What is Node.js?
Node.js is an open-source, cross-platform Javascript runtime environment based on the Chrome V8 engine. It allows developers to use Javascript to construct high-performance and extensible web applications which can make Javascript run in the server side, not just on the browser side.
Node.js package manager npm is the largest open-source library ecosystem in the world. Front-end development and back-end development can be achieved using Node.js , which has features such as event-driven and non-blocking IO models.
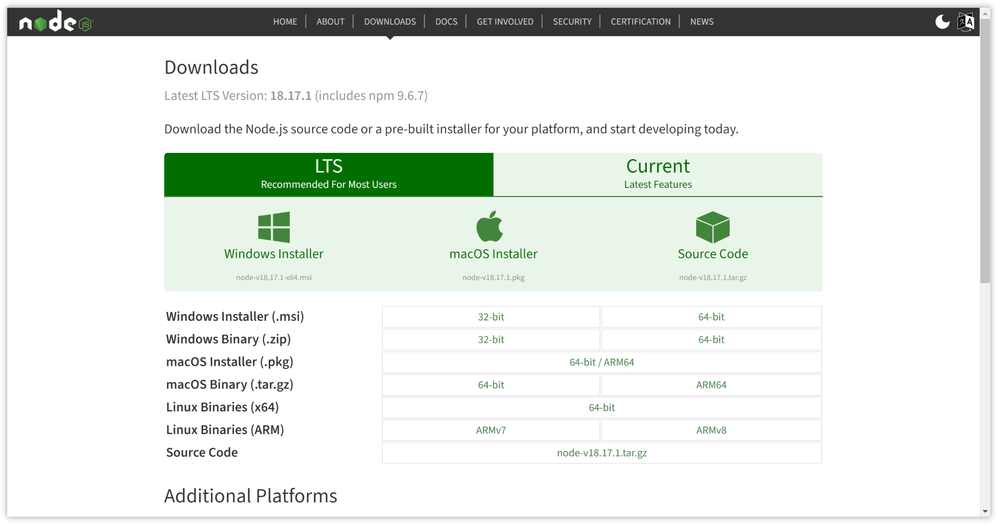
Install Node.js and Environment Configuration
To get started with Node.js, first, you need to install it on your computer. The common ways are as follows.
- Download the Node.js package from the official website: view here: https://nodejs.org/en
choose the system fit for you. Then follow the instructions always next is ok. You can custom install the directory and input node -v
and npm -v
to check whether the installation is it success.
- Using package manager npm: (suitable for Linux/macOS) If your are using Linux or macOS, you can run this command in the terminal:
For Linux:
sudo apt install nodejs
sudo apt install npm
For macOS:
brew install node
Configure Node.js Environment:
After installing Node.js, you may also need to configure some environment settings to better use it:
1. Check the installation: Run the following command in the command line to check whether Node.js and npm (Node.js package manager) are installed correctly:
node -v
npm -v
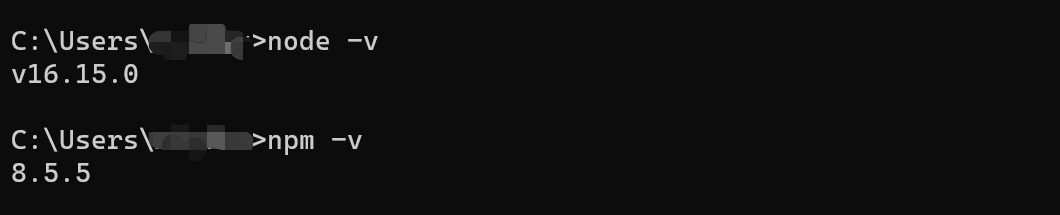
2. Update npm: npm releases updates frequently. You can update npm to the latest version by running the following command:
npm install -g npm
3. Choose a version management tool: For different projects, you may need to use different versions of Node.js. You can use tools such as nvm
(Node Version Manager) to manage multiple Node.js versions. How to install nvm will not be described here.

Practical example: Building a simple Node.js server
The following is a basic Node.js practical example that demonstrates how to create a simple server and handle basic routing and requests:
// Import the http module
const http = require('http');
// Create a server
const server = http.createServer((req, res) => {
if (req.url === '/') {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, Node.js Server!');
} else if (req.url === '/about') {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('About Page');
} else {
res.writeHead(404, { 'Content-Type': 'text/plain' });
res.end('404 Not Found');
}
});
// Listen on port
server.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
Create a file named in your IDE editor server.js
and paste the above code into it. Then navigate to the directory where the file is located in the terminal and run the following command to start the server:
node server.js
Now you can view the server's response by visiting http://localhost:3000
and in your browser .http://localhost:3000/about
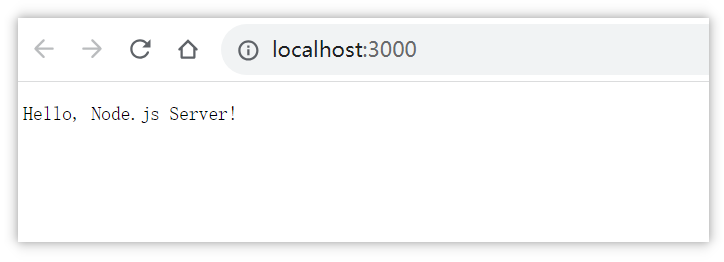
Apidog: An ultimate API Tool
Apidog is an all-in-one API platform, providing comprehensive documentation, debugging, mocking, and automated testing functionalities. It serves as a centralized hub for teams to craft detailed API documentation, ensuring clarity and accessibility throughout the development process. With integrated debugging features, developers can swiftly identify and resolve issues, minimizing downtime and maximizing efficiency.
If you want to explore the different methods for calling REST APIs from Node.js applications. Check this article:
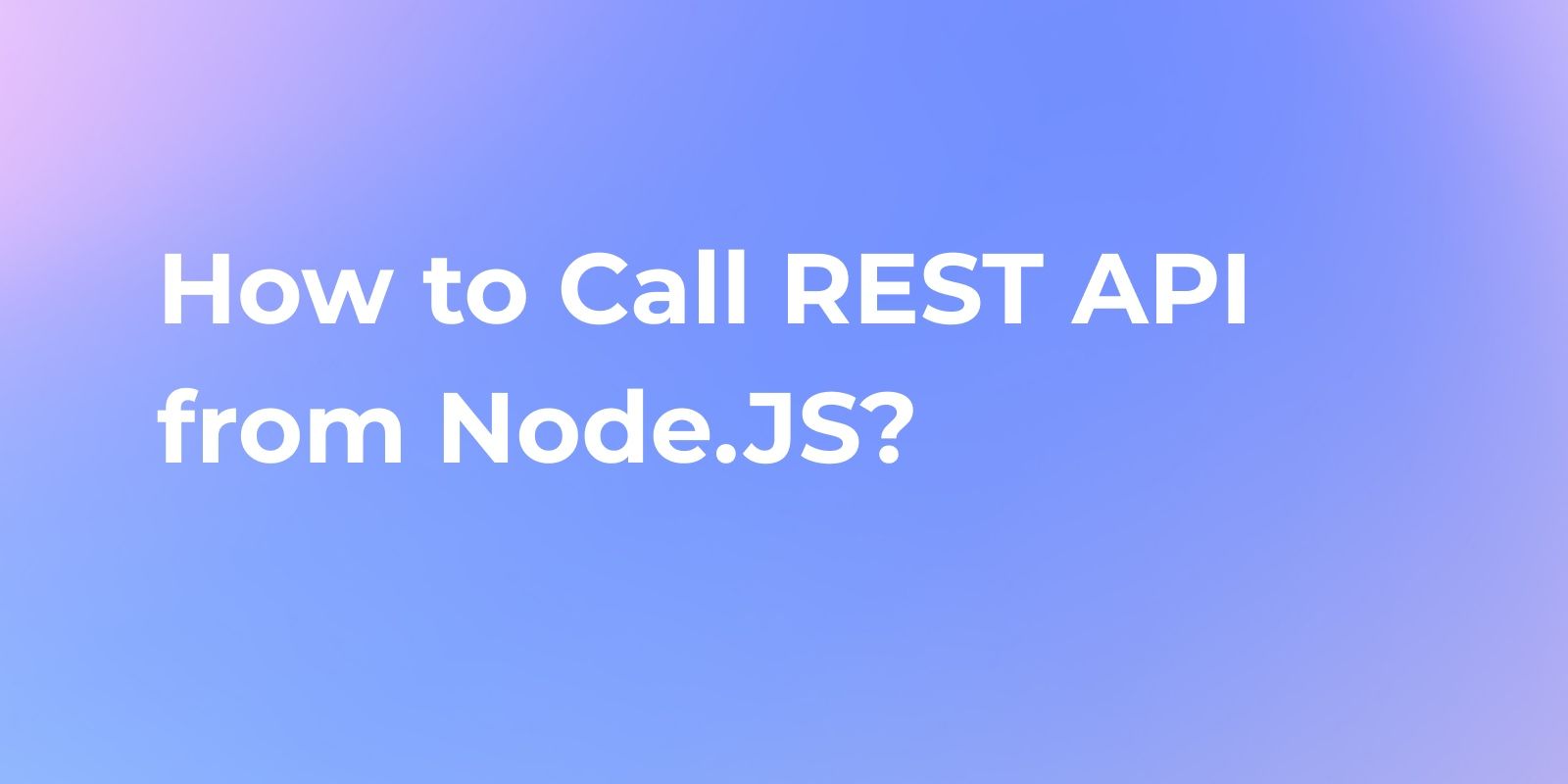
Bonus Tips of Node.js
- Learning resources: Node.js has a wealth of learning resources, including official documentation, tutorials, blog posts, and online courses. A deep understanding of the core concepts and features of Node.js will have a huge impact on your development experience.
- Package management: Use npm to easily install, manage, and share JavaScript packages.
npm init
You can use the command to create a file in the project folderpackage.json
, and then use tonpm install
install the required packages. - Asynchronous programming: Node.js adopts a non-blocking asynchronous programming model, which means that most operations are non-blocking, which can improve the performance of the application. But you also need to pay attention to correctly handling callbacks, Promise or async/await to avoid callback hell and asynchronous errors.