_video_streamer
function simulates a video stream by generating a new frame every second. The StreamingResponse
class is used to send these frames to the client as they’re generated, rather than waiting for all frames to be ready.
Breaking It Down
- fake_video_streamer(): This generator function simulates the creation of video frames. Each
yield
sends a new chunk of data to the client. - StreamingResponse: This FastAPI class takes a generator (or any iterable) as input and streams it to the client. The
media_type
parameter defines the type of data being sent—in this case, plain text.
Real-World Application: Streaming a Large File
Streaming small text responses is one thing, but what if you need to send a large file? Here’s how you can do that:
from fastapi import FastAPI
from fastapi.responses import StreamingResponse
app = FastAPI()
def file_reader(file_path):
with open(file_path, "rb") as file:
while chunk := file.read(1024):
yield chunk
@app.get("/download")
async def download_file():
file_path = "large_file.zip"
return StreamingResponse(file_reader(file_path), media_type="application/octet-stream")
In this example, the file_reader
function reads a large file in chunks of 1024 bytes and streams it to the client.
Optimizing Streaming Responses
Managing Memory Usage
One of the primary benefits of streaming responses is reduced memory usage. However, if not handled properly, streaming can still consume a lot of memory, especially when dealing with multiple clients or very large datasets.
- Chunk Size: The size of each chunk you stream can have a significant impact on performance. Larger chunks mean fewer requests but more memory usage. Smaller chunks reduce memory usage but increase the number of requests. Finding the right balance is key.
- Lazy Loading: If your data source supports it, use lazy loading techniques to load data only as it’s needed, rather than loading everything into memory at once.
Asynchronous Streaming
FastAPI’s asynchronous nature makes it well-suited for streaming responses. By using async
and await
, you can ensure that your streaming doesn’t block other parts of your application, allowing you to handle multiple clients simultaneously without sacrificing performance.
Here’s an example:
from fastapi import FastAPI
from fastapi.responses import StreamingResponse
import aiofiles
app = FastAPI()
async def async_file_reader(file_path):
async with aiofiles.open(file_path, 'rb') as file:
while chunk := await file.read(1024):
yield chunk
@app.get("/async-download")
async def async_download_file():
file_path = "large_file.zip"
return StreamingResponse(async_file_reader(file_path), media_type="application/octet-stream")
In this example, we use aiofiles
to read the file asynchronously, ensuring that the server can continue processing other requests while the file is being streamed.
Using Background Tasks
Sometimes, you might want to process data in the background while streaming the response. FastAPI’s background tasks are perfect for this.
from fastapi import FastAPI, BackgroundTasks
from fastapi.responses import StreamingResponse
app = FastAPI()
def background_data_processor():
# Process data in the background
pass
def data_streamer():
for i in range(10):
yield f"data {i}\n"
@app.get("/data")
async def stream_data(background_tasks: BackgroundTasks):
background_tasks.add_task(background_data_processor)
return StreamingResponse(data_streamer(), media_type="text/plain")
In this example, the background_data_processor
function processes data in the background while data_streamer
streams the response to the client.
Challenges and Considerations
Error Handling
When dealing with streaming responses, error handling becomes crucial. Since the data is being sent in chunks, any error that occurs during the process can result in an incomplete or corrupted response.
- Graceful Shutdowns: Ensure that your application can handle shutdowns gracefully, completing or aborting streams as necessary.
- Client Disconnects: Be prepared for scenarios where the client disconnects mid-stream. Your application should detect this and clean up resources accordingly.
Security Considerations
Streaming responses can introduce security challenges, especially when dealing with large files or sensitive data.
- Rate Limiting: Implement rate limiting to prevent abuse, especially for public APIs.
- Data Validation: Ensure that all data being streamed is properly validated and sanitized to prevent injection attacks.
Best Practices for FastAPI Streaming Responses
Use Appropriate Media Types
Always specify the correct media_type
when using StreamingResponse
. This not only helps the client understand how to handle the data but can also improve performance by allowing the client to process data more efficiently.
Monitoring and Logging
Streaming responses can be tricky to debug, especially when things go wrong. Implement thorough logging and monitoring to track the performance of your streaming endpoints and quickly identify any issues.
Advanced Techniques: Combining Streaming with WebSockets
For more advanced use cases, you can combine FastAPI streaming responses with WebSockets. This combination allows you to create highly interactive real-time applications, such as live dashboards, multiplayer games, or collaborative tools.
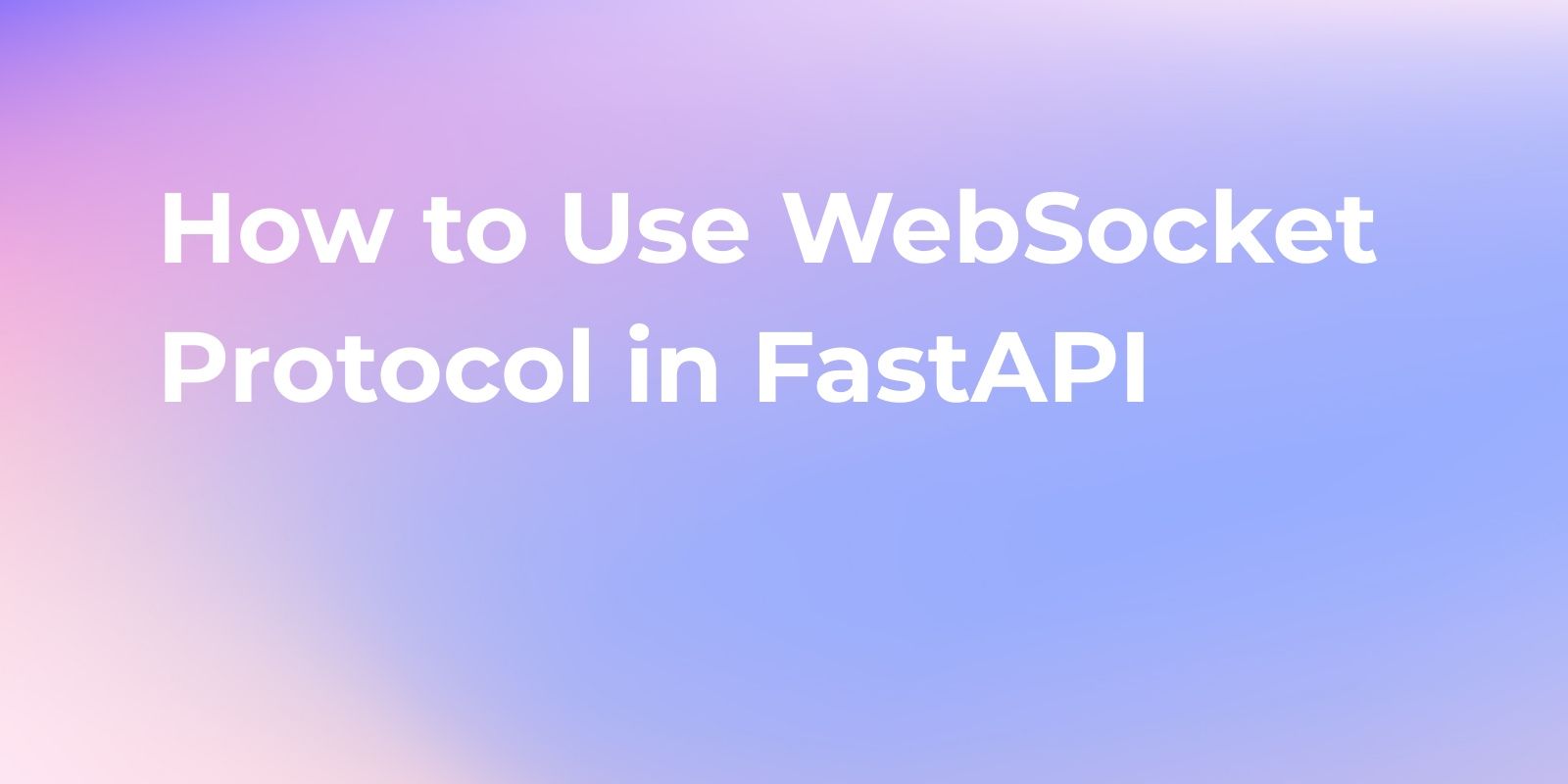
Example: Streaming with WebSockets
Here’s a simple example of how you can use WebSockets for a real-time chat application:
from fastapi import FastAPI, WebSocket
from fastapi.responses import StreamingResponse
app = FastAPI()
clients = []
@app.websocket("/ws")
async def websocket_endpoint(websocket: WebSocket):
await websocket.accept()
clients.append(websocket)
try:
while True:
data = await websocket.receive_text()
for client in clients:
await client.send_text(f"Message: {data}")
except Exception as e:
clients.remove(websocket)
Why Use WebSockets with Streaming?
- Bidirectional Communication: WebSockets allow for real-time, two-way communication between the client and server, which is perfect for applications like chat or live updates.
- Low Latency: WebSockets are designed for low-latency communication, making them ideal for time-sensitive applications.
Combining WebSockets with streaming responses enables you to build highly interactive and efficient real-time applications.
Conclusion
FastAPI’s streaming responses offer a powerful tool for handling real-time data, large files, and improving overall API performance. Whether you’re building a live sports application, a financial data service, or just need to send large files efficiently, FastAPI has you covered.
And don’t forget—if you’re looking to take your API development to the next level, check out Apidog. It’s a powerful tool that can streamline your FastAPI projects and much more. Download it for free and see how it can make your development process smoother and more efficient.