How to make a PUT Request in Python (2024 Guide)
Learn how to make PUT requests using Python . This blog post covers everything you need to know about PUT requests, including how to customize your requests and test them using apidog.
In this blog post, we will explore the basics of PUT requests, how to make them using Python Requests, and some best practices to keep in mind. Whether you’re a seasoned developer or just starting out, this guide will provide you with everything you need to know about PUT requests.
HTTP Request Basics
Before we dive into the PUT request, let’s first understand what an HTTP request is. HTTP stands for Hypertext Transfer Protocol, which is a protocol used to transfer data over the internet. An HTTP request is a message sent by a client to a server, requesting for a specific resource. The server then responds with the requested resource.
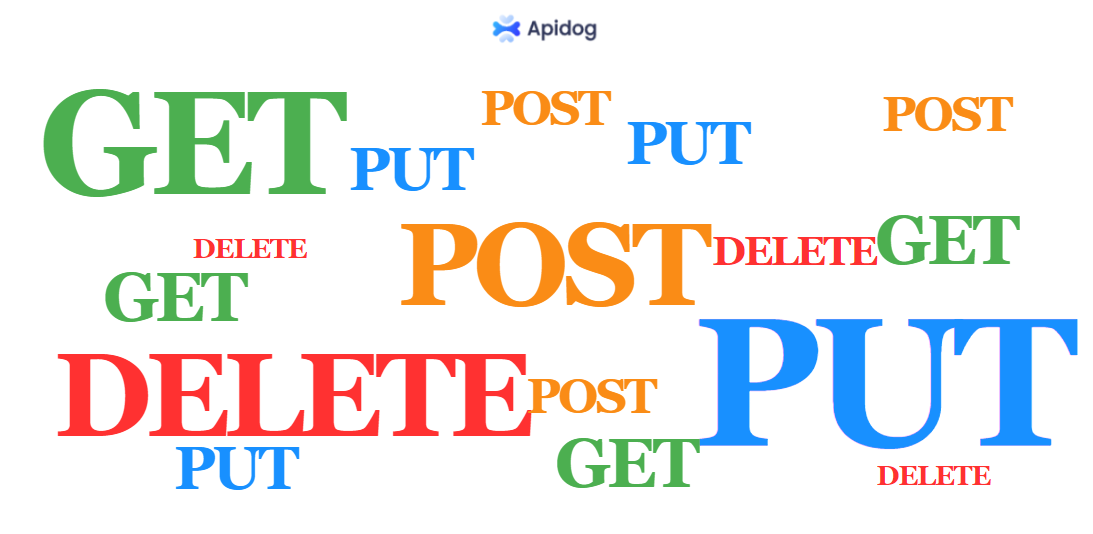
There are various HTTP methods, also referred to as HTTP requests, each serving a distinct purpose and conveying the nature of the request. The most prevalent HTTP methods include GET, POST, PUT and DELETE.
What is a PUT request?
A PUT request is an HTTP method used to update or replace an existing resource on a server. It is similar to the POST method, but instead of creating a new resource, it updates an existing one. PUT requests are idempotent, meaning that multiple requests will have the same result.
In simple terms, a PUT request is used to update a resource on the server. It sends data to the server to be stored at a specified resource or URL. Think of it as the command that tells the server, "Hey, I've got some new information for you; replace the old stuff with this!"
What is Python?
Now that we've covered the basics of HTTP requests, let's talk about the programming language that's going to be our trusty companion on this journey – Python. Python is renowned for its simplicity, readability, and versatility. It's a high-level language that enables developers to write clear, logical code for projects of all sizes. The latest version of Python can be obtained by visiting the official website and downloading it from there.
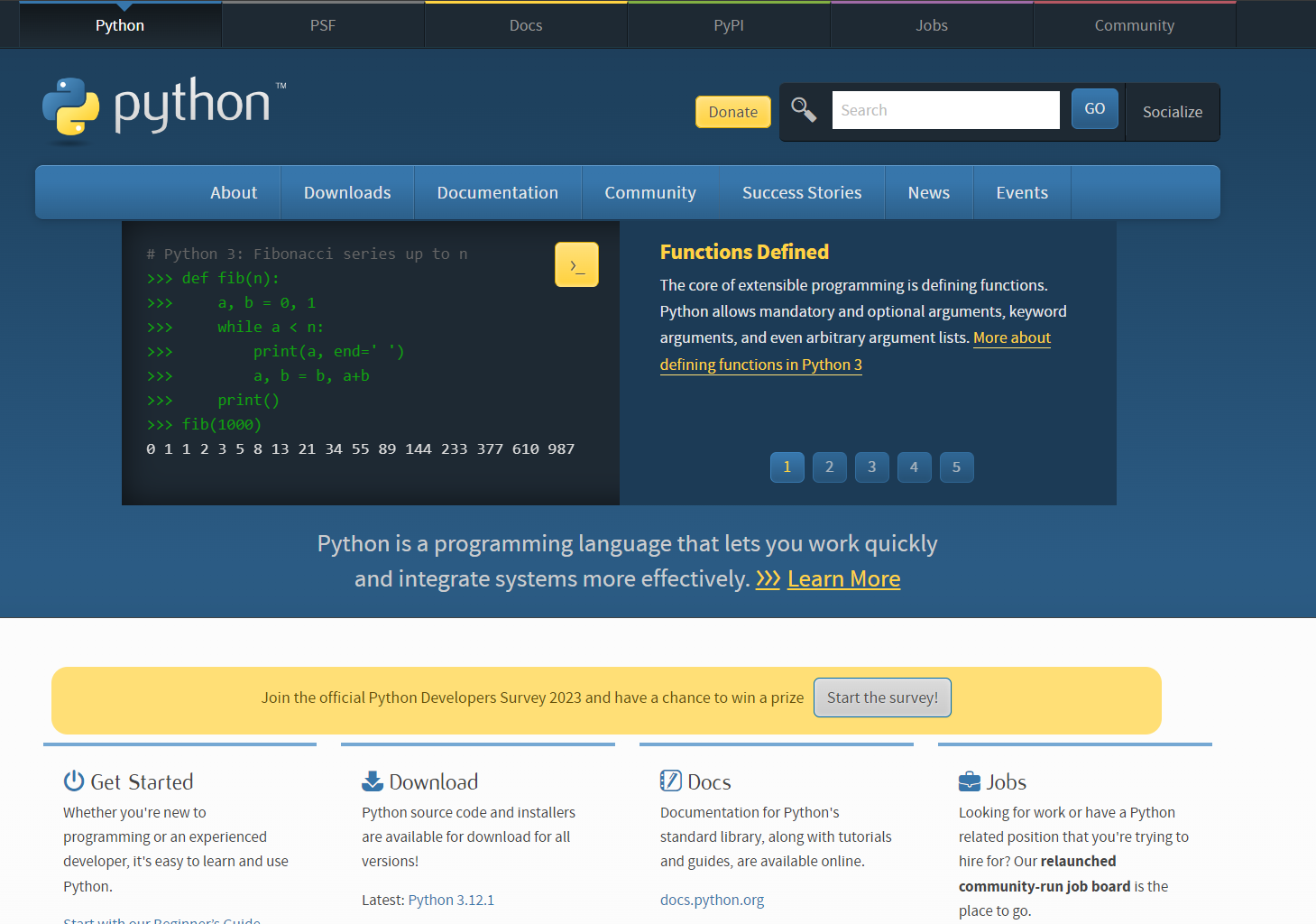
Python's extensive libraries make it a favorite among developers, and its syntax ensures that even beginners can grasp its concepts quickly. So, why not harness the power of Python to make our PUT requests smoother than ever?
How to make a PUT request using Python
To make a PUT request using Python, you will need to use the requests
library. This library allows you to send HTTP/1.1 requests extremely easily.
In Python, you can make a PUT request using the requests
library, which is a popular HTTP library for making HTTP requests. If you don't have it installed, you can install it using:
pip install requests
Here's an example of how to make a PUT request using the requests
library:
import requests
url = "https://example.com/api/resource"
data = {"key1": "value1", "key2": "value2"}
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer your_access_token" # Include any necessary headers
}
response = requests.put(url, json=data, headers=headers)
# Check the response
if response.status_code == 200:
print("PUT request successful")
print("Response:", response.json())
else:
print("PUT request failed")
print("Status code:", response.status_code)
print("Response:", response.text)
In this example:
url
is the endpoint where you want to send the PUT request.data
is the data you want to send in the request body. You can change it based on your API requirements.headers
is a dictionary containing any additional headers you need to include, such as authentication headers.response
contains the server's response to the PUT request.
Make sure to replace the URL, data, and headers with your actual values. Adjust the content type and authentication headers according to your API specifications.
Note: This example assumes you are working with JSON data. If your API requires a different content type or data format, you may need to modify the code accordingly.
Here are examples of different content types and data formats:
Example 1: Sending Form Data
import requests
url = "https://example.com/api/resource"
data = {"key1": "value1", "key2": "value2"}
headers = {
"Content-Type": "application/x-www-form-urlencoded",
"Authorization": "Bearer your_access_token"
}
response = requests.put(url, data=data, headers=headers)
if response.status_code == 200:
print("PUT request successful")
print("Response:", response.text)
else:
print("PUT request failed")
print("Status code:", response.status_code)
print("Response:", response.text)
Example 2: Sending XML Data
import requests
url = "https://example.com/api/resource"
data = """
<root>
<key1>value1</key1>
<key2>value2</key2>
</root>
"""
headers = {
"Content-Type": "application/xml",
"Authorization": "Bearer your_access_token"
}
response = requests.put(url, data=data, headers=headers)
if response.status_code == 200:
print("PUT request successful")
print("Response:", response.text)
else:
print("PUT request failed")
print("Status code:", response.status_code)
print("Response:", response.text)
Example 3: Sending Plain Text
import requests
url = "https://example.com/api/resource"
data = "This is plain text data."
headers = {
"Content-Type": "text/plain",
"Authorization": "Bearer your_access_token"
}
response = requests.put(url, data=data, headers=headers)
if response.status_code == 200:
print("PUT request successful")
print("Response:", response.text)
else:
print("PUT request failed")
print("Status code:", response.status_code)
print("Response:", response.text)
Adjust the Content-Type
and the format of the data
parameter based on your API requirements. Always refer to the API documentation to ensure you're using the correct content type and data format.
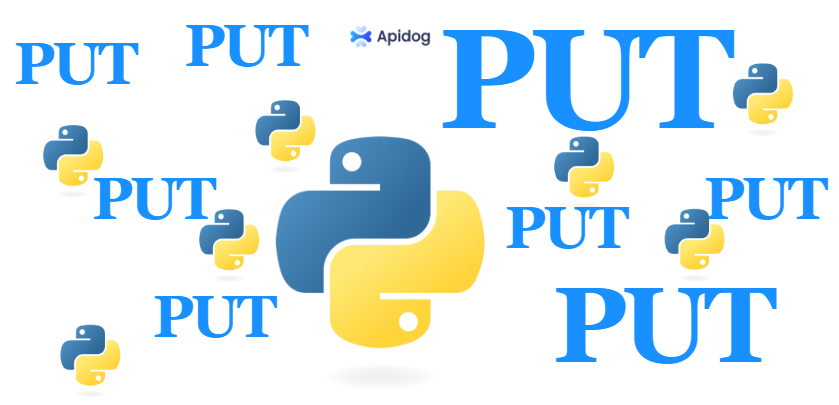
Understanding the PUT request parameters in Python.
When making a PUT request, there are several parameters that you can use to customize your request. Here are some of the most common parameters:
- URL: The URL of the resource you want to update.
- Data: The data you want to send in the PUT request. You can use the
json
parameter to send JSON data or thedata
parameter to send form-encoded data. - Headers: The headers you want to include in the PUT request. You can use the
headers
parameter to specify headers such asContent-Type
,Authorization
, etc. - Authentication: If the endpoint requires authentication, you can use the
auth
parameter to provide authentication credentials. - Timeout: You can set a timeout for the request using the
timeout
parameter. This specifies the number of seconds to wait for a response before timing out. - Proxies: If you need to use a proxy to make the request, you can use the
proxies
parameter to specify the proxy URL. - Verify: If you want to verify the SSL certificate of the server, you can set the
verify
parameter toTrue
. If you want to disable certificate verification, you can set it toFalse
. - Allow redirects: By default,
requests
will automatically follow redirects. If you want to disable this behavior, you can set theallow_redirects
parameter toFalse
.
Here is an example of how to use the data
parameter to send a PUT request:
import requests
url = 'https://api.example.com/resource'
payload = {'key1': 'value1', 'key2': 'value2'}
r = requests.put(url, data=payload)
In this example, we are sending a PUT request to https://api.example.com/resource
with a payload of {'key1': 'value1', 'key2': 'value2'}
.
Using Apidog to Test Your Python PUT Request
Apidog is a powerful tool for testing APIs. It allows you to create and save API requests, organize them into collections, and share them with your team.
Here is how you can use Apidog to test your PUT request:
- Open Apidog and create a new request.
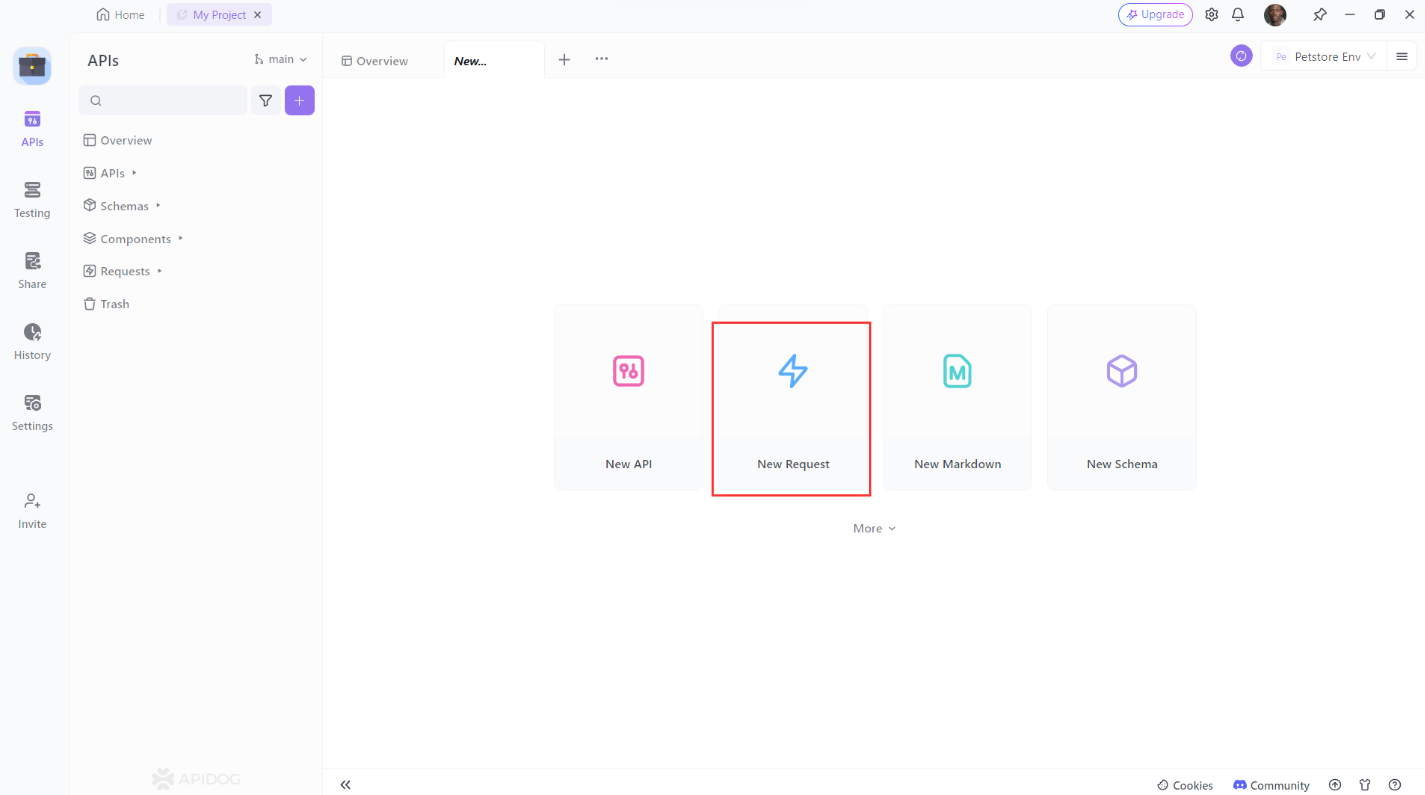
2. Set the request method to PUT.
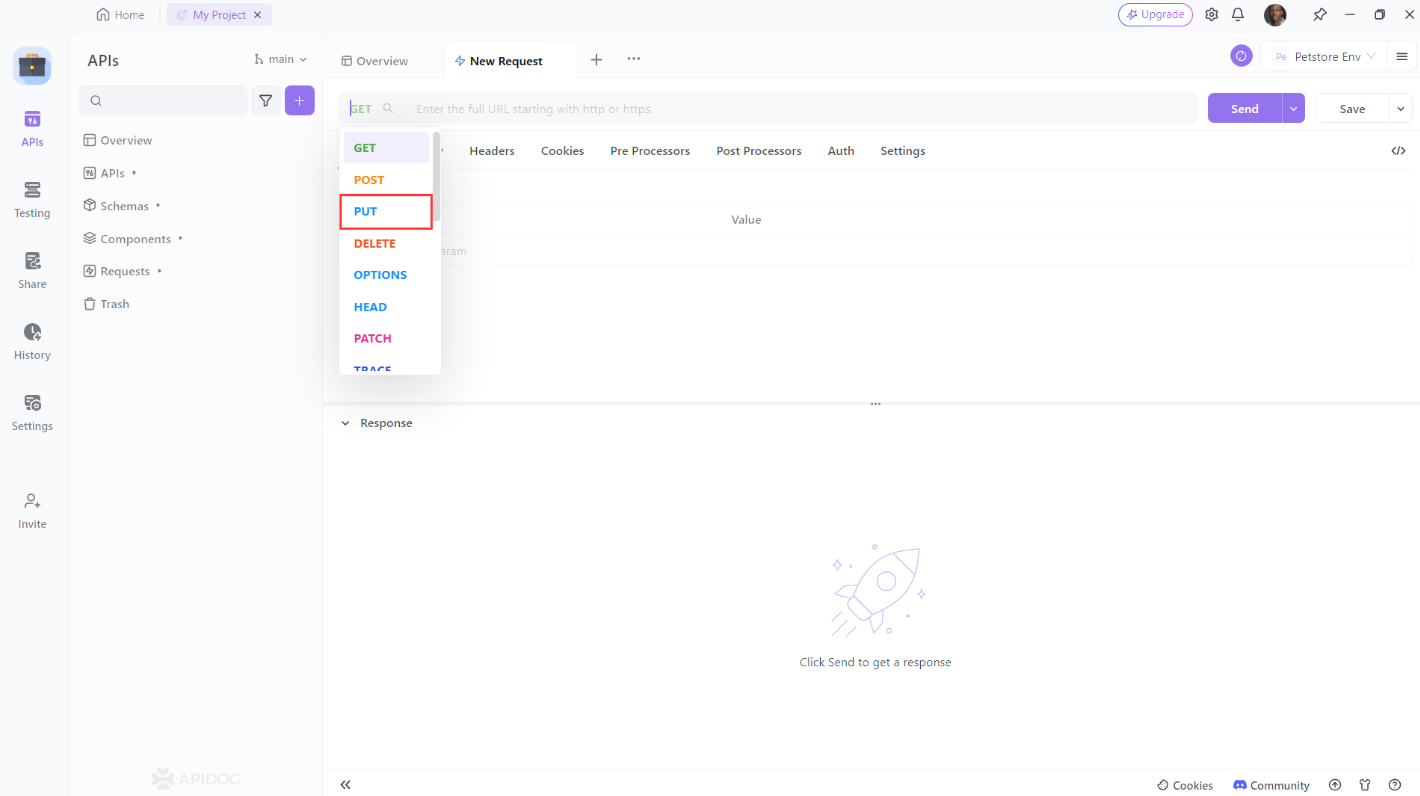
3. Enter the URL of the resource you want to update. Add any additional headers or parameters you want to include then click the “Send” button to send the request.
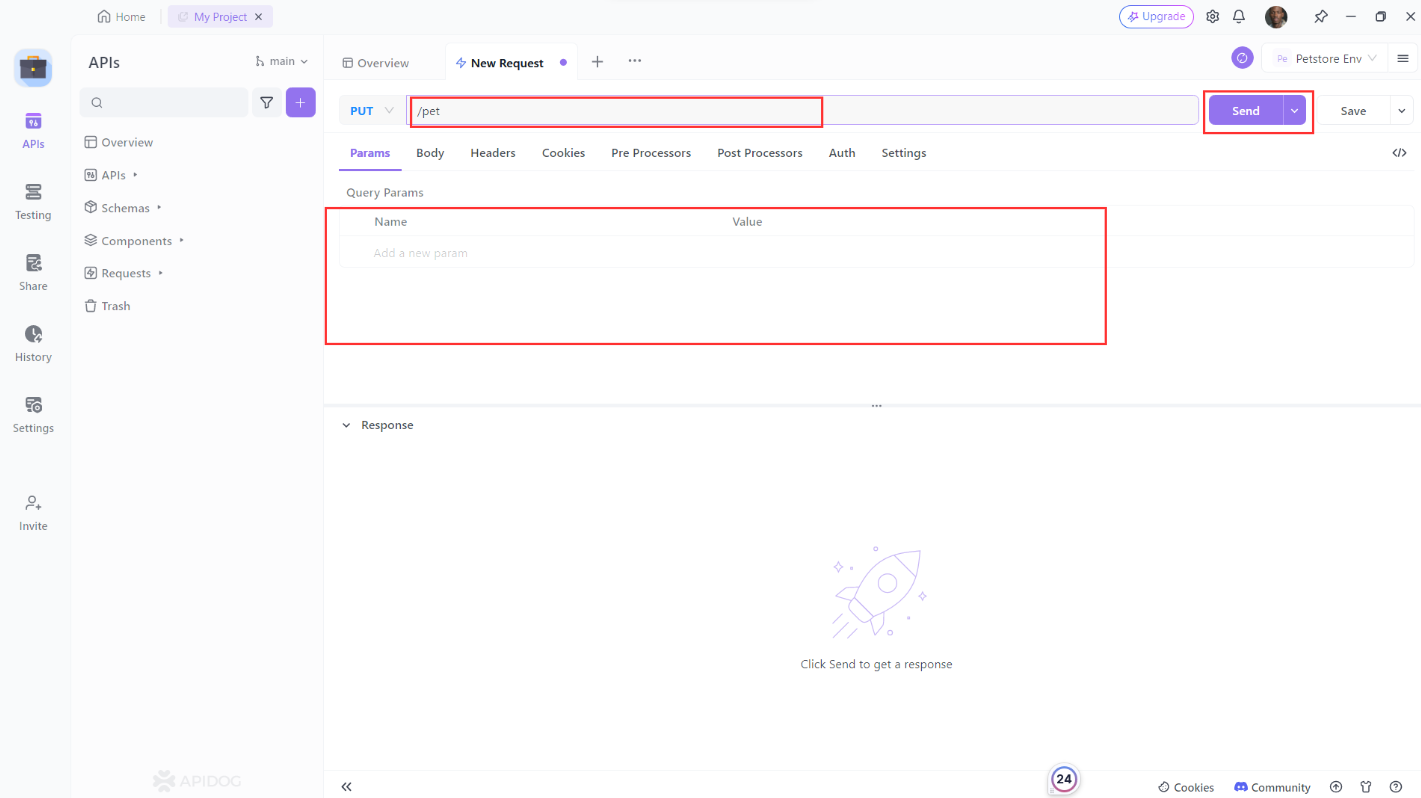
4. Verify that the response is what you expected.
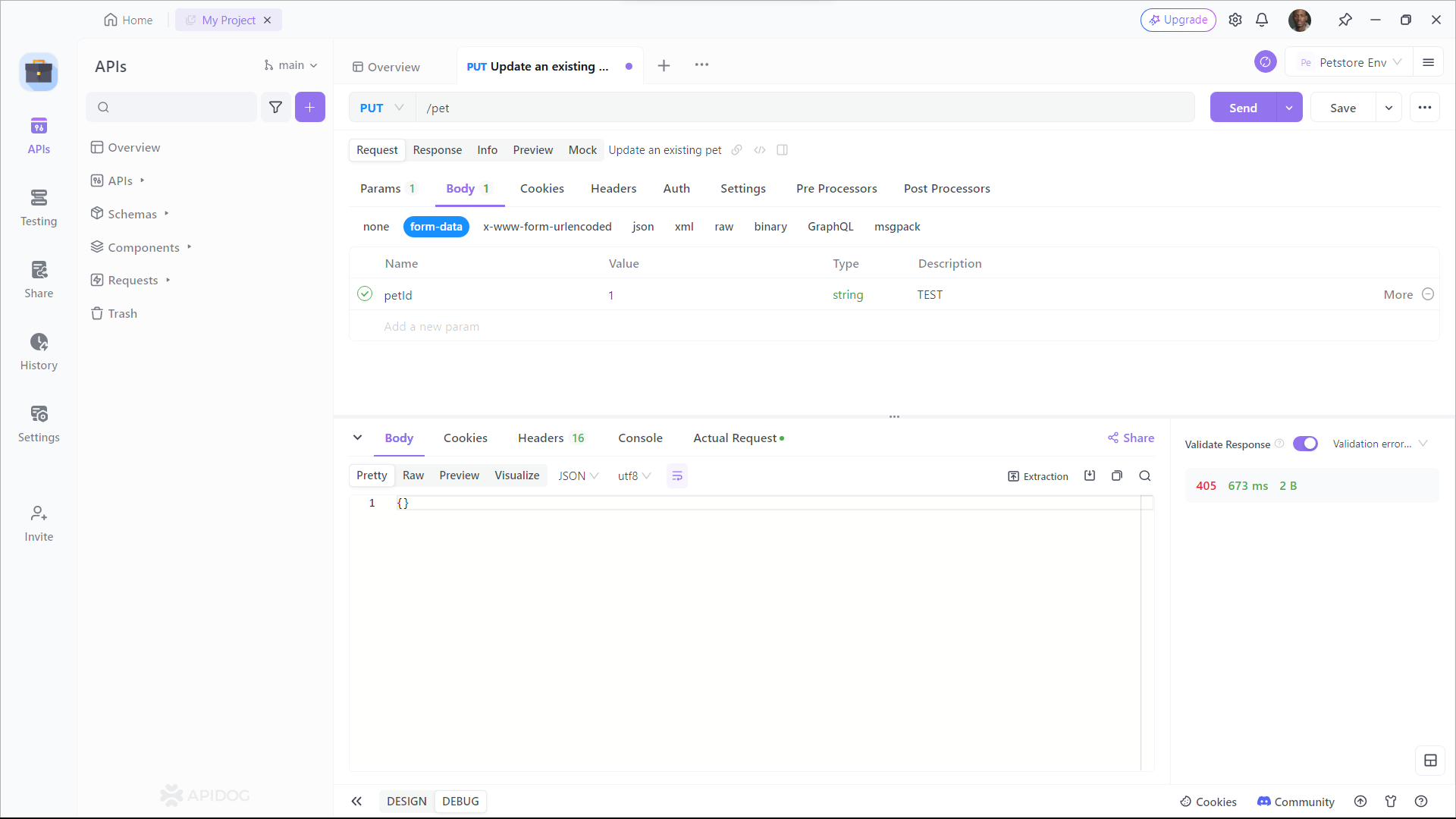
Best practices for making a PUT Request.
When making a PUT request, it is important to handle errors properly. You should always check the response status code to ensure that the request was successful. You should also handle any errors that may occur during the request.
Here are some best practices for making a PUT request in Python:
- Error handling: Always include appropriate error handling in your code to ensure that your program does not crash when an error occurs. You can use the
try
andexcept
statements to handle errors in Python. For example, you can catch all exceptions using the base-class exceptionException
or catch them separately and do different things. - Authentication: Some endpoints require authentication using headers, tokens, or user credentials. Ensure your request includes these when needed. You can use the
auth
parameter in therequests
library to provide authentication credentials. For example, you can use basic authentication with theHTTPBasicAuth
class provided by therequests
library. If you are accessing a URL that requires Windows authentication, you can use theRequests-NTLM
library. - Security: When making a PUT request, ensure that the data is sent securely. If you are sending data over HTTP, it is recommended to use HTTPS instead. You can verify that your certificate is good by running
openssl s_client -showcerts -connect example.com:443
from the command line and checking that it reportsVerify return code: 0 (ok)
. You can also disable the security certificate check in Python requests by using a context manager that monkey patches requests and changes it so thatverify=False
is the default and suppresses the warning.
Conclusion
In this blog post, we explored what a PUT request is, how to make a PUT request using Python, and how to test your PUT request using Apidog. We also discussed the various parameters you can use to customize your PUT request. With this knowledge, you should be able to confidently use PUT requests in your own web development projects.