Choosing the right programming language for building APIs is a crucial decision that can significantly impact your project's performance, scalability, and maintainability. In this comprehensive guide, we'll explore four popular languages C#, Java, Golang, and Python—to help you determine which is best suited for your API development needs. Additionally, we'll introduce you to Apidog, an all-in-one collaborative API development platform that can streamline your API testing process.
Introduction to API Development
APIs (Application Programming Interfaces) are the backbone of modern software applications, enabling different systems to communicate and share data seamlessly. Choosing the appropriate programming language for building APIs is vital, as it affects development speed, performance, and scalability.
Overview of Programming Languages
Let's delve into each of the four languages, examining their strengths and weaknesses in the context of API development.
C#
C# is a statically typed, object-oriented programming language developed by Microsoft as part of the .NET framework.It's known for its robustness and is widely used in enterprise-level applications.
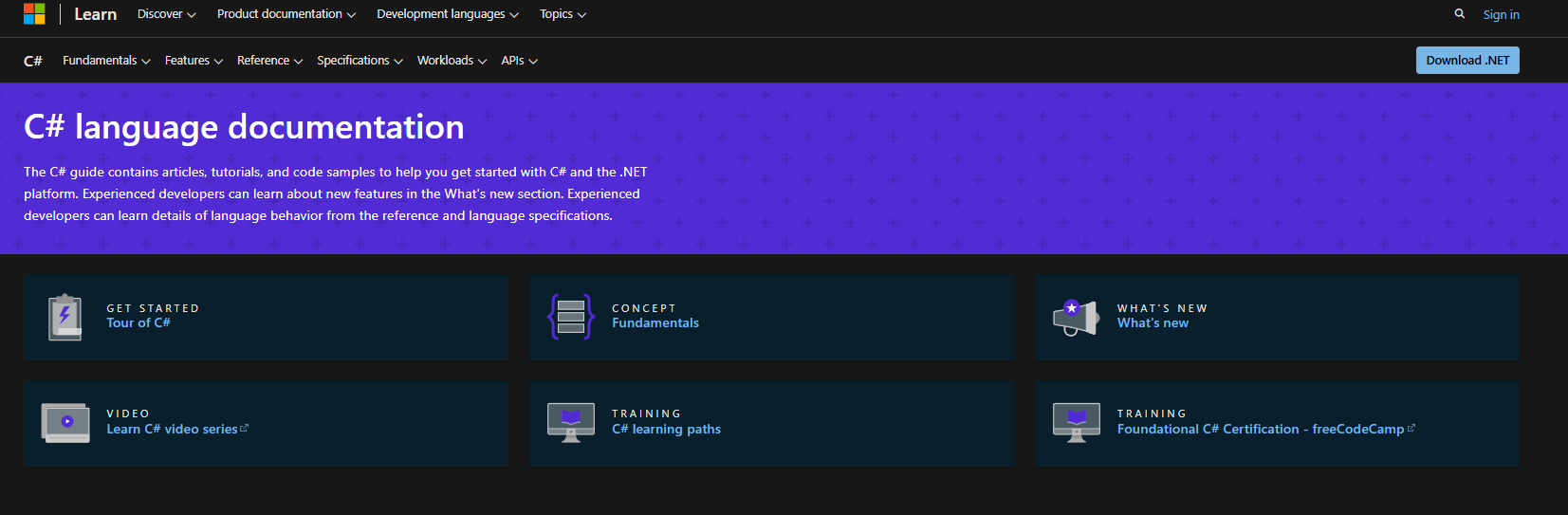
Pros:
- Performance: C# offers high performance, especially with the .NET Core framework, which is optimized for building scalable web applications.
- Tooling: The language boasts a rich set of development tools, including Visual Studio, which provides an integrated development environment (IDE) with debugging and testing capabilities.
- Cross-Platform: With the introduction of .NET Core, C# applications can now run on Windows, Linux, and macOS, enhancing flexibility in deployment.
Cons:
- Complexity: The language's extensive features can be overwhelming for beginners, leading to a steeper learning curve.
- Memory Management: While C# provides garbage collection, developers still need to be cautious about memory leaks, especially in large applications.
Java
Java is a versatile, object-oriented programming language that has been a staple in the software industry for decades.It's renowned for its portability across platforms, thanks to the Java Virtual Machine (JVM).
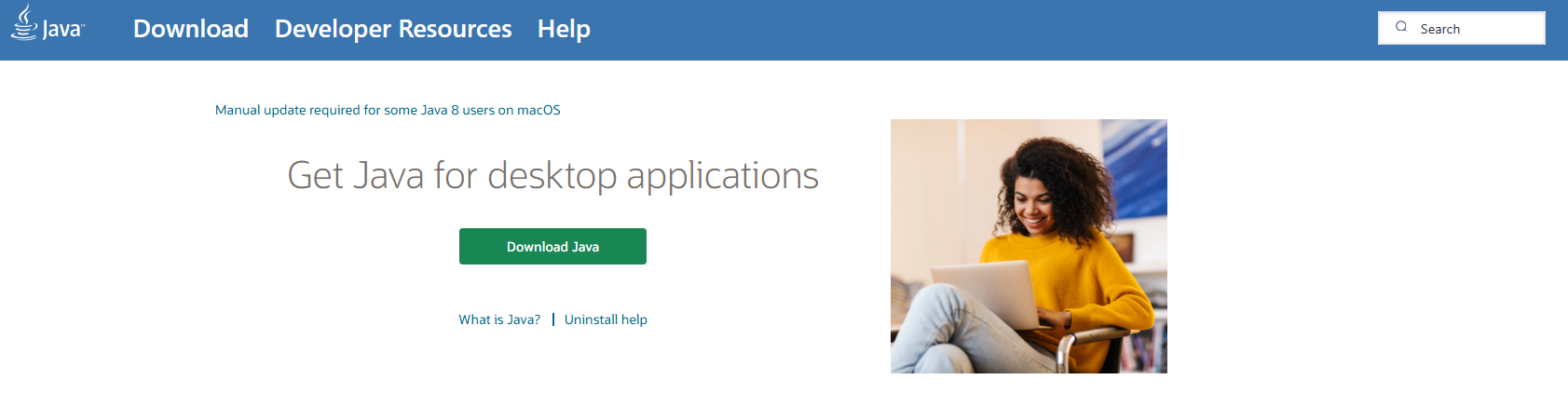
Pros:
- Scalability: Java's architecture allows for building large-scale, distributed systems with ease.
- Community Support: A vast community of developers contributes to a rich ecosystem of libraries and frameworks, facilitating rapid development.
- Performance: With Just-In-Time (JIT) compilation, Java offers respectable performance suitable for high-throughput applications.
Cons:
- Verbosity: Java's syntax can be verbose, leading to longer codebases that may be harder to maintain.
- Memory Consumption: The JVM can be resource-intensive, which might be a consideration for applications with limited resources.
Golang
Developed by Google, Golang (or Go) is a statically typed, compiled language designed for simplicity and efficiency.It's particularly well-suited for building concurrent applications.
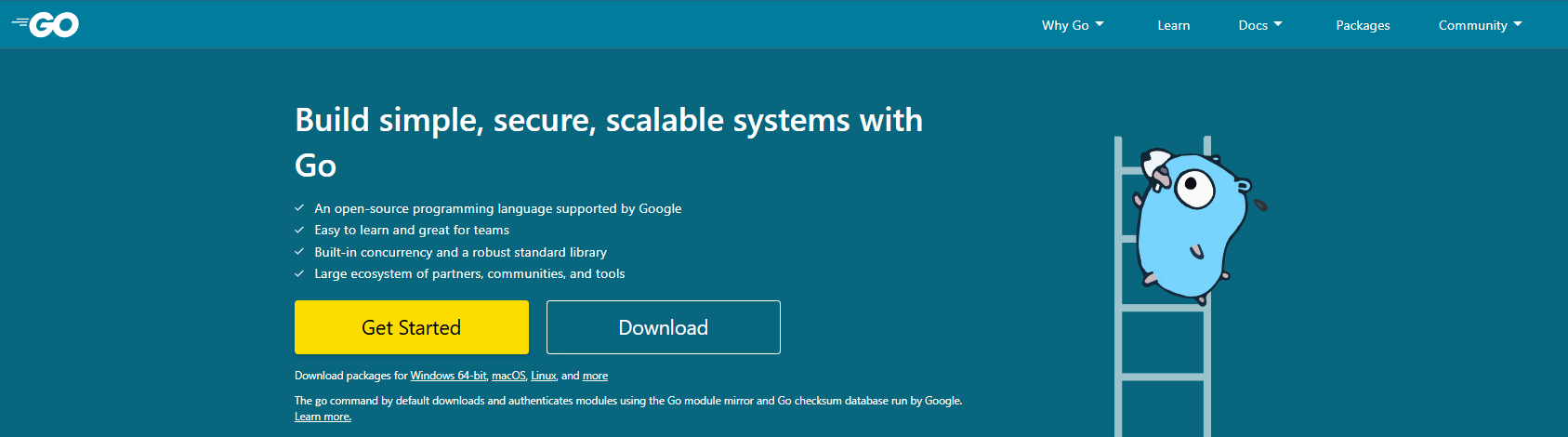
Pros:
- Performance: As a compiled language, Go offers excellent performance, often comparable to lower-level languages like C++.
- Concurrency: Go's goroutines provide a simple and efficient way to handle concurrent operations, making it ideal for networked applications.
- Simplicity: The language's minimalist design promotes readability and maintainability.
Cons:
- Maturity: Being relatively new, Go has a smaller ecosystem compared to more established languages.
- Generics: Until recently, Go lacked support for generics, which could lead to code duplication. However, this is being addressed in newer versions.
Python
Python is an interpreted, dynamically typed language known for its simplicity and readability.It's a popular choice for rapid application development.
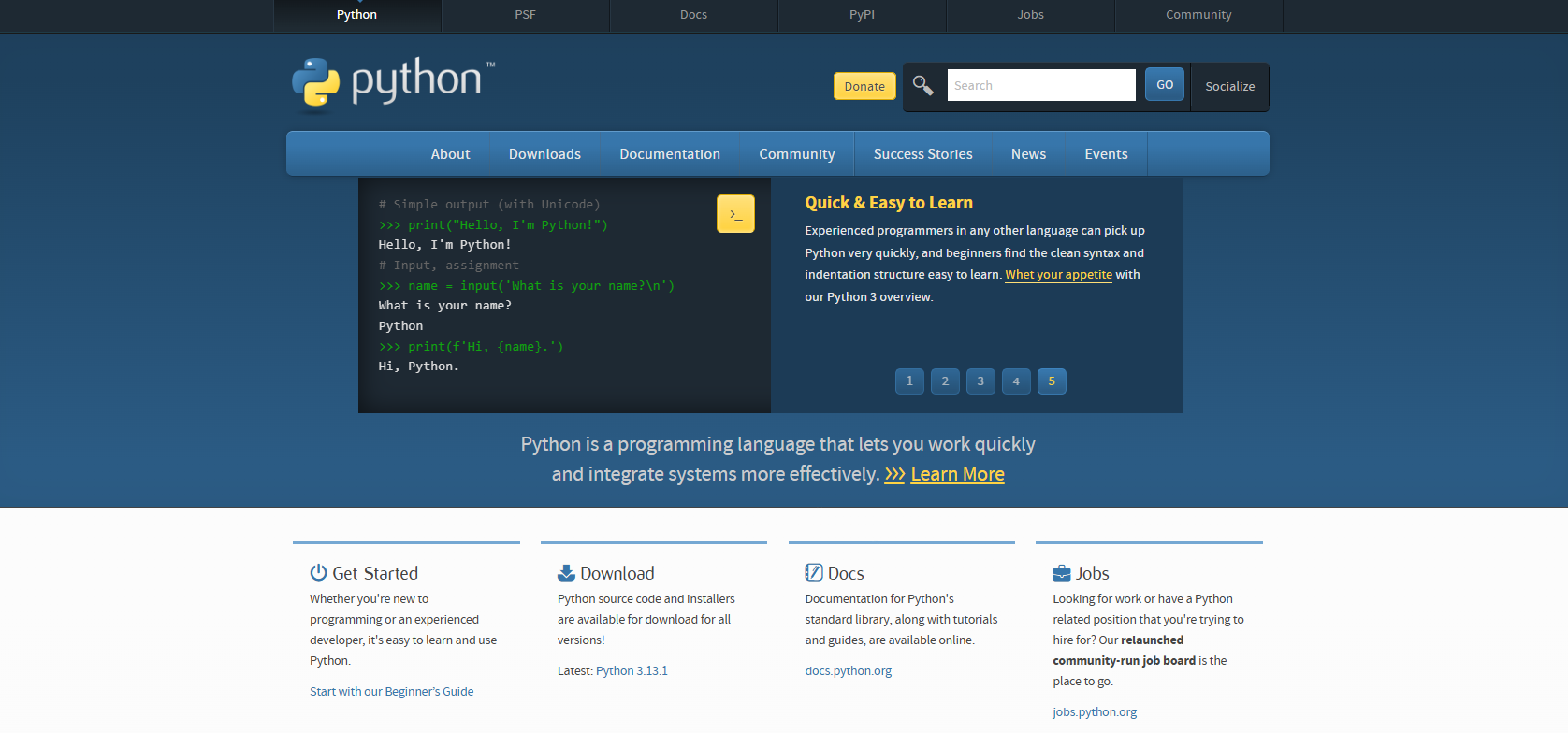
Pros:
- Ease of Use: Python's straightforward syntax makes it accessible to beginners and allows for quick development cycles.
- Libraries: A vast standard library and numerous third-party packages facilitate various aspects of development, including web frameworks like Django and Flask.
- Community: A large and active community ensures continuous improvement and support.
Cons:
- Performance: As an interpreted language, Python may not match the performance of compiled languages, which can be a drawback for CPU-intensive tasks.
- Concurrency: Python's Global Interpreter Lock (GIL) can be a bottleneck in multi-threaded applications, affecting scalability.
Performance Comparison
Performance is a critical factor in API development, influencing response times and the ability to handle multiple requests.
- Golang: Excels in performance due to its compiled nature and efficient memory management, making it suitable for high-performance APIs.
- C#: With .NET Core, C# offers competitive performance, handling intensive tasks effectively.
- Java: Provides solid performance, though it may require fine-tuning and optimization for resource-intensive applications.
- Python: While sufficient for many applications, Python's performance may lag behind compiled languages like Go, C#, and Java, especially when dealing with high-throughput or compute-intensive operations. However, it shines in areas where ease of development and rapid iteration are more important than raw speed. For example, Python is often used for data-driven APIs and machine learning models where the overhead is acceptable.
In general, if performance is your primary concern, Golang and C# (with .NET Core) are top contenders, with Java coming in as a strong alternative. Python, while great for prototyping and certain use cases, may not be the best option when dealing with very high load environments.
Scalability and Concurrency
When building APIs, especially in the context of microservices, scalability and the ability to handle concurrent requests efficiently are key factors to consider.
- Golang: One of Golang's standout features is its concurrency model. With goroutines (lightweight threads), Go makes it incredibly easy to handle multiple requests concurrently, which is essential for APIs that need to scale across many users or services. It’s also memory efficient, as each goroutine consumes very little memory compared to traditional threads in other languages. This makes Go a great choice for high-traffic APIs and microservices architectures.
- C#: C# with async/await and the Task Parallel Library (TPL) provides excellent support for asynchronous programming. You can handle a large number of concurrent requests with ease. The performance of C# in handling concurrency has been improved drastically with .NET Core, making it suitable for scalable applications. However, C# still relies heavily on threading, which can be a bit heavier on resources compared to Go's goroutines.
- Java: Java has traditionally been used for large-scale, enterprise-level applications, and its concurrency model is robust. It uses threads and provides various concurrency utilities (e.g.,
ExecutorService
) to manage them efficiently. While Java’s thread management is quite powerful, it can be resource-heavy compared to Go’s goroutines. Nevertheless, Java has proven itself in building scalable systems that handle thousands of concurrent requests, especially with frameworks like Spring Boot that simplify the development of scalable web applications. - Python: Python, by default, is not ideal for handling large numbers of concurrent tasks due to its Global Interpreter Lock (GIL), which prevents multiple threads from executing Python bytecodes simultaneously. However, Python compensates with tools like asyncio and Celery for asynchronous tasks and distributed processing. For APIs that don't require extensive concurrency, Python can still handle concurrent requests adequately, though it may not be as efficient as Go or C# in highly concurrent environments.
Conclusion on Scalability and Concurrency
If you're looking for easy scalability and efficient concurrency, Go is the clear winner. It’s lightweight, easy to scale, and perfect for high-traffic APIs. C# and Java both handle concurrency well, with C# benefiting from .NET Core's improvements in async programming. Python, while not the best for concurrency-heavy applications, can still perform well for many use cases, especially when scalability requirements aren't too high.
Ease of Use and Learning Curve
The ease of use and the learning curve of each language are vital when choosing a language for API development, especially if you're working with a small team or want to quickly iterate on your APIs.
- C#: While C# has a steep learning curve, especially for developers new to the .NET ecosystem, it’s a well-structured language with strong tooling. Microsoft’s support and the comprehensive documentation make it easier to overcome the initial hurdles. The development environment (Visual Studio) also provides a robust experience with features like IntelliSense, debugging, and performance profiling.
- Java: Java’s syntax is relatively verbose, which can be frustrating for beginners. However, the language has a huge body of resources and tutorials, so learning it is not difficult. Java’s massive community and long-standing presence in the industry ensure that developers will find ample support.
- Golang: Go’s syntax is intentionally simple and minimalistic, which makes it very easy for developers to pick up, even for beginners. It eliminates many of the complexities found in other languages (like C# and Java), which makes Go one of the easiest languages to learn for building APIs. Despite its simplicity, it’s a very powerful language that can handle complex tasks.
- Python: Python is widely recognized as one of the easiest languages to learn, especially for beginners. Its syntax is clean, readable, and concise, making it a great language for rapid development. Many developers choose Python for their API projects due to how quickly they can get started and build functional APIs.
Conclusion on Ease of Use and Learning Curve
If you're looking for a language that’s easy to pick up, Python and Golang are excellent choices. Go offers simplicity without sacrificing performance, while Python allows for rapid development but may face scalability limitations. C# and Java, while more complex, provide powerful tools and frameworks for larger and more enterprise-level API systems.
Community Support and Ecosystem
The size and strength of a language’s community and ecosystem can drastically influence your development process. A large community means better support, more libraries, and more resources.
- C#: The .NET ecosystem is vast, and Microsoft’s backing ensures strong long-term support. There are numerous libraries for building APIs, including ASP.NET Core, which is one of the most popular frameworks for creating APIs in C#. There’s also a rich set of tools available for testing, debugging, and monitoring APIs.
- Java: Java has one of the largest and most mature ecosystems. From web frameworks like Spring to libraries for serialization, authentication, and more, Java offers a comprehensive toolkit for building APIs. The Java community is vast, with numerous open-source projects and libraries available to speed up development.
- Golang: While Go is relatively new, it’s rapidly gaining popularity, particularly in the microservices space. It has a growing ecosystem, and the community is very active in creating open-source tools for API development. Popular frameworks like Gin and Echo make it easy to build APIs quickly in Go.
- Python: Python has a massive community and a rich ecosystem of libraries and frameworks. For building APIs, Django and Flask are the two most popular frameworks. Python's versatility means you can easily find libraries for nearly any use case, including machine learning, data analysis, and more.
Conclusion on Community Support and Ecosystem
If community and ecosystem support are your primary concerns, Java and C# are the best choices. Both have mature ecosystems and vast communities. However, Golang is growing quickly, and Python has a rich ecosystem for rapid development.
Testing APIs with Apidog
Now that we’ve explored the pros and cons of each language for building APIs, let’s look at how you can efficiently test your API using Apidog. Apidog is an all-in-one API design and testing platform that streamlines the API development process. With Apidog, you can design, debug, and test your APIs with ease.
Steps to Test Your API with Apidog:
Sign Up: Start by downloading Apidog.
Create a New Project: Once logged in, create a new API project.
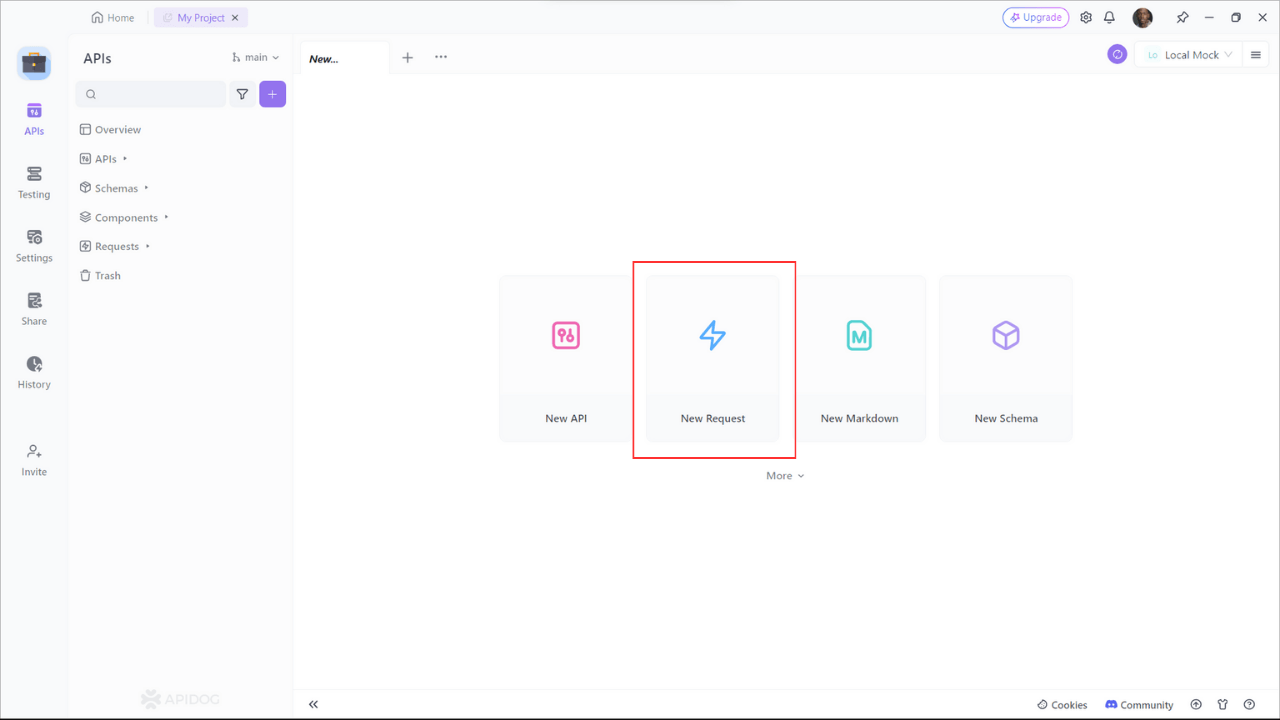
Design Your API: Use Apidog’s intuitive interface to define your API endpoints, including request methods, parameters, and responses.
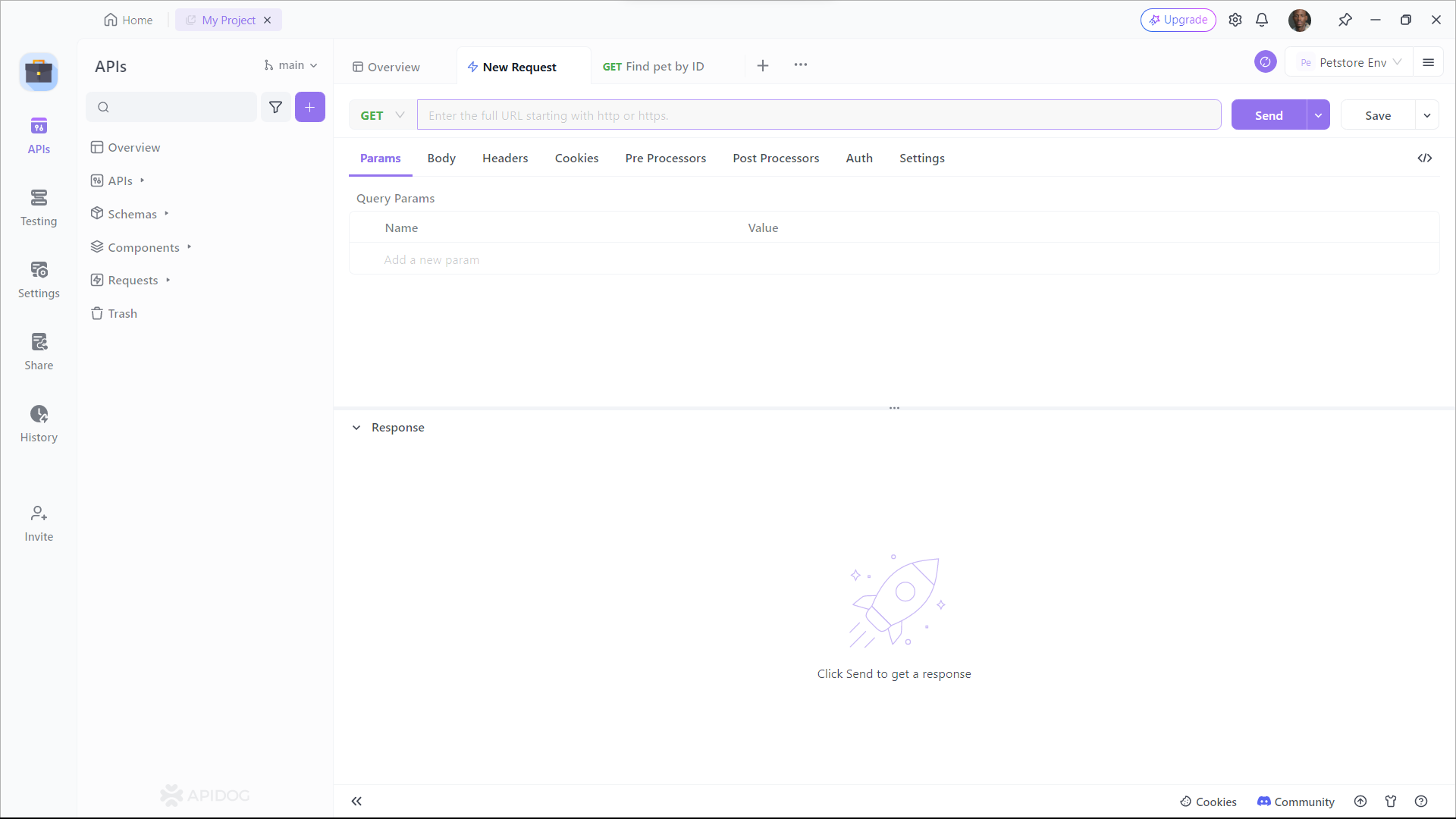
Test Your Endpoints: Apidog allows you to send requests to your API and view real-time responses. You can test different scenarios, validate response data, and ensure that your API behaves as expected.
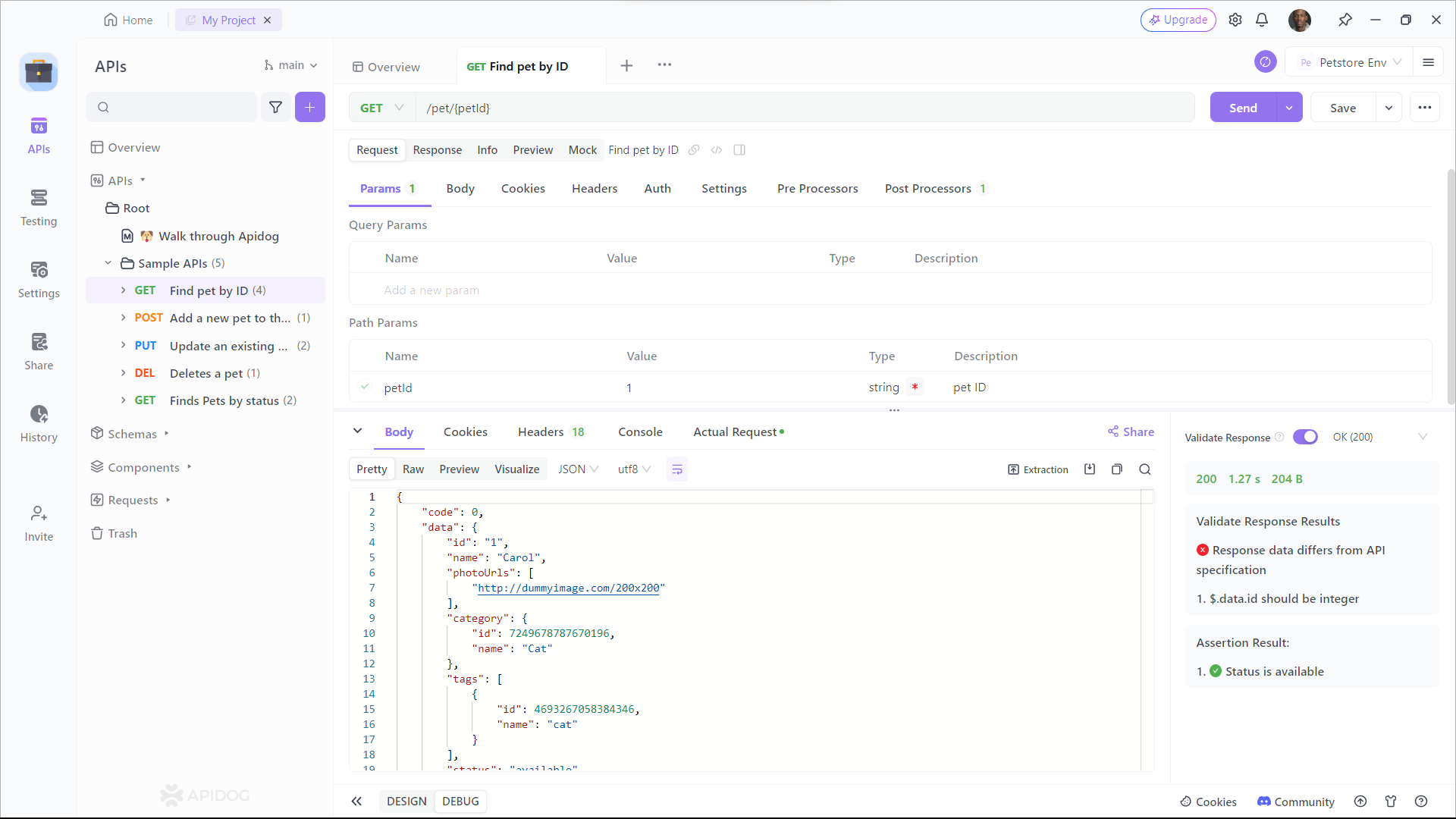
Apidog also supports team collaboration, so your team members can join the project and test APIs collaboratively.
By integrating Apidog into your development workflow, you can save valuable time and reduce errors during testing. Download Apidog for free and start testing your APIs today!
Conclusion
In this blog post, we've compared four of the most popular programming languages for building APIs: C#, Java, Golang, and Python. Each language has its strengths and weaknesses, depending on your specific needs. For high-performance, scalable APIs, Golang is a top contender. If you need a robust ecosystem and community support, Java or C# are excellent choices. Python is perfect for rapid development, but may not be ideal for high-concurrency, performance-critical APIs.
Ultimately, the best language for your API depends on factors such as performance requirements, scalability, development speed, and your team’s expertise.