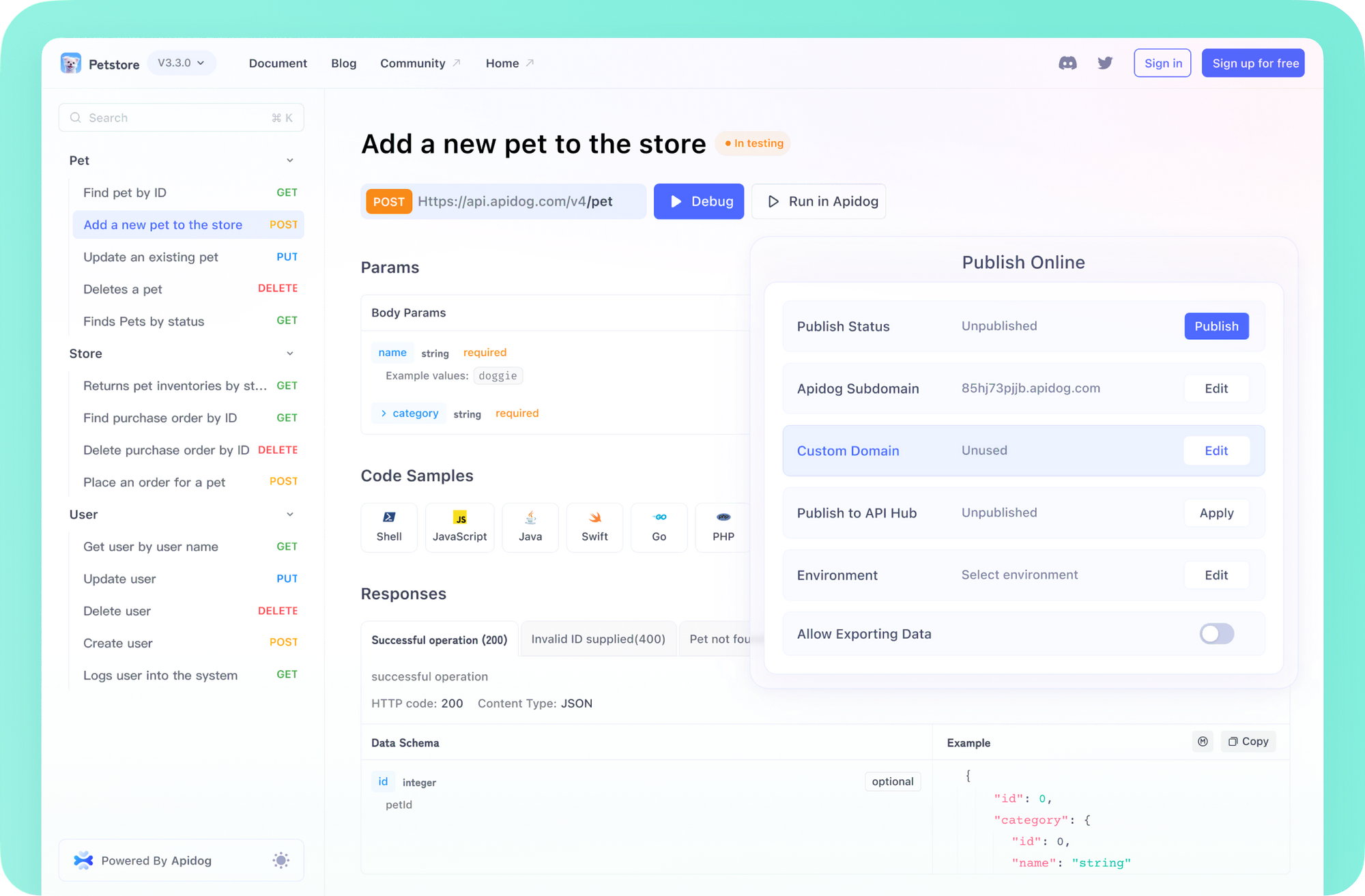
Introduction to the Datadog API
Datadog's API provides programmatic access to the platform's robust monitoring and analytics capabilities. This RESTful API allows developers to send data, build visualizations, and manage their Datadog accounts through code. Whether you're integrating custom applications, automating workflows, or extending Datadog's functionality, understanding how to leverage the API is essential for maximizing the platform's potential.
The Datadog API is designed with a resource-oriented architecture that uses standard HTTP response codes, accepts and returns JSON in all requests, and utilizes standard HTTP methods. This makes it intuitive for developers familiar with RESTful web services. The API's comprehensive functionality enables both read and write operations, allowing you to not only retrieve monitoring data but also configure various aspects of your Datadog environment.
By mastering the Datadog API, you'll be able to:
- Programmatically create and manage dashboards, monitors, and alerts
- Submit custom metrics from any application or infrastructure component
- Automate incident management workflows
- Integrate with CI/CD pipelines for continuous monitoring
- Build custom tools and solutions around your monitoring data
Getting Started with the Datadog API Authentication
Before making any API calls, you'll need to properly set up authentication to ensure secure access to your Datadog resources.
Obtaining Datadog API and Application Keys
To start using the Datadog API, you'll need two types of keys:
- API Key: This identifies your Datadog account and is required for all API requests.
- Navigate to your Datadog account: Organization Settings > API Keys
- Click "New Key" to create a new API key
- Give it a meaningful name that indicates its purpose and usage
- Store the key securely as it grants access to your Datadog account
- Application Key: Required for many management endpoints, this provides additional authentication and specifies access permissions.
- Navigate to Organization Settings > Application Keys
- Click "New Key" to generate an application key
- Name it appropriately based on its intended use
- Optionally, restrict its access to specific Datadog applications
Setting Up Datadog API Authentication Headers
When making API requests, you'll need to include these keys as headers:
- Include your API key in the header using
DD-API-KEY: your_api_key_here
- For endpoints requiring additional authentication, include
DD-APPLICATION-KEY: your_application_key_here
Here's an example of a basic authenticated request:
curl -X GET "<https://api.datadoghq.com/api/v1/dashboard>" \\\\
-H "Content-Type: application/json" \\\\
-H "DD-API-KEY: your_api_key_here" \\\\
-H "DD-APPLICATION-KEY: your_app_key_here"
Managing Datadog API Key Security
Given the significant access these keys provide, following security best practices is crucial:
- Rotate keys regularly, especially API keys
- Set appropriate permissions for application keys
- Never hardcode keys in your application source code
- Use environment variables or secure vaults to store keys
- Audit API key usage periodically
- Revoke unused or potentially compromised keys immediately
Core Datadog API Concepts
Understanding the foundational concepts of the Datadog API will help you navigate its extensive capabilities more effectively.
Datadog API Endpoints Structure
The Datadog API is logically organized into functional areas that mirror the platform's capabilities:
- Metrics API: For submitting and querying metrics data, enabling you to send custom metrics or retrieve historical metric values. These endpoints are central to monitoring application and infrastructure performance.
- Events API: Used to post and retrieve events from the Datadog event stream. Events can represent deployments, alerts, or any significant occurrences in your environment.
- Monitors API: Allows programmatic creation and management of monitoring alerts, including configuration of notification settings and downtime scheduling.
- Dashboards API: For building, modifying, and retrieving visualization dashboards, enabling automated dashboard creation based on templates or application needs.
- Logs API: Provides endpoints for sending logs directly to Datadog, configuring log processing pipelines, and managing log archives.
- Synthetics API: For managing synthetic tests, retrieving test results, and scheduling test runs.
- Users & Organizations API: Enables management of team members, permissions, and organization settings.
- Service Level Objectives (SLOs) API: For creating and tracking SLOs to measure service reliability.
Making Your First Datadog API Call
Let's start with a common use case: submitting a custom metric. This example demonstrates how to send a simple gauge metric with tags:
import requests
import time
import json
api_key = "your_api_key_here"
current_time = int(time.time())
payload = {
"series": [
{
"metric": "custom.application.performance",
"points": [[current_time, 100]],
"type": "gauge",
"tags": ["environment:production", "application:web", "region:us-east"]
}
]
}
headers = {
"Content-Type": "application/json",
"DD-API-KEY": api_key
}
response = requests.post("<https://api.datadoghq.com/api/v1/series>",
headers=headers,
data=json.dumps(payload))
print(f"Response Status Code: {response.status_code}")
print(f"Response Body: {response.json()}")
This code snippet:
- Creates a payload with a custom metric named "custom.application.performance"
- Sets the current timestamp and a value of 100
- Adds tags for better organization and filtering
- Sends the data to Datadog's metrics endpoint
- Prints the API response
Understanding Datadog API Response Format
Datadog API responses typically follow a consistent JSON format:
{
"status": "ok",
"errors": [],
"data": {
"id": "abc-123-xyz",
"name": "Example Resource",
"created_at": "2023-06-01T12:00:00.000Z",
"modified_at": "2023-06-02T15:30:00.000Z",
...
}
}
Key fields include:
status
: Indicates the success or failure of the requesterrors
: Contains error messages if the request faileddata
: The actual resource data returned by the API
Common Datadog API Use Cases and Examples
Let's explore practical applications of the Datadog API through detailed examples covering key functionality.
Retrieving Datadog API Dashboard Information
Dashboards are central to Datadog's visualization capabilities. Here's how to retrieve details about a specific dashboard:
curl -X GET "<https://api.datadoghq.com/api/v1/dashboard/dashboard_id>" \\\\
-H "Content-Type: application/json" \\\\
-H "DD-API-KEY: your_api_key_here" \\\\
-H "DD-APPLICATION-KEY: your_app_key_here"
To create a new dashboard programmatically:
import requests
import json
api_key = "your_api_key_here"
app_key = "your_app_key_here"
dashboard_payload = {
"title": "API Generated Dashboard",
"description": "Created via the Datadog API",
"widgets": [
{
"definition": {
"type": "timeseries",
"requests": [
{
"q": "avg:system.cpu.user{*} by {host}",
"display_type": "line"
}
],
"title": "CPU Usage by Host"
}
}
],
"layout_type": "ordered"
}
headers = {
"Content-Type": "application/json",
"DD-API-KEY": api_key,
"DD-APPLICATION-KEY": app_key
}
response = requests.post("<https://api.datadoghq.com/api/v1/dashboard>",
headers=headers,
data=json.dumps(dashboard_payload))
print(f"Dashboard created with ID: {response.json().get('id')}")
Creating a Monitor with the Datadog API
Monitors are essential for proactive alerting. Here's how to create a monitor that alerts when CPU usage exceeds a threshold:
import requests
import json
api_key = "your_api_key_here"
app_key = "your_app_key_here"
monitor_payload = {
"name": "High CPU Usage Alert",
"type": "metric alert",
"query": "avg(last_5m):avg:system.cpu.user{*} > 80",
"message": "CPU usage is above 80% for the last 5 minutes. @slack-alerts-channel @email.address@example.com",
"tags": ["app:web", "env:production", "team:infrastructure"],
"priority": 3,
"options": {
"notify_no_data": True,
"no_data_timeframe": 10,
"new_host_delay": 300,
"evaluation_delay": 60,
"thresholds": {
"critical": 80,
"warning": 70
},
"include_tags": True,
"notify_audit": False,
"require_full_window": False
}
}
headers = {
"Content-Type": "application/json",
"DD-API-KEY": api_key,
"DD-APPLICATION-KEY": app_key
}
response = requests.post("<https://api.datadoghq.com/api/v1/monitor>",
headers=headers,
data=json.dumps(monitor_payload))
print(f"Response Status: {response.status_code}")
print(f"Monitor created: {response.json()}")
This example:
- Creates a metric alert monitor
- Sets thresholds for warning (70%) and critical (80%)
- Includes notification settings with mentions for Slack and email
- Adds detailed configuration options like evaluation delay and new host delay
Integrating with AWS using the Datadog API
Connecting Datadog with cloud services extends its monitoring capabilities. Here's how to create an AWS integration:
curl -X POST "<https://api.datadoghq.com/api/v1/integration/aws>" \\\\
-H "Content-Type: application/json" \\\\
-H "DD-API-KEY: your_api_key_here" \\\\
-H "DD-APPLICATION-KEY: your_app_key_here" \\\\
-d '{
"account_id": "your_aws_account_id",
"role_name": "DatadogAWSIntegrationRole",
"access_key_id": "your_access_key",
"secret_access_key": "your_secret_key",
"filter_tags": ["env:production", "service:critical"],
"host_tags": ["account:main", "region:us-east-1"],
"account_specific_namespace_rules": {
"auto_scaling": true,
"opsworks": false,
"elasticache": true
},
"excluded_regions": ["us-west-2", "ca-central-1"]
}'
This integration setup:
- Connects to your AWS account using either a role or access keys
- Configures filtering to focus on specific resources
- Automatically applies tags to monitored hosts
- Enables specific AWS service namespaces
- Excludes regions you don't want to monitor
Sending Logs via the Datadog API
Logs provide critical contextual information for troubleshooting. Here's how to send logs directly to Datadog:
import requests
import json
import datetime
api_key = "your_api_key_here"
logs_payload = [{
"ddsource": "python",
"ddtags": "env:production,service:payment-processor,version:1.2.3",
"hostname": "payment-service-01",
"message": "Payment transaction completed successfully for order #12345",
"service": "payment-service",
"status": "info",
"timestamp": datetime.datetime.now().isoformat(),
"attributes": {
"transaction_id": "tx_789012345",
"amount": 99.95,
"currency": "USD",
"customer_id": "cust_123456",
"payment_method": "credit_card"
}
}]
headers = {
"Content-Type": "application/json",
"DD-API-KEY": api_key
}
response = requests.post("<https://http-intake.logs.datadoghq.com/v1/input>",
headers=headers,
data=json.dumps(logs_payload))
print(f"Log submission response: {response.status_code}")
This example:
- Sends a structured log with rich metadata
- Includes source, service, and environment information
- Adds custom attributes for the specific business context
- Uses tags for better filtering and correlation
Working with Datadog API Rate Limits
Datadog enforces rate limits to ensure platform stability and fair usage across customers. Understanding and respecting these limits is crucial for reliable API integration.
Understanding Datadog API Rate Limiting
Different endpoints have different rate limits based on their resource intensity and typical usage patterns:
- Read operations generally have higher limits than write operations
- Some endpoints may have per-organization limits while others have per-key limits
- API keys shared across multiple applications may hit limits faster
Monitoring Datadog API Rate Limit Headers
When making API requests, check these response headers to understand your current rate limit status:
X-RateLimit-Limit
: The maximum number of requests allowed in the rate limit windowX-RateLimit-Remaining
: The number of requests remaining in the current rate limit windowX-RateLimit-Reset
: The time in seconds until the rate limit resetsX-RateLimit-Period
: The length of the rate limit window in seconds
Implementing Rate Limit Handling in Datadog API Calls
Here's a robust implementation for handling rate limits with exponential backoff:
import requests
import time
import random
def make_api_request_with_backoff(url, headers, payload=None, max_retries=5):
retries = 0
while retries < max_retries:
response = requests.post(url, headers=headers, json=payload) if payload else requests.get(url, headers=headers)
if response.status_code == 429: # Too Many Requests
# Extract rate limit information
limit = response.headers.get('X-RateLimit-Limit', 'Unknown')
remaining = response.headers.get('X-RateLimit-Remaining', 'Unknown')
reset = int(response.headers.get('X-RateLimit-Reset', 60))
print(f"Rate limit hit: {remaining}/{limit} requests remaining. Reset in {reset} seconds.")
# Calculate backoff time with jitter
backoff_time = min(2 ** retries + random.uniform(0, 1), reset)
print(f"Backing off for {backoff_time:.2f} seconds")
time.sleep(backoff_time)
retries += 1
else:
return response
raise Exception(f"Failed after {max_retries} retries due to rate limiting")
Using Datadog API Client Libraries
For convenience, Datadog offers official client libraries in multiple languages, simplifying authentication and request formatting.
Python Datadog API Client
The official Python client provides a clean, idiomatic interface to the Datadog API:
pip install datadog-api-client
Here's an example of submitting metrics using the client:
from datadog_api_client import ApiClient, Configuration
from datadog_api_client.v1.api.metrics_api import MetricsApi
from datadog_api_client.v1.model.metrics_payload import MetricsPayload
from datadog_api_client.v1.model.metrics_series import MetricsSeries
from datadog_api_client.v1.model.point import Point
import time
configuration = Configuration()
configuration.api_key["apiKeyAuth"] = "your_api_key_here"
configuration.api_key["appKeyAuth"] = "your_app_key_here"
with ApiClient(configuration) as api_client:
api_instance = MetricsApi(api_client)
body = MetricsPayload(
series=[
MetricsSeries(
metric="application.request.duration",
points=[
Point([int(time.time()), 250.0])
],
type="gauge",
host="web-server-01",
tags=["endpoint:login", "environment:staging"]
)
]
)
response = api_instance.submit_metrics(body=body)
print(f"Metrics submission successful: {response}")
Ruby Datadog API Client
For Ruby applications, the official client library streamlines API interactions:
gem install datadog_api_client -v 2.31.1
Example usage for creating a monitor:
require 'datadog_api_client'
DatadogAPIClient.configure do |config|
config.api_key = 'your_api_key_here'
config.application_key = 'your_app_key_here'
end
api_instance = DatadogAPIClient::V1::MonitorsAPI.new
body = {
'name' => 'API test monitor',
'type' => 'metric alert',
'query' => 'avg(last_5m):avg:system.cpu.user{*} > 75',
'message' => 'CPU usage is high',
'tags' => ['test:api', 'monitor:automated'],
'options' => {
'thresholds' => {
'critical' => 75,
'warning' => 65
}
}
}
begin
result = api_instance.create_monitor(body)
puts "Monitor created successfully with ID: #{result['id']}"
rescue DatadogAPIClient::APIError => e
puts "Error creating monitor: #{e}"
end
Best Practices for Datadog API Usage
Following these guidelines will help you build more reliable, secure, and efficient integrations with the Datadog API.
Securing Your Datadog API Keys
The security of your API keys is paramount:
- Store keys in environment variables or secure vaults, never in code repositories
- Implement key rotation policies and regularly refresh API keys
- Use different API keys for different applications or purposes
- Apply the principle of least privilege by restricting application key permissions
- Use IP allowlisting where possible to limit access to known IP addresses
Using Tags Effectively in Datadog API Calls
Tags are a powerful mechanism for organizing and filtering your Datadog data:
- Design a consistent tagging taxonomy before starting API implementations
- Include environment, service, and version tags in all metrics and logs
- Use hierarchical tags (e.g.,
region:us-east
,availability-zone:us-east-1a
) - Make tags consistent across all your telemetry (metrics, logs, traces)
- Avoid high-cardinality tags in metrics (e.g., unique user IDs)
Implementing Error Handling for Datadog API Requests
Robust error handling ensures your integrations remain reliable:
- Check HTTP status codes and handle different error types appropriately
- Parse error response bodies for detailed error information
- Implement retry logic with exponential backoff for transient errors
- Log failed API calls with sufficient context for debugging
- Set reasonable timeouts to prevent hung requests
def send_to_datadog(endpoint, payload, headers):
try:
response = requests.post(endpoint,
json=payload,
headers=headers,
timeout=10)
response.raise_for_status()
return response.json()
except requests.exceptions.Timeout:
print("Request timed out - Datadog API may be experiencing delays")
except requests.exceptions.HTTPError as e:
if e.response.status_code == 400:
print(f"Bad request: {e.response.json().get('errors', [])}")
elif e.response.status_code == 403:
print("Authentication error - check your API and application keys")
elif e.response.status_code == 429:
print("Rate limit reached - implement backoff and retry")
else:
print(f"HTTP error: {e.response.status_code}")
except requests.exceptions.RequestException as e:
print(f"Request failed: {e}")
return None
Testing in Sandbox Environments with the Datadog API
Before implementing in production:
- Create a dedicated development/staging organization in Datadog
- Use separate API keys for testing and production
- Create test monitors with notifications directed only to the development team
- Simulate load testing to understand rate limit impacts
- Document API usage patterns for future reference
Monitoring Datadog API Usage
Track your API usage to detect issues early:
- Create dashboards to visualize API call volumes and error rates
- Set up monitors for excessive API errors or approaching rate limits
- Implement logging for all API operations with appropriate detail
- Audit API key usage periodically through Datadog's audit logs
- Track the cost impact of custom metrics submitted through the API
Conclusion: Mastering the Datadog API
The Datadog API provides powerful capabilities for extending and customizing your monitoring and analytics platform. By understanding the authentication process, core concepts, and best practices outlined in this guide, you'll be well-equipped to integrate Datadog into your applications and automate your workflows effectively.
Whether you're sending custom metrics, creating monitors, or building complex dashboards, the API offers the flexibility to tailor Datadog to your specific needs. As your usage matures, consider implementing more advanced patterns like:
- Template-based dashboard generation for new services
- Automated monitor maintenance and tuning
- Custom integrations with internal tools and systems
- Scheduled reporting and data extraction workflows
- Synthetic monitoring for critical business processes
The programmatic capabilities provided by the Datadog API enable you to build a monitoring ecosystem that scales with your infrastructure and adapts to your organization's unique requirements.
Remember to check Datadog's official API documentation regularly, as new endpoints and features are frequently added to expand the platform's capabilities. With the knowledge gained from this guide, you're ready to build sophisticated, automated monitoring solutions that leverage the full power of Datadog's observability platform.