Ever found yourself digging through a massive JSON response, trying to find that one piece of data you need? If you have, then you know it can be a bit like searching for a needle in a haystack. Enter JSONPath - a nifty little tool that can make your life so much easier when dealing with JSON data in APIs.
What is JSONPath?
JSONPath is a query language for JSON, similar to XPath for XML. It allows you to navigate through and extract specific parts of your JSON data using a simple, intuitive syntax. Think of it as a way to run queries on your JSON data and get exactly what you need without wading through the entire response.
Why Use JSONPath?
Imagine you have a huge JSON response from an API; all you need is the value of a specific field nested deep inside. Without JSONPath, you'd typically have to write a bunch of code to parse through the JSON, which can be tedious and error-prone. JSONPath simplifies this by letting you specify exactly what you want to extract, making your code cleaner and easier to maintain.
How Does JSONPath Work?
JSONPath uses a syntax similar to JavaScript object notation, with some added features for more complex queries. Here's a quick rundown of the basic syntax:
$
: Represents the root object or array..
or[]
: Child operator; used to access a property of the object or an element of the array.*
: Wildcard; matches all elements or fields...
: Recursive descent; searches for matching nodes at any level.?()
: Applies a filter (expression must evaluate to true).()
: Script expression, using the underlying script engine.
Let’s dive into some examples to see JSONPath in action.
Basic JSONPath Examples
Consider the following JSON data from a movie database API:
{
"movies": {
"action": [
{ "title": "Mad Max: Fury Road", "director": "George Miller", "year": 2015, "rating": 8.1 },
{ "title": "John Wick", "director": "Chad Stahelski", "year": 2014, "rating": 7.4 }
],
"drama": [
{ "title": "The Shawshank Redemption", "director": "Frank Darabont", "year": 1994, "rating": 9.3 },
{ "title": "Forrest Gump", "director": "Robert Zemeckis", "year": 1994, "rating": 8.8 }
],
"comedy": [
{ "title": "The Grand Budapest Hotel", "director": "Wes Anderson", "year": 2014, "rating": 8.1 }
]
}
}
-
Extracting all action movies:
$.movies.action[*]
This will give you an array of all the action movie objects.
-
Extracting the titles of all drama movies:
$.movies.drama[*].title
This will return an array of the titles of all the drama movies:
["The Shawshank Redemption", "Forrest Gump"]
. -
Extracting the rating of "Mad Max: Fury Road":
$.movies.action[?(@.title == "Mad Max: Fury Road")].rating
This will return the rating of "Mad Max: Fury Road":
8.1
. -
Finding all movies released in 2014:
$.movies..[?(@.year == 2014)]
This will return all movies released in 2014, which in this case are:
["John Wick", "The Grand Budapest Hotel"]
.
JSONPath in APIs
Using JSONPath in APIs is incredibly useful when you want to extract specific information from a JSON response. Many programming languages and tools have libraries that support JSONPath, making it easy to integrate into your projects.
For instance, in Javascript, you can use the jsonpath
library:
const jsonpath = require('jsonpath');
// Sample JSON data
const data = {
movies: {
action: [
{ title: "Mad Max: Fury Road", director: "George Miller", year: 2015, rating: 8.1 },
{ title: "John Wick", director: "Chad Stahelski", year: 2014, rating: 7.4 }
],
drama: [
{ title: "The Shawshank Redemption", director: "Frank Darabont", year: 1994, rating: 9.3 },
{ title: "Forrest Gump", director: "Robert Zemeckis", year: 1994, rating: 8.8 }
],
comedy: [
{ title: "The Grand Budapest Hotel", director: "Wes Anderson", year: 2014, rating: 8.1 }
]
}
};
// Extracting titles of all drama movies
const titles = jsonpath.query(data, '$.movies.drama[*].title');
console.log(titles); // Output: ["The Shawshank Redemption", "Forrest Gump"]
Similarly, in Python, you can use the jsonpath-ng
library:
from jsonpath_ng import jsonpath, parse
# Sample JSON data
data = {
"movies": {
"action": [
{"title": "Mad Max: Fury Road", "director": "George Miller", "year": 2015, "rating": 8.1},
{"title": "John Wick", "director": "Chad Stahelski", "year": 2014, "rating": 7.4}
],
"drama": [
{"title": "The Shawshank Redemption", "director": "Frank Darabont", "year": 1994, "rating": 9.3},
{"title": "Forrest Gump", "director": "Robert Zemeckis", "year": 1994, "rating": 8.8}
],
"comedy": [
{"title": "The Grand Budapest Hotel", "director": "Wes Anderson", "year": 2014, "rating": 8.1}
]
}
}
# Extracting titles of all drama movies
jsonpath_expr = parse('$.movies.drama[*].title')
titles = [match.value for match in jsonpath_expr.find(data)]
print(titles) # Output: ["The Shawshank Redemption", "Forrest Gump"]
Using JSONPath In Apidog
Apidog is a versatile tool designed to simplify the process of working with APIs, and it supports JSONPath out of the box.
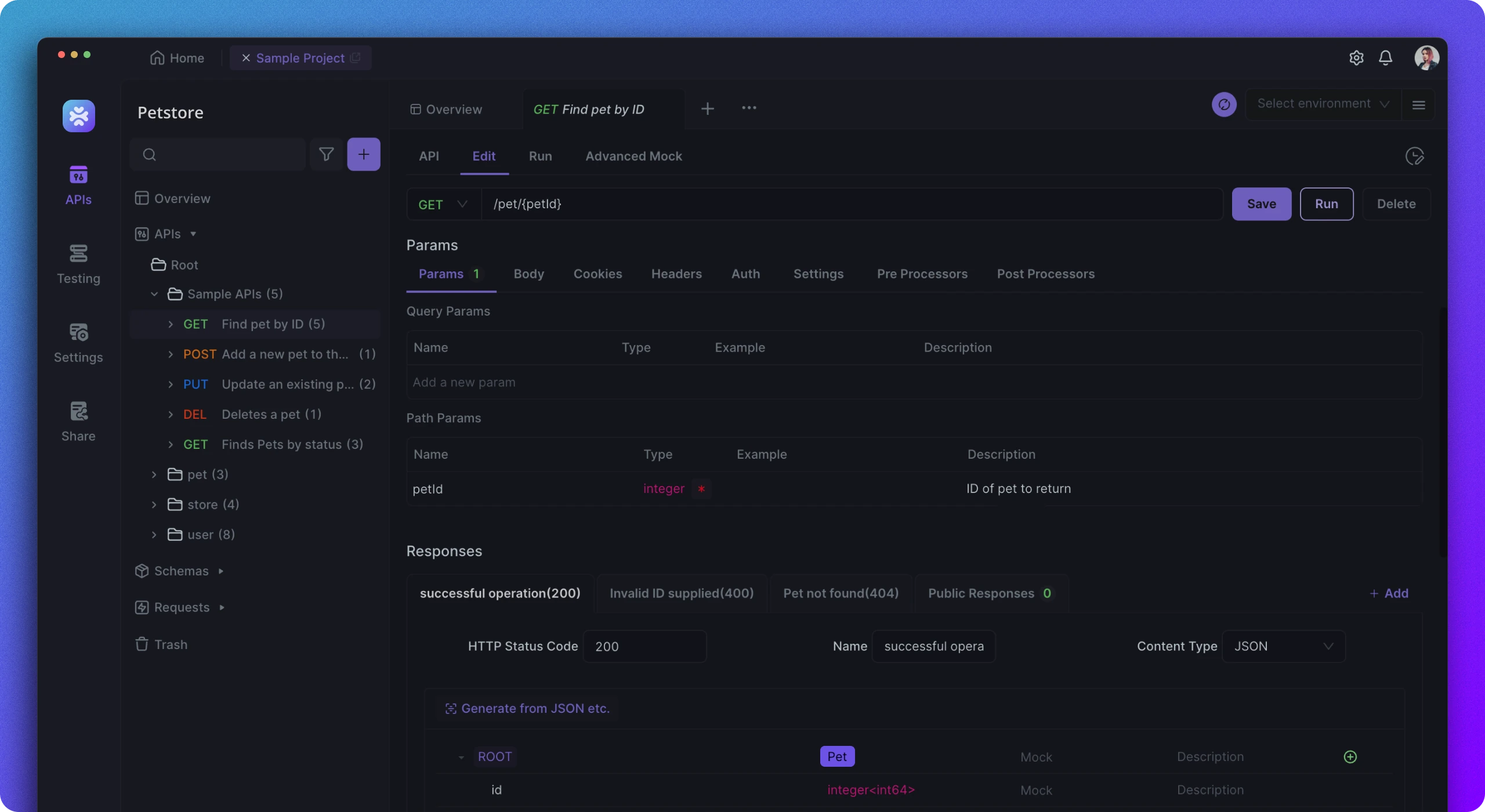
Here’s how Apidog can help you harness the power of JSONPath effectively:
First. you'll need to send requests to your APIs and view the responses directly with Apidog. Then you can use the interface provided to apply a JSONPath query to extract the titles of all action movies from the response.
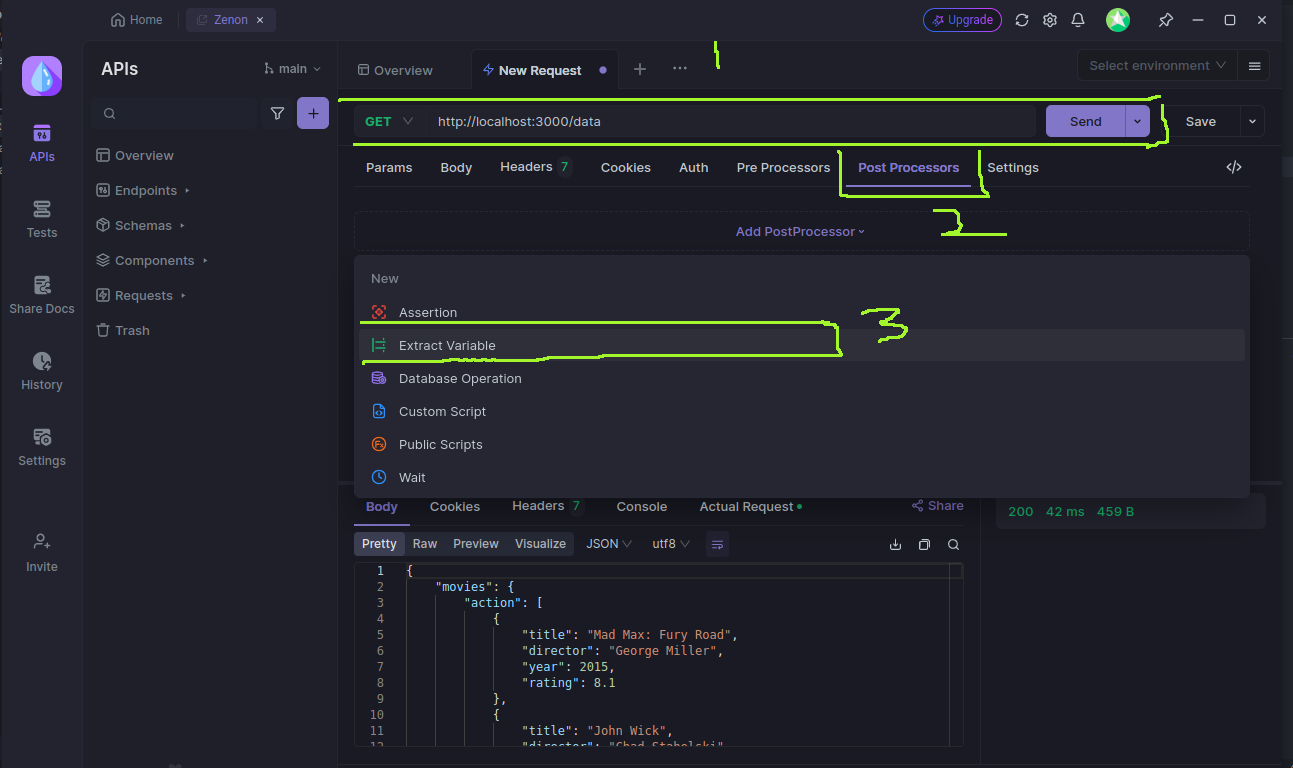
From the screenshot above, you'll see that (1) we send a GET
request to our server - I created a simple express server using the JSON data we got above as the response. Then I switched to the Post Processors tab (2), and added a new process - Extract Variable
(3).
Upon clicking on the Extract Variable
, you'd be shown the below page;
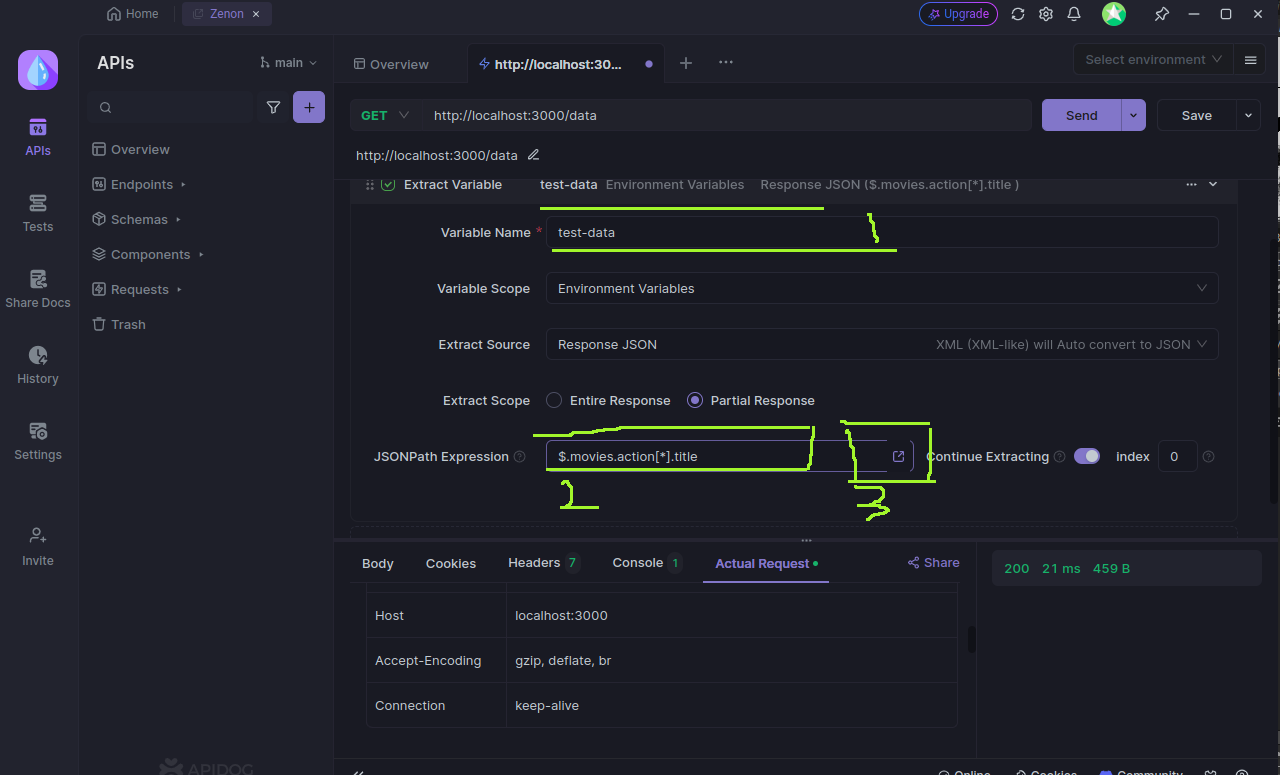
You can set a variable name (1) if you like. Variables are useful if you want to monitor or build different APIs and you need a single place to manage the general configuration. The variables here are like .env
files.
The other step is to type the JSONPath you want to filter out (2). In our case here, we want to get the titles of movies in the action array of our JSON. Once you type in the path currently, click on the arrow button (3) to open up the response page of the query as shown below.
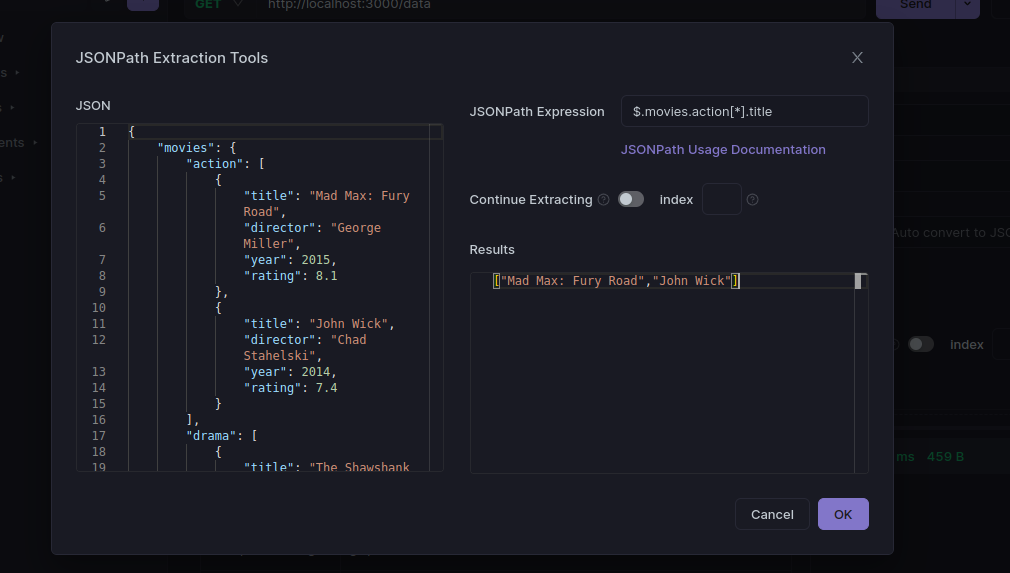
As you can see from the response above, Apidog filtered out the titles of the movies in the action array and displayed them to us.
If you want to try this out, then make sure to download and install Apidog and send your first request with it.
Conclusion
JSONPath is an invaluable tool for navigating and extracting data from JSON responses in APIs. It simplifies the process of working with complex JSON structures, making your development workflow more efficient. By integrating JSONPath with tools like Apidog, you can easily filter and retrieve the exact data you need, enhancing your API testing and debugging processes. Whether you’re extracting movie titles, filtering specific records, or validating responses, JSONPath offers a clear, concise way to handle JSON data. Give it a try in your next project and experience the streamlined simplicity it brings.