APIs are the essential backbone of our modern web, allowing different applications to communicate and exchange data with each other. With every application having its niche, some malicious people tend to abuse these useful applications, therefore negatively impacting other users' experience. However, what if there was a way to prevent this?
Introducing to you Apidog, an all-in-one API development tool that allows you to unlimitedly test out APIs. The only restriction Apidog has is the restriction that thrid-party APIs have (whether they throttle their APIs or not).
If you are interested in trying out Apidog, start for free today (or in the future) by clicking on the button below! 👇 👇 👇
To find out what the difference is between throttling and rate limiting, we will individually define them.
What is Throttling?
In the context of APIs, throttling is considered a dynamic approach to manage API access and prevent the API from overloading. Throttling APIs regulate the flow of incoming requests to ensure the API's stability and performance.
API Throttling Key Features
1. Dynamic Adjustment:
- Unlike rate limiting's fixed limits, throttling dynamically adjusts response times based on real-time traffic conditions. Imagine a highway with variable speed limits. Traffic triggers adjustments (throttling) to maintain smooth flow (API stability).
2. Techniques and Algorithms:
- Leaky Bucket: Incoming requests fill a virtual bucket with a small leak at the bottom. Response times increase (leak slows) as the bucket fills (system nears overload), and vice versa.
- Token Buckets: Users have a limited number of tokens (requests) per timeframe. Each request consumes a token. If no tokens are available (bucket empty), the request is throttled until a token is replenished.
3. Configuration Options:
- Granularity: Throttling can be applied globally or to specific API endpoints based on their resource usage.
- Thresholds: Customizable thresholds determine when throttling kicks in. These can be based on factors like concurrent requests or resource utilization.
- Time Windows: Throttling behavior can be configured for specific time windows (e.g., peak hours).
4. Response Mechanisms:
- Slowing Down: The most common approach, increasing response times for subsequent requests.
- Error Codes: Returning specific HTTP error codes (e.g., 429 Too Many Requests) to indicate throttling and potential retry options.
- Waiting Queues: Temporarily holding requests in a queue until resources become available.
5. Advanced Features:
- Whitelisting: Granting specific users or applications exemption from throttling for critical operations.
- Blacklisting: Throttling more aggressively for users exhibiting abusive behavior.
- Integration with Monitoring: Throttling parameters can be adjusted dynamically based on real-time API usage data.
API Throttling Code Sample
1. Simple API throttling with delay (Python):
def handle_request(user_id):
# Simulate checking a shared resource counter
if resource_counter > threshold:
time.sleep(delay_time) # Throttle by introducing a delay
# Process request logic here...
2. Token bucket throttling for API requests (Python)
from threading import Lock
class TokenBucket:
"""
A simple token bucket class for rate limiting.
"""
def __init__(self, capacity, refill_rate):
"""
Initialize the token bucket with a specific capacity and refill rate.
Args:
capacity (int): The maximum number of tokens the bucket can hold.
refill_rate (float): The rate at which tokens are added to the bucket (tokens per second).
"""
self.capacity = capacity
self.refill_rate = refill_rate
self.tokens = capacity # Start with a full bucket
self.last_refill_time = time.time()
self.lock = Lock()
def consume(self, amount):
"""
Attempts to consume a specified number of tokens from the bucket.
Args:
amount (int): The number of tokens to consume.
Returns:
bool: True if the tokens were consumed successfully, False otherwise.
"""
with self.lock:
self._refill()
if self.tokens >= amount:
self.tokens -= amount
return True
return False
def _refill(self):
"""
Refills the bucket based on the elapsed time and refill rate.
"""
now = time.time()
elapsed_time = now - self.last_refill_time
self.tokens = min(self.capacity, self.tokens + (elapsed_time * self.refill_rate))
self.last_refill_time = now
# Example usage
bucket = TokenBucket(capacity=5, refill_rate=1) # 5 tokens, refilled at 1 token/second
def access_api():
# Simulate API request logic here...
print("Accessing API...")
if bucket.consume(2):
access_api()
else:
print("Request throttled, not enough tokens!")
# Try again after a short delay
time.sleep(1)
if bucket.consume(1):
access_api()
else:
print("Request throttled, not enough tokens!")
Code explanation (step-by-step):
- First define a
TokenBucket
class that manages the token pool - Takes capacity (maximum tokens) and refill rate (tokens per second) as arguments.
consume
method attempts to remove a specified number of tokens from the bucket.- Calls private
_refill
method to ensure bucket is updated based on past time. - If tokens are enough, they are consumed - the method returns
True
- Else, the method returns
False
- indicates throttling.
What is Rate Limiting?
In the context of APIs, rate limiting refers to a set restriction on the number of requests a user or application can make within a specific period. Imagine it like a ticket booth at a popular attraction, where only a certain number of requests are allowed per minute.
API Rate Limiting Key Features
1. Limit Configuration:
Request Limits: API providers define the maximum number of requests allowed per user or application within a specific time window (e.g., 100 requests per hour). These limits can be based on factors like:
- User Tiers: Free vs. paid plans might have different limits.
- API Endpoints: Different functionalities might have varying resource requirements, leading to different limits.
Time Windows: Limits are applied within specific timeframes, typically seconds, minutes, or hours. This allows for controlled bursts of activity while preventing sustained overload.
2. Counting Mechanisms:
User Identification: Requests are associated with users or applications. This can be achieved through:
- API Keys: Unique identifiers provided to developers for authentication and usage tracking.
- IP Addresses: While less secure, IP addresses can be used for basic rate limiting.
Request Counters: The API keeps track of the number of requests received from each user/application within the current time window.
3. Enforcement Strategies:
- Blocking: When a user reaches the limit, their subsequent requests might be entirely blocked until the time window resets. This is a stricter approach suitable for preventing abuse.
- Throttling: Throttling, often used in conjunction with rate limiting, slows down subsequent requests instead of completely blocking them. This allows some level of access while preventing overload. (Throttling is a separate concept but can be used alongside rate limiting)
4. Advanced Features:
- Burst Limits: Short-term allowances for exceeding the average rate limit to accommodate bursts of activity. This offers flexibility for legitimate use cases.
- Leaky Bucket: A metaphorical approach where requests are like water filling a bucket with a small leak. The leak represents the rate limit. Requests are processed as long as the bucket isn't full.
- Token Buckets: Users are allocated a set of tokens (requests) that replenish over time. Requests consume tokens, and users are throttled if no tokens are available.
5. Communication and Monitoring:
- API Documentation: Clear documentation outlines rate limits, including specific limits, time windows, and enforcement methods.
- Monitoring and Alerts: API providers monitor usage patterns and adjust rate limits as needed to maintain stability.
API Rate Limiting Code Samples
1. Tracking limits and time windows (Python):
# Simulate storing API rate limit information retrieved from API documentation
rate_limit = 100 # Requests per hour
time_window = 3600 # Seconds in an hour
last_request_time = None
def make_api_request():
global last_request_time
# Check if within the time window and enough requests remaining (hypothetical)
if last_request_time is None or (time.time() - last_request_time) >= time_window:
# Make the API request
last_request_time = time.time()
# ... (API request logic)
else:
print("API rate limit reached, waiting for reset...")
# Implement backoff strategy (see point 3)
# Example usage
make_api_request()
The code example above displays a situation where you store retrieved rate limit information (requests and time window), and track the last request time. The code then checks if a request can be made based on the remaining time and allowed requests within the window.
2. Utilizing API response headers (Python):
import requests
def make_api_request():
response = requests.get("https://api.example.com/data")
if response.status_code == 429: # Rate limit exceeded code
# Extract rate limit information from headers (X-RateLimit-Remaining, X-RateLimit-Reset)
# Implement backoff strategy (see point 3)
else:
# Process successful response
# ...
The code example above checks the response status code for a common rate limit error code 429
and attempts to extract relevant information from the response headers if encountered.
Summarized Differences Between Throttling VS. Rate Limiting
Feature | Throttling | Rate Limiting |
---|---|---|
Goal | Manage API traffic flow to maintain performance | Control API access to prevent abuse and overload |
Mechanism | Dynamically adjusts response times based on traffic | Sets a hard limit on requests per time window |
Enforcement | Slows down requests during peak periods (more flexible) | Blocks requests exceeding the limit (stricter) |
Focus | Maintaining stability and performance | Fairness and preventing abuse |
Configuration | Thresholds, time windows, response mechanisms | Limits and time windows |
Use Case | Preventing overload during peak traffic, prioritizing urgent requests | Protecting against DoS attacks, controlling usage |
Apidog - Unlimited Requests to Perfect Your Application
The only thing that is stopping you from creating the best APIs is the limitations of your tools - most API tools today all have paywalls. If you do not pay, you cannot get the features essential for API development. However, one API development tool goes a step further to provide the best services for developers.
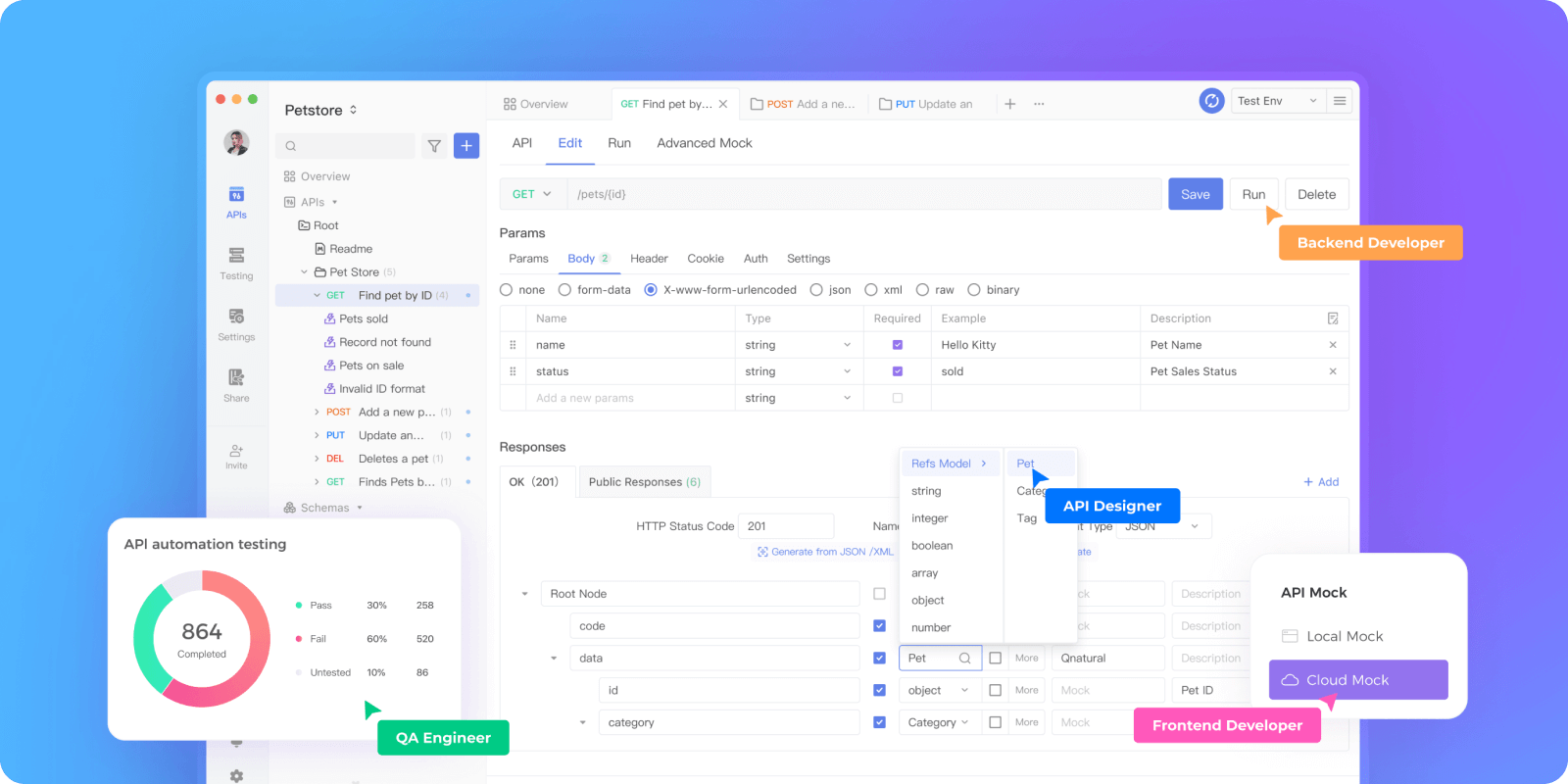
Meet Apidog, an all-in-one API development tool that facilitates every API development process for the entire API lifecycle. With Apidog, you can create new APIs and modify pre-existing APIs, and carry out tests, mocks, and documentation to ensure that your APIs will run flawlessly.
Building APIs with Apidog
With Apidog, you can create APIs by yourself. This means you can also set your API's own rate limit, and decide if you want to throttle your API with the help of additional coding.
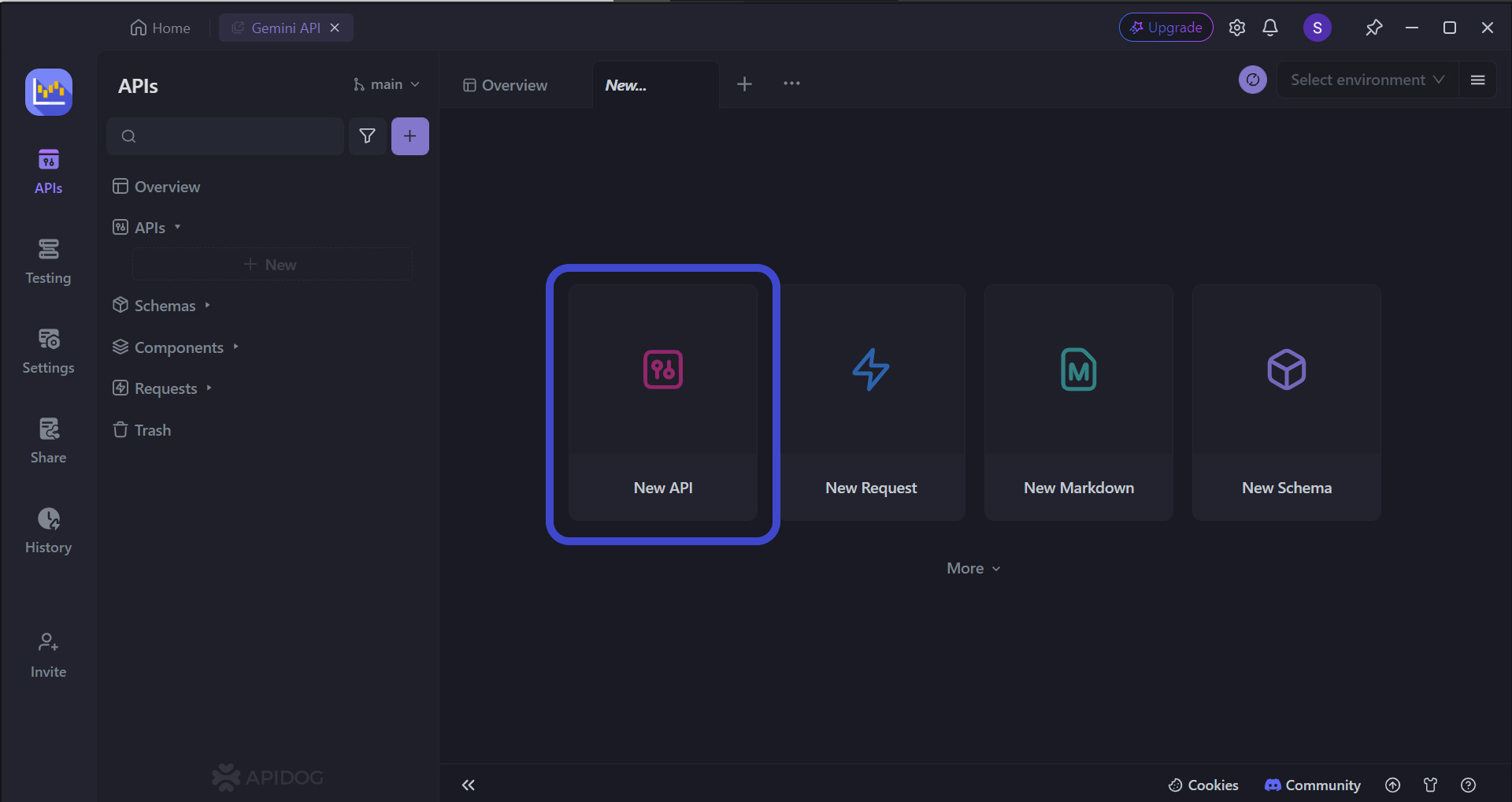
Begin by pressing the New API
button, as shown in the image above.
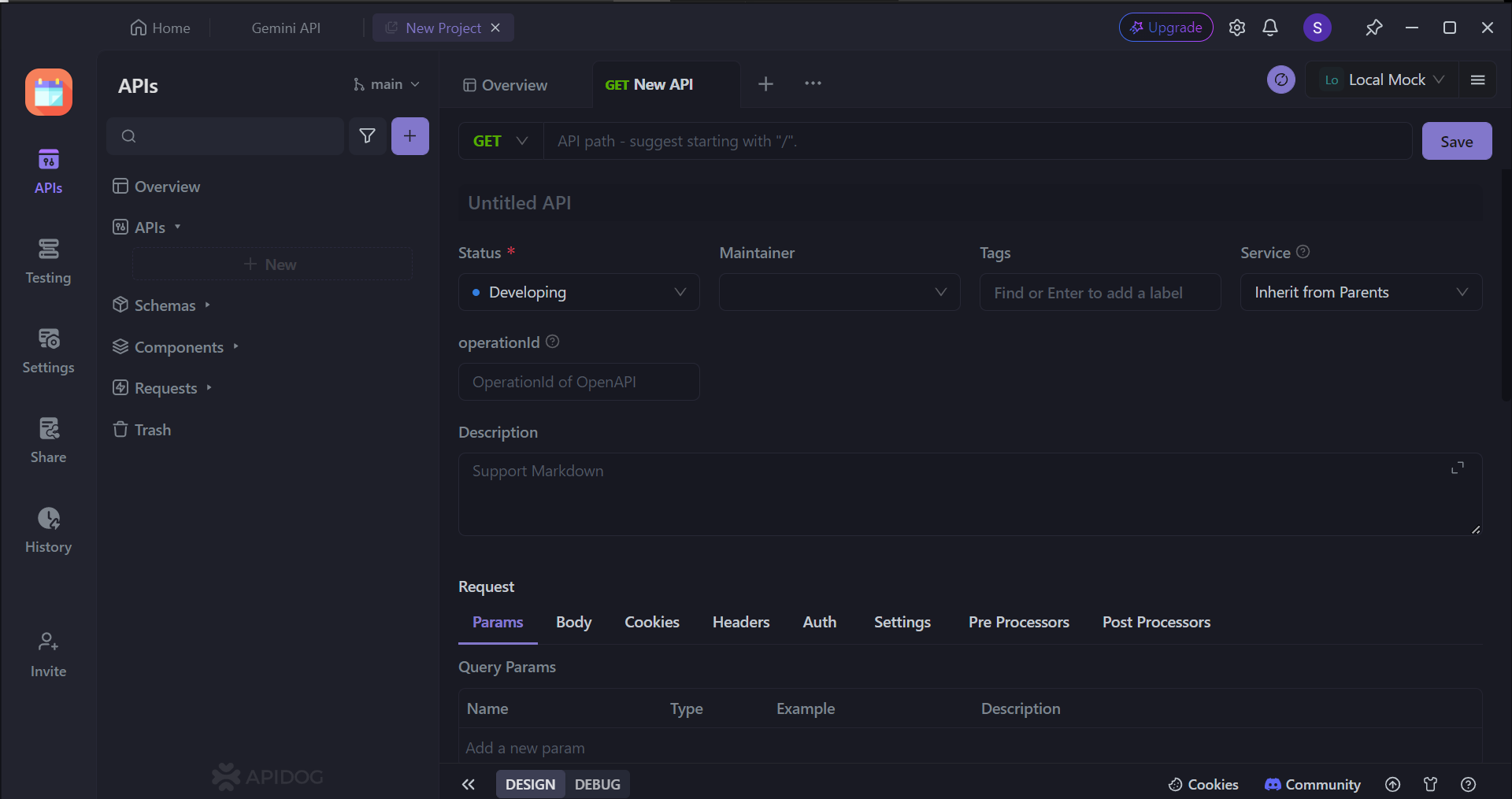
Next, you can select many of the API's characteristics. On this page, you can:
- Set the HTTP method (GET, POST, PUT, or DELETE)
- Set the API URL (or API endpoint) for client-server interaction
- Include one/multiple parameters to be passed in the API URL
- Provide a description of what functionality the API aims to provide. Here, you can also describe the rate limit you plan to implement on your API.
The more details you can provide to the designing stage, the more descriptive your API documentation will be, as shown in the next section of this article.
Make sure to also include whether there are any rate limits imposed on the API, as users will require that knowledge in order to work with the API.
To provide some assistance in creating APIs in case this is your first time creating one, you may consider reading these articles.
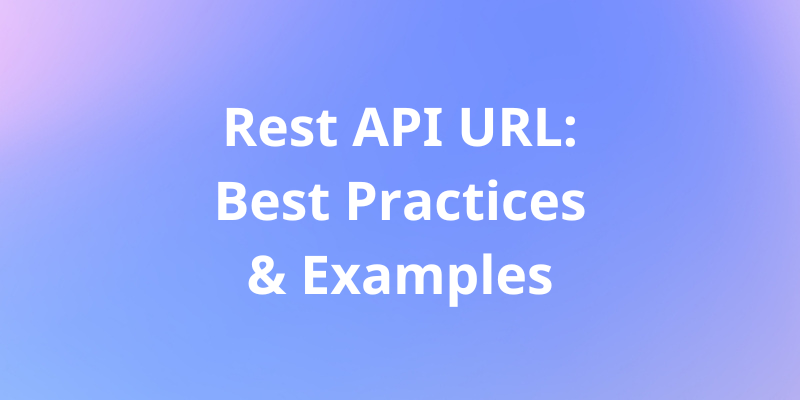
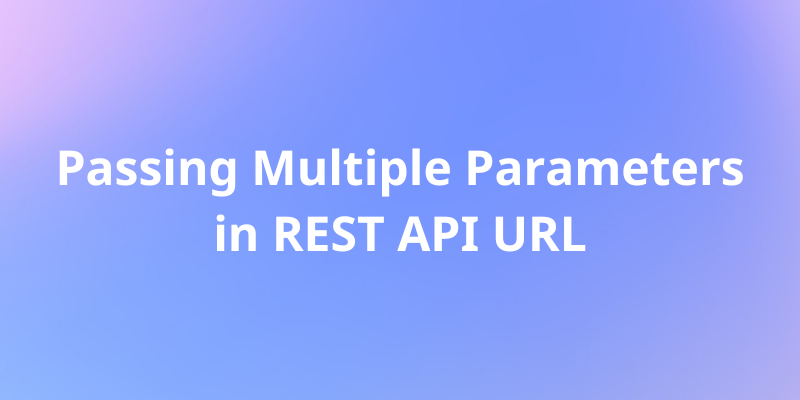
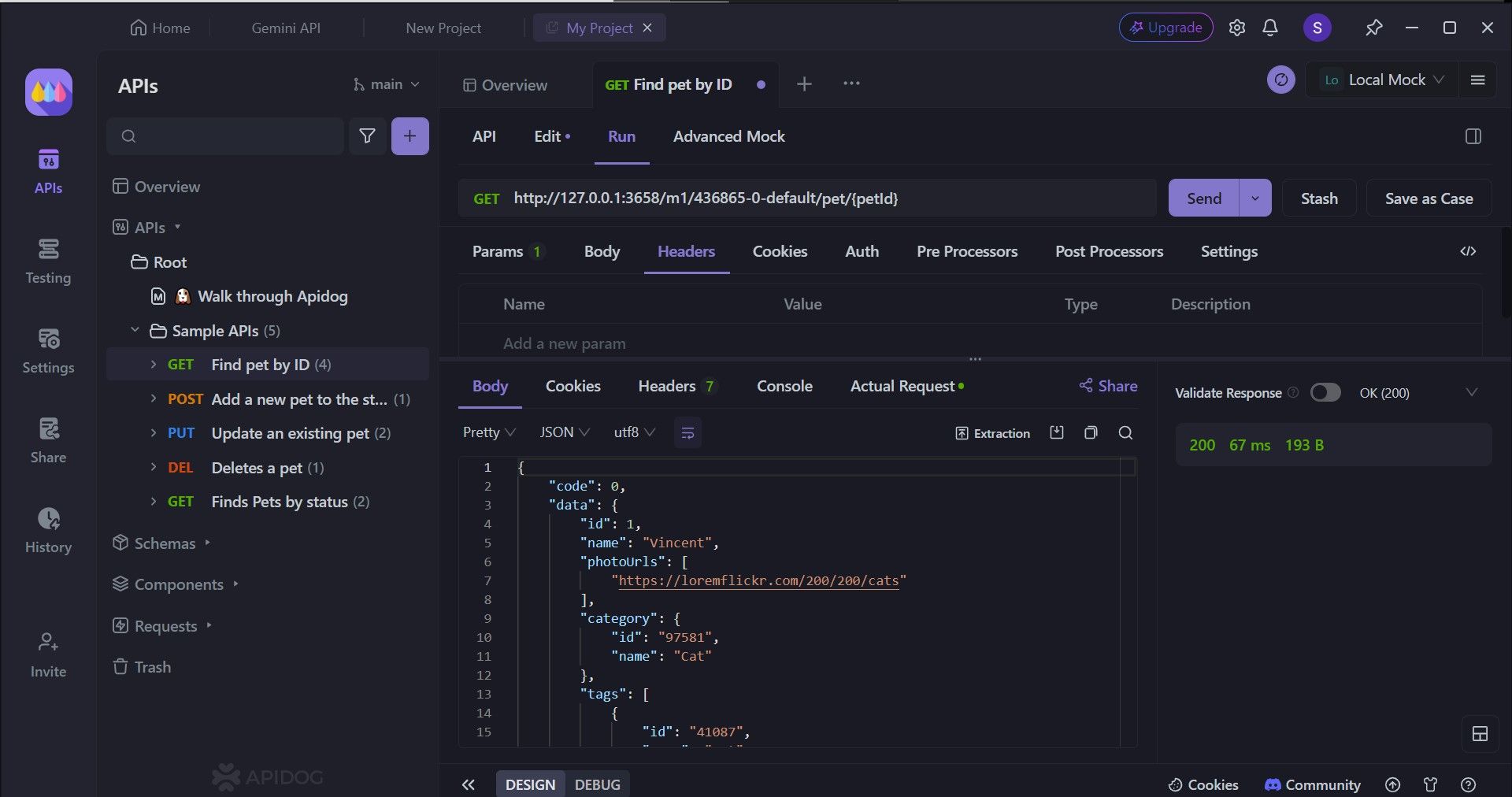
Once you have finalized all the basic necessities to make a request, you can try to make a request by clicking Send
. You should then receive a response on the bottom portion of the Apidog window, as shown in the image above.
The simple and intuitive user interface allows users to easily see the response obtained from the request. It is also important to understand the structure of the response as you need to match the code on both the client and server sides.
Generate Descriptive API Documentation with Apidog
With Apidog, you can quickly create API documentation that includes everything software developers need within just a few clicks.
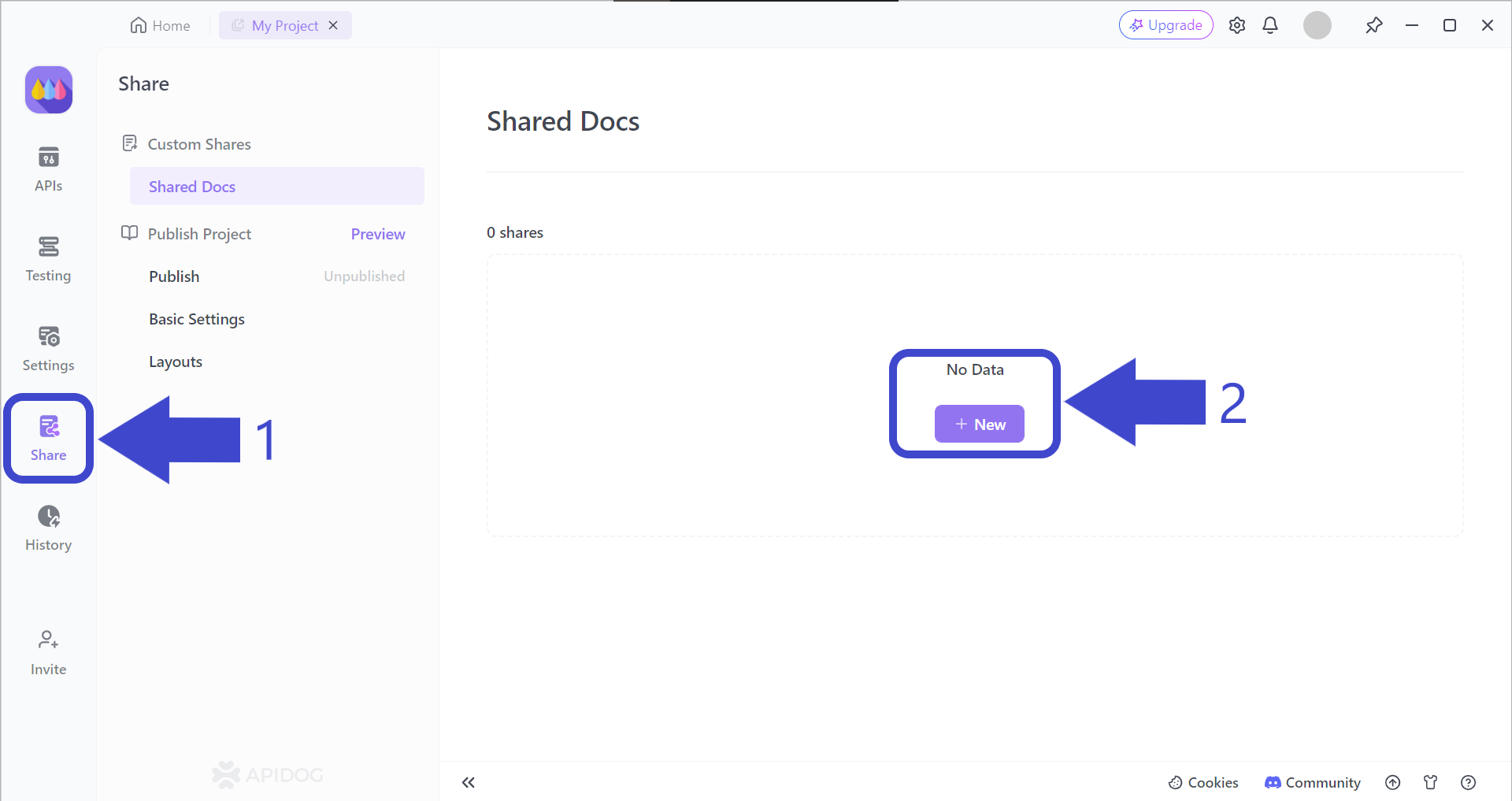
Arrow 1 - First, press the Share
button on the left side of the Apidog app window. You should then be able to see the "Shared Docs" page, which should be empty.
Arrow 2 - Press the + New
button under No Data
to begin creating your very first Apidog API documentation.
Select and Include Important API Documentation Properties
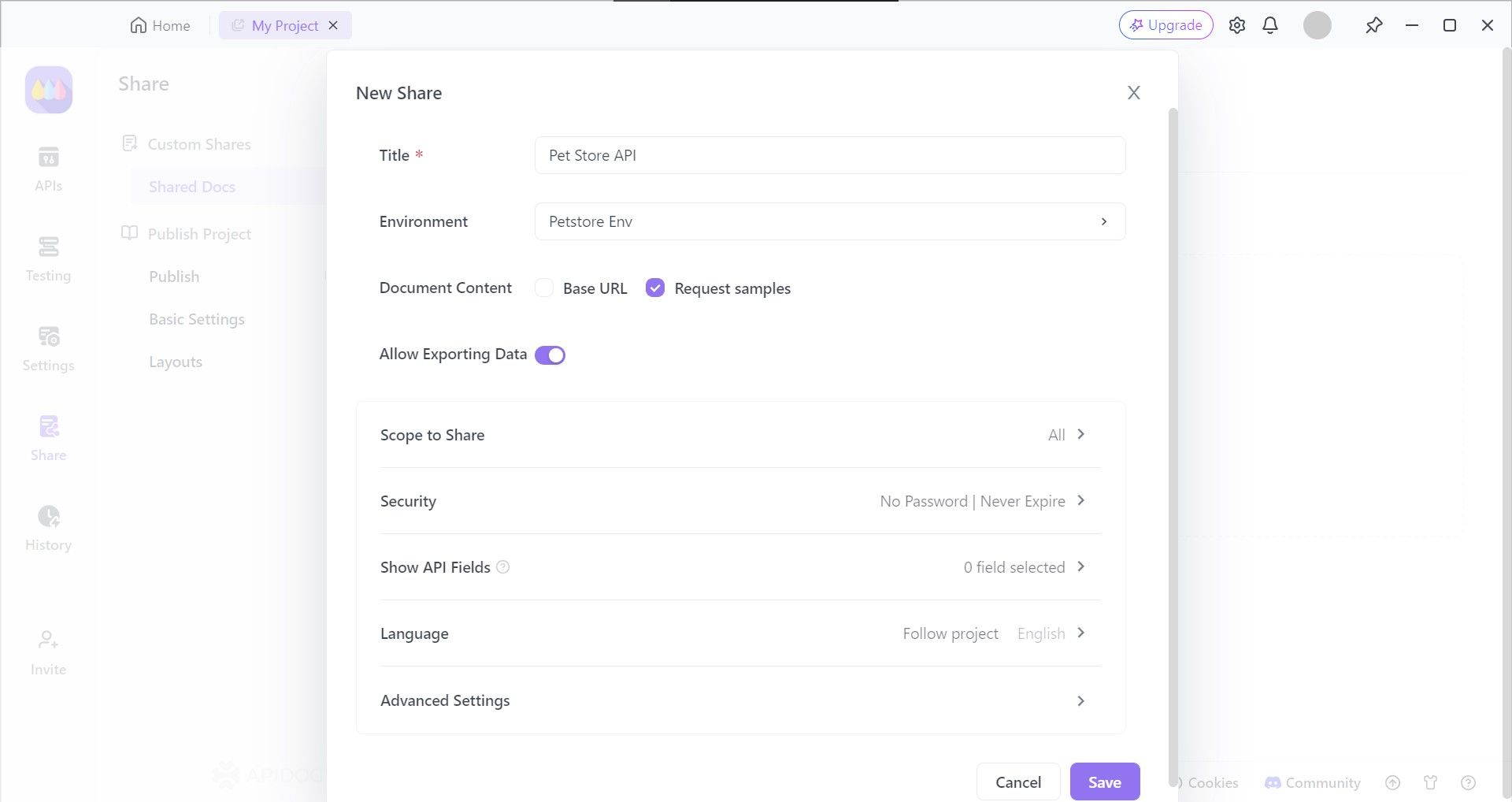
Apidog provides developers with the option of choosing the API documentation characteristics, such as who can view your API documentation and setting a file password, so only chosen individuals or organizations can view it.
View or Share Your API Documentation
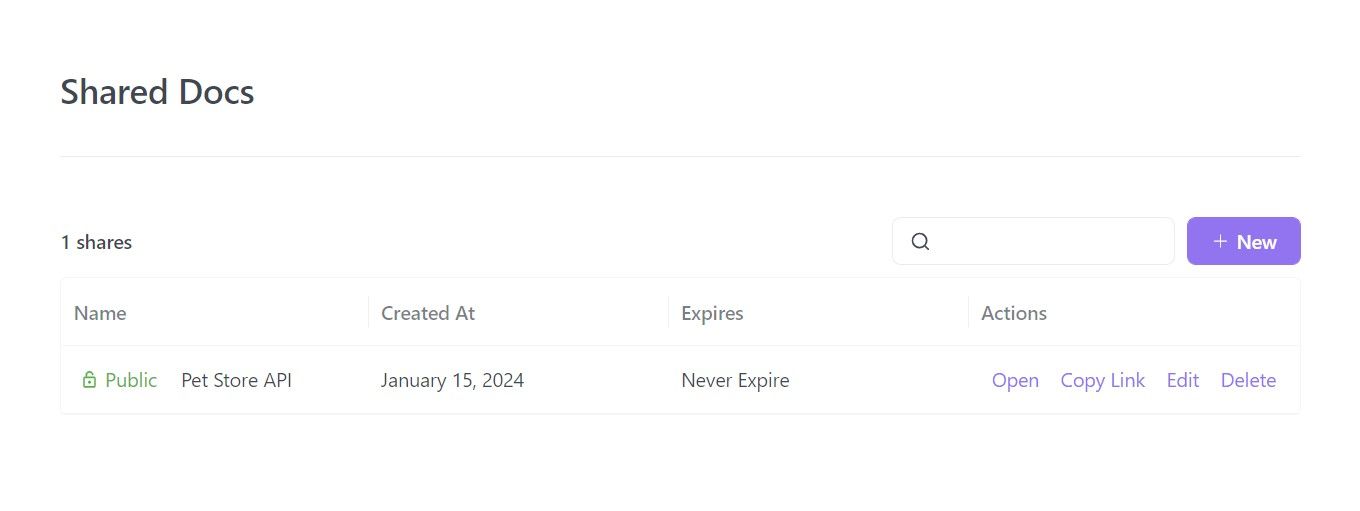
Apidog compiles your API project's details into an API documentation that is viewable through a website URL. All you have to do is distribute the URL so that others can view your API documentation!
If more details are required, read this article on how to generate API documentation using Apidog:
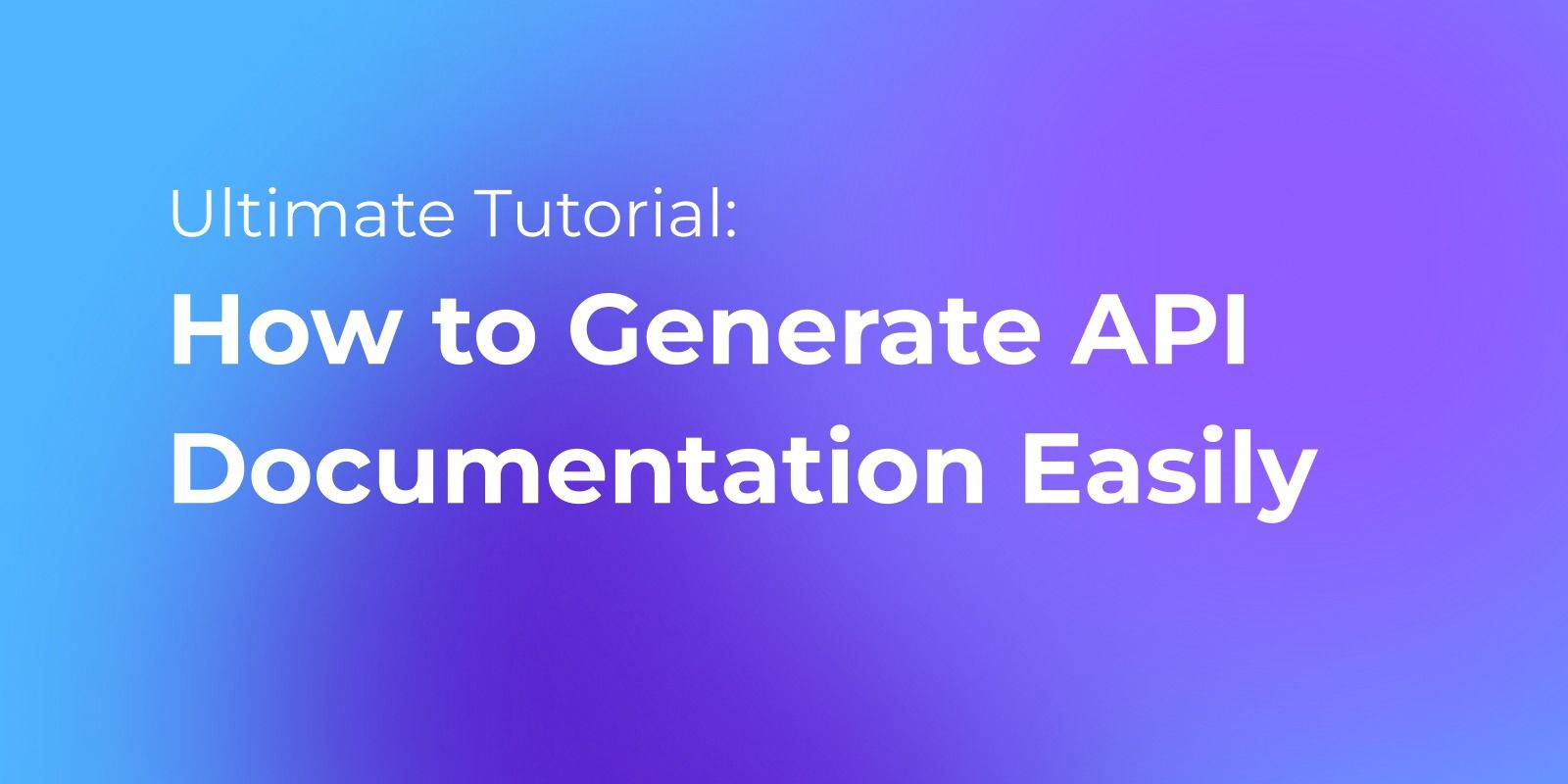
Conclusion
Throttling and rate limiting are both essential tools for managing API access and ensuring smooth operation. While they share the common goal of preventing overload, they differ in their approach.
Rate limiting acts like a strict gatekeeper, setting a hard limit on requests within a time frame. This prioritizes fairness and prevents abuse. Throttling, on the other hand, functions like a dimmer switch, dynamically adjusting response times based on traffic. This ensures stability and performance by gracefully handling surges in requests.
Understanding the strengths of each approach allows API providers to create a robust access control system that balances user needs with the API's capacity, leading to a secure and performant experience for everyone.
With Apidog, you do not have to worry about limited requests. You can also import APIs that you want to understand and analyse them using Apidog's simple yet intuitive design. Begin your API development journey with Apidog today!