How to make a Delete Request in Python ?
Discover the process of executing DELETE requests in Python with the `requests` library. This tutorial encompasses all aspects, from configuring your environment to adhering to best practices, ensuring secure and efficient API interactions.
In this article, we delve into the fundamentals of DELETE requests, demonstrate their implementation via Python’s requests
library, and highlight essential best practices. This comprehensive guide is designed to equip developers of all levels with the necessary knowledge to proficiently utilize DELETE requests.
HTTP Request Basics
Before we dive into the DELETE request, let’s first understand what an HTTP request is. HTTP stands for Hypertext Transfer Protocol, which is a protocol used to transfer data over the internet. An HTTP request is a message sent by a client to a server, requesting for a specific resource. The server then responds with the requested resource.
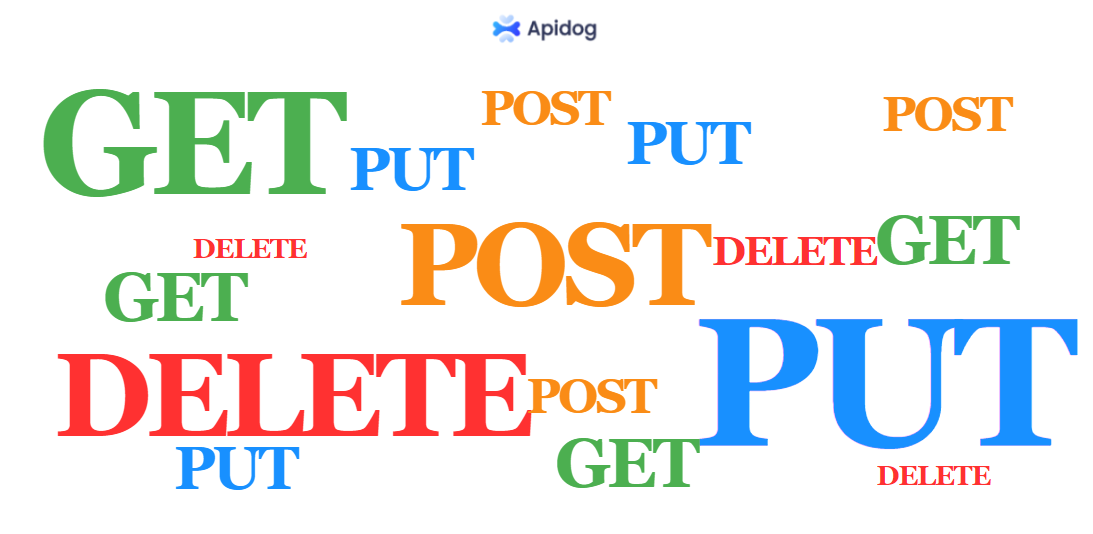
There are various HTTP methods, also referred to as HTTP requests, each serving a distinct purpose and conveying the nature of the request. The most prevalent HTTP methods include GET, POST, PUT and DELETE.
What is a DELETE request?
A DELETE request is a method used in the HTTP protocol to indicate that a client wants to delete a specific resource from a server. When a DELETE request is successful, it can result in various response status codes, such as:
- 202 (Accepted): The action will likely succeed but has not yet been enacted.
- 204 (No Content): The action has been enacted, and no further information is to be supplied.
- 200 (OK): The action has been enacted, and the response message includes a representation describing the status.
What is Python?
Now that we've covered the basics of HTTP requests, let's talk about the programming language that's going to be our trusty companion on this journey – Python. Python is renowned for its simplicity, readability, and versatility. It's a high-level language that enables developers to write clear, logical code for projects of all sizes. The latest version of Python can be obtained by visiting the official website and downloading it from there.
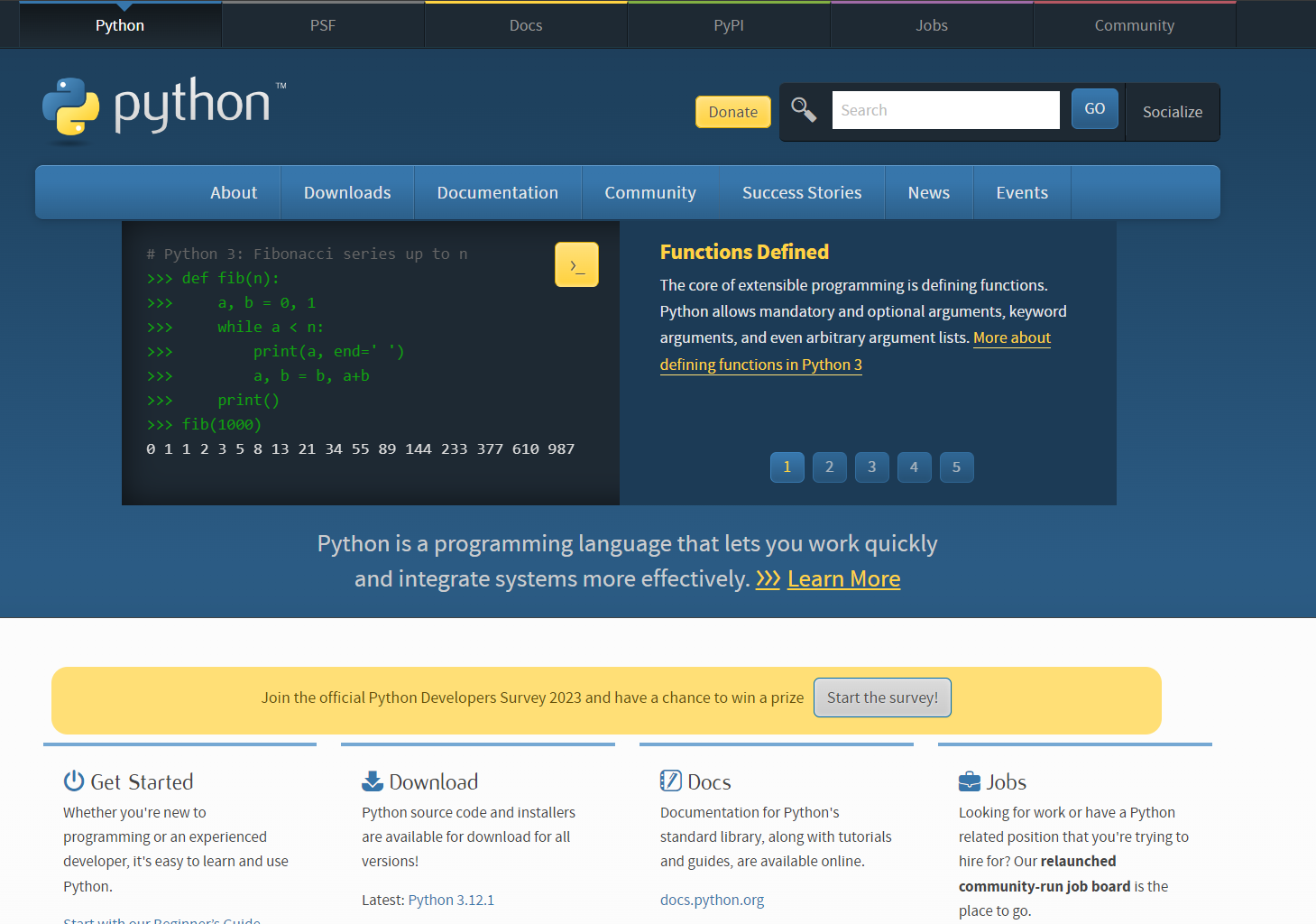
Python's extensive libraries make it a favorite among developers, and its syntax ensures that even beginners can grasp its concepts quickly.
How to make a DELETE request using Python
To make a DELETE request using Python, you can use the requests
library, which provides a simple method to perform this action. Here’s a basic example of how to use it:
import requests
# Function to make a DELETE request with detailed response
def make_delete_request(url):
try:
# Make the DELETE request
response = requests.delete(url)
# Check if the request was successful
if response.status_code in [200, 202, 204]:
print(f"DELETE request to {url} was successful.")
print(f"Status Code: {response.status_code}")
if response.content:
print("Response Content:")
print(response.content.decode())
else:
print("No content returned.")
else:
print(f"DELETE request to {url} failed.")
print(f"Status Code: {response.status_code}")
if response.content:
print("Response Content:")
print(response.content.decode())
except requests.exceptions.RequestException as e:
# Print any error that occurs during the request
print(f"An error occurred: {e}")
# URL to send the DELETE request to
url = 'http://example.com/api/resource'
# Call the function with the URL
make_delete_request(url)
This function attempts to send a DELETE request to the specified URL. It prints out a message indicating whether the request was successful or not, along with the status code. If there’s any content returned in the response, it will print that out as well. In case of any exceptions, such as network issues, it will print out the error encountered.
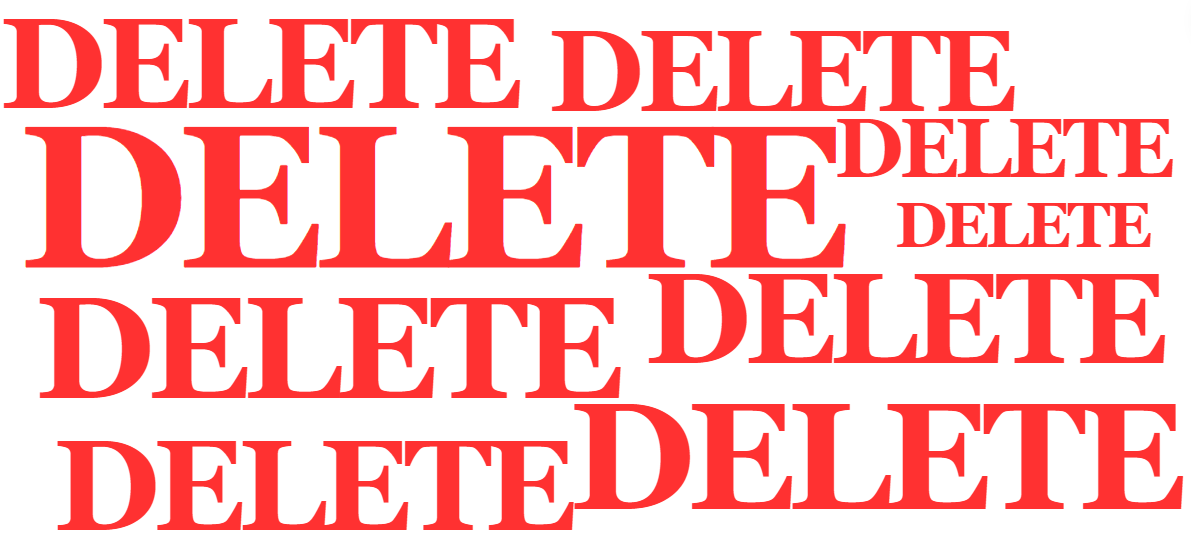
Understanding the DELETE request parameters in Python.
In Python, when making a DELETE request using the requests
library, you can pass several parameters to customize the request. Here’s a breakdown of the most common parameters:
- url: The URL for the request. This is the only required parameter.
- data: The data to send in the body of the request. For a DELETE request, this is typically not used since the URL should identify the resource to be deleted.
- json: A JSON serializable Python object to send in the body of the request.
- headers: A dictionary of HTTP headers you want to send with the request.
- params: A dictionary or bytes to be sent in the query string of the new request.
- auth: An auth tuple to enable Basic/Digest/Custom HTTP Auth.
- cookies: A dictionary of cookies to send with the request.
- files: A dictionary of ‘filename’ to file-like-objects for multipart encoding upload.
- timeout: How many seconds to wait for the server to send data before giving up.
- allow_redirects: Boolean. Enable/disable GET/OPTIONS/POST/PUT/PATCH/DELETE/HEAD redirection.
- proxies: A dictionary of the protocol to the proxy URL.
- verify: Either a boolean, in which case it controls whether we verify the server’s TLS certificate, or a string, in which case it must be a path to a CA bundle to use.
- stream: If
False
, the response content will be immediately downloaded.
Here’s an example of how you might use some of these parameters in a DELETE request:
import requests
# The URL of the resource you want to delete
url = 'http://example.com/api/resource'
# Additional headers
headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
}
# Query parameters
params = {
'param1': 'value1',
'param2': 'value2'
}
# Make the DELETE request with additional parameters
response = requests.delete(url, headers=headers, params=params)
# Check the response
if response.ok:
print('Resource deleted successfully.')
else:
print(f'Failed to delete resource. Status code: {response.status_code}')
In this example, we’re sending a DELETE request with additional headers and query parameters. The response.ok
will check if the response status code is less than 400, indicating that the request was successful. Remember to replace 'YOUR_ACCESS_TOKEN'
with your actual access token and the url
with the resource you wish to delete.
Using Apidog to Test Your Python DELETE Request
Apidog is a powerful tool for testing APIs. It allows you to create and save API requests, organize them into collections, and share them with your team.
Here is how you can use Apidog to test your DELETE request:
- Open Apidog and create a new request.
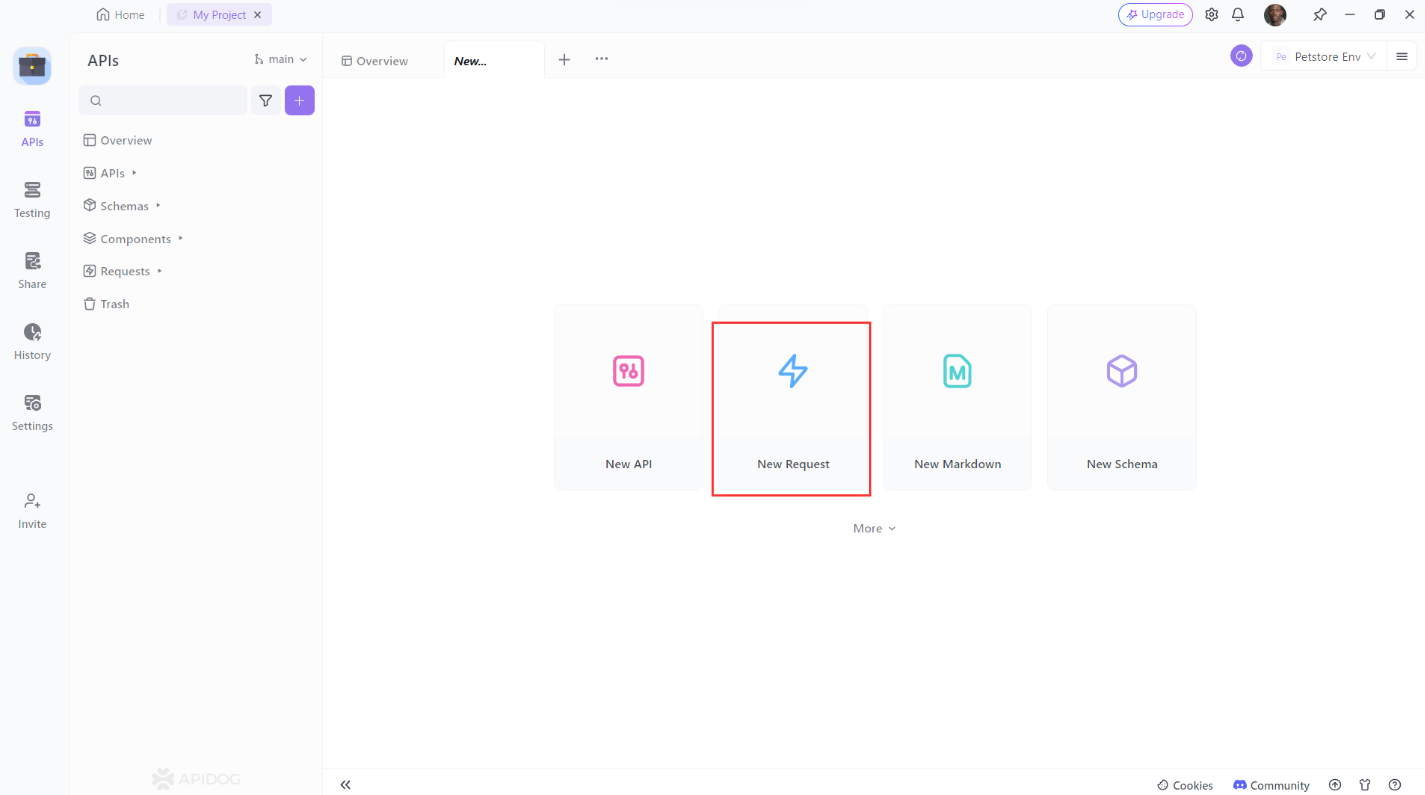
2. Set the request method to DELETE .
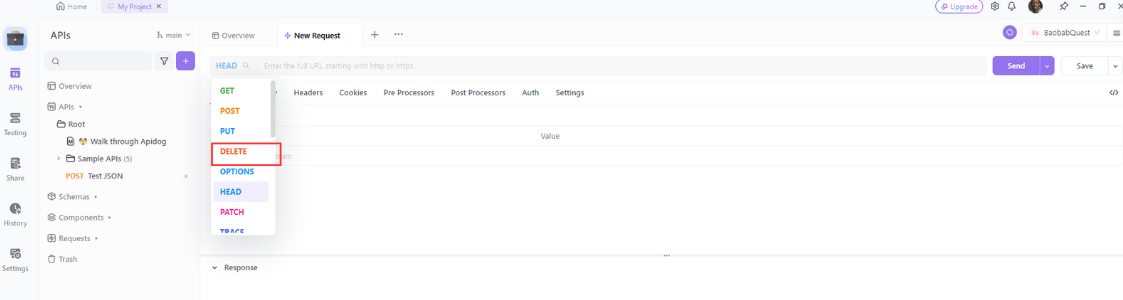
3. Enter the URL of the resource you want to update. Add any additional headers or parameters you want to include then click the “Send” button to send the request.

4. Verify that the response is what you expected.
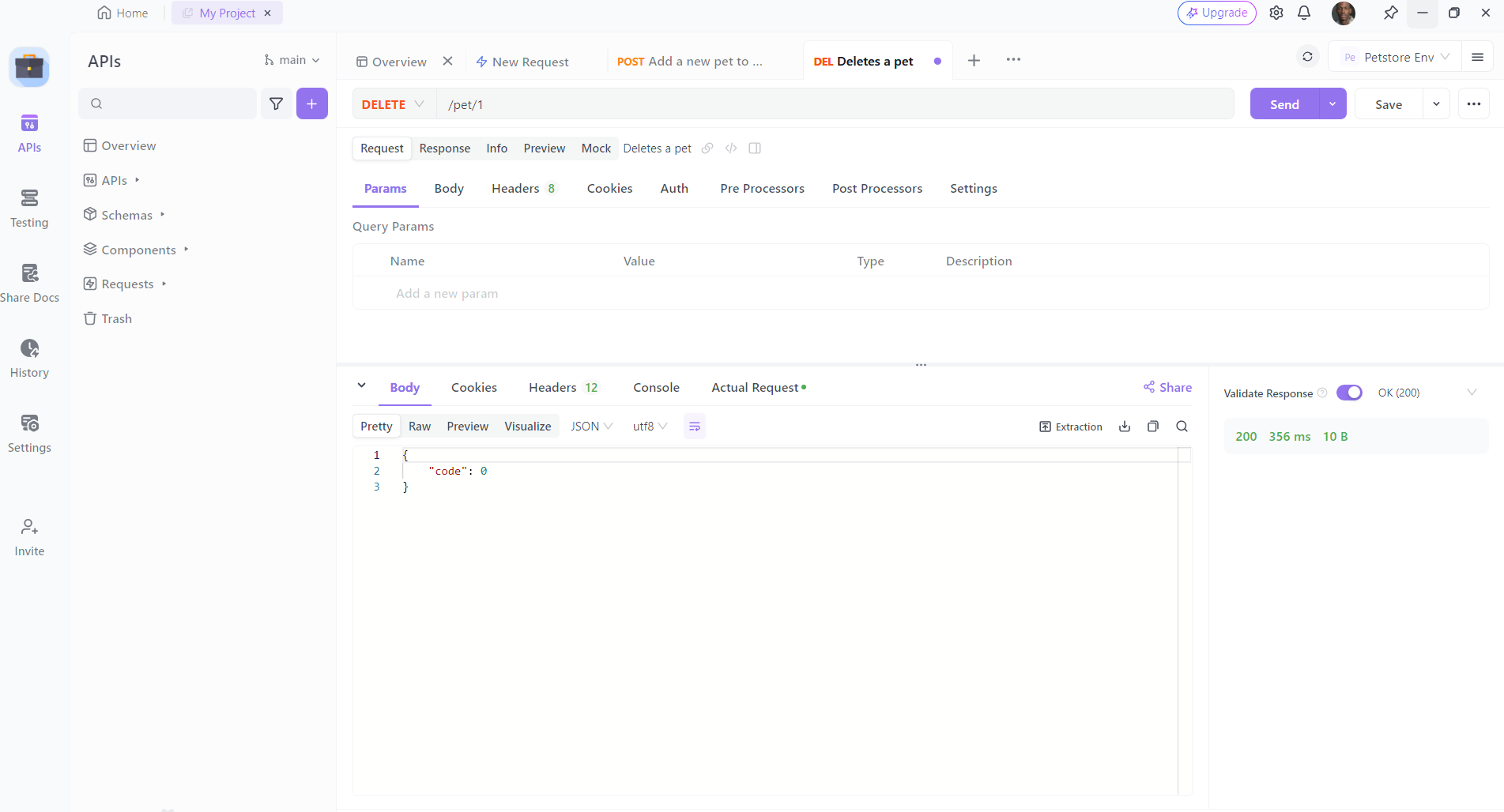
Best practices for making a DELETE Request.
When making a DELETE request, it’s important to follow best practices to ensure the request is handled properly and securely. Here are some key best practices:
- Idempotency: Ensure that your DELETE implementation is idempotent, meaning that multiple identical DELETE requests have the same effect as a single request.
- Authorization: Implement proper access controls and authentication mechanisms to prevent unauthorized users from deleting resources.
- Validation: Validate all input data to avoid unintended deletions or security vulnerabilities.
- Error Handling: Provide clear error messages for failed deletions, including why the deletion failed and what the client can do about it.
- Logging: Keep logs of DELETE requests for auditing purposes, including the identity of the requester, the time of the request, and the outcome.
- Resource Verification: Before performing the deletion, verify that the resource exists and that the client has permission to delete it.
- Response Codes: Use appropriate HTTP status codes to indicate the outcome of the request. For example, return a
404 Not Found
if the resource doesn’t exist, or a403 Forbidden
if the user is not authorized to delete the resource. - API Documentation: Clearly document how the DELETE method should be used in your API, including any required parameters or headers.
Following these practices will help maintain the integrity and security of the application or API you’re working with.
Conclusion
Mastering the DELETE request in Python is essential for developers who manage server resources via APIs. Utilizing the requests
library streamlines the process of communicating with web services, allowing for the seamless removal of resources. Adherence to best practices, including thorough input validation, secure API usage, and comprehensive testing, is crucial for building resilient and secure applications. Equipped with these methodologies, developers can confidently employ Python to orchestrate effective HTTP interactions within their web development endeavors.