In the fast-paced world of web development, real-time communication is no longer a luxury—it’s a necessity. Imagine building a chat application where users don’t have to refresh the page to see new messages or creating a live dashboard where data updates automatically without any action from the user. Sounds cool, right? That's where SignalR comes in.
SignalR is a game-changer for developers who want to incorporate real-time features into their web applications. But what exactly is SignalR? How does it work? And why should you, as a developer, care about it? Let’s dive into the world of SignalR and explore how this technology can revolutionize the way you build web applications.
What is SignalR?
SignalR is a library for ASP.NET developers that simplifies the process of adding real-time web functionality to applications. This means that it allows server-side code to push content to connected clients instantly as it becomes available. You don’t need to rely on complex mechanisms like polling the server every few seconds for updates. Instead, SignalR provides a way for your server to notify your clients whenever something new happens.
SignalR was first introduced by Microsoft as part of the ASP.NET framework. Over the years, it has grown in popularity due to its simplicity and efficiency in handling real-time communication. Whether you’re building a chat app, a live dashboard, or a collaborative tool, SignalR makes it easier to add real-time features without breaking a sweat.
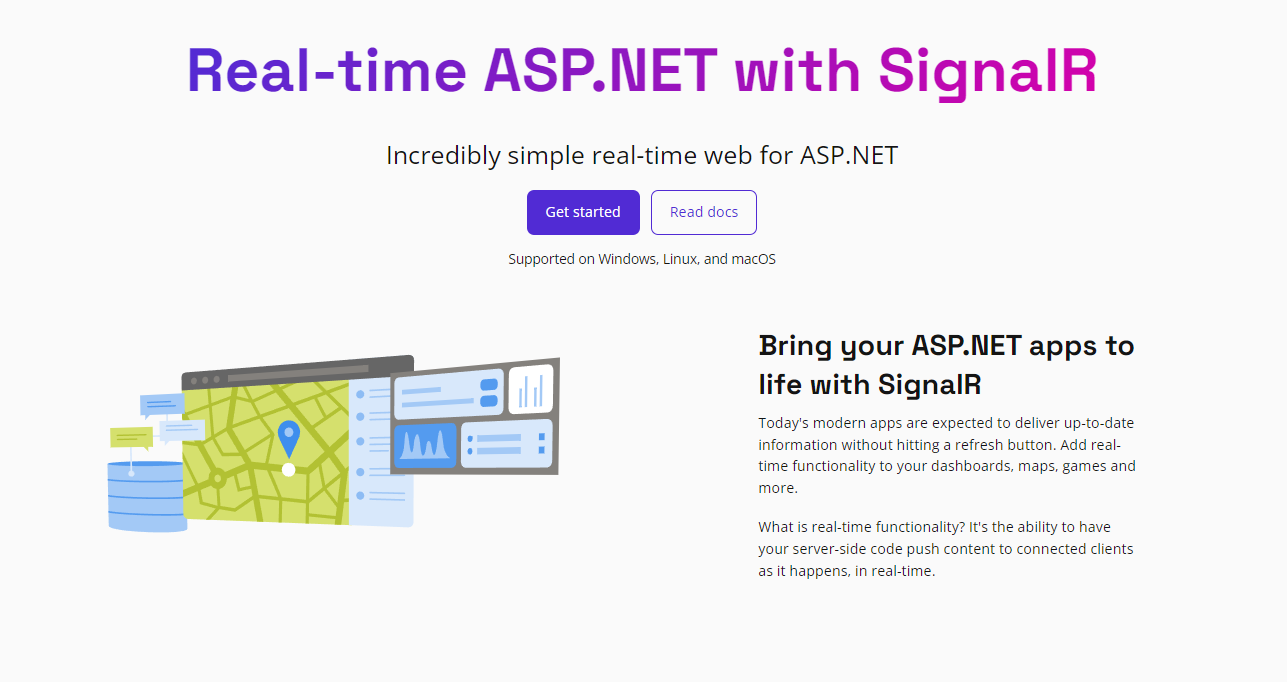
How SignalR Works
To understand how SignalR works, it’s essential to grasp the concept of real-time communication in web applications. Traditionally, web pages communicate with the server using HTTP requests. The client (your browser) sends a request to the server, and the server responds with data. This is a one-way communication model, where the client has to initiate every interaction.
But what if you want the server to push updates to the client without waiting for the client to ask? That’s where SignalR comes into play. SignalR provides a two-way communication channel between the server and the client, enabling real-time data exchange.
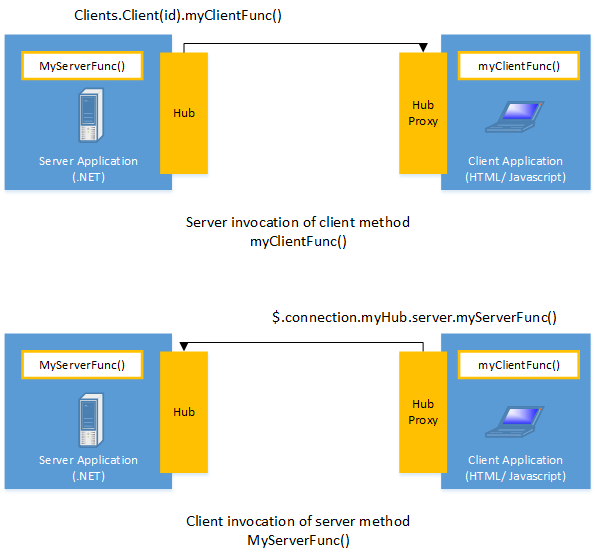
The Magic Behind SignalR: Transports
SignalR supports multiple transport mechanisms to handle real-time communication:
WebSockets: The preferred and most efficient transport for real-time communication. WebSockets establish a persistent connection between the server and the client, allowing data to be sent in both directions simultaneously.
Server-Sent Events (SSE): This is a one-way communication channel where the server sends updates to the client, but the client cannot send data back.
Long Polling: This method involves the client repeatedly polling the server for updates. If WebSockets or SSE aren’t supported, SignalR falls back to long polling.
SignalR automatically chooses the best transport method available, depending on the client’s capabilities and server configuration.
Real-World Applications of SignalR
SignalR isn’t just some fancy tool—it has real-world applications that can enhance the user experience and make your applications stand out. Here are some scenarios where SignalR can be a game-changer:
Live Chat Applications: Whether it’s a customer support chat or a group messaging app, SignalR enables messages to be sent and received in real-time, providing a seamless communication experience.
Live Dashboards: Need to display real-time data? SignalR can push updates to your dashboard as soon as they happen, making it perfect for monitoring systems, stock tickers, or live sports scores.
Collaborative Tools: Think of applications like Google Docs, where multiple users can edit a document simultaneously. SignalR enables real-time collaboration by syncing changes across all users instantly.
Online Gaming: For multiplayer games, real-time communication is crucial. SignalR ensures that game state updates are communicated to all players without delay.
IoT Applications: SignalR can be used to monitor IoT devices in real-time, pushing updates to a dashboard as soon as new data is available.
SignalR vs WebSockets: What’s the Difference?
You might be wondering, “Isn’t SignalR just another name for WebSockets?” Well, not exactly. While WebSockets are a crucial part of SignalR, they’re not the whole story.
WebSockets are a low-level protocol that enables two-way communication between a client and a server over a single, long-lived connection. They’re fast, efficient, and perfect for real-time communication. However, not all environments support WebSockets, and this is where SignalR shines.
SignalR is a high-level abstraction that sits on top of WebSockets, providing additional features like automatic reconnection, message broadcasting, and fallback to other transports if WebSockets aren’t available. In short, SignalR makes working with WebSockets (and other transports) easier and more reliable.
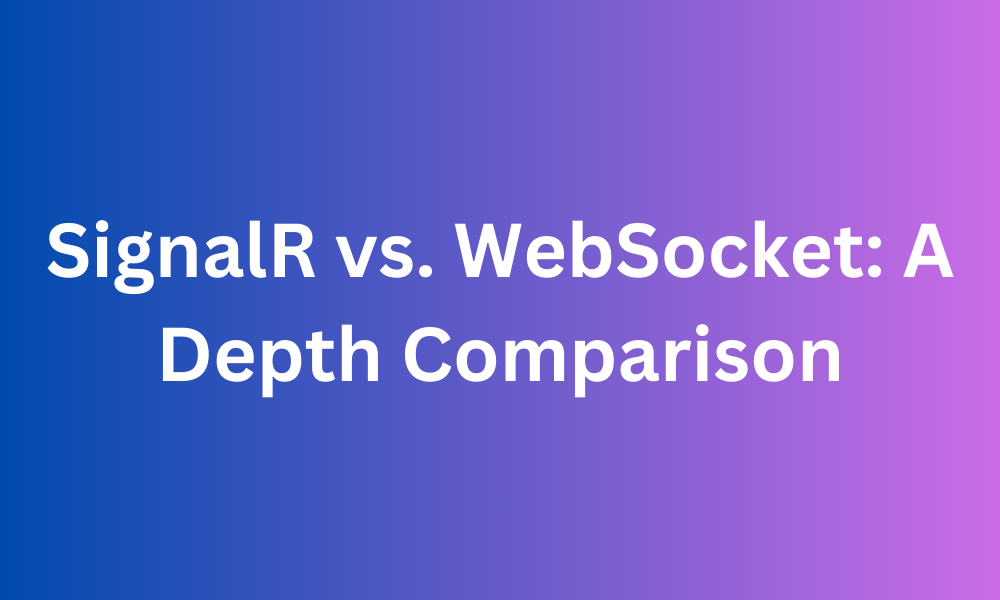
Setting Up SignalR in Your Application
Getting started with SignalR is straightforward. Here’s a step-by-step guide to integrating SignalR into your ASP.NET Core application.
Step 1: Install the SignalR Package
First, you need to install the SignalR NuGet package. You can do this via the Package Manager Console in Visual Studio:
Install-Package Microsoft.AspNetCore.SignalR
Or, if you prefer the .NET CLI:
dotnet add package Microsoft.AspNetCore.SignalR
Step 2: Create a Hub
SignalR uses hubs to manage connections and communication between the client and server. A hub is a class that inherits from Hub
and contains methods that clients can call.
Here’s a simple example of a hub:
public class ChatHub : Hub
{
public async Task SendMessage(string user, string message)
{
await Clients.All.SendAsync("ReceiveMessage", user, message);
}
}
Step 3: Configure SignalR in Startup.cs
Next, you need to configure SignalR in your Startup.cs
file. Add the following code to the ConfigureServices
method:
public void ConfigureServices(IServiceCollection services)
{
services.AddSignalR();
// Other services
}
Then, in the Configure
method, set up the SignalR middleware:
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapHub<ChatHub>("/chathub");
});
}
Step 4: Create a Client
Finally, create a client that connects to the SignalR hub. Here’s an example using JavaScript:
const connection = new signalR.HubConnectionBuilder()
.withUrl("/chathub")
.build();
connection.on("ReceiveMessage", (user, message) => {
const msg = `${user}: ${message}`;
console.log(msg);
});
connection.start().catch(err => console.error(err));
document.getElementById("sendButton").addEventListener("click", () => {
const user = document.getElementById("userInput").value;
const message = document.getElementById("messageInput").value;
connection.invoke("SendMessage", user, message).catch(err => console.error(err));
});
And that’s it! You’ve now got a basic SignalR setup that can handle real-time communication.
Key Features of SignalR
SignalR isn’t just about real-time communication. It comes packed with features that make it a robust solution for a wide range of applications. Here are some of the key features:
Automatic Reconnect: SignalR can automatically reconnect clients if the connection is lost, ensuring a seamless user experience.
Scalability: SignalR supports scaling out across multiple servers, making it suitable for large-scale applications with many users.
Security: SignalR provides built-in support for authentication and authorization, ensuring that only authorized clients can connect to your hubs.
Broadcasting: SignalR makes it easy to broadcast messages to all connected clients or to specific groups of clients.
Compatibility: SignalR works with a wide range of clients, including web browsers, mobile devices, and desktop applications.
SignalR Performance and Scalability
When it comes to performance and scalability, SignalR is designed to handle a large number of concurrent connections. However, there are some considerations to keep in mind.
Performance Tips
Use WebSockets When Possible: WebSockets offer the best performance for real-time communication. SignalR will automatically use WebSockets if the client supports it.
Optimize Message Size: Keep your messages as small as possible to reduce latency and improve performance.
Use Groups Wisely: SignalR allows you to broadcast messages to specific groups of clients. Use groups to reduce the number of messages sent and improve performance.
Scaling Out
If you’re building a large-scale application, you might need to scale out
SignalR across multiple servers. SignalR supports several scaling options, including:
Azure SignalR Service: A fully managed SignalR service that automatically handles scaling and load balancing.
Redis: Use Redis as a backplane to coordinate messages between SignalR servers in a scale-out environment.
SQL Server: Use SQL Server as a backplane to scale out SignalR across multiple servers.
Common Use Cases for SignalR
SignalR is incredibly versatile and can be used in a variety of scenarios. Here are some common use cases:
Real-Time Notifications: Whether it’s a new message, a system alert, or a social media update, SignalR can push notifications to users in real-time.
Live Data Feeds: Display live data feeds, such as stock prices, news updates, or sports scores, using SignalR.
Interactive User Interfaces: Create interactive UIs that respond to user actions in real-time, such as live polls, quizzes, or voting systems.
Collaborative Tools: Build collaborative tools where multiple users can work together in real-time, such as document editors, whiteboards, or project management apps.
Real-Time Monitoring: Use SignalR to monitor systems, networks, or IoT devices in real-time, providing instant updates when something goes wrong.
Integrating SignalR with APIs
SignalR can be easily integrated with APIs to provide real-time updates. For example, if you have an API that handles orders, you can use SignalR to notify users when their order status changes.
Here’s a simple example:
API Endpoint: Create an API endpoint that updates the order status.
SignalR Hub: Use SignalR to broadcast the order status change to the client.
Client-Side Integration: The client listens for updates from the SignalR hub and displays the new order status in real-time.
This integration can be particularly useful in e-commerce applications, where customers expect real-time updates on their orders.
Comparison Table WebSocket & SignalR:
Feature/Aspect | WebSocket | SignalR |
---|---|---|
Type | Protocol | Library/Framework |
Communication | Full-duplex, bi-directional | Full-duplex, bi-directional; additional features for broadcasting and grouping |
Connection | Persistent | Persistent; automatic management and reconnect capabilities |
Compatibility | Universal (any platform/language supporting TCP) | Primarily for .NET applications |
Fallback Mechanisms | None | Automatic fallback to other techniques (long polling, server-sent events) if WebSockets are unavailable |
Transport | TCP | WebSocket, Server-Sent Events, Long Polling |
Message Formats | Binary and Text | Text, JSON, and custom protocols |
Supported Platforms | Browsers, Servers, IoT devices | .NET, JavaScript, Java, and others through custom clients |
State Management | Client and Server responsible for state | Built-in state management and reconnection support |
Security | SSL/TLS encryption | SSL/TLS encryption, plus additional authentication mechanisms |
In the dynamic world of web development, where real-time communication is vital, Apidog emerges as an indispensable tool for WebSocket API testing. Its design specifically caters to the unique challenges of WebSocket APIs, setting it apart in several key areas:
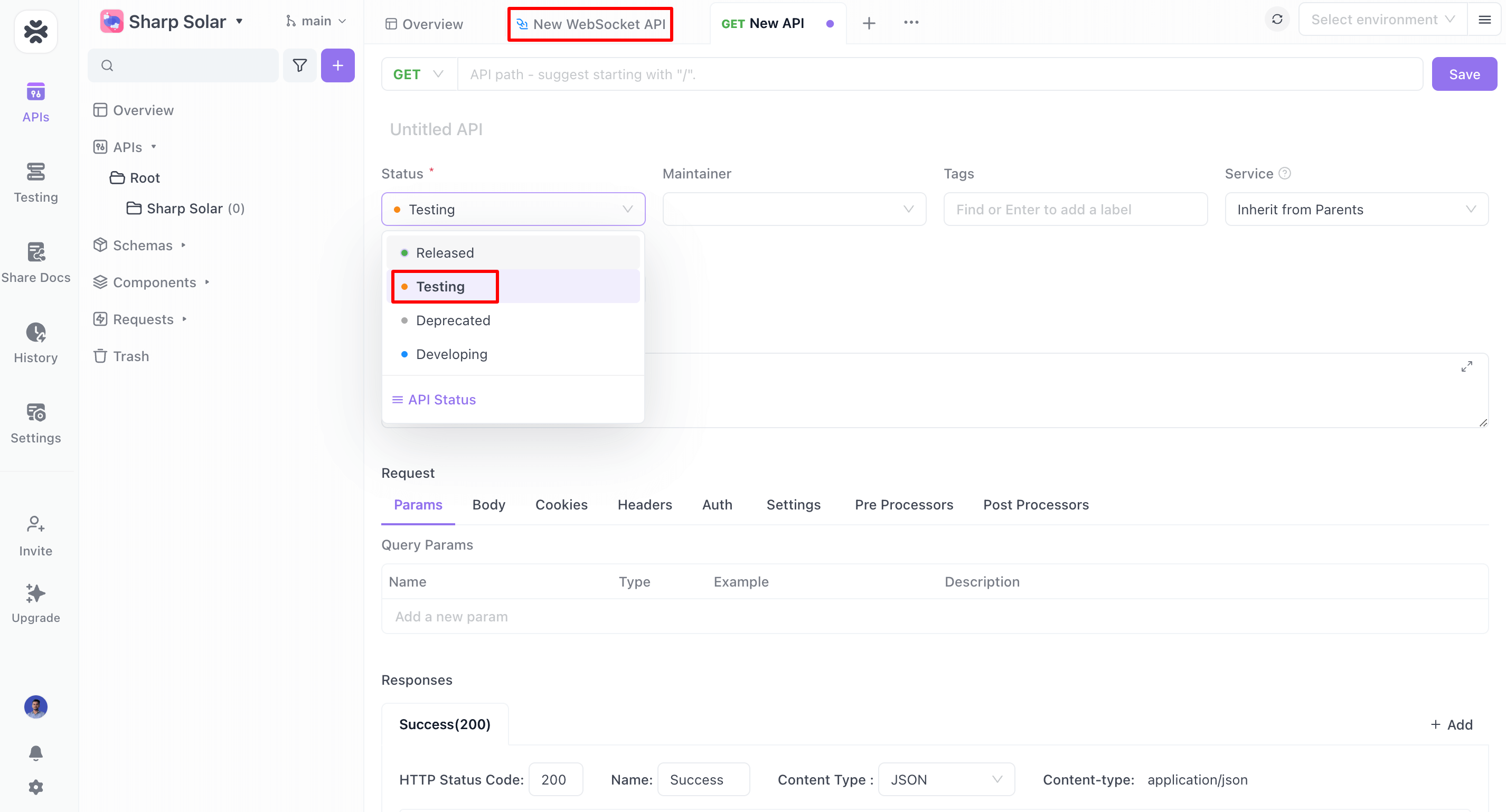
Real-Time Interaction: Apidog enables live interaction with WebSocket APIs, allowing testers to send messages and receive immediate responses, mirroring real-world application behavior.
Intuitive User Interface: Its user-friendly design simplifies the testing process, making it accessible for both novice and experienced testers and developers. This ease of use is crucial for efficient setup, execution, and analysis of tests.
Comprehensive Testing Features: Apidog goes beyond basic functionality checks, offering extensive testing capabilities including connection stability, message formatting, concurrency handling, and response time analysis.
Collaboration Enhancement: Recognizing the importance of teamwork in modern development, Apidog facilitates the sharing of test configurations and results, promoting effective communication and a cohesive testing strategy.
Automation for Efficiency: It supports test automation, a key feature for maintaining continuous oversight of API performance and functionality, thus enhancing efficiency.
Efficient Error Detection and Resolution: With detailed analysis tools, Apidog aids in swiftly identifying and addressing issues, reducing the time and effort spent in troubleshooting.
Why Choose SignalR for Real-Time Communication?
You might still be wondering, “Why should I choose SignalR for real-time communication?” Here are a few reasons:
Simplicity: SignalR abstracts away the complexities of real-time communication, making it easy to implement in your applications.
Flexibility: With support for multiple transports, SignalR can adapt to different environments and client capabilities.
Scalability: Whether you’re building a small app or a large-scale application, SignalR can scale to meet your needs.
Community and Support: As part of the ASP.NET ecosystem, SignalR has a large community and extensive documentation, making it easy to find help and resources.
Integration: SignalR integrates seamlessly with other ASP.NET technologies, such as MVC, Web API, and Identity, providing a cohesive development experience.
Conclusion
SignalR is a powerful tool that simplifies the process of adding real-time communication to your web applications. Whether you’re building a chat app, a live dashboard, or a collaborative tool, SignalR provides the features and flexibility you need to create a seamless user experience.
And remember, if you’re working with APIs and want to make your life easier, don’t forget to check out Apidog. It’s the ultimate tool for managing, testing, and documenting your APIs—perfect for developers working with real-time applications like those powered by SignalR.