Want to level up your coding game with Deepseek V3 right inside Cursor? I recently set up Deepseek V3 on my windows computer and let me tell you—it’s like having a coding wizard by your side, whipping up solutions faster than you can say “debug”! In this beginner’s guide, I’ll walk you through setting up Deepseek V3 with Cursor to make coding a breeze, with a simple example: writing a Python function to calculate a factorial. No tech wizardry required—just a sprinkle of curiosity! Ready to team up Deepseek V3 and Cursor for some coding magic? Let’s get started!
What is Deepseek V3 with Cursor?
Deepseek V3 is a powerful open-source AI model by DeepSeek AI. It’s a Mixture-of-Experts (MoE) model that rivals top models like GPT-4o and Claude 3.5 Sonnet, excelling in coding, math, and reasoning tasks. Cursor is an AI-powered code editor built on VS Code, featuring a chat interface and Composer for seamless code generation. Together, Deepseek V3 and Cursor let you generate code, debug, and refactor with ease. We’ll set it up and write a factorial function to see it in action—let’s roll!
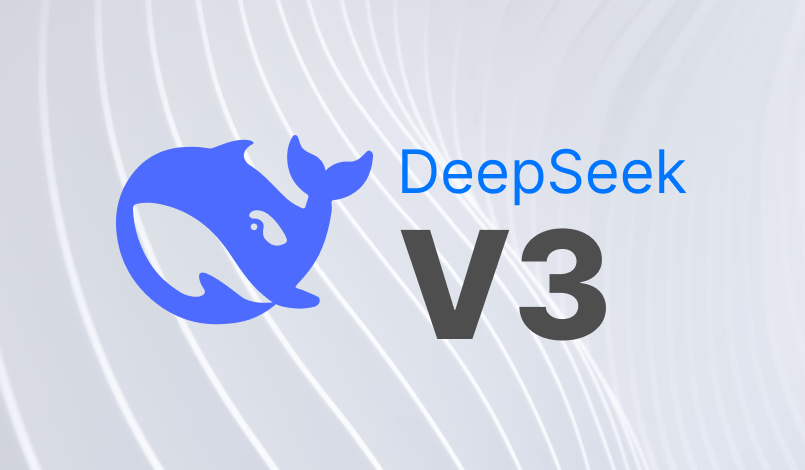
Setting Up Your Environment to Run DeepSeek V3: The Basics
Before we get Deepseek V3 running in Cursor, let’s prep your system. This is totally beginner-friendly, with each step explained so you’re never lost.
Check Prerequisites: Make sure you’ve got these ready:
- Python: Version 3.10 or higher. Run
python --version
in your terminal. If it’s missing, download from python.org. Python is handy for testing scripts. - VS Code: Cursor is built on VS Code, so you’ll need it as a base. Check with
code --version
or install from code.visualstudio.com. - Node.js: Required for Cursor’s dependencies. Verify with
node --version
or get it from nodejs.org.
Missing something? Install it now to keep things smooth.
Install Cursor: Download Cursor from cursor.com for macOS, Windows, or Linux. Install and launch it—it’s a VS Code-like editor with AI superpowers built in.
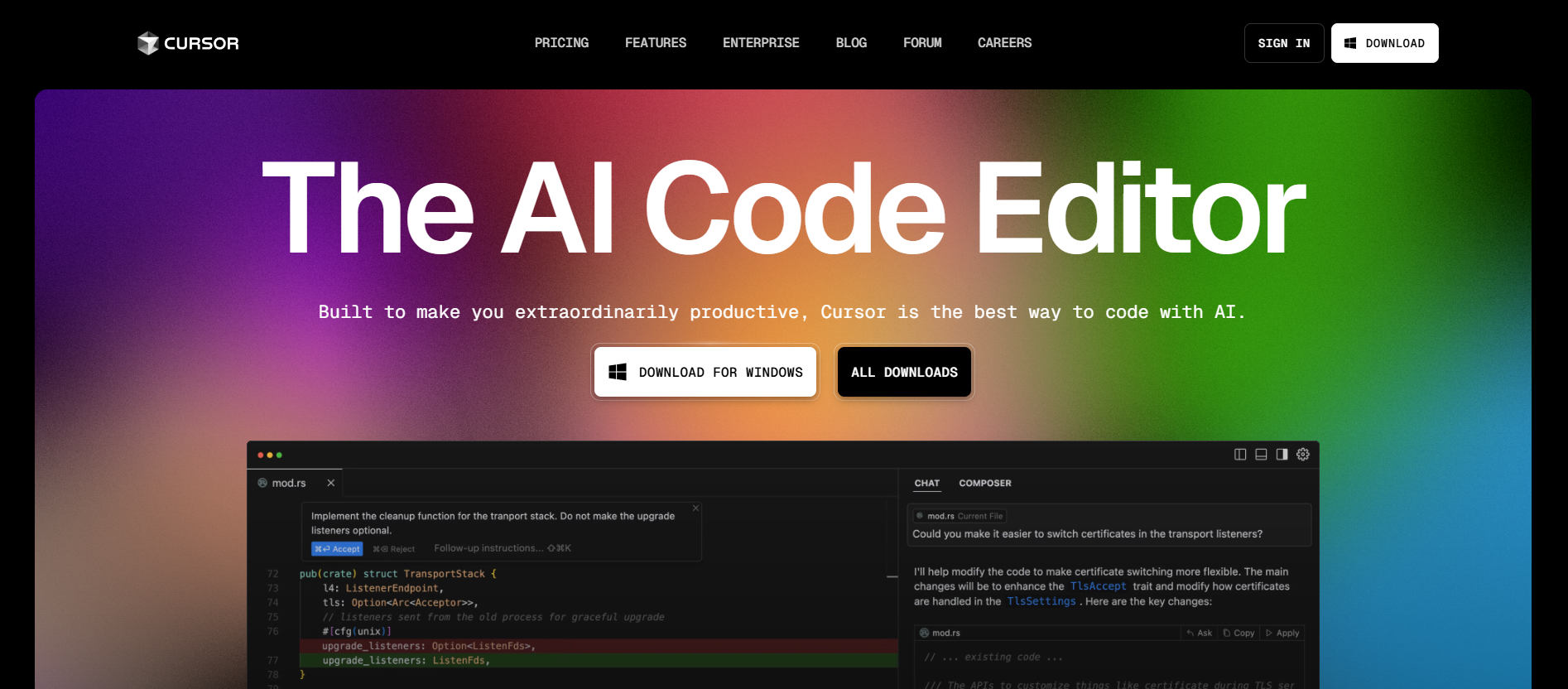
Create a Project Folder: Let’s stay organized:
mkdir deepseek-coding
cd deepseek-coding
This folder will hold your Deepseek V3 projects, and cd
gets you ready for the next steps.
Set Up a Virtual Environment: To keep things tidy, create a Python virtual environment:
python -m venv venv
Activate it:
- Mac/Linux:
source venv/bin/activate
- Windows:
venv\Scripts\activate
Seeing (venv)
in your terminal means you’re in a clean Python environment, avoiding dependency clashes.
Open in Cursor: Launch Cursor with your project folder:
Open Cursor, then use File > Open Folder to select deepseek-coding
. This sets up your workspace for coding with Deepseek V3.
Installing Dependencies
Let’s get your environment ready for Deepseek V3. Since Cursor handles most of the heavy lifting, we just need a few basics for testing.
Install Python Dependencies (optional): While our test is simple, let’s install a dependency for future Deepseek V3 projects:
pip install requests
The requests
library is useful for API-based tasks, though our factorial example won’t need it. This ensures your setup is ready for more complex coding later.
Verify Cursor Installation: Open Cursor and ensure it’s running smoothly. You should see the chat sidebar (Ctrl+L) and Composer (Ctrl+I). If not, restart Cursor or reinstall from cursor.com.
Configuring Deepseek V3 with Cursor
Good news—Cursor directly supports Deepseek V3 as of version 0.44 and higher (we’re on 0.45 in April 2025). We just need to enable it in settings and grab an API key if you’re using a custom setup. Let’s get Deepseek V3 ready to roll!
Check Cursor Version: Ensure you’re on Cursor version 0.44 or 0.45, where Deepseek V3 is supported:
- Go to Help > About in Cursor.
- If you’re on an older version, update Cursor via the app or download the latest from cursor.com.
Enable Deepseek V3 in Cursor:
- Open Cursor and go to Settings (
Ctrl+,
orCmd+,
on Mac). - Navigate to the “Models” section, where you can select available AI models.
- Find
deepseek-v3
in the list and enable it by clicking the toggle or selecting it. Cursor hosts Deepseek V3 on US servers (via Fireworks.ai), so no external API key is needed for the default setup. - Save the settings. To verify, open the Chat UI (Ctrl+L), switch the model to
deepseek-v3
, and type “Hello”—it should respond. This direct integration makes using Deepseek V3 in Cursor a breeze!
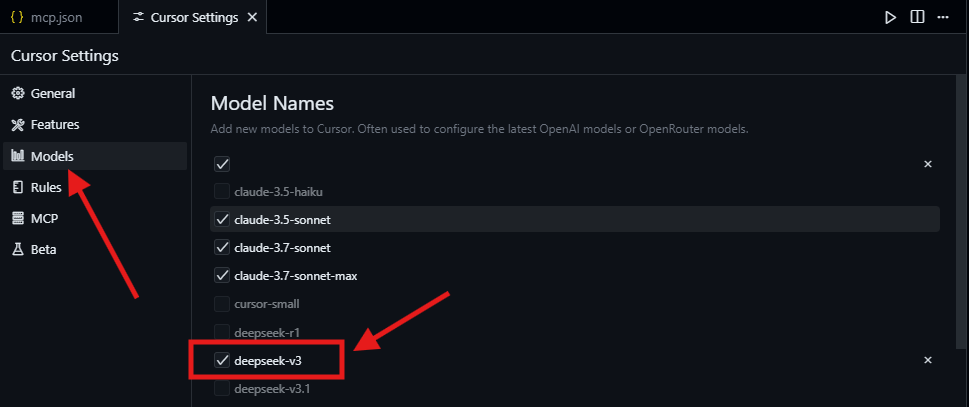
Optional: Use a Custom Deepseek API Key:
- If you prefer using the official Deepseek V3 API (e.g., for better performance), you can set it up via OpenRouter. However, DeepSeek has restricted sign-ups outside mainland China due to security issues, so this might not be an option for everyone (feel free to give it a try).
- Instead, you can use a local setup with Ollama (as noted in some guides). Install Ollama, pull the
deepseek-v3
model, and configure Cursor to usehttp://localhost:11434/v1
as the OpenAI Base URL with a placeholder API key. For simplicity, we’ll stick with Cursor’s hosted version here.
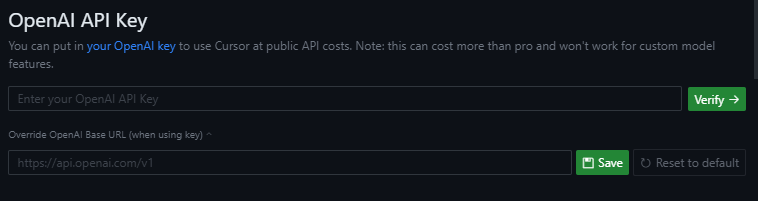
Understand Deepseek V3 Pricing:
- Cursor’s default Deepseek V3 usage is covered under its pricing: the Pro Plan ($20/month) includes 500 “Fast Use” credits, switching to unlimited “Slow Use” after that. Deepseek V3 is considered a non-premium model, so it’s FREE for use.
- If using the official DeepSeek API (via OpenRouter or direct), DeepSeek V2.5 is priced at $0.27 per 1M tokens, and Deepseek V3 may be similar. A typical prompt (e.g., 500 input tokens, 200 output) would cost ~$0.000189 ($0.27/1M * 700). Check your Cursor plan or OpenRouter dashboard for exact usage.
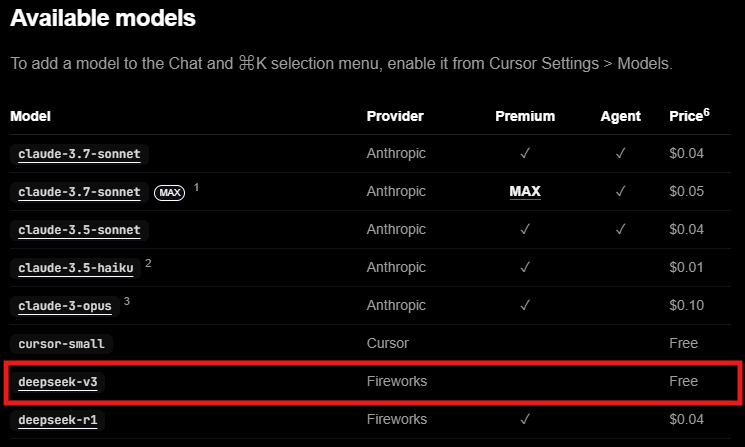
Testing Deepseek V3 in Cursor
Let’s test Deepseek V3 in Cursor with a simple task: “Write a Python function to calculate the factorial of a number.” This keeps things straightforward, showcasing Deepseek V3’s coding skills without complex steps.
Test in Cursor Chat:
- Open Cursor, go to the Chat UI (Ctrl+L), and ensure
deepseek-v3
is selected as the model. - Type: “Write a Python function to calculate the factorial of a number.”
- Deepseek V3 will respond with something like:
def factorial(n):
if n < 0:
raise ValueError("Factorial is not defined for negative numbers")
elif n == 0 or n == 1:
return 1
else:
result = 1
for i in range(2, n + 1):
result *= i
return result
- Add a test line to verify:
print(factorial(5)) # Outputs: 120
- Copy the code into a new file in Cursor (e.g.,
factorial.py
), and run it by clicking the “Run” button or pressingCtrl+Enter
. I got120
(5! = 5 * 4 * 3 * 2 * 1)—nailed it! If it fails, check your model selection or internet connection.
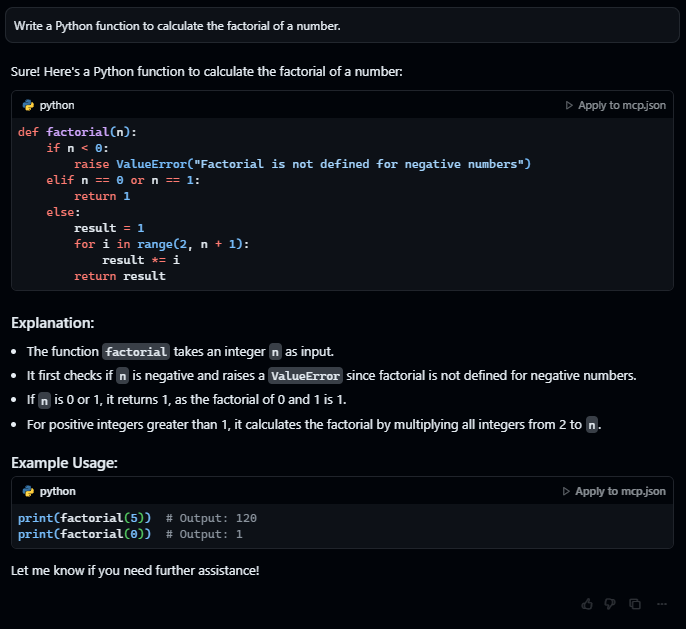
Test in Cursor Composer:
- Open Composer (Ctrl+I) and type the same prompt: “Write a Python function to calculate the factorial of a number.”
- Deepseek V3 will generate the same function. Click “Accept” to add it to your current file.
- Add the test line and run it again—I got
120
once more. If Composer doesn’t work, ensure Deepseek V3 is selected and your Cursor credits aren’t depleted (switch to “Slow Use” if needed).
However, take note that:
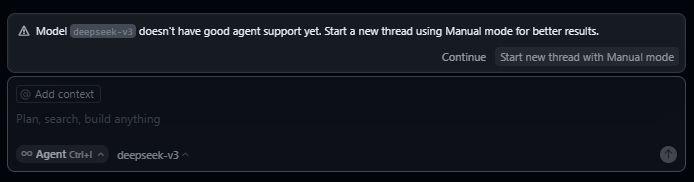
But non the less, you could still try it out on simpler tasks!
Understand the Test Results: The factorial function is a great test—it’s simple but shows Deepseek V3’s ability to generate correct, recursive code. The output 120
confirms it understood the task perfectly. If you see errors, ensure Cursor is using Deepseek V3 and your setup matches the steps above.
Tips for Using Deepseek V3 Effectively
To make the most of Deepseek V3 in Cursor:
- Clear Prompts: “Write a Python function to calculate the factorial of a number” works better than “Do math.” Specificity helps Deepseek V3 deliver spot-on code.
- Use Composer for Edits: For refining code, use Composer (Ctrl+I) to iterate—Deepseek V3 can show diffs and improve your code seamlessly.
- Monitor Usage: If on Cursor’s Pro Plan, track your 500 “Fast Use” credits to avoid switching to “Slow Use” during peak times.
- Explore Chat UI: Use the Chat UI (Ctrl+L) for quick questions or brainstorming before coding in Composer.
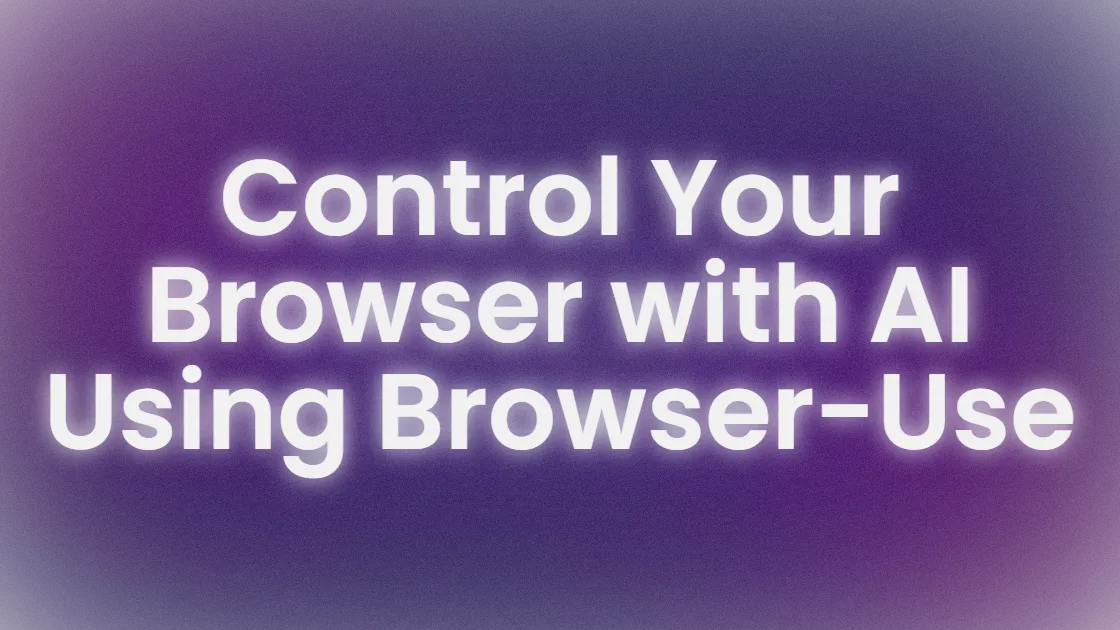
My Takes on Deepseek V3 with Cursor
After testing Deepseek V3, here’s my vibe:
- Super Fast: Deepseek V3 generated the factorial function in seconds, with perfect code.
- Cursor’s Magic: The Chat UI and Composer make coding feel effortless, and direct Deepseek V3 support is a win.
- Cost-Effective: As a non-premium model in Cursor, Deepseek V3 saves on API costs compared to pricier models.
- Minor Hiccups: Some users note Deepseek V3’s 64k token limit can be a constraint for large projects—keep prompts concise.
If you hit issues, double-check your model selection or update Cursor.
Wrapping Up: Your Deepseek V3 Coding Adventure
You’ve just unlocked Deepseek V3 in Cursor, turning your coding sessions into AI-powered awesomeness! From coding a factorial function to tackling bigger projects, you’re ready to roll. Try generating a web scraper or debugging code next and share your wins. For more, check out Cursor’s community forums, and keep coding with Deepseek V3 and Cursor!