Testing is a very important phase in the life cycle of software, which ensures an application will be able to provide expected performance under continuously changing conditions. From this perspective, Java offers a wide range of testing tools that correspond to various types of testing. Whether you are an experienced developer or just an up-and-coming one, it is worth recognizing these tools, since they may make your life easier and allow enriching your possibilities of testing.
In this blog post, we take an in-depth look at the top 10 Java testing tools every developer should know. We will also introduce Apidog and its recent updates to see how it fits into the landscape.
What is a Java Testing?
Java testing refers to the process of verifying and validating Java applications to ensure they function correctly and meet specified requirements. It involves various techniques and tools to identify bugs, ensure code quality, and improve software reliability. Key aspects of Java testing include:
Unit Testing: Testing individual components or methods in isolation. Frameworks like JUnit and TestNG are commonly used.
Integration Testing: Testing the interaction between different modules or services to ensure they work together as expected.
Automated Testing: Using scripts and tools to automate the testing process, which helps in saving time and increasing coverage.
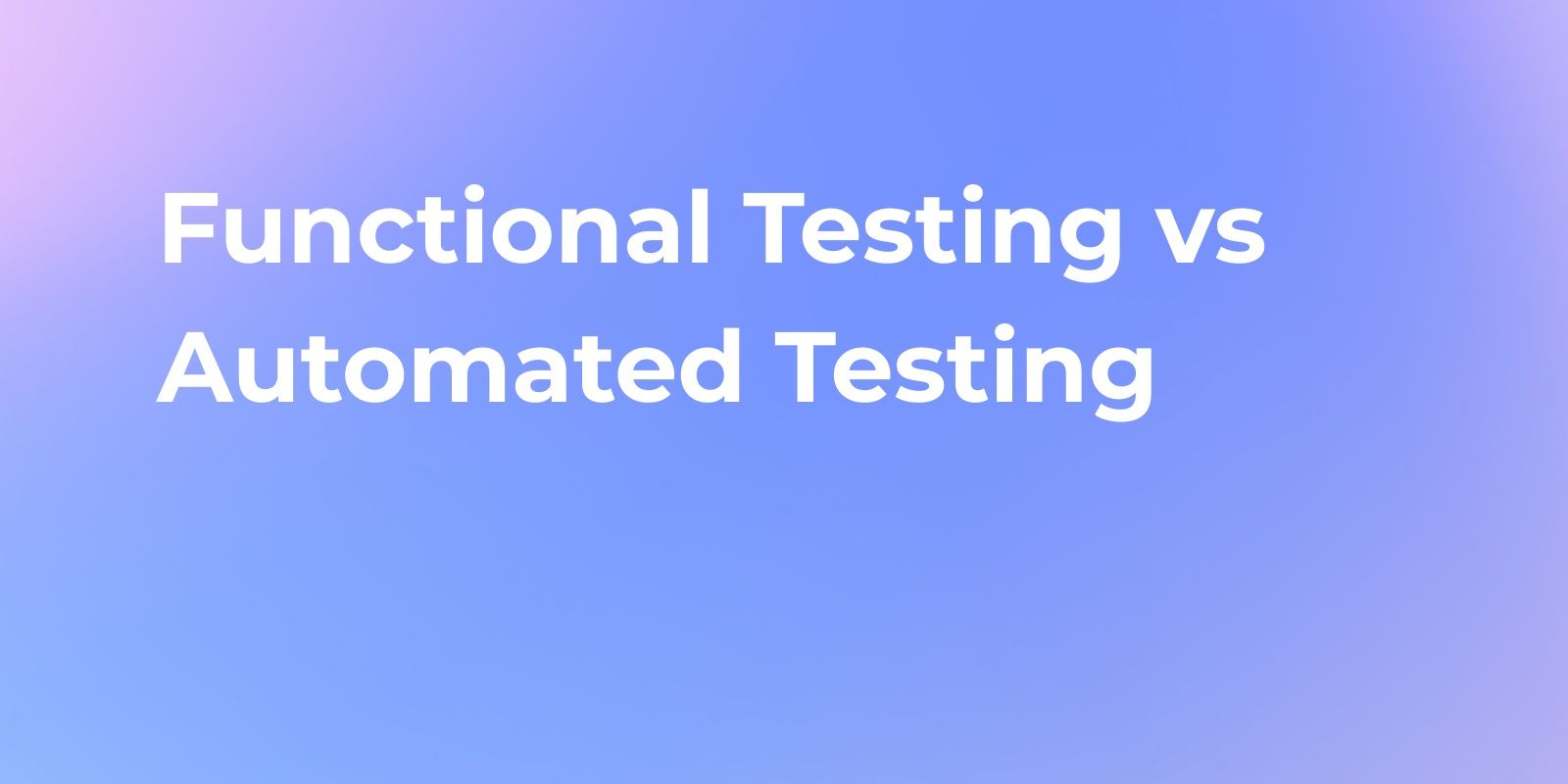
Functional Testing: Validating the software against functional requirements. This can be done using tools like Selenium for web applications.
Performance Testing: Assessing how the application performs under load. Tools like JMeter are often used for this purpose.
Regression Testing: Re-running tests after changes to the codebase to ensure that existing functionality is not broken.
Mocking and Stubbing: Creating mock objects to simulate the behavior of real objects in controlled ways, often used in unit testing.
Java testing is an essential part of the software development lifecycle, ensuring that applications are reliable, maintainable, and meet user expectations. By employing a combination of unit, integration, functional, performance, and regression testing, developers can create robust applications that perform well under various conditions. Utilizing the right tools and following best practices can significantly enhance the effectiveness of the testing process.
1. JUnit
JUnit is arguably the most well-known Java testing framework. As a cornerstone of test-driven development (TDD), JUnit provides a simple and elegant way to write repeatable tests. Its widespread adoption is due to its simplicity and robust support for unit testing.
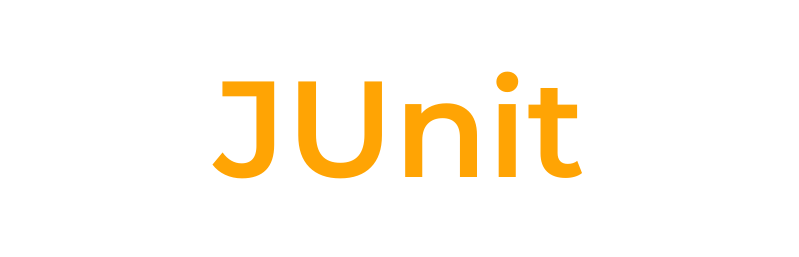
Key Features:
- Annotations for Test Configuration: JUnit uses annotations such as
@Test
,@Before
, and@After
to manage test execution order and setup. - Assertions for Testing Expected Results: With a comprehensive set of assertions, JUnit allows developers to verify that their code behaves as expected.
- Test Runners: JUnit provides various test runners to execute tests, making it easy to integrate with build tools like Maven and Gradle.
- The JUnit platform facilitates launching java testing frameworks on it.
- JUnit Vintage assists in maintaining backward compatibility.
- JUnit enables the creation of a test framework that can define the TestEngine API.
- JUnit Jupiter provides a Test Engine for executing Jupiter-based tests by merging programming and extension models.
Pros:
- Widely used with extensive community support.
- Integrates seamlessly with IDEs like IntelliJ IDEA and Eclipse.
- Supports parameterized tests for running the same test with different inputs.
- Enables simpler and more efficient testing with assertions and annotations.
- Ability to create self-verifying tests
Cons:
- Primarily focused on unit testing; may require additional tools for integration or end-to-end testing.
- No support for GUI and dependency testing
- Not suitable for dealing with a large number of test suites
JUnit's simplicity and effectiveness make it an essential tool for any Java developer looking to implement TDD practices in their projects. Its ability to integrate with CI/CD pipelines further enhances its utility in modern software development environments.
2. TestNG
Inspired by JUnit, TestNG introduces several new functionalities that make it more powerful and easier to use for large-scale testing scenarios. It is designed to cover all categories of tests: unit, functional, end-to-end, integration, etc.
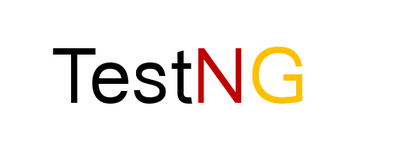
Key Features:
- Parallel Test Execution: TestNG allows tests to be run in parallel, which can significantly reduce execution time.
- Test Configuration Flexibility: It supports annotations similar to JUnit but offers additional ones like
@BeforeSuite
and@AfterSuite
for more complex setups. - Detailed HTML Reports: After executing tests, TestNG generates comprehensive HTML reports that provide insights into test outcomes.
- TestNG offers annotation support.
- A user can create test suites based on test methods, classes, test groups, and packages using an XML file.
- TestNG is capable of grouping test methods and executing dependent test methods.
- Using TestNG's dependencies option, we can add the necessary software versions and change them according to user preferences.
Pros:
- Flexible and supports various types of testing including unit, functional, and end-to-end.
- Allows dependency testing where you can specify methods that should be executed before others.
- Able to extract HTML reports post-test implementation
- Supports dependent test methods for application server testing
- Open and flexible API plugin for users to create custom extensions
- Supported by a wide range of tools such as IDEA, Maven, Eclipse, etc.
Cons:
- Slightly steeper learning curve compared to JUnit due to its broader feature set.
- Requires time and experienced resources
- Not suitable if the project doesn’t require test case prioritization
TestNG's ability to handle complex test configurations makes it a preferred choice for developers working on large projects with intricate testing requirements. Its compatibility with Selenium makes it particularly useful for automated web application testing.
3. Selenium
Selenium is a powerful tool for automating web applications for testing purposes. It supports multiple browsers and platforms, making it ideal for cross-browser testing. Selenium's suite includes WebDriver, IDE, and Grid, each serving different purposes within the automation ecosystem.
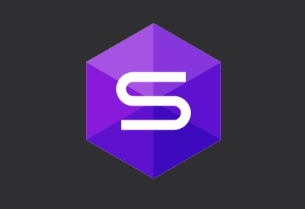
Key Features:
- WebDriver API: Provides a programming interface to create robust browser-based regression automation suites and tests.
- Cross-Browser Compatibility: Selenium supports all major browsers like Chrome, Firefox, Safari, and Edge.
- Integration with Other Tools: Seamlessly integrates with tools like TestNG and JUnit for enhanced test management.
- Selenium includes a native web browser that can be executed locally or through a Selenium server.
- With the run command, one test case can be utilized within another.
- Selenium facilitates the control flow of the structure.
- Selenium features a grid that enables running tests across multiple machines.
Pros:
- Highly flexible with robust community support.
- Supports multiple programming languages including Java.
Cons:
- Requires knowledge of programming for effective use.
- Managing dynamic web elements can be challenging without additional libraries like Selenide.
Selenium's versatility in automating browser tasks makes it indispensable for web application developers aiming to ensure compatibility across various platforms. Its open-source nature means it's continually evolving with contributions from developers worldwide.
4. Mockito
Mockito is a popular mocking framework used in conjunction with other Java testing frameworks like JUnit and TestNG. It simplifies the process of creating mock objects for unit tests by allowing developers to focus on the behavior they want to test rather than setting up complex object states.
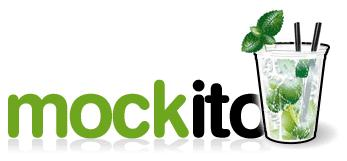
Key Features:
- Mock Creation Using Annotations: Mockito simplifies mock creation using annotations such as
@Mock
and@InjectMocks
. - Behavior Verification: Allows verification of method calls on mock objects using intuitive syntax.
- Supports behavior-driven testing with clear syntax.
- Integrates seamlessly with JUnit and TestNG.
Pros:
- Easy to integrate with existing Java projects.
- Supports behavior-driven development (BDD) style tests which enhance readability.
- Annotation report for mock generation
- Safe refactoring as mock artifacts are formed during runtime.
Cons:
- Limited to unit testing scenarios involving mock objects; not suitable for integration or system-level tests.
Mockito's ease of use and integration capabilities make it an excellent choice for developers looking to enhance their unit testing with realistic mock objects. Its ability to verify interactions between objects ensures that dependencies are correctly managed within the application logic.
5. Selenide
Selenide builds on top of Selenium, providing an easier way to write concise and stable UI tests. It handles dynamic content effectively, making it ideal for modern web applications where elements may load asynchronously or change frequently during user interactions.
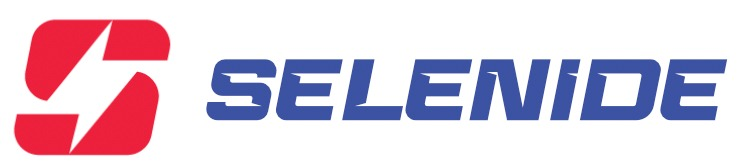
Key Features:
- Automatic Waiting for Elements: Selenide automatically waits for elements to appear or disappear before interacting with them, reducing flakiness in tests.
- Concise API: Offers a more readable API compared to raw Selenium WebDriver commands.
Pros:
- Ensures precise and stable tests by solving Ajax issues
- More readable and shorter tests with concise API
- Supports app tests developed using AngularJS
- Automatic screenshots
Cons:
- Customization can be complex for advanced scenarios requiring specific browser configurations or network conditions.
Selenide's focus on simplicity without sacrificing power makes it a great tool for developers who need reliable UI tests without extensive setup. Its automatic waiting mechanism significantly reduces false negatives caused by timing issues during test execution.
6. Spock
Spock is a testing framework that leverages Groovy syntax to offer expressive and readable tests. It's particularly known for its data-driven testing capabilities which allow developers to run the same test logic against different sets of input data efficiently.
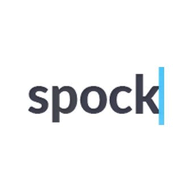
Key Features:
- Combines JUnit’s stability with Groovy expressive syntax.
- Supports data-driven testing out-of-the-box.
- Powerful mocking and stubbing capabilities.
- Readable and maintainable test specifications.
Pros:
- Compatibility with most IDEs, build tools, and integration servers
- Great code readability and documentation
- Built-in stubbing and mocking
- Simple and expressive Domain-Specific Language (DSL)
Cons:
- A basic understanding of Groovy is required
Spock's expressive syntax makes it an attractive option for developers who prioritize readability maintainability their-test-suites Its seamless integration existing-Java-projects coupled-with-powerful-mocking-stubbing-capabilities ensures comprehensive-test-coverage minimal-effort
7 .Serenity
Serenity designed behavior-driven-development (BDD) extends WebDriver capabilities provides comprehensive reporting features help visualize test results effectively
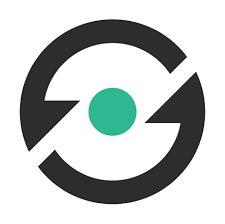
Key Features:
- Serenity enables writing cleaner and more structured code.
- It creates descriptive reports with a business-readable format for each test.
- Serenity is highly effective for behavior-driven testing.
Pros:
- Integrated with multiple automated testing frameworks like Selenium WebDriver, JBehave, JUnit, and Appium
- Rich built-in support for web testing with Selenium
- Highly readable, manageable, and scalable automated testing with the Screenplay pattern
Cons:
- Regular communication is required to make the most of BDD
- Takes more time to create feature files
Serenity’s ability create detailed-test-documentation makes invaluable teams practicing BDD those needing comprehensive-reporting-capabilities By integrating seamlessly-JBehave-Cucumber Serenity enables teams leverage power-BDD while maintaining clear concise documentation throughout development lifecycle
8. Gauge
Gauge open-source tool focuses acceptance-testing low-code environments emphasizes readability using markdown syntax writing-tests
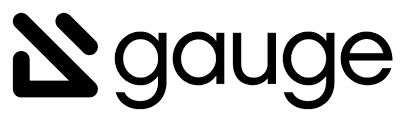
Key Features:
Supports multiple languages CI/CD tools Offers parallel execution features scalability
Pro’s: Easy integration cloud-based solutions
Con’s: Limited community support compared established frameworks
Gauge’s focus simplicity scalability makes ideal teams looking integrate acceptance-tests into CI/CD pipelines effortlessly Its markdown-based approach ensures-tests remain-readable-understandable even nontechnical stakeholders fostering collaboration across entire-team
9. Cucumber
Cucumber another BDD framework uses plain-language specifications describe software behavior bridges gap between technical-teams nontechnical stakeholders using Gherkin syntax
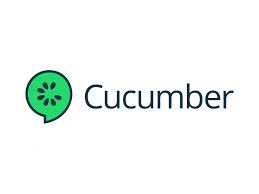
Key Features:
Supports writing-tests plain English Gherkin language Integrates well Selenium other-Java-frameworks
Pros:
- Provides an intuitive way to describe requirements in natural language
- Code reusability for faster development time
- User-friendly interface to reduce the technical entry barrier
Cons:
- Complexity arising from the combination with Gherkin
- The given-when-then format can cause unnecessary elaborations
Cucumber’s ability create shared understanding among team members makes valuable-tool agile-development environments where communication key By enabling-tests written plain-language understood everyone involved project Cucumber fosters greater alignment around desired outcomes ensuring successful-delivery high-quality-software-products consistently over time!
10. Apidog
While primarily known API-development tool Apidog recently introduced features enhance utility Java ecosystem particularly around API-debugging automated-testing
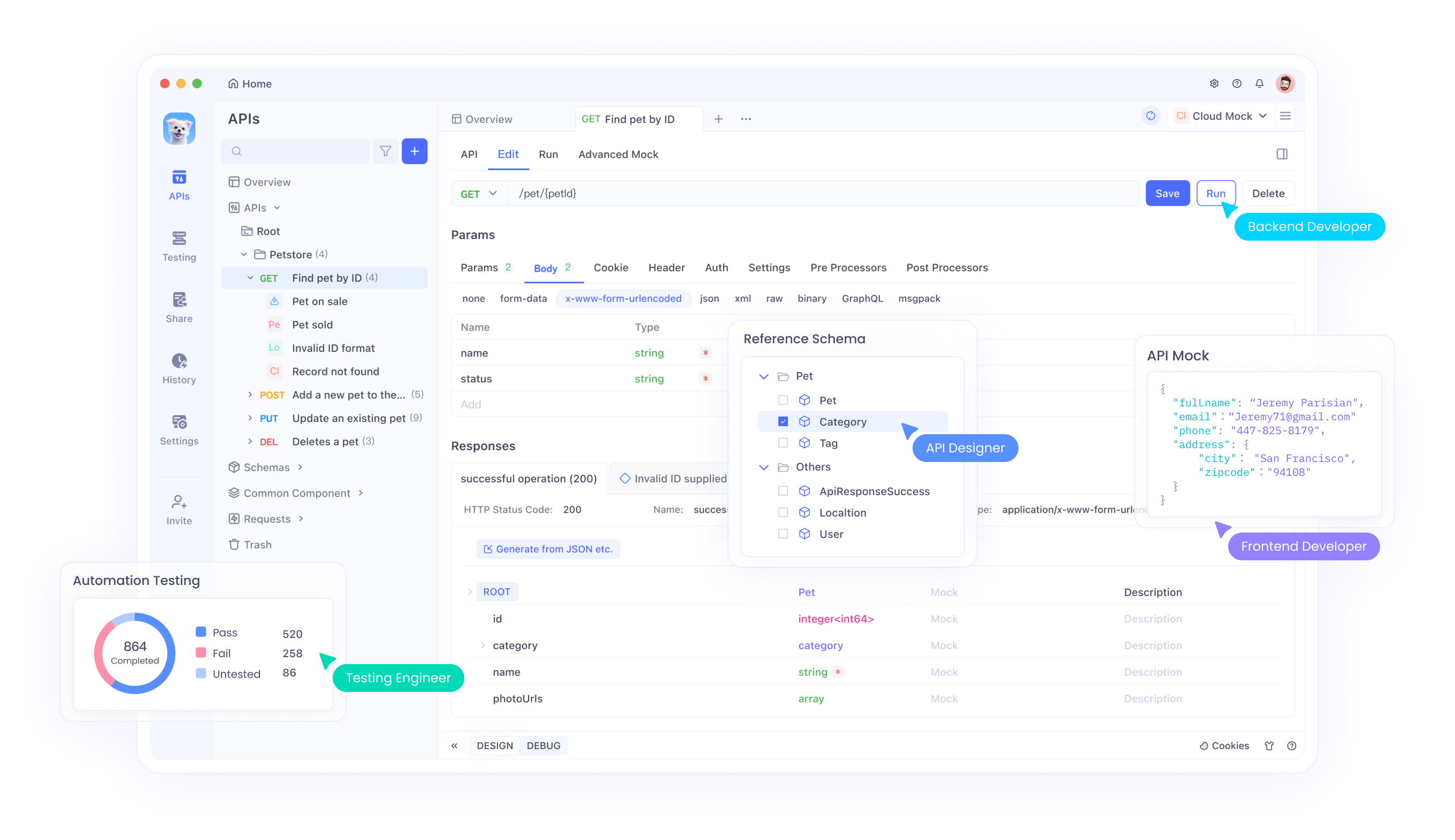
Recent Updates: New "Debug Mode" simplifies API-debugging allowing direct parameter modifications without predefined documentation Enhanced support loops conditionals error handling-test-cases
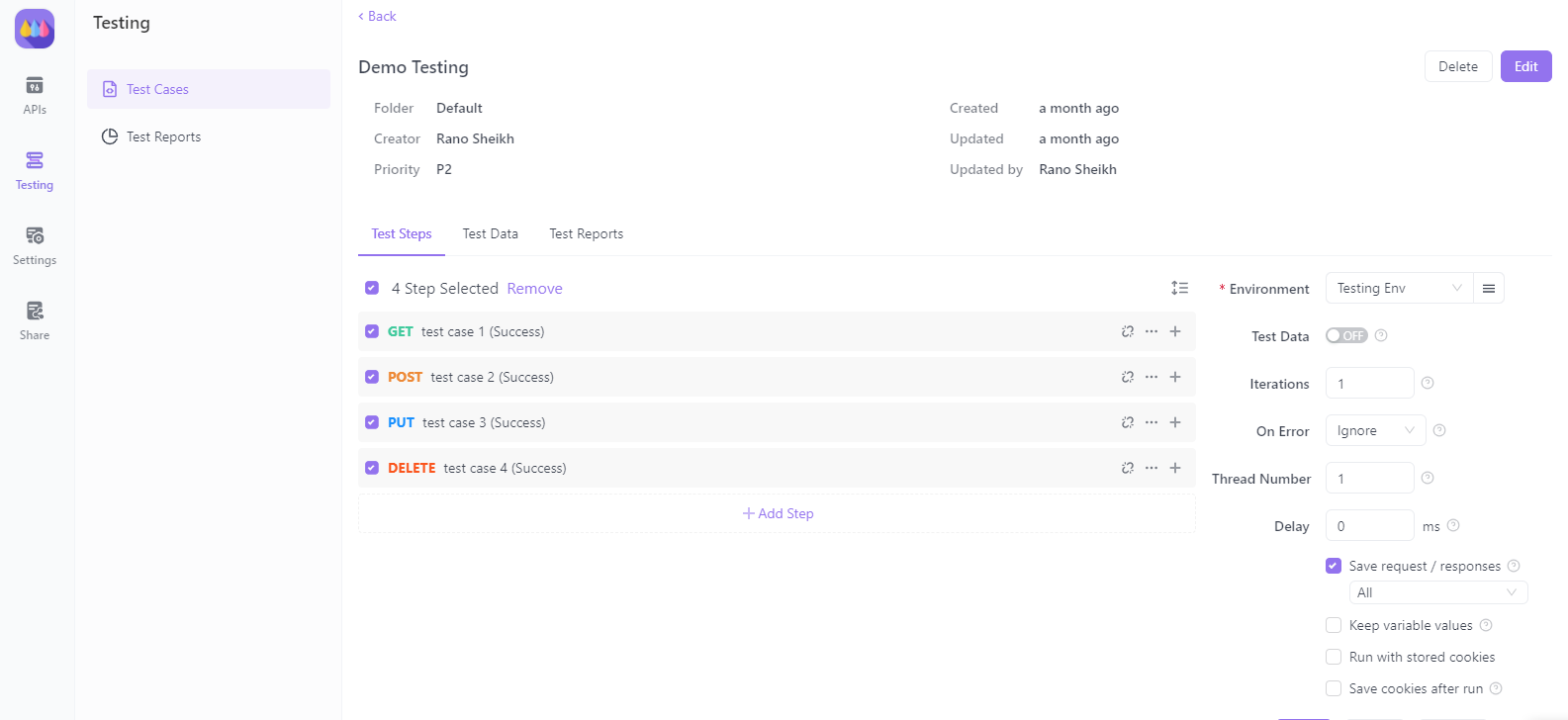
Apidog recent updates make exciting addition any developer’s toolkit especially those working extensively APIs their-Java-applications Its intuitive interface powerful-debugging-capabilities streamline process developing robust APIs while ensuring they meet quality standards through automated-tests By integrating seamlessly existing-development-environments Apidog empowers-developers deliver high-performance reliable APIs faster than ever before!
Conclusion
Choosing the proper Java testing tool will dramatically affect your development workflow by improving efficiency, enhancing code quality, and reducing a lot of time spent on manual testing tasks. Each of the tools listed above has unique features, ranging from unit tests with JUnit and Mockito, UI automation with Selenium and Selenide, and from behavior-driven development provided by Serenity and Cucumber to Apidog and its really innovative approach toward API debugging within Java ecosystems.
Learning how to use these tools effectively in the context of projects' needs, from small-scale applications requiring simple unit checks through large enterprise systems that require end-to-end validations, you will be well-equipped to maintain high-quality products and also efficiently support them over time.