Welcome to the fascinating world of WebSockets! Today, we’re diving deep into the often-debated topic of WS on HTTP vs WSS on HTTPS. If you're building an API or working with Apidog, understanding the differences and benefits of these two WebSocket protocols is crucial. Let's break it down in a friendly, conversational way.
What are WebSockets?
Before we jump into the nitty-gritty, let's briefly recap what WebSockets are. WebSockets are a communication protocol providing full-duplex communication channels over a single TCP connection. This essentially means that both the client and the server can send and receive messages simultaneously, making it perfect for real-time applications.
Understanding WS on HTTP
WebSocket (WS) operates over HTTP. It’s the standard, non-secure version of the WebSocket protocol. When you initiate a WebSocket connection using WS, your data is sent in plain text. This can be a suitable choice for applications where security isn't a major concern. However, in today's world, security is almost always a priority.
Benefits of WS on HTTP
- Simplicity: WS on HTTP is straightforward to implement and does not require the overhead of encryption.
- Lower Latency: Without the encryption overhead, you might experience slightly lower latency.
Drawbacks of WS on HTTP
- Security: The primary downside is the lack of encryption. Data is transferred in plain text, making it susceptible to eavesdropping and man-in-the-middle attacks.
- Trust Issues: Users might be wary of using applications that do not secure their data, potentially affecting user trust and engagement.
Diving into WSS on HTTPS
WebSocket Secure (WSS) operates over HTTPS. This means that your WebSocket connection is encrypted using SSL/TLS, the same protocols that secure your browsing on the web.
Benefits of WSS on HTTPS
- Enhanced Security: WSS encrypts the data transmitted between the client and the server, providing protection against eavesdropping and man-in-the-middle attacks.
- Trust: Users generally trust HTTPS connections more, as the "secure" indicator in browsers gives a sense of safety.
- Compliance: Many industries have regulatory requirements for data security that WSS can help meet.
Drawbacks of WSS on HTTPS
- Complexity: Implementing WSS requires managing SSL/TLS certificates, which adds complexity to your setup.
- Latency: The encryption process can introduce a slight latency compared to non-encrypted connections.
WS on HTTP vs WSS on HTTPS: A Comparative Analysis
Now that we understand the basics, let's compare WS on HTTP and WSS on HTTPS side-by-side.
Feature | WS on HTTP | WSS on HTTPS |
---|---|---|
Security | None | SSL/TLS Encryption |
Implementation | Simple | More Complex |
Latency | Lower | Slightly Higher |
User Trust | Lower | Higher |
Regulatory Compliance | Rarely sufficient | Often necessary |
When to Use WS on HTTP
WS on HTTP might be appropriate in scenarios where:
- Internal Networks: The application is running within a secure, internal network where the risk of interception is minimal.
- Performance Critical: Latency is a critical factor, and the slight overhead introduced by encryption is unacceptable.
- Prototyping: During the early stages of development or for prototyping purposes where security is not yet a priority.
When to Use WSS on HTTPS
On the other hand, WSS on HTTPS should be your choice when:
- Public Networks: The application operates over the internet, where data interception risks are higher.
- Sensitive Data: The application deals with sensitive data such as personal information, financial transactions, or health records.
- User Trust: Building trust with users is important, and a secure connection helps in achieving this.
- Compliance Requirements: Your application needs to comply with data protection regulations such as GDPR, HIPAA, etc.
How to Implement WS on HTTP
Implementing WS on HTTP is fairly straightforward. Here’s a simple example using Node.js:
const WebSocket = require('ws');
const server = new WebSocket.Server({ port: 8080 });
server.on('connection', socket => {
socket.on('message', message => {
console.log(`Received: ${message}`);
socket.send(`Hello, you sent -> ${message}`);
});
socket.send('Welcome to WebSocket over HTTP!');
});
How to Implement WSS on HTTPS
Implementing WSS on HTTPS involves a few more steps. You need to set up SSL/TLS certificates. Here’s an example:
const https = require('https');
const fs = require('fs');
const WebSocket = require('ws');
const server = https.createServer({
cert: fs.readFileSync('/path/to/cert.pem'),
key: fs.readFileSync('/path/to/key.pem')
});
const wss = new WebSocket.Server({ server });
wss.on('connection', socket => {
socket.on('message', message => {
console.log(`Received: ${message}`);
socket.send(`Hello, you sent -> ${message}`);
});
socket.send('Welcome to WebSocket over HTTPS!');
});
server.listen(8080);
Enhancing Your API with Apidog
If you're using Apidog to develop your API, integrating WebSockets can be a game-changer for real-time communication. Apidog provides tools to test and manage your WebSocket connections easily. Whether you choose WS on HTTP or WSS on HTTPS, Apidog can simplify the process.
Testing WS on HTTP with Apidog
Step 1: Create a new WebSocket request: In Apidog, set up a new WebSocket request using your WS endpoint.
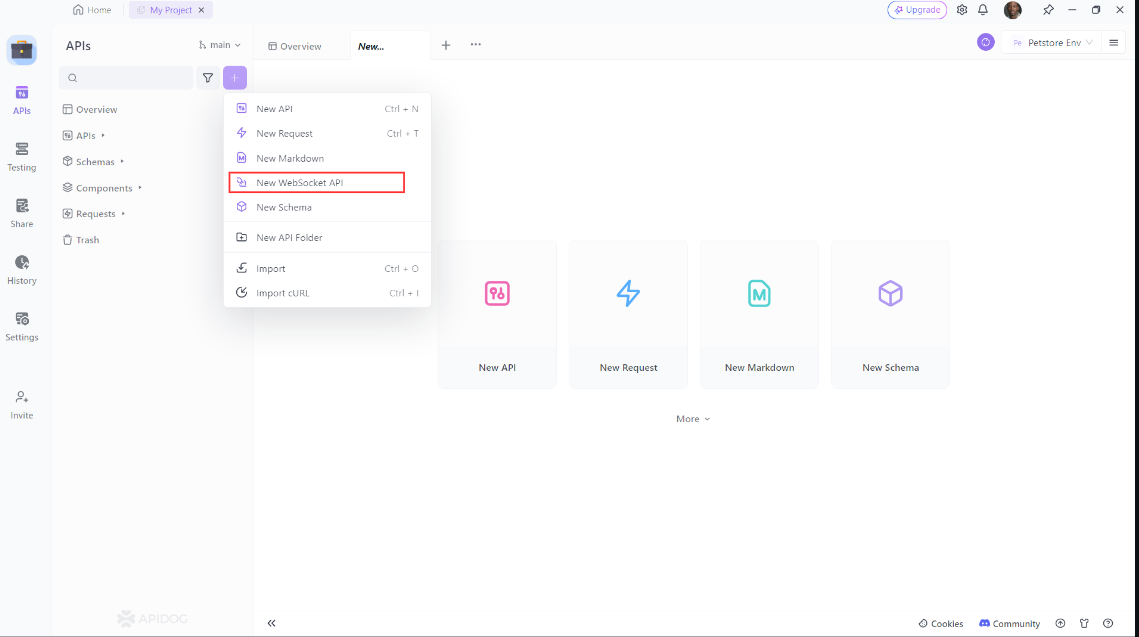
Step 2: Send Messages: Easily send messages and view responses in real-time.
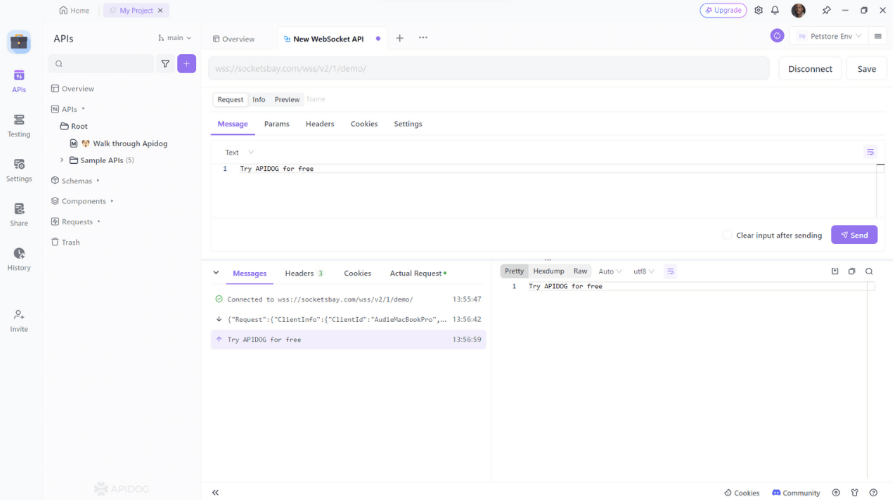
Step 3: Monitor Connections: Keep track of connection status and message flow.
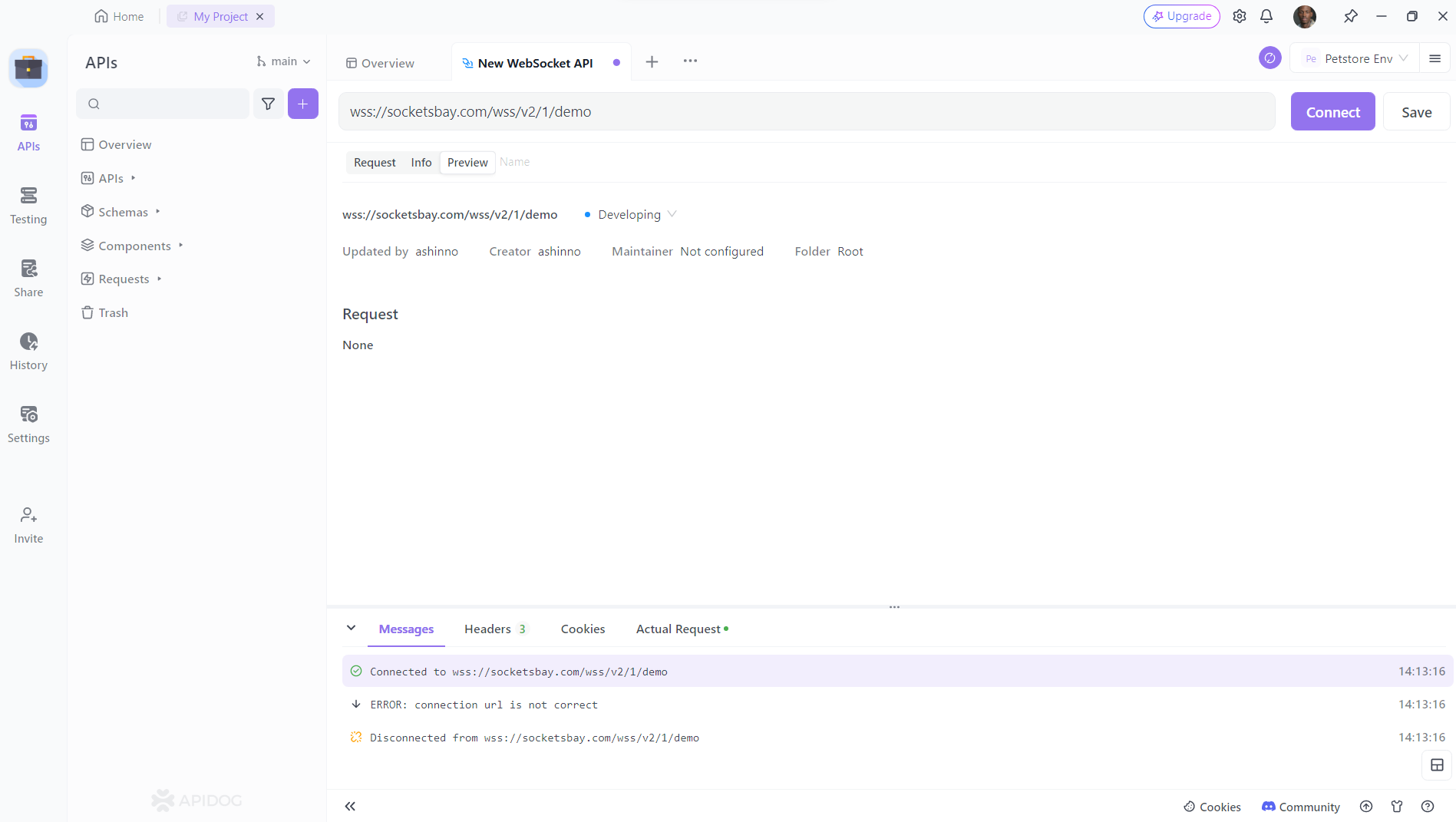
Testing WSS on HTTPS with Apidog
Step 1: Create WebSocket Request: Similar to WS on HTTP, but using the WSS endpoint.
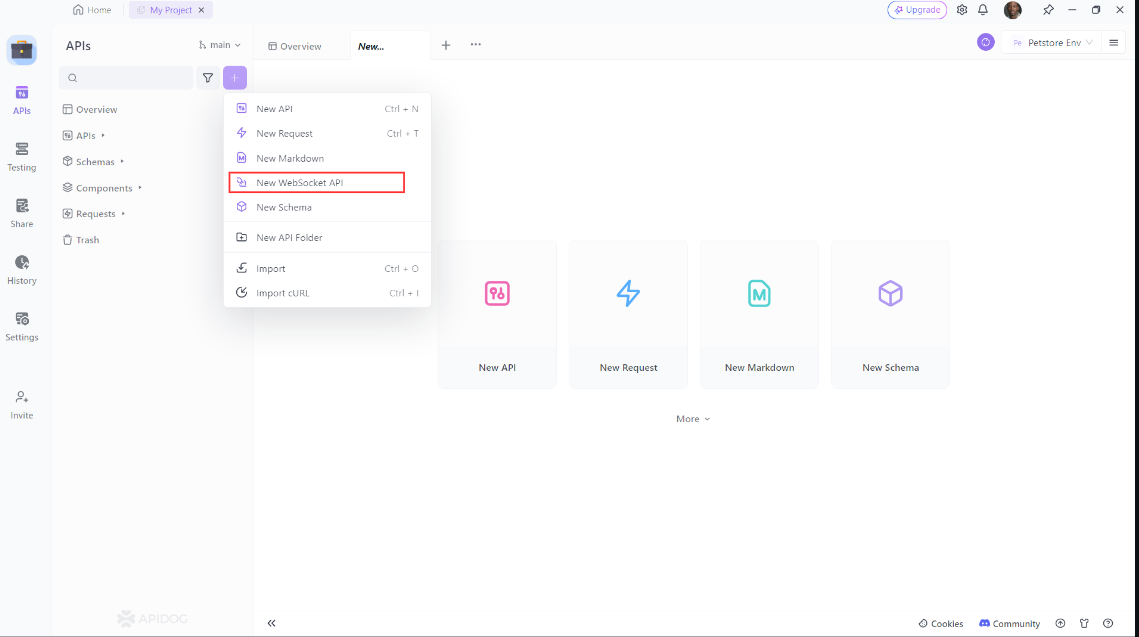
Step 2: Setup SSL/TLS: Ensure your server is configured with the necessary SSL/TLS certificates.
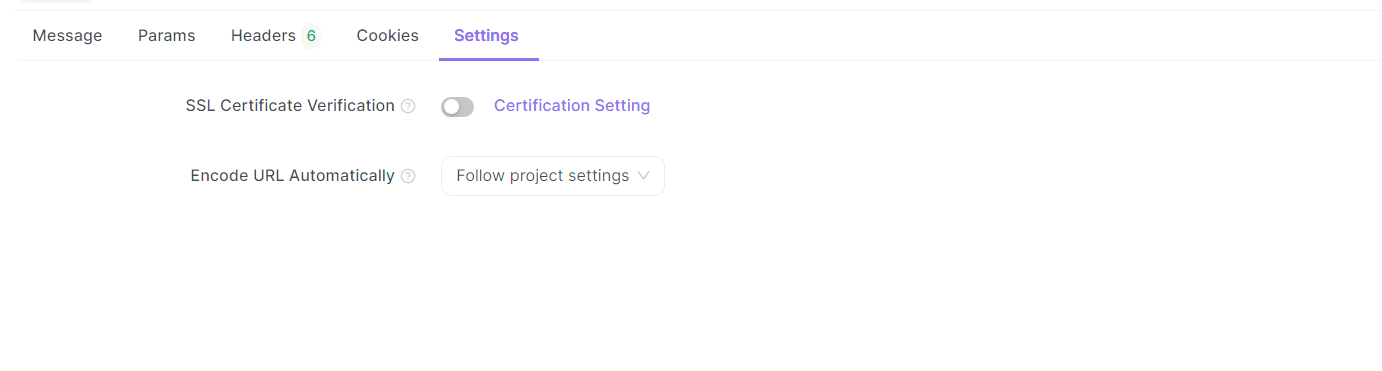
Step 3: Send Messages: Easily send messages and view responses in real-time.
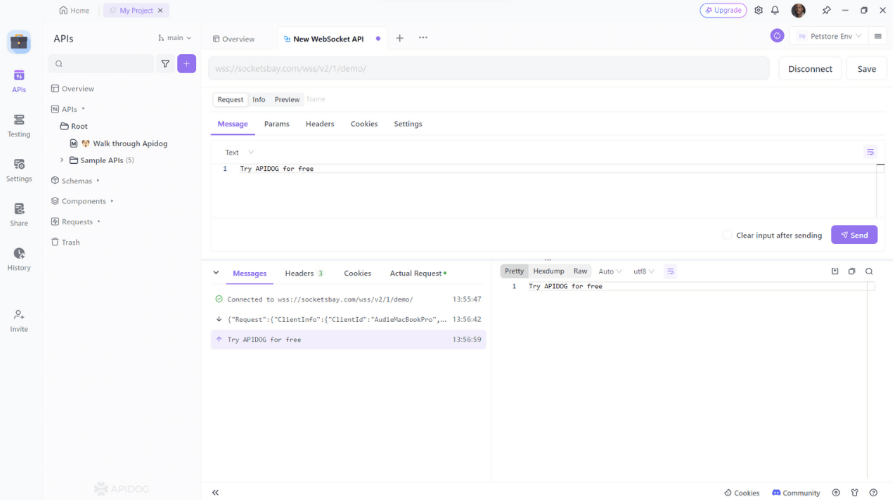
Conclusion: Making the Right Choice
In the debate of WS on HTTP vs WSS on HTTPS, the choice ultimately depends on your specific needs and context. For most modern applications, especially those handling sensitive data or operating over public networks, WSS on HTTPS is the preferred choice due to its enhanced security and user trust.
However, there are scenarios where WS on HTTP might be suitable, particularly in controlled environments where performance is critical, and security concerns are minimal.
By understanding the pros and cons of each protocol and leveraging tools like Apidog for development and testing, you can make an informed decision that best suits your application's requirements.