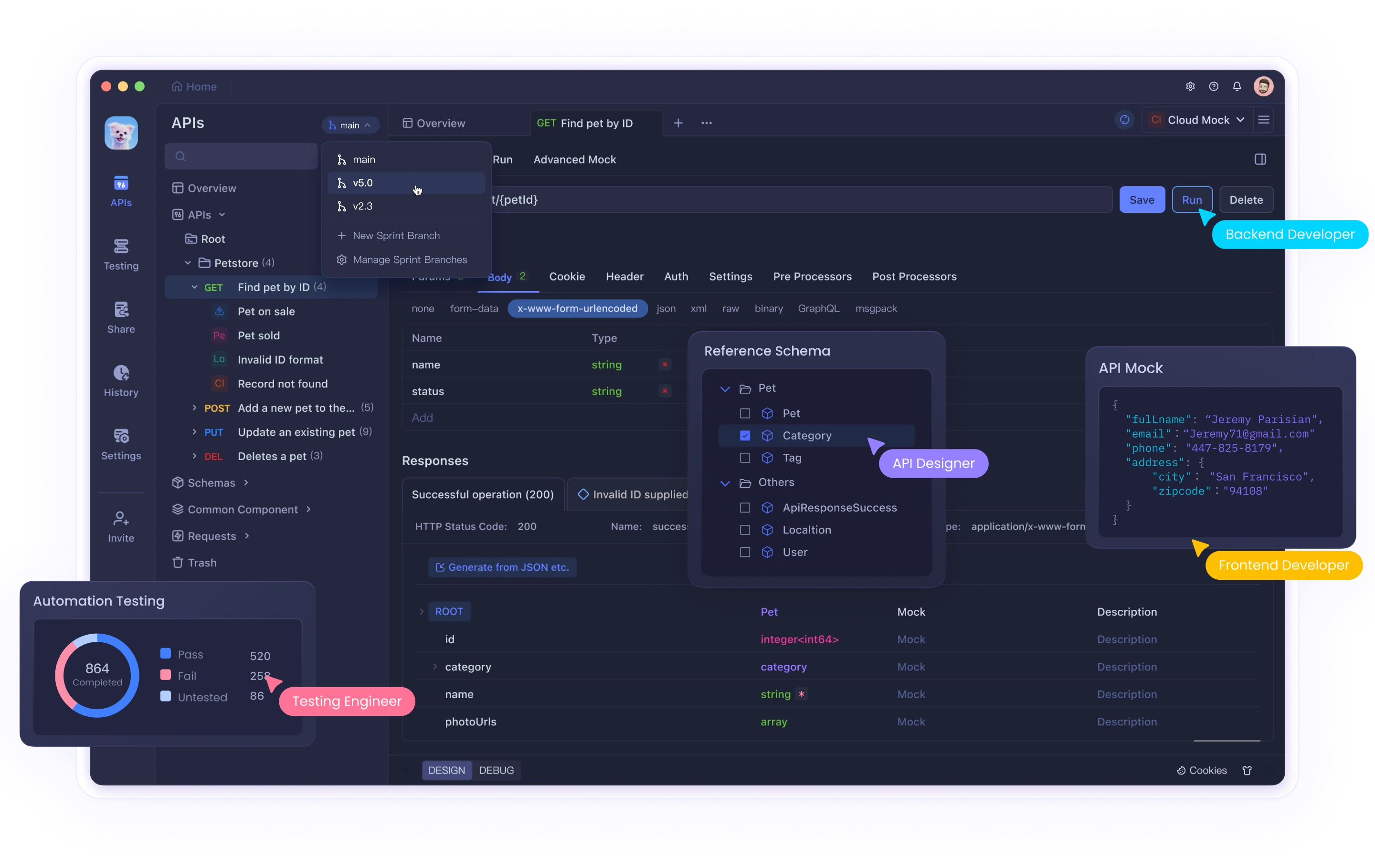
In my journey as a data scientist working with document intelligence, I've often encountered the challenging task of extracting and processing text from large, complex PDFs. Traditional OCR (Optical Character Recognition) pipelines typically involve multiple steps, specialized tools, and considerable processing time. However, the introduction of Gemini 2.0 Flash has transformed my approach to handling these documents. In this article, I'll share my personal workflow for using Gemini 2.0 Flash as a powerful OCR solution for large PDFs without resorting to complex RAG (Retrieval-Augmented Generation).
My Introduction to Gemini 2.0 Flash
My first encounter with Gemini 2.0 Flash came after struggling with a particularly challenging project involving hundreds of legacy financial reports in scanned PDF format. Traditional OCR tools were either failing to capture tables correctly or requiring extensive post-processing. When Google released Gemini 2.0 Flash with its impressive 1 million token context window and multimodal capabilities, I immediately recognized its potential.
Gemini 2.0 Flash isn't just another language model—it's a multimodal powerhouse capable of understanding both text and visual content within documents. This makes it uniquely suited for OCR tasks where context and visual understanding matter. With its ability to process up to approximately 1,500 pages in a single operation, it has become my go-to solution for large document OCR.
Setting Up My OCR Environment
Before diving into my OCR workflow, I needed to establish a reliable technical foundation. Here's how I set up my environment:
- Install Essential Libraries: First, I installed the necessary Python packages to interface with Gemini and handle PDFs:
pip install google-generativeai
pip install pypdf
pip install pdf2image
pip install pillow
pip install python-dotenv
- Configure API Access: I created a project in Google Cloud Console, enabled the Gemini API, and generated an API key. I store this key securely using environment variables:
import os
import google.generativeai as genai
from dotenv import load_dotenv
# Load API key from .env file
load_dotenv()
api_key = os.getenv('GOOGLE_API_KEY')
# Configure the Gemini API
genai.configure(api_key=api_key)
- Initialize the Model: I access Gemini 2.0 Flash which supports multimodal inputs:
# Initialize Gemini 2.0 Flash
model = genai.GenerativeModel('gemini-2.0-flash')
My PDF Conversion and Image Extraction Workflow
For effective OCR with Gemini 2.0 Flash, I've developed a systematic approach to handling PDFs:
- Conversion to Images: First, I convert PDF pages to high-resolution images:
from pdf2image import convert_from_path
import os
def convert_pdf_to_images(pdf_path, output_folder, dpi=300):
# Create output directory if it doesn't exist
if not os.path.exists(output_folder):
os.makedirs(output_folder)
# Convert PDF pages to images
images = convert_from_path(pdf_path, dpi=dpi)
# Save images to the output folder
image_paths = []
for i, image in enumerate(images):
image_path = os.path.join(output_folder, f'page_{i+1}.jpg')
image.save(image_path, 'JPEG')
image_paths.append(image_path)
return image_paths
- Batch Processing for Large PDFs: For especially large documents, I process images in batches:
def batch_images(image_paths, batch_size=50):
"""Group images into batches for processing"""
for i in range(0, len(image_paths), batch_size):
yield image_paths[i:i + batch_size]
My OCR Pipeline Using Gemini 2.0 Flash
Here's where the power of Gemini 2.0 Flash truly shines in my workflow:
- Direct Image-to-Text Conversion: I process images directly through the multimodal capabilities:
from PIL import Image
from IPython.display import display
import base64
import io
def ocr_with_gemini(image_paths, instruction):
"""Process images with Gemini 2.0 Flash for OCR"""
images = [Image.open(path) for path in image_paths]
prompt = f"""
{instruction}
These are pages from a PDF document. Extract all text content while preserving the structure.
Pay special attention to tables, columns, headers, and any structured content.
Maintain paragraph breaks and formatting.
"""
response = model.generate_content([prompt, *images])
return response.text
- My Approach to Handling Complex Document Elements:
For documents with complex layouts that include tables, charts, and multi-column text, I use specific instructions:
def ocr_complex_document(image_paths):
instruction = """
Extract ALL text content from these document pages.
For tables:
1. Maintain the table structure using markdown table format
2. Preserve all column headers and row labels
3. Ensure numerical data is accurately captured
For multi-column layouts:
1. Process columns from left to right
2. Clearly separate content from different columns
For charts and graphs:
1. Describe the chart type
2. Extract any visible axis labels, legends, and data points
3. Extract any title or caption
Preserve all headers, footers, page numbers, and footnotes.
"""
return ocr_with_gemini(image_paths, instruction)
- Specialized OCR for Financial Documents:
Many of the PDFs I work with contain financial data, which requires special attention:
def ocr_financial_document(image_paths):
instruction = """
Extract ALL text content from these financial document pages.
Pay particular attention to:
1. All numerical values and ensure they're accurately transcribed
2. Currency symbols and their correct association with numbers
3. Financial tables - maintain their exact structure and alignment
4. Balance sheets, income statements, and cash flow statements
5. Footnotes and disclosures - these often contain crucial information
6. Any dates associated with financial periods
Format tables using markdown table syntax to preserve their structure.
"""
return ocr_with_gemini(image_paths, instruction)
My Quality Assurance Process
I've found that OCR quality can vary based on document characteristics, so I implement a quality check process:
def verify_ocr_quality(image_path, extracted_text):
"""Verify the quality of OCR results for a specific page"""
image = Image.open(image_path)
prompt = f"""
I have a document page and text that was extracted from it using OCR.
Compare the original image with the extracted text and identify any errors or omissions.
Focus on:
1. Missing text
2. Incorrectly recognized characters
3. Table structure issues
4. Issues with special characters or symbols
Extracted text:
{extracted_text}
"""
response = model.generate_content([prompt, image])
return response.text
I use this function to spot-check random pages from large documents to ensure quality.
My Process for Handling Large Documents Beyond Context Limits
While Gemini 2.0 Flash has an impressive 1 million token limit, some of my documents still exceed this capacity. For these cases, I've developed a sequential processing approach:
- Process PDF in Meaningful Segments:
def process_large_pdf(pdf_path, output_folder, output_file):
# Convert PDF to images
image_paths = convert_pdf_to_images(pdf_path, output_folder)
# Create batches of images (e.g., by chapter or section)
batches = batch_images(image_paths, 30) # Adjust batch size based on document complexity
full_text = ""
for i, batch in enumerate(batches):
print(f"Processing batch {i+1}...")
batch_text = ocr_with_gemini(batch, "Extract all text, maintaining document structure")
full_text += f"\n\n--- BATCH {i+1} ---\n\n{batch_text}"
# Save the full extracted text
with open(output_file, 'w', encoding='utf-8') as f:
f.write(full_text)
return full_text
- Post-Processing for Document Coherence:
After extracting text from all batches, I use Gemini 2.0 Flash to ensure consistency:
def harmonize_document(extracted_text):
prompt = """
The following text was extracted from a large PDF document in batches.
Harmonize the content by:
1. Removing any batch separation markers
2. Ensuring consistent formatting throughout
3. Fixing any table structure issues at batch boundaries
4. Ensuring paragraph and section flow is natural across batch boundaries
Original extracted text:
"""
response = model.generate_content(prompt + extracted_text)
return response.text
Specialized Use Case: My Approach to Historical Document OCR
Some of my most challenging OCR projects involve historical documents with aged paper, faded text, or unusual fonts. For these, I've developed a specialized approach:
def historical_document_ocr(image_paths):
instruction = """
Extract text from these historical document images.
Consider the following challenges:
1. Aged paper with stains or discoloration
2. Faded ink or typefaces
3. Old-fashioned typography and ligatures
4. Handwritten annotations
5. Non-standard page layouts
Prioritize accuracy over format preservation when necessary.
Note any text that appears uncertain with [?].
"""
extracted_text = ocr_with_gemini(image_paths, instruction)
# Additional context-based correction
correction_prompt = f"""
The following text was extracted from a historical document that may have quality issues.
Review the text for typical OCR errors in historical documents:
1. Fix words that were likely misinterpreted due to aged paper or faded ink
2. Correct archaic spellings only if they appear to be OCR errors (not if they're actually period-appropriate)
3. Resolve any uncertain text marked with [?] if context makes the correct reading clear
Original text:
{extracted_text}
"""
corrected_text = model.generate_content(correction_prompt)
return corrected_text.text
Practical Results From My OCR Projects
In my experience using Gemini 2.0 Flash for OCR across dozens of large documents, I've seen remarkable improvements over traditional OCR methods:
- Accuracy Improvements: For complex financial reports with tables and charts, my accuracy rates improved from around 85% with traditional OCR to over 95% with Gemini 2.0 Flash. The model's ability to understand context helps it correctly interpret ambiguous characters based on surrounding content.
- Processing Time Reduction: What used to take me multiple hours of processing and manual correction now frequently completes in minutes. By eliminating the need for separate OCR and text understanding steps, my workflow has become significantly more efficient.
- Table Structure Preservation: One of the most impressive capabilities I've seen is Gemini 2.0 Flash's ability to maintain complex table structures. In financial documents, this has been invaluable for ensuring data integrity.
Conclusion: The Impact on My Document Processing Workflow
Adopting Gemini 2.0 Flash for OCR of large PDFs has transformed my document processing workflow. The elimination of complex RAG architectures for most use cases has simplified my technical infrastructure while improving results. The model's multimodal capabilities allow it to understand both the visual elements and textual content of documents simultaneously, resulting in more intelligent text extraction.
What impresses me most is the model's ability to handle edge cases that traditional OCR systems struggle with—handwritten annotations, watermarks, unusual fonts, and complex layouts. By leveraging Gemini 2.0 Flash's massive context window, I can process substantial portions of documents in a single operation, maintaining coherence and contextual understanding throughout.
For data scientists, researchers, and professionals who regularly work with large document collections, Gemini 2.0 Flash represents not just an incremental improvement but a fundamental shift in how we approach OCR and document understanding. The ability to "see" and "read" documents the way a human would—considering layout, context, and visual elements holistically—opens new possibilities for document intelligence applications.
As I continue to refine my workflow, I'm constantly discovering new ways this technology can streamline document processing tasks that were previously labor-intensive or technologically challenging. The future of document OCR is here, and it's revolutionizing how I work with the massive PDF archives that were once the bane of my professional existence.