Introduction to VSCode MCP Server
MCP (Model Context Protocol) is an innovative open standard that transforms how AI models interact with external tools and services through a unified interface. In VSCode, MCP support enhances GitHub Copilot's agent mode by allowing you to connect any MCP-compatible server to your AI-powered coding workflows. This tutorial will guide you through everything you need to know about using MCP servers with VSCode, from initial setup to advanced configurations and troubleshooting.
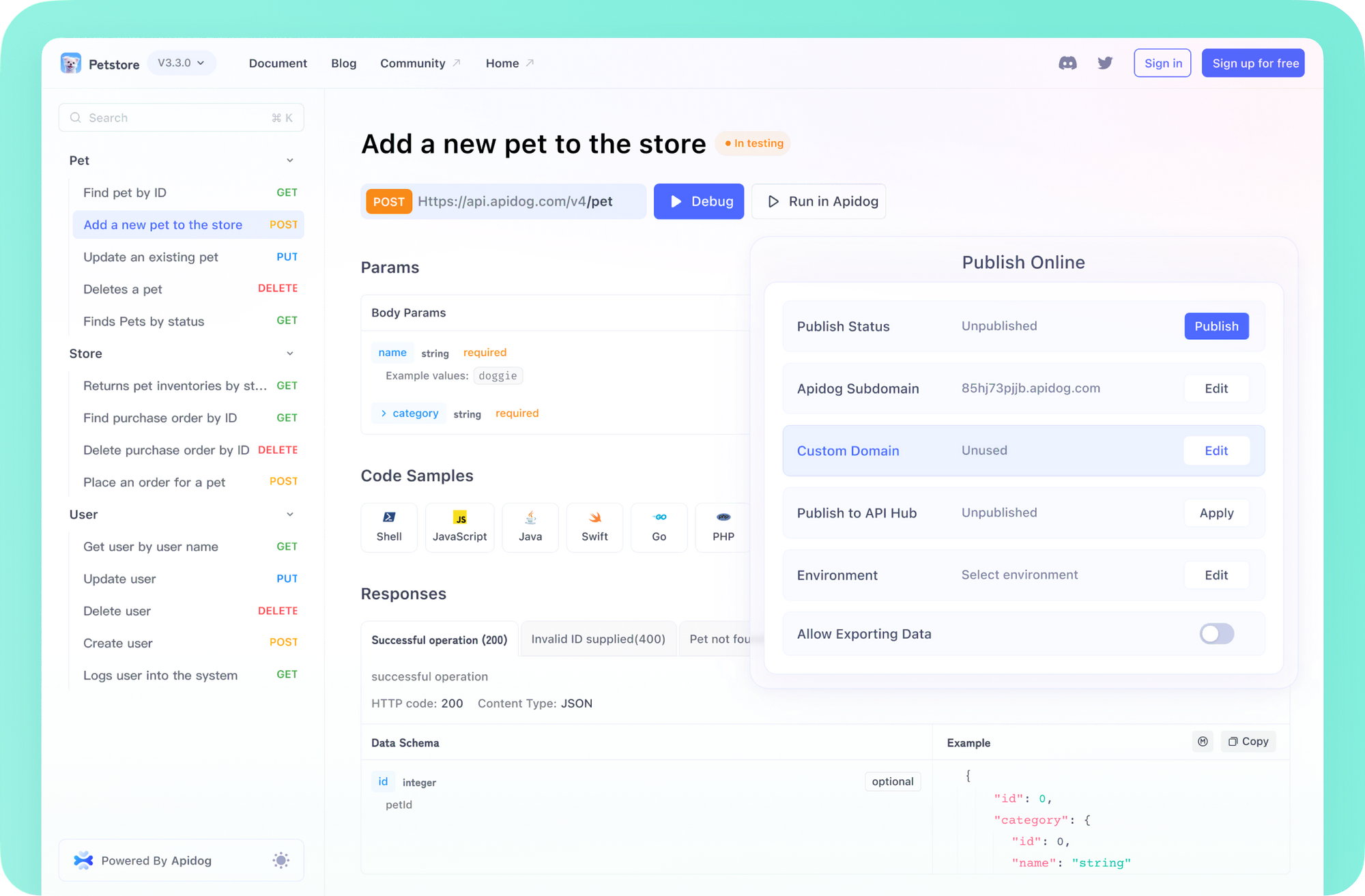
What is VSCode MCP Server
The Model Context Protocol enables AI models to discover and interact with external tools, applications, and data sources through a standardized interface. When using VSCode with Copilot's agent mode, the AI can leverage various tools to perform tasks such as file operations, accessing databases, or calling APIs based on your requests.
MCP follows a client-server architecture:
- MCP clients (like VSCode) connect to MCP servers and request actions on behalf of the AI model
- MCP servers provide tools that expose specific functionalities through well-defined interfaces
- The protocol defines message formats for communication between clients and servers, covering tool discovery, invocation, and response handling
For example, a file system MCP server might provide tools for reading, writing, or searching files and directories. GitHub's MCP server offers tools to list repositories, create pull requests, or manage issues. These servers can run locally on your machine or be hosted remotely.
Setting Up Your First VSCode MCP Server
Step 1: Adding an MCP Server to Your Workspace
There are several ways to add an MCP server to VSCode:
Using the workspace configuration file:
- Create a
.vscode/mcp.json
file in your workspace - Select the "Add Server" button to add a template for a new server
- VSCode provides IntelliSense for the MCP server configuration
Using the Command Palette:
- Run the
MCP: Add Server
command - Choose "Workspace Settings" to create the
.vscode/mcp.json
file in your workspace
Using User Settings for global access:
- Open the Command Palette and run
MCP: Add Server
- Select "User Settings" to add the MCP server configuration globally
Step 2: Understanding VSCode MCP Server Configuration Format
The MCP server configuration follows a specific JSON format:
{
"inputs": [
{
"type": "promptString",
"id": "api-key",
"description": "API Key",
"password": true
}
],
"servers": {
"MyServer": {
"type": "stdio",
"command": "npx",
"args": ["-y", "@modelcontextprotocol/server-example"],
"env": {
"API_KEY": "${input:api-key}"
}
}
}
}
The key elements are:
- inputs: Define custom placeholders for configuration values
- servers: Contains the list of MCP servers
- type: Connection type ("stdio" or "sse")
- command: Command to start the server executable
- args: Array of arguments passed to the command
- env: Environment variables for the server
VSCode MCP Server Connection Types and Configuration Options
STDIO Connection for VSCode MCP Server
For standard input/output connections:
"MyServer": {
"type": "stdio",
"command": "npx",
"args": ["server.js", "--port", "3000"],
"env": {"API_KEY": "${input:api-key}"}
}
SSE Connection for VSCode MCP Server
For server-sent events connections:
"MyRemoteServer": {
"type": "sse",
"url": "http://api.example.com/sse",
"headers": {"VERSION": "1.2"}
}
Using Variables in VSCode MCP Server Configuration
You can use predefined variables in your configuration:
"MyServer": {
"type": "stdio",
"command": "node",
"args": ["${workspaceFolder}/server.js"]
}
Advanced VSCode MCP Server Configuration Examples
Here's a more comprehensive example showing multiple servers and input variables:
{
"inputs": [
{
"type": "promptString",
"id": "perplexity-key",
"description": "Perplexity API Key",
"password": true
}
],
"servers": {
"Perplexity": {
"type": "stdio",
"command": "docker",
"args": ["run", "-i", "--rm", "-e", "PERPLEXITY_API_KEY", "mcp/perplexity-ask"],
"env": {
"PERPLEXITY_API_KEY": "${input:perplexity-key}"
}
},
"Fetch": {
"type": "stdio",
"command": "uvx",
"args": ["mcp-server-fetch"]
},
"RemoteServer": {
"type": "sse",
"url": "http://api.contoso.com/sse",
"headers": {"VERSION": "1.2"}
}
}
}
Using VSCode MCP Tools in Agent Mode
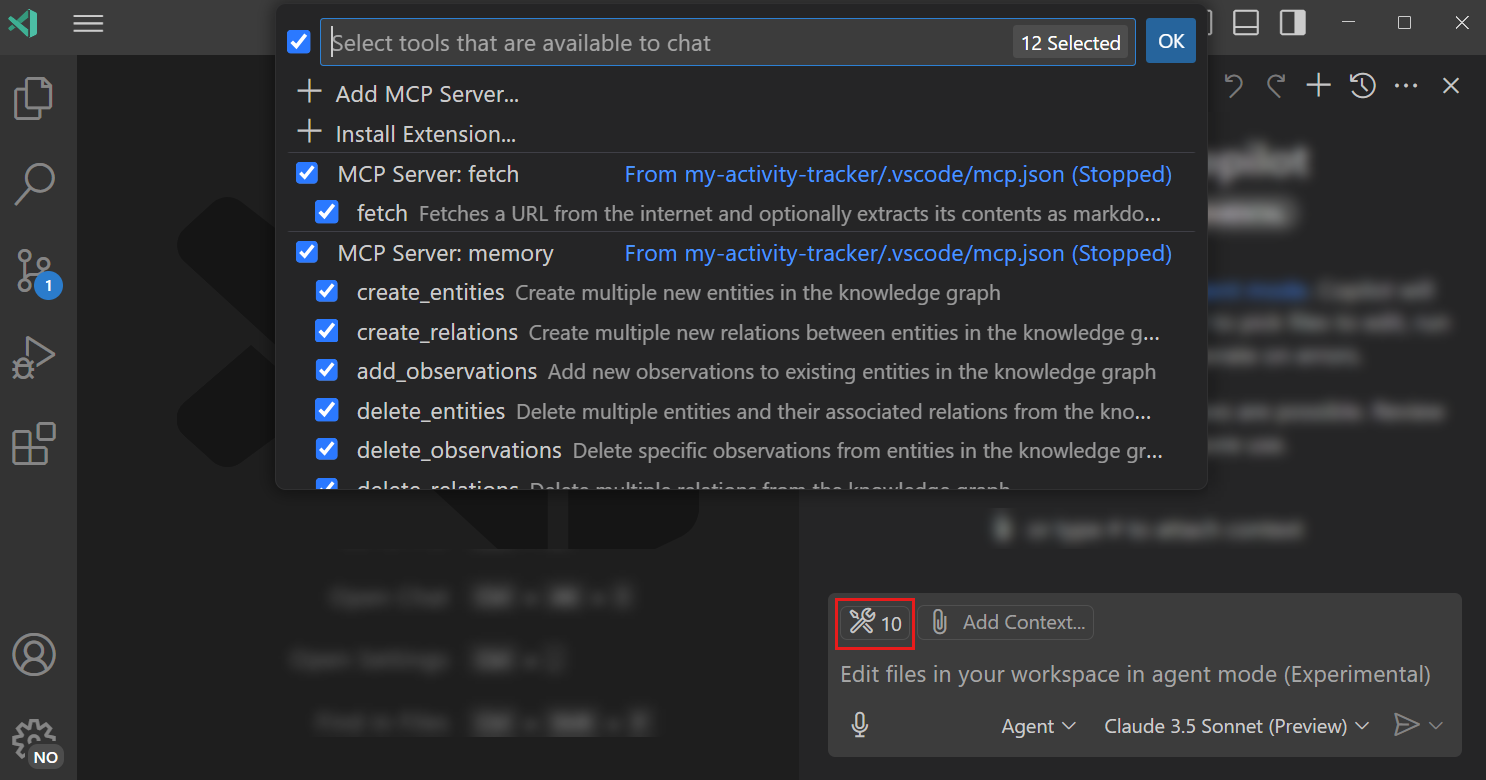
Once you've configured your MCP servers, you can use their tools in Copilot's agent mode:
- Open the Chat view (Windows, Linux:
Ctrl+Alt+I
, Mac:⌃⌘I
) - Select "Agent" mode from the dropdown
- Click the "Tools" button to view available tools
- Select or deselect tools as needed
- Enter your prompt in the chat input
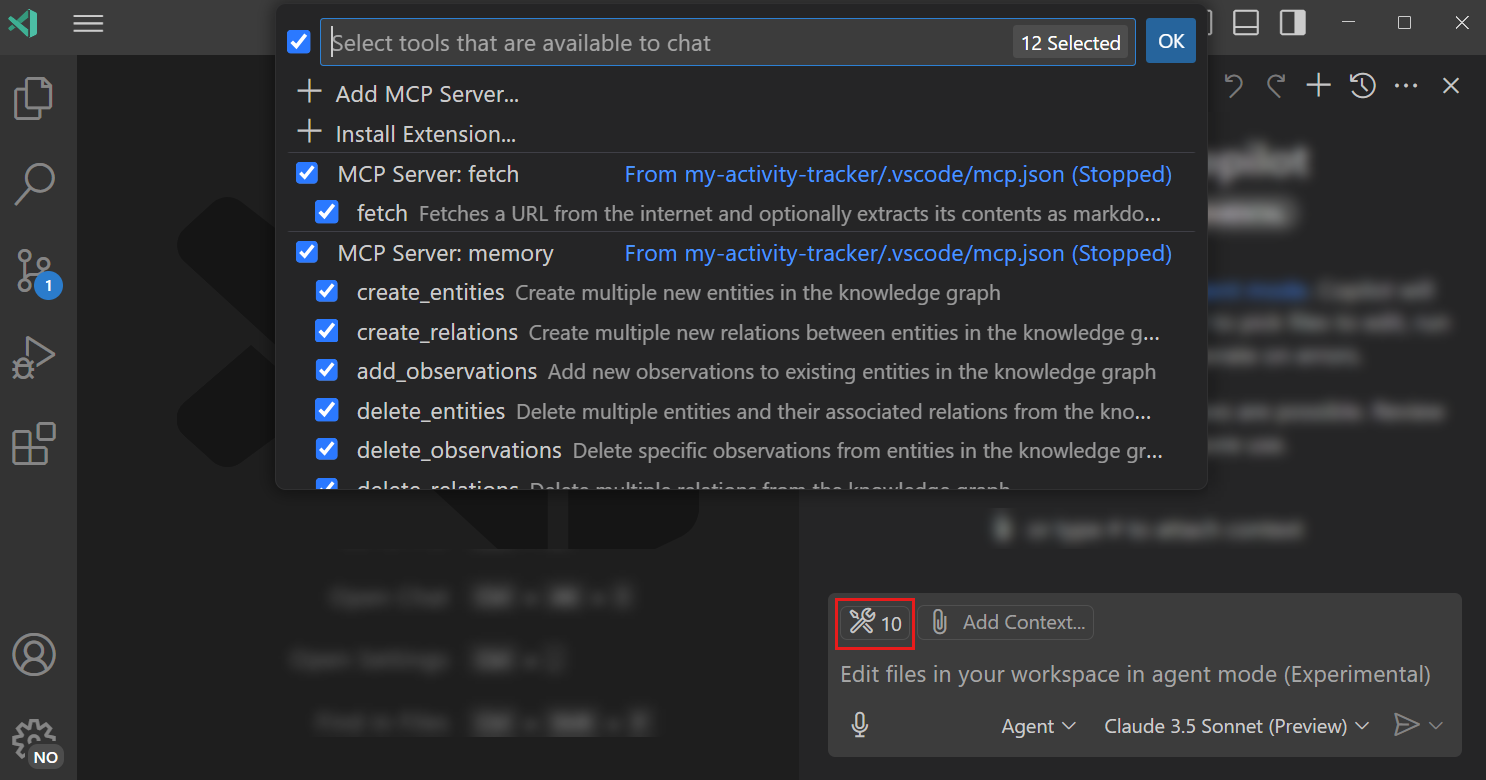
When a tool is invoked, you'll need to confirm the action before it runs. You can configure VSCode to automatically confirm specific tools for the current session, workspace, or all future invocations using the "Continue" button dropdown.
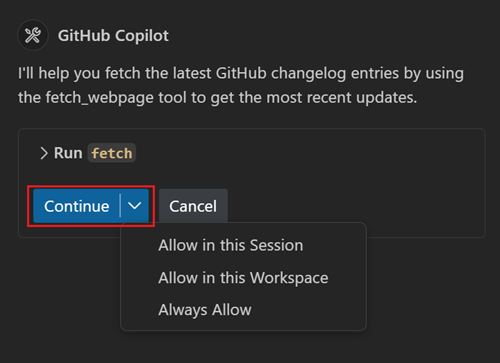
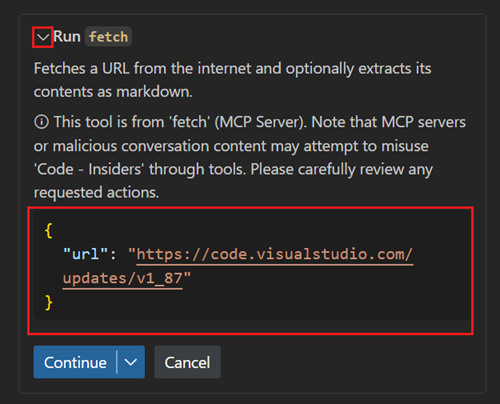
Managing VSCode MCP Servers and Tools
Viewing and Managing Servers
Run the MCP: List Servers
command from the Command Palette to view your configured MCP servers. From this view, you can:
- Start, stop, or restart servers
- View server configurations
- Access server logs for troubleshooting
Direct Tool References in VSCode MCP Server
You can directly reference a tool in your prompt by typing #
followed by the tool name. This works in all chat modes (ask, edit, and agent mode).
Command-line Configuration for VSCode MCP Server
You can add MCP servers using the VSCode command line:
code --add-mcp "{\"name\":\"my-server\",\"command\":\"uvx\",\"args\":[\"mcp-server-fetch\"]}"
Troubleshooting VSCode MCP Server Issues
When VSCode encounters MCP server issues, it displays an error indicator in the Chat view. To diagnose problems:
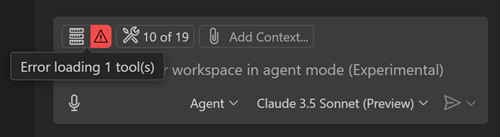
- Click the error notification in the Chat view
- Select "Show Output" to view server logs
- Alternatively, run
MCP: List Servers
from the Command Palette, select the server, and choose "Show Output"
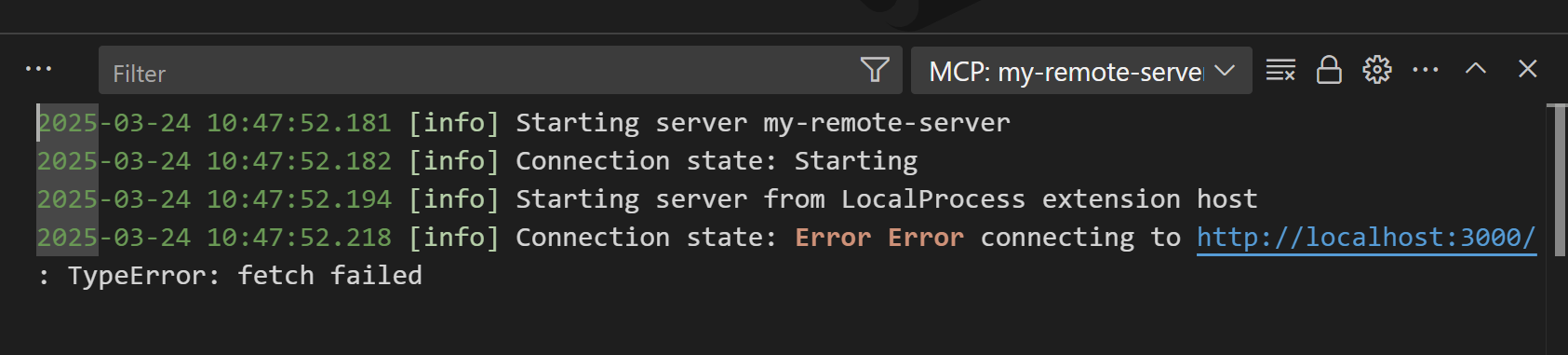
Common issues include:
- Incorrect server configuration
- Missing dependencies
- Network connectivity problems for remote servers
- Permission issues for local executables
Creating Your Own VSCode MCP Server
If you want to develop your own MCP server, you can use any programming language capable of handling stdout. Several official SDKs are available:
- TypeScript SDK
- Python SDK
- Java SDK
- Kotlin SDK
- C# SDK
Your server should implement the MCP standard which defines how tools are discovered, invoked, and how responses are formatted.
VSCode MCP Server Auto-Discovery and Integration
VSCode can automatically detect and reuse MCP servers defined in other tools, such as Claude Desktop. You can enable auto-discovery with the chat.mcp.discovery.enabled
setting in your VSCode settings.
FAQ: Common Questions about VSCode MCP Server
Can I control which MCP tools are used?
Yes, you can toggle specific tools on/off in the Agent mode interface, directly reference tools with the #
prefix in your prompts, or use .github/copilot-instructions.md
for more advanced control.
Are MCP servers secure?
VSCode implements security measures such as requiring confirmation before running tools and securely storing sensitive inputs like API keys. However, you should only use MCP servers from trusted sources.
Can I use MCP servers with other AI assistants?
MCP is an open standard designed to work with multiple AI models. While VSCode currently integrates it with GitHub Copilot, the protocol is compatible with other AI systems that support the standard.
Conclusion: Maximizing VSCode MCP Server Potential
MCP servers significantly extend the capabilities of AI assistants in VSCode by providing standardized access to external tools and services. By following this guide, you can now:
- Set up and configure MCP servers in your VSCode environment
- Use MCP tools with GitHub Copilot's agent mode
- Manage and troubleshoot your MCP servers
- Create your own MCP servers if needed
The Model Context Protocol is still evolving, with a growing ecosystem of servers and tools available for integration with your projects. By mastering VSCode MCP servers, you're positioning yourself at the forefront of AI-assisted development, enabling more powerful and efficient coding workflows.
Keep exploring the MCP ecosystem and experiment with different servers to find the tools that best enhance your development process. With proper configuration and understanding of the protocol, you can transform your AI assistant into a truly powerful coding partner.