Everything You Must Know About NodeJS Express PUT Request
Thinking about building web apps or APIs? NodeJS Express is a well-known framework that makes development easier and more consistent. This article will show you how to handle POST requests using NodeJS Express!
The NodeJS runtime environment has become a popular choice for building modern web applications due to its efficient event-driven architecture and JavaScript foundation. Within this ecosystem, Express stands out as a prominent web framework, offering a robust and streamlined approach to development.
Introducing Apidog, a sophisticated API tool that provides developers with a code generation tool for more efficient app and API development.
To learn more about what Apidog has to offer, click the button below.
One of the cornerstones of effective web APIs lies in the utilization of HTTP methods that dictate how resources are interacted with. The PUT method plays a crucial role in this context, enabling developers to perform targeted updates on existing resources.
Before diving deeper into making PUT requests with NodeJS Express, let us fully understand what NodeJS and Express are separate.
What is NodeJS?
NodeJS is an open-source software that breaks the mold by allowing JavaScript to run outside of web browsers. This flexibility extends across different operating systems, making it a versatile tool for developers. This document dives deep into what NodeJS offers, exploring its capabilities and how it has changed the way we approach software development.
NodeJS Key Features
Event-Driven Architecture
Unlike traditional web servers, NodeJS doesn't use threads. Instead, it relies on an event loop to handle multiple requests efficiently. This event-driven approach makes NodeJS ideal for applications that deal with a high volume of concurrent connections, like chat applications or real-time data streaming.
JavaScript Everywhere
NodeJS uses the V8 JavaScript engine, the same one that powers Google Chrome, to execute code. This allows developers to use JavaScript for both front-end and back-end development, reducing the need to learn and manage multiple languages.
Non-Blocking I/O
NodeJS utilizes a non-blocking I/O model. This means it doesn't wait for slow operations (like reading from a database) to finish before handling other requests. Instead, it moves on to the next request while the slow operation completes in the background. This keeps the server responsive and efficient.
Rich Ecosystem and NPM
NodeJS boasts a vast and active community that contributes to a massive package manager called npm (Node Package Manager). NPM offers a wide variety of pre-written modules for various functionalities, saving developers time and effort by providing reusable code components.
Scalability
NodeJS applications can be easily scaled horizontally. This means you can add more worker servers to handle increased traffic, making NodeJS suitable for building large and complex applications.
What is Express?
Express, AKA ExpressJS, is a popular web framework for NodeJS that empowers developers to build web applications and APIs with greater ease. It acts as a layer of abstraction, providing a more convenient and structured approach compared to working directly with NodeJS's underlying functionalities. This translates to a significant reduction in repetitive code (boilerplate code), allowing developers to focus on the core logic of their applications and accelerate the development process.

ExpressJS Key Features
Routing
ExpressJS excels at defining routes, which map URLs to specific functions that handle incoming requests. This organization makes your application code more readable and maintainable.
Middleware
A core strength of ExpressJS is its middleware functionality. Middleware acts like mini-applications that intercept requests and responses, allowing you to perform common tasks like logging, authentication, or static file serving before the request reaches its final destination.
Templating Engines
ExpressJS integrates seamlessly with various templating engines like EJS, Pug, or Handlebars. These engines allow you to create dynamic HTML pages by combining HTML with server-side-generated content.
Error Handling
ExpressJS provides a structured approach to error handling. You can define custom error handlers to catch and respond to errors gracefully, improving the overall user experience of your application.
Community and Ecosystem
ExpressJS benefits from a large and active developer community. This translates to a vast amount of readily available middleware, libraries, and resources, saving developers time and effort when building web applications.
How to Make NodeJS Express PUT Requests?
To ensure that you can run the PUT requests, you will have to first ensure that your system has NodeJS and NPM installed.
Prerequisites
First, create a project directory and initialize a NodeJS project within it using NPM:
mkdir nodejs-put-request
cd nodejs-put-request
npm init -y
Next, install the ExpressJS framework as a dependency for your project:
npm install express
Step 1 - Constructing a PUT Route
Within your project directory, create a file named server.js
. This file will contain the ExpressJS application logic. The code snippet below demonstrates a basic PUT route:
const express = require('express');
const app = express();
const port = 3000;
// In-memory data store (replace with a database for production)
let data = {
id: 1,
name: "John Doe",
age: 30
};
// PUT route for updating data based on ID
app.put('/api/data/:id', (req, res) => {
const id = req.params.id;
const updatedData = req.body;
// Validate data existence before update
if (data.id !== parseInt(id)) {
return res.status(404).send("Data not found");
}
// Update data properties using object spread syntax
data = { ...data, ...updatedData };
res.json(data);
});
app.listen(port, () => {
console.log(`Server listening on port ${port}`);
});
Code Explanation
- We import Express and create an Express application instance.
- A sample data object serves as a placeholder (a database would be used in production).
- The PUT route (
/api/data/:id
) captures an ID parameter from the URL. - Data existence is verified before attempting an update.
- If the data is found, the updated data from the request body is merged with the existing data object using object spread syntax (
...
). - Finally, the updated data is sent back in the response as JSON.
Step 2 - Sending a PUT Request (Using Apidog)
To simulate sending a PUT request, you can utilize a tool like Apidog.
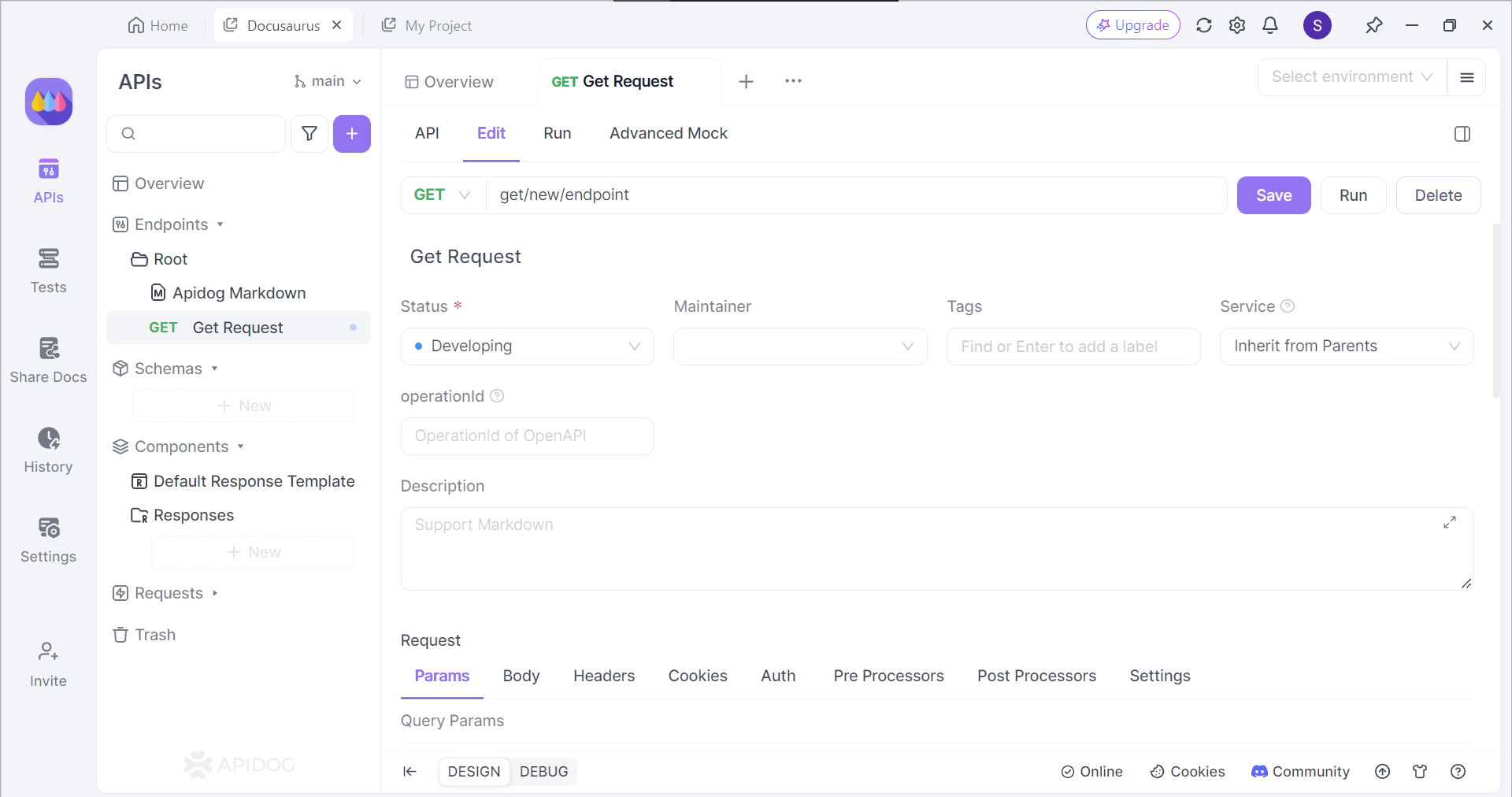
What you need to do is:
- Set the HTTP method to PUT.
- Define the URL as
http://localhost:3000/api/data/1
(replace 1 with the desired ID). - In the body tab, set the content-type to JSON and provide the data you want to update (e.g., {"name": "Jane Doe"}).
- Send the request.
The section below will highlight more on what Apidog has to offer.
Important Considerations and Best Practices
This example serves as a foundational illustration for making PUT requests with NodeJS Express. Remember to incorporate additional features into your real-world applications:
- Error Handling: Implement robust error handling mechanisms to gracefully manage potential issues during the update process.
- Validation: Validate incoming data to ensure it adheres to your defined schema and constraints.
- Database Integration: For production applications, integrate a database solution like MongoDB or PostgreSQL for persistent data storage.
- Middleware: Explore middleware functionalities within ExpressJS to handle tasks like authentication, authorization, and logging for a more secure and well-structured application.
Apidog - Making a PUT Request
As introduced before, you can make a PUT request in no time with Apidog, a comprehensive API development tool that has complete tools for the entire API lifecycle.
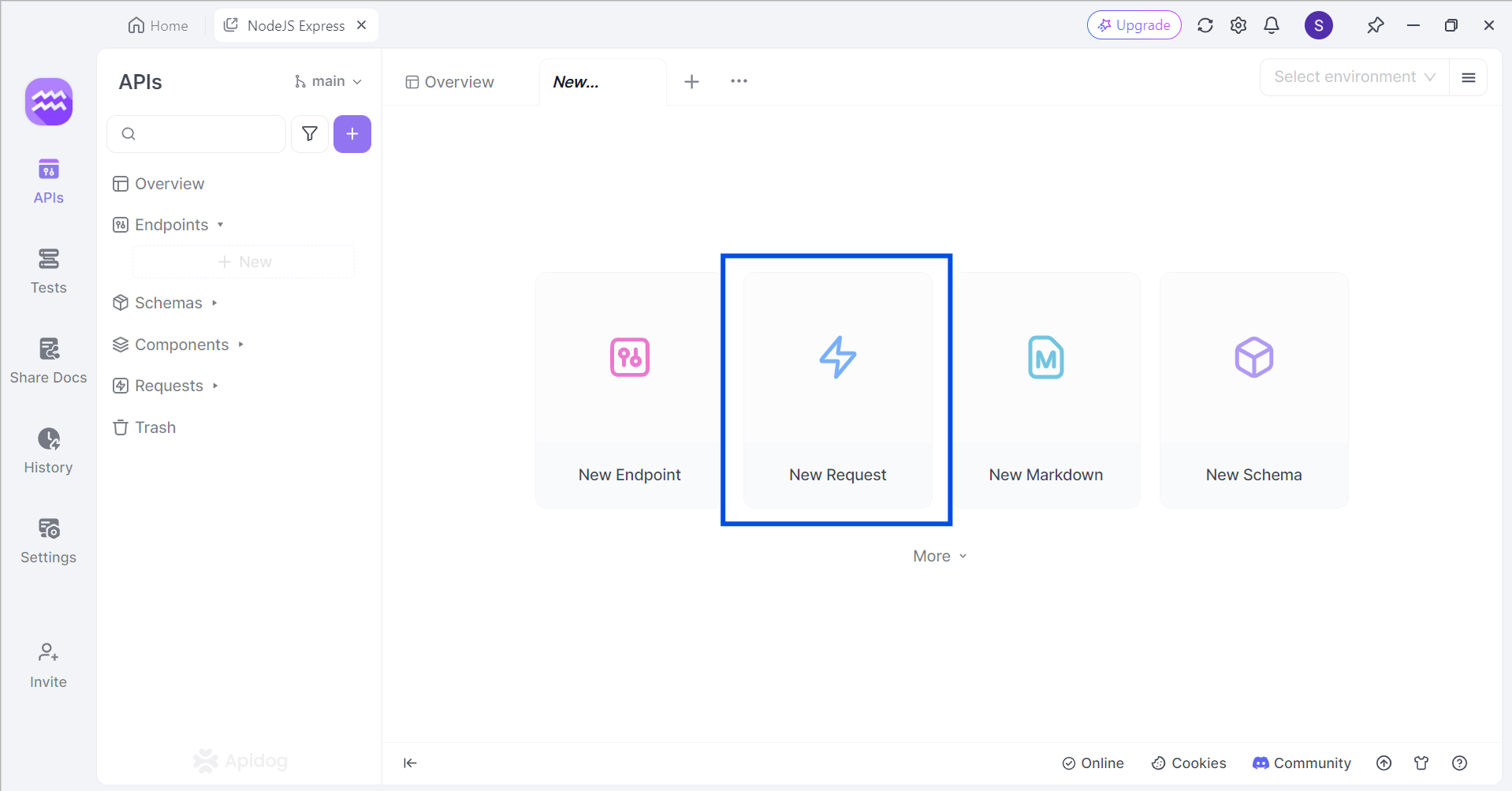
First, click the New Request
button to create a new request for the NodeJS Express PUT request.
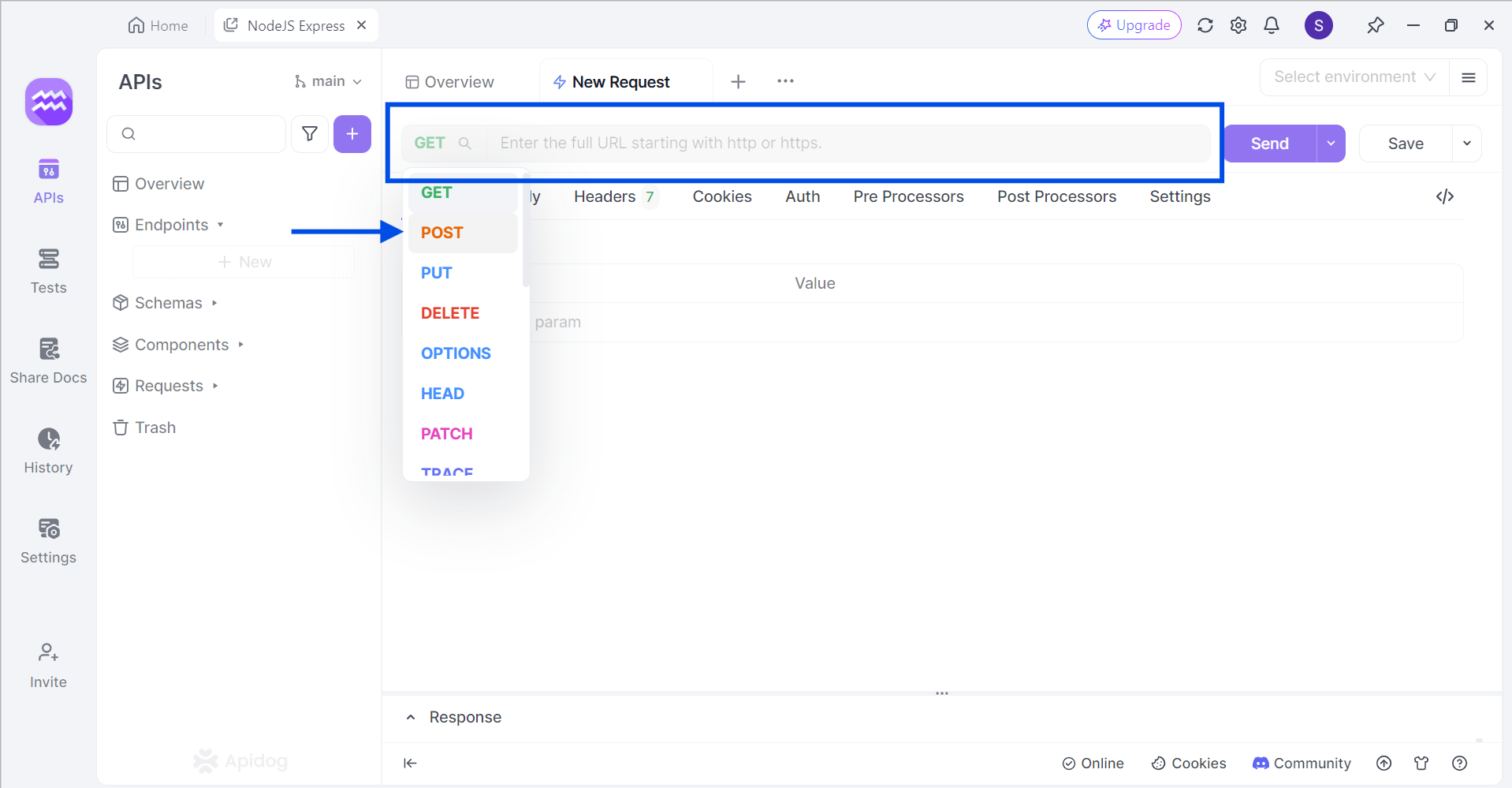
Next, make sure to change the request into a PUT request. On the highlighted box in the image, insert the API endpoint you wish to access.
Proceed by including the necessary details for your PUT request.
Generate JavaScript Code for Your App with Apidog
As NodeJS and Express are written using JavaScript programming language, you will need to have a grasp of the language. Luckily, Apidog has a code generation feature.
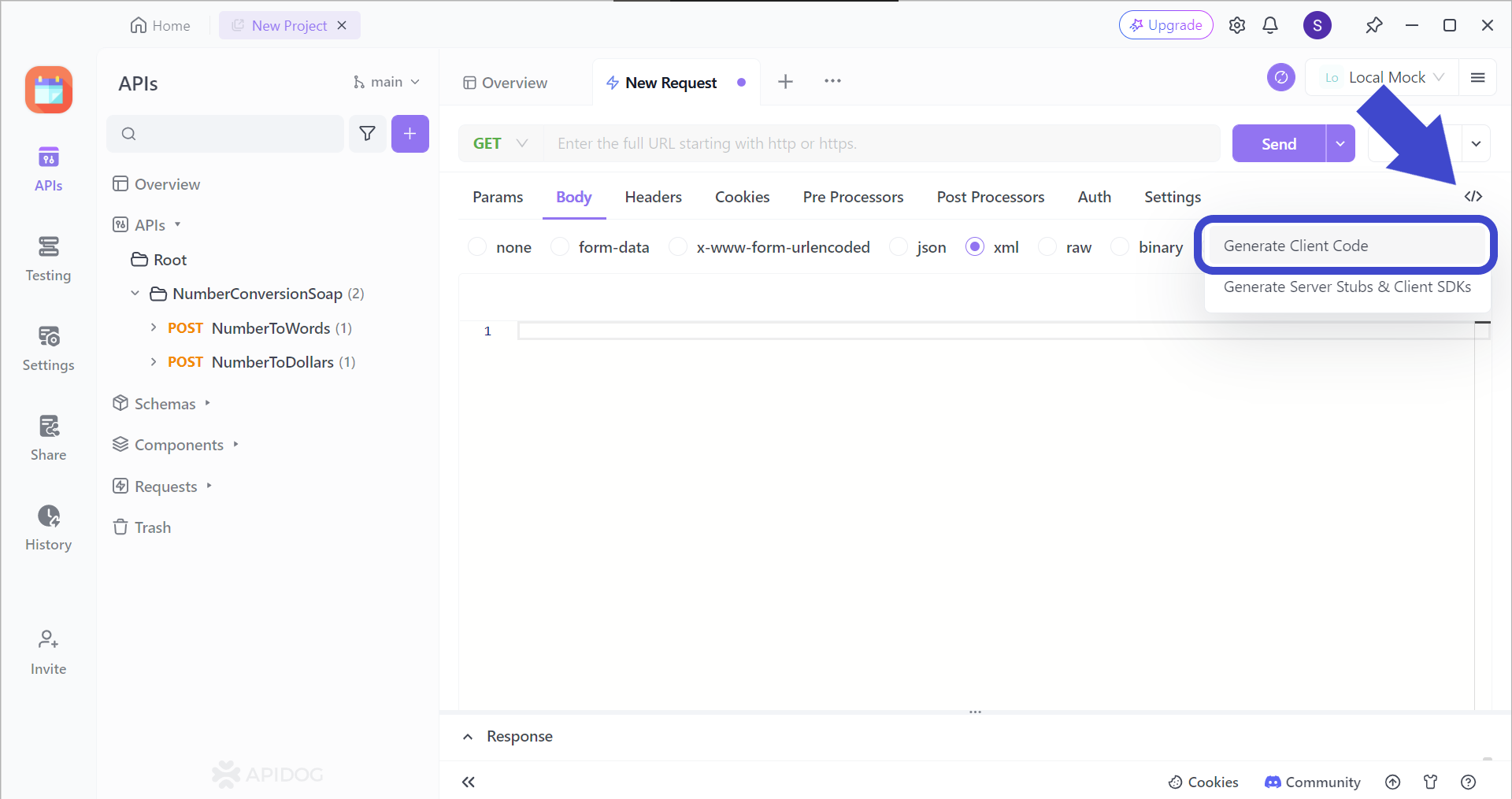
First, locate the </>
button found around the top right corner of the screen. If you are struggling to locate the button, you can refer to the image above.
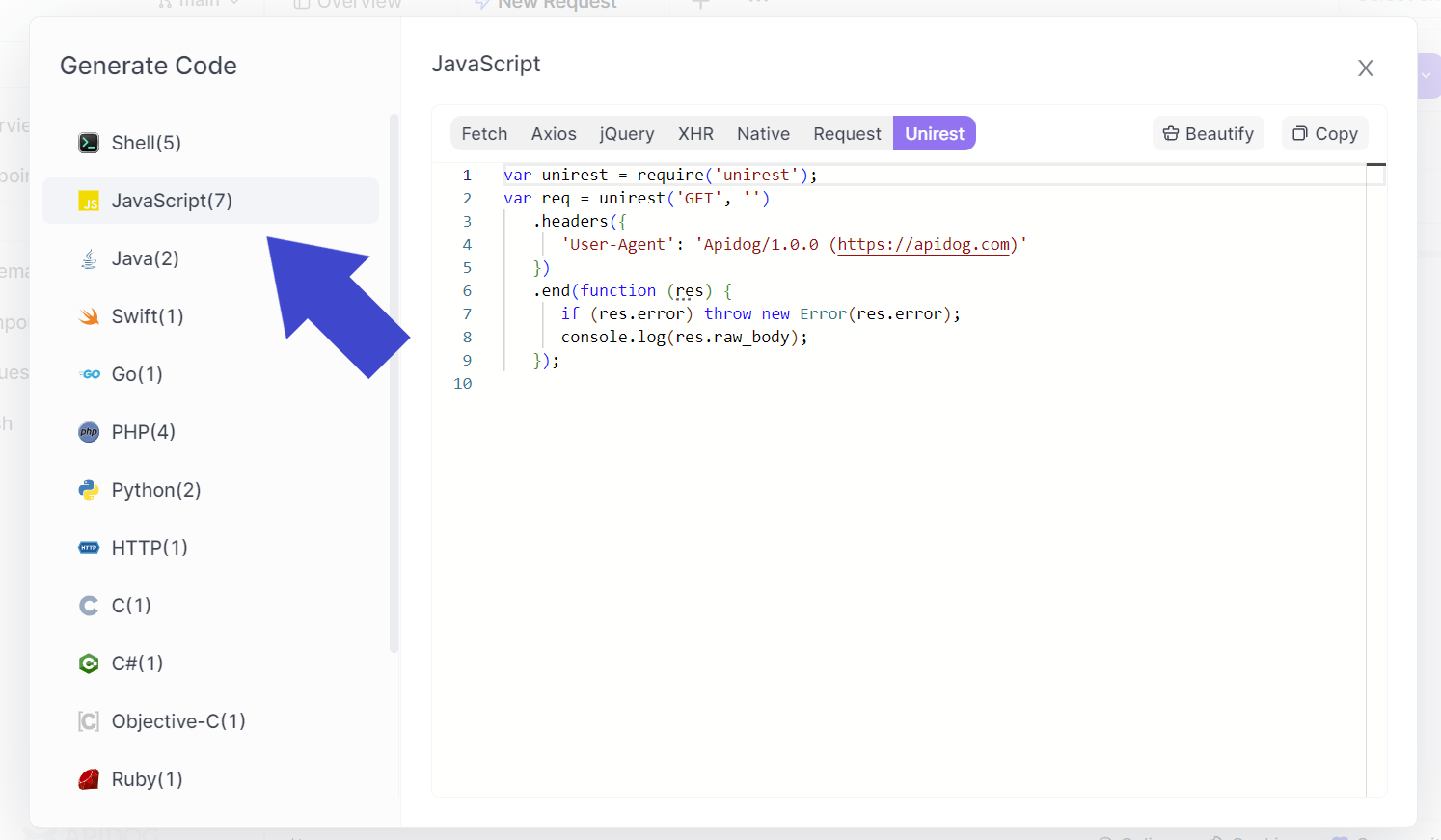
Proceed by selecting the client-side programming language that you need. You have the power to choose which JavaScript library you are more comfortable with.
All you need to do is copy and paste the code to your IDE, and continue with editing to ensure it fits your NodeJS app! However, if you're keen on using Unirest as shown in the image above, you can refer to the article here:
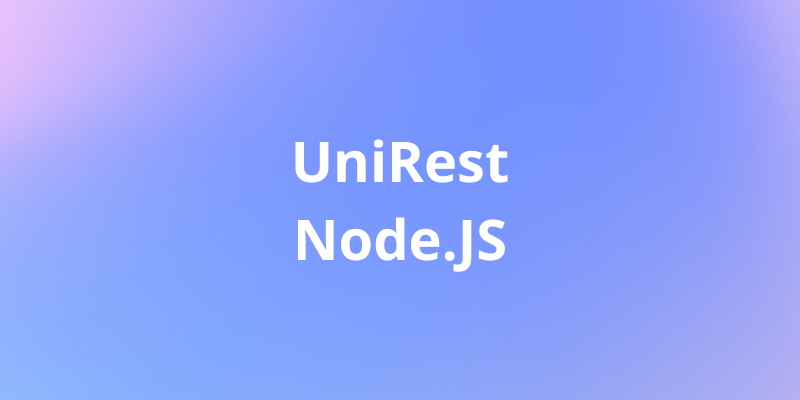
Conclusion
NodeJS Express empowers developers to construct robust and versatile APIs that seamlessly integrate PUT requests for targeted resource updates. This guide explored the core concepts involved in handling PUT requests within NodeJS Express applications. We walked through establishing a project, crafting a PUT route, and simulating a request using Postman.
Remember, this serves as a foundation. Real-world applications should incorporate best practices like error handling, data validation, database integration, and middleware for a comprehensive and secure development approach. By mastering PUT requests within NodeJS Express, you can effectively manage and update resources within your web applications and APIs.