In today's digital landscape, the way we build and interact with APIs (Application Programming Interfaces) has become a critical skill for developers. APIs serve as the backbone of modern web applications, enabling seamless communication between different services. When it comes to working with APIs, two powerful tools that often come into play are Axios and GraphQL. These tools, when used together, can greatly simplify and enhance your development experience. In this blog post, we'll dive into the world of Axios and GraphQL, exploring how they work, why they're beneficial, and how you can leverage them to build more efficient and robust applications. Plus, we'll introduce you to a fantastic tool called Apidog.
What is Axios?
Let's start with Axios. Axios is a promise-based HTTP client for JavaScript, primarily used for making HTTP requests from the browser. It's often preferred over the native fetch
API due to its ease of use and additional features. With Axios, you can make GET
, POST
, PUT
, DELETE
, and other types of requests with just a few lines of code.
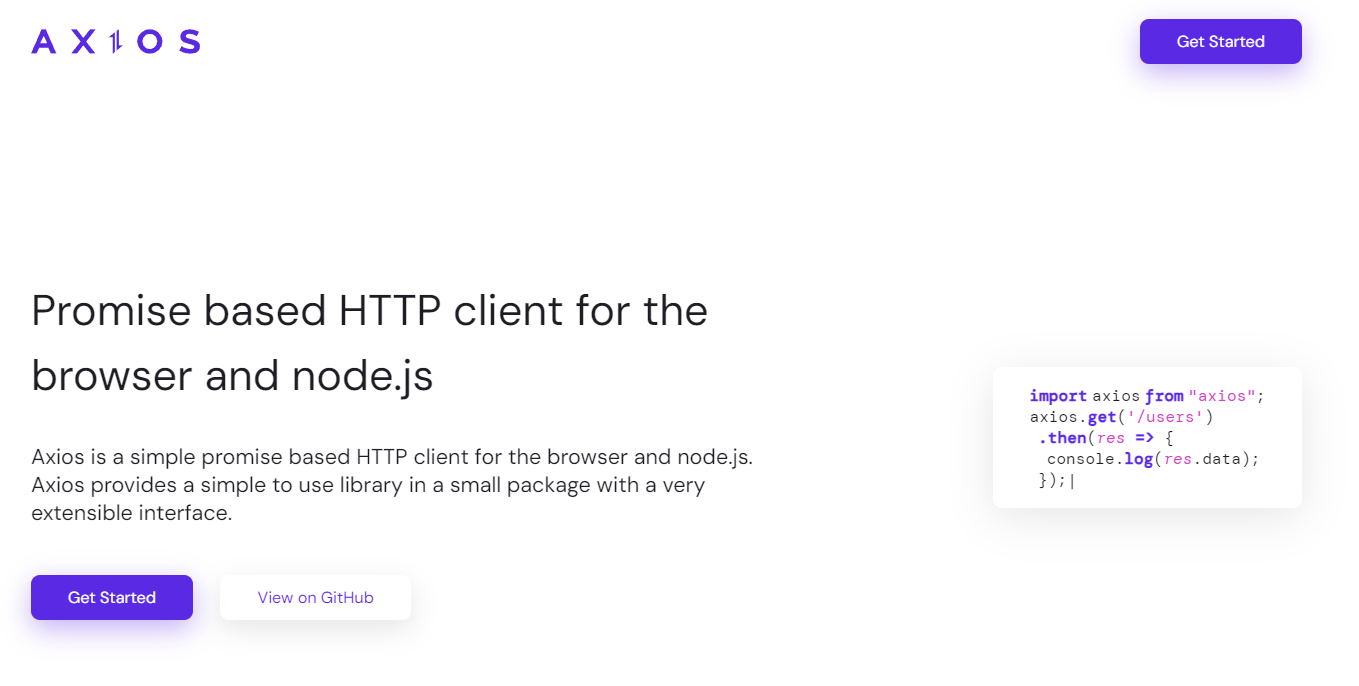
Key Features of Axios:
- Promise-based: Axios uses promises, which makes your asynchronous code easier to read and write.
- Interceptors: These allow you to modify requests or responses before they are handled by
then
orcatch
. - Automatic transformations: Axios automatically transforms JSON data.
- Cancellation: You can cancel requests using Axios' cancellation tokens.
- Browser compatibility: Axios works in all major browsers, including Internet Explorer 11.
What is GraphQL?
GraphQL , on the other hand, is a query language for APIs and a runtime for executing those queries. Developed by Facebook, GraphQL provides a more efficient and flexible alternative to REST by allowing clients to request exactly the data they need. This reduces over-fetching and under-fetching of data.
Key Features of GraphQL:
- Declarative data fetching: Clients can specify the exact data requirements.
- Strongly typed schema: GraphQL APIs are defined by a schema, which provides a clear structure and type system.
- Real-time updates: GraphQL supports real-time updates through subscriptions.
- Introspective: GraphQL APIs can be queried for their schema, making them self-documenting.
- Single endpoint: All interactions occur through a single endpoint, simplifying network requests.
Why Use Axios with GraphQL?
Combining Axios with GraphQL can be a game-changer for your development workflow. Axios simplifies the process of making HTTP requests, while GraphQL provides a more flexible and efficient way to query and manipulate data. Together, they offer several advantages:
- Efficient Data Fetching: With GraphQL, you can fetch only the data you need, and Axios makes it easy to send these queries.
- Simplified Codebase: Using Axios' promise-based syntax along with GraphQL queries can make your code cleaner and more maintainable.
- Enhanced Error Handling: Axios' interceptors combined with GraphQL's detailed error responses can improve your error handling strategies.
Getting Started with Axios and GraphQL
Now that we understand the basics, let's get our hands dirty with some code. We'll walk through setting up a simple project that uses Axios to fetch data from a GraphQL API.
Step 1: Set Up Your Project
First, let's set up a new Node.js project. Open your terminal and run the following commands:
mkdir axios-graphql
cd axios-graphql
npm init -y
npm install axios graphql
This will create a new directory, initialize a Node.js project, and install Axios and the GraphQL library.
Step 2: Create a Simple GraphQL API
For the sake of this tutorial, we'll use a simple GraphQL API. You can either set up your own using tools like Apollo Server or use a public GraphQL API. For simplicity, we'll use a public API in this example.
Step 3: Write Your Axios Request
Create a new file named index.js
and add the following code:
const axios = require('axios');
const GRAPHQL_API = 'https://countries.trevorblades.com/';
const query = `
{
countries {
code
name
capital
currency
}
}
`;
axios.post(GRAPHQL_API, { query })
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
In this code, we're using Axios to make a POST
request to a public GraphQL API that provides information about countries. The query we're sending requests the code
, name
, capital
, and currency
fields for all countries.
Step 4: Run Your Code
Run your code by executing node index.js
in your terminal. You should see a JSON response with the requested data.
Advanced Usage: Integrating Apidog
While Axios and GraphQL provide a powerful combination, there's another tool that can take your API development to the next level: Apidog. Apidog is a comprehensive API management tool that simplifies the process of designing, testing, and documenting APIs. It integrates seamlessly with tools like Axios and GraphQL, making it a valuable addition to your toolkit. Apidog can be used alongside Axios and GraphQL.
Why Use Apidog?
- API Design: Create and manage API specifications with an intuitive graphical interface.
- Testing: Automate API testing and ensure your endpoints are working as expected.
- Documentation: Generate detailed API documentation that stays up-to-date with your code.
- Collaboration: Share your API designs and documentation with your team.
Apidog with Axios
Step 1: Open Apidog and select new request
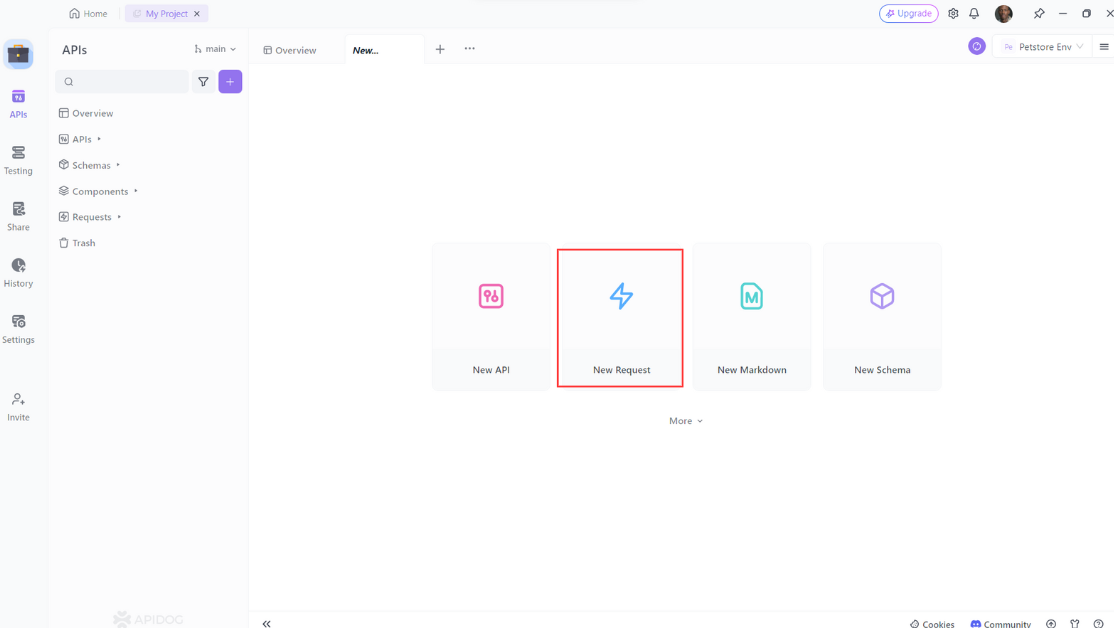
Step 2: Enter the URL of the API endpoint you want to send a request to,input any headers or query string parameters you wish to include with the request, then click on the "Design" to switch to the design interface of Apidog.
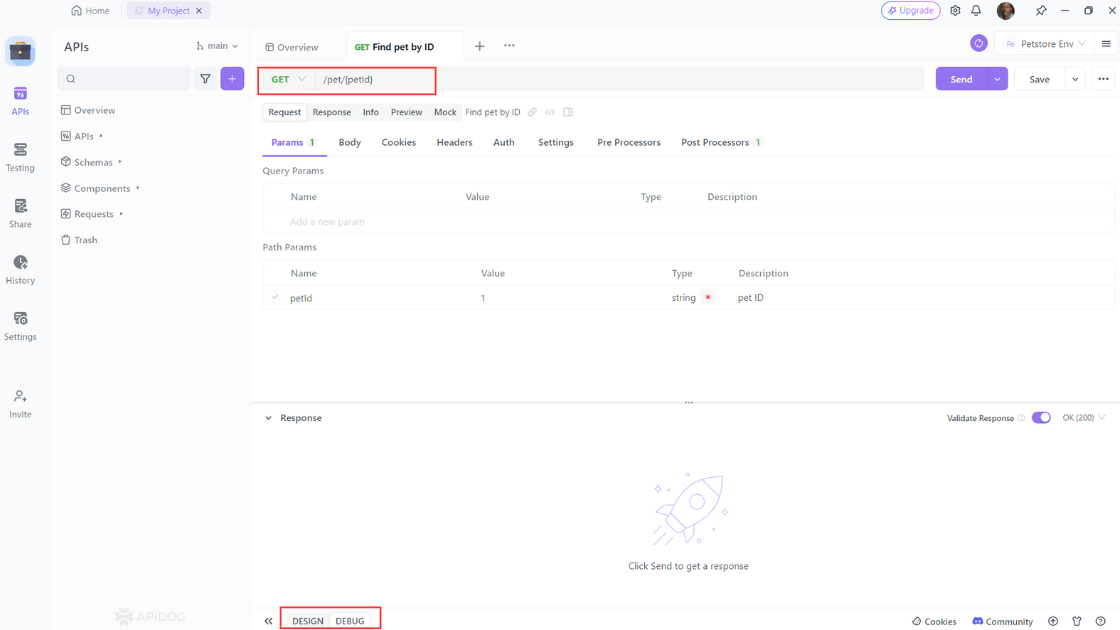
Step 3: Select "Generate client code " to generate your code.
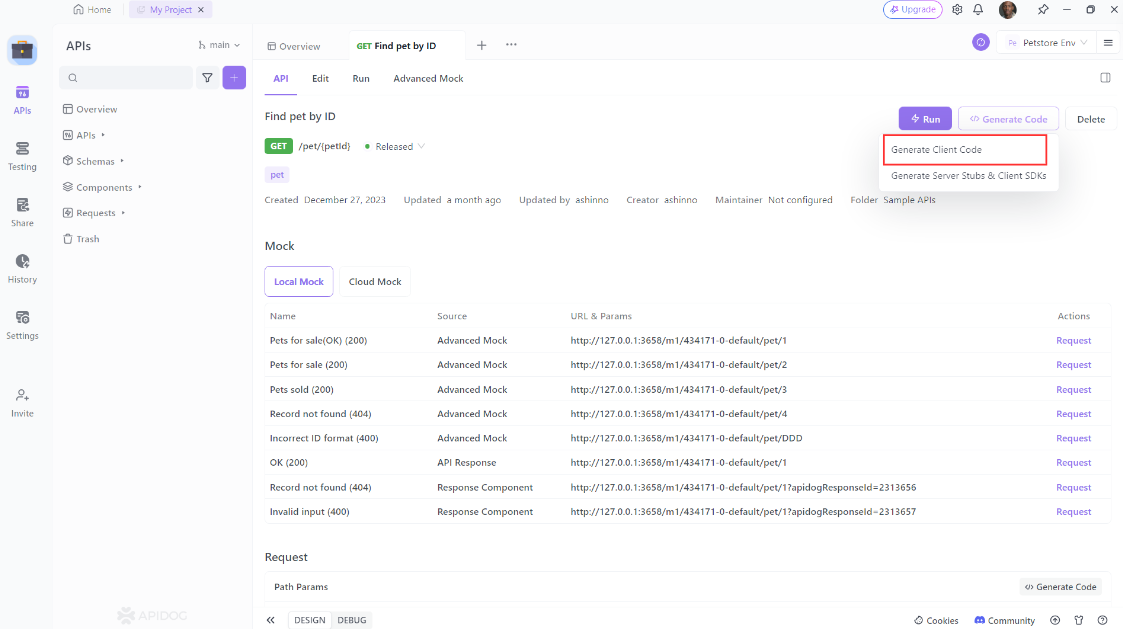
Step 4: Copy the generated Axios code and paste it into your project.
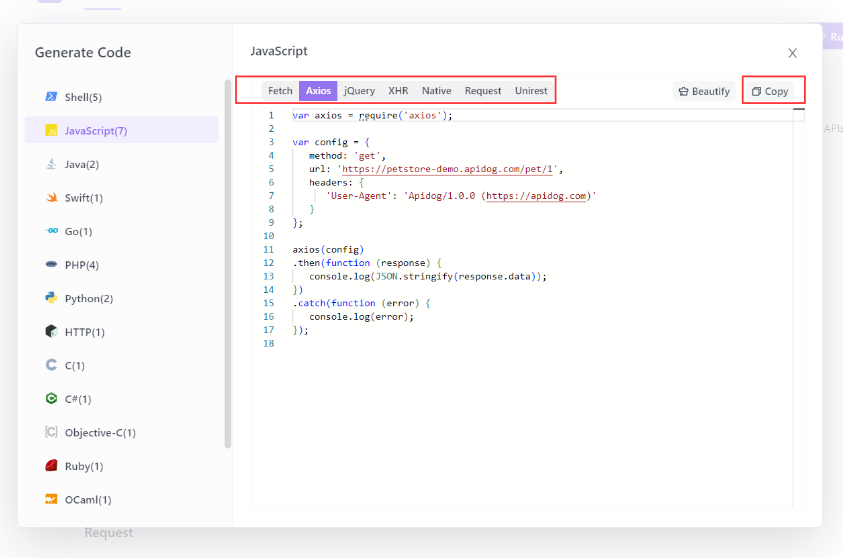
HTTP Requests with Apidog
Apidog offers several advanced features that further enhance its ability to test HTTP requests. These features allow you to customize your requests and handle more complex scenarios effortlessly.
Step 1: Open Apidog and create a new request.
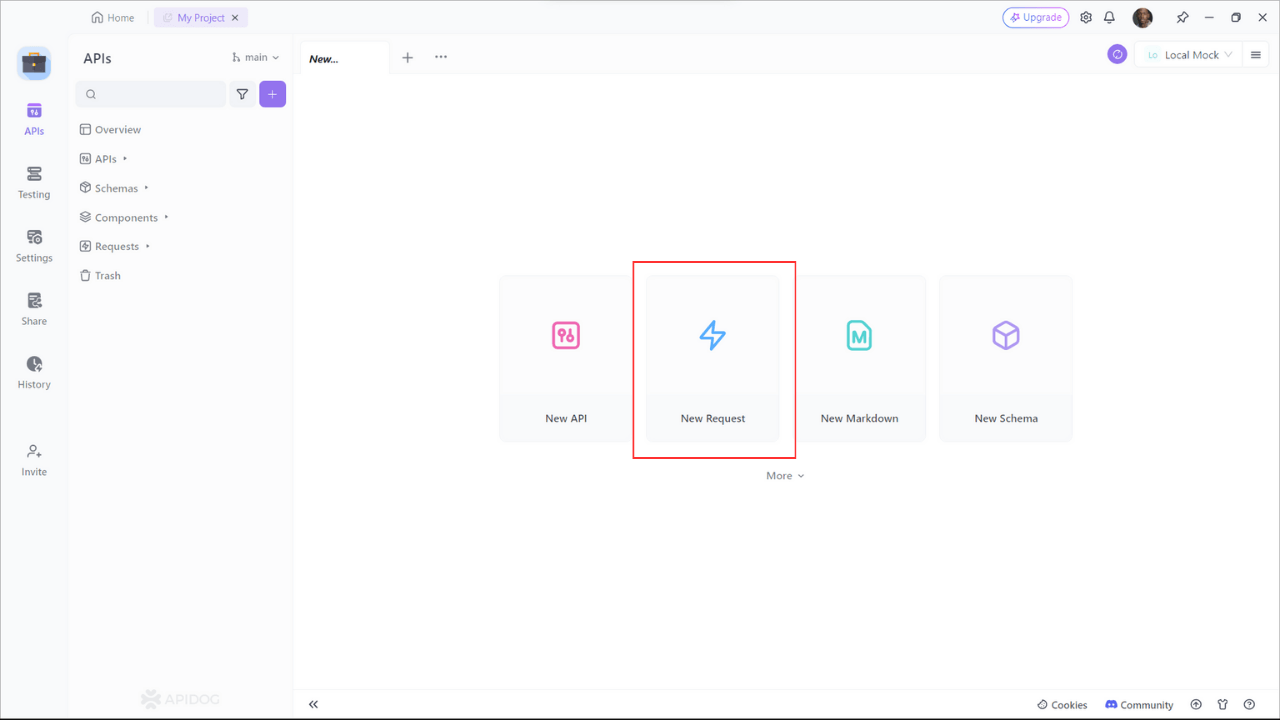
Step 2: Find or manually input the API details for the POST request you want to make.
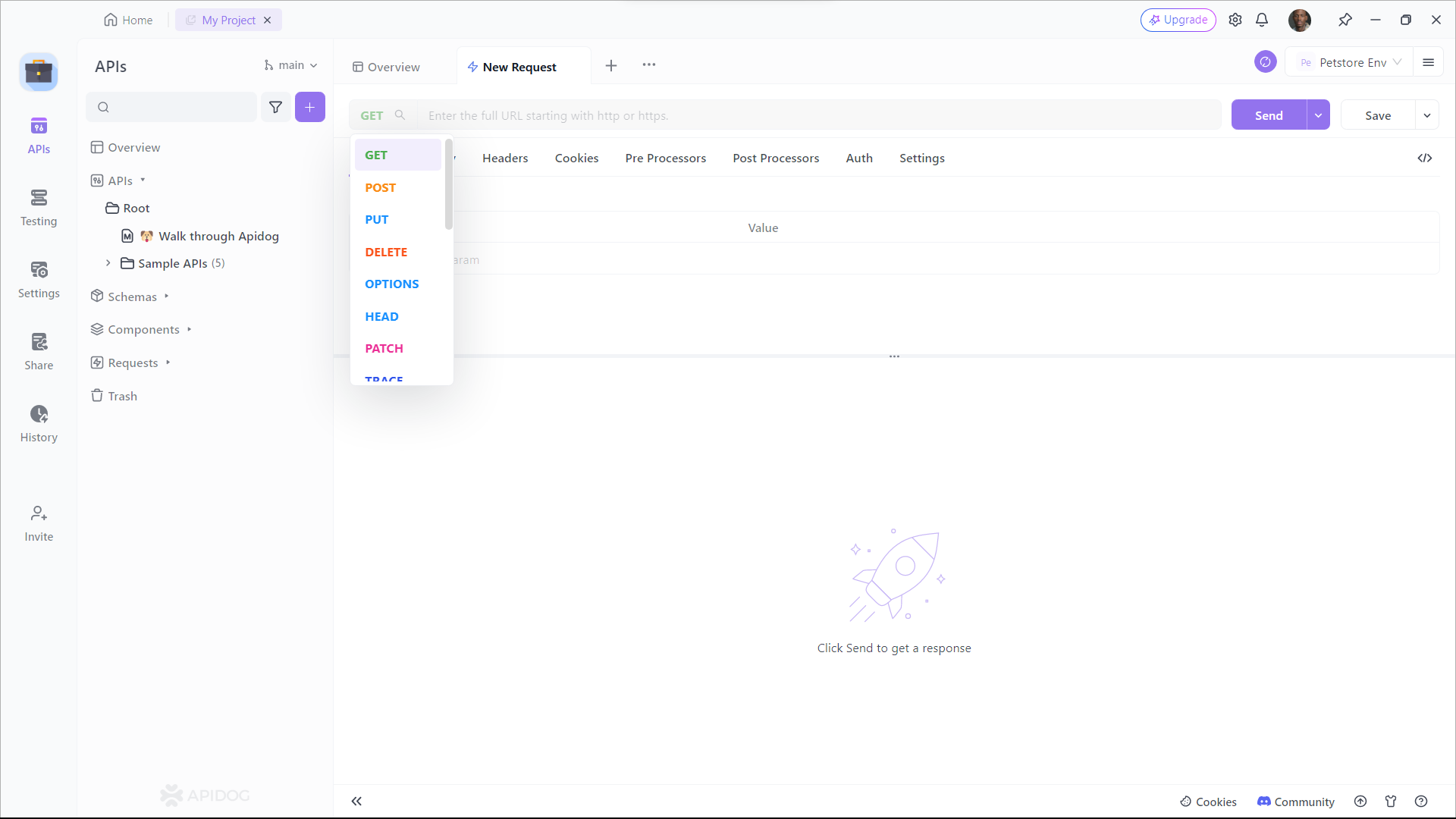
Step 3: Fill in the required parameters and any data you want to include in the request body.
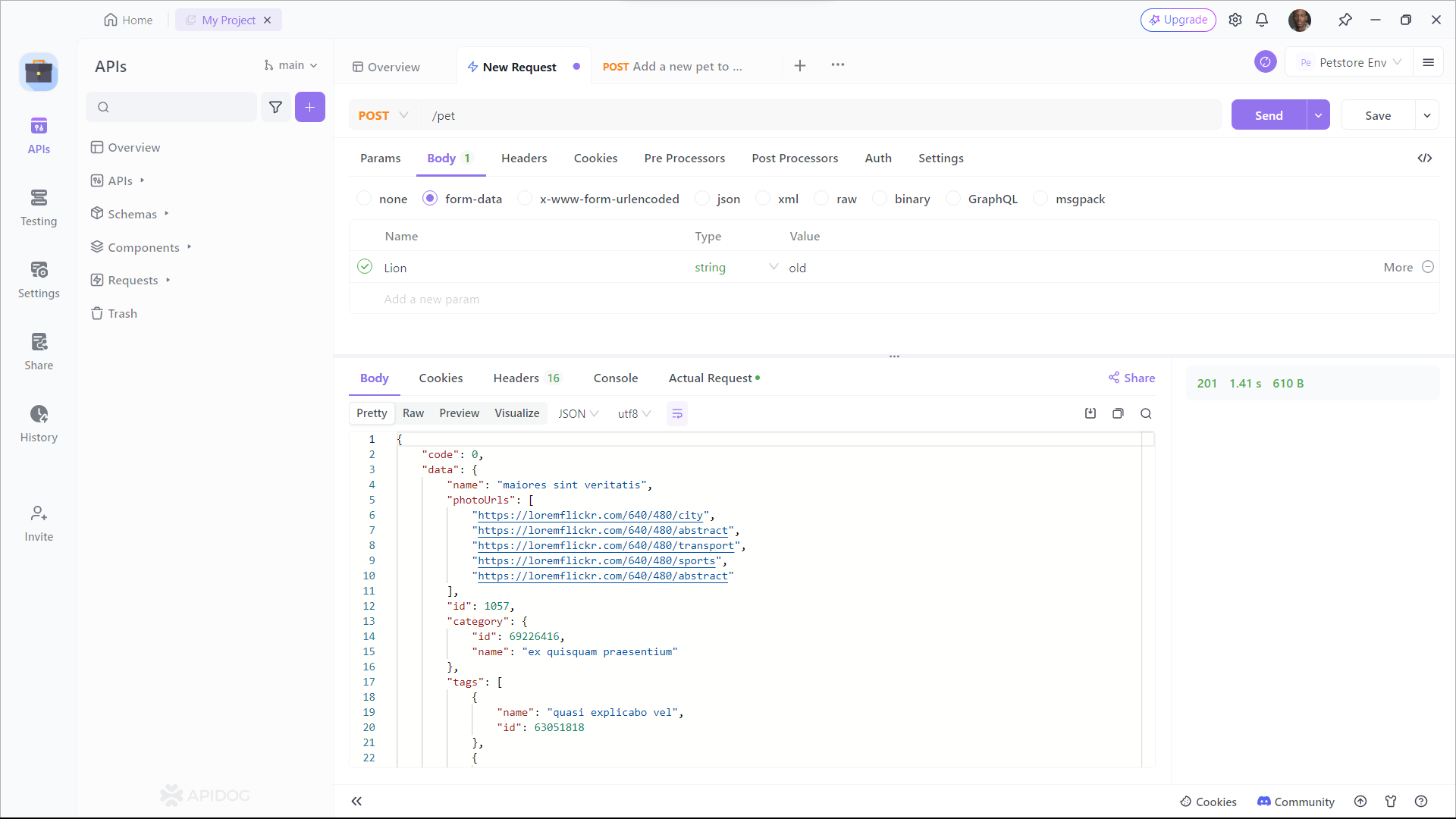
Integrating Apidog with Your GraphQL
Once you have Apidog installed, you can import your GraphQL schema to manage and test your API more efficiently. Navigate to the import section in Apidog and upload your schema.graphqls
file.
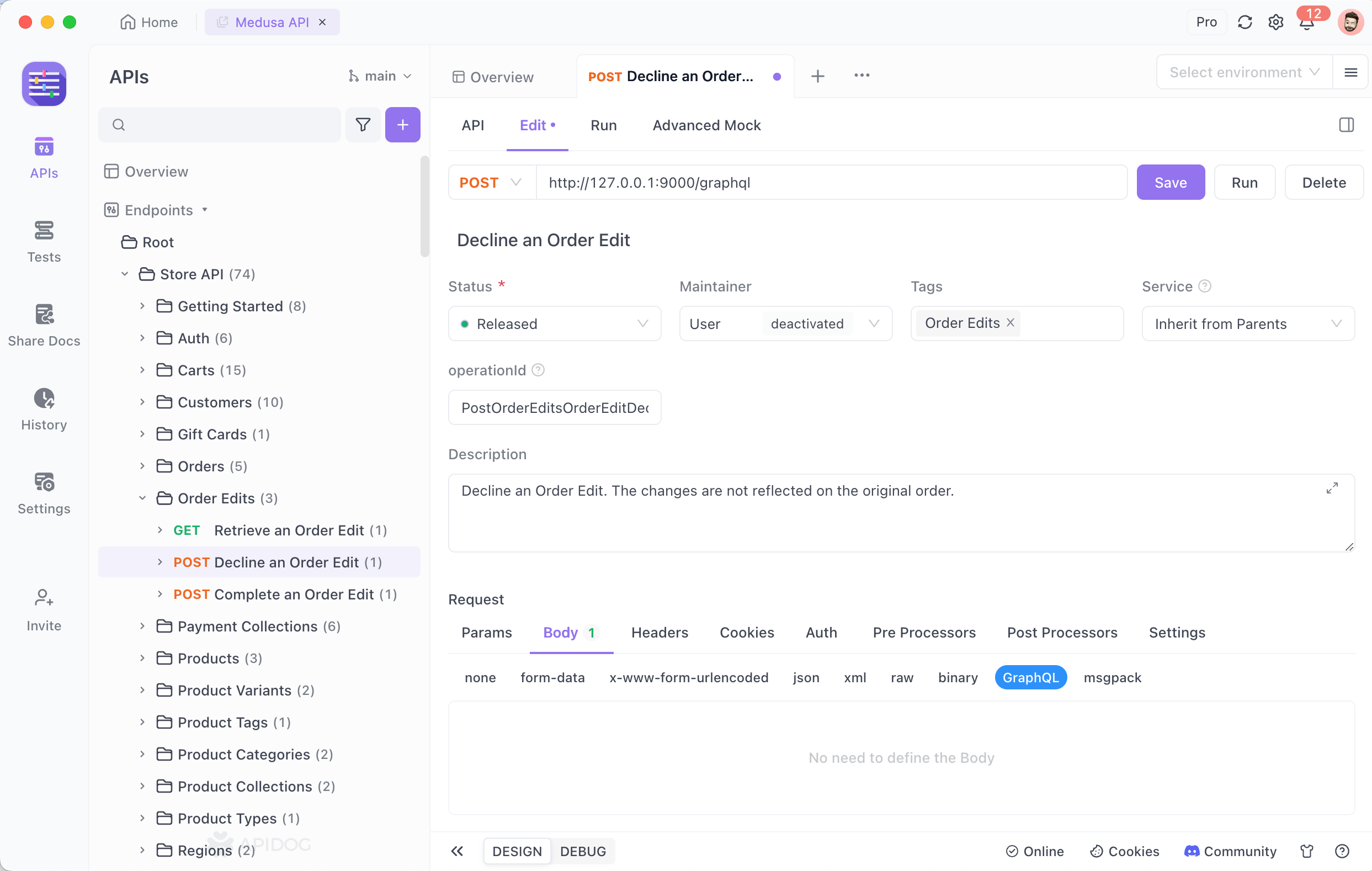
Enter your query in the Query box on the "Run" tab. You can also click the manual Fetch Schema button in the input box to enable the "code completion" feature for Query expressions, assisting in entering Query statements.
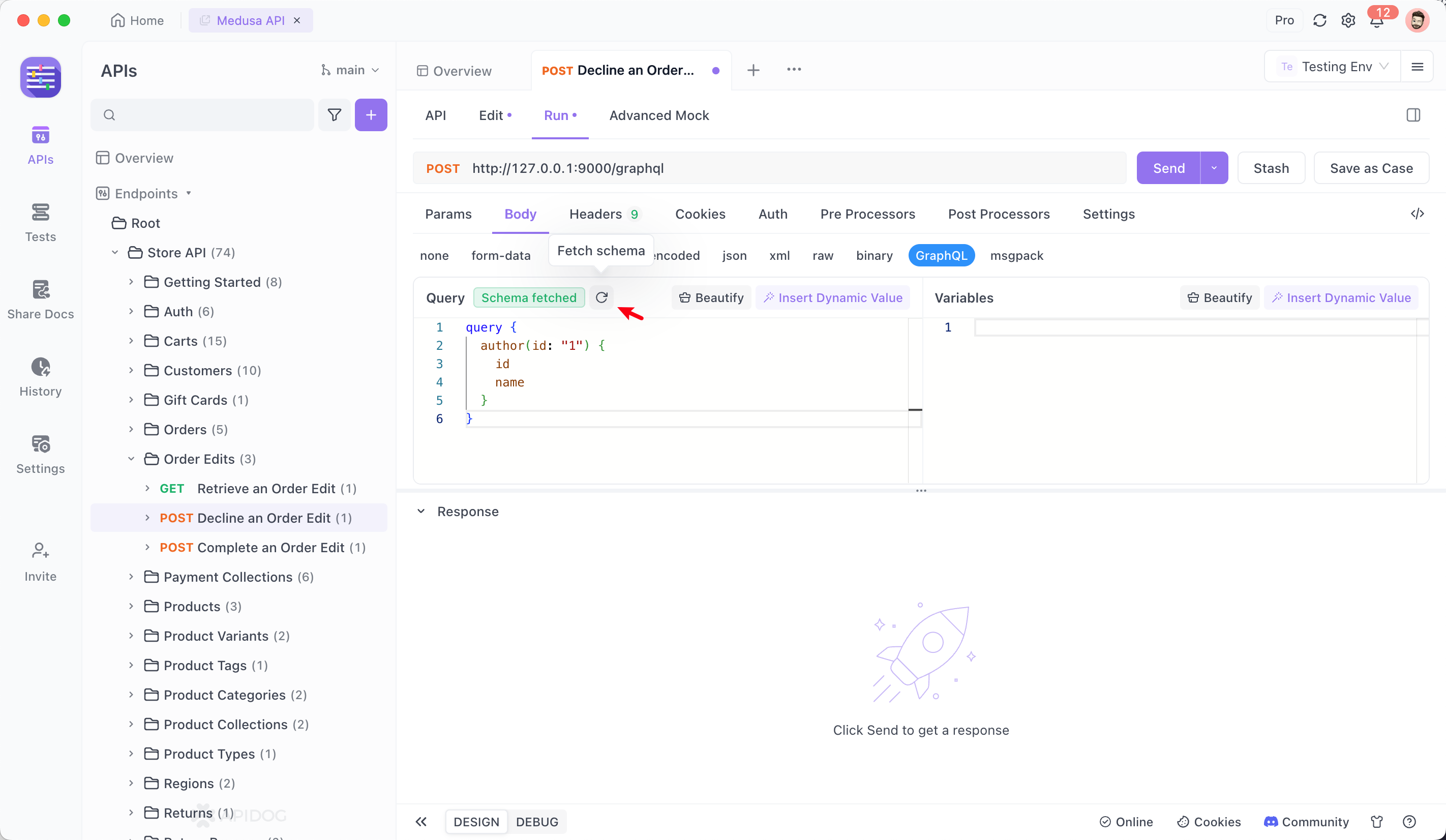
With your schema imported, you can use Apidog to test your queries and mutations, generate documentation, and even mock responses. This will help you ensure your API is working as expected and provide a comprehensive guide for your API users.
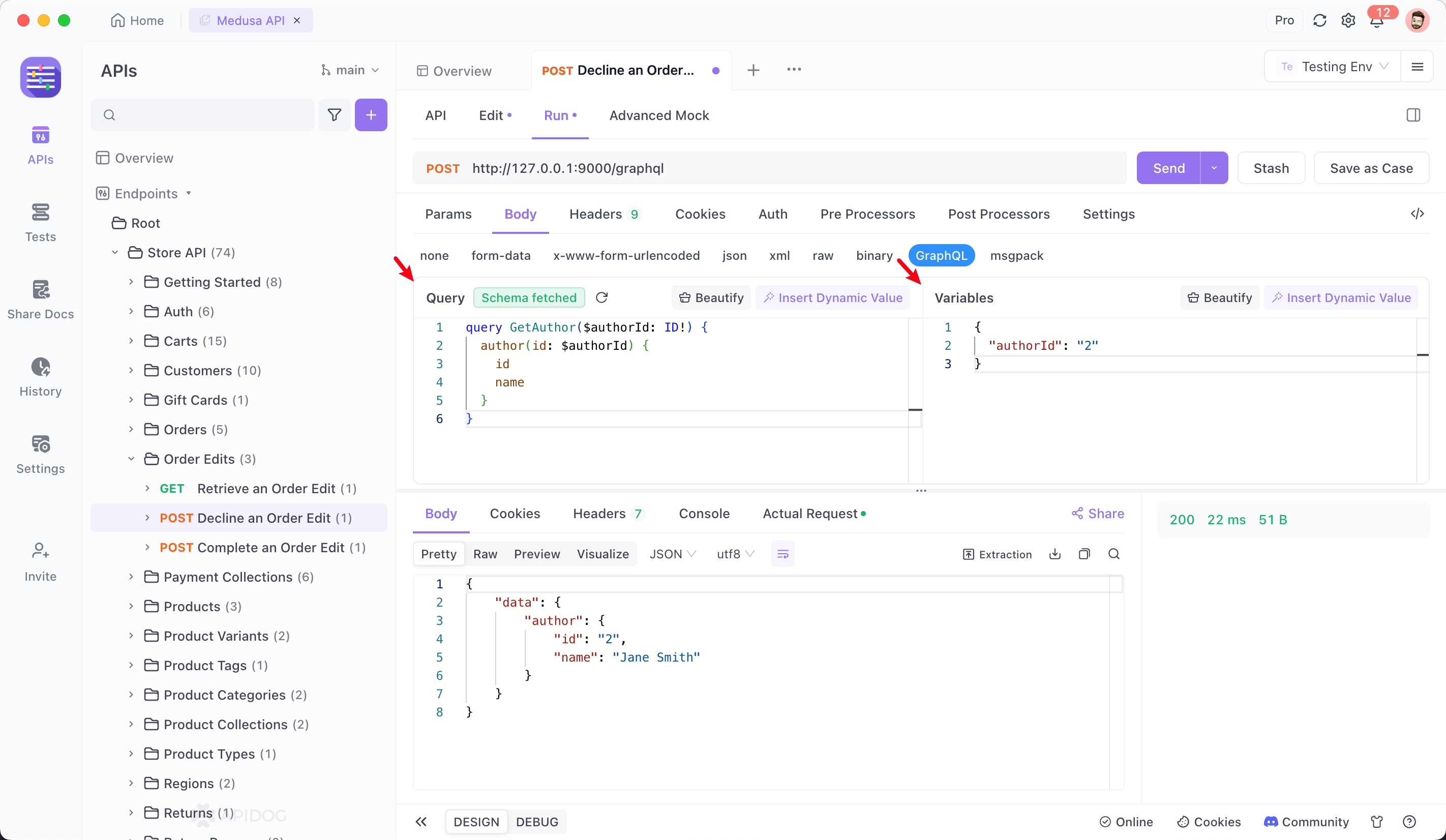
Real-World Applications
Using Axios and GraphQL together, along with a tool like Apidog, opens up a world of possibilities for real-world applications. Here are a few examples of how you can leverage these technologies:
Building a Weather App
Imagine building a weather app that provides real-time weather updates for different cities. With GraphQL, you can query only the data you need (e.g., temperature, humidity, wind speed) for a specific city, and Axios can handle the HTTP requests. Apidog can help you design and test your API, ensuring it works flawlessly.
E-commerce Platform
For an e-commerce platform, you might need to fetch product details, user reviews, and inventory status. GraphQL allows you to request all this information in a single query, and Axios makes it easy to send the request. Apidog can automate testing for different scenarios, such as out-of-stock products or invalid product IDs.
Social Media Dashboard
A social media dashboard might need to pull data from multiple sources, such as user profiles, posts, and comments. GraphQL can aggregate this data into a single response, and Axios can handle the requests. Apidog ensures your API remains reliable by running automated tests.
Best Practices for Using Axios and GraphQL
To get the most out of Axios and GraphQL, keep these best practices in mind:
Optimize Queries
With GraphQL, it's easy to over-fetch data. Always request only the fields you need to minimize the amount of data transferred over the network.
Handle Errors Gracefully
Both Axios and GraphQL provide detailed error information. Use this information to handle errors gracefully and provide meaningful feedback to your users.
Secure Your API
Security is paramount. Use HTTPS to encrypt data in transit, and implement authentication and authorization mechanisms to protect your API endpoints.
Use Interceptors
Leverage Axios' interceptors to add custom logic to your requests and responses. This can include adding authentication tokens, logging requests, or modifying responses.
Stay Up-to-Date
Both Axios and GraphQL are actively maintained. Stay up-to-date with the latest versions to benefit from new features and security patches.
Conclusion
In conclusion, Axios and GraphQL are powerful tools that, when used together, can significantly enhance your API development process. By leveraging the simplicity of Axios for HTTP requests and the flexibility of GraphQL for querying data, you can build more efficient and maintainable applications. Additionally, integrating Apidog into your workflow can further streamline API design, testing, and documentation, ensuring your APIs are robust and reliable.
So, what are you waiting for? Start exploring the world of Axios and GraphQL today, and don't forget to check out Apidog for an even smoother development experience. Download Apidog for free and see how it can revolutionize your API workflow.