Stripe is a powerful platform that provides a suite of APIs for handling online payments and financial transactions. With its user-friendly interface and robust features, Stripe has become a popular choice for developers looking to integrate payment processing into their applications. Additionally, tools like Apidog can help streamline the testing and integration process, making it easier to work with the Stripe API effectively. This article will explore the Stripe API in detail, covering its features, integration process, best practices, and more.
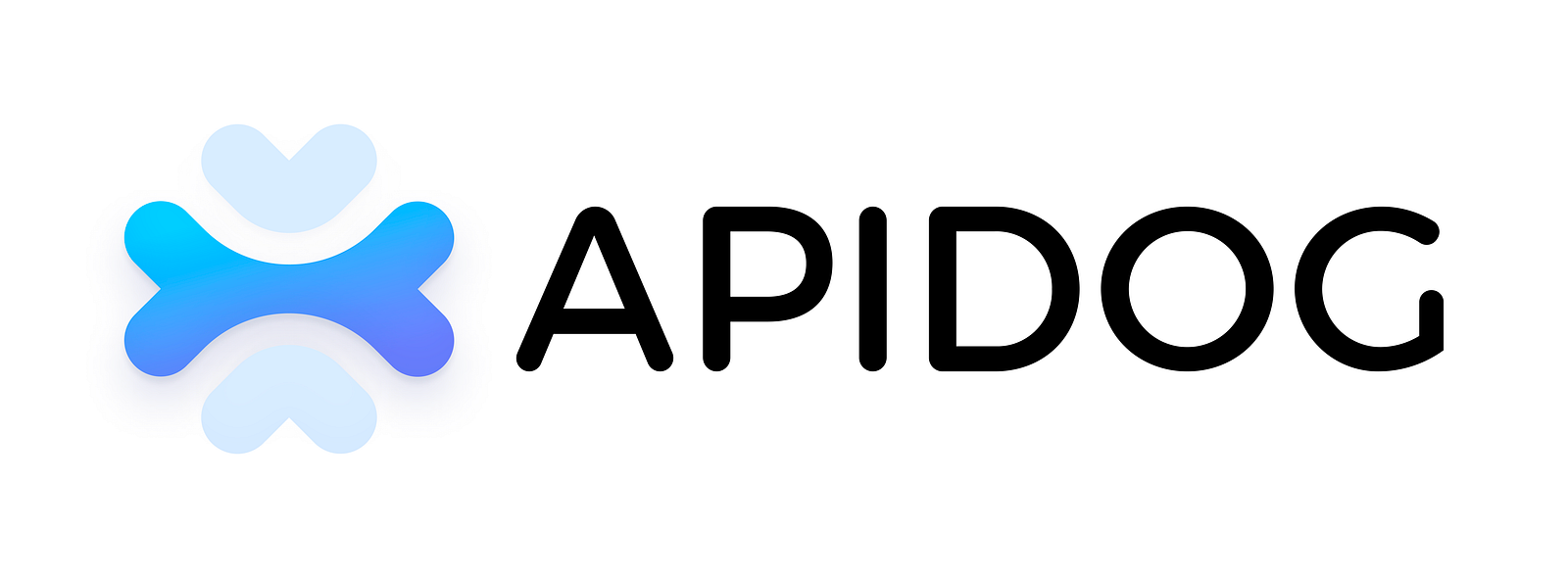
What is the Stripe API?
The Stripe API is a set of programming interfaces that allow developers to integrate payment processing capabilities into their applications. It enables businesses to accept payments, manage subscriptions, send invoices, and handle various financial transactions seamlessly.
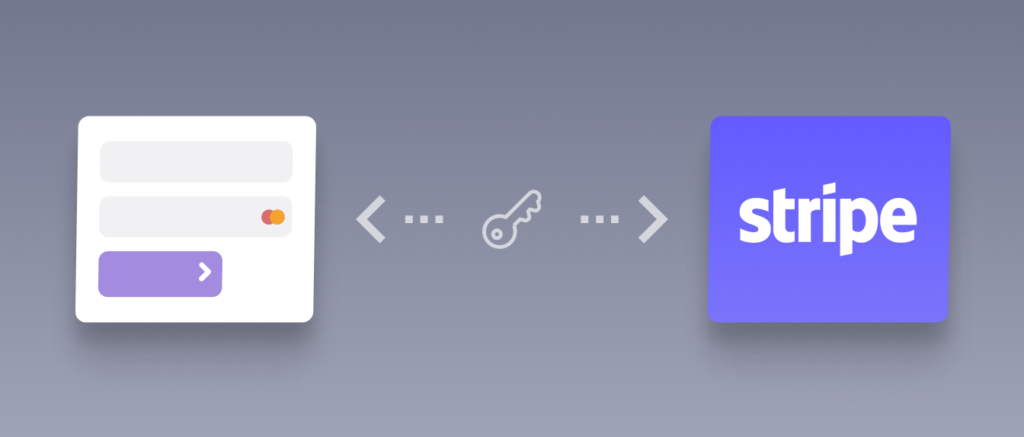
Key Features of the Stripe API
- Payment Processing: Accept payments from various sources, including credit cards, debit cards, and digital wallets.
- Subscription Management: Create and manage subscription plans for recurring billing.
- Invoicing: Generate and send invoices to customers with automated reminders.
- Fraud Prevention: Use machine learning algorithms to detect and prevent fraudulent transactions.
- Reporting and Analytics: Access detailed reports on transactions, revenue, and customer behavior.
Getting Started with the Stripe API
To begin using the Stripe API, you need to create an account on the Stripe platform. Once you have an account, you can access your API keys from the dashboard. These keys are essential for authenticating your requests to the Stripe servers.
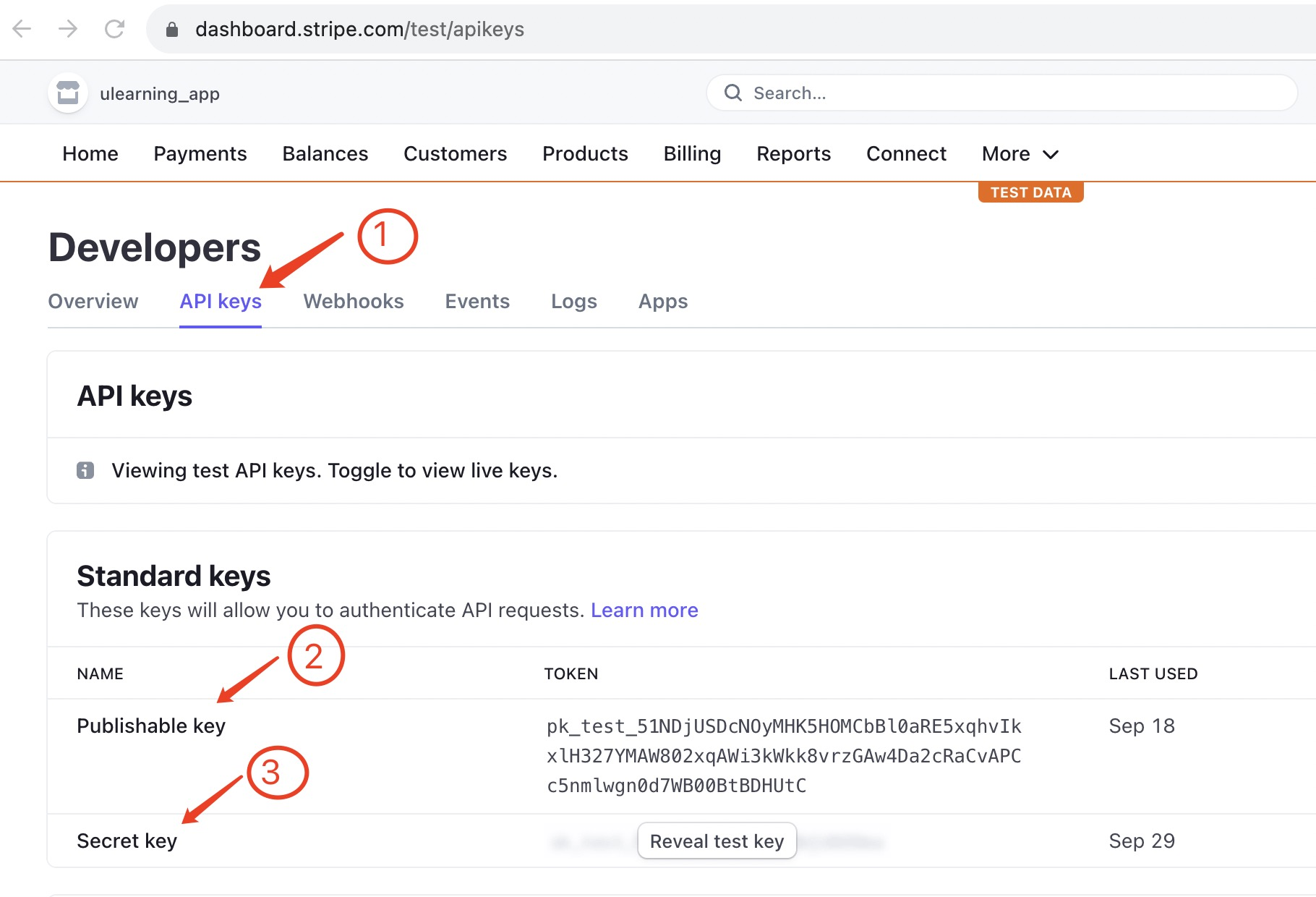
Setting Up Your Environment
- Create a Stripe Account: Sign up at Stripe.
- Obtain API Keys: Navigate to the Developers section in your dashboard to find your publishable key and secret key.
- Install SDKs: Depending on your programming language (e.g., Node.js, Python), install the relevant Stripe SDK using package managers like npm or pip.
Example of Installing Stripe SDK
For a Node.js application, you can install the Stripe SDK using npm:
npm install stripe
Integrating the Stripe API into Your Application
Once you have set up your environment, you can start integrating the Stripe API into your application. Below are some common use cases for integrating with Stripe.
Accepting Payments
To accept payments via credit or debit cards, you need to create a payment intent. The payment intent represents a payment that you plan to process.
Step-by-Step Process
Create a Payment Intent:
Use the following code snippet to create a payment intent in your server-side code:
const stripe = require('stripe')('your_secret_key');
const paymentIntent = await stripe.paymentIntents.create({
amount: 2000, // Amount in cents
currency: 'usd',
payment_method_types: ['card'],
});
Collect Payment Details:
Use Stripe Elements or Checkout to securely collect card details from your customers.
Confirm Payment:
Once you have collected the payment details, confirm the payment intent:
const confirmedPayment = await stripe.paymentIntents.confirm(paymentIntent.id);
Managing Subscriptions
Stripe makes it easy to manage subscriptions for recurring billing. You can create subscription plans and assign customers to these plans.
Creating a Subscription Plan
Define Your Product:
First, create a product in your Stripe dashboard or via the API:
const product = await stripe.products.create({
name: 'Gold Special',
description: 'Monthly subscription for Gold members',
});
Create a Price for Your Product:
Create a recurring price for this product:
const price = await stripe.prices.create({
unit_amount: 1500,
currency: 'usd',
recurring: { interval: 'month' },
product: product.id,
});
Subscribe a Customer:
Finally, subscribe a customer to this plan:
const subscription = await stripe.subscriptions.create({
customer: 'customer_id',
items: [{ price: price.id }],
});
Handling Invoices
Stripe allows you to generate invoices for one-time payments or recurring subscriptions.
Creating an Invoice
Create an Invoice Item:
Add an item to the invoice:
await stripe.invoiceItems.create({
customer: 'customer_id',
amount: 2500,
currency: 'usd',
description: 'One-time setup fee',
});
Create and Finalize the Invoice:
Create the invoice and finalize it for sending:
const invoice = await stripe.invoices.create({
customer: 'customer_id',
auto_advance: true,
});
Using Webhooks with Stripe
Webhooks are essential for receiving real-time notifications about events that occur in your Stripe account (e.g., successful payments, subscription updates).
Setting Up Webhooks
Create an Endpoint:
Set up an endpoint in your application to listen for webhook events:
app.post('/webhook', express.json(), (req, res) => {
const event = req.body;
switch (event.type) {
case 'payment_intent.succeeded':
console.log('PaymentIntent was successful!');
break;
case 'invoice.paid':
console.log('Invoice was paid!');
break;
// Handle other events
default:
console.log(`Unhandled event type ${event.type}`);
}
res.status(200).send('Received');
});
Register Your Webhook URL:
In your Stripe dashboard under Developers -> Webhooks, register your endpoint URL.
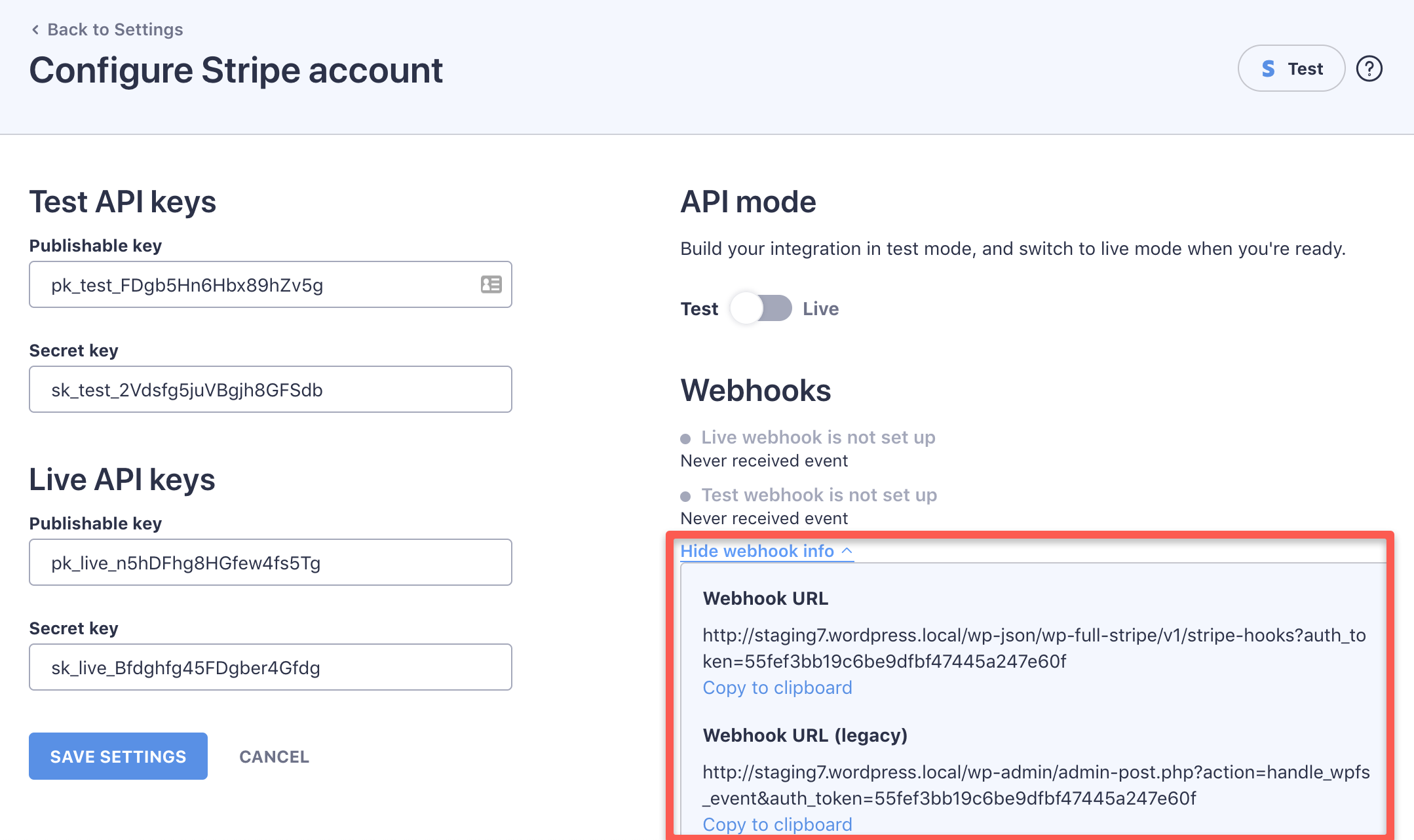
Best Practices for Using the Stripe API
When integrating with the Stripe API, consider these best practices:
- Use Test Mode: Always test your integration in test mode before going live.
- Secure Your Keys: Keep your secret keys confidential and never expose them on client-side code.
- Handle Errors Gracefully: Implement error handling for failed requests or unexpected responses.
- Monitor Webhook Events: Regularly check logs for webhook events to ensure they are being processed correctly.
- Stay Updated: Keep track of updates and changes in the Stripe API documentation.
Advanced Features of the Stripe API
Beyond basic payment processing and subscription management, Stripe offers several advanced features that can enhance your application's capabilities.
3D Secure Authentication
Stripe supports 3D Secure authentication for card payments, adding an extra layer of security during transactions. This feature helps reduce fraud by requiring customers to complete additional verification steps during checkout.
Custom Payment Flows
With Stripe's extensive customization options, you can create tailored payment flows that suit your business needs. This includes setting up custom fields on payment forms or integrating with third-party services.
Multi-Currency Support
Stripe allows businesses to accept payments in multiple currencies, making it ideal for international transactions. You can easily configure currency settings within your account dashboard.
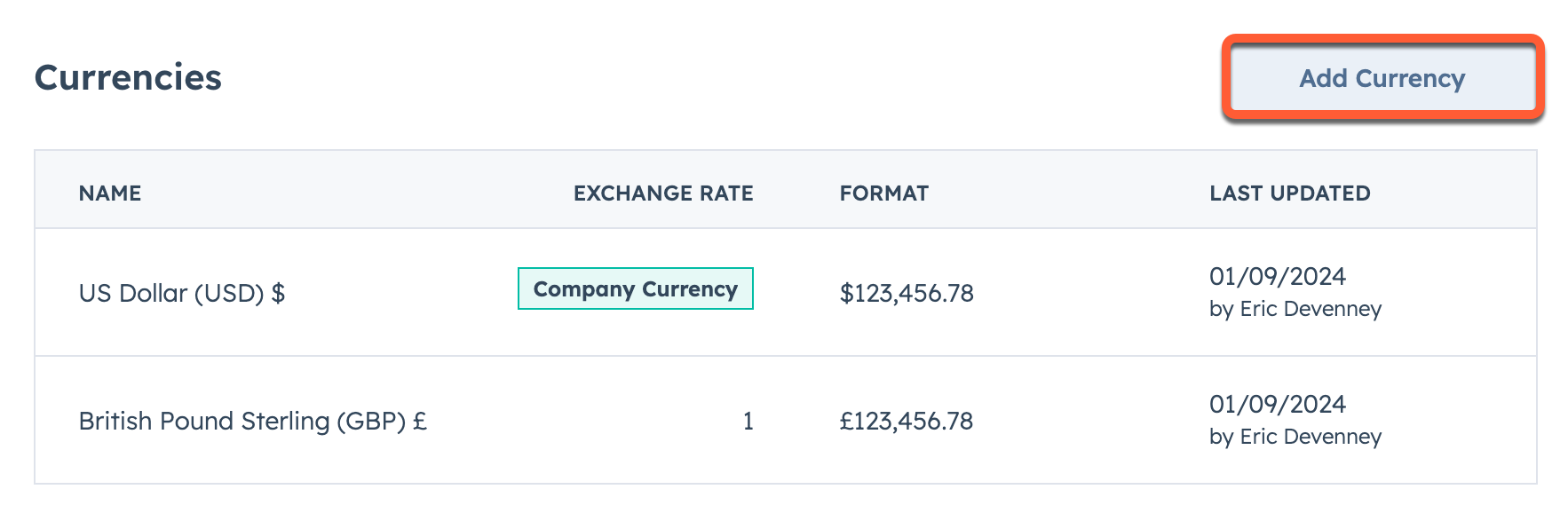
Reporting and Analytics Tools
Stripe provides robust reporting tools that allow businesses to gain insights into their transaction history, revenue trends, and customer behavior. These analytics can help inform business decisions and optimize revenue strategies.
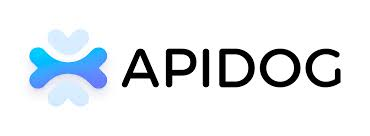
Integrating Apidog with Stripe API Testing
When developing applications that use the Stripe API, ensuring robust testing is crucial. The Stripe API handles sensitive financial transactions, making thorough testing essential to avoid issues that could lead to lost revenue or customer dissatisfaction. Apidog is an excellent tool for testing APIs efficiently, providing a range of features that enhance the testing process for developers working with Stripe.
Benefits of Using Apidog for Testing Stripe APIs
User-Friendly Interface
One of the standout features of Apidog is its user-friendly interface. The platform is designed with simplicity in mind, allowing developers to navigate through various functionalities without a steep learning curve. This intuitive design is particularly beneficial for teams that may not have extensive experience in API testing.
For example, Apidog's dashboard provides clear visual cues and organized sections for managing endpoints, request parameters, and test cases. This design helps developers quickly set up tests for different Stripe API endpoints, such as payment intents or subscriptions.
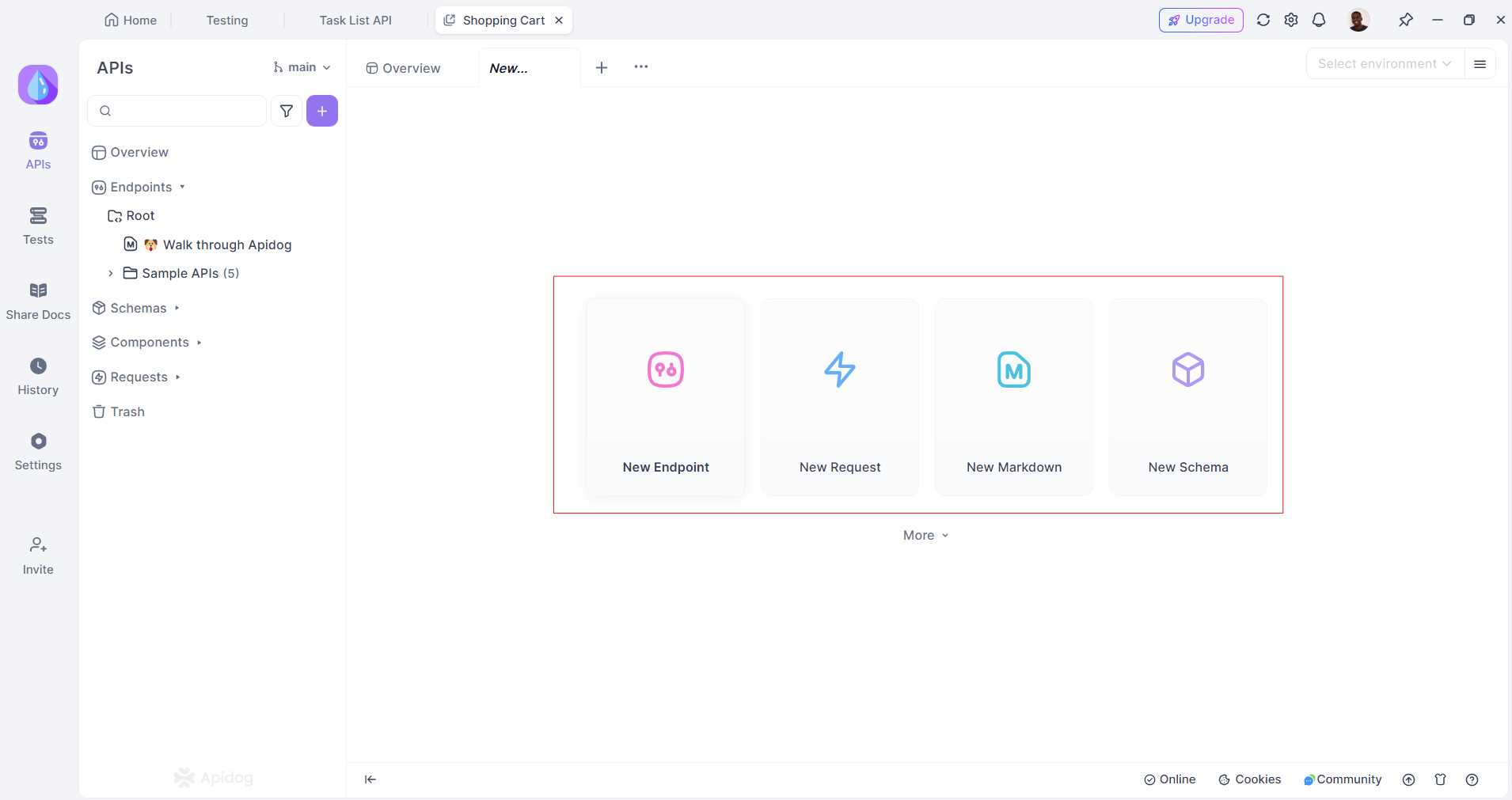
As highlighted in the article on API GUI Tools, Apidog simplifies the API testing process by enabling users to create mock APIs and manage test cases efficiently. This ease of use ensures that developers can focus on writing effective tests rather than getting bogged down by complex interfaces.
Automated Testing
Automated testing is another significant advantage of using Apidog for Stripe API integration. With Apidog, developers can easily automate tests for various endpoints of the Stripe API, which is vital for maintaining consistent quality across releases.
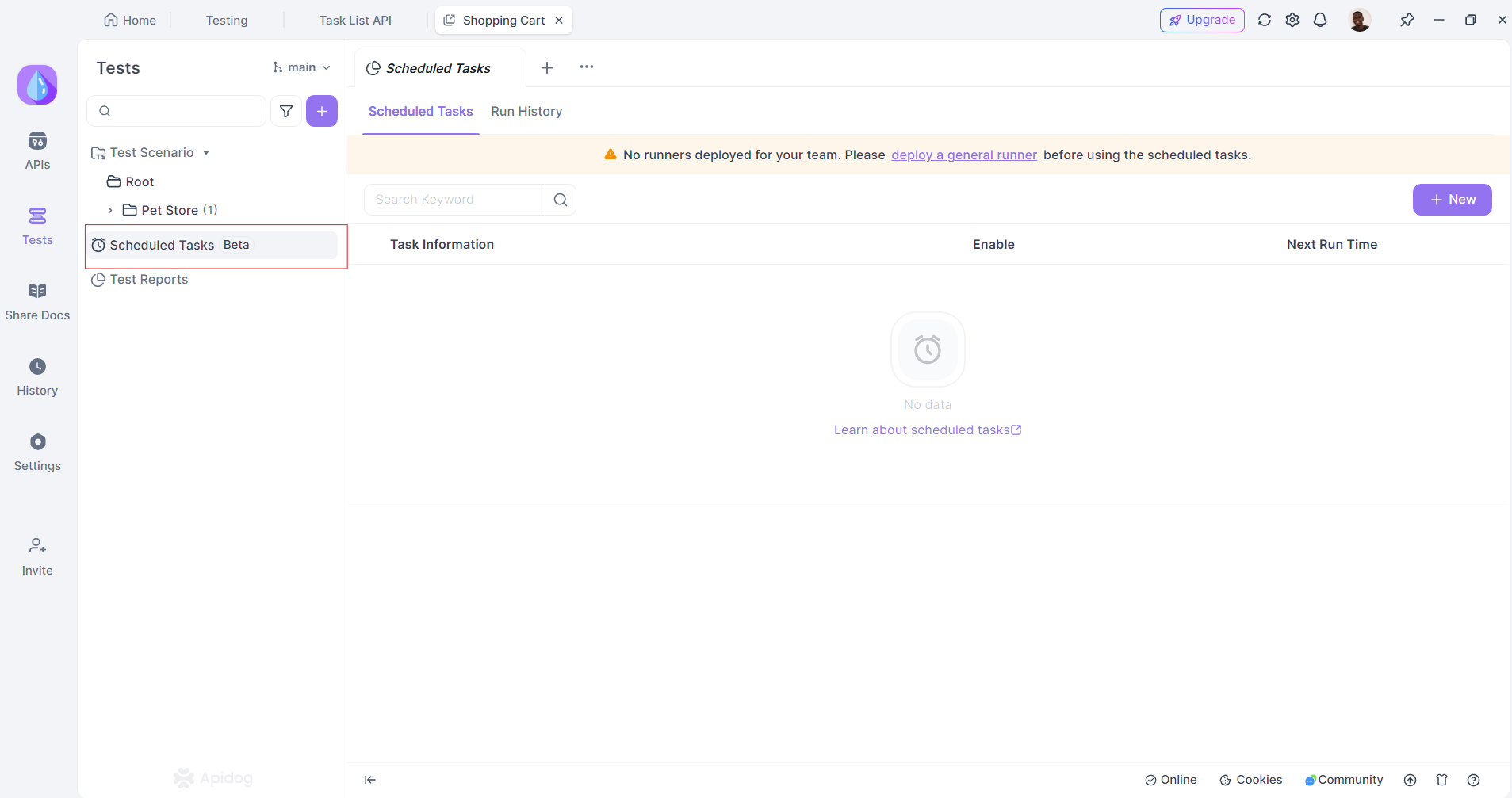
Automated tests can be set up to run at scheduled intervals or triggered by specific events within the development cycle. This capability allows teams to catch issues early and ensure that new code changes do not break existing functionality.
The article on How to Automate Your API Testing emphasizes how Apidog facilitates automation through its straightforward setup process. By defining test cases once and running them automatically, teams can save time and reduce manual effort while increasing test coverage.
Collaboration Features
Collaboration is key in software development, especially when multiple team members are involved in building and testing applications. Apidog offers robust collaboration features that enable users to share test cases and results seamlessly.
With Apidog, team members can easily access shared projects, review test cases, and contribute to the testing process without confusion. This feature is particularly useful when working on complex integrations like those with the Stripe API, where multiple endpoints and scenarios need to be tested collaboratively.
The importance of collaboration tools in API testing is highlighted in the article on How to Build CI/CD Pipeline. By integrating Apidog into your CI/CD workflow, teams can ensure that everyone stays aligned on testing objectives and results.
Integration with CI/CD Pipelines
Incorporating testing into continuous integration (CI) and continuous deployment (CD) workflows is essential for modern software development practices. Apidog supports this integration by allowing developers to automate their API tests as part of their deployment pipeline.
When using Apidog with Stripe, you can set up automated tests that run every time new code is pushed to your repository. This ensures that any changes made do not negatively impact existing functionality or introduce new bugs.
The article on API Testing Automation discusses how automating your testing processes can lead to more reliable deployments and faster release cycles. By integrating Apidog into your CI/CD pipeline, you can maintain high-quality standards while accelerating your development process.
Example of Testing a Payment Intent with Apidog
To illustrate how you can use Apidog to test creating a payment intent with the Stripe API, follow these steps:
Define Your Endpoint: Start by defining the endpoint for creating a payment intent in Apidog. You would typically use the following URL:
POST https://api.stripe.com/v1/payment_intents
Set Up Request Parameters: In the request body, specify parameters such as amount (in cents) and currency:
{
"amount": 2000,
"currency": "usd",
"payment_method_types": ["card"]
}
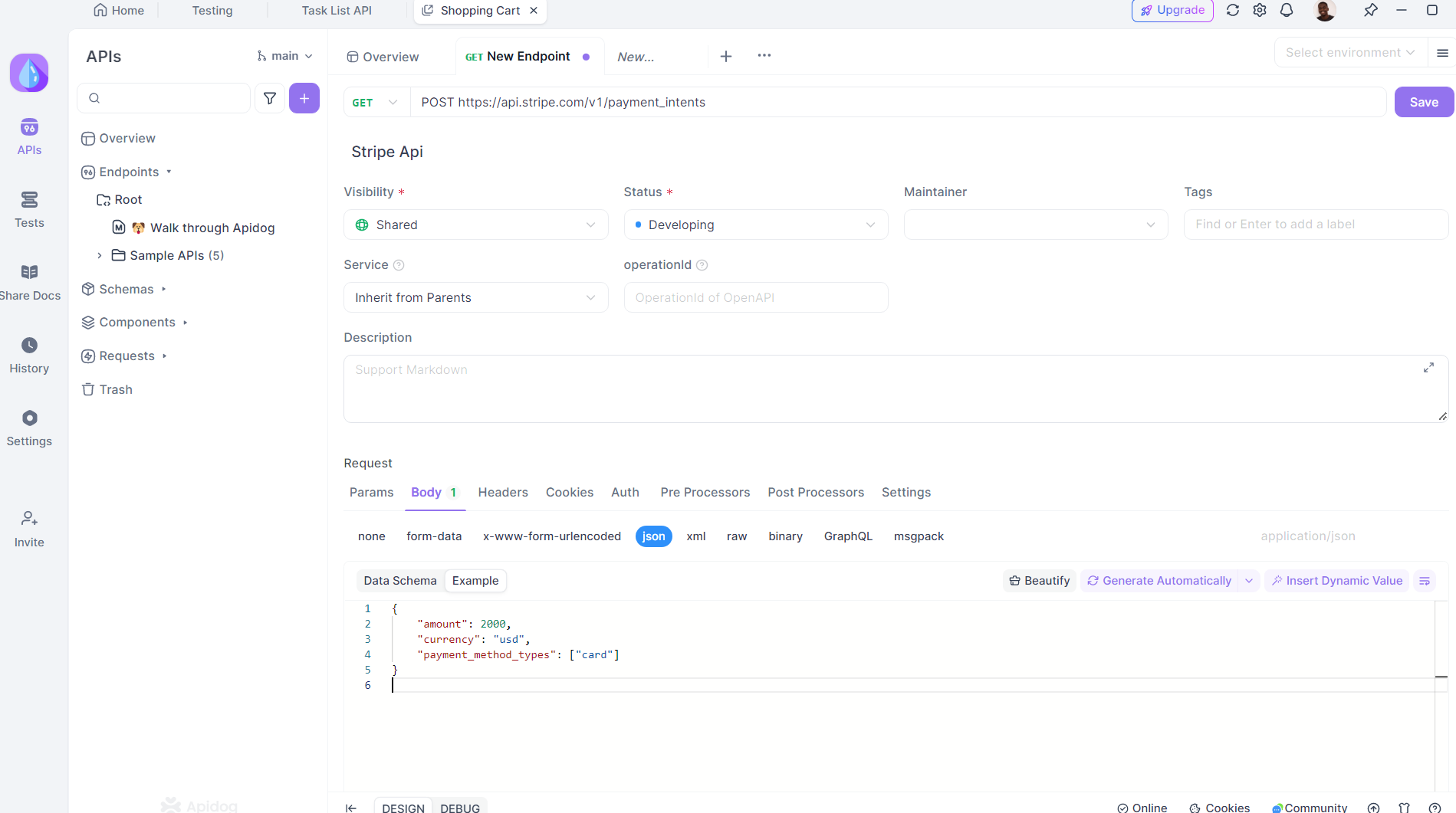
Execute the Test: Once you've defined your endpoint and set up your parameters, execute the test directly within Apidog's interface. The platform will send the request to Stripe's servers and return a response.
Analyze Responses: After executing the test, you can analyze the response provided by Stripe within Apidog’s interface. This includes checking for successful payment intents or handling errors if they occur.
Using Apidog not only streamlines testing but also helps catch issues early in development by allowing developers to validate their integrations against expected behaviors. By leveraging its intuitive interface and automation capabilities, teams can ensure their implementations of the Stripe API are robust and reliable.
Common Challenges When Using the Stripe API
While integrating with the Stripe API offers many benefits, developers may encounter several challenges along the way:
Handling Errors Effectively
Errors can occur during any interaction with an external service like Stripe. It's crucial to implement robust error-handling mechanisms within your application:
- Network Issues: Handle network timeouts gracefully by retrying requests when appropriate.
- API Rate Limits: Be aware of rate limits imposed by Stripe; implement exponential backoff strategies when encountering rate limit errors.
- Invalid Requests: Validate user inputs before sending requests to avoid unnecessary errors caused by invalid data formats or missing fields.
Keeping Up with Changes in Documentation
The world of APIs is constantly evolving; therefore:
- Regularly review Stripe's official documentation for updates on new features or changes in existing functionality.
- Subscribe to update notifications from Stripe or follow their blog for announcements regarding breaking changes or deprecations.
Ensuring Security Compliance
When dealing with sensitive information such as credit card data:
- Utilize HTTPS endpoints when communicating with both your server and Stripe’s servers.
- Follow PCI compliance guidelines provided by Stripe when handling card information directly on your website or application.
Real-world Use Cases of Using the Stripe API
Understanding how other businesses utilize the capabilities of the Stripe API can provide insights into best practices and innovative implementations:
E-Commerce Platforms
Many e-commerce platforms leverage Stripe's capabilities to facilitate seamless checkout experiences:
- Customizable checkout forms allow businesses to match their branding while securely processing payments.
- Subscription models enable businesses like subscription box services or SaaS companies to charge customers on a recurring basis effortlessly.
Mobile Applications
Mobile apps often integrate with payment gateways like Stripe for in-app purchases or services:
- Using mobile SDKs provided by Stripe allows developers to securely collect payment information directly within their apps while maintaining PCI compliance.
Non-Profit Organizations
Non-profits utilize donation forms powered by Stripe's APIs:
- They can easily set up one-time donations as well as recurring donations through subscription models while providing donors with receipts automatically generated by invoices created through the API.
Conclusion
The Stripe API is a powerful tool that empowers developers to integrate seamless payment processing into their applications. With its extensive features like subscription management, invoicing capabilities, and robust security measures like 3D Secure authentication, it caters effectively to various business needs.
By following best practices during integration and utilizing tools like Apidog for testing, developers can ensure their applications are reliable and user-friendly. Whether you're building an e-commerce platform or managing subscriptions for SaaS products, leveraging the capabilities of the Stripe API will enhance user experience while simplifying financial operations.
By leveraging these resources alongside this guide on the Stripe API, you'll be well-equipped to build effective payment solutions tailored to your business needs.