Effective communication between applications is essential in modern software development. This communication often relies on well-defined protocols like Hypertext Transfer Protocol (HTTP). Within HTTP, specific methods dictate the type of interaction intended between a client and server.
To observe how applications behave with APIs, consider using Apidog - a one-stop solution to your API problems. With Apidog, you can build, test, mock, and document APIs, thus providing a more convenient and efficient developing environment for developers.
To learn more about Apidog, click the button below!
To understand what UniRest HTTP methods are available, this article will dive into the core functionalities of four essential HTTP methods—GET, POST, PUT, and DELETE—as implemented by the UniRest library. Understanding these methods is crucial for building robust and versatile web applications.
What are HTTP Methods?
HTTP methods are a defined set of instructions that dictates the intended action on a specific resource. HTTP methods are verbs that establish clear communication channels between client applications and server-side resources.
UniRest HTTP Methods and Example
UniRest GET HTTP Method
Retrieves data from a specified URL. UniRest provides a get(url)
method for this purpose. You can optionally include query parameters using the queryString(String key, Object value)
method to refine your request.
UniRest POST HTTP Method
Creates a new resource on the server. UniRest offers a post(url)
method for initiating a POST request. You can include data to be created within the request body using methods like body(Object body)
or field(String key, Object value)
for form data.
UniRest PUT HTTP Method
Updates an existing resource entirely. UniRest's put(url)
method facilitates sending PUT requests. Similar to POST, you can provide the updated data within the request body using body(Object body)
.
UniRest DELETE HTTP Method
Removes a specified resource from the server. This irreversible action is initiated using the delete(url)
method in UniRest.
UniRest HEAD HTTP Method
Retrieves only the header information associated with a resource, excluding the actual content. UniRest offers a head(url)
method for this purpose.
UniRest OPTIONS HTTP Method
Queries the server to determine the supported HTTP methods for a specific resource. UniRest provides an options(url)
method for this functionality.
UniRest PATCH HTTP Method
Applies partial modifications to an existing resource. UniRest's patch(url)
method allows for sending PATCH requests. The specific changes are typically defined in a format like JSON Patch.
UniRest CONNECT HTTP Method
Establishes a tunnel connection to the server. UniRest offers a connect(url)
method for this purpose, though it's less commonly used compared to other methods.
UniRest TRACE HTTP Method
Traces the path taken by a request through the network of intermediaries. This method is primarily used for debugging purposes, and UniRest provides a trace(url)
method for it.
In addition to these core methods, UniRest provides functionalities for:
- Basic Authentication: Setting username and password for authorization using
basicAuth(String username, String password)
. - Form Uploads: Uploading files within a POST request using
field(String key, File file)
. - Asynchronous Requests: Non-blocking requests are made using asynchronous methods provided by UniRest.
By leveraging these methods and functionalities, UniRest empowers developers to construct dynamic and efficient interactions with web services, streamlining the process of data retrieval, creation, manipulation, and deletion.
Example Code Snippets for UniRest HTTP Methods
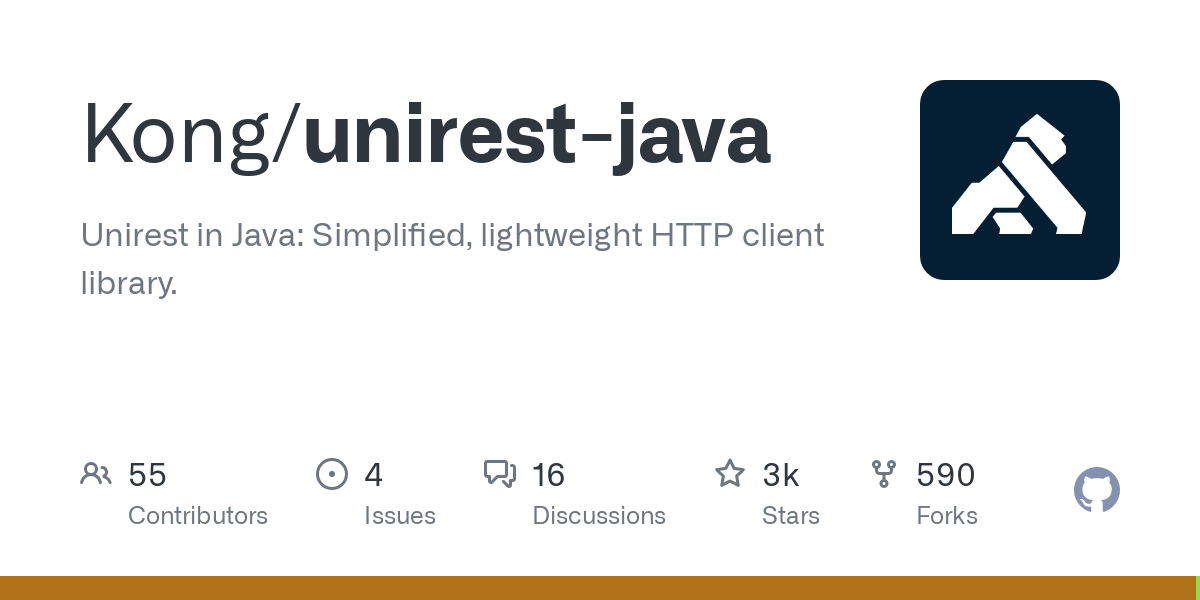
Here are a few examples of UniRest HTTP Methods in UniRest Java.
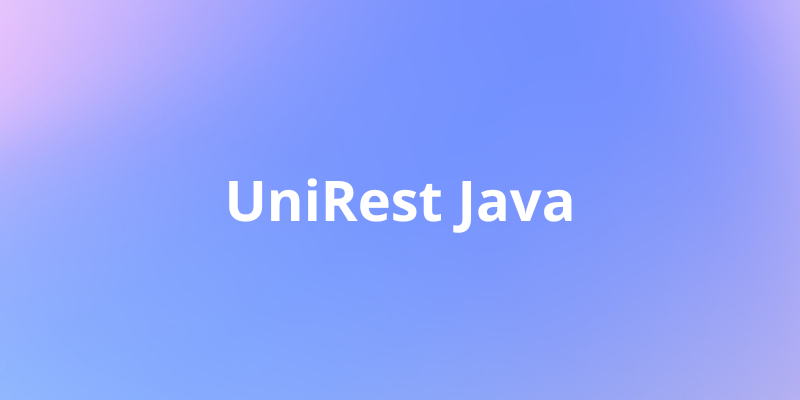
GET Request for Retrieving Data
// Import necessary classes
import com.mashape.unirest.http.HttpResponse;
import com.mashape.unirest.http.JsonNode;
import com.mashape.unirest.http.Unirest;
public class GetExample {
public static void main(String[] args) throws Exception {
// Define the URL and optional query parameter
String url = "https://api.example.com/users";
int userId = 123;
// Build the GET request with query parameter
HttpResponse<JsonNode> response = Unirest.get(url)
.queryString("id", userId)
.asJson();
// Check for successful response
if (response.getStatus() == 200) {
JsonNode user = response.getBody();
System.out.println("Retrieved user data: " + user);
} else {
System.out.println("Error retrieving user: " + response.getStatus());
}
}
}
POST Request to Create a Resource
public class PostExample {
public static void main(String[] args) throws Exception {
// Define the URL and data for the new resource
String url = "https://api.example.com/posts";
String title = "My New Post";
String content = "This is some content for the new post.";
// Build the POST request with body data
HttpResponse<String> response = Unirest.post(url)
.body("{\"title\": \"" + title + "\", \"content\": \"" + content + "\"}")
.asString();
// Check for successful response
if (response.getStatus() == 201) {
System.out.println("Post created successfully!");
} else {
System.out.println("Error creating post: " + response.getStatus());
}
}
}
PUT Request to Update a Resource
public class PutExample {
public static void main(String[] args) throws Exception {
// Define the URL and updated data for the resource
String url = "https://api.example.com/posts/10";
String newTitle = "Updated Title";
// Build the PUT request with body data
HttpResponse<String> response = Unirest.put(url)
.body("{\"title\": \"" + newTitle + "\"}")
.asString();
// Check for successful response
if (response.getStatus() == 200) {
System.out.println("Post updated successfully!");
} else {
System.out.println("Error updating post: " + response.getStatus());
}
}
}
DELETE Request to Remove a Resource
public class DeleteExample {
public static void main(String[] args) throws Exception {
// Define the URL of the resource to delete
String url = "https://api.example.com/comments/5";
// Build the DELETE request
HttpResponse<String> response = Unirest.delete(url)
.asString();
// Check for successful response
if (response.getStatus() == 204) {
System.out.println("Comment deleted successfully!");
} else {
System.out.println("Error deleting comment: " + response.getStatus());
}
}
}
Apidog - Start Integrating Apps with UniRest!
UniRest is a powerful tool that provides developers with the necessary functionalities for bridging code and servers through the power of APIs. It is therefore crucial for developers to understand how to implement both APIs and their tools.
If you are new to API development, we recommend you Apidog.
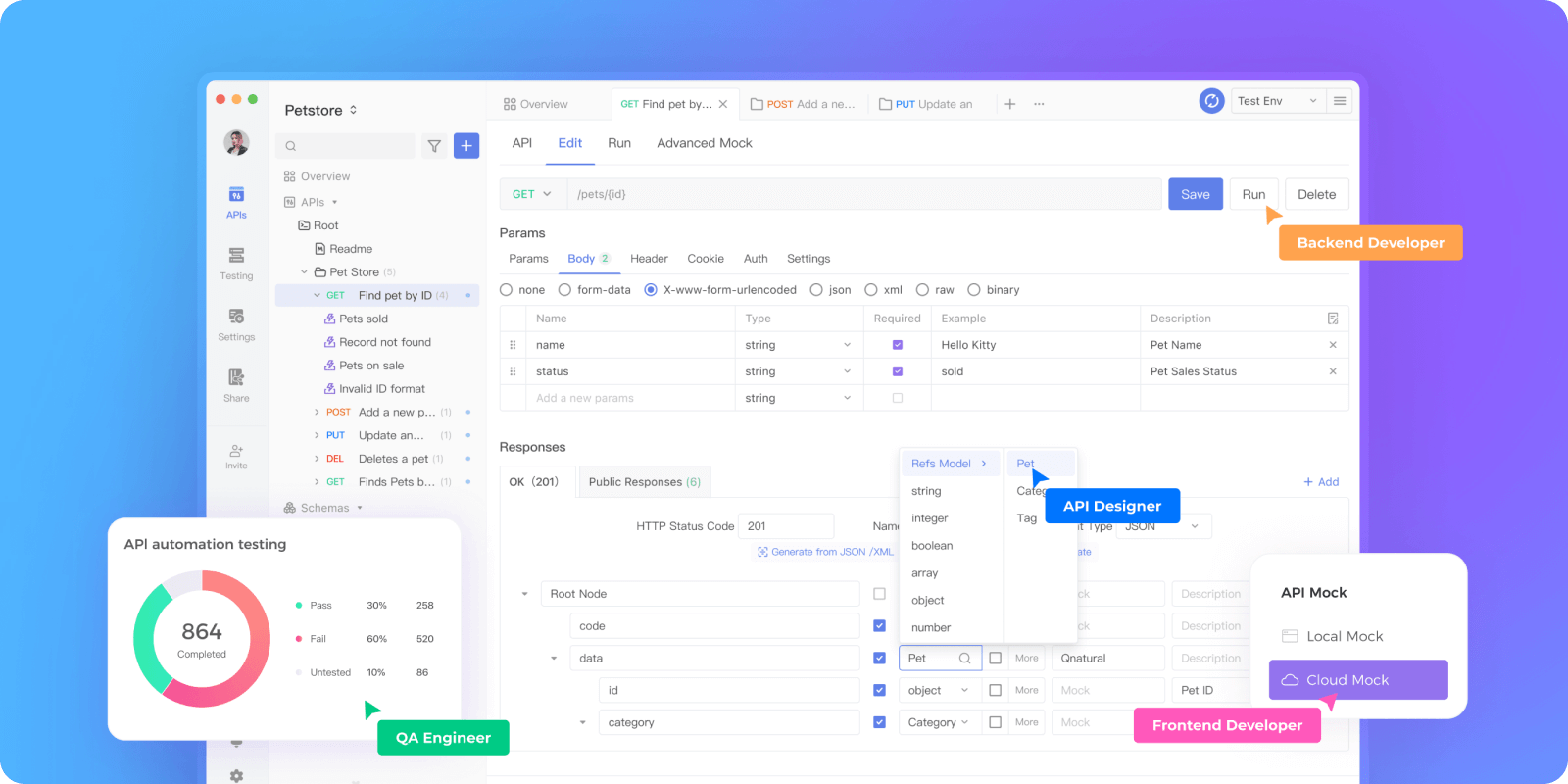
Apidog is a comprehensive API development tool that empowers developers to build, test, mock, and document APIs by equipping them with all the necessary tools.
Through a simple and intuitive user interface, Apidog users, whether new or experienced with the app, can navigate around the app in no time. With a low learning curve, new users will not be intimidated by the complexity of API development.
Importing Existing APIs onto Apidog
If you have an existing API project that you would like to observe, you can import it over into Apidog with just a few clicks of your cursor.
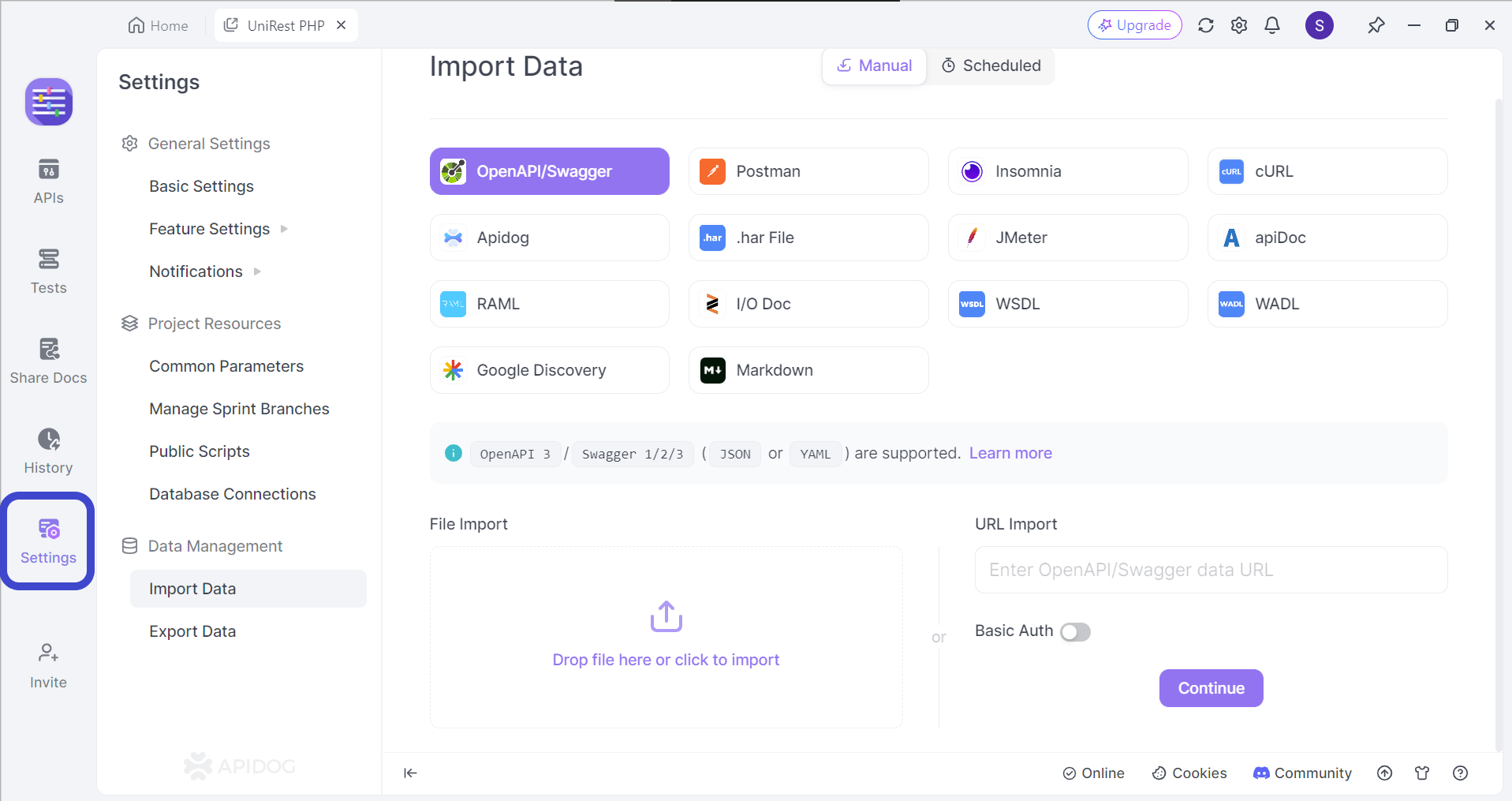
Once you have downloaded Apidog, you can proceed to the Settings
section of your project. Locate the Import Data
button so you can choose which type of API file type you have. If you do not see one, you can drag and drop it into the box below.
Generate Client Code in a Few Seconds with Apidog
A major advantage that Apidog users can enjoy is the code generation feature.
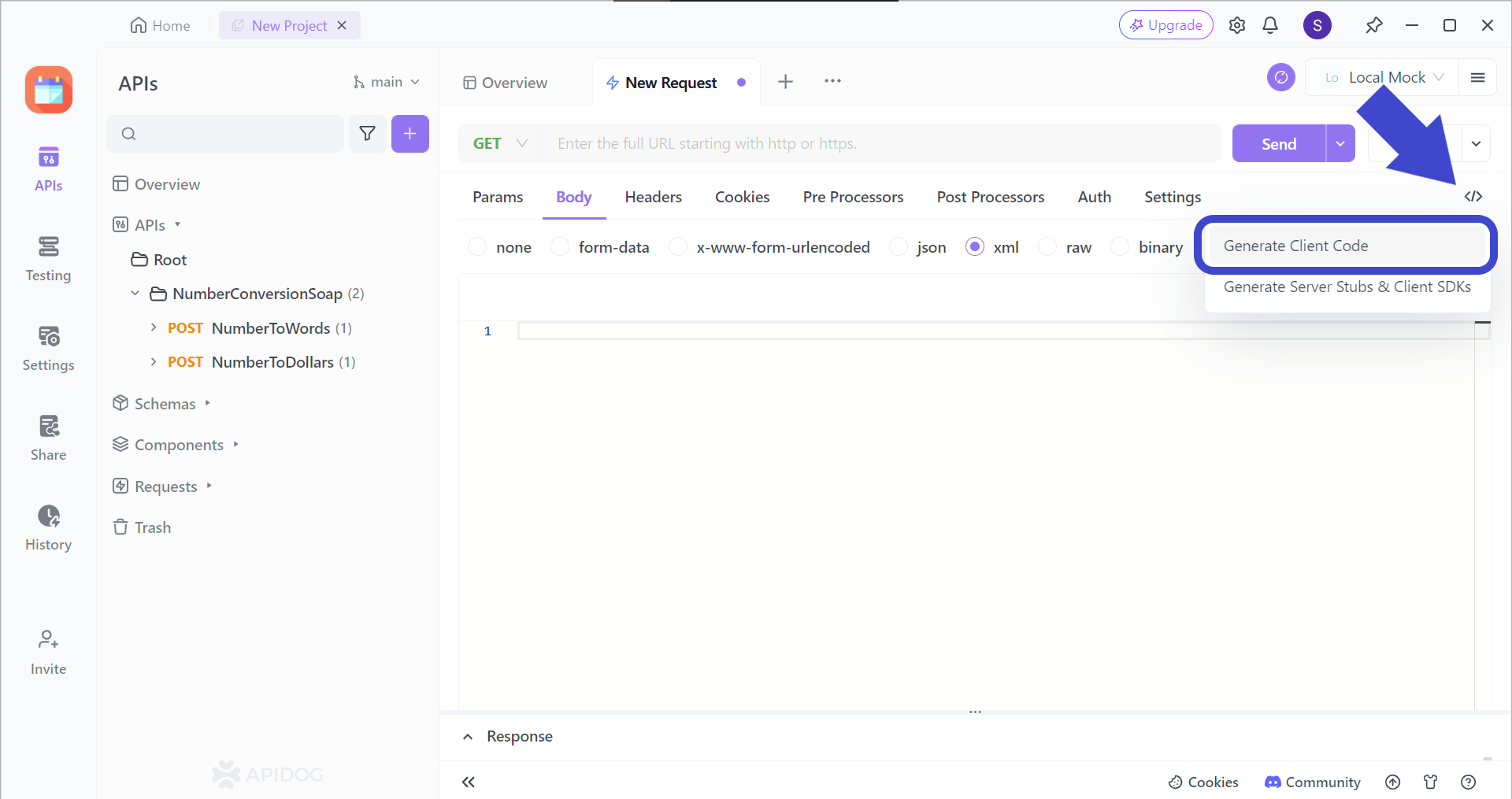
First, install and open Apidog, and make sure to have a project open. Proceed by locating the </>
button around the top right of the Apidog window as shown in the image above.
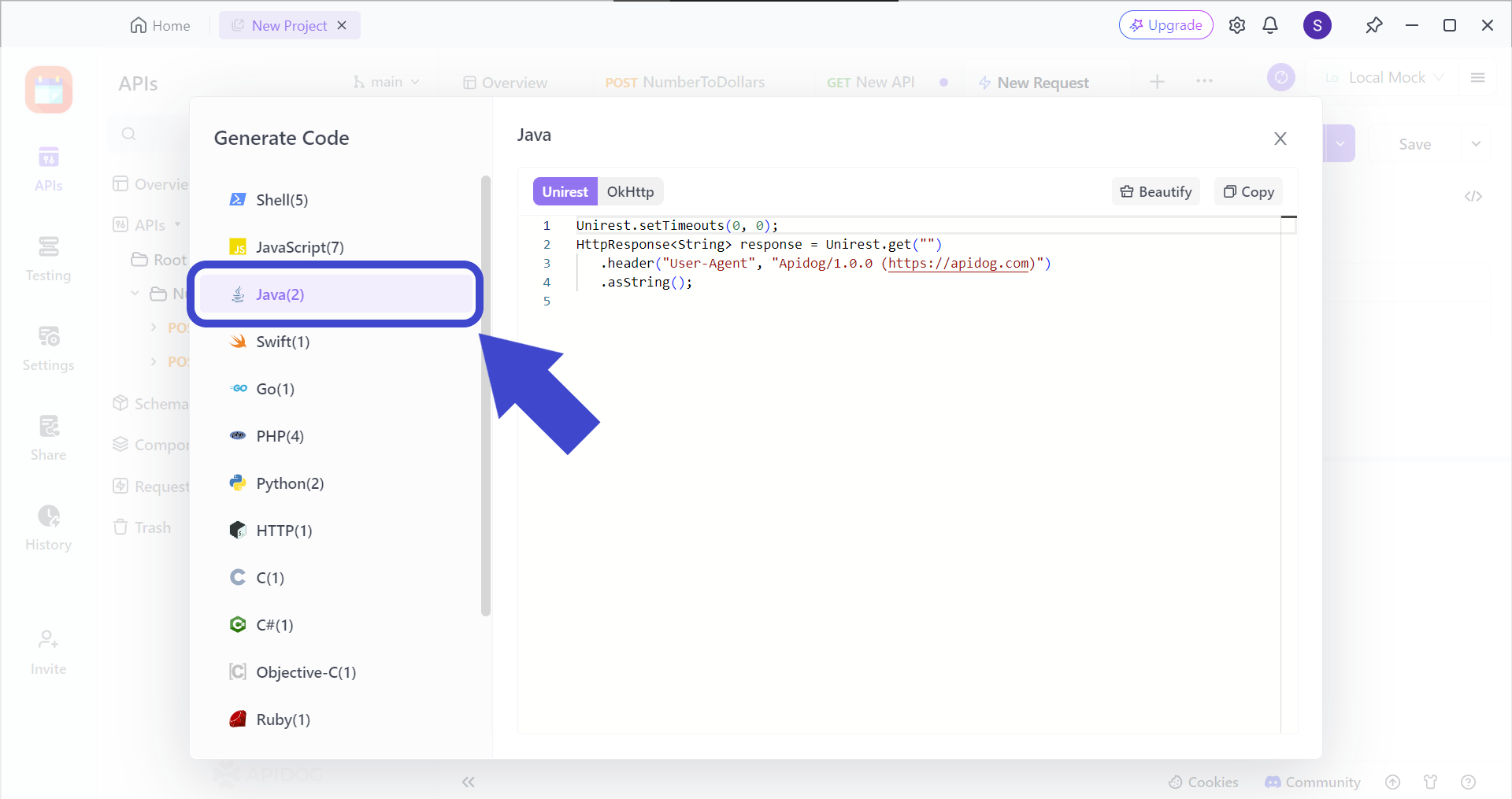
A pop-up window containing a list of programming languages will be provided. The image above shows the code generation for UniRest Java.
Conclusion
The UniRest library equips developers with a powerful and user-friendly toolkit for interacting with web services. By offering a clear and concise API for core HTTP methods like GET, POST, PUT, and DELETE, UniRest simplifies the process of data retrieval, creation, manipulation, and deletion. Additionally, its support for features like authentication, form uploads, and asynchronous requests empowers developers to construct robust and efficient web applications.
For in-depth exploration and mastery of UniRest's functionalities, consulting the official documentation is highly recommended. The documentation provides comprehensive explanations, illustrative code examples, and best practices for effectively utilizing UniRest in your development endeavors. By leveraging both the library and its documentation, developers can streamline web service interactions and expedite the creation of dynamic and feature-rich applications.