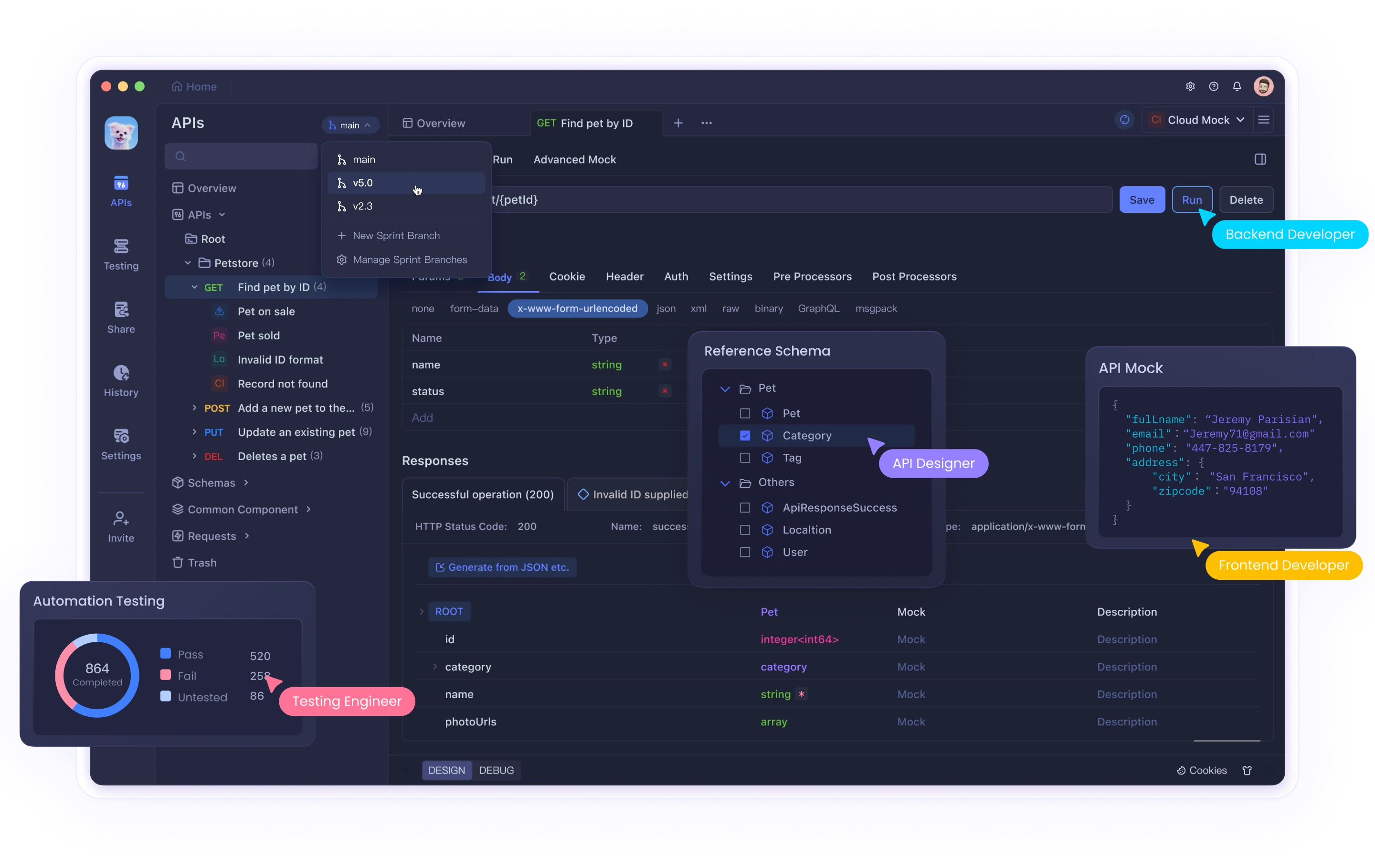
In 2025, many developers and data enthusiasts are looking for robust ways to integrate powerful search or data processing APIs into their applications without incurring steep costs. Deepseek API offers an innovative approach to unlocking advanced data search capabilities and insights. In this guide, we will explore how to make the most of the Deepseek API key for free in 2025. Whether you’re a beginner or a seasoned developer, we will walk you through three distinct methods, each with detailed steps and sample code, so you can choose the option that best fits your needs.
By leveraging these methods, you can experiment and prototype seamlessly, build upon open-source projects, or even deploy serverless functions that interact with the Deepseek API. Let’s break down the different options below.
Introduction
Deepseek API is designed to offer state-of-the-art search and data extraction capabilities that are crucial for building modern applications. For 2025, many providers are focusing on making these APIs accessible, even for developers who might have budget constraints or are experimenting with new ideas. The free methods provided by Deepseek encourage innovation and allow you to integrate high-quality search functionality without upfront investment.
In this article, you will learn:
- How to sign up and obtain an API key using the official Deepseek free trial.
- Ways to integrate the Deepseek API key into an open source project with minimal configuration.
- How to deploy serverless functions on a free cloud platform to harness the Deepseek API in production-like environments.
Each option is broken down into detailed steps and illustrated with sample code so you can implement the solutions right away. Whether you prefer coding in Python, Node.js, or another environment, you'll find a method that suits your workflow.
Option 1: Using the Official Deepseek Free Trial Account
One of the simplest ways to work with the Deepseek API key for free in 2025 is to use the official free trial version provided by Deepseek. This option is perfect for those who want to quickly experiment with the API without any setup overhead aside from creating an account.
Step-by-Step Guide for Option 1
Create Your Account
- Navigate to the Deepseek website.
- Click on the “Sign Up” or “Free Trial” button.
- Complete the registration form using your email address and other required details.
- Verify your email address by clicking on the verification link sent to you.
Obtain Your API Key
- Once your account is activated, log in to your dashboard.
- Locate the section named “API Keys” or “Integrations.”
- Copy the provided API key which will be your gateway to accessing Deepseek features.
Review Documentation
- It is a good idea to review any available documentation on your dashboard. This will provide you with endpoints, rate limits, and usage examples.
- Familiarize yourself with how the authentication and query parameters work.
Start Experimenting with the API
- Use the API key in your code by including it in your HTTP request headers.
- Experiment with querying the Deepseek API using different parameters to understand the data it returns.
Code Setup and Testing
- Prepare your development environment with your favorite language and tools.
- Write some test code to make a GET or POST request to a Deepseek endpoint.
- Review the results and iterate on your query designs.
Sample Code (Python) for Option 1
Below is a sample Python script that demonstrates how to use the Deepseek API key in a simple GET request:
import requests
# Replace with your actual Deepseek API key
API_KEY = "YOUR_DEEPSEEK_API_KEY"
# API endpoint for the search or data extraction
API_URL = "https://api.deepseek.ai/v1/search"
# Parameters for the API call
params = {
"query": "latest technology trends", # Your custom search query
"limit": 10 # Number of results to retrieve
}
# Headers including authorization information
headers = {
"Authorization": f"Bearer {API_KEY}"
}
def main():
try:
# Send a GET request to the Deepseek API endpoint
response = requests.get(API_URL, headers=headers, params=params)
response.raise_for_status()
# Retrieve and display JSON data from the API response
results = response.json()
print("Search results:", results)
except requests.exceptions.HTTPError as errh:
print("HTTP Error:", errh)
except requests.exceptions.ConnectionError as errc:
print("Connection Error:", errc)
except requests.exceptions.Timeout as errt:
print("Timeout Error:", errt)
except requests.exceptions.RequestException as err:
print("Request Exception:", err)
if __name__ == '__main__':
main()
This code demonstrates the essential steps: setting up your API key, forming a query, and handling the HTTP response. You can further modify the code to meet your specific requirements or integrate it into a larger project.
Option 2: Integrating with an Open Source Project
For developers who are working on open-source projects, there’s an alternative approach: integrating the Deepseek API with an existing framework or project. This option allows you to build upon community-driven code bases while taking advantage of the free API key.
Step-by-Step Guide for Option 2
Fork or Clone an Open Source Repository
- Identify and fork a project that could greatly benefit from advanced search capabilities.
Clone the repository to your local development environment:
git clone https://github.com/username/open-source-project.git
cd open-source-project
Locate the Configuration File or Module
- Search within the project for configuration files (like
.env
orconfig.js
) where API keys and credentials are stored. - This is usually where you can set environmental variables for external APIs.
Insert Your Deepseek API Key
Add your Deepseek API key to the configuration file. For instance, if you are using a .env
file, add the following line:
DEEPSEEK_API_KEY=YOUR_DEEPSEEK_API_KEY
Modify the Code to Make API Calls
- Find in the code base where external data is retrieved.
- Insert the logic to call the Deepseek API. This may involve integrating additional API endpoints or modifying existing functions.
Test the Integration Locally
- Run the project locally to ensure that the new API integration works as expected.
- Debug any issues and validate that data is being correctly fetched from Deepseek.
Contribute Back
- If your integration improves the project, consider contributing your changes back to the main repository as a pull request.
- This collaborative approach benefits both your own project and the open source community at large.
Sample Code (Node.js) for Option 2
Below is a simple Node.js example that demonstrates how to utilize the Deepseek API within an open source project setting. This code snippet assumes you are using the node-fetch
package to handle HTTP requests:
const fetch = require('node-fetch');
// Load your environmental variables (using dotenv for example)
require('dotenv').config();
const API_KEY = process.env.DEEPSEEK_API_KEY;
const API_URL = 'https://api.deepseek.ai/v1/search';
// Function to execute a search query using Deepseek API
async function deepseekSearch(query) {
const url = `${API_URL}?query=${encodeURIComponent(query)}&limit=10`;
try {
const response = await fetch(url, {
headers: {
'Authorization': `Bearer ${API_KEY}`
}
});
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const data = await response.json();
console.log("Search results:", data);
} catch (error) {
console.error("Error fetching data from Deepseek:", error);
}
}
// Example usage
deepseekSearch('open source search integration');
In this example, the API key is stored in an environmental variable, and a search query is executed by calling deepseekSearch
. This approach allows you to incorporate the API seamlessly into projects that are built with Node.js.
Option 3: Serverless Deployment on a Free Cloud Platform
For developers looking to scale their applications or create microservices without managing infrastructure, serverless deployment is an appealing option. Many free cloud platforms support serverless functions, making it possible to call the Deepseek API without incurring significant costs. This option is ideal for developers who want to integrate the API in production-like environments with minimal maintenance overhead.
Step-by-Step Guide for Option 3
Set Up Your Cloud Environment
- Sign up for a free tier account on a cloud platform (e.g., AWS, Google Cloud, or Azure).
- Create a new project or serverless function service following the platform’s documentation.
- Make sure your account settings allow you to deploy functions without exceeding the free tier quota.
Configure Environment Variables
- In your cloud console, set up environment variables for your function.
- Add your DEEPSEEK API key as an environment variable (e.g., DEEPSEEK_API_KEY). This ensures your sensitive credentials remain secure and separate from your code.
Create a New Serverless Function
- Follow the instructions to create a new function.
- Choose your preferred runtime (Node.js is popular for serverless functions).
Implement the Function to Call Deepseek API
- Write the function’s code so that it receives a request, calls the Deepseek API using your API key, and returns the resulting data.
- Ensure proper error handling and logging are in place.
Deploy and Test the Function
- Deploy the function using the platform’s deployment tools.
- Test it by triggering the endpoint (e.g., via the browser or Postman) to ensure it calls Deepseek correctly and handles responses appropriately.
Sample Code (AWS Lambda using Node.js) for Option 3
Below is an example AWS Lambda function written in Node.js that utilizes the Deepseek API:
// Import axios for making HTTP requests
const axios = require('axios');
// The Lambda handler function
exports.handler = async (event) => {
// Retrieve the API key from environment variables
const apiKey = process.env.DEEPSEEK_API_KEY;
// Extracting query parameters from the event
const query = event.queryStringParameters && event.queryStringParameters.query ? event.queryStringParameters.query : 'default query';
// Construct the Deepseek API URL with encoded query parameters
const url = `https://api.deepseek.ai/v1/search?query=${encodeURIComponent(query)}&limit=10`;
try {
// Make the API call using axios
const response = await axios.get(url, {
headers: {
'Authorization': `Bearer ${apiKey}`
}
});
// Return a successful response with the data received from Deepseek
return {
statusCode: 200,
body: JSON.stringify(response.data),
headers: {
'Content-Type': 'application/json'
}
};
} catch (error) {
console.error("Deepseek API error:", error);
// Return an error response in case of failure
return {
statusCode: 500,
body: JSON.stringify({ message: 'Unable to retrieve data from Deepseek API.' }),
headers: {
'Content-Type': 'application/json'
}
};
}
};
Explanation of the Code
- Importing Axios:
The code importsaxios
for handling HTTP requests in a concise manner. Ensure you include theaxios
package in your deployment package. - Environment Variables:
The Deepseek API key is retrieved from the Lambda’s environment variables usingprocess.env.DEEPSEEK_API_KEY
. This keeps your key secure and makes your code cleaner. - API Request:
It constructs the URL by encoding the query parameter and includes the API key in the request headers. - Error Handling:
Proper error handling is implemented to catch issues during the API call and return a suitable HTTP response.
By deploying a serverless function like this, you can easily integrate Deepseek API capabilities into your applications in a scalable and cost-effective manner. This option is especially effective when you want to decouple your application logic from the backend infrastructure.
Conclusion
In this guide, we explored three distinct methods to use the Deepseek API key for free in 2025. Each option provides a unique set of advantages:
- Option 1: Using the Official Free Trial Account
This method is perfect for quickly getting started with the API. It enables you to sign up, obtain your API key, and experiment with code examples in a straightforward manner. The emphasis is on speed and ease of setup, ideal for those who want to prototype or learn directly from official documentation. - Option 2: Integration with an Open Source Project
This option offers greater flexibility and the opportunity to contribute to community-driven projects. By integrating the Deepseek API key into an existing open source code base, you can enhance your project with powerful search functionalities while learning from real-world examples. This method is particularly suited for developers who enjoy collaboration and contributing improvements back to the community. - Option 3: Serverless Deployment on a Free Cloud Platform
For those aiming to build production-like environments or deploy microservices quickly, serverless deployment is ideal. By using a free cloud platform, you can set up scalable functions without managing server infrastructure. This option also demonstrates how the latest cloud technologies can be leveraged to deliver robust applications powered by modern APIs.
Each option described in this article is designed to meet different needs and skill levels. Whether you prefer working in Python, Node.js, or a serverless environment, there is an option available that can help you integrate Deepseek seamlessly into your projects. The provided sample codes are meant to serve as starting points – feel free to adapt and expand them based on your unique requirements.
As you embark on your journey using Deepseek API in 2025, remember that experimenting with different integration approaches not only broadens your technical skills but also ensures that your applications remain flexible and future-proof. Enjoy the process of discovery, keep iterating on your code, and embrace the wide range of possibilities that modern APIs and cloud platforms offer.
Happy coding and may your applications be ever-evolving and innovative!