Hey there, fellow web developers! Today, we're going to dive into the world of XMLHttpRequest (XHR), a powerful tool that has revolutionized the way we interact with web APIs and build dynamic web applications. Buckle up, because we're about to embark on a journey through the fundamentals, practical applications, and advanced techniques of XHR.
First, let's start with a brief overview. XMLHttpRequest is a built-in JavaScript object that allows you to make HTTP requests to a server and handle the response asynchronously, without reloading the entire web page. This means you can fetch data, update content, and create seamless user experiences, all while keeping your application responsive and efficient.
The importance of XHR in modern web development cannot be overstated. With the rise of APIs and the increasing demand for real-time data, XHR has become a fundamental building block for creating rich, interactive web applications. From social media feeds to e-commerce platforms, XHR is the unsung hero that powers the dynamic features we've come to expect.
In this blog post, we'll cover the following:
- Understanding the Basics of XMLHttpRequest
- Making API Calls with XMLHttpRequest
- Advanced Techniques in XMLHttpRequest
- Using Apidog to Generate XMLHttpRequest Code
Understanding the Basics of XMLHttpRequest
Before we dive into the nitty-gritty of XHR, let's take a step back and understand what it is and how it fits into the web APIs ecosystem. XMLHttpRequest was initially introduced by Microsoft in the late 1990s as an ActiveX object and later adopted by other browsers as a standard API. Despite its name, XHR can handle data formats beyond XML, including JSON, which has become the de facto standard for API communication.
The basic syntax of XHR is relatively straightforward. You create a new instance of the XMLHttpRequest object, configure the request (method, URL, headers, etc.), define event handlers to handle the response, and finally send the request. XHR provides several methods and properties to interact with the request and response data, such as open()
, send()
, onreadystatechange
, and status
.
Making API Calls with XMLHttpRequest
Now that we've covered the basics, let's dive into a practical example of making an API call with XMLHttpRequest. We'll walk through a step-by-step guide on how to fetch data from an external API and update the DOM with the retrieved information.
First, we'll create a new instance of the XMLHttpRequest object and configure the request:
const xhr = new XMLHttpRequest();
xhr.open('GET', 'https://api.example.com/data', true);
Next, we'll define an event handler to handle the response when it's ready:
xhr.onreadystatechange = function() {
if (xhr.readyState === XMLHttpRequest.DONE && xhr.status === 200) {
// Handle the response data here
const data = JSON.parse(xhr.responseText);
// Update the DOM with the retrieved data
document.getElementById('result').innerHTML = data.message;
}
};
Finally, we'll send the request:
xhr.send();
In this example, we're making a GET
request to https://api.example.com/data
. When the response is ready, we parse the JSON data and update the DOM with the message
property.
While this example is straightforward, there are common pitfalls to be aware of, such as handling errors, setting appropriate headers (e.g., for authentication), and managing cross-origin resource sharing (CORS) when making requests to external domains.
Advanced Techniques in XMLHttpRequest
As you become more comfortable with XHR, you'll likely encounter scenarios that require more advanced techniques. In this section, we'll explore working with different data formats, managing asynchronous operations with callbacks, and handling cross-origin resource sharing.
Working with different data formats:
While JSON is the most common data format used in API communication, you may encounter scenarios where you need to handle XML or other formats. XHR provides methods like responseXML
and overrideMimeType()
to work with different data formats.
Managing asynchronous operations with callbacks:
XHR is inherently asynchronous, which means that your code needs to handle the response when it's ready, rather than blocking the execution flow. This is typically achieved using callbacks or, in more modern JavaScript, Promises and async/await.
Cross-origin resource sharing (CORS):
When making requests to external domains, you may encounter CORS restrictions that prevent your XHR requests from succeeding. To overcome this, you'll need to configure the server to allow cross-origin requests or use alternative techniques like server-side proxies or JSONP (for older browsers).
Use Apidog to Generate XMLHttpRequest Code
While XHR is a powerful tool, writing the code from scratch can be time-consuming and error-prone, especially for complex API interactions. That's where Apidog comes in – a handy tool that can generate XMLHttpRequest code for you, saving you valuable development time.
Here's the process for using Apidog to generate Axios code:
Step 1: Open Apidog and select new request
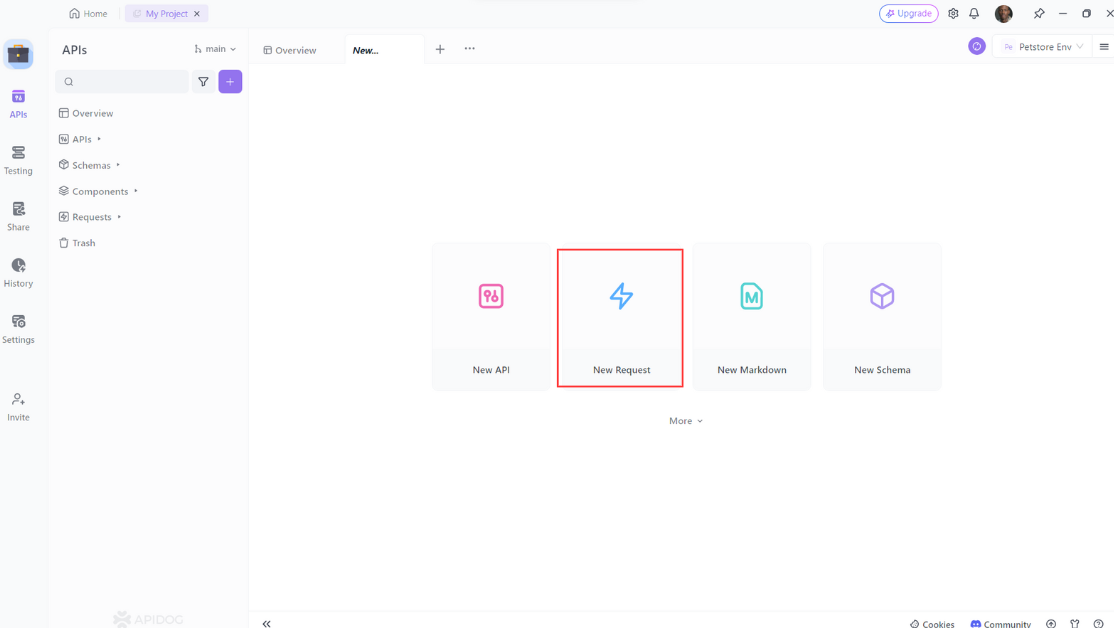
Step 2: Enter the URL of the API endpoint you want to send a request to,input any headers or query string parameters you wish to include with the request, then click on the "Design" to switch to the design interface of Apidog.
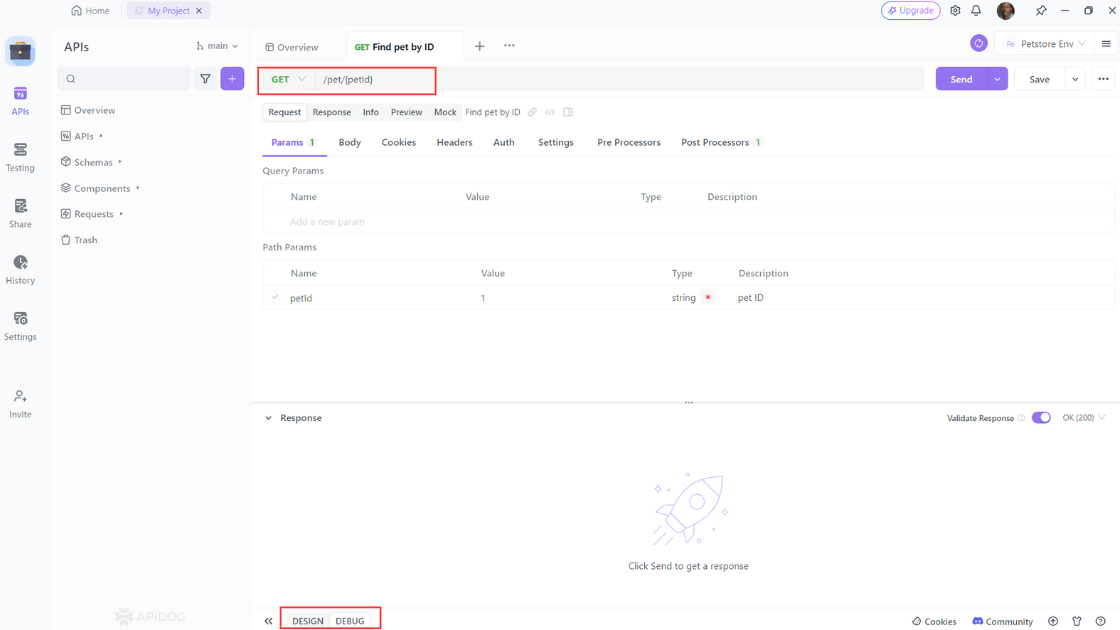
Step 3: Select "Generate client code " to generate your code.
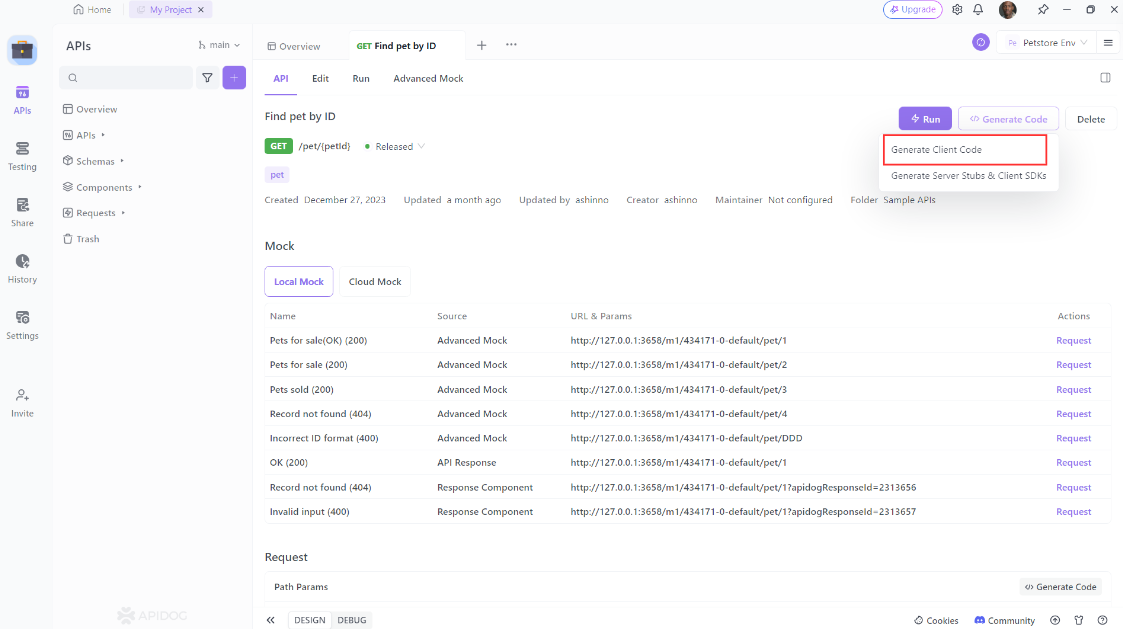
Step 4: Copy the generated Axios code and paste it into your project.
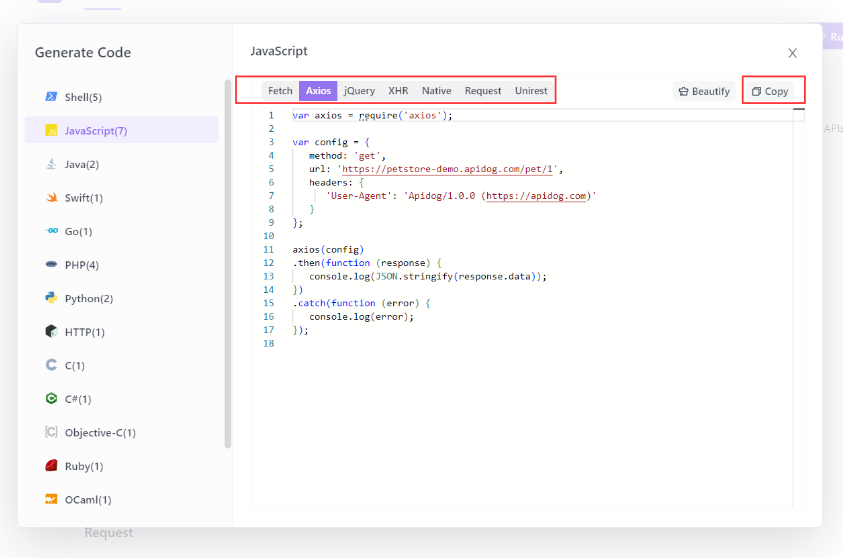
Using Apidog, you can focus on building your application logic rather than worrying about the low-level details of API communication. It's a game-changer for developers who want to streamline their workflow and boost productivity.
Conclusion
XMLHttpRequest has been a cornerstone of modern web development, enabling developers to build rich, interactive web applications that leverage the power of APIs and real-time data. From understanding the basics to mastering advanced techniques, XHR is a versatile tool that every web developer should have in their arsenal.
While XHR has served us well, the future of API interactions in JavaScript lies with the Fetch API and newer standards like HTTP/3. These modern APIs provide a more streamlined and efficient way of making network requests, addressing some of the limitations and complexity of XHR.
However, XHR remains a crucial part of the web development ecosystem, and mastering it will not only deepen your understanding of how the web works but also prepare you for the next generation of API interactions.
So, what are you waiting for? Start practicing with XMLHttpRequest today, and don't forget to check out Apido – your secret weapon for generating efficient XHR code and streamlining your API integration workflow.
Happy coding!