Hey there, fellow developers! Today, we're diving into the world of unit testing with FastAPI. Whether you're a seasoned pro or a newcomer to FastAPI, this guide will help you ensure your API is as robust as possible. We’ll cover everything from the basics of unit testing to specific techniques for testing FastAPI applications. Plus, we'll introduce you to a handy tool called Apidog that can streamline your testing process.
Why Unit Testing Matters
Before we dive into the nitty-gritty of unit testing FastAPI, let’s talk about why unit testing is essential. In simple terms, unit testing allows you to verify that each part of your application works as intended. By testing individual components, you can catch bugs early and ensure that your application behaves correctly.
Here are some compelling reasons to embrace unit testing:
- Catch Bugs Early: By testing small units of code, you can identify and fix issues before they become significant problems.
- Ensure Code Quality: Unit tests act as a safety net, helping you maintain high-quality code as your project evolves.
- Facilitate Refactoring: With a solid set of tests, you can confidently refactor your code, knowing that any issues will be caught immediately.
- Improve Documentation: Tests serve as additional documentation, showing how different parts of your application are supposed to work.
Now that we understand the importance of unit testing, let’s dive into the specifics of unit testing with FastAPI.
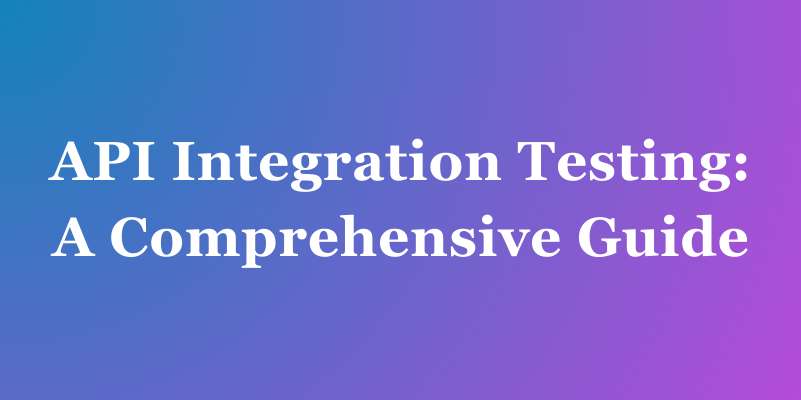
Setting Up Your FastAPI Project
First things first, you need to have a FastAPI project set up. If you haven’t already, you can create a new FastAPI project by following these steps:
Install FastAPI and Uvicorn: FastAPI is the web framework, and Uvicorn is the ASGI server.
pip install fastapi uvicorn
Create Your FastAPI Application: Create a file called main.py
and add the following code:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def read_root():
return {"Hello": "World"}
Run Your Application: Start the server using Uvicorn.
uvicorn main:app --reload
Your FastAPI application is now up and running!
Getting Started with Unit Testing
Installing Test Dependencies
To start unit testing, you'll need a testing framework. We recommend using pytest because it’s easy to use and widely adopted.
Install pytest using pip:
pip install pytest
Additionally, install httpx
for making HTTP requests to your FastAPI application during tests:
pip install httpx
Writing Your First Test
Let’s write a simple test to verify that our root endpoint returns the expected response. Create a file named test_main.py
and add the following code:
from fastapi.testclient import TestClient
from main import app
client = TestClient(app)
def test_read_root():
response = client.get("/")
assert response.status_code == 200
assert response.json() == {"Hello": "World"}
In this test, we use FastAPI’s TestClient
to send a GET request to the root endpoint and assert that the response is correct. Run the test using pytest:
pytest
Structuring Your Tests
To keep your tests organized, consider the following structure for your test files:
project/
├── app/
│ ├── main.py
│ └── ... (other application code)
├── tests/
│ ├── __init__.py
│ └── test_main.py
By placing your tests in a dedicated tests
directory, you can maintain a clean and organized codebase.
Advanced Unit Testing Techniques
Testing Endpoints with Parameters
FastAPI makes it easy to handle parameters in your endpoints. Let’s add an endpoint that takes a parameter and write a test for it.
Update your main.py
with a new endpoint:
@app.get("/items/{item_id}")
def read_item(item_id: int):
return {"item_id": item_id}
Next, add a test for this endpoint in test_main.py
:
def test_read_item():
item_id = 42
response = client.get(f"/items/{item_id}")
assert response.status_code == 200
assert response.json() == {"item_id": item_id}
This test checks that the endpoint correctly returns the item ID passed in the URL.
Testing Dependency Injection
FastAPI’s dependency injection system is powerful and flexible. Let’s see how you can test endpoints that use dependencies.
Add a dependency to main.py
:
from fastapi import Depends
def get_query(q: str = None):
return q
@app.get("/search/")
def search(query: str = Depends(get_query)):
return {"query": query}
Write a test for this endpoint in test_main.py
:
def test_search():
query = "fastapi"
response = client.get(f"/search/?q={query}")
assert response.status_code == 200
assert response.json() == {"query": query}
Here, we test that the dependency injection correctly handles the query parameter.
Introducing Apidog for Enhanced Testing
What is Apidog?
Apidog is a fantastic tool that simplifies API testing and documentation. It integrates seamlessly with FastAPI, allowing you to automate and streamline your testing process.
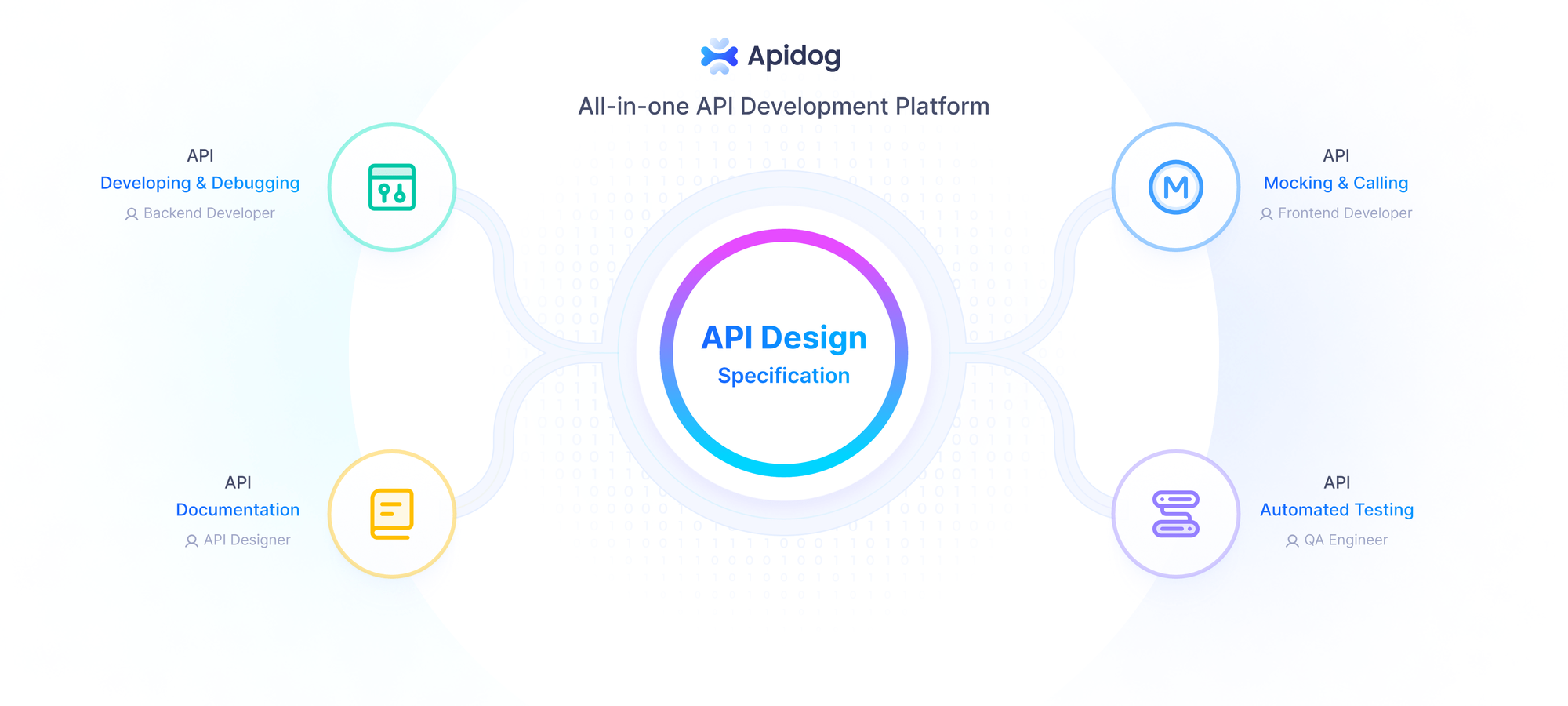
Using Apidog in Your Tests
Apidog provides decorators to easily document and test your endpoints. Here’s how you can use Apidog with your FastAPI application.
Set Up Your Test Environment: First things first, set up your test environment. This includes the systems you want to test and Apidog. Open Apidog and switch to the test Tab
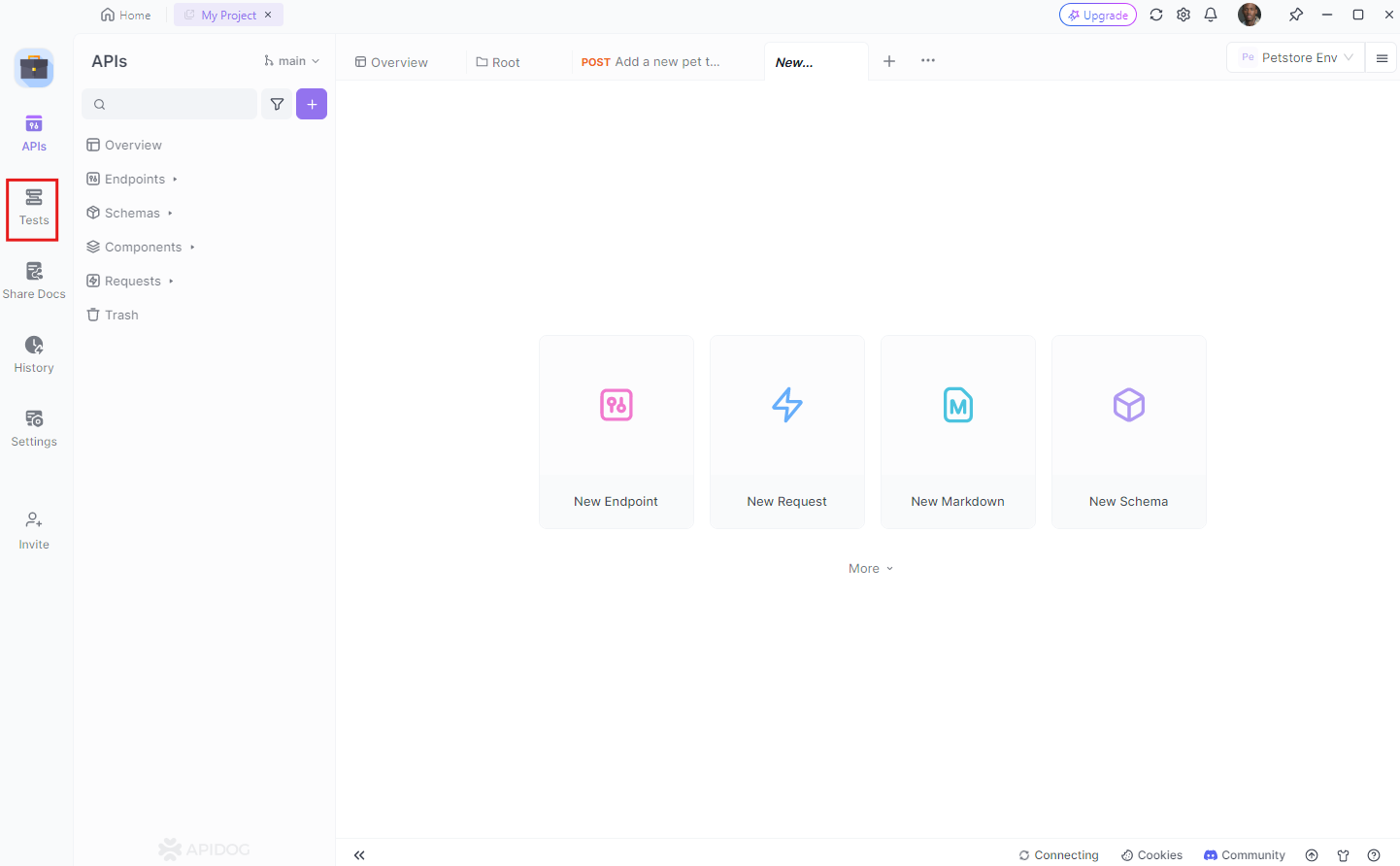
Define Your Test Cases: Next, define your test cases. Think about the different scenarios you want to test and write them down.
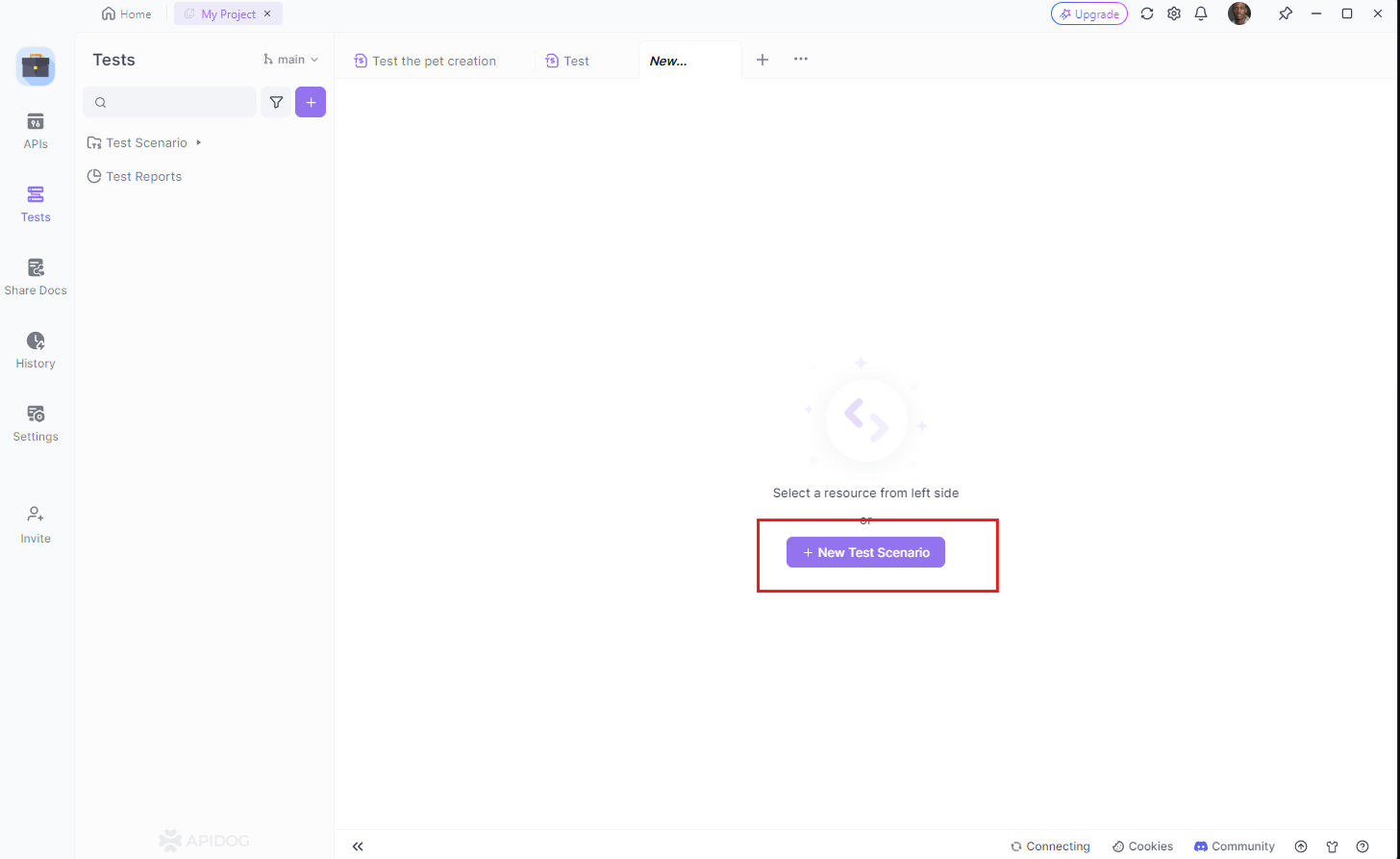
Run Your Tests: Now, it’s time to let Apidog do its magic! Run your tests and wait for the results.
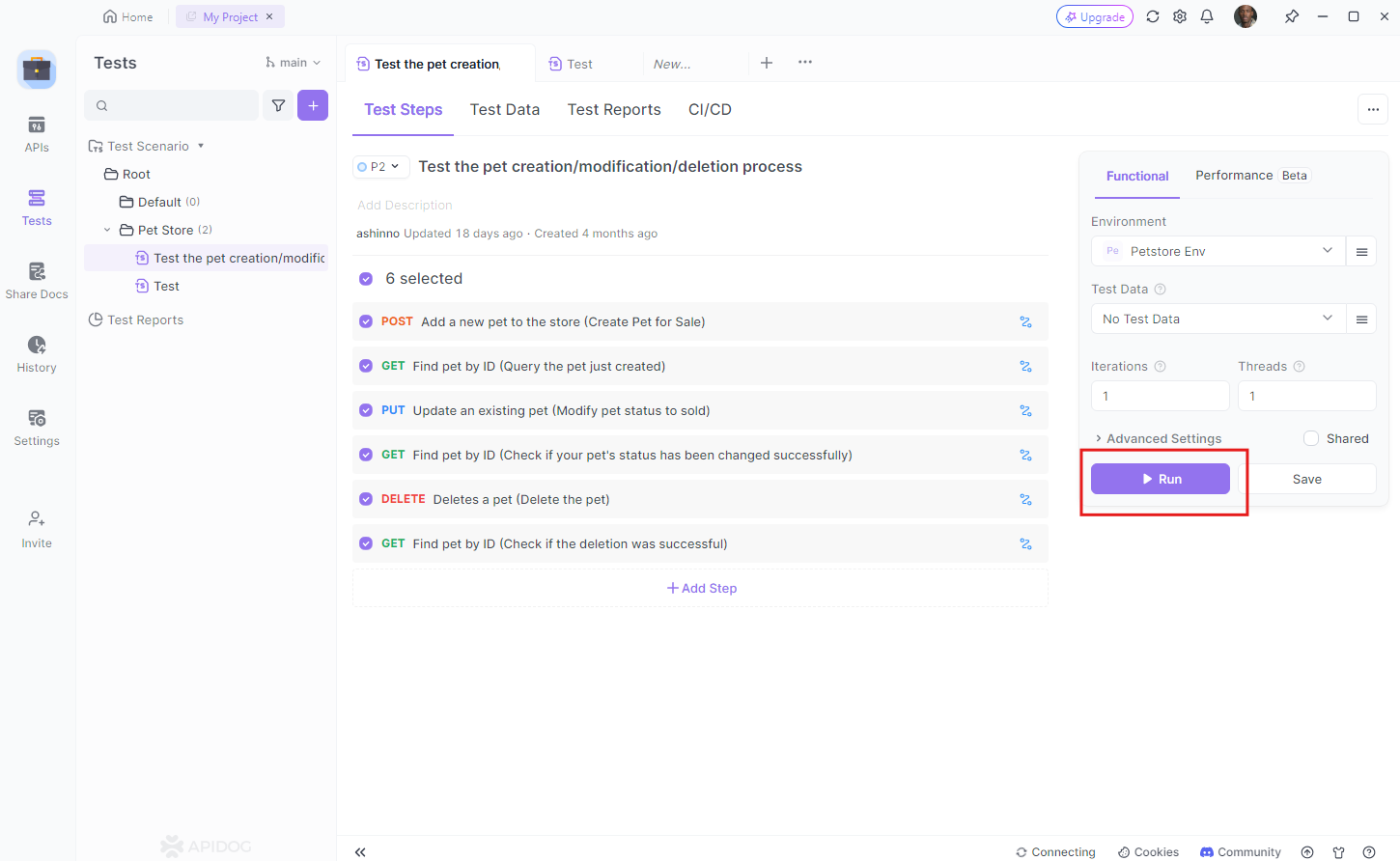
Analyze Your Results: Once your tests are done, analyze your results. Look for any errors or unexpected behavior.
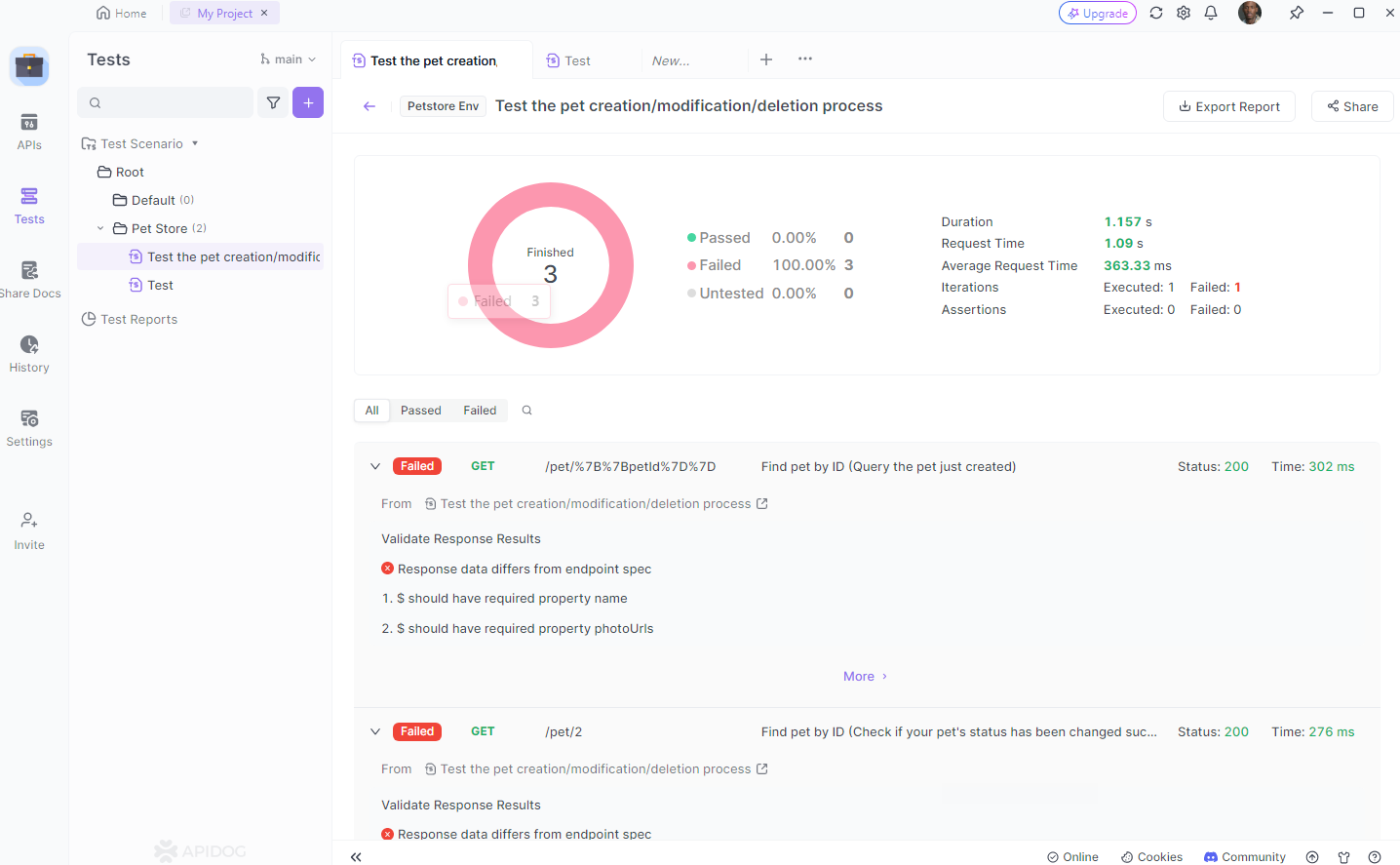
If you find any issues, fix them and run your tests again. Repeat this process until you’re satisfied with the results.
With Apidog, you can ensure your tests are not only correct but also well-documented.
Best Practices for Unit Testing FastAPI
Keep Tests Isolated
Ensure that each test is independent and does not rely on the state of other tests. This isolation makes tests easier to debug and maintain.
Use Fixtures
Fixtures in pytest can help you set up and tear down test environments. Here’s an example fixture for your FastAPI app:
import pytest
from fastapi.testclient import TestClient
from main import app
@pytest.fixture
def client():
return TestClient(app)
def test_with_fixture(client):
response = client.get("/")
assert response.status_code == 200
assert response.json() == {"Hello": "World"}
Mock External Dependencies
When your endpoints depend on external services or databases, use mocking to simulate those dependencies during testing. This approach helps you focus on testing your application logic without being affected by external factors.
Conclusion
Unit testing FastAPI applications doesn’t have to be daunting. By following the steps outlined in this guide, you can ensure your API is reliable, maintainable, and well-documented. Remember to use tools like Apidog to streamline your testing process and keep your tests organized and isolated.
Now, it's your turn to put these tips into practice and make your FastAPI application bulletproof. Happy testing!