Three.jsは、Webブラウザで3Dグラフィックスを作成するための最も人気のあるJavaScriptライブラリの1つです。WebGLの上に構築されており、低レベルのWebGLを学ぶことなく、アニメーションされた3Dコンピュータグラフィックスを作成して表示するプロセスを簡素化します。インタラクティブなビジュアライゼーション、ゲーム、または没入型のWebエクスペリエンスを作成しようとしている場合でも、Three.jsはアイデアを形にするために必要なツールを提供します。
Three.jsの開発に入る前に、APIテストのニーズに対応するためにPostmanの代替としてApidogを試してみることを検討してください。Apidogは、API開発、テスト、ドキュメント作成のための強力な機能を備えた直感的なインターフェースを提供します。多くのユーザーがPostmanでイライラしている機能の肥大化はありません。APIと対話するWebアプリケーションを開発する際に、優れた補助ツールとなります。
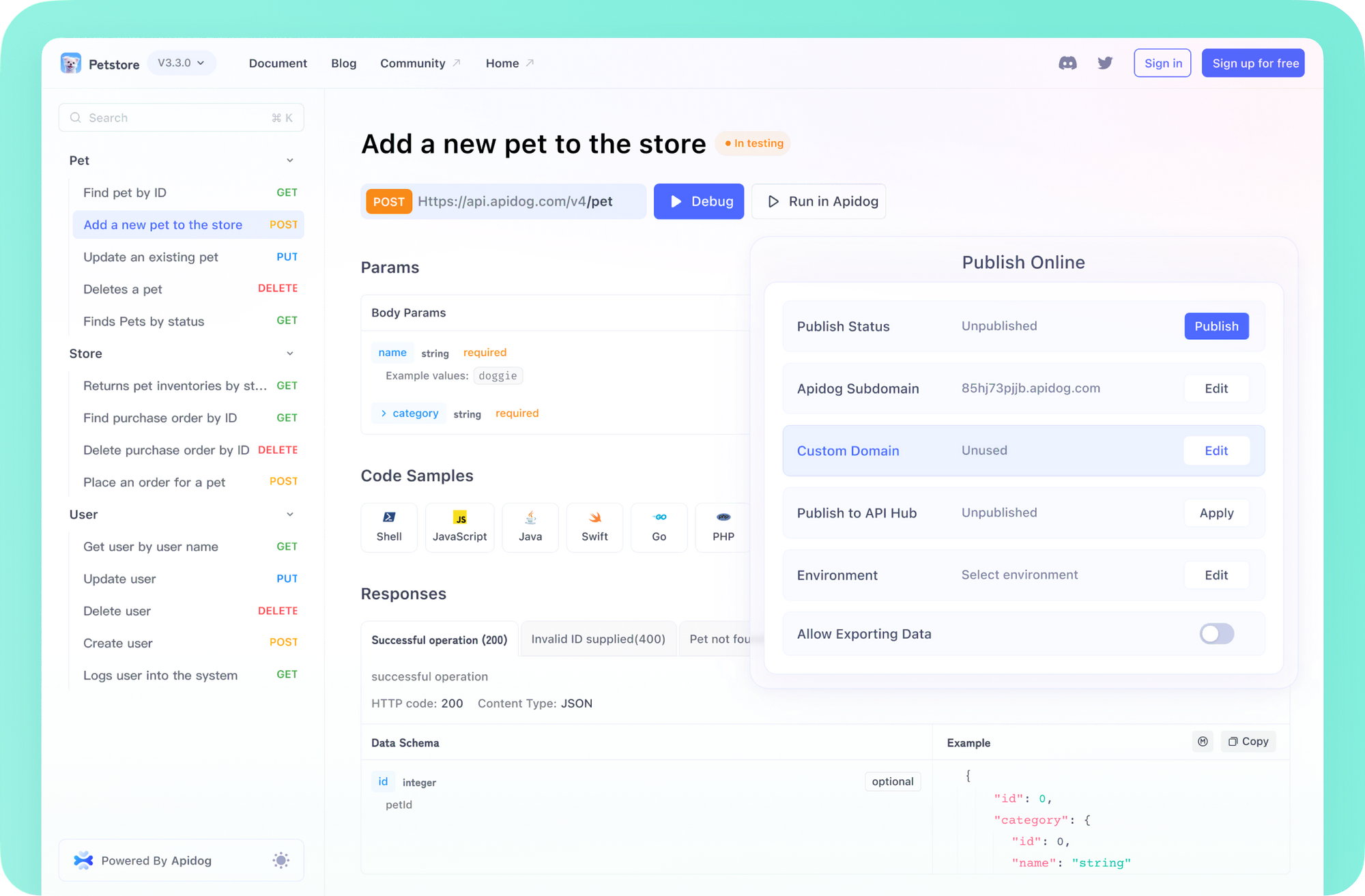
さあ、Three.jsの旅を始めましょう!
1. 環境設定
インストール
Three.jsをプロジェクトに含める方法はいくつかあります:
オプション1: npmを使用(推奨)
npm install three
次に、JavaScriptファイルにインポートします:
import * as THREE from 'three';
オプション2: CDNを使用
これをHTMLファイルに追加してください:
<script src="https://cdn.jsdelivr.net/npm/three@0.157.0/build/three.min.js"></script>
オプション3: ローカルにダウンロードして含める
Three.jsのウェブサイトからライブラリをダウンロードし、HTMLに含めます:
<script src="path/to/three.min.js"></script>
プロジェクト構造
基本的なThree.jsプロジェクトには、以下が必要です:
project-folder/
├── index.html
├── style.css
└── script.js
あなたのindex.html
ファイルには以下を含める必要があります:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>私のThree.jsアプリ</title>
<style>
body { margin: 0; }
canvas { display: block; }
</style>
</head>
<body>
<script src="https://cdn.jsdelivr.net/npm/three@0.157.0/build/three.min.js"></script>
<script src="script.js"></script>
</body>
</html>
2. 基本の理解
Three.jsは、理解するために重要な数種類の主要な概念に基づいています:
コアコンポーネント
- シーン: すべてのオブジェクト、ライト、カメラを保持するコンテナと考えてください。
- カメラ: シーン内のどの部分が見えるのかを定義します(あなたの視点)。
- レンダラー: カメラが見るものを画面に描画します。
- オブジェクト(メッシュ): シーン内の3Dオブジェクトで、通常はジオメトリとマテリアルで構成されています。
- ライト: シーンの照明源です。
最初のシーンの作成
回転する立方体を作成する基本的な例を示します:
// シーンの作成
const scene = new THREE.Scene();
// カメラを作成
const camera = new THREE.PerspectiveCamera(
75, // 視野角
window.innerWidth / window.innerHeight, // アスペクト比
0.1, // 近接クリッピングプレーン
1000 // 遠方クリッピングプレーン
);
camera.position.z = 5;
// レンダラーを作成
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
// 立方体を作成
const geometry = new THREE.BoxGeometry();
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(geometry, material);
scene.add(cube);
// アニメーションループ
function animate() {
requestAnimationFrame(animate);
// 立方体を回転
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
renderer.render(scene, camera);
}
animate();
3. アプリをレスポンシブにする
Three.jsアプリケーションがすべてのデバイスで良く見えるように、ウィンドウのサイズ変更を処理する必要があります:
// ウィンドウのサイズ変更を処理
window.addEventListener('resize', () => {
const width = window.innerWidth;
const height = window.innerHeight;
// レンダラーを更新
renderer.setSize(width, height);
// カメラを更新
camera.aspect = width / height;
camera.updateProjectionMatrix();
});
4. マテリアルとライティングを追加
基本的なシーンは始まりにすぎません。マテリアルとライティングで視覚品質を向上させましょう:
マテリアル
// 反射のある標準マテリアル
const material = new THREE.MeshStandardMaterial({
color: 0x0088ff, // 青色
roughness: 0.5, // やや光沢あり
metalness: 0.5, // やや金属的
});
// 簡単な光沢表面用のフォンマテリアル
const phongMaterial = new THREE.MeshPhongMaterial({
color: 0xff0000, // 赤色
shininess: 100, // 非常に光沢あり
specular: 0x111111 // 鏡面ハイライトの色
});
ライト
// 環境光を追加
const ambientLight = new THREE.AmbientLight(0x404040);
scene.add(ambientLight);
// 照明(太陽のような)を追加
const directionalLight = new THREE.DirectionalLight(0xffffff, 1);
directionalLight.position.set(1, 1, 1);
scene.add(directionalLight);
// ポイントライト(電球のような)を追加
const pointLight = new THREE.PointLight(0xffffff, 1, 100);
pointLight.position.set(10, 10, 10);
scene.add(pointLight);
5. 様々なジオメトリを作成
Three.jsには、多くの組み込みのジオメトリがあります:
// 球体
const sphereGeometry = new THREE.SphereGeometry(1, 32, 32);
const sphere = new THREE.Mesh(sphereGeometry, material);
sphere.position.x = -3;
scene.add(sphere);
// 円柱
const cylinderGeometry = new THREE.CylinderGeometry(1, 1, 2, 32);
const cylinder = new THREE.Mesh(cylinderGeometry, material);
cylinder.position.x = 0;
scene.add(cylinder);
// トーラス(ドーナツ)
const torusGeometry = new THREE.TorusGeometry(0.8, 0.3, 16, 100);
const torus = new THREE.Mesh(torusGeometry, material);
torus.position.x = 3;
scene.add(torus);
6. Three.jsでのテキスト作成
TextGeometryを使用してシーンにテキストを追加できます:
// 最初にフォントをロード
const loader = new THREE.FontLoader();
loader.load('https://threejs.org/examples/fonts/helvetiker_regular.typeface.json', function(font) {
const textGeometry = new THREE.TextGeometry('Hello Three.js!', {
font: font,
size: 0.5,
height: 0.1,
curveSegments: 12,
bevelEnabled: true,
bevelThickness: 0.03,
bevelSize: 0.02,
bevelOffset: 0,
bevelSegments: 5
});
const textMaterial = new THREE.MeshStandardMaterial({ color: 0xffff00 });
const text = new THREE.Mesh(textGeometry, textMaterial);
// テキストを中央に配置
textGeometry.computeBoundingBox();
const textWidth = textGeometry.boundingBox.max.x - textGeometry.boundingBox.min.x;
text.position.x = -textWidth / 2;
scene.add(text);
});
7. 線の描画
Three.jsで線を作成してワイヤーフレームやパスのビジュアライゼーションを行えます:
// 線のためのポイントを作成
const points = [];
points.push(new THREE.Vector3(-2, 0, 0));
points.push(new THREE.Vector3(0, 2, 0));
points.push(new THREE.Vector3(2, 0, 0));
// 線ジオメトリを作成
const lineGeometry = new THREE.BufferGeometry().setFromPoints(points);
// マテリアルを作成
const lineMaterial = new THREE.LineBasicMaterial({ color: 0xffffff });
// 線を作成
const line = new THREE.Line(lineGeometry, lineMaterial);
scene.add(line);
8. インタラクションとコントロール
ユーザーが3Dシーンとインタラクトできるようにするために、コントロールを追加できます:
// OrbitControlsをインポート
import { OrbitControls } from 'three/examples/jsm/controls/OrbitControls.js';
// コントロールを作成
const controls = new OrbitControls(camera, renderer.domElement);
controls.enableDamping = true; // スムーズダンピング効果を追加
controls.dampingFactor = 0.05;
// アニメーションループを更新
function animate() {
requestAnimationFrame(animate);
// コントロールを更新
controls.update();
renderer.render(scene, camera);
}
9. 3Dモデルの読み込み
Blenderのようなソフトウェアで作成されたモデルをインポートできます:
import { GLTFLoader } from 'three/examples/jsm/loaders/GLTFLoader.js';
const loader = new GLTFLoader();
loader.load(
'path/to/model.gltf',
// 読み込み完了時に呼び出される
function(gltf) {
scene.add(gltf.scene);
},
// 読み込み中に呼び出される
function(xhr) {
console.log((xhr.loaded / xhr.total * 100) + '% 読み込み完了');
},
// エラー時に呼び出される
function(error) {
console.error('エラーが発生しました', error);
}
);
10. デバッグとパフォーマンスのヒント
ステータスパネル
import Stats from 'three/examples/jsm/libs/stats.module.js';
const stats = new Stats();
document.body.appendChild(stats.dom);
function animate() {
requestAnimationFrame(animate);
stats.begin();
// ここにアニメーションコードを記述
stats.end();
renderer.render(scene, camera);
}
パフォーマンスのヒント
- Geometryの代わりにBufferGeometryを使用(非推奨)
- 可能な限りマテリアルとジオメトリを再利用
- より良いGPU使用のために
renderer.powerPreference = 'high-performance'
を設定 Object3D.frustumCulled = true
を使用してカメラビューの外にあるオブジェクトを非表示にする- 可能な限りポリゴン数を減らしてモデルの複雑さを最適化
結論
これで、環境設定からインタラクティブな3Dシーンの作成まで、Three.jsの基本を学びました。これは始まりに過ぎません。Three.jsは、驚くべき3D Webエクスペリエンスを作成するためのさまざまな機能を提供しています。
知識を深めるために、公式のThree.jsドキュメントと例ギャラリーを探索してください。コミュニティもフォーラムやGitHubで活発に活動しており、すべてのレベルの開発者を対象としたリソースやサポートを提供しています。
覚えておいてください。学ぶ最良の方法は実験です。簡単なプロジェクトから始めて、徐々により複雑なシーンに進んでください。練習と忍耐で、すぐに印象的な3D Webエクスペリエンスを作成できるようになるでしょう!
Three.jsでのコーディングを楽しんでください!