Have you ever wondered how to update data on a web server using Python? If so, you’re not alone. Many developers struggle with the concept of PUT requests, which are one of the most common methods of the HTTP protocol. PUT requests allow you to send data to a web server and replace the existing resource with the new one. Sounds simple, right?
In this blog post, we’ll cover the basics of APIs, HTTP requests, and PUT requests. We’ll also show you how to use the requests library to make a PUT request in Python with headers and test it using Apidog.
Apidog can simplify the process of sending PUT requests with headers and make API development more efficient, so click the download button below to use Apidog for completely free.
What is an API?
Before we dive into making a PUT request in Python with headers, let’s first discuss what an API is. An API, or Application Programming Interface, is a set of protocols, routines, and tools for building software applications. APIs specify how software components should interact and APIs are used to enable communication between different software systems. APIs are used to retrieve data from a server, send data to a server, or perform other actions.
What is an HTTP request?
Let’s first understand what an HTTP request is. HTTP stands for Hypertext Transfer Protocol, which is a protocol used to transfer data over the internet. An HTTP request is a message sent by a client to a server, requesting for a specific resource. The server then responds with the requested resource.
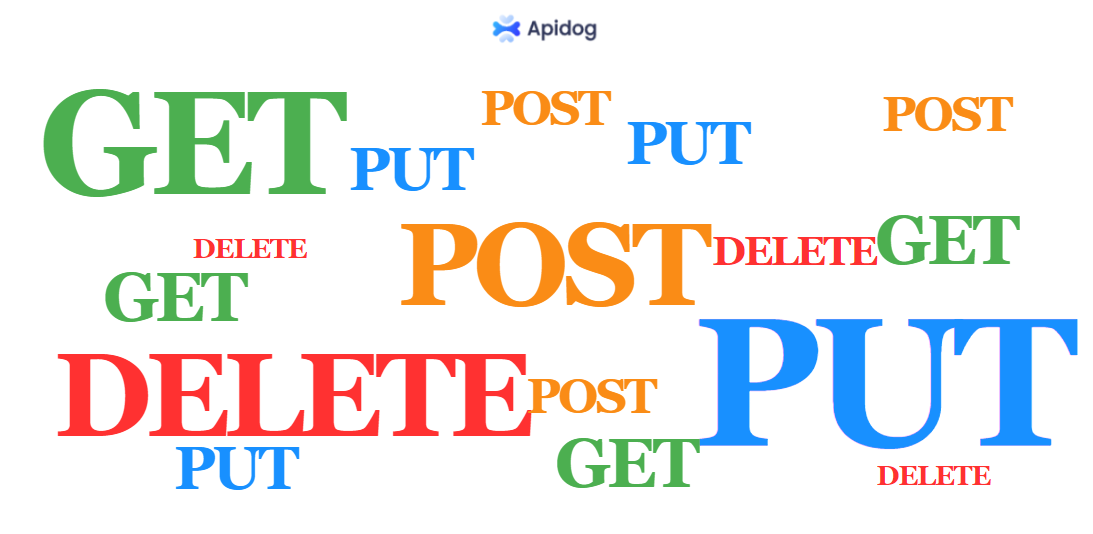
There are various HTTP methods, also referred to as HTTP requests, each serving a distinct purpose and conveying the nature of the request. The most prevalent HTTP methods include GET, POST, PUT and DELETE.
What is a PUT request?
A PUT request is an HTTP request method that is used to update or replace an existing resource on the server. A PUT request creates a new resource or replaces a representation of the target resource with the request payload. The request has a body and the successful response has a body. The PUT request is not safe and is idempotent. It is not allowed in HTML forms.
What are Headers?
Headers are additional information that is sent along with an HTTP request or response. Headers can be used to provide information about the request or response, such as the content type, encoding, or authentication information. Headers can also be used to provide additional information about the client or server, such as the user agent or server software.
Why is it convenient to use Python to send put requests with headers?
Now that we've covered the basics of HTTP requests, let's talk about the programming language that's going to be our trusty companion on this journey – Python. Python is renowned for its simplicity, readability, and versatility. It's a high-level language that enables developers to write clear, logical code for projects of all sizes. The latest version of Python can be obtained by visiting the official website and downloading it from there.
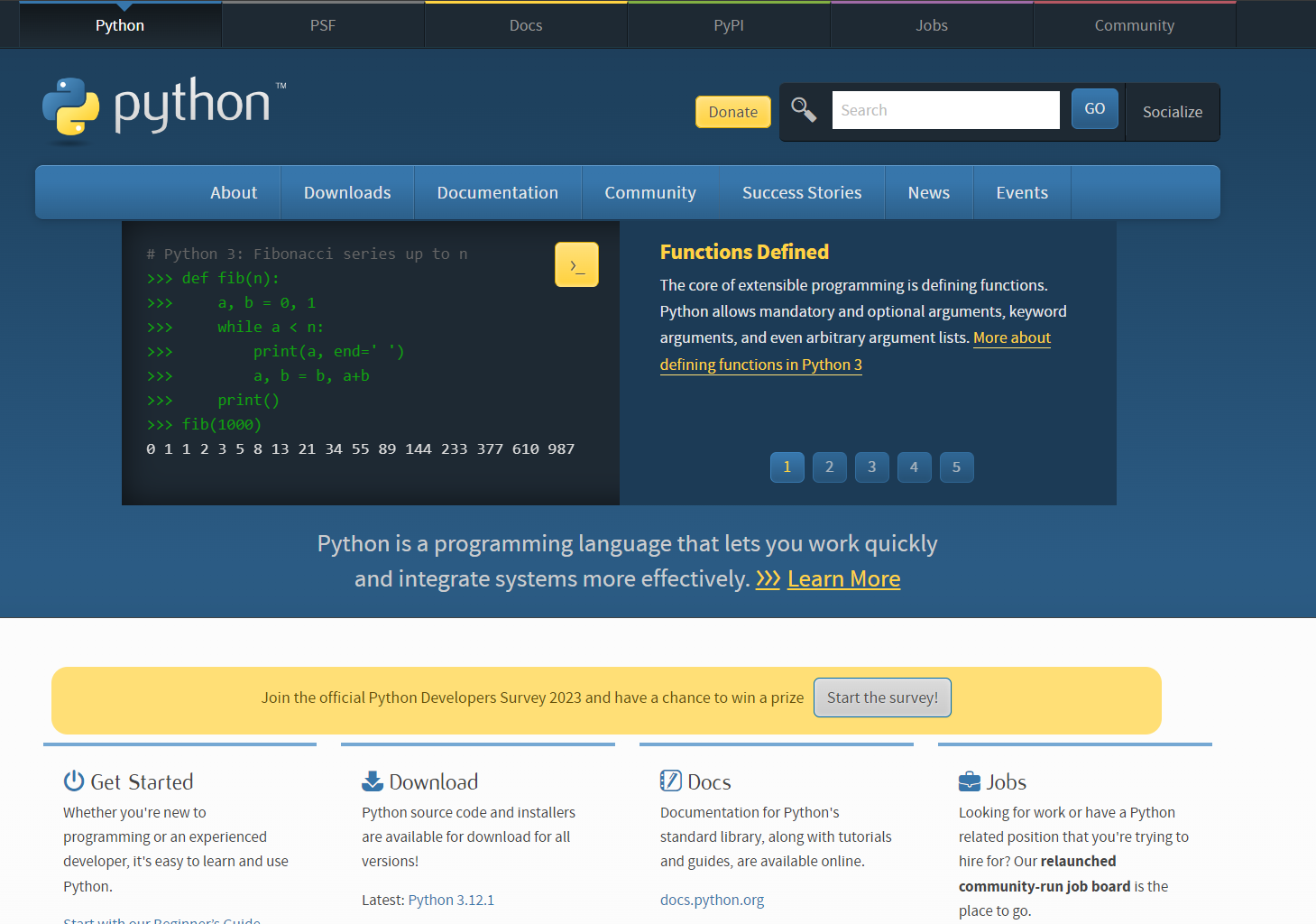
Python's extensive libraries make it a favorite among developers, and its syntax ensures that even beginners can grasp its concepts quickly. So, why not harness the power of Python to make our PUT requests with headers smoother than ever?
How to Make a PUT Request in Python with Headers
Now that we’ve covered the basics of APIs, HTTP requests, PUT requests, and headers, let’s dive into how to make a PUT request in Python with headers. In Python, you can make a PUT request with headers using the requests
library, which is a popular HTTP library for making HTTP requests. If you don't have it installed, you can install it using:
pip install requests
Here are examples of how to make a PUT request in Python with headers with different content types and data formats.
- PUT Request in python with JSON Data:
import requests
import json
url = 'https://api.example.com/data'
headers = {'Content-Type': 'application/json'}
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.put(url, headers=headers, data=json.dumps(data))
print(response.status_code)
print(response.json())
In this example, we're making a PUT request to the specified URL with JSON data. We set the 'Content-Type' header to 'application/json' to specify the data format.
- PUT Request in python with Form Data:
import requests
url = 'https://api.example.com/data'
headers = {'Content-Type': 'application/x-www-form-urlencoded'}
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.put(url, headers=headers, data=data)
print(response.status_code)
print(response.text)
In this example, we're making a PUT request with form data. We set the 'Content-Type' header to 'application/x-www-form-urlencoded' to specify the data format.
- PUT Request in Python with XML Data:
import requests
url = 'https://api.example.com/data'
headers = {'Content-Type': 'application/xml'}
data = '<xml><key1>value1</key1><key2>value2</key2></xml>'
response = requests.put(url, headers=headers, data=data)
print(response.status_code)
print(response.text)
In this example, we're making a PUT request with XML data. We set the 'Content-Type' header to 'application/xml' to specify the data format.
These examples demonstrate how to make PUT requests with different content types and data formats using the requests library in Python. Each example specifies the content type in the request headers and includes the data in the request body.
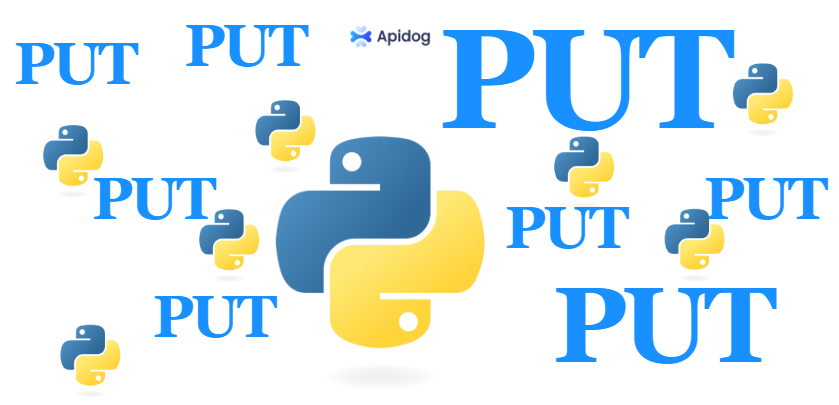
Understanding the PUT request with headers in Python.
A PUT request is an HTTP request method that is used to update or replace an existing resource on the server. It is not safe and is idempotent, meaning that calling it once or several times successively has the same effect (that is no side effect), whereas successive identical POST requests may have additional effects, akin to placing an order several times. The request has a body and the successful response has a body. The PUT request is not allowed in HTML forms.
Headers are additional information that is sent along with an HTTP request or response. Headers can be used to provide information about the request or response, such as the content type, encoding, or authentication information. Headers can also be used to provide additional information about the client or server, such as the user agent or server software.
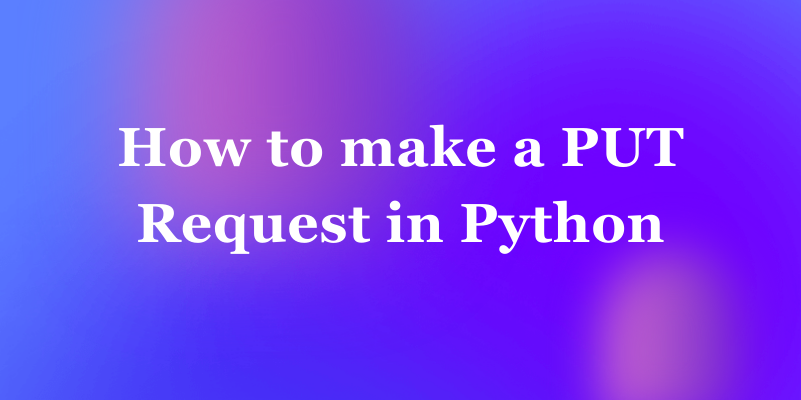
Using Apidog to test your Python PUT Request with headers
Apidog is a robust API testing tool that enables you to generate and store API requests, categorize them into collections, and collaborate with your team.
Here is how you can use Apidog to test your PUT request with headers:
- Open Apidog and create a new request.
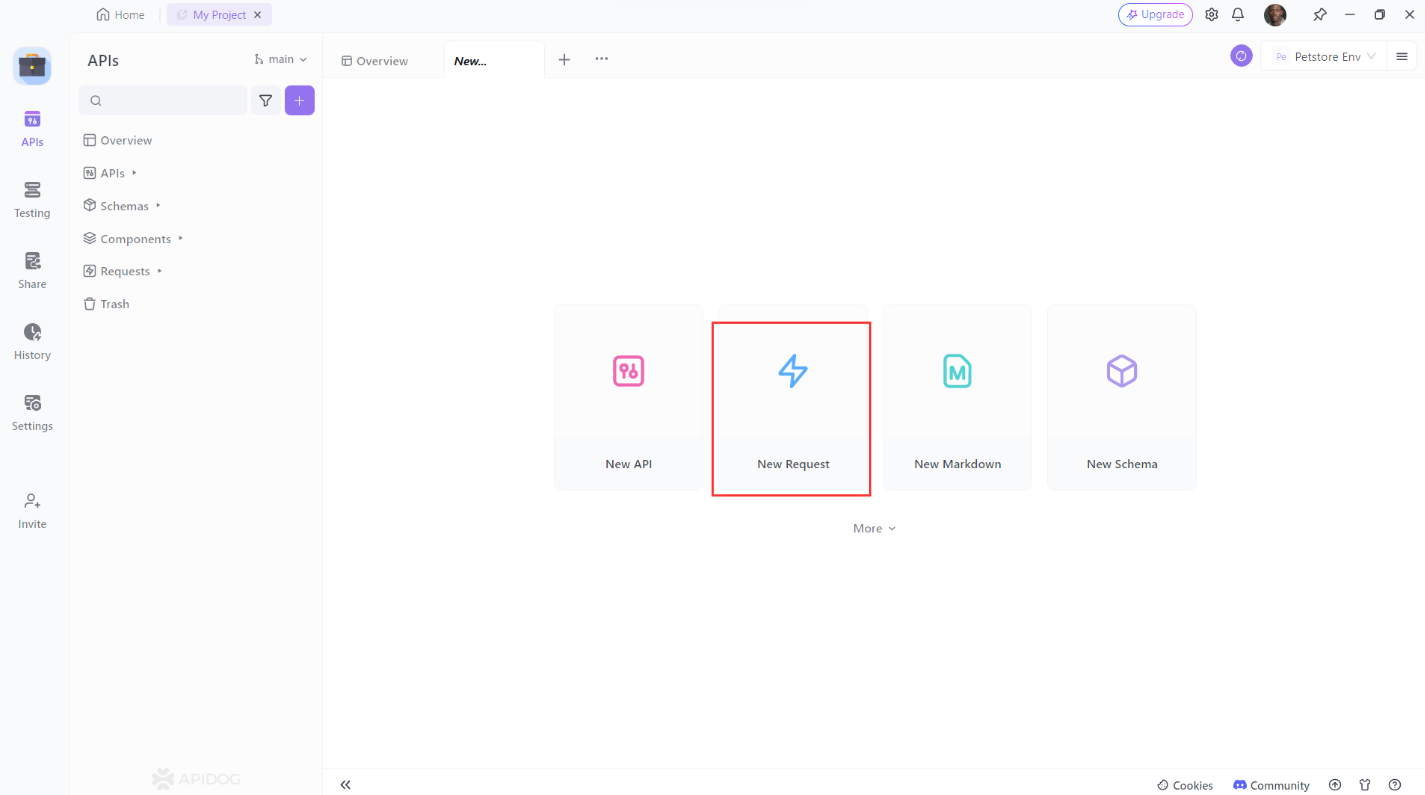
2. Set the request method to PUT.
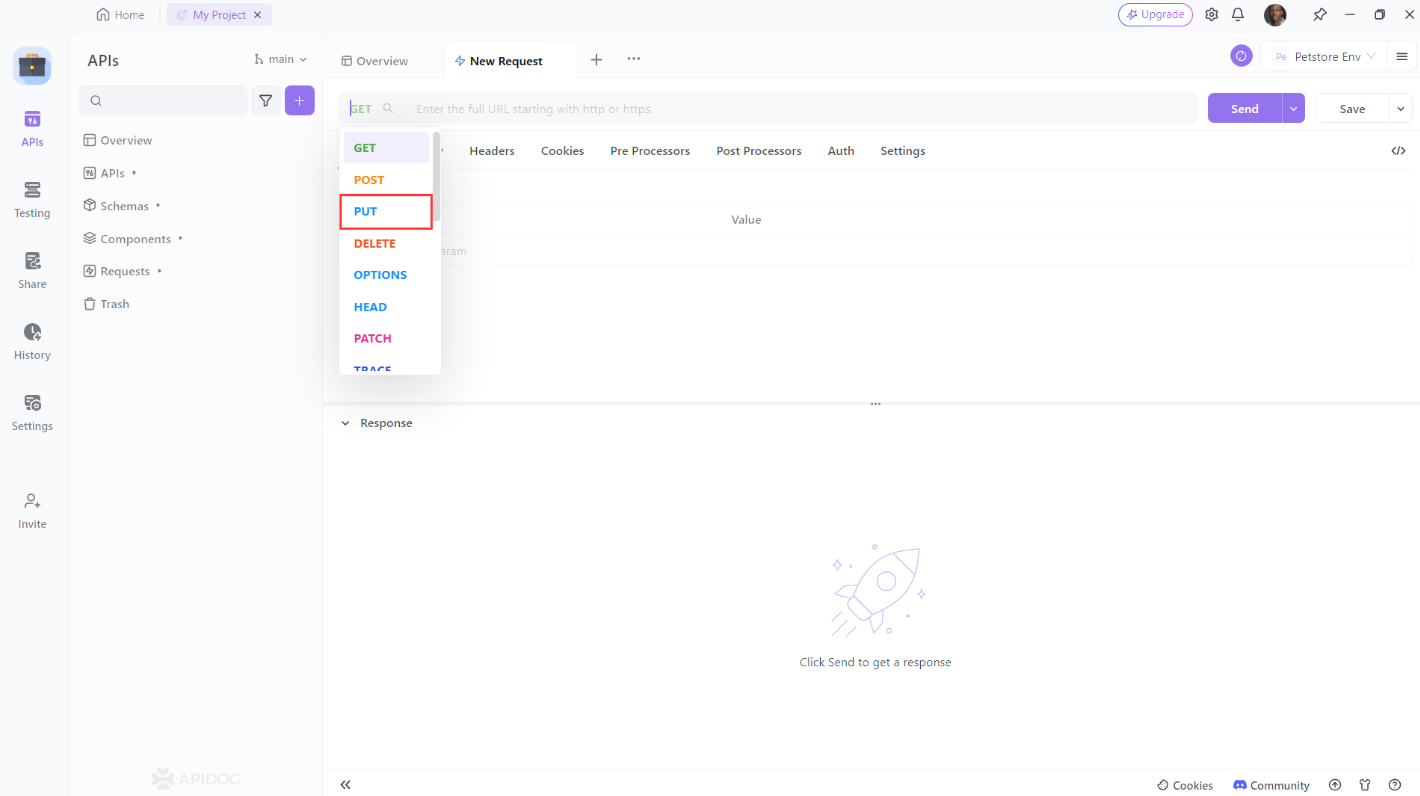
3. Enter the URL of the resource you want to update. Add your headers and or additional parameters you want to include then click the “Send” button to send the request.
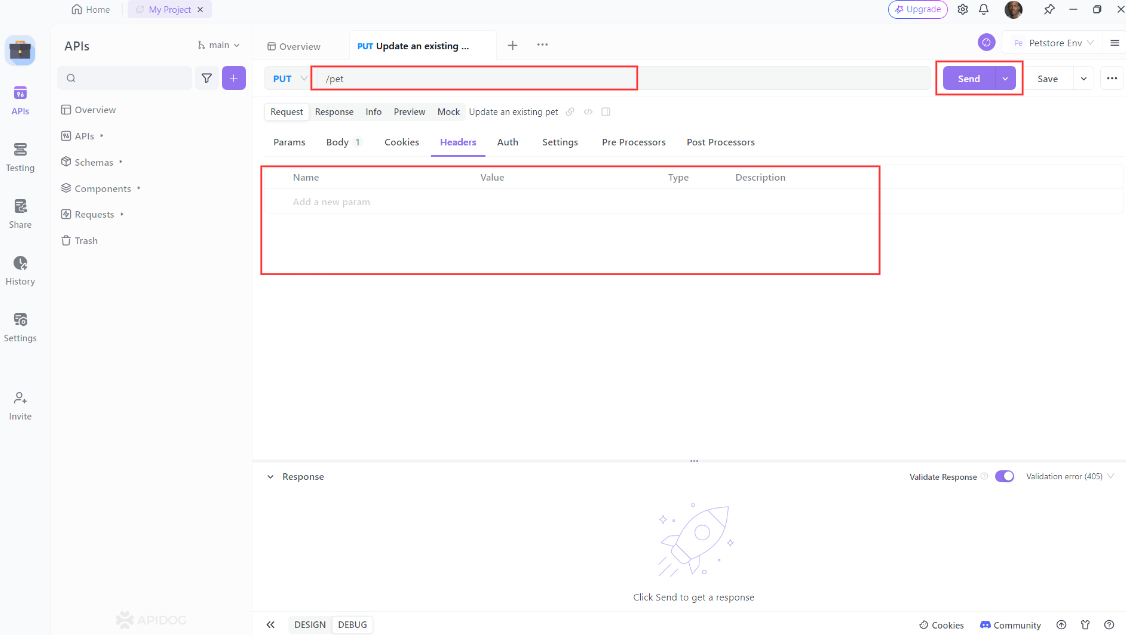
4. Verify that the response is what you expected.
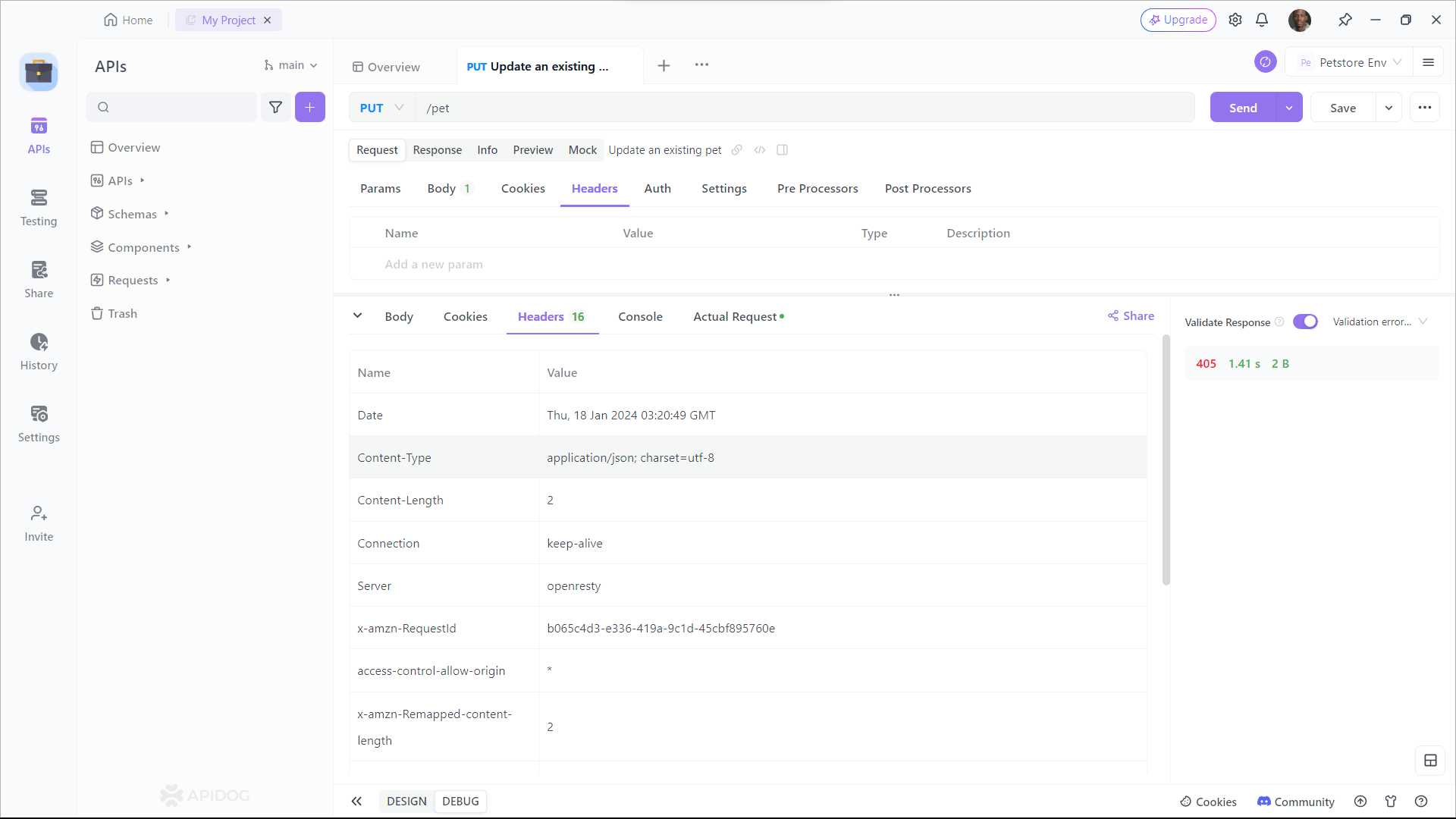
Best Practices for Making a PUT Request in Python with Headers
it is important to handle errors properly. You should always check the response status code to ensure that the request was successful. You should also handle any errors that may occur during the request. There are a few best practices to keep in mind.
1. First, always include appropriate error handling in your code to ensure that your program does not crash when an error occurs. You can use the try and except statements to handle errors in Python. For example, you can catch all exceptions using the base-class exception Exception or catch them separately and do different things.
2. Second, some endpoints require authentication using headers, tokens, or user credentials. Ensure your request includes these when needed. You can use the auth parameter in the requests library to provide authentication credentials. For example, you can use basic authentication with the HTTPBasicAuth class provided by the requests library. If you are accessing a URL that requires Windows authentication, you can use the Requests-NTLM library.
3. Third, when making a PUT request, ensure that the data is sent securely. If you are sending data over HTTP, it is recommended to use HTTPS instead. You can verify that your certificate is good by running openssl s_client -showcerts -connect example.com:443
from the command line and checking that it reports Verify return code: 0 (ok). You can also disable the security certificate check in Python requests by using a context manager that monkey patches requests and changes it so that verify=False is the default and suppresses the warning.
Conclusion
In this blog post, we’ve covered everything you need to know about making a PUT request in Python with headers. We’ve discussed the basics of APIs, HTTP requests, and PUT requests. We’ve also shown you how to use the requests library to make a PUT request in Python with headers and how to test your PUT request using Apidog.