Creating APIs seamlessly integrating with evolving data structures is crucial in API development. JSON Schema, a widely adopted standard for API definition, provides a robust framework for defining data structures and validation rules in combination with OpenAPI.
Within this framework, the keywords oneOf, anyOf, and allOf emerge as powerful tools for building flexible and adaptable APIs. oneOf helps specify multiple valid schemas for a single data structure.
anyOf provides a mechanism for validating a data structure against other available schemas. allOf combines multiple schema options into a single validation rule, ensuring a data structure adheres to all specified constraints. Apidog helps APIs seamlessly adapt to diverse data needs.
What is oneOf
The term "oneOf” refers to a construct or keyword used in the context of schema definitions like JSON schemas. "oneOf" allows you to specify multiple subschemas, and a valid instance of the schema must adhere to the constraints of exactly one of these subschemas. For example, consider a JSON schema for a person, where a person can be either an adult or a child.
The "oneOf" keyword could specify two subschemas, one for adults and one for children. The document being validated must satisfy the constraints of either the adult or child schema but not both. Here is how it would look in a JSON schema.
{
"type": "object",
"oneOf": [
{
"properties": {
"age": { "type": "integer", "minimum": 18 }
},
"required": ["age"]
},
{
"properties": {
"age": { "type": "integer", "maximum": 17 }
},
"required": ["age"]
}
]
}
In this example, the "oneOf" keyword is used to define two subschemas: one for adults and one for children. The document being validated must satisfy the constraints of the adult or child schema, but not both. The "age" property is a discriminator to determine which subschema should be applied.
This flexibility is beneficial when you have diverse valid structures for a JSON document based on different conditions. The "oneOf" keyword ensures mutual exclusivity among the defined subschemas, guaranteeing that a valid instance conforms to only one of the specified structures. This capability makes "oneOf" a valuable tool for accurately describing complex data models and maintaining data integrity in various scenarios.
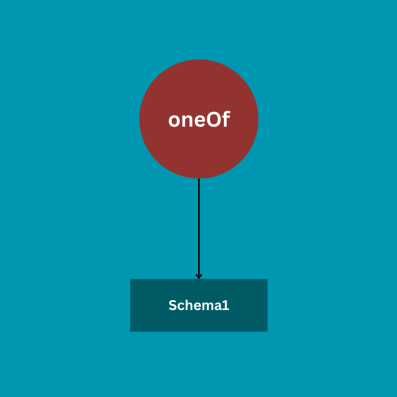
What is anyOf
The “anyOf" keyword is another construct to define a schema where the validated instance must conform to at least one specified subschema. Unlike "oneOf," which enforces exclusive adherence to one subschema, "anyOf" offers more flexibility by permitting the instance to satisfy the constraints of at least one of the specified subschemas.
Let's consider a practical scenario where "anyOf" might be beneficial. Imagine working with JSON data representing individuals, some adults, and others students. Both adults and students have distinct properties, and you want to create a schema that accommodates both cases.
The "anyOf" keyword enables you to express this flexibility, allowing the JSON document to be valid if it conforms to the schema for adults or students. Here is how this example is represented in a JSON schema.
{
"type": "object",
"anyOf": [
{
"properties": {
"name": { "type": "string" },
"age": { "type": "integer", "minimum": 18 }
},
"required": ["name", "age"]
},
{
"properties": {
"name": { "type": "string" },
"grade": { "type": "string", "enum": ["A", "B", "C"] }
},
"required": ["name", "grade"]
}
]
}
In this example, the "anyOf" keyword is used to define two subschemas: one for adults and one for students. The JSON document is considered valid if it satisfies the constraints of the adult or student schema. This allows for a flexible representation of diverse data structures within the same overarching schema, making "anyOf" a powerful tool for handling varied data scenarios.
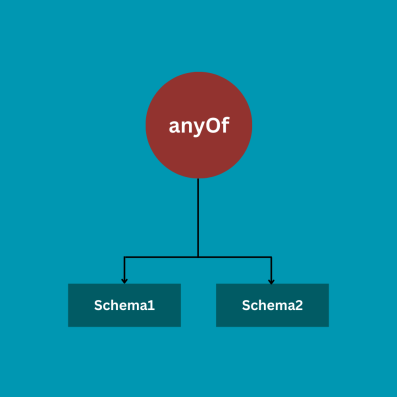
OneOf vs anyof: What is the difference in z schema
In Z Schema, OneOf
and AnyOf
are both used to define multiple schemas, but they serve different purposes.
OneOf:
OneOf
is used when exactly one of the defined schemas must match.
It ensures that the data validates against only one of the specified schemas.
Example:
{
"OneOf": [
{"type": "string"},
{"type": "number"}
]
}
This schema ensures that the data is either a string or a number, but not both.
AnyOf:
AnyOf
is used when any one or more of the defined schemas can match.
It allows the data to validate against multiple specified schemas.
Example:
{
"AnyOf": [
{"type": "string"},
{"type": "number"}
]
}
What is allOf
The "allOf" keyword in JSON Schema defines a schema where the validated instance must conform to all specified subschemas. Essentially, it represents a logical AND operation for the subschemas. This means that the validated JSON document must satisfy the constraints of every subschema listed under "allOf." Here is an example:
{
"allOf": [
{
"properties": {
"name": { "type": "string" },
"age": { "type": "integer", "minimum": 18 }
},
"required": ["name", "age"]
},
{
"properties": {
"hasDegree": { "type": "boolean", "default": true }
},
"required": ["hasDegree"]
}
]
}
In this example, the "allOf" keyword combines two subschemas. The first subschema defines properties for adults, including a name and age with specific constraints. The second subschema introduces a property for individuals with a degree, in this case, a boolean property "hasDegree" with a default value of true. The combined effect of "allOf" is that the validated JSON document must adhere to both constraints. It must have a name, age (satisfying the first subschema), and also include the "hasDegree" property (satisfying the second subschema).
The "allOf" keyword is useful when creating a schema that encompasses the properties and constraints from multiple subschemas, ensuring that the validated instance meets all specified conditions. This logical AND operation differs from "anyOf" and "oneOf," allowing flexibility in satisfying at least one or exactly one of the specified subschemas.
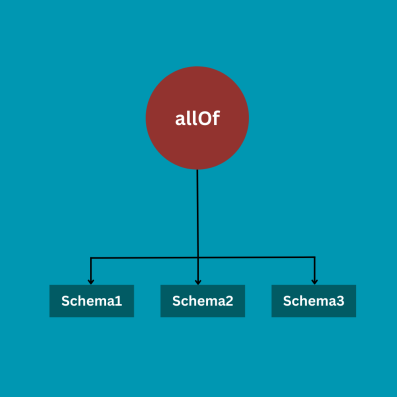
What is Apidog?
Apidog is a versatile API integration platform that simplifies API testing, debugging, design, mocking, and documentation. It offers a user-friendly interface, collaborative tools, and features for thorough API evaluations.
Apidog excels in documenting API responses with customizable layouts and facilitates collaborative efforts within teams. Its testing tools enable visual assertion addition and testing branch creation. The platform also aids in monitoring API activity and provides efficient mocking capabilities, eliminating the need for scripting in development.
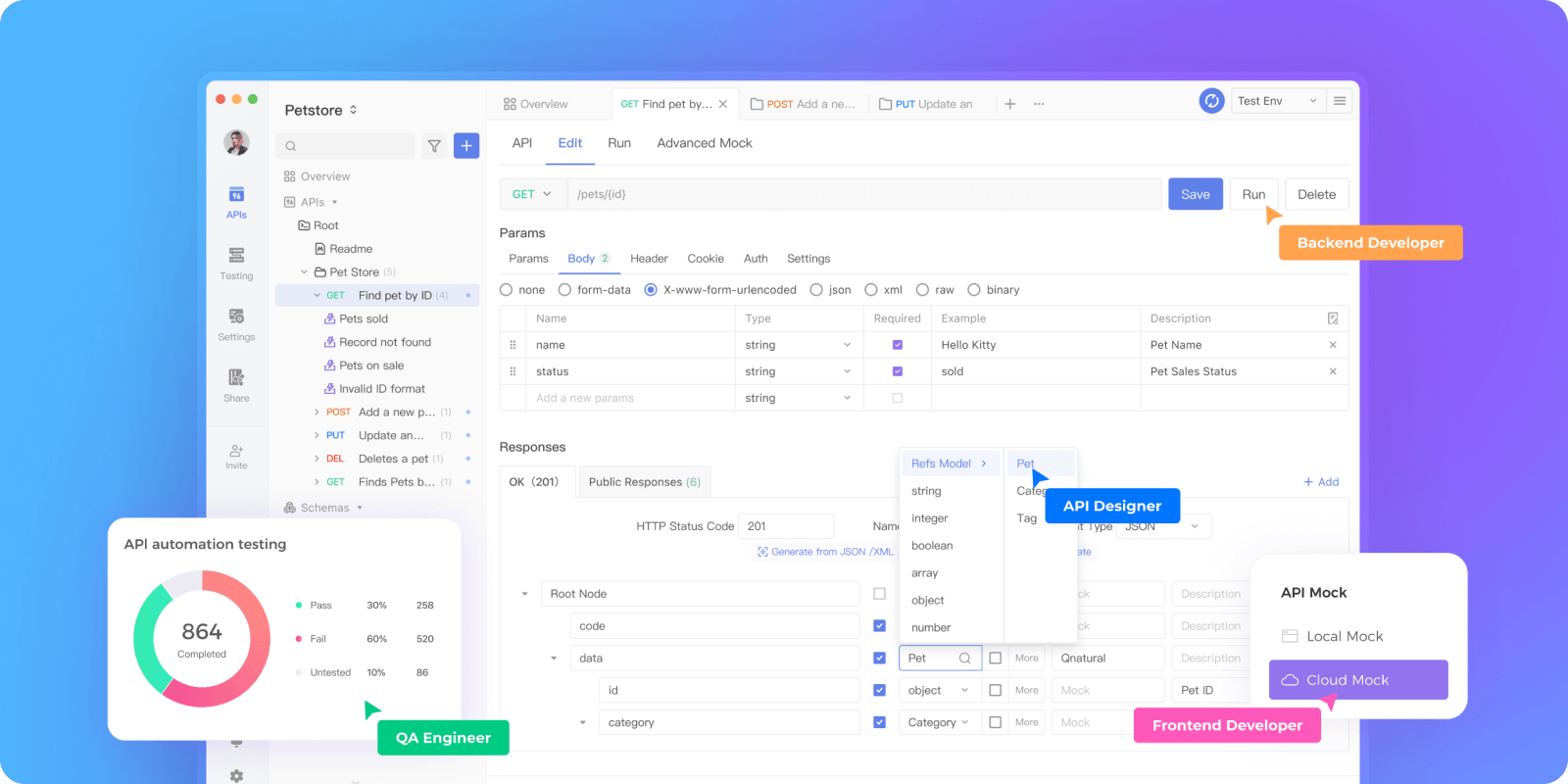
Apidog JSON schemas with oneOf, anyOf and allOf
Now that you know what JSON schemas are and how they employ oneOf, anyOf, and allOf in their structure, we will define them in Apidog. To efficiently create these schemas in Apidog, ensure you have Apidog downloaded in your system or have an account to use Apidog services online.
How to Create OneOf
- Create a new project in Apidog with HTTP type.
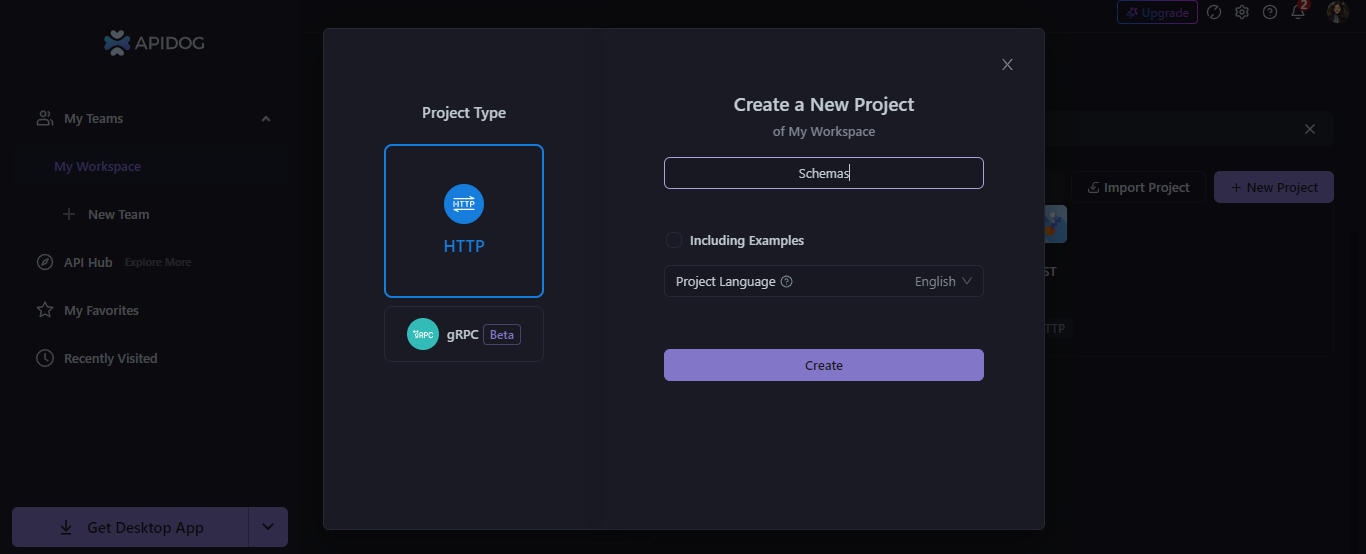
2. Create a new Schema by clicking on the new Schema button.
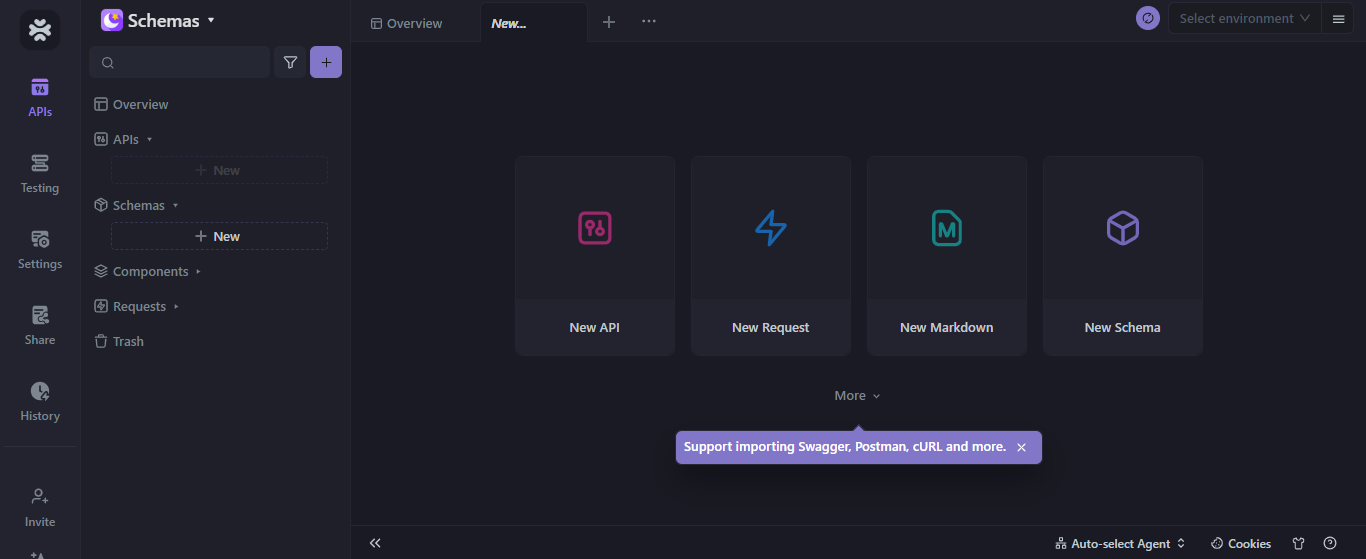
3. Click on Generate from JSON option and go to the JSON schema option.
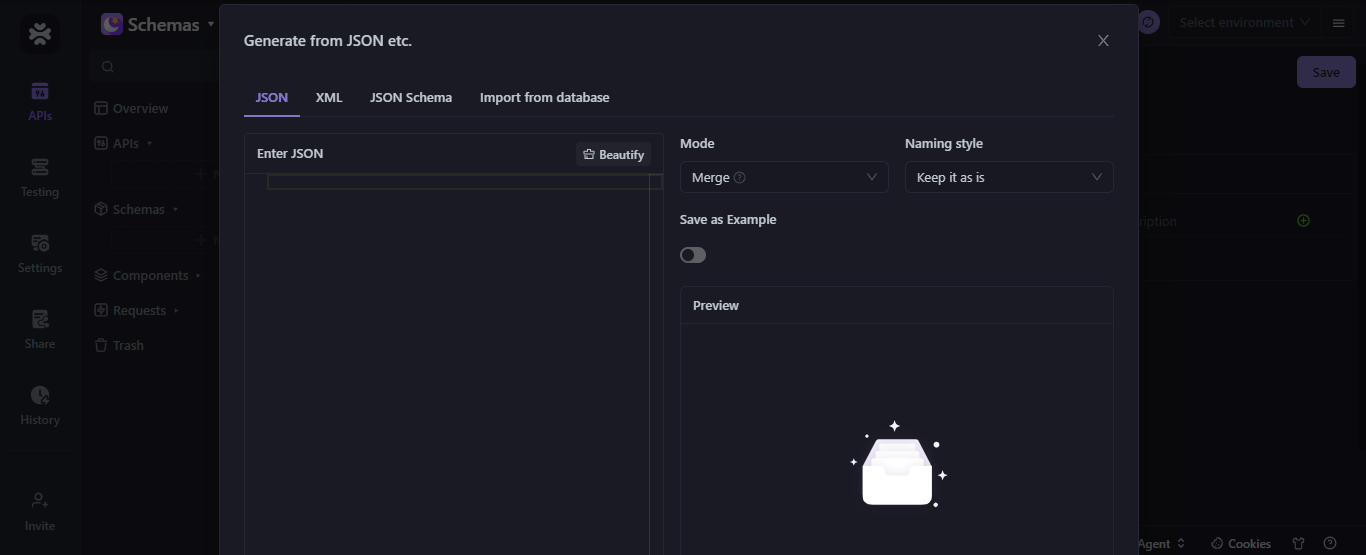
4. Enter the following code in the editor.
{
"title": "Payment Schema Example",
"type": "object",
"properties": {
"payment": {
"oneOf": [
{
"type": "object",
"properties": {
"amount": {
"type": "number"
},
"currency": {
"type": "string"
}
},
"required": ["amount", "currency"]
},
{
"type": "object",
"properties": {
"amount": {
"type": "number"
},
"currency": {
"type": "string"
},
"cardDetails": {
"type": "object",
"properties": {
"cardNumber": {
"type": "string"
},
"expirationDate": {
"type": "string"
},
"cvv": {
"type": "string"
}
},
"required": ["cardNumber", "expirationDate", "cvv"]
}
},
"required": ["amount", "currency", "cardDetails"]
}
]
}
},
"required": ["payment"]
}
5. Save your schema.
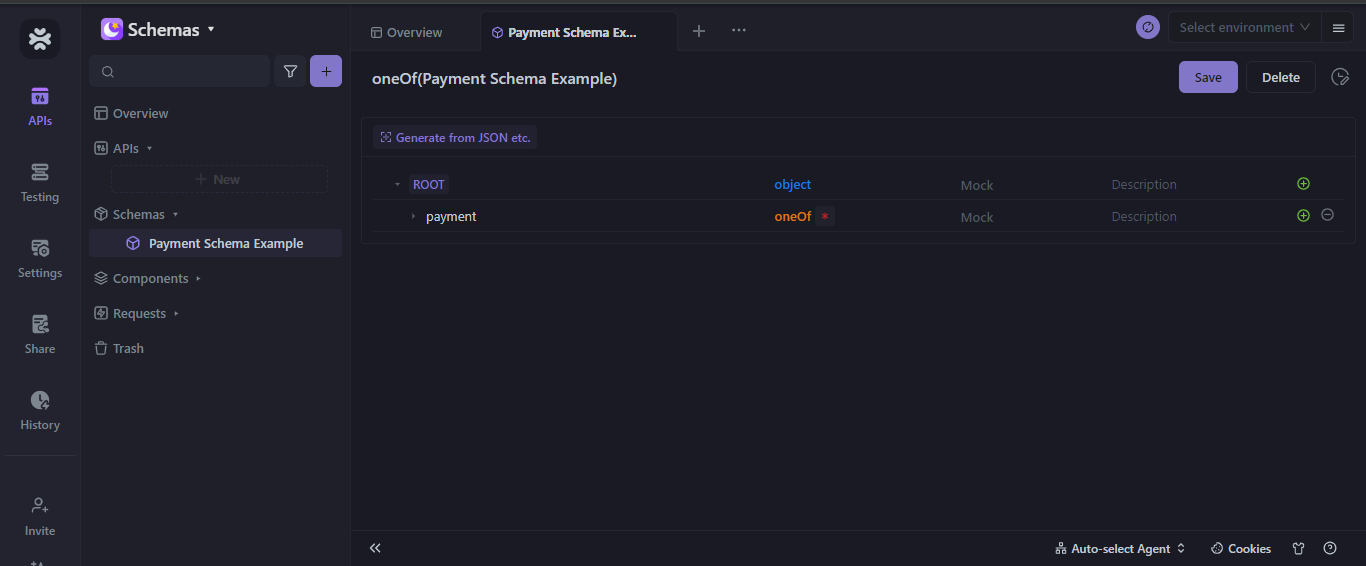
Your oneOf schema has been successfully created!
How to Create anyOf
Follow all the steps above for oneOf, but the only change you will make for your anyOf schema is to change the code. Input the following code in the editor to create your schema.
{
"type": "object",
"properties": {
"address": {
"anyOf": [
{
"type": "object",
"properties": {
"street": {
"type": "string"
},
"city": {
"type": "string"
},
"state": {
"type": "string"
},
"zip": {
"type": "string"
}
},
"required": ["street", "city", "state", "zip"]
},
{
"type": "object",
"properties": {
"country": {
"type": "string"
}
},
"required": ["country"]
}
]
}
},
"required": ["address"]
}
allOf
Follow all the steps above for oneOf, but the only change you will make for your allOf schema is to change the code. Input the following code in the editor to create your schema.
{
"type": "object",
"properties": {
"product": {
"allOf": [
{
"type": "object",
"properties": {
"name": {
"type": "string"
},
"description": {
"type": "string"
}
},
"required": ["name", "description"]
},
{
"type": "object",
"properties": {
"price": {
"type": "number"
},
"stock": {
"type": "integer"
}
},
"required": ["price", "stock"]
}
]
}
},
"required": ["product"]
}
Benefits of creating Schemas with Apidog
You can do several useful things now that you have created your JSON schemas with Apidog. Some of the benefits are mentioned below.
API Documentation
You can integrate your JSON Schema into your API documentation to provide clear and comprehensive descriptions of your API endpoints' data structures and validation rules. This improves developer understanding and reduces the risk of errors in API usage.
Code generation
You can utilize tools like Apidog's code generation capabilities to automatically generate client libraries and server-side code from your JSON Schema. This streamlines the development process and ensures consistency between your API definition and its implementation.
Data validation and error handling
You can employ JSON Schema validation libraries to validate incoming API requests and responses, ensuring the data conforms to the defined structure and constraints. This helps prevent invalid data from entering your system and reduces the risk of application errors.
API testing
You can leverage JSON Schema to generate test cases for your API endpoints and create mock servers for testing. This facilitates comprehensive API testing and ensures that your API functions as intended.
API design
You can continuously refine your JSON Schema as your API evolves to accommodate new data requirements and usage patterns. This ensures that your API remains flexible and adaptable to changing demands.
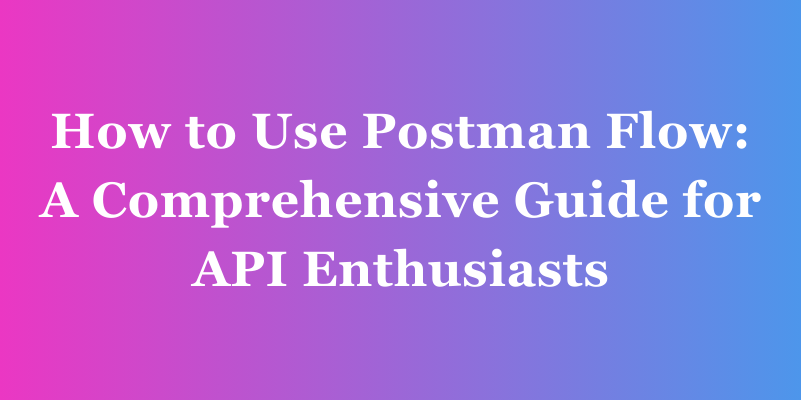
Conclusion
In conclusion, integrating JSON schemas with Apidog can greatly enhance the documentation process for APIs. JSON Schema provides a standardized way to describe the structure and constraints of JSON data, and when integrated with API documentation tools, it ensures that the documentation accurately reflects the expected request and response formats.
Apidog supports features that leverage JSON schemas. For instance, it might automatically generate documentation based on the defined schemas, making it easier to keep the documentation up-to-date as the API evolves.