Django is a high-level Python web framework that is designed to help developers build web applications quickly and easily. It is free, open-source, and has a large and active community of developers.
In this blog post, we will focus on one of the most important aspects of Django - handling GET requests and how to get requests with Django using Apidog, a fantastic tool for API development and testing, to make your life easier.
Ready to experience the future of API development? Download Apidog for free and start testing it now!
What is Django?
Django is a powerful and popular web framework that allows you to create amazing web applications with ease. One of the most common tasks that you will encounter as a Django developer is handling get requests from your users. A get request is a way of sending data to the server through the URL. For example, if you want to search for something on Google, you can use a get request like this:
https://www.google.com/search?q=django
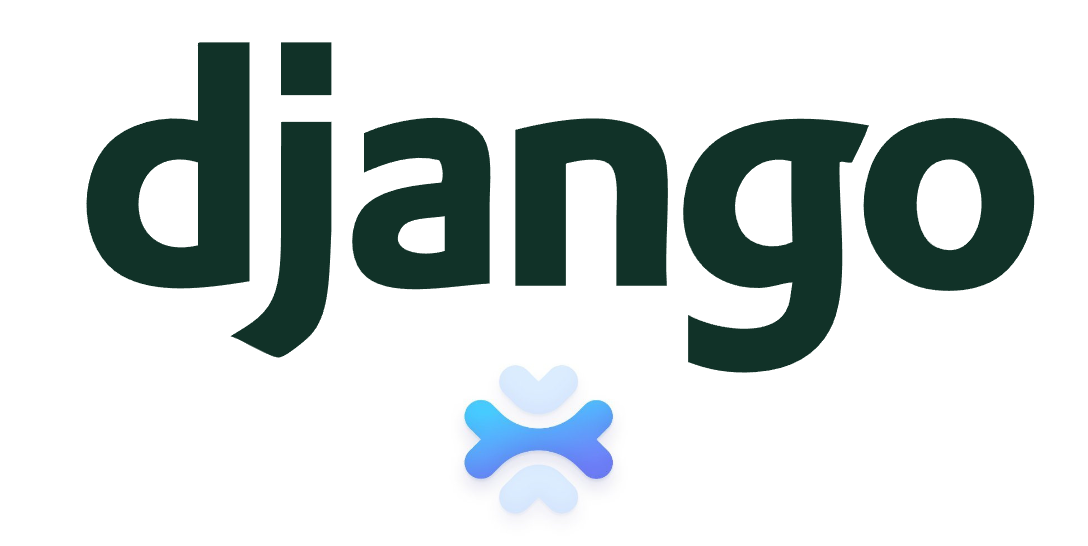
HTTP Request Basics
Before we dive into Django GET request, let's review some basic concepts of HTTP requests. HTTP stands for Hypertext Transfer Protocol, and it is the standard way of communicating between a web browser and a web server. HTTP requests are messages that the browser sends to the server, asking for some data or action. HTTP responses are messages that the server sends back to the browser, providing the requested data or action.
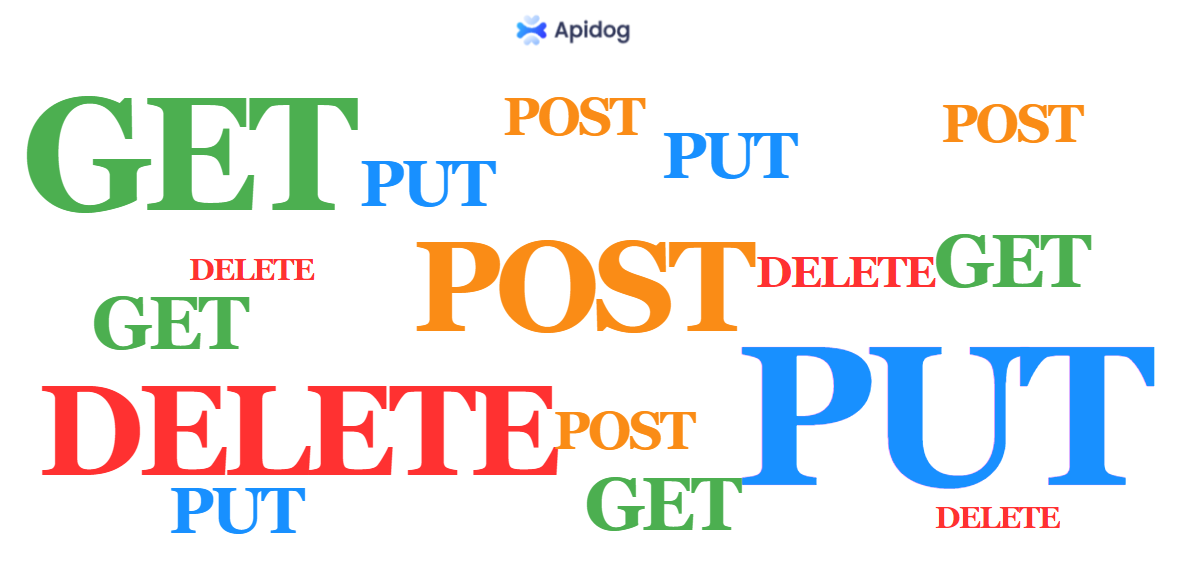
There are different types of HTTP requests, also known as HTTP methods, that indicate the purpose and nature of the request. The most common HTTP methods are:
- GET: used to request data from the server, such as a web page, an image, or a JSON file. The GET method does not modify any data on the server, it only retrieves it.
- POST: used to send data to the server, such as a form submission, a file upload, or a login request. The POST method usually modifies data on the server, such as creating, updating, or deleting a resource.
- PUT: used to update or replace data on the server, such as modifying an existing resource. The PUT method is similar to POST, but it is more specific and idempotent, meaning that multiple identical requests will have the same effect as a single request.
- DELETE: used to delete data from the server, such as removing a resource. The DELETE method is also idempotent, meaning that multiple identical requests will have the same effect as a single request.
Understanding the Basics of GET Requests in Django
In web development, GET and POST are the two most commonly used HTTP methods. While POST is primarily used for sending data to the server, GET is used to retrieve data from the server. A get request is one of the most common types of HTTP requests that you can use to communicate with a web server. For example, if you want to get the latest news from a website, you can use a get request like this:
https://www.example.com/news
A get request can also include parameters that specify what kind of data you want to get. For example, if you want to get the news for a specific category, you can use a get request like this:
https://www.example.com/news?category=sports
When a user clicks on a link or submits a form with the method set to GET, a GET request is sent to the server. The server then processes the request and returns the requested data to the user. In Django, handling GET requests involves capturing the parameters passed in the URL and using them to retrieve the relevant data from the database or perform certain actions.
Now that you know what a get request is and why use it, let’s see how to get data from get requests in Django.
Setting Up Your Django Project for GET Requests
To begin handling GET requests in Django, you first need to set up your Django project. If you're new to Django, don't worry! We'll guide you through the process step by step.
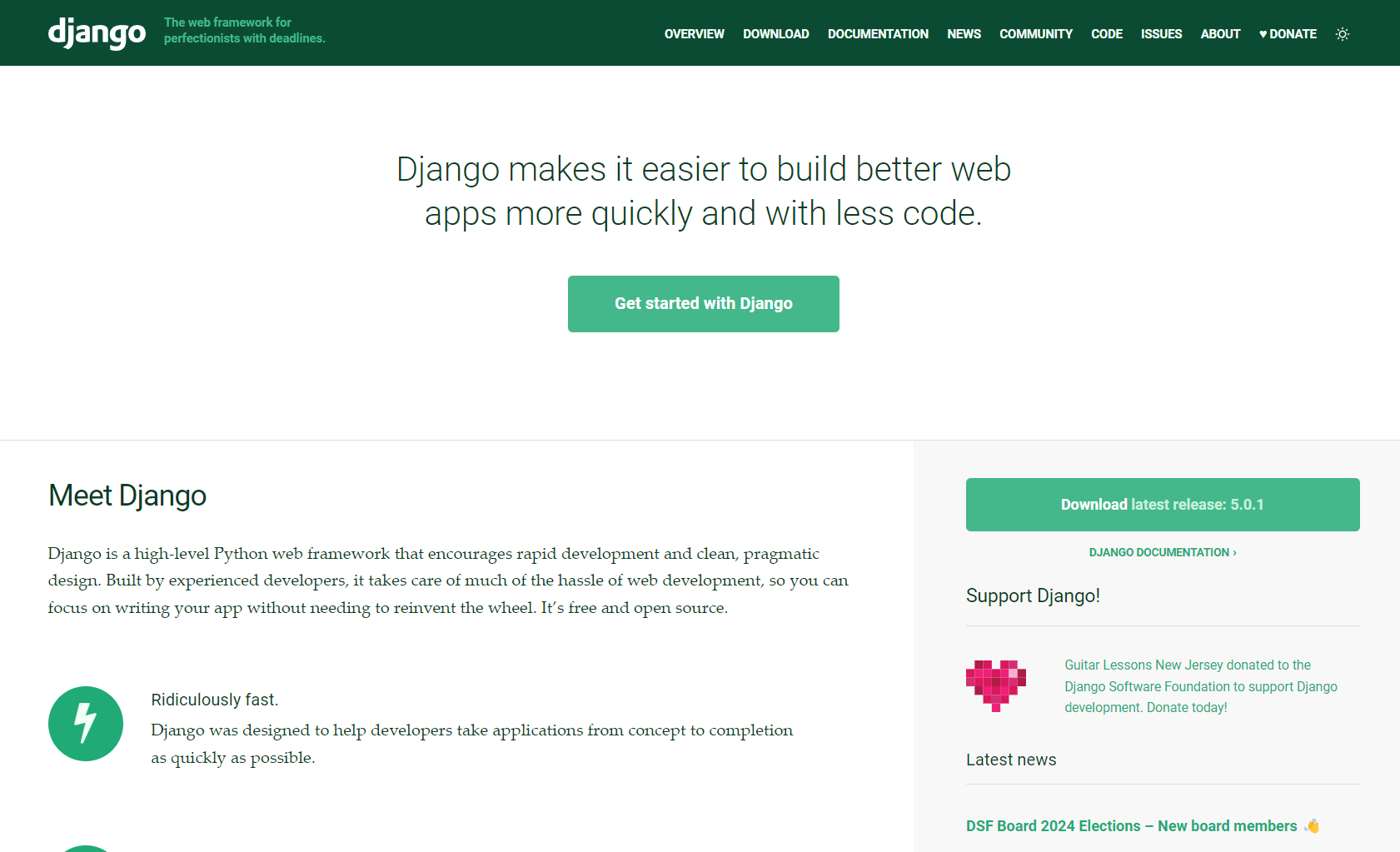
- Install Django: Start by installing Django on your system. You can do this by running the following command in your terminal:
pip install Django
- Create a Django Project: Once Django is installed, create a new Django project by running the following command:
django-admin startproject myproject
- Create a Django App: After creating the project, navigate to the project's directory and create a new Django app:
cd myproject
python manage.py startapp myapp
- Configure URL Routing: Next, you need to configure URL routing in your Django project. Open the
urls.py
file located in your project's directory and define the URL patterns for your app.
Implementing GET Requests in Django
Now that you have your Django project set up, let's dive into implementing GET requests. There are several ways to handle GET requests in Django, depending on the complexity of your application and the desired functionality. Here, we will cover two commonly used methods: using function-based views and class-based views.
Using Function-Based Views
Function-based views are the simplest way to handle GET requests in Django. They allow you to define a Python function that takes a request as an argument and returns a response. Here's an example of a function-based view that handles a GET request:
from django.http import HttpResponse
def my_view(request):
if request.method == 'GET':
# Retrieve data from the server
data = retrieve_data()
return HttpResponse(data)
In this example, the my_view
function checks if the request method is GET and retrieves the data from the server using the retrieve_data
function. It then returns an HTTP response containing the retrieved data.
Using Class-Based Views
Class-based views provide a more structured and reusable way to handle GET requests in Django. They allow you to define a class that inherits from Django's View
class and implement various methods for different HTTP methods. Here's an example of a class-based view that handles a GET request:
from django.views import View
from django.http import HttpResponse
class MyView(View):
def get(self, request):
# Retrieve data from the server
data = retrieve_data()
return HttpResponse(data)
In this example, the MyView
class defines a get
method that handles the GET request. Inside the get
method, you can retrieve the data from the server and return an HTTP response.
Advanced Techniques for Handling GET Requests in Django
Once you have a basic understanding of handling GET requests in Django, you can explore some advanced techniques to enhance your application's functionality. Here are a few techniques you can consider:
Query Parameters
Query parameters allow you to pass additional information in the URL and retrieve it in your Django view. For example, you can pass a search query as a query parameter and use it to filter the retrieved data. Here's an example of how to handle query parameters in Django:
def my_view(request):
search_query = request.GET.get('q')
if search_query:
# Filter data based on the search query
filtered_data = filter_data(search_query)
return HttpResponse(filtered_data)
In this example, the request.GET.get('q')
statement retrieves the value of the q
query parameter. If a search query is provided, the filter_data
function filters the data based on the query and returns the filtered data.
Working with APIs
Django provides powerful tools for working with APIs and consuming data from external sources. You can use Django's built-in requests
library or popular third-party libraries like requests
or http.client
to make API requests and retrieve data. Here's an example of how to retrieve data from an API in Django:
import requests
def my_view(request):
response = requests.get('https://api.example.com/data')
if response.status_code == 200:
data = response.json()
return HttpResponse(data)
else:
return HttpResponse("Error retrieving data")
In this example, the requests.get
function sends a GET request to the specified API endpoint. If the request is successful (status code 200), the retrieved data is returned as an HTTP response. Otherwise, an error message is returned.
How to Use Apidog to GET request in Django
Apidog is a tool that helps you design, debug, test, and document your APIs in a fast and fun way. Apidog is based on the concept of API design-first, which means that you start by defining the structure and behavior of your API before you write any code. This way, you can ensure that your API is consistent, clear, and easy to use.
Here’s how to use Apidog to send GET requests with params:
- Open Apidog, Click on the New Request button.
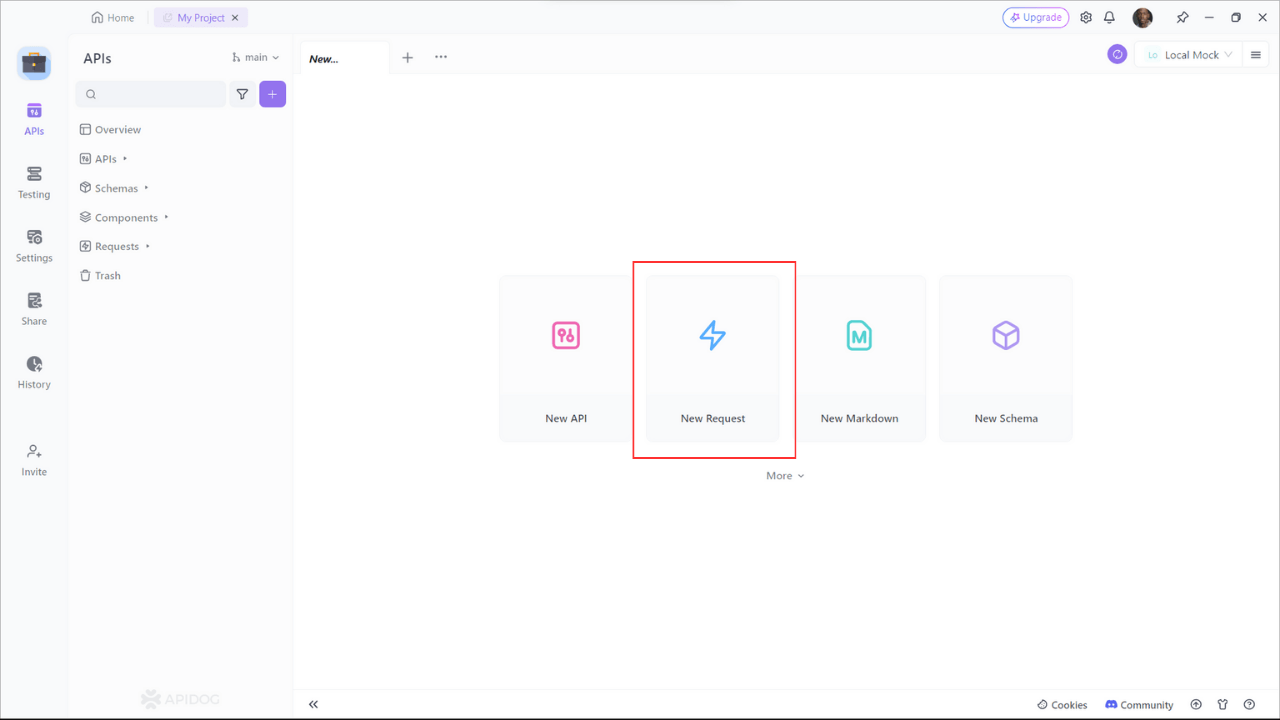
2. Enter the URL of the API endpoint you want to send a GET request to
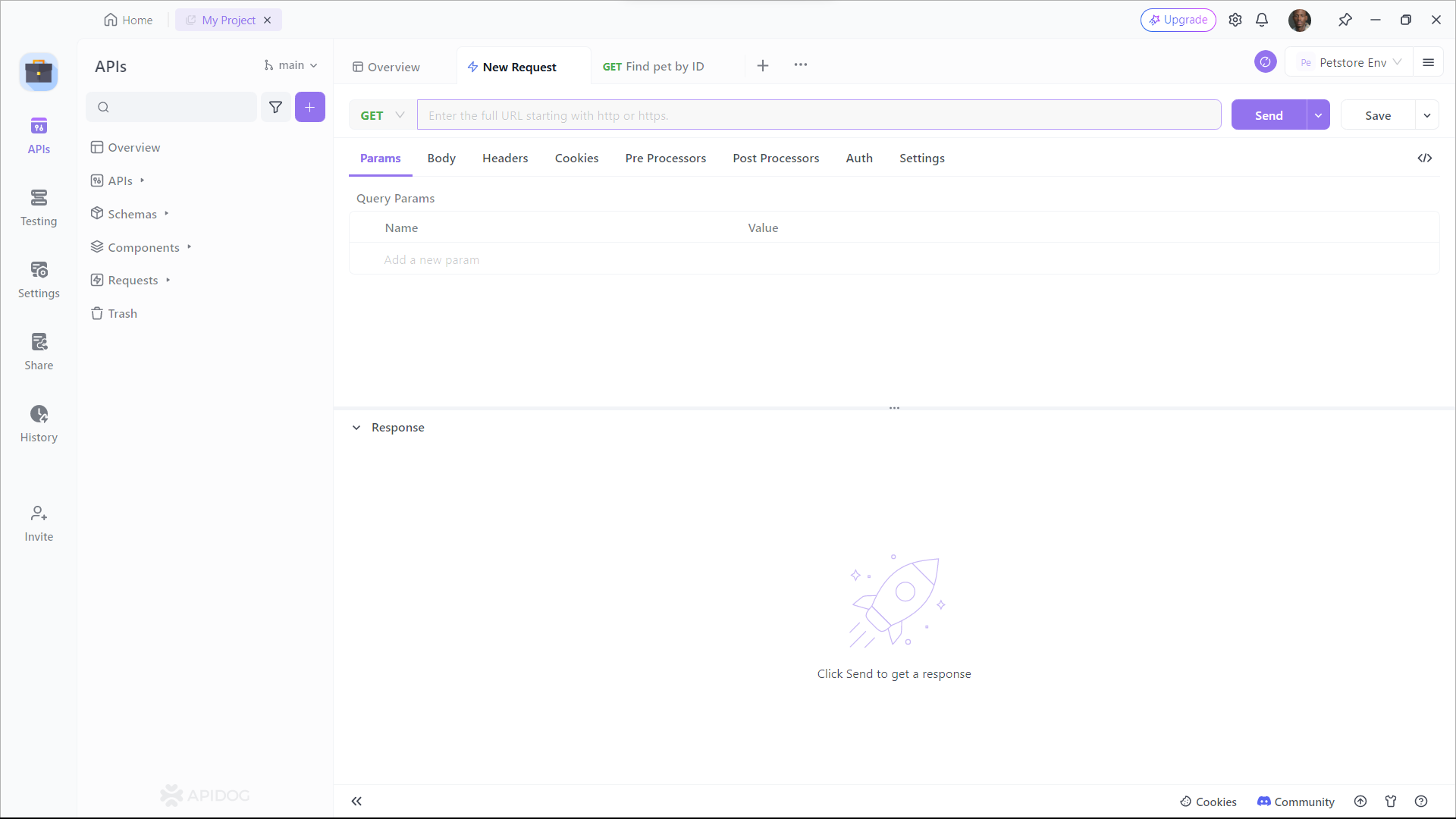
3. Click on the Send button to send the request and get the result
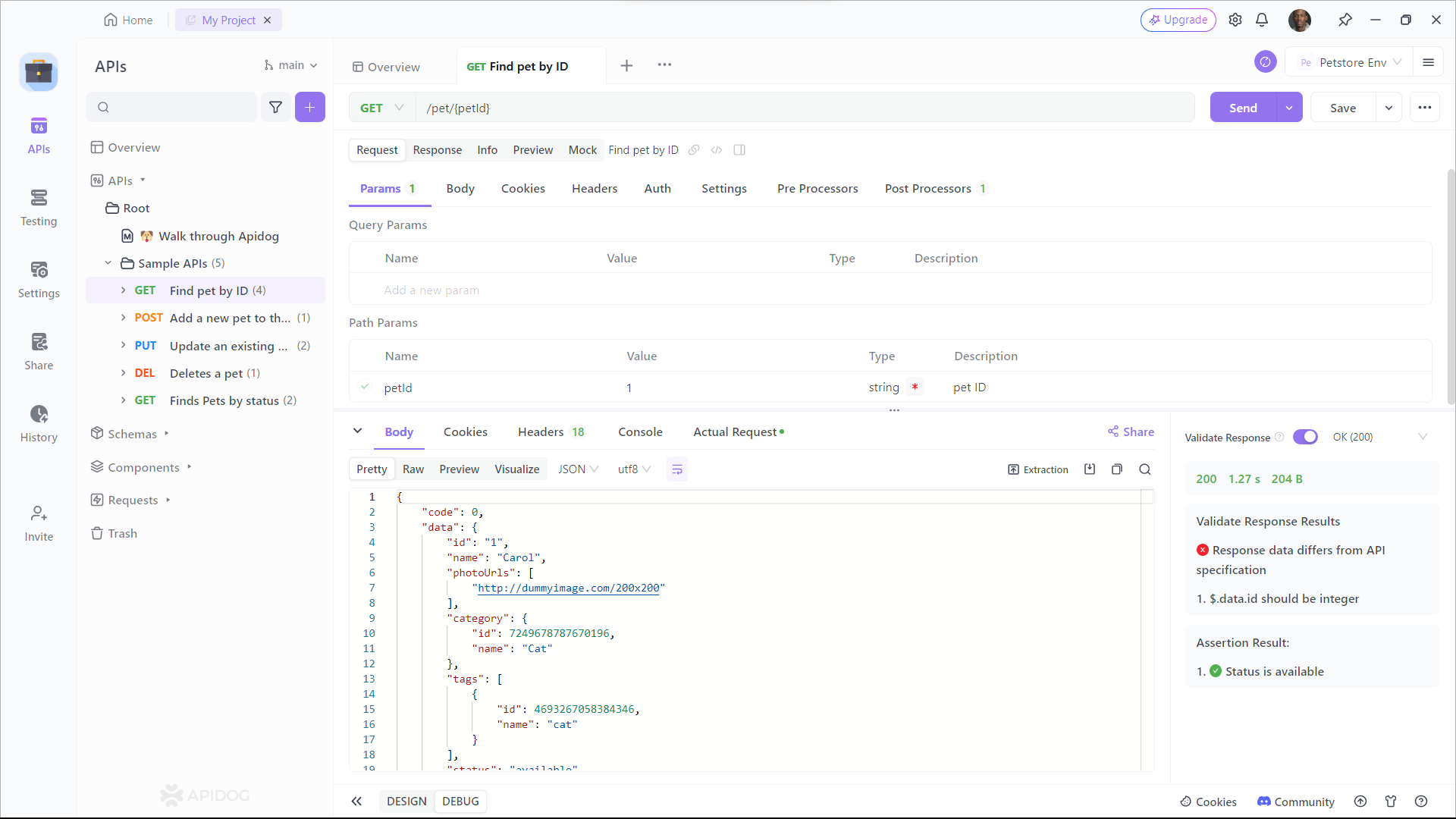
Conclusion
In this blog post, we have learned how to get data from get requests in Django and how to do it with Apidog, a fantastic tool for API development and testing, to make your life easier.
We hope that you have enjoyed this blog post and that you have learned something new and useful. If you want to try Apidog for yourself, you can sign up for a free account and start creating your own APIs.
Thank you for reading and happy coding!