Hey there, Java enthusiasts! In today's interconnected world, APIs (Application Programming Interfaces) are the secret sauce that powers many of the cool applications we use daily. But have you ever wondered how these APIs work behind the scenes and how you can leverage them in your own Java projects? Well, fret no more! This post is your one-stop guide to conquering APIs in Java.
What exactly is an API?
Think of an API as a friendly waiter at a restaurant. You (the developer) tell the waiter (the API) what you want (data, functionality) from the kitchen (the web service), and the waiter delivers it to your table (your program). APIs provide a structured way for your Java code to communicate with external services and exchange information.
Why Use APIs in Java?
There are several compelling reasons to embrace APIs in your Java projects:
- Boost Functionality: APIs grant you access to a vast array of features without reinventing the wheel. Imagine needing weather data or social media integration – instead of building it yourself, you can leverage existing APIs.
- Save Time and Resources: Why waste time building something that's already readily available? APIs empower you to focus on your core application logic, saving valuable development time and resources.
- Stay Updated: APIs are constantly evolving, keeping your applications at the forefront of technology advancements. No need to constantly rewrite code to stay current – the API takes care of that.
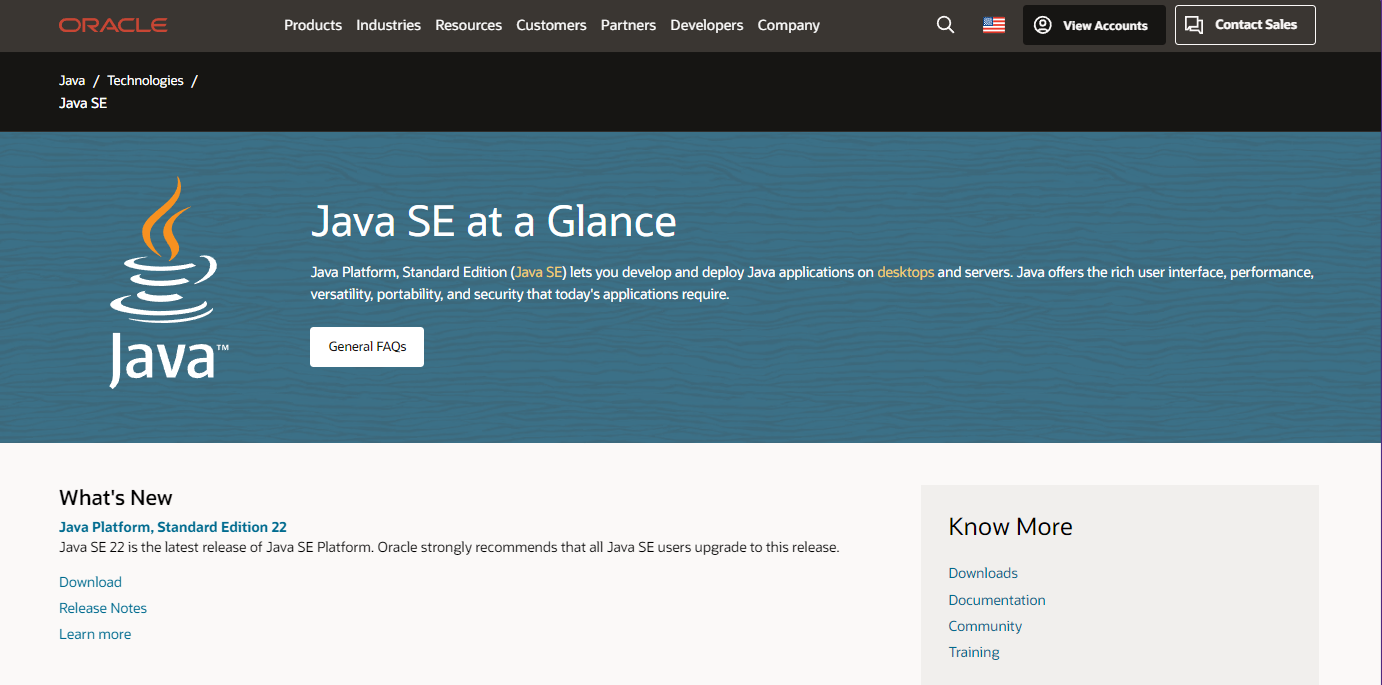
Getting Started with APIs in Java
Now that you're pumped up about APIs, let's dive into the practical steps of using them in your Java code:
Find Your Perfect API: The web is brimming with APIs catering to diverse needs. Explore popular API directories like RapidAPI to discover APIs that align with your project's requirements.
Devour the API Documentation: Every API comes with its own set of instructions – the API documentation. This holy grail provides crucial details like available endpoints (specific URLs for accessing data), request formats (how to structure your requests), response formats (how data is returned), and authentication methods (how to gain access to the API). Consider the documentation your best friend – read it thoroughly to avoid any hiccups.
Set Up Your Java Environment: Ensure you have a robust Java Development Kit (JDK) installed on your system. You can download it from the official Java website Java SE: https://www.oracle.com/java/technologies/javase/. Popular IDEs like Eclipse or IntelliJ IDEA also come bundled with JDKS, making setup a breeze.
Choose Your HTTP Client Library: To interact with APIs, you'll need an HTTP client library. These libraries handle the nitty-gritty of sending requests and receiving responses over the internet. Common choices include the built-in HttpURLConnection
class, Apache HttpClient, or the user-friendly OkHttp library.
Making Your First API Call in Java
Here's a breakdown of the typical workflow for making an API call in Java using OkHttp (remember to replace placeholders with your specific API details):
- Import Necessary Libraries:Java
import okhttp3.*;
- Construct the URL:Java
String url = "https://api.example.com/data"; // Replace with actual API endpoint
- Create an HTTP Client:Java
OkHttpClient client = new OkHttpClient();
- Build the Request:Java
Request request = new Request.Builder()
.url(url)
.build();
- Execute the Request and Process the Response:Java
try (Response response = client.newCall(request).execute()) {
if (response.isSuccessful()) {
String responseData = response.body().string();
// Parse the response data (JSON, XML, etc.) and use it in your program
} else {
// Handle errors!
}
} catch (Exception e) {
// Handle exceptions!
}
Parsing the API Response
The format of the data returned by an API can vary. Popular formats include JSON (JavaScript Object Notation) and XML (Extensible Markup Language). To make sense of this data, you'll need to use parsing libraries like Jackson (for JSON) or XStream (for XML) to convert the raw response into a format your Java program can understand.
Handling Complex JSON Responses
When dealing with APIs, you’ll often encounter complex JSON structures. It’s crucial to understand how to navigate and extract the data you need. Using a library like Jackson, you can deserialize complex JSON responses into Java objects. Here’s an example of how you might do this:
import com.fasterxml.jackson.databind.ObjectMapper;
// Assume 'response' is the JSON string you received from the API
ObjectMapper mapper = new ObjectMapper();
MyResponseObject responseObject = mapper.readValue(response, MyResponseObject.class);
// Now 'responseObject' contains the structured data from the JSON response
Managing Asynchronous Requests
As your application grows, you’ll need to handle multiple API calls simultaneously. Java’s CompletableFuture
class allows you to work with asynchronous programming patterns. This way, you can make non-blocking API calls that won’t freeze your application while waiting for a response.
Efficient API Calls
Efficiency is key when making API calls. You want to minimize the load on both your application and the API server. Here are some tips:
- Cache responses when possible to avoid unnecessary calls.
- Use webhooks if the API supports them, to get notified of changes instead of polling.
- Limit the data you request to only what you need by using API parameters.
Advanced Authentication Techniques
For APIs that require authentication, you might need to go beyond basic API keys. OAuth is a common authentication standard that provides more security and control. Implementing OAuth in Java can be complex, but libraries like ScribeJava can simplify the process.
Monitoring and LoggingKeep an eye on your API usage. Use monitoring tools to track the performance and health of your API integrations. Logging is also essential; make sure to log your API requests and responses to troubleshoot issues when they arise.
How to test your JAva API with Apidog
Testing your Java API with Apidog can streamline the process and ensure that your API functions as expected. Apidog is a tool that can help you design, develop, debug, and test your APIs.
- Open Apidog and create a new request.
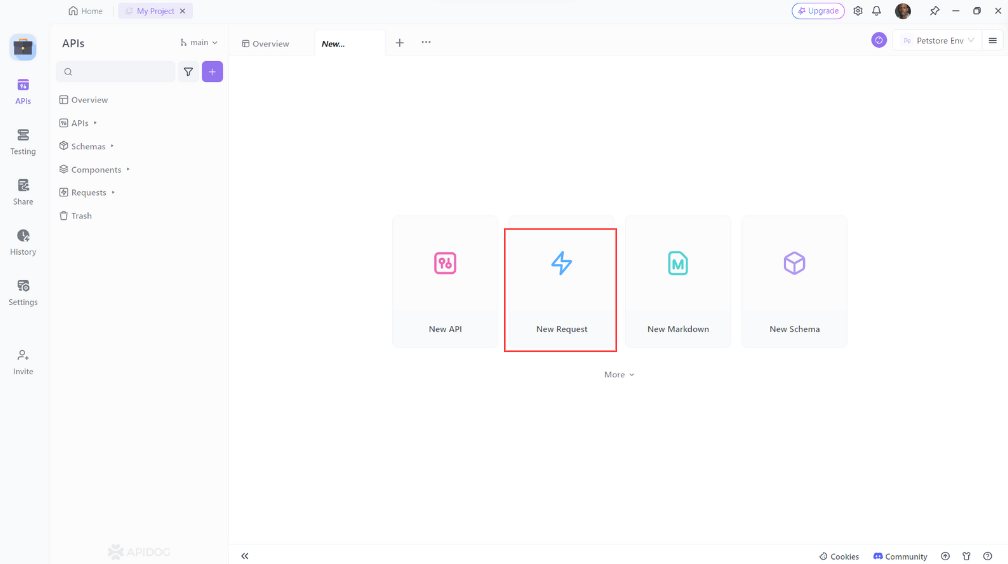
2. Set the request method to GET.
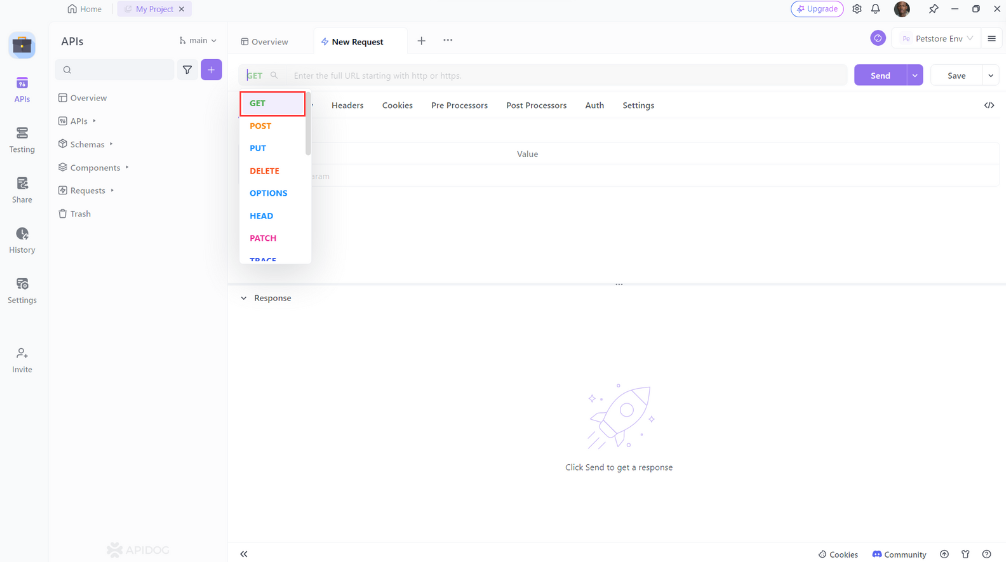
3. Enter the URL of the resource you wish to update. You can also add any additional headers or parameters you want to include, and then click the 'Send' button to send the request
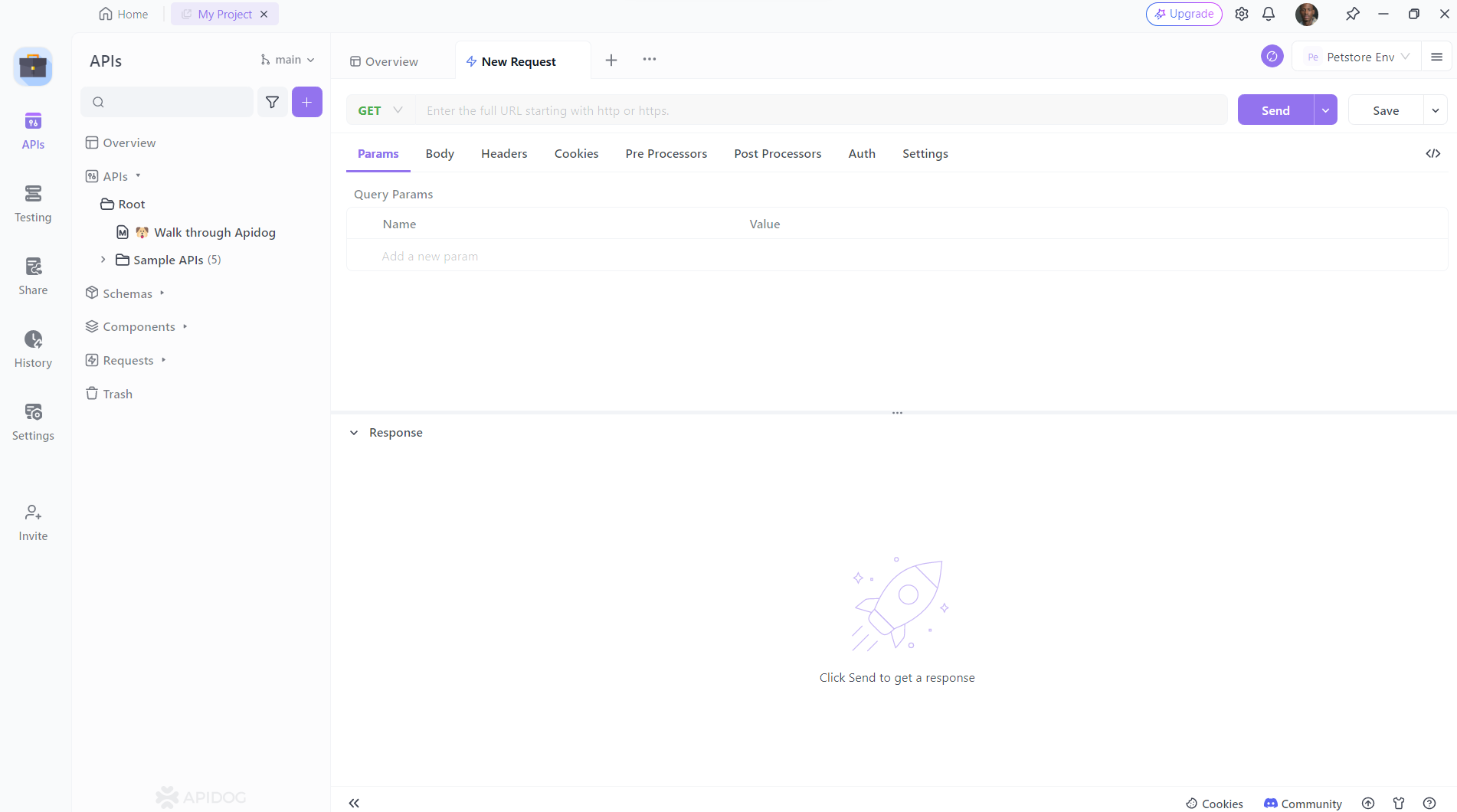
4. Confirm that the response matches your expectations.
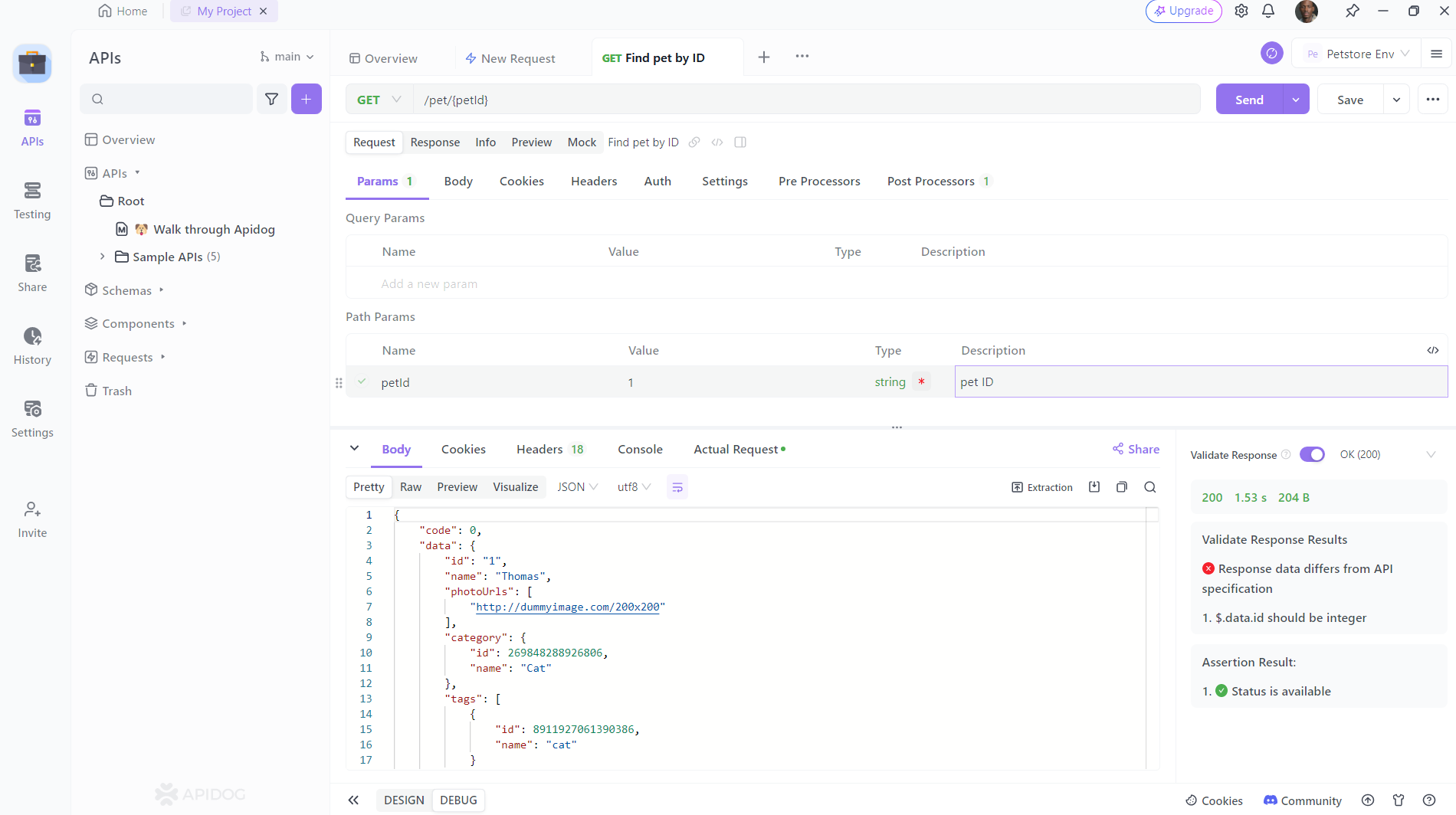
Best Practices for API Integration
To wrap up, here are some best practices to follow:
- Read the API documentation thoroughly.
- Handle errors and exceptions gracefully.
- Stay updated with any changes to the API.
- Respect the API rate limits to avoid being blocked.
- Secure sensitive data, such as API keys and user credentials.
Conclusion
Integrating APIs into your Java applications can be a game-changer. It allows your applications to be more dynamic, powerful, and interconnected. With the tips and techniques we’ve discussed, you’re well-equipped to take on the challenge.
By integrating Apidog into your workflow, you can efficiently design, execute, and manage tests, ensuring that your API is well-prepared for real-world scenarios.