In the age of AI-powered applications, integrating advanced language models into your software can unlock unprecedented capabilities—from intelligent chatbots to data analysis tools. DeepSeek’s APIs offer cutting-edge AI functionalities, but the real challenge lies in seamlessly integrating them into your app without getting bogged down by debugging or boilerplate code.
This guide walks you through the entire process of integrating DeepSeek APIs into your application, leveraging an intuitive API platform — Apidog to test, debug, and generate ready-to-use code snippets in minutes. Whether you’re building a Python backend or a JavaScript frontend, by the end of this article, you’ll have a fully functional API integration with minimal effort.
Prerequisites
- A DeepSeek account (sign up here).
- Basic knowledge of REST APIs and programming (Python/JavaScript used here).
- A tool like Apidog to test APIs.
Step 1: Get the DeepSeek API Key
Every API request requires authentication. Here’s how to get your key:
- Log into your DeepSeek account.
- Navigate to
API Keys
under your account settings. - Click
Create new APl key
and copy it.
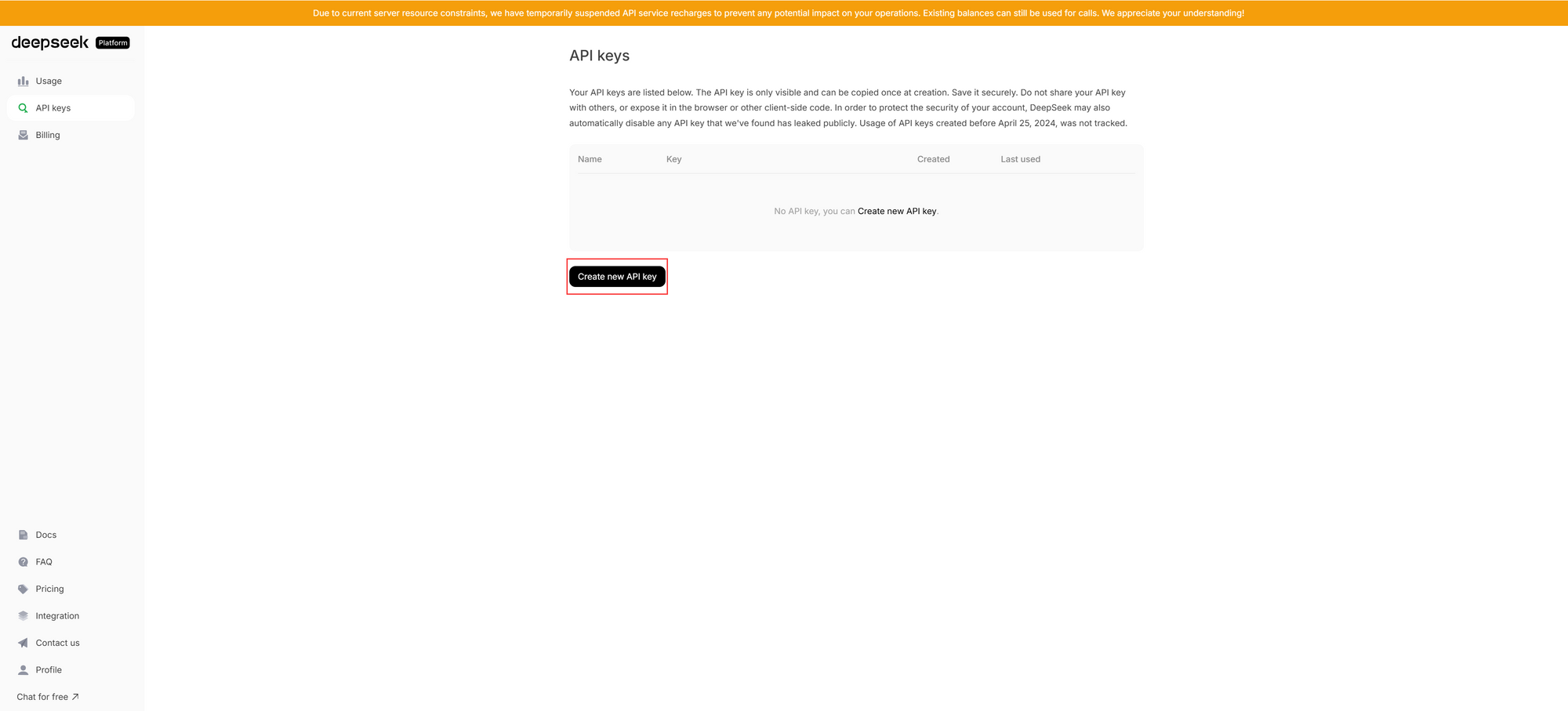
⚠️ Important: Treat this key like a password. Never expose it in client-side code or public repositories.
Step 2: Test the DeepSeek API
DeepSeek’s API documentation created by Apidog provides an interactive playground to test endpoints directly on the documentation without writing a single line of code. Here’s how:
1. Open the Documentation: Navigate to the Create Chat Completion endpoint.
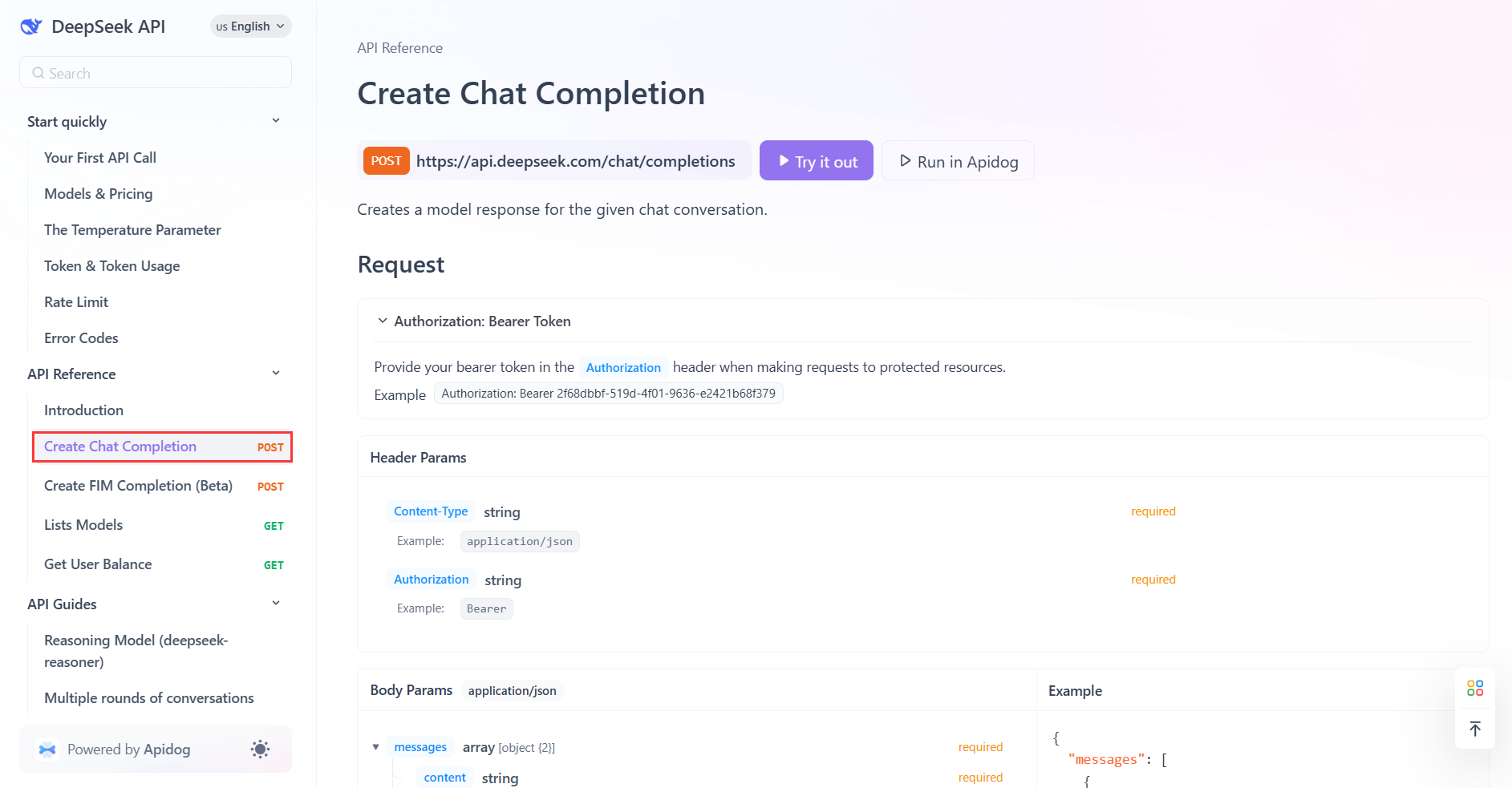
2. Authenticate:
- Click on
Try it out
orRun in Apidog
. - On the pop-out request panel, navigate to the Headers or Auth section, and add your API key:
Authorization: Bearer YOUR_API_KEY
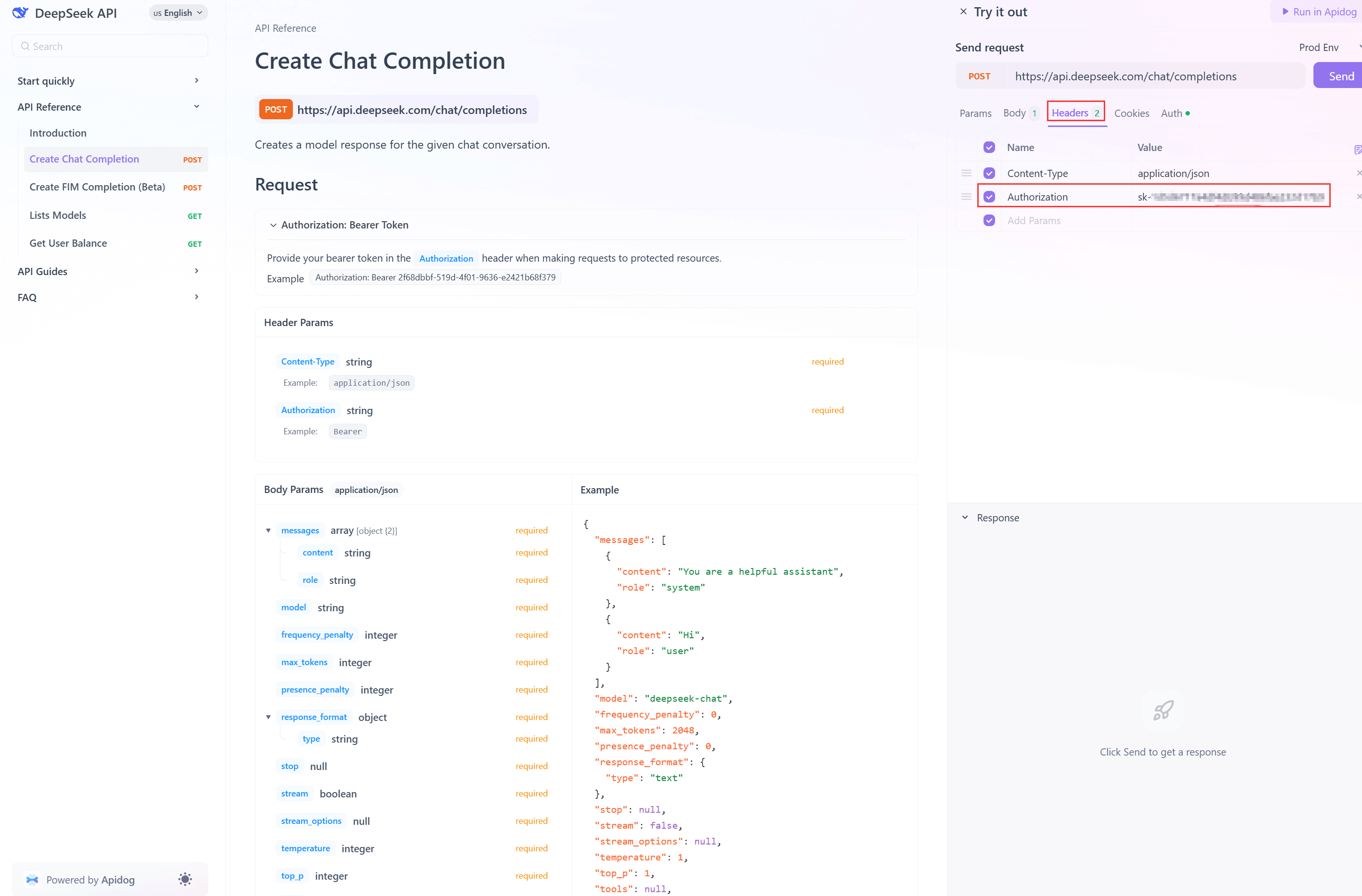
3. Craft Your Request:
In the request body, specify the model (e.g., deepseek-chat
) and add a message:
{
"model": "deepseek-chat",
"messages": [
{"role": "user", "content": "Explain quantum computing in 3 sentences."}
]
}
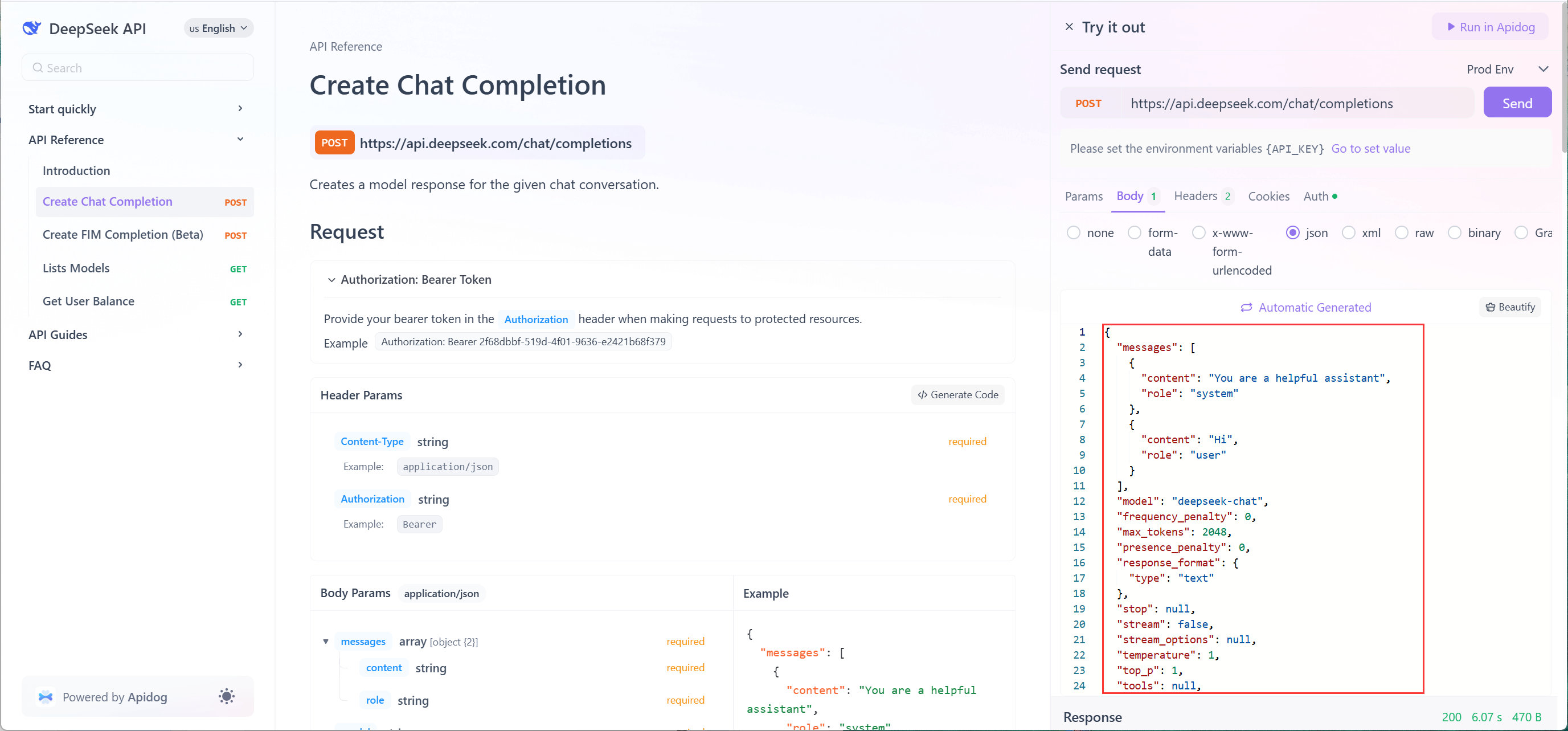
4. Send the Request: Click Send
and view the response in the real time.
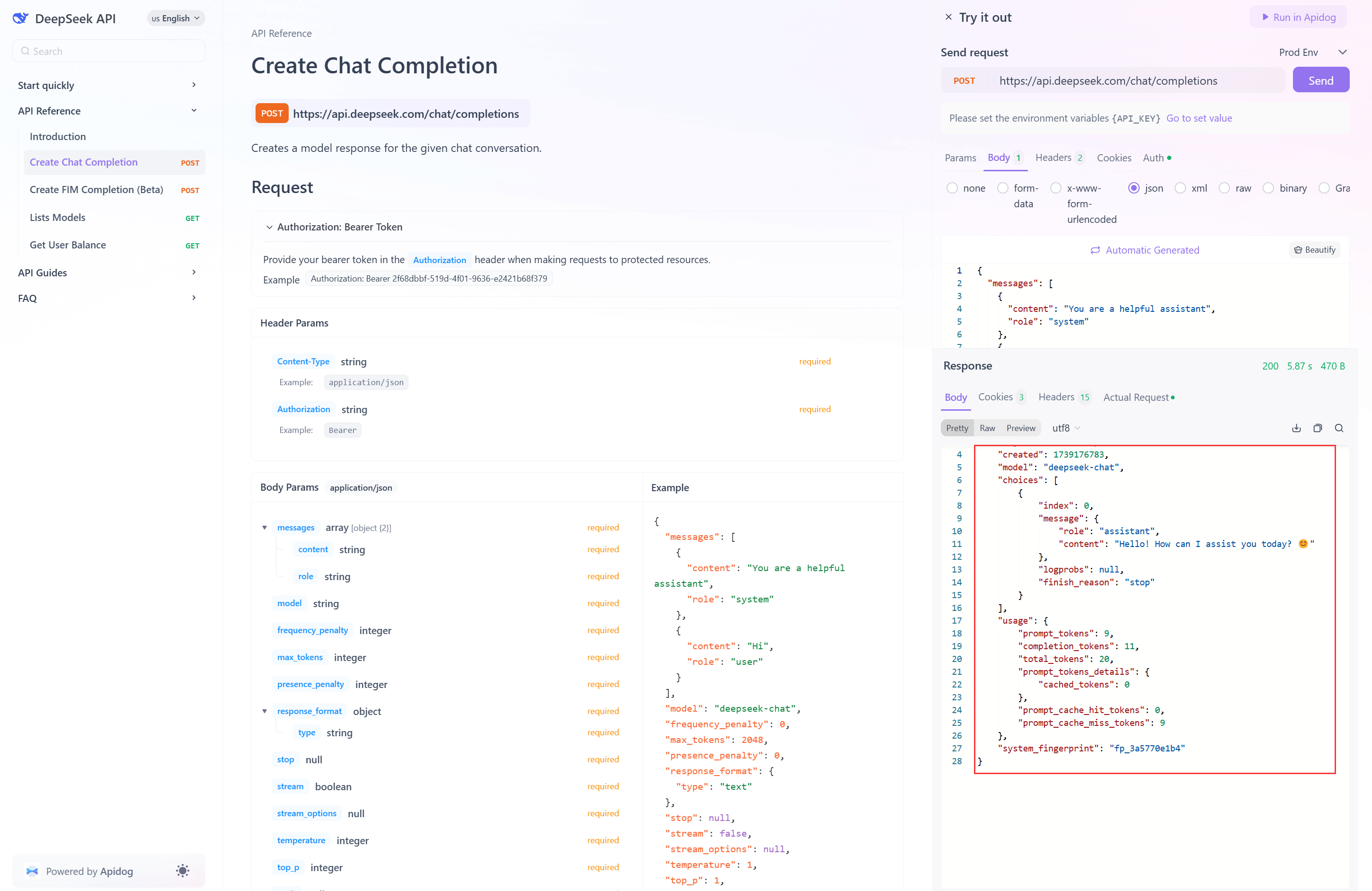
Why This Matters: Testing APIs manually helps you validate payload structures and error handling before writing integration code.
Step 3: Generate Code Snippets for Your Stack
Apidog allows you to generate out-of-box business codes for different languages and frameworks based on the API documentation that can be directly used in your stack. Here is how:
1. Open the DeekSeek project on the Apidog app:
- To open the DeepSeek API project in the Apidog web app, simply click "Run in Apidog" at the top right of the documentation.
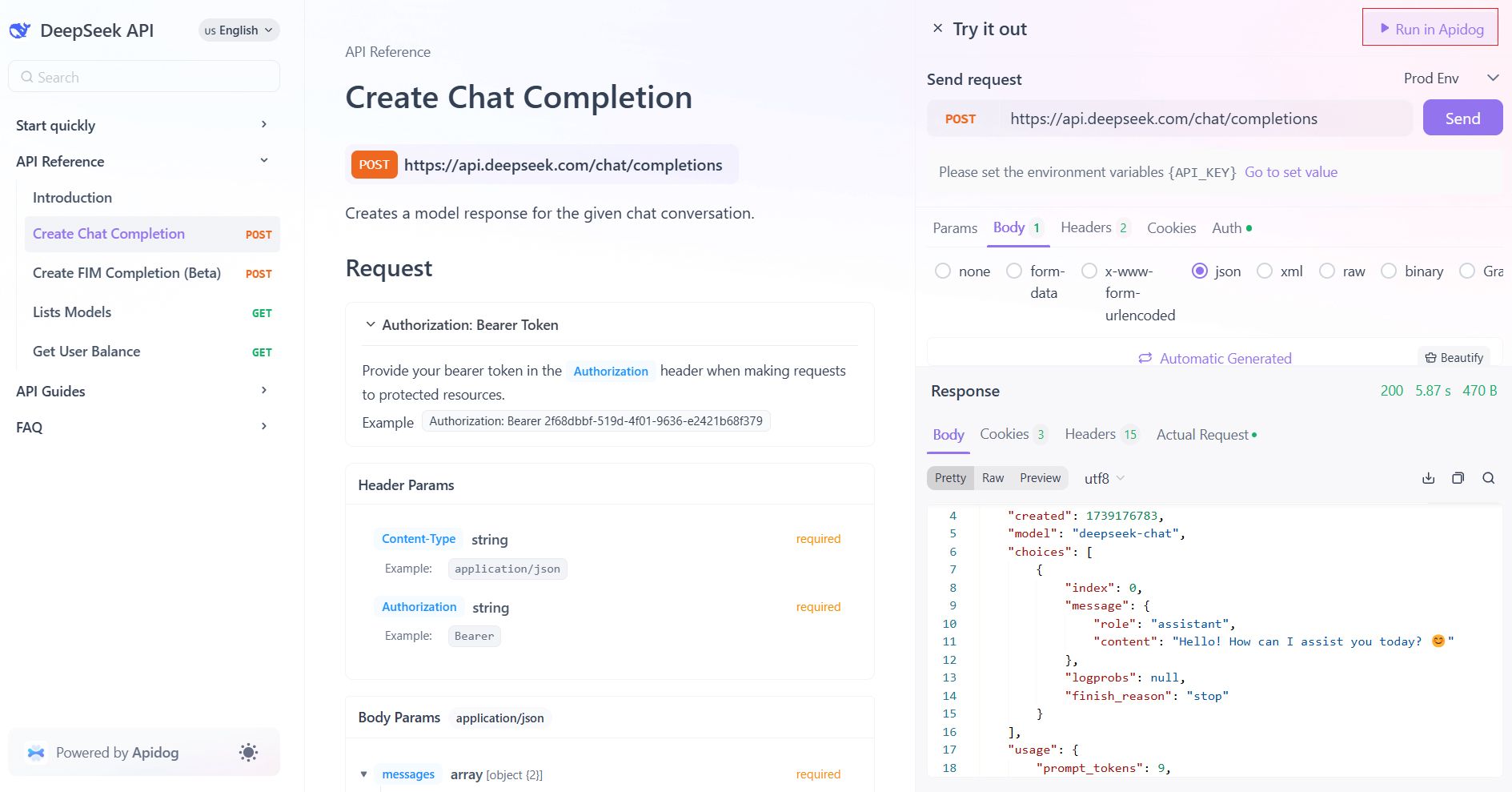
- PRO TIP: To open the project in the Apidog desktop app, click "Open on desktop" at the top right after that.
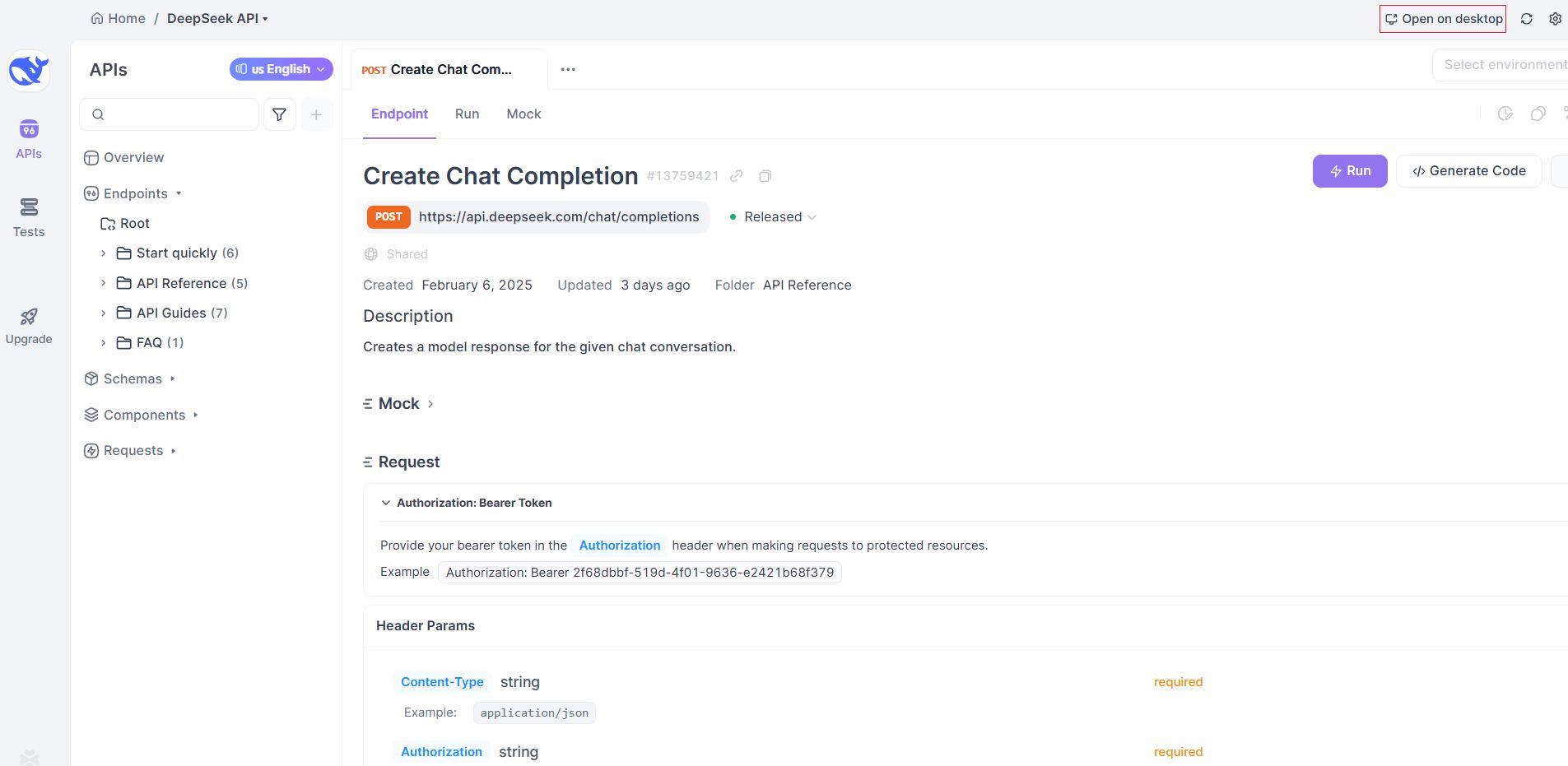
2. Choose Your Language:
- On the DeepSeek API documentation page, click the
</> Generate Code
button.
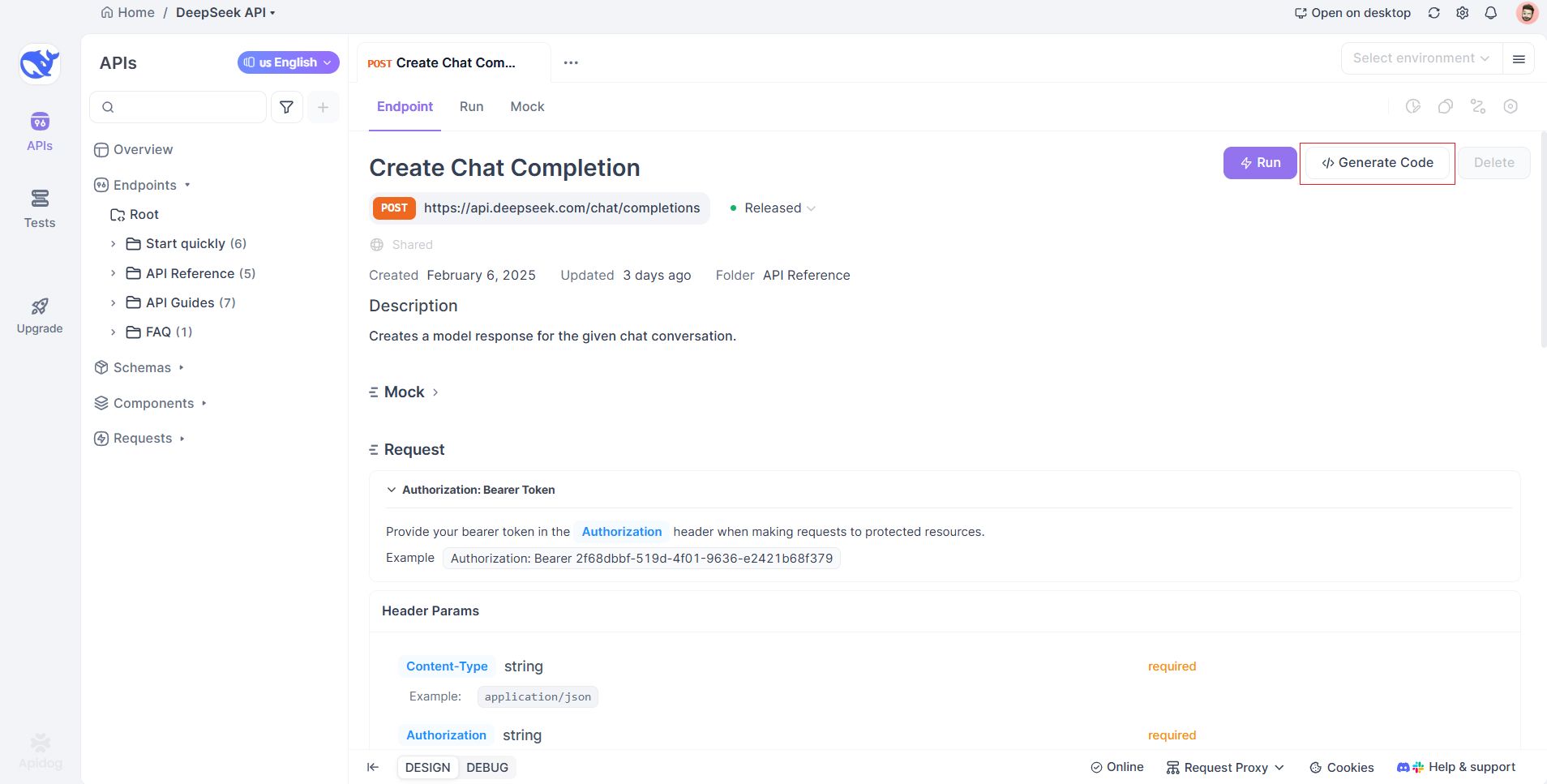
- Select your language (e.g., Python, JavaScript, Go, and more).
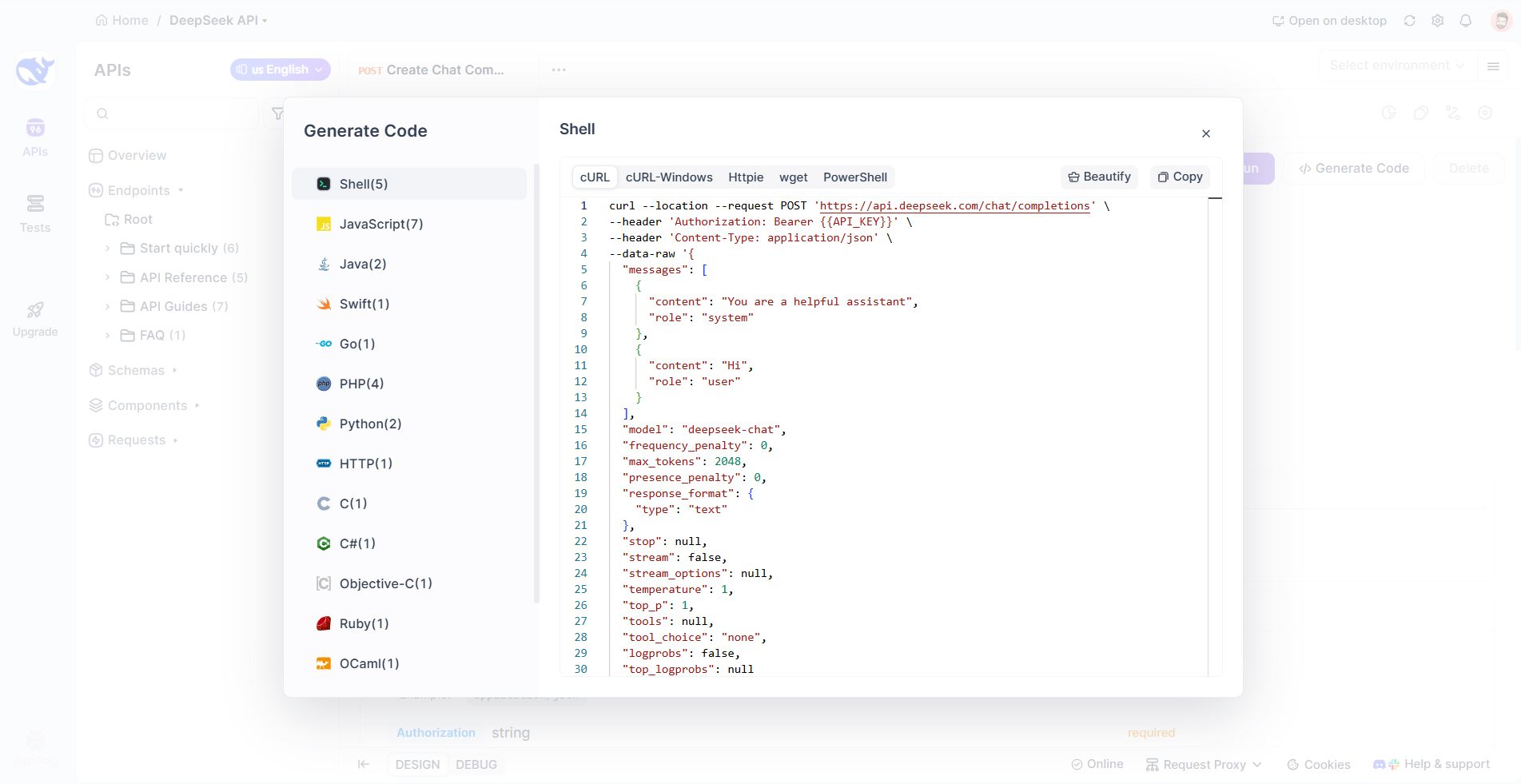
3. Copy-Paste the Code:
- For Python (using
requests
):
import requests
import json
url = "https://api.deepseek.com/chat/completions"
payload = json.dumps({
"messages": [
{
"content": "You are a helpful assistant",
"role": "system"
},
{
"content": "Hi",
"role": "user"
}
],
"model": "deepseek-chat",
"frequency_penalty": 0,
"max_tokens": 2048,
"presence_penalty": 0,
"response_format": {
"type": "text"
},
"stop": None,
"stream": False,
"stream_options": None,
"temperature": 1,
"top_p": 1,
"tools": None,
"tool_choice": "none",
"logprobs": False,
"top_logprobs": None
})
headers = {
'Authorization': 'Bearer {{API_KEY}}',
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
- For JavaScript (using
fetch
):
var myHeaders = new Headers();
myHeaders.append("Authorization", "Bearer {{API_KEY}}");
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({
"messages": [
{
"content": "You are a helpful assistant",
"role": "system"
},
{
"content": "Hi",
"role": "user"
}
],
"model": "deepseek-chat",
"frequency_penalty": 0,
"max_tokens": 2048,
"presence_penalty": 0,
"response_format": {
"type": "text"
},
"stop": null,
"stream": false,
"stream_options": null,
"temperature": 1,
"top_p": 1,
"tools": null,
"tool_choice": "none",
"logprobs": false,
"top_logprobs": null
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://api.deepseek.com/chat/completions", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
4. Customize the Code: Replace YOUR_API_KEY
and modify the message content.
Step 4: Integrate into Your Application
Let’s build a simple Python CLI app that uses DeepSeek’s API to answer user questions.
Project Setup
1. Create a directory and install dependencies:
mkdir deepseek-cli && cd deepseek-cli
pip install requests python-dotenv
2. Store your API key securely using .env
:
echo "DEEPSEEK_API_KEY=your_api_key_here" > .env
Code Implementation
import argparse
import asyncio
import json
import os
import sys
from typing import AsyncGenerator, Generator
import httpx
import requests
async def async_deepseek(
api_key: str,
model: str,
messages: list,
temperature: float
) -> AsyncGenerator[str, None]:
"""
Asynchronous generator for streaming DeepSeek responses
"""
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {api_key}",
}
data = {
"model": model,
"messages": messages,
"temperature": temperature,
"stream": True,
}
async with httpx.AsyncClient() as client:
async with client.stream(
"POST",
"https://api.deepseek.com/v1/chat/completions",
headers=headers,
json=data,
) as response:
response.raise_for_status()
async for line in response.aiter_lines():
if line.startswith("data: "):
try:
json_data = json.loads(line[6:])
if chunk := json_data["choices"][0]["delta"].get("content", ""):
yield chunk
except json.JSONDecodeError:
pass
def sync_deepseek(
api_key: str,
model: str,
messages: list,
temperature: float
) -> Generator[str, None, None]:
"""
Synchronous generator for streaming DeepSeek responses
"""
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {api_key}",
}
data = {
"model": model,
"messages": messages,
"temperature": temperature,
"stream": True,
}
with requests.post(
"https://api.deepseek.com/v1/chat/completions",
headers=headers,
json=data,
stream=True,
) as response:
response.raise_for_status()
for line in response.iter_lines():
if line:
decoded_line = line.decode("utf-8")
if decoded_line.startswith("data: "):
try:
json_data = json.loads(decoded_line[6:])
if chunk := json_data["choices"][0]["delta"].get("content", ""):
yield chunk
except json.JSONDecodeError:
pass
def main():
parser = argparse.ArgumentParser(
description="DeepSeek CLI Client - Chat with DeepSeek models"
)
parser.add_argument(
"prompt",
type=str,
help="Your message to send to DeepSeek"
)
parser.add_argument(
"--model",
type=str,
default="deepseek-chat",
help="Model to use (default: deepseek-chat)"
)
parser.add_argument(
"--temperature",
type=float,
default=0.7,
help="Temperature parameter (default: 0.7)"
)
parser.add_argument(
"--async-mode",
action="store_true",
help="Use asynchronous mode (requires Python 3.7+)"
)
args = parser.parse_args()
api_key = os.getenv("DEEPSEEK_API_KEY")
if not api_key:
print("Error: Set your API key in DEEPSEEK_API_KEY environment variable")
sys.exit(1)
messages = [{"role": "user", "content": args.prompt}]
try:
if args.async_mode:
async def run_async():
try:
async for chunk in async_deepseek(
api_key=api_key,
model=args.model,
messages=messages,
temperature=args.temperature
):
print(chunk, end="", flush=True)
print()
except httpx.HTTPStatusError as e:
print(f"\nHTTP error occurred: {e.response.status_code} {e.response.reason_phrase}")
except Exception as e:
print(f"\nAn error occurred: {str(e)}")
asyncio.run(run_async())
else:
try:
for chunk in sync_deepseek(
api_key=api_key,
model=args.model,
messages=messages,
temperature=args.temperature
):
print(chunk, end="", flush=True)
print()
except requests.exceptions.HTTPError as e:
print(f"\nHTTP error occurred: {e.response.status_code} {e.response.reason_phrase}")
except Exception as e:
print(f"\nAn error occurred: {str(e)}")
except KeyboardInterrupt:
print("\n\nOperation interrupted by user")
sys.exit(0)
if __name__ == "__main__":
main()
Run the App
Type a question (e.g., “What’s the capital of France?”) and see the response!
Step 5: Handle Errors and Edge Cases
APIs can fail due to rate limits, invalid keys, or network issues. Implement robust error handling:
1. Check HTTP Status Codes:
401 Unauthorized
: Invalid API key.429 Too Many Requests
: Rate limit exceeded.500 Internal Server Error
: Server-side issue.
2. Retry Mechanisms:
from time import sleep
def get_deepseek_response(prompt, retries=3):
# ... existing code ...
except requests.exceptions.RequestException as e:
if retries > 0:
sleep(2)
return get_deepseek_response(prompt, retries - 1)
else:
return f"Error: {str(e)}"
3. Validate Inputs:
Ensure prompts are within token limits and properly formatted.
Step 6: Optimize for Production
- Cache Responses: Use Redis or Memcached to store frequent queries.
- Monitor Usage: Track API calls to avoid exceeding quotas.
- Async Processing: For high-throughput apps, use async libraries like
aiohttp
(Python) oraxios
(JavaScript).
Conclusion
Integrating DeepSeek’s APIs into your app doesn’t have to be a time sink. By using interactive documentation to test endpoints, generate code, and debug on the fly, you can ship AI-powered features in hours—not days.
The example above is just the tip of the iceberg. With DeepSeek’s models, you can build anything from automated content generators to AI tutors. Now that you’ve got the blueprint, go ahead and transform your app into an AI powerhouse!