Best Examples of WSDL Files (Updated 2024)
Enrich your knowledge by getting to know simple and complex examples of WSDL files.
Software these days is more powerful than ever. They can provide so much information and functionalities for users. With applications' increasingly powerful abilities, have you wondered how it is all achievable?
One type of file that appears often in the hindsight of application development is a WSDL file, but what is it?
What is a WSDL File?
WSDL (Web Services Description Language) files allow different applications to communicate with each other over a network in a standardized way. It connects web service providers and consumers, allowing data exchanges between, but not limited to, the two parties.
WSDL files are also known for providing ample description regarding a web service's capabilities, specifically for SOAP-based web services. WSDL files are written in XML (eXtensible Markup Language) language and are vital for SOAP APIs' testing.
More importantly, WSDL files are significant due to the definition of a formal agreement between the web service provider and the service consumer. WSDL files show what operations are available, the format and structure of messages, and other important details required when using the web service.
Structure of WSDL Files With Examples
WSDL files have key components that provide information for using it. This article will demonstrate parts of a WSDL file in XML language, using a weather web service as an example.
Types type
WSDL files type
section is used to define the data types that will be used in the web service operations.
<types>
<schema xmlns="http://www.w3.org/2001/XMLSchema" targetNamespace="http://example.com/weather">
<element name="City" type="string"/>
<element name="Weather" type="string"/>
</schema>
</types>
This example specifies the structure of the elements, including their names City
and Weather
being of the string
type.
Message message
The message
section defines the data elements that are being exchanged between the web service provider and the service user. In the examples above, you can see the message names GetWeatherRequest
and GetWeatherResponse
.
<message name="GetWeatherRequest">
<part name="City" element="tns:City"/>
</message>
<message name="GetWeatherResponse">
<part name="Weather" element="tns:Weather"/>
</message>
Here, GetWeatherRequest
and GetWeatherResponse
messages are defined with a part specifying the data elements being sent or received.
Port Type portType
The portType
section describes a set of operations that can be performed by the web service, such as the operation name GetWeather
. It defines the abstract interface for the service, listing the operations along with their input tns:GetWeatherRequest
and output tns:GetWeatherResponse
messages.
<portType name="WeatherServicePortType">
<operation name="GetWeather">
<input message="tns:GetWeatherRequest"/>
<output message="tns:GetWeatherResponse"/>
</operation>
</portType>
The example above shows WeatherServicePortType
a defined operation GetWeather
with specified input and output messages.
Binding binding
The binding
section specifies how the abstract operations defined in portType
are mapped to a concrete protocol for communication. It defines details such as the message format and the protocol (e.g., SOAP).
<binding name="WeatherServiceSoapBinding" type="tns:WeatherServicePortType">
<soap:binding style="document" transport="http://schemas.xmlsoap.org/soap/http"/>
<operation name="GetWeather">
<soap:operation soapAction="http://example.com/weather/GetWeather"/>
<input>
<soap:body use="literal"/>
</input>
<output>
<soap:body use="literal"/>
</output>
</operation>
</binding>
In this example, WeatherServiceSoapBinding
is defined with SOAP as the binding protocol, specifying details about the message format and transport.
Port port
The port
section specifies the network address where the web service can be accessed.
<service name="WeatherService">
<port name="WeatherServicePort" binding="tns:WeatherServiceSoapBinding">
<soap:address location="http://example.com/weather/service"/>
</port>
</service>
Here, WeatherService
is defined with WeatherServicePort
that uses the SOAP binding, and its address is specified as http://example.com/weather/service
.
Examples of WSDL Files
Simple Weather Web Service WSDL File Example
Compiling the different key component code snippets provided in the previous section, we can combine them together and create a simple weather web service WSDL file.
<?xml version="1.0" encoding="UTF-8"?>
<definitions xmlns="http://schemas.xmlsoap.org/wsdl/"
xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/"
xmlns:tns="http://example.com/weather"
targetNamespace="http://example.com/weather">
<types>
<schema xmlns="http://www.w3.org/2001/XMLSchema" targetNamespace="http://example.com/weather">
<element name="City" type="string"/>
<element name="Weather" type="string"/>
</schema>
</types>
<message name="GetWeatherRequest">
<part name="City" element="tns:City"/>
</message>
<message name="GetWeatherResponse">
<part name="Weather" element="tns:Weather"/>
</message>
<portType name="WeatherServicePortType">
<operation name="GetWeather">
<input message="tns:GetWeatherRequest"/>
<output message="tns:GetWeatherResponse"/>
</operation>
</portType>
<binding name="WeatherServiceSoapBinding" type="tns:WeatherServicePortType">
<soap:binding style="document" transport="http://schemas.xmlsoap.org/soap/http"/>
<operation name="GetWeather">
<soap:operation soapAction="http://example.com/weather/GetWeather"/>
<input>
<soap:body use="literal"/>
</input>
<output>
<soap:body use="literal"/>
</output>
</operation>
</binding>
<service name="WeatherService">
<port name="WeatherServicePort" binding="tns:WeatherServiceSoapBinding">
<soap:address location="http://example.com/weather/service"/>
</port>
</service>
</definitions>
In this simple weather web service example, it can be observed that:
- The web service operates under the namespace
http://example.com/weather
. - It defines two simple data types:
City
andWeather
. - There are two messages,
GetWeatherRequest
andGetWeatherResponse
, specifying the input and output data for theGetWeather
operation. WeatherServicePortType
port type defines theGetWeather
operation.WeatherServiceSoapBinding
specifies that the communication will use SOAP with a specific style and transport.WeatherService
service has a port namedWeatherServicePort
using the SOAP binding, and its address ishttp://example.com/weather/service
.
Complex E-Commerce Web Service WSDL File Example
Here is a more complicated e-commerce web service WSDL file example that includes multiple operations, data types, and features.
?xml version="1.0" encoding="UTF-8"?>
<definitions xmlns="http://schemas.xmlsoap.org/wsdl/"
xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/"
xmlns:tns="http://example.com/ecommerce"
targetNamespace="http://example.com/ecommerce">
<types>
<schema xmlns="http://www.w3.org/2001/XMLSchema" targetNamespace="http://example.com/ecommerce">
<element name="Product">
<complexType>
<sequence>
<element name="ProductId" type="string"/>
<element name="ProductName" type="string"/>
<element name="Price" type="decimal"/>
</sequence>
</complexType>
</element>
<element name="Order">
<complexType>
<sequence>
<element name="OrderId" type="string"/>
<element name="CustomerName" type="string"/>
<element name="Products" type="tns:Product" minOccurs="0" maxOccurs="unbounded"/>
</sequence>
</complexType>
</element>
</schema>
</types>
<message name="GetProductRequest">
<part name="ProductId" element="tns:ProductId"/>
</message>
<message name="GetProductResponse">
<part name="Product" element="tns:Product"/>
</message>
<message name="PlaceOrderRequest">
<part name="Order" element="tns:Order"/>
</message>
<message name="PlaceOrderResponse">
<part name="Confirmation" type="string"/>
</message>
<portType name="ECommerceServicePortType">
<operation name="GetProduct">
<input message="tns:GetProductRequest"/>
<output message="tns:GetProductResponse"/>
</operation>
<operation name="PlaceOrder">
<input message="tns:PlaceOrderRequest"/>
<output message="tns:PlaceOrderResponse"/>
</operation>
</portType>
<binding name="ECommerceServiceSoapBinding" type="tns:ECommerceServicePortType">
<soap:binding style="document" transport="http://schemas.xmlsoap.org/soap/http"/>
<operation name="GetProduct">
<soap:operation soapAction="http://example.com/ecommerce/GetProduct"/>
<input>
<soap:body use="literal"/>
</input>
<output>
<soap:body use="literal"/>
</output>
</operation>
<operation name="PlaceOrder">
<soap:operation soapAction="http://example.com/ecommerce/PlaceOrder"/>
<input>
<soap:body use="literal"/>
</input>
<output>
<soap:body use="literal"/>
</output>
</operation>
</binding>
<service name="ECommerceService">
<port name="ECommerceServicePort" binding="tns:ECommerceServiceSoapBinding">
<soap:address location="http://example.com/ecommerce/service"/>
</port>
</service>
</definitions>
In this complex e-commerce web service example, it can be observed that:
- Complex data types
Product
andOrder
are defined with nested elements (likeProductID
,ProductName
, andPrice
) and sequences. - Multiple operations
GetProduct
andPlaceOrder
are defined within theECommerceServicePortType
. - Messages
GetProductRequest
,GetProductResponse
,PlaceOrderRequest
,PlaceOrderResponse
are defined for each operation, specifying the input and output data. - The SOAP binding
ECommerceServiceSoapBinding
specifies the formatting details for communication using SOAP. - The service
ECommerceService
has a port namedECommerceServicePort
using the SOAP binding, and its address is specified ashttp://example.com/ecommerce/service
.
Editing WSDL Files Using Apidog
As WSDL files are relatively common in the software development scene, you might have to edit or create one yourself. With tons of API platforms out there, Apidog is an excellent choice.
Apidog allows developers, web service providers, and web service users of any background to make a seamless transition from other API platforms over to Apidog.
Fortunately, Apidog also covers WSDL file imports! If you are interested in trying it out, make sure to download Apidog using the buttons below.
Don't forget to sign in and start a new project once you enter. If you need help, take a look at Apidog's Help Docs.
Importing Your SOAP API Onto Apidog from a WSDL File
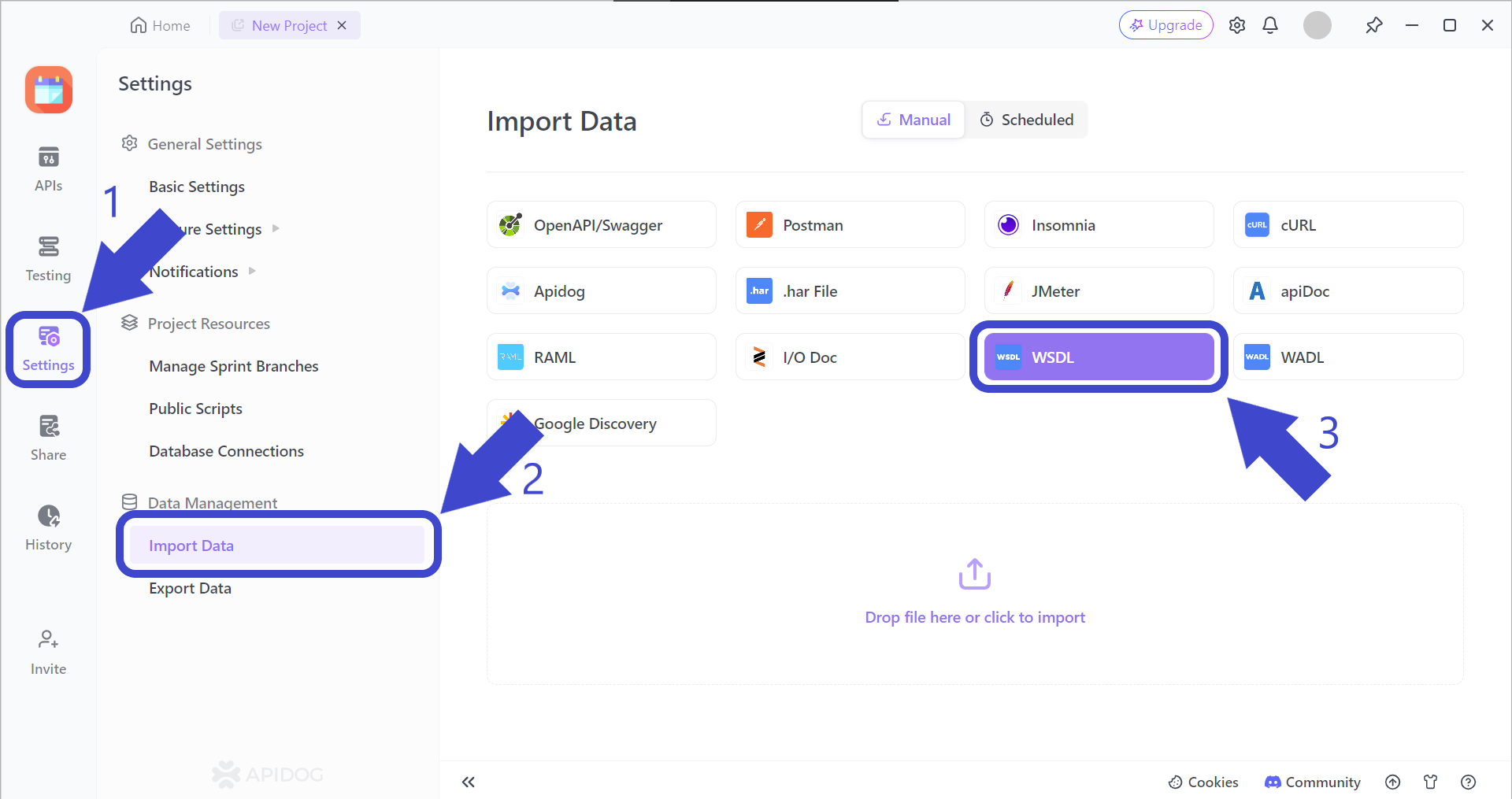
Once you have opened a new project, click the "Settings" button on the vertical toolbar found on the left side of the Apidog window. Then, click on Arrow 2 and Arrow 3 in that order.
Testing SOAP API Easily on Apidog
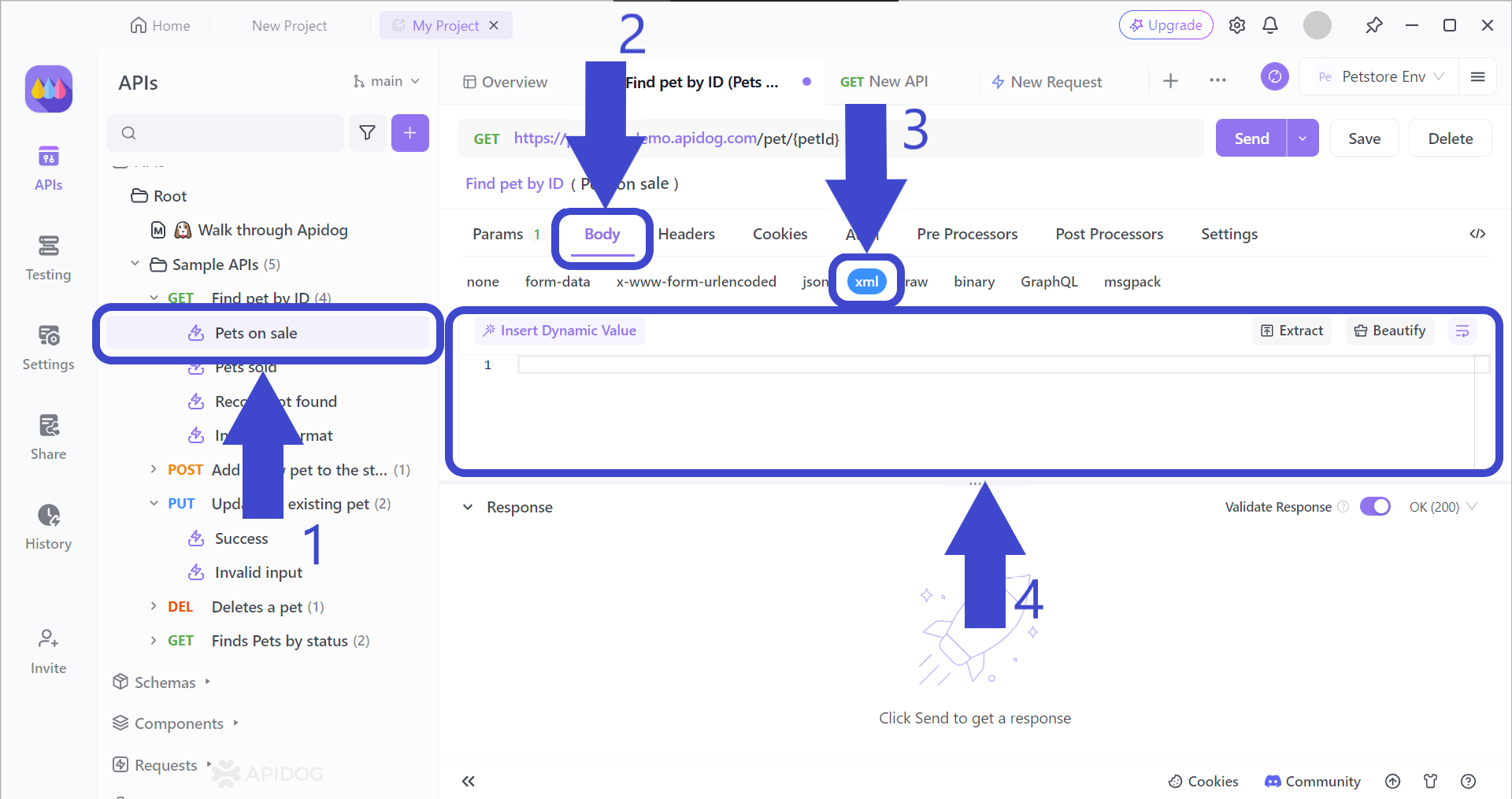
If you have successfully imported the WSDL file, you should see its name on the left side of the screen, as shown around Arrow 1.
To start editing the WSDL file, click Arrows 2 to 4 in ascending order, and you're good to go!
Conclusion
With WSDL files based on XML (eXtensible Markup Language), it is slightly easier to understand. WSDL files may vary in complexity depending on how specific the descriptions and functionalities of the web service are.