WebSockets are a powerful communication protocol that enables real-time, two-way data exchange between web browsers and servers. This constant connection streamlines communication for various applications, including live chat features, stock tickers, and collaborative editing tools. However, network interruptions or server-side issues can occasionally lead to connection breakage.
With Apidog, you can also test, mock, and document the WebSocket API all within a single application. To learn more about Apidog, do not miss clicking the button below.
To ensure uninterrupted data flow and maintain application functionality, WebSocket reconnect mechanisms are implemented. These mechanisms automatically attempt to re-establish the connection upon encountering a disconnect, minimizing disruption for the user and ensuring data continues to be exchanged seamlessly.
Why does WebSocket Disconnect?
WebSockets are known to be fragile due to their sensitivity to network disruptions and other issues that cause connections to drop. here are a few common causes and their impact on WebSockets:
Network Issues
Unstable or unreliable Internet connection: Fluctuations in network quality, weak Wi-Fi signals, or packet loss can lead to connection breaks. This is especially common for mobile users on cellular data.
Firewall or proxy interference: Security measures like firewalls or proxy servers might unintentionally block WebSocket connections due to misconfigured rules.
Network congestion: During periods of high network traffic, data packets can be delayed or dropped, leading to disconnects.
Server-Side Problems
Server overload: A surge in user traffic or resource-intensive server processes can overload the server, causing it to drop connections.
Server crashes or restarts: Unexpected server crashes or scheduled maintenance can abruptly terminate WebSocket connections.
Application bugs: Software bugs within the server-side application handling WebSockets can lead to unexpected disconnects.
Client-Side Issues
Browser crashes or extensions: Browser crashes or malfunctioning extensions can disrupt WebSocket connections.
JavaScript errors: Bugs within the client-side JavaScript code responsible for managing the WebSocket connection can lead to disconnects.
Tab/window closure: Users closing the browser tab or window hosting the application can intentionally terminate the connection.
Impact of Disconnects
Disrupted user experience: Disconnects can cause interruptions in real-time data flow, impacting features like live chat, stock tickers, or collaborative editing. This can lead to frustration and a loss of productivity for users.
Data loss: If disconnects occur during data transmission, partial messages or entire data packets may be lost, causing inconsistencies in the application state.
Resource strain: Frequent reconnection attempts can put additional strain on server resources and potentially lead to further instability.
WebSocket Reconnect Strategies
WebSocket reconnect mechanisms are crucial for maintaining reliable communication in the face of disconnects. Here, we explore various strategies with their advantages and disadvantages to help you choose the most suitable approach for your application:
Immediate Reconnect
This strategy attempts to re-establish the WebSocket connection immediately upon detecting a disconnect. It doesn't involve any delay between retries.
Advantages
Fast recovery: This approach prioritizes minimizing downtime by attempting to reconnect as quickly as possible. Users experience minimal disruption, especially for short-lived disconnects.
Simplicity: Implementation is straightforward, requiring minimal code to trigger reconnection upon encountering a closed connection event.
Disadvantages
Server overload: During widespread outages or server issues, a flood of immediate reconnection attempts can overwhelm the server, causing further instability.
Potential for thrashing: If the server remains unavailable for an extended period, continuous retries without backoff can lead to a cycle of connection attempts and immediate failures, putting unnecessary strain on both client and server resources.
Exponential Backoff
This strategy implements a gradual increase in the wait time between reconnect attempts after each failed attempt. This approach aims to balance responsiveness with server resource management.
Advantages
Reduced server load: By increasing the delay between retries, exponential backoff prevents overwhelming the server with reconnection requests during outages.
Eventual reconnection: This strategy ensures that the client will eventually re-establish the connection even if the server is down for an extended period.
Disadvantages
Slower recovery: Compared to immediate reconnect, users might experience longer periods of disconnection, especially after the first few failed attempts. This can be undesirable for applications requiring real-time data with minimal latency.
Potential data gaps: Disconnects lasting longer than the backoff intervals can lead to missed data updates on the client side.
Custom Logic
This approach allows developers to tailor the reconnect behavior to the specific needs of their application.
Considerations
Retry limits: Implement a maximum number of retries to prevent infinite loops in case the server remains persistently unavailable.
Timeouts: Set a timeout for each reconnection attempt to avoid waiting indefinitely for unresponsive servers.
Server status checks (optional): If the server exposes an API endpoint indicating its availability, the client can check its status before attempting reconnection. This can be helpful in avoiding unnecessary retries when the server is intentionally down for maintenance.
Best Practices for WebSocket Reconnection
Implementing robust WebSocket reconnect mechanisms involves more than just choosing a strategy. Here are some best practices to ensure a smooth user experience and optimal resource utilization:
User Feedback and Transparency
Visual Cues: Inform users about connection status through clear visual cues. Display messages or icons indicating a disconnect, ongoing reconnection attempts, and successful reconnection.
Error Handling: Provide informative error messages in case reconnection fails after exceeding retry limits or timeouts. This helps users understand the situation and take appropriate actions (e.g., refreshing the page or contacting support).
Managing Retries and Timeouts
Retry Limits: Define a maximum number of retries to prevent the client from endlessly attempting to reconnect to an unavailable server. This avoids unnecessary resource usage on both the client and server sides.
Timeouts: Set a reasonable timeout value for each reconnection attempt. Â This prevents the client from waiting indefinitely for a response from an unresponsive server. You can adjust the timeout based on the expected server response time or application requirements.
Server Load Management
Exponential Backoff: As discussed earlier, utilize exponential backoff to space out reconnection attempts after failures. This helps to avoid overwhelming the server with a surge of reconnect requests during outages.
Monitoring and Logging
Logging: Implement logging mechanisms to track reconnect attempts, successes, and failures. This data can be invaluable for troubleshooting connection issues and identifying potential bottlenecks.
Monitoring: Consider monitoring tools to visualize reconnection behavior and server health over time. This can help identify recurring issues and optimize reconnect strategies for better performance.
Leverage Existing Libraries
WebSocket Libraries: Many popular JavaScript libraries for WebSockets offer built-in reconnect functionality. These libraries often handle common scenarios and provide configuration options for customizing retry behavior and timeouts. Explore these options to simplify implementation and benefit from maintained code.
Apidog - Start Creating WebSocket APIs
WebSocket APIs are very useful for creating applications that require real-time, two-way communication between browsers and servers. To accommodate WebSocket APIs, you will need to have a proper API tool. This is where Apidog comes in.
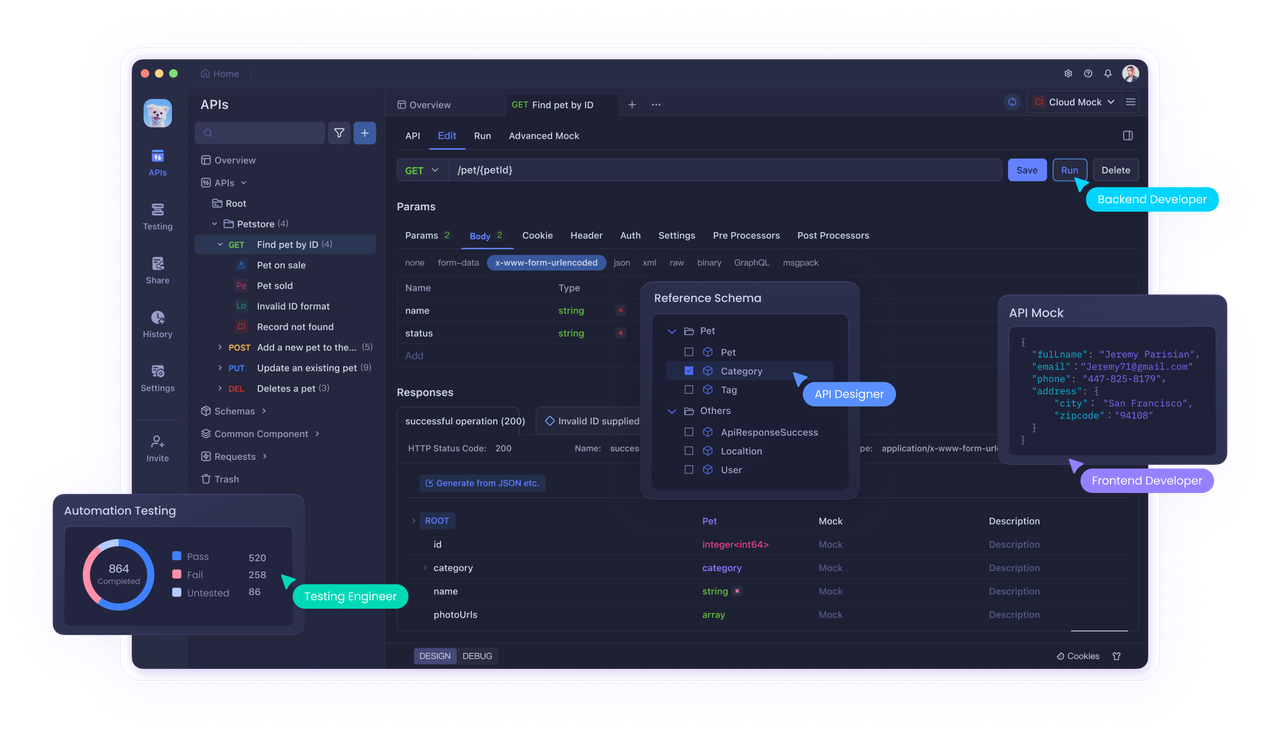
Creating a WebSocket API Using Apidog
You can easily begin creating a WebSocket API in an HTTP project.
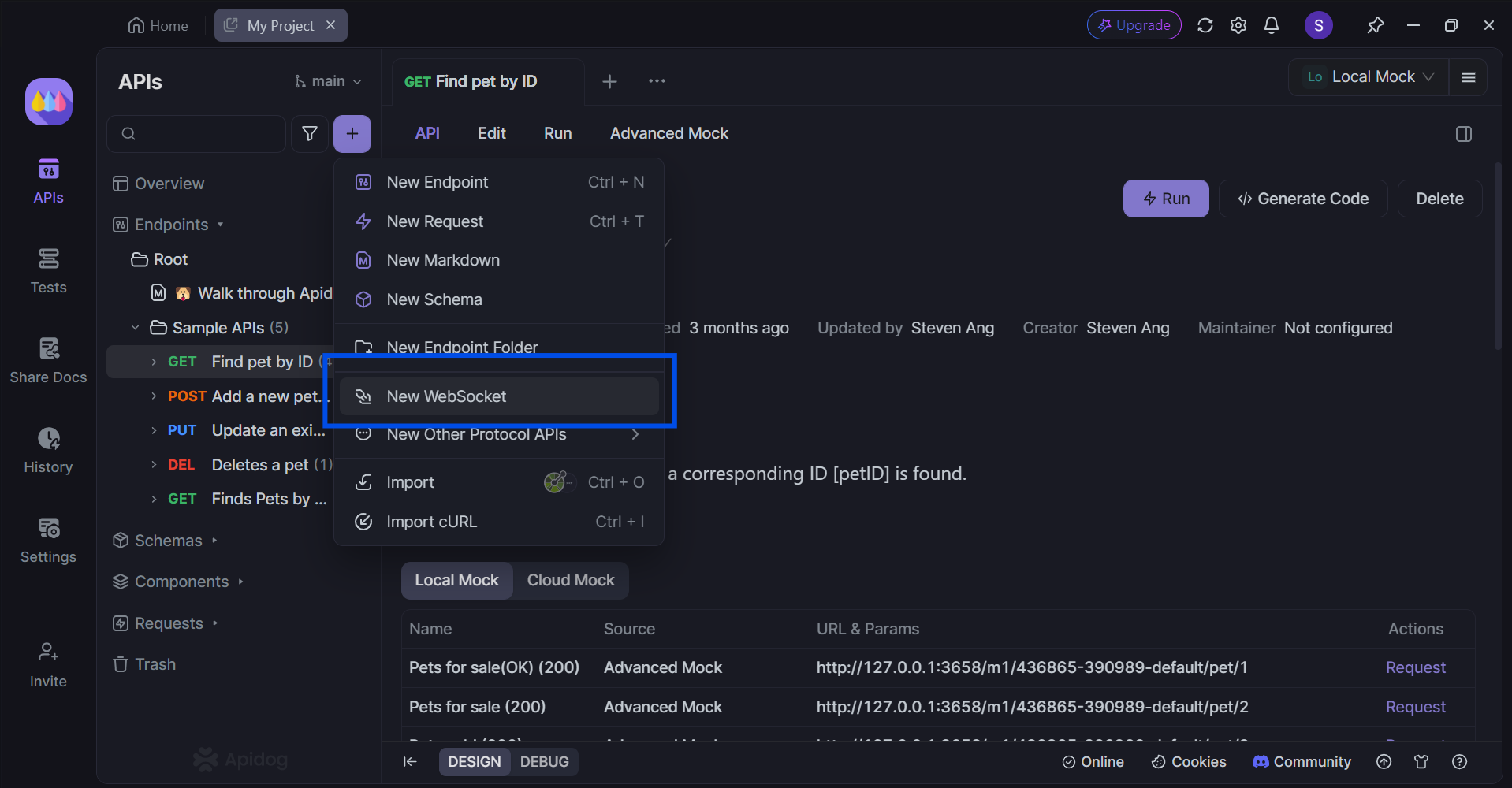
First, create a new API, and hover above the purple +
button, as shown in the image above. This will show a drop-down menu. Proceed by selecting New WebSocket
.
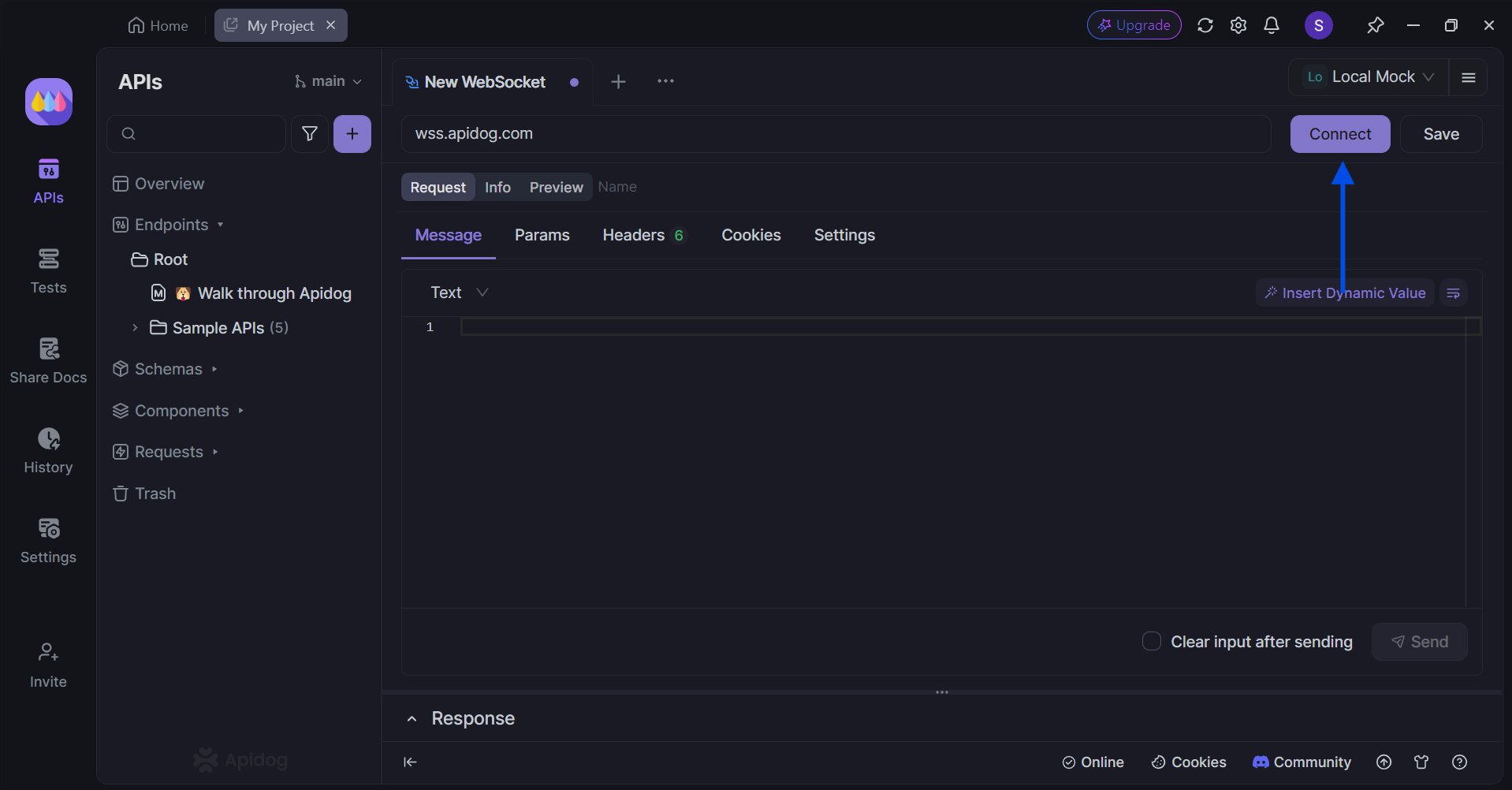
Once you have included the URL, press the Connect
button to establish a WebSocket connection.
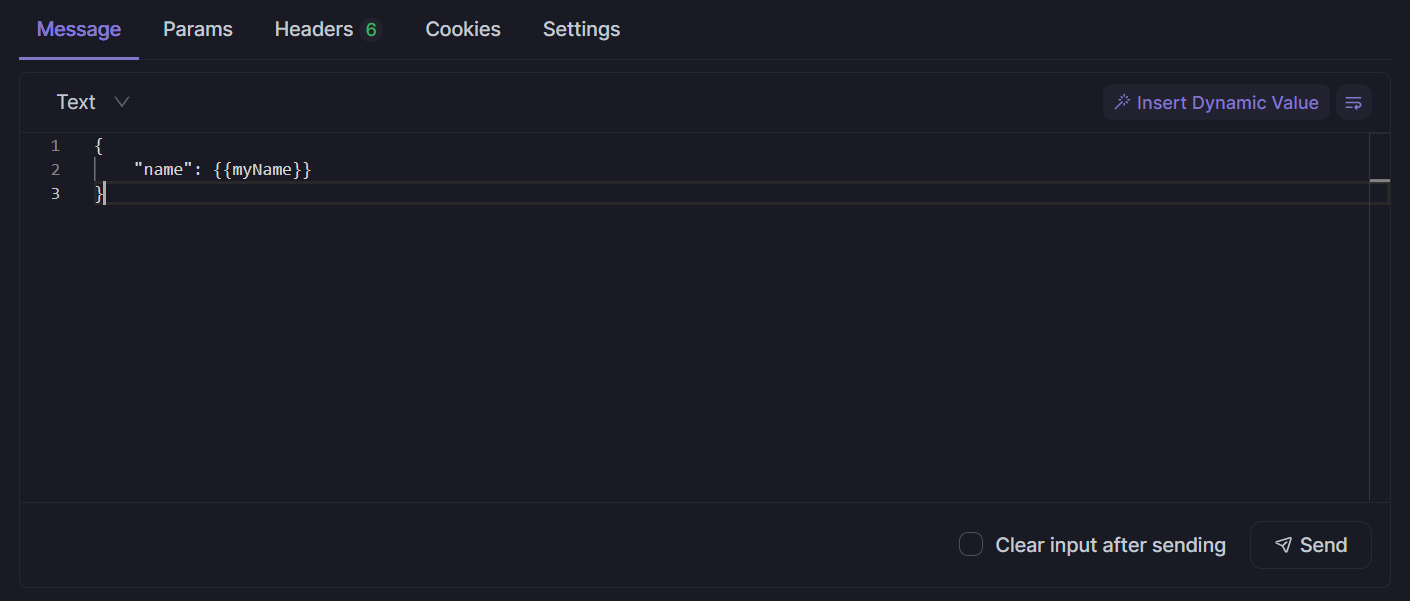
Finally, you can compose a message that you wish to send over. This includes text formats such as Text
, JSON
, XML
, and HTML
. You can also compose them in binary formats using Base64
or Hexadecimal
.
Apidog will syntax highlight the message content based on the selected message format. If the message is in JSON
, XML
, or HTML
format, you can also format the input content.
Adding Handshake Request Parameters
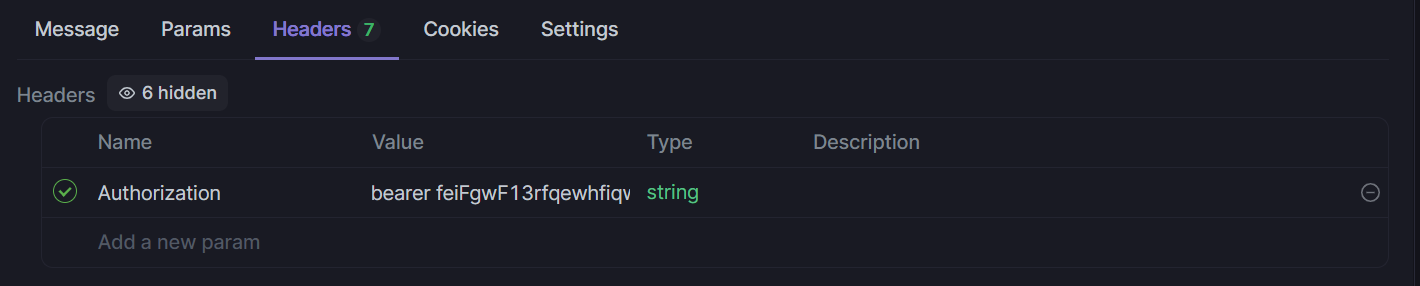
With Apidog, you can also customize the parameters required to pass during WebSocket handshakes, such as Params
,Headers
,Cookies
to meet authentication or other complex scenarios.
Conclusion
WebSocket reconnect mechanisms play a critical role in ensuring reliable and seamless real-time communication for web applications. By understanding the causes of disconnects and the various reconnect strategies available, developers can implement robust solutions that minimize disruption for users and maintain a smooth data flow. Â Choosing the right approach involves balancing responsiveness with server load management. Â
Consider factors like application requirements, latency tolerance, and server resource constraints when selecting and configuring your reconnect strategy. Additionally, following best practices for user feedback, retry management, and monitoring will further enhance the overall user experience and application stability. By employing these techniques, developers can leverage the full potential of WebSockets to create dynamic and engaging web applications.