In this blog post, we’ll be exploring the basics of GET requests and learning how to use Python Requests to make them. We’ll also pick up some cool tips and tricks along the way. Whether you’re a seasoned developer or just starting out on your coding adventure, this guide will equip you with all the juicy details about GET requests.
If you’re interested in the topic of PUT requests, you can check out the previous article by clicking on the link below.
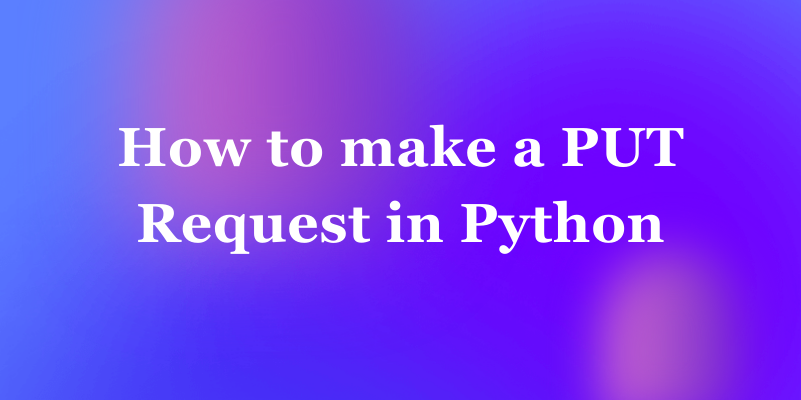
Fundamentals of HTTP Requests
Before delving into the intricacies of GET requests, let's first grasp the concept of an HTTP request. HTTP, short for Hypertext Transfer Protocol, serves as the protocol for transmitting data across the internet. An HTTP request entails a message dispatched by a client to a server, seeking a particular resource. Subsequently, the server issues a response containing the requested resource.
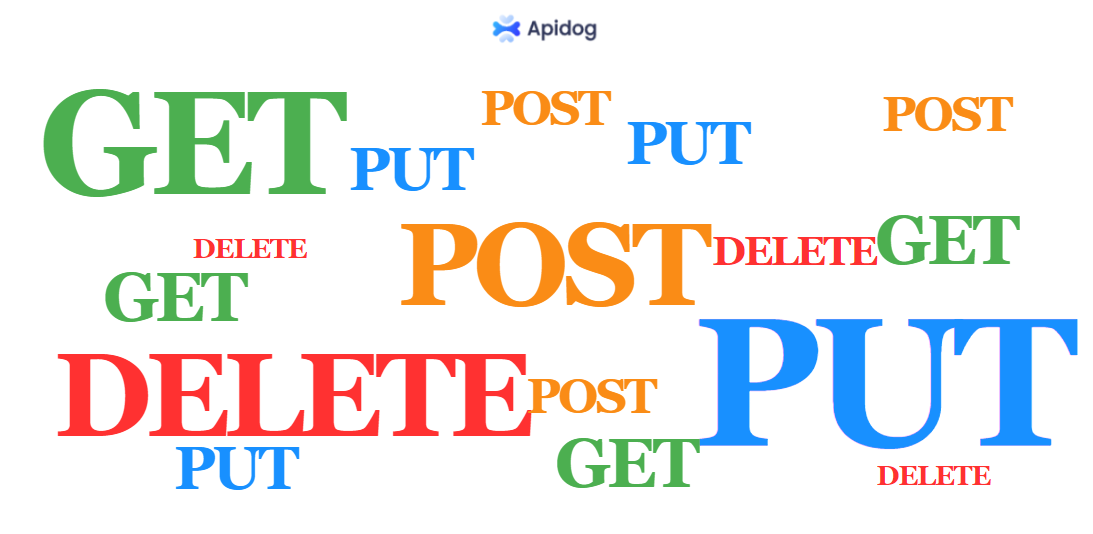
There are different HTTP methods, each with a specific purpose and indicating the nature of the request. The most common HTTP methods are GET, POST, PUT, and DELETE.
What is a GET request?
A GET request is an HTTP method employed to retrieve information from a server. Unlike other HTTP methods, such as PUT or POST, the primary purpose of a GET request is to fetch data rather than modify or update it. When you send a GET request to a server, you're essentially asking for specific information or resources located at a particular URL.
In simpler terms, a GET request is akin to making an inquiry to the server, saying, "Hey, I'd like to receive the data or resource located at this specific URL." It doesn't involve altering or replacing the existing information; instead, it's all about obtaining the requested data from the server.
What is Python?
Having covered the basics of HTTP requests, let’s now focus on Python, a high-level programming language that is renowned for its simplicity, readability, and versatility. Python enables developers to write clear, logical code for projects of all sizes. You can obtain the latest version of Python by visiting the official website and downloading it from there.
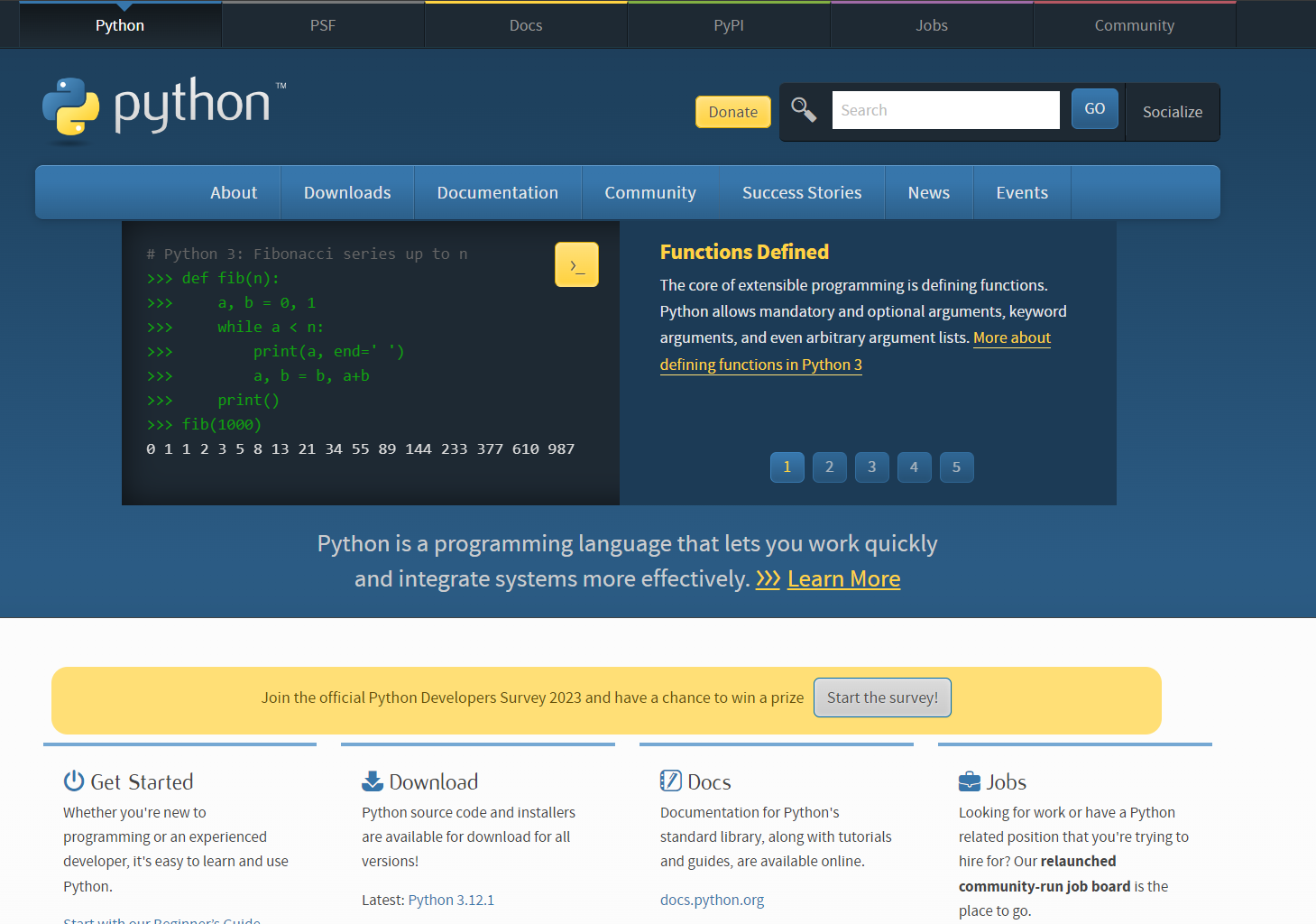
Python’s extensive libraries make it a favorite among developers, and its syntax ensures that even beginners can grasp its concepts quickly. So, why not harness the power of Python to make your GET requests smoother than ever?
How to make a GET request in Python
To make HTTP requests in Python, we often turn to the popular requests
library. If you don't have it installed, you can do this by running the following command in your terminal:
pip install requests
Once you have installed the requests library, you can import it into your Python script using the following code:
import requests
Now, let's delve into the code. Making a basic GET request involves importing the requests
module and using the get
method with the desired URL. Here's a simple example:
import requests
url = "https://api.example.com/data"
response = requests.get(url)
# Check if the request was successful (status code 200)
if response.status_code == 200:
print("GET request successful!")
print("Response:")
print(response.text)
else:
print(f"GET request failed with status code {response.status_code}")
In this example, we specify the URL we want to retrieve data from, use requests.get(url)
to make the GET request, and then check the status code of the response. A status code of 200 indicates success, and we print the response content.
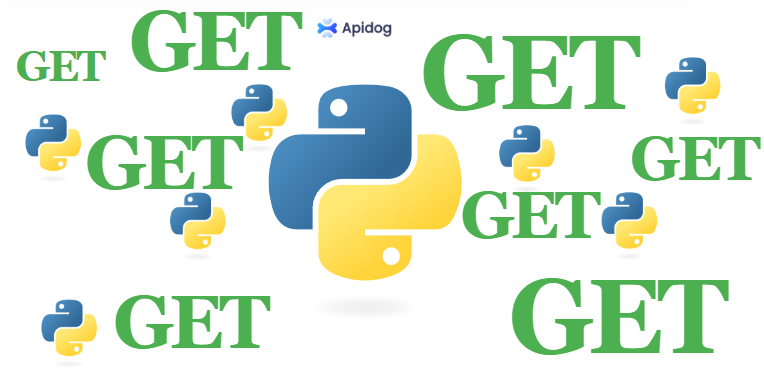
Understanding the GET request parameters in Python.
understanding the intricacies of GET request parameters is pivotal for tailoring your requests to specific needs. Let's explore the essential parameters and their roles in shaping your GET requests for seamless interactions with web resources.
URL:
The URL parameter specifies the location of the resource you intend to retrieve using the GET request.
Data:
Unlike PUT requests, GET requests typically don't send data in the request body. Instead, parameters are often included in the URL itself. For example, appending ?key1=value1&key2=value2
to the URL.
Headers:
Similar to PUT requests, headers in a GET request can be customized using the headers parameter. This is useful for specifying details like Content-Type or Authorization.
Authentication:
If the target endpoint demands authentication, the auth parameter enables you to provide the necessary credentials for secure access.
Timeout:
The timeout parameter allows you to set a maximum duration for the GET request, specifying the number of seconds to wait for a response before timing out.
Proxies:
For scenarios requiring proxy usage, the proxies parameter allows you to specify the proxy URL to facilitate the GET request through a designated intermediary.
Verify:
Similar to PUT requests, the verify parameter plays a role in SSL certificate verification. Setting it to True verifies the SSL certificate, while False disables the verification.
Allow Redirects:
By default, GET requests follow redirects automatically. If you wish to disable this behavior, set the allow_redirects parameter to False.
Example: Utilizing the URL Parameter in a GET Request:
import requests
url = "https://api.example.com/resource?key1=value1&key2=value2"
response = requests.get(url)
if response.status_code == 200:
print("GET request successful!")
print("Response:")
print(response.text)
else:
print(f"GET request failed with status code {response.status_code}")
In this example, the data is included in the URL to retrieve a resource with specific key-value parameters.
As you embark on your Python journey, incorporating these insights will undoubtedly enhance your capabilities in making GET requests.
Using Apidog to Test Your Python GET Request
Apidog is a robust API testing tool that enables you to generate and store API requests, categorize them into collections, and collaborate with your team. Here's a guide on using Apidog to test your GET request:
- Open Apidog and create a new request.
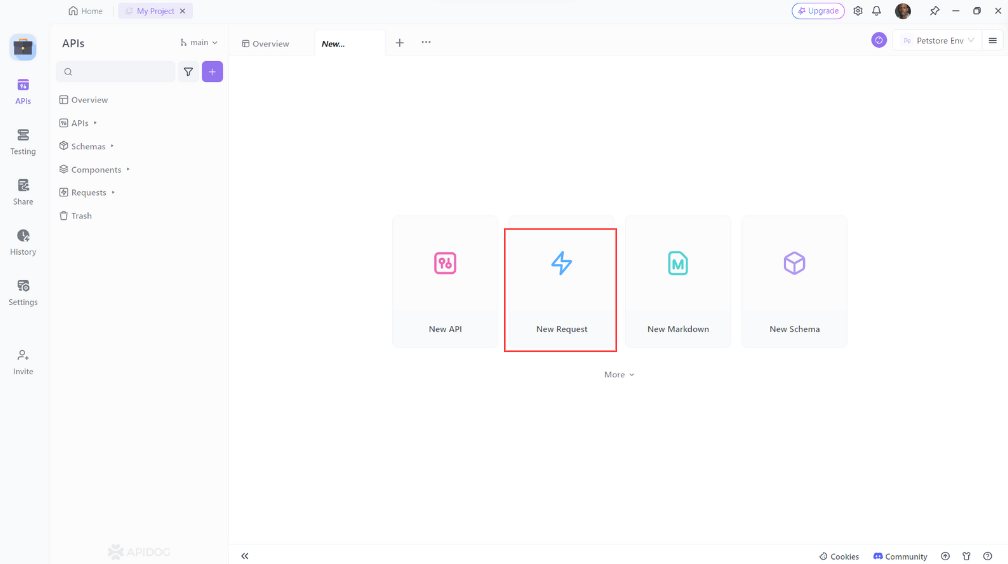
2. Set the request method to GET.
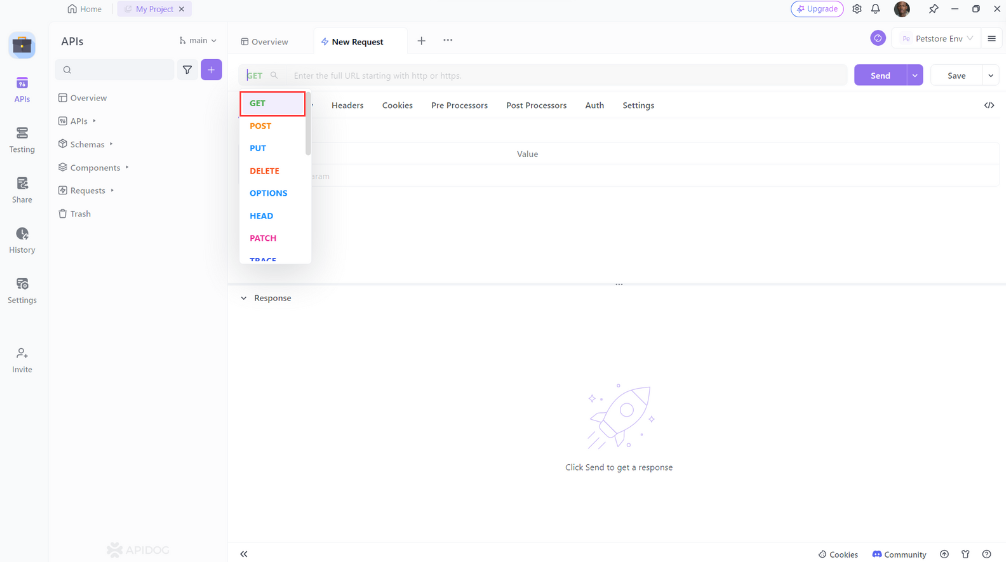
3. Enter the URL of the resource you wish to update. You can also add any additional headers or parameters you want to include, and then click the 'Send' button to send the request
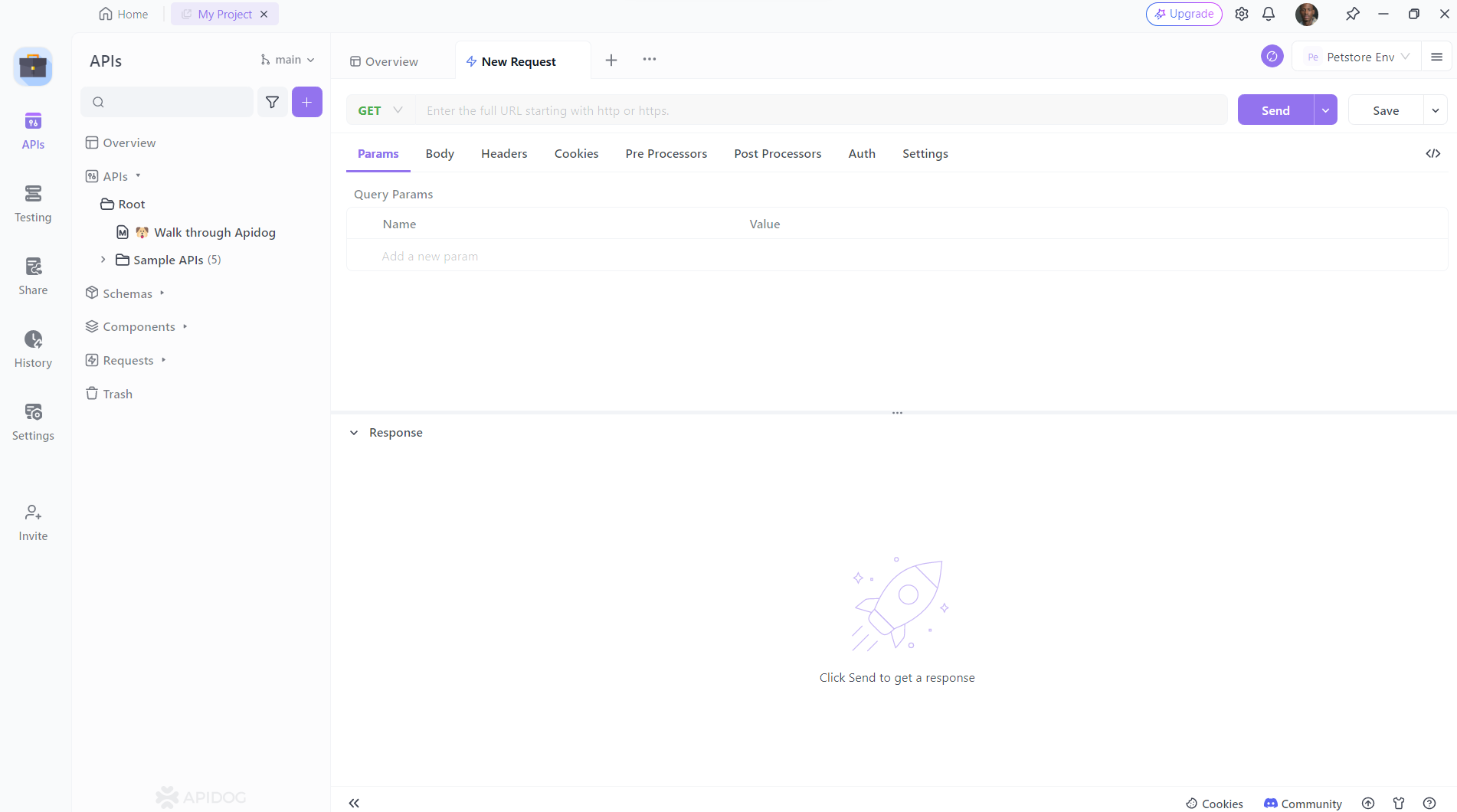
4. Confirm that the response matches your expectations.
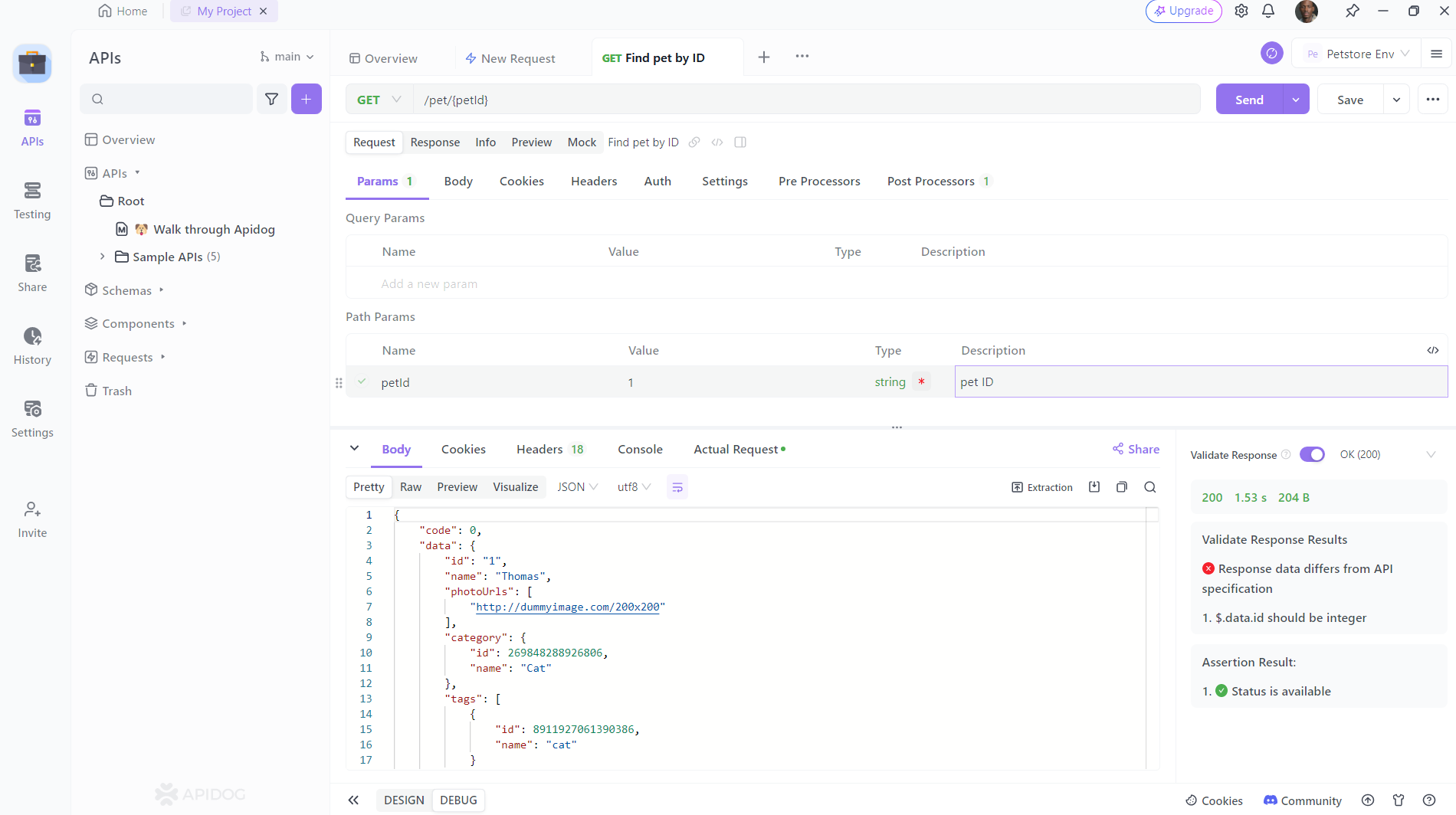
Best practices for making a GET Request.
When engaging in a GET request, it becomes crucial to manage errors effectively. It is imperative to consistently examine the response status code to ascertain the success of the request. Additionally, it is essential to adeptly address any potential errors that might arise during the execution of the request. Taking these measures ensures a robust and reliable handling of errors, promoting a smoother and more resilient GET request process.
There are several best practices to keep in mind. Here are some of them:
- Use HTTPS: Always use HTTPS instead of HTTP to ensure that your data is encrypted and secure.
- Keep URLs short: URLs should be kept as short as possible to make them easy to read and share. Avoid using long query strings or parameters in the URL.
- Use query parameters: Use query parameters to pass data to the server. This makes it easy to filter, sort, and search for data.
- Limit the number of parameters: Limit the number of parameters you use in your GET requests. Too many parameters can make your requests difficult to read and understand.
- Avoid sensitive data: Do not include sensitive data in your GET requests. Sensitive data should be sent using POST requests instead.
- Cache responses: If possible, cache the responses from your GET requests to improve performance.
- Use pagination: If you are requesting a large amount of data, use pagination to break it up into smaller chunks.
Conclusion
In this blog post, we've gone on a little adventure into the world of GET requests. We've learned how to make a GET request in Python and had some fun testing it out with Apidog. We've also explored the cool ways you can tweak and customize your GET request using different parameters.
Now that you've got this awesome new knowledge under your belt, you'll be all set to rock those GET requests in your projects. So go ahead, give it a try, and let those GET requests work their magic!
Happy Coding !🚀🚀