The ability to adeptly handle data exchange has become a cornerstone of software development. This blog post offers a deep dive into the mechanics of posting JSON data using C#, a skill that stands at the forefront of modern web development practices.
Structured as a practical guide, this post aims to equip developers with the knowledge and tools necessary to navigate the complexities of data serialization and transmission. By focusing on C#, a language renowned for its robustness and versatility, we provide insights into crafting efficient, secure, and scalable data exchange solutions.
What is JSON?
JSON stands for JavaScript Object Notation. It’s a lightweight data interchange format that’s easy for humans to read and write, and easy for machines to parse and generate. JSON is often used when data is sent from a server to a web page or in various programming environments for data exchange.
Here’s a simple example of JSON data representing a person:
{
"firstName": "John",
"lastName": "Doe",
"age": 30,
"isEmployed": true
}
In this example, data is organized in key-value pairs, with keys like firstName
and lastName
. JSON is text-based and language-independent, so it can be used in many different programming scenarios. It’s particularly popular in web development for transmitting data between a client and a server.
Understanding C#
C# (pronounced “See Sharp”) is a modern, object-oriented, and type-safe programming language developed by Microsoft. It is part of the .NET framework and is designed to build a variety of secure and robust applications, including desktop, web, and mobile apps.
C# is known for its simplicity and power, making it a popular choice among developers. It has roots in the C family of languages, so it’s familiar to those with experience in C, C++, Java, and JavaScript. The language supports many contemporary programming paradigms, such as asynchronous programming, generics, and LINQ (Language Integrated Query).
Here’s a basic example of a “Hello World” program in C#:
using System;
class Program
{
static void Main()
{
Console.WriteLine("Hello, World!");
}
}
This program demonstrates the structure of a C# application, which includes using directives, a class, a Main method, and the use of the Console
class to output text to the console. C# is versatile and continues to evolve, with the latest version being C# 12 as of November 2023.
Why Post JSON Data with C#?
Posting JSON data with C# is common in web development when you need to send data from a client application to a server in a format that’s both easy to understand and process. JSON is a popular choice due to its simplicity and compatibility with many programming languages, including C#.
In C#, you might post JSON data for various reasons:
- Web APIs: Many web services accept and return data in JSON format.
- Cross-platform compatibility: JSON is text-based and language-independent, making it ideal for systems that involve multiple programming languages.
- Data interchange: JSON is great for serializing C# objects to a format that can be stored or transmitted and then deserialized back into objects by the server.
Posting JSON Data with C#
To post JSON data with C#, you can use the HttpClient
class from the .NET
framework. Here’s a step-by-step guide on how to do it:
- Create a C# class that represents the data you want to send.
- Serialize the object to a JSON string using a library like
Newtonsoft.Json
. - Create an instance of
HttpClient
and set up the necessary headers, such asContent-Type: application/json
. - Send the POST request with the JSON data as the content.
Here’s an example code snippet:
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json;
public class MyData
{
public int Id { get; set; }
public string Name { get; set; }
}
public async Task<string> PostJsonDataAsync(string url, MyData data)
{
using (var client = new HttpClient())
{
var json = JsonConvert.SerializeObject(data);
var content = new StringContent(json, Encoding.UTF8, "application/json");
var response = await client.PostAsync(url, content);
if (response.IsSuccessStatusCode)
{
return await response.Content.ReadAsStringAsync();
}
else
{
// Handle the error
return null;
}
}
}
In this code:
MyData
is the class that holds the data you want to send.PostJsonDataAsync
is an asynchronous method that takes a URL and aMyData
object as parameters.JsonConvert.SerializeObject
converts theMyData
object into a JSON string.StringContent
is used to encapsulate the JSON data and set the media type toapplication/json
.HttpClient.PostAsync
sends the POST request to the specified URL with the JSON content.- The response is checked for success, and the content is read if the request was successful.
Remember to include error handling for scenarios where the request may fail, and ensure that any sensitive data is securely transmitted.
How to test JSON Data with c# using Apidog
Apidog is an all-in-one API collaboration platform that provides comprehensive tools for API documentation, API debugging, API mocking, and API automated testing. It’s designed to streamline the API lifecycle by integrating functionalities typically found in separate tools like Postman, Swagger, and JMeter into a single system. This centralization solves the problem of data synchronization across different systems, making the API development process more efficient and consistent.
To post JSON data with C# using Apidog, you can follow these general steps:
Establish a New Project: In your project, initiate a new request
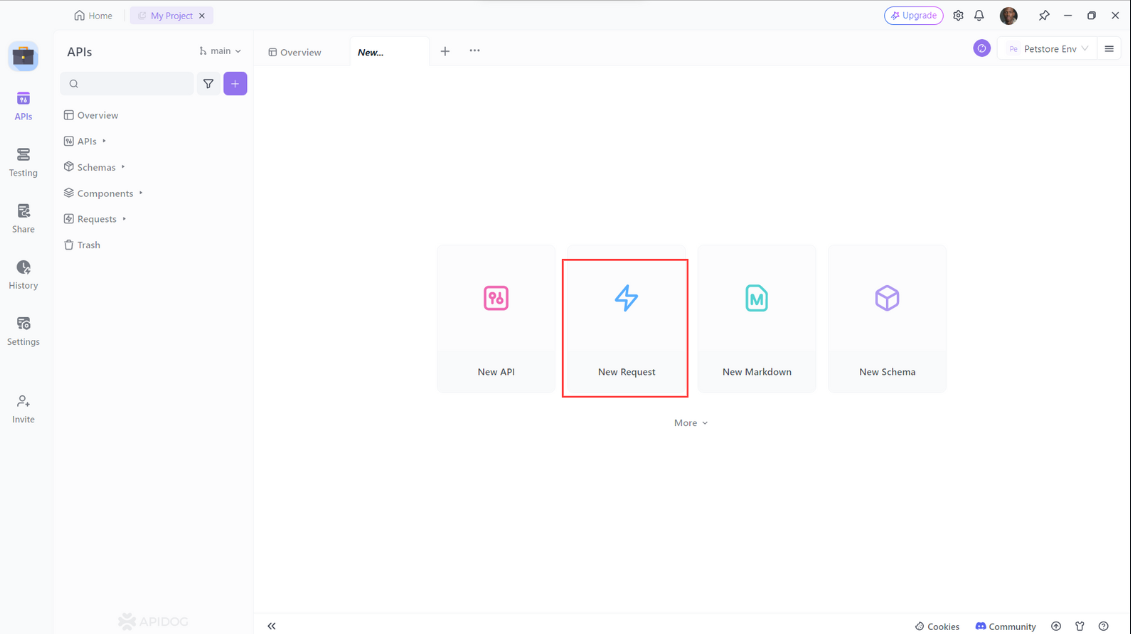
Enter POST Request Details: Select the request type as POST.
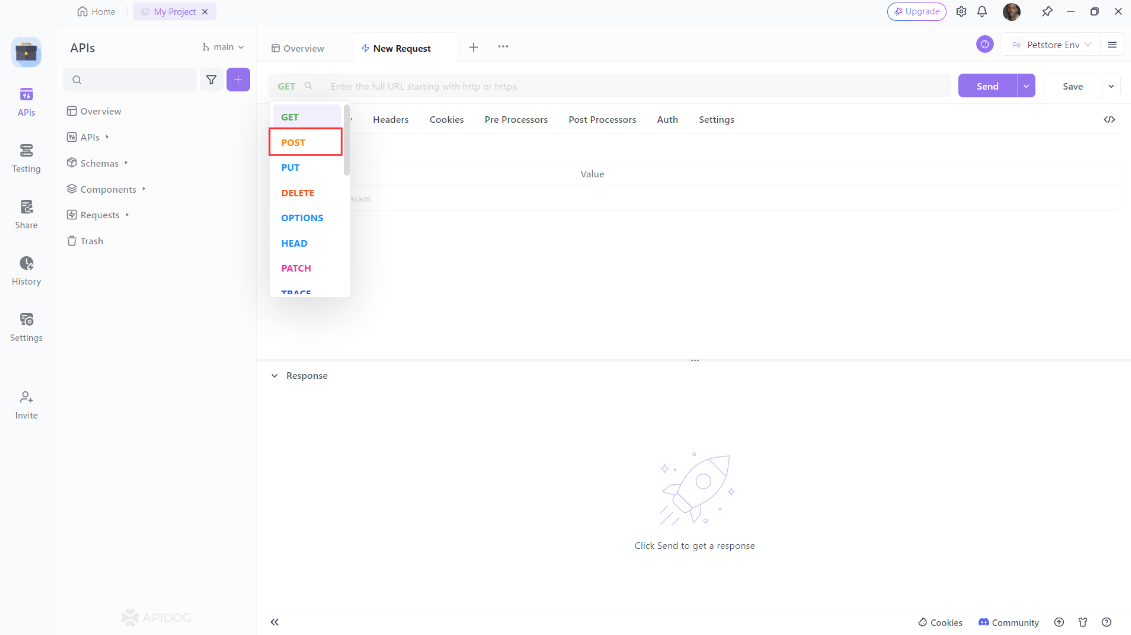
Input JSON Data: Navigate to the “Body” tab in your request setup, choose “json” as the format, and input the JSON data you wish to send.
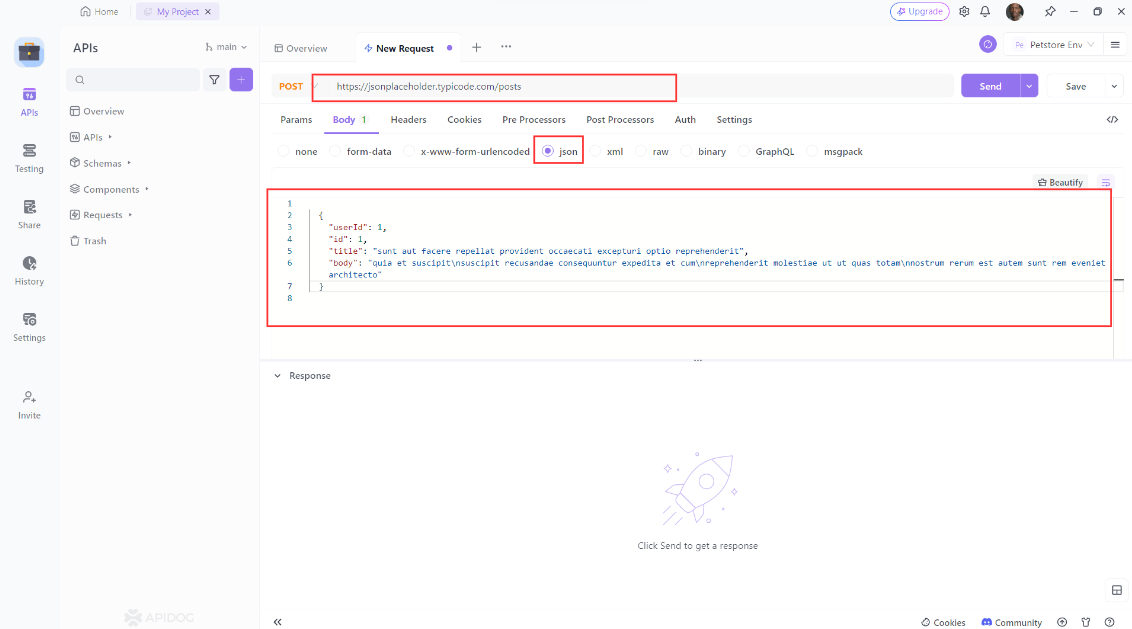
Send Post Request: After setting up your request with the JSON data, you can send the POST request and observe the response from the server.
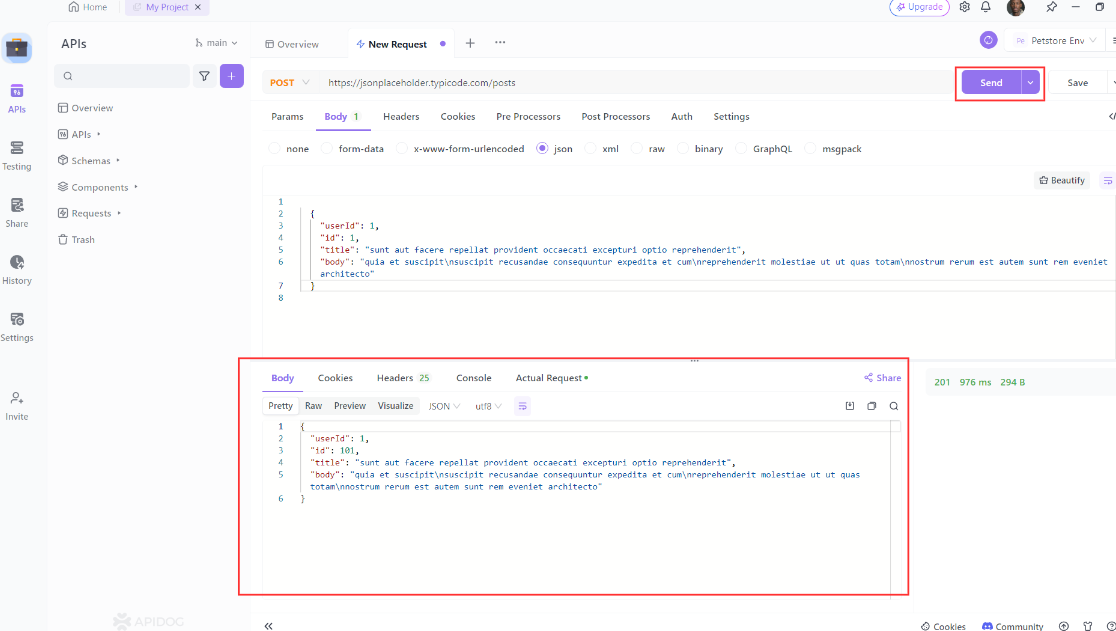
Conclusion
In conclusion, posting JSON data with C# is a common practice in web development for sending structured data from a client to a server. It involves serializing C# objects into JSON format and using the HttpClient
class to send the data as part of an HTTP POST request. This process is essential for creating interactive and dynamic applications that communicate with web services and APIs.
Run your tests within Apidog to ensure your API behaves as expected.