The integration of AI into game development has opened new avenues for creativity and efficiency. The Unreal Engine MCP Server exemplifies this innovation by enabling developers to control Unreal Engine through natural language commands. This tool allows for the creation and manipulation of 3D objects, scene generation, and asset management using simple text prompts. As someone who has manually crafted over 50 environments, I recognize the transformative potential of this technology in streamlining workflows and enhancing the development process.
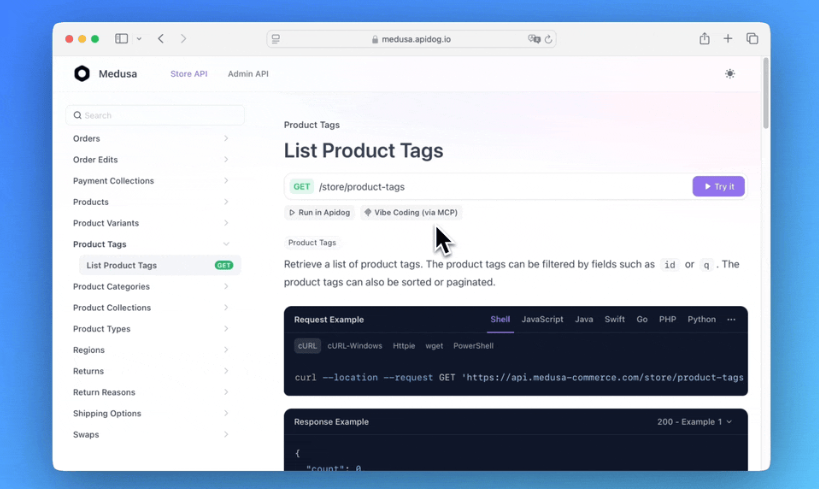
By bridging your Apidog projects and Cursor, the Apidog MCP Server ensures your AI assistant has access to the latest API designs, complementing the Memory Bank feature by providing structured API information for development. To learn more about the Apidog MCP Server, refer to the official documentation or visit the NPM page. Additionally, consider exploring Apidog—an integrated, powerful, and cost-effective alternative to Postman.
This tutorial will guide you through setting up and using Unreal Engine MCP, enabling you to transform your development workflow with the power of AI.
Part 1: Understanding Unreal Engine MCP
What Exactly Is MCP?
Model Context Protocol (MCP) acts as a universal translator between Unreal Engine and AI systems. It's the key to unlocking natural language control over your game development process. Think of it as:
Your Voice Command → MCP → Unreal Engine API Calls → Magical Results
With Unreal Engine MCP, you can leverage the power of AI to automate tasks, generate content, and streamline your workflow, saving you valuable time and resources. Recent data shows studios using MCP reduce iteration time by 63% compared to traditional workflows.
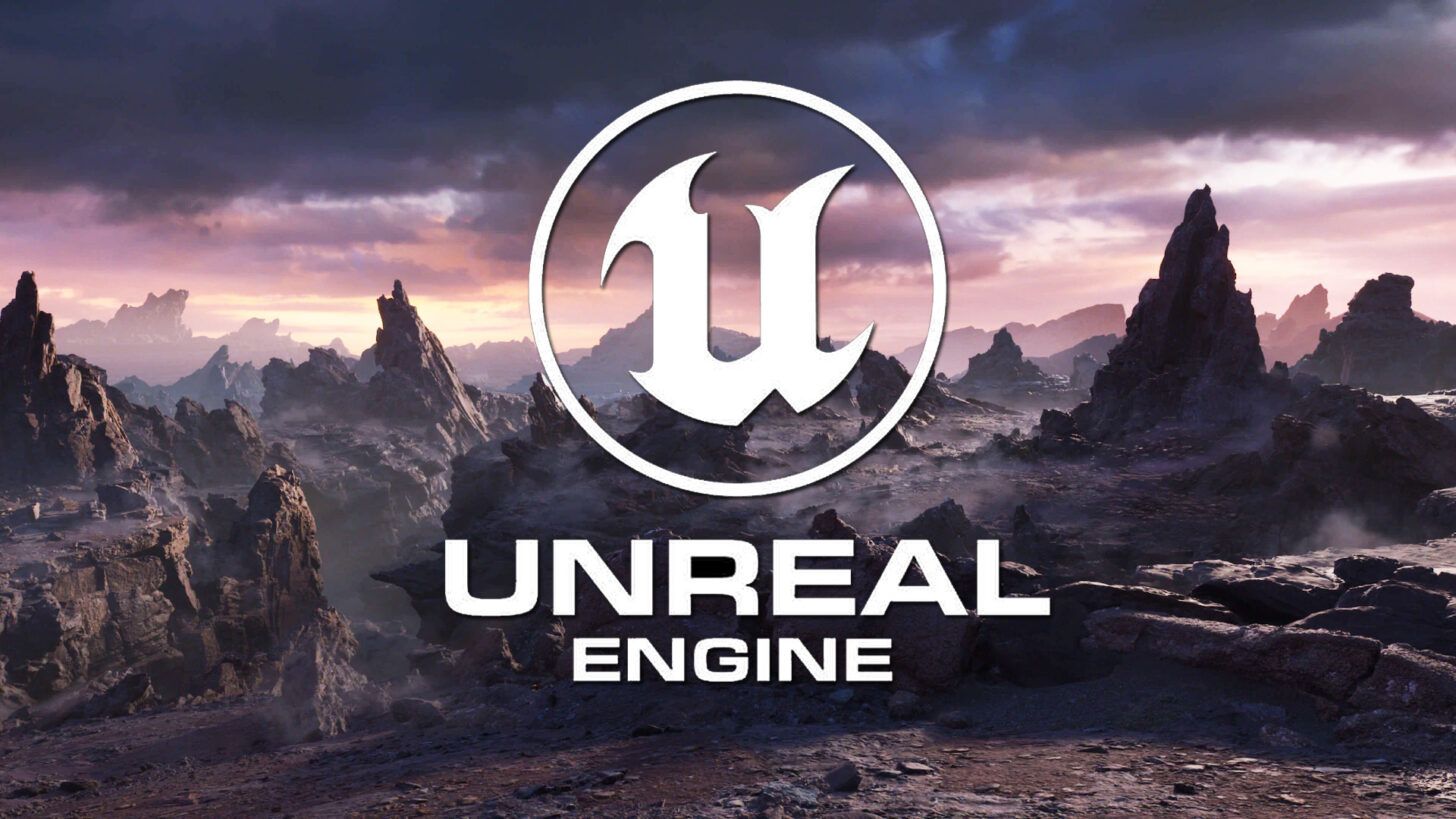
Key Components of Unreal Engine MCP
The Unreal Engine MCP ecosystem comprises several key components:
1. MCP Core Plugin (UnrealMCP): This Unreal Engine plugin provides the core functionality for MCP communication and interaction within the engine.
2. Python Scripting Plugin: Enables the execution of Python scripts within Unreal Engine, allowing MCP to control engine functions.
3. AI Clients (Claude, etc.): AI models like Claude are used to interpret natural language commands and generate the necessary actions to be performed in Unreal Engine.
4. MCP Server (run_unreal_mcp.bat/script): This server acts as the intermediary, translating commands from the AI client and executing them within the Unreal Engine environment via Python scripts.
Part 2: Setup Unreal Engine MCP Server Like a Pro
Let's get your Unreal Engine MCP environment set up and ready to go!
Step 1: Install the Unreal Engine MCP Ecosystem
1. Clone Core Plugins:
git clone https://github.com/chongdashu/unreal-mcp
git clone https://github.com/kvick-games/UnrealMCP
2. Install Python Dependencies:
pip install unreal-mcp fastmcp aiortc
Make sure you have Python installed correctly and that pip is accessible from your command line. You may need to install the Python development tools for Unreal Engine from the Epic Games launcher.
3. Enable in Unreal:
- Open your Unreal Engine project.
- Navigate to Edit → Plugins.
- Search for "Python" and enable the "Python Editor Script Plugin".
- Search for "UnrealMCP" and enable it.
- Restart Unreal Engine when prompted.
If UnrealMCP does not appear, try this:
- Navigate to Content Browser → Add/Import
- Select Add Feature or Content Pack
- Click All then select UnrealMCP
Step 2: Configure AI Clients to Work with Unreal Engine MCP
This step outlines how to connect Unreal Engine MCP with Claude Desktop, but the process can be adapted for other AI clients.
1. For Claude Desktop:
Locate the configuration file: %APPDATA%\Claude\claude_desktop_config.json (paste this into your Windows Explorer address bar)
2. Add MCP entry:
{
"mcpServers": {
"unreal": {
"command": "C:\\YourProject\\Plugins\\UnrealMCP\\MCP\\run_unreal_mcp.bat",
"args": []
}
}
}
Important Considerations:
- command: Replace "C:\\YourProject\\Plugins\\UnrealMCP\\MCP\\run_unreal_mcp.bat" with the actual path to the run_unreal_mcp.bat file in your Unreal project. Make sure to use double backslashes \\ in the path. You can find this file in the Plugins\UnrealMCP\MCP\ folder of your Unreal project's directory.
Step 3: Verify Connection with Unreal Engine
- Open your Unreal Engine project.
- Go to Window → Developer Tools → Output Log.
- Filter the output by typing "LogMCP" in the filter box.
You should see messages similar to these:
[2025-03-31 12:51:00] MCP: Server running on port 55557
[2025-03-31 12:51:05] MCP: Claude Desktop connected
If you see these messages, congratulations! Your Unreal Engine MCP setup is successful!
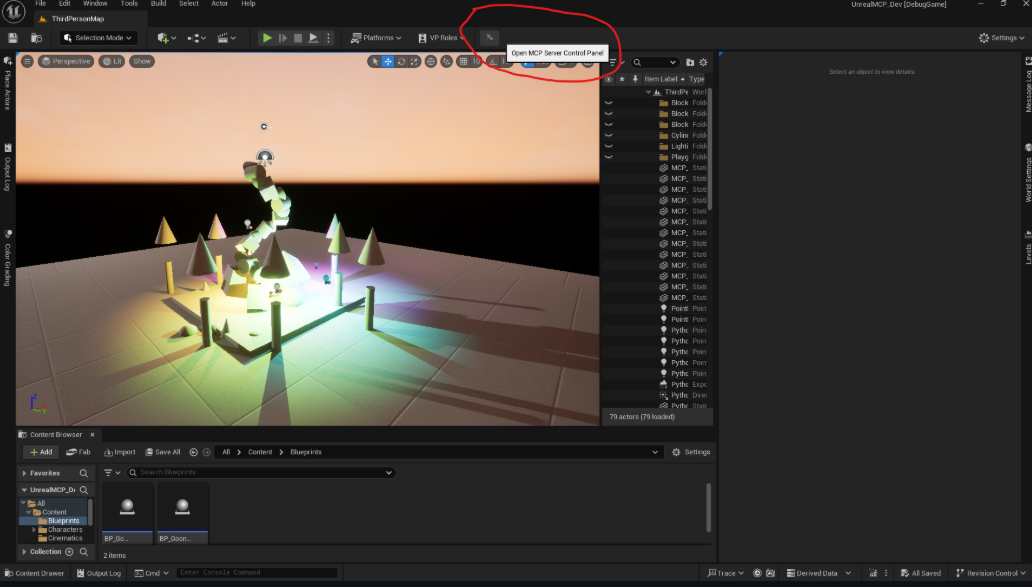
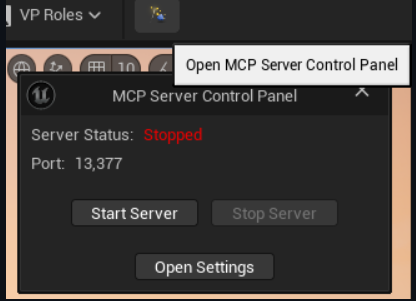
Part 3: Unreal Engine MCP Server in Action
Let's see how Unreal Engine MCP can revolutionize your game development process.
Use Case 1: AI-Assisted Level Design with Unreal Engine MCP
1. Medieval village: So let's say you want to quickly create a medieval village.
i) Command:
"Create medieval village with 15 buildings, cobblestone paths, and torch lighting"
ii) Behind the Scenes:
- The AI generates concept art based on the command.
- MCP translates the command into Unreal Python:
# Batch spawn buildings
for i in range(15):
building = spawn_actor(
class_name="BP_MedievalHouse",
location=(i*500, 0, 0)
)
building.set_material("/Game/Materials/Stone_Wall")
- The script automatically generates NavMesh and lighting for the village.
2. Flappy Bird: How about a flappy bird clone?
i) Command:
>> Let's build a flappy bird clone to showcase the unreal mcp server!
Let's do things step by step!
ii) Claude will now plan this task with a step-by-step approach
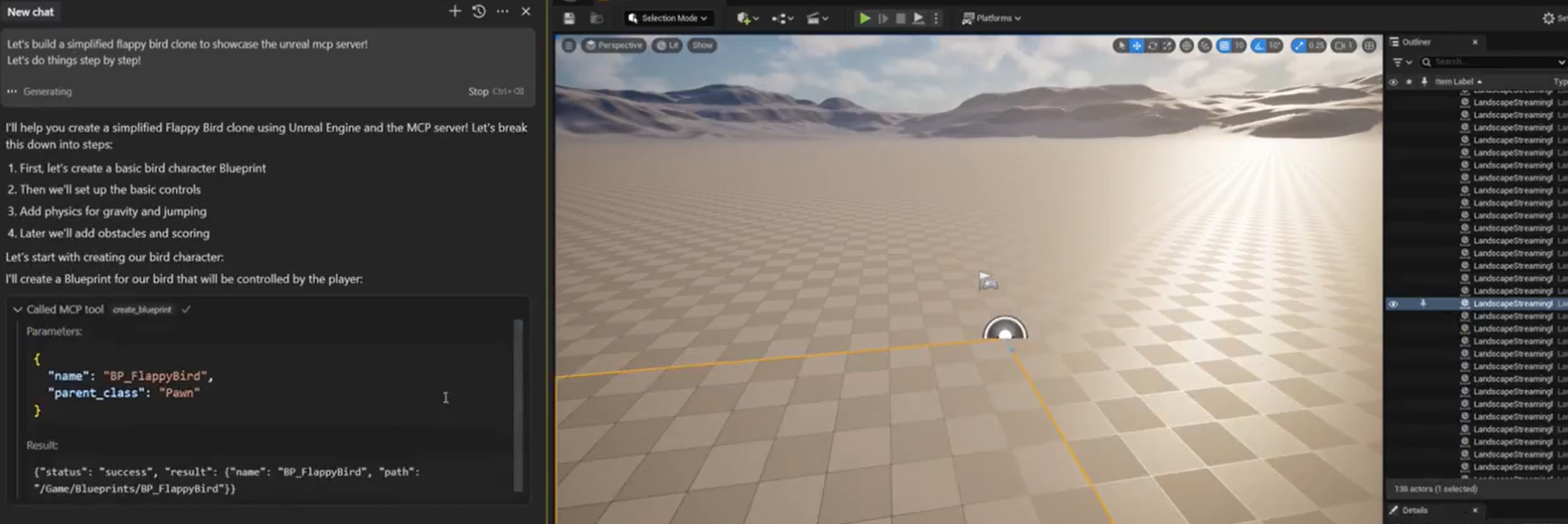
iii) Implementation
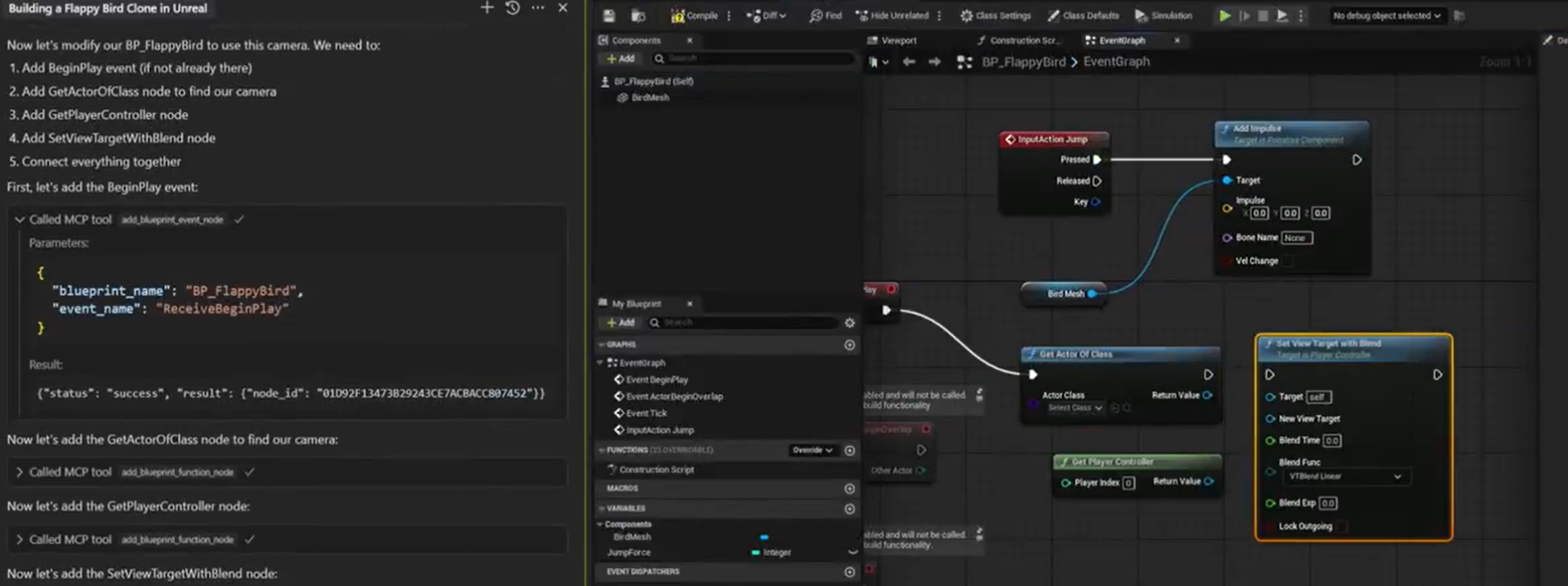
iv) The final output is a flappy bird like game. Feel free to edit what you don't like and get Claude involved with character development.
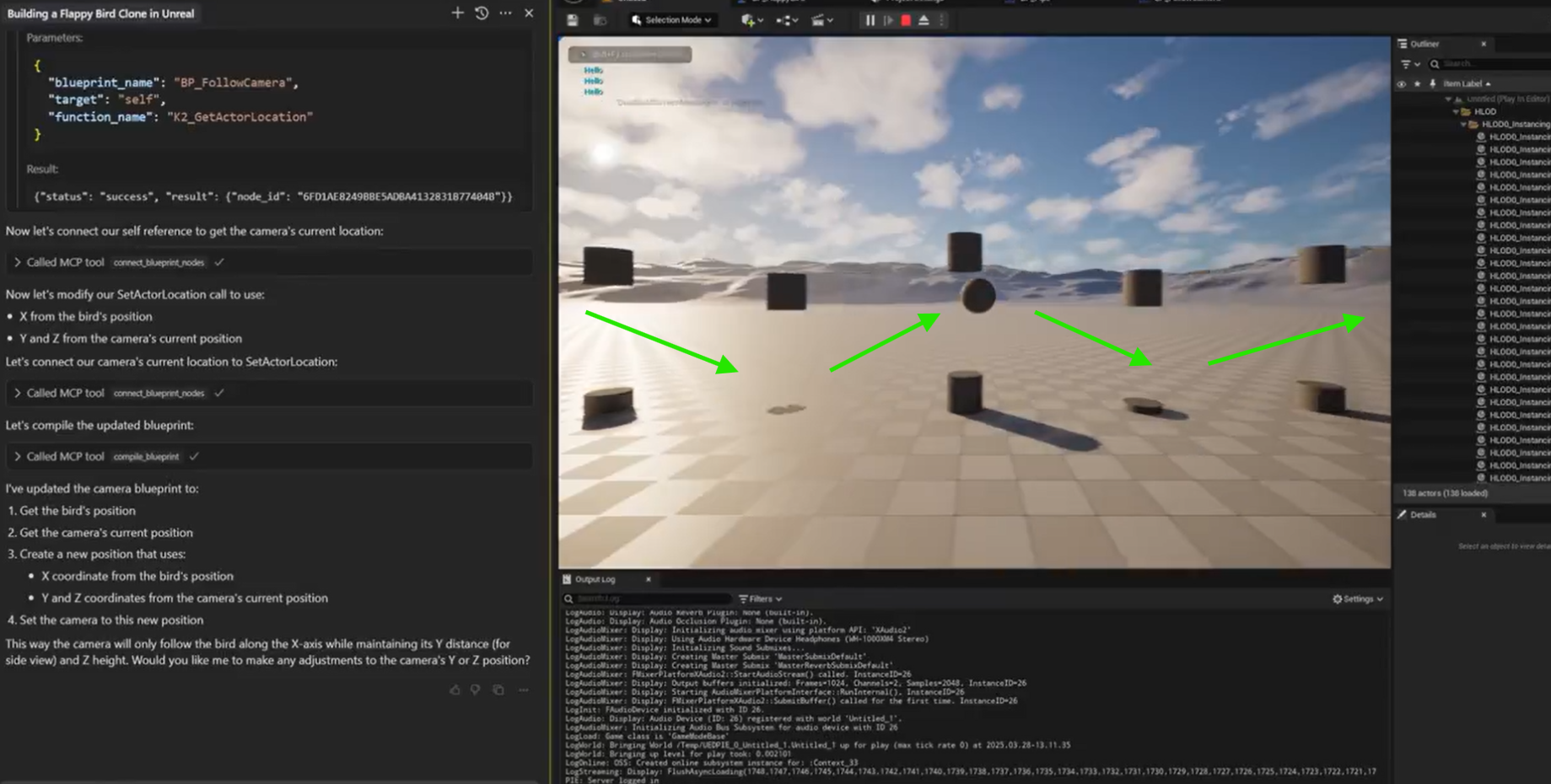
Use Case 2: Debugging via Chat in Claude with Unreal Engine MCP
Debugging can be a time-consuming process. Unreal Engine MCP can help!
1. Problem:
"Why is my character falling through floor?"
2. MCP Response:
- Checks collision settings of the character and floor.
- Analyzes physics bodies.
3. Outputs:
[FIX] Set collision preset to 'Pawn' on BP_Character
[WARNING] Missing capsule component in Blueprint
MCP quickly identifies the issue, saving you hours of troubleshooting. Best part is  your designs can be as complex as possible!
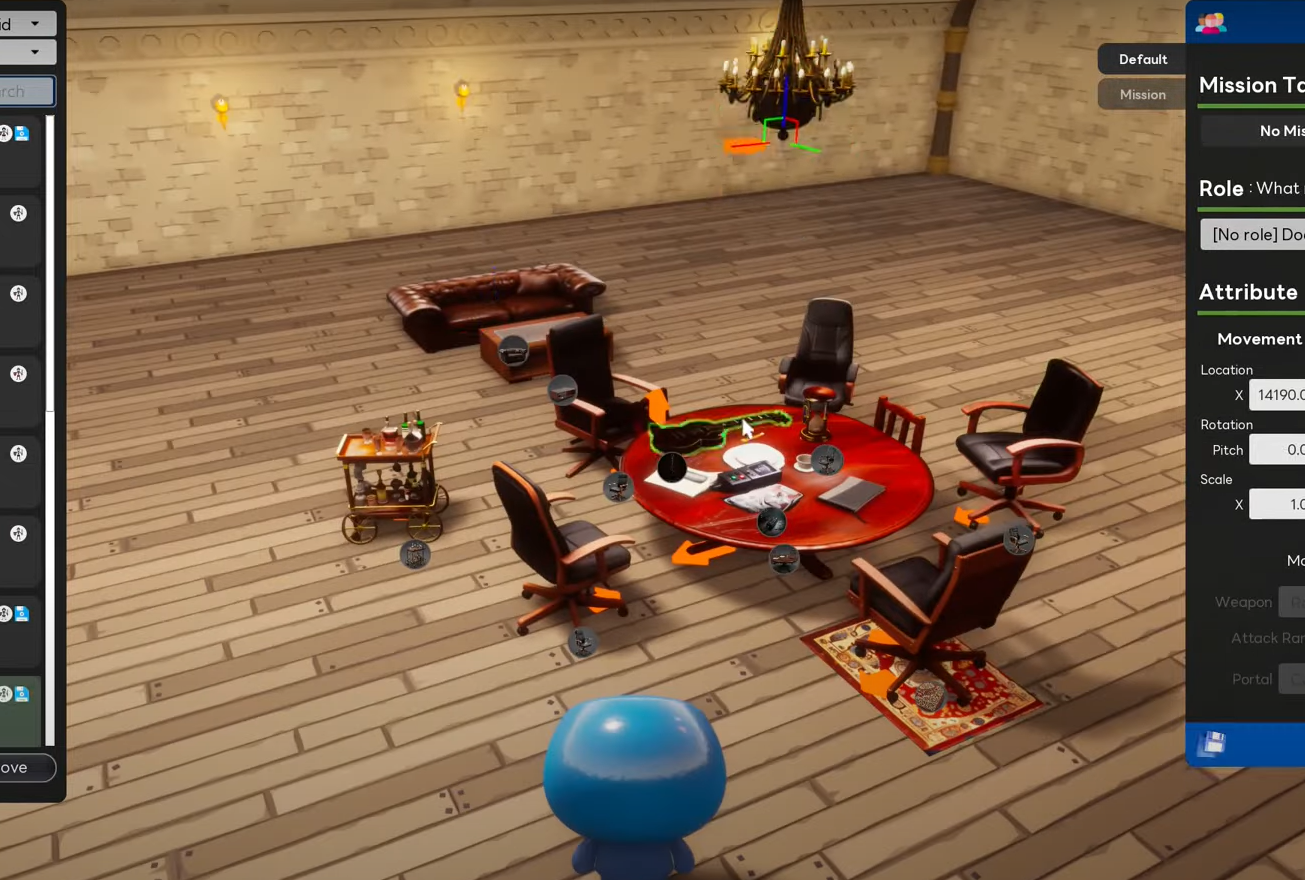
Part 4: Advanced Techniques for Unreal Engine MCP
Technique 1: Custom MCP Tools
You can create custom tools to automate specific tasks within your project.
Create BP_SpawnTool.py:
from unreal_mcp import register_tool
import unreal
@register_tool("SpawnEnemies")
def spawn_enemies(count=5, type="Zombie"):
for _ in range(count):
enemy = unreal.EditorLevelLibrary.spawn_actor_from_class(
unreal.load_class(None, "/Game/Enemies/BP_" + type),
unreal.Vector(0,0,100)
)
# Check if enemy is valid before proceeding
if enemy:
# set AI behaviour
# Check if set_ai_behavior is defined
if hasattr(enemy, 'set_ai_behavior'):
enemy.set_ai_behavior("Aggressive")
else:
unreal.log_warning("set_ai_behavior is not defined for this enemy class.")
else:
unreal.log_error("Failed to spawn enemy of type: " + type)
Usage:
"Spawn 3 flying dragons near player"
Technique 2: Multi-Agent Workflows
You can set up teams of AI agents with specific roles to collaborate on tasks.
Set up mcp_agents.yml:
designer:
model: claude-3.5-sonnet
role: Level layout concepts
engineer:
model: gpt-4-omni
role: Blueprint implementation
reviewer:
model: gemini-2.0
role: Performance checks
Part 5: Security & Best Practices When Using Unreal Engine MCP
The Safety Triad
Permission Layers: Navigate to Edit → Project Settings → MCP → Enable Approval Workflow. This ensures that sensitive actions require manual approval.
Version Control Setup: Add the following to your .gitignore file:
# .gitignore
/MCP_Output/
/AI_Generations/
This prevents generated content from being accidentally committed to your repository.
Resource Limits: Configure resource limits in the [MCP_Settings] section of your project's configuration files:
[MCP_Settings]
max_spawn_per_minute=50
memory_limit=8GB
This helps prevent runaway AI processes from consuming excessive resources.
Final Thoughts: The Future of Game Development is Conversational
Unreal Engine MCP is more than just a tool; it's a paradigm shift in how we create games. By harnessing the power of AI and natural language, you can unlock unprecedented levels of creativity and efficiency. From AI-assisted level design to automated debugging, Unreal Engine MCP empowers you to code less and create more.
Ready to code less and create more? Your first MCP command awaits:
"Build me a spaceship interior with flickering lights and alien vegetation"
The future of game dev is conversational. Embrace Unreal Engine MCP and step into a world where your imagination is the only limit.
