In the rapidly evolving landscape of web development, real-time communication has become essential for creating dynamic, responsive applications. Socket.IO stands at the forefront of this revolution, offering developers a powerful solution for bidirectional communication. This article delves into what Socket.IO is, how it functions, and introduces Apidog's groundbreaking Socket.IO debugging tool that transforms the development workflow.
Understanding Socket.IO: The Foundation of Real-time Web Applications
Socket.IO emerged as a solution to a critical problem in web development: enabling instant, two-way communication between clients and servers. Unlike traditional HTTP requests where clients initiate all communication, Socket.IO creates persistent connections that allow servers to push data to clients without solicitation.
At its core, Socket.IO is a JavaScript library that enables low-latency, bidirectional, and event-based communication. It consists of two parts: a client-side library that runs in the browser, and a server-side library for Node.js. This powerful combination creates a seamless channel for data exchange that feels instantaneous to users.
What makes Socket.IO particularly valuable is its pragmatic approach to connectivity. While WebSockets offer the ideal transport mechanism for real-time communication, they aren't universally supported or accessible. Socket.IO addresses this challenge by implementing an automatic fallback system:
- Primary transport: WebSocket connection whenever possible
- Fallback transport: HTTP long-polling when WebSockets are unavailable
This fallback capability ensures applications work reliably across different browsers, networks, and environments—even when corporate firewalls or proxies block WebSocket connections. The library handles this complexity transparently, allowing developers to focus on building features rather than managing connection logistics.
Socket.IO also provides several features that elevate it beyond a simple WebSocket implementation:
- Automatic reconnection: If connections drop, Socket.IO attempts to reestablish them with exponential back-off
- Packet buffering: Messages sent during disconnection are queued and delivered upon reconnection
- Acknowledgements: Confirmation mechanisms to verify message delivery
- Broadcasting: Ability to send messages to all connected clients or specific subgroups
- Namespaces and rooms: Logical separation of concerns within a single connection
These capabilities make Socket.IO an ideal choice for applications requiring real-time updates such as chat platforms, collaborative tools, gaming, live dashboards, and notification systems. By abstracting away the complexities of maintaining persistent connections, Socket.IO allows developers to create responsive, interactive experiences with relatively straightforward code.
How Socket.IO Works: The Technical Architecture Behind Real-time Communication
Understanding how Socket.IO
works requires examining its layered architecture. The library operates through two distinct layers that work in concert to deliver its functionality:
The Engine.IO Layer: The Communication Foundation
At the lower level, Engine.IO handles the establishment and maintenance of the connection between client and server. This layer manages:
- Transport selection and upgrades: Initially establishing connection via HTTP long-polling, then attempting to upgrade to WebSocket
- Connection state management: Tracking whether connections are open, closing, or closed
- Heartbeat mechanism: Sending regular PING/PONG packets to verify connection health
- Disconnection detection: Identifying when connections have terminated
The connection lifecycle begins with a handshake, where the server sends critical information:
{
"sid": "FSDjX-WRwSA4zTZMALqx",
"upgrades": ["websocket"],
"pingInterval": 25000,
"pingTimeout": 20000
}
This handshake establishes the session ID, available transport upgrades, and heartbeat parameters. The client then maintains this connection or attempts to upgrade it to WebSocket when possible.
What makes Engine.IO particularly robust is its upgrade mechanism. Rather than immediately attempting a WebSocket connection (which might fail and cause delays), it establishes a reliable HTTP long-polling connection first. Then, in the background, it attempts to upgrade to WebSocket. This approach prioritizes user experience by ensuring immediate connectivity while optimizing for better performance when possible.
The Socket.IO Layer: The Developer-Facing API
Built atop Engine.IO, the Socket.IO layer provides the high-level, event-based API that developers interact with. This layer implements:
- Event emission and handling: The
.emit()
and.on()
methods for sending and receiving events - Reconnection logic: Automatically attempting to reconnect with exponential back-off
- Packet buffering: Storing messages during disconnection for later delivery
- Multiplexing: Supporting multiple "namespaces" over a single connection
- Room management: Grouping clients for targeted broadcasting
The Socket.IO protocol transforms messages into a specific format before transmission. For example, socket.emit("hello", "world")
becomes a WebSocket frame containing 42["hello","world"]
, where:
4
indicates an Engine.IO "message" packet2
denotes a Socket.IO "message" packet["hello","world"]
represents the JSON-stringified arguments
This structured approach enables the rich feature set that makes Socket.IO more than just a WebSocket wrapper. It provides a consistent API regardless of the underlying transport mechanism, allowing developers to build with confidence across diverse environments.
Socket.IO Debugging Challenges: Why Traditional Tools Fall Short
Debugging Socket.IO applications presents unique challenges that conventional development tools struggle to address effectively. The real-time, event-driven nature of Socket.IO communication creates scenarios where traditional debugging approaches prove inadequate.
The primary challenges developers face when debugging Socket.IO include:
- Asynchronous event flow: Events can fire in unpredictable sequences, making it difficult to trace execution paths
- Bidirectional communication: Messages flow in both directions, requiring simultaneous monitoring of client and server
- Ephemeral data: Messages appear and disappear quickly, often without leaving traces in standard logs
- Connection lifecycle issues: Problems during connection establishment, upgrade, or reconnection can be difficult to diagnose
- Environment-specific behavior: Applications may work differently across browsers or networks due to transport fallback mechanisms
Conventional browser developer tools provide limited visibility into Socket.IO operations. While network panels can show WebSocket frames, they typically don't decode the Socket.IO protocol or organize messages by event types. Console logging helps but clutters code and requires manual instrumentation of each event.
Server-side debugging tools face similar limitations. Standard logging approaches capture events but struggle to correlate them with specific clients or visualize the bidirectional flow. This fragmented view forces developers to mentally reconstruct the communication sequence across different tools and logs.
These challenges extend beyond development into testing and production monitoring:
- Reproducing issues: Intermittent connection problems or race conditions prove notoriously difficult to replicate
- Testing event handlers: Verifying that all event handlers respond correctly requires manual triggering or complex test setups
- Performance analysis: Identifying bottlenecks in message processing or transmission requires specialized instrumentation
- Cross-environment verification: Ensuring consistent behavior across different browsers and network conditions demands extensive testing
The lack of specialized tools has historically forced developers to create custom debugging solutions or rely on a patchwork of general-purpose tools. This approach consumes valuable development time and often leaves blind spots in the debugging process.
A comprehensive Socket.IO debugging solution requires capabilities beyond what traditional development tools offer—specifically, the ability to monitor connections, decode protocol messages, trigger events manually, and visualize the bidirectional communication flow in real time.
Introducing Apidog's Socket.IO Debugging Tool
The landscape of Socket.IO development has fundamentally changed with Apidog's introduction of its dedicated Socket.IO debugging tool. This purpose-built solution addresses the unique challenges of real-time application development, offering unprecedented visibility and control over Socket.IO communications.
Apidog's Socket.IO debugging tool represents a significant advancement for developers working with real-time applications. It transforms the debugging experience from a fragmented, multi-tool process into a streamlined workflow within a single, intuitive interface.
Key Capabilities of Apidog's Socket.IO Debugging Tool
The tool provides a comprehensive set of features designed specifically for Socket.IO development:
- Connection management: Establish, monitor, and terminate Socket.IO connections with complete control over connection parameters
- Event listening: Subscribe to specific events and view incoming messages in real-time with automatic decoding
- Message sending: Trigger events with custom payloads, including support for acknowledgments and multiple arguments
- Timeline visualization: Chronological display of all Socket.IO communications with clear distinction between sent and received events
- Protocol inspection: Detailed view of the underlying Socket.IO and Engine.IO protocols, including handshake parameters
- Environment configuration: Fine-grained control over client version, handshake path, and connection parameters
- Variable support: Use environment variables and dynamic values in messages for testing different scenarios
These capabilities address the core challenges of Socket.IO debugging by providing a unified interface for monitoring and interacting with Socket.IO connections. Developers gain the ability to:
- Observe the complete communication flow between client and server
- Trigger events manually to test server responses
- Verify event handlers by sending various payload formats
- Troubleshoot connection issues by examining handshake parameters and transport selection
- Document Socket.IO endpoints for team collaboration
The tool integrates seamlessly with Apidog's broader API development ecosystem, allowing teams to manage Socket.IO endpoints alongside REST, GraphQL, and other API types. This integration creates a unified workflow for all API development activities, from design and testing to documentation and collaboration.
Real-world Impact on Development Workflow
For development teams, Apidog's Socket.IO debugging tool delivers tangible benefits:
- Reduced debugging time: Problems that previously required hours of investigation can often be identified in minutes
- Improved collaboration: Shared Socket.IO endpoint configurations ensure consistent testing across team members
- Better documentation: Automatically generated Socket.IO endpoint documentation improves knowledge sharing
- Faster development cycles: The ability to quickly test Socket.IO interactions accelerates feature development
- Higher quality: More thorough testing of real-time features leads to more reliable applications
By providing specialized tools for Socket.IO debugging, Apidog eliminates the need for custom debugging solutions or complex test setups. This allows developers to focus on building features rather than creating and maintaining debugging infrastructure.
Step-by-Step Guide: Debugging Socket.IO Endpoints with Apidog
Apidog's Socket.IO debugging tool transforms the development experience through an intuitive, powerful interface. This comprehensive guide walks through the process of using this tool to debug Socket.IO applications effectively.
Setting Up Your Socket.IO Debugging Environment
1. Create a new Socket.IO endpoint
- Launch Apidog (version 2.7.0 or higher)
- Hover over the
+
button in the left panel - Select "New Socket.IO" from the dropdown menu
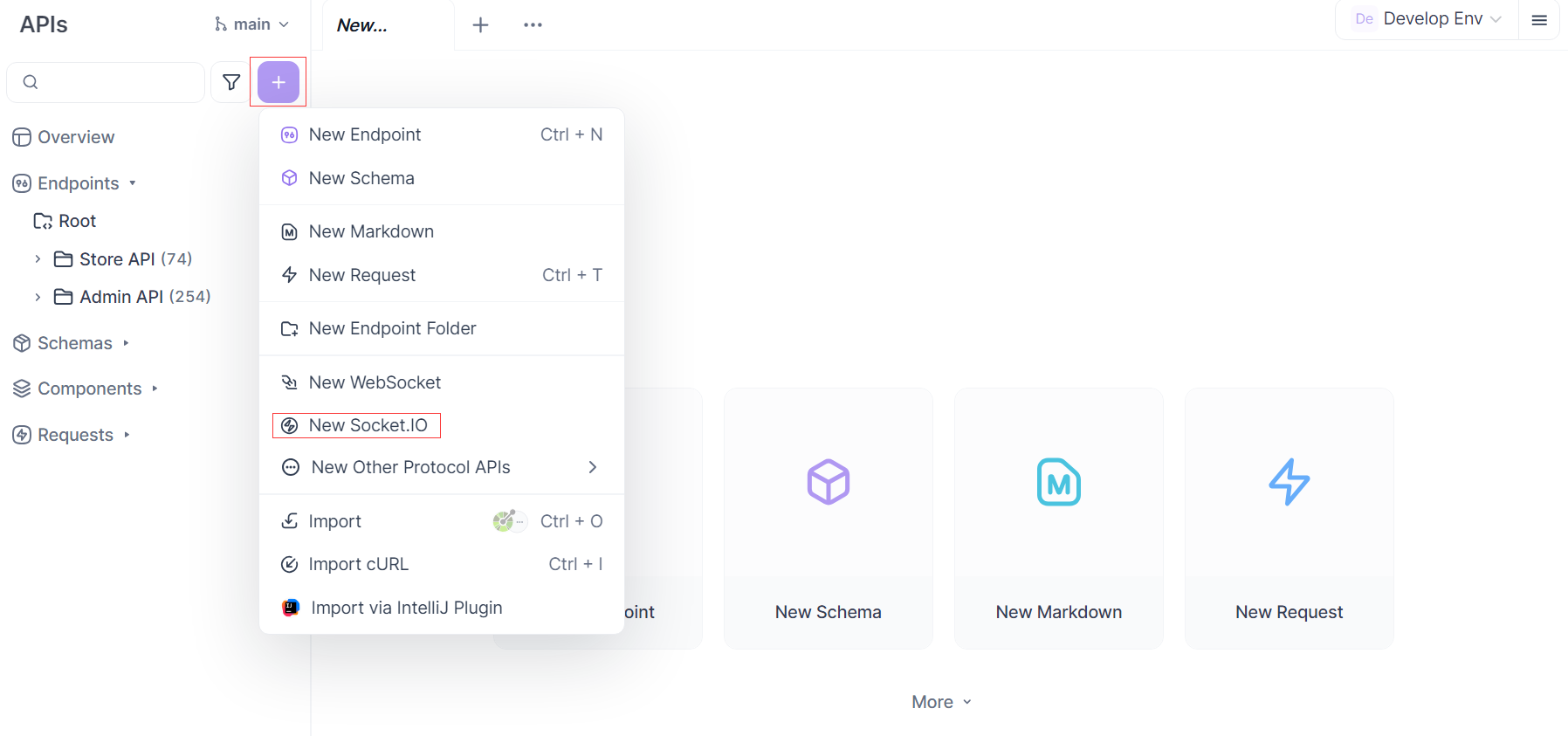
2. Configure the connection parameters
- Enter the server address (e.g.,
ws://localhost:3000
orwss://example.com
)
- Add any required handshake parameters:
- URL parameters directly in the address
- Additional parameters in the "Params" tab
- Authentication headers in the "Headers" tab
- Cookies in the "Cookies" tab
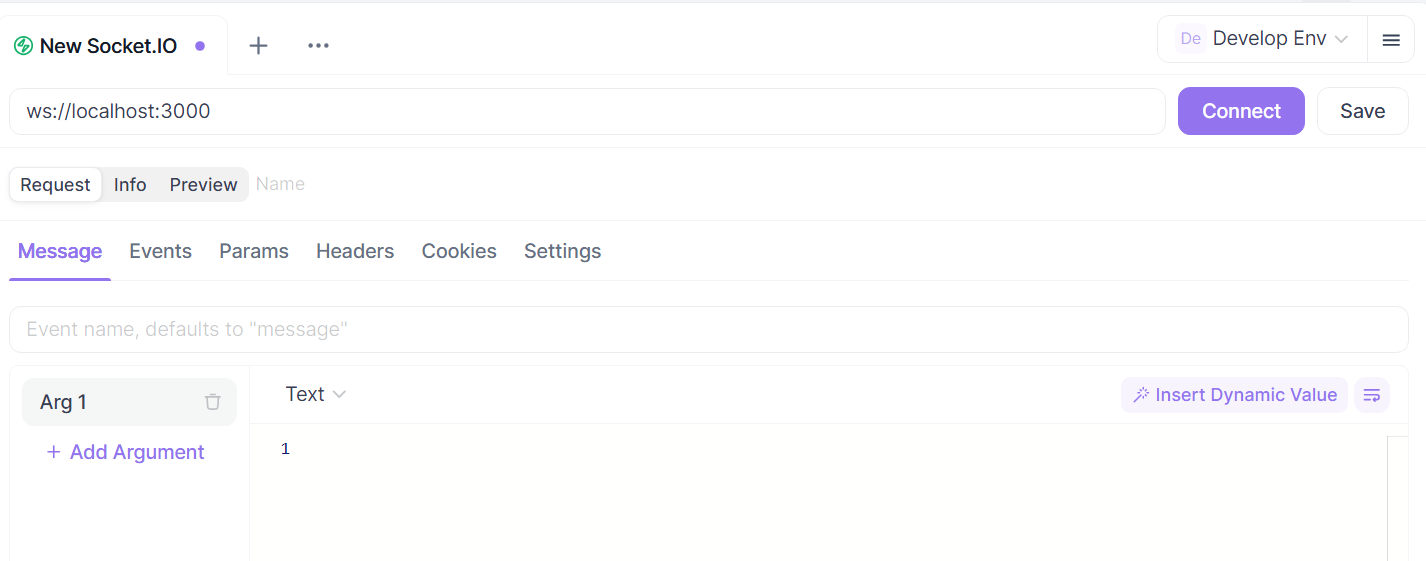
3. Adjust advanced settings if necessary
- Click "Settings" under the "Request" section
- Select the appropriate client version (default is v4, but v2/v3 are supported)
- Modify the handshake path if your server uses a custom path (default is
/socket.io
)
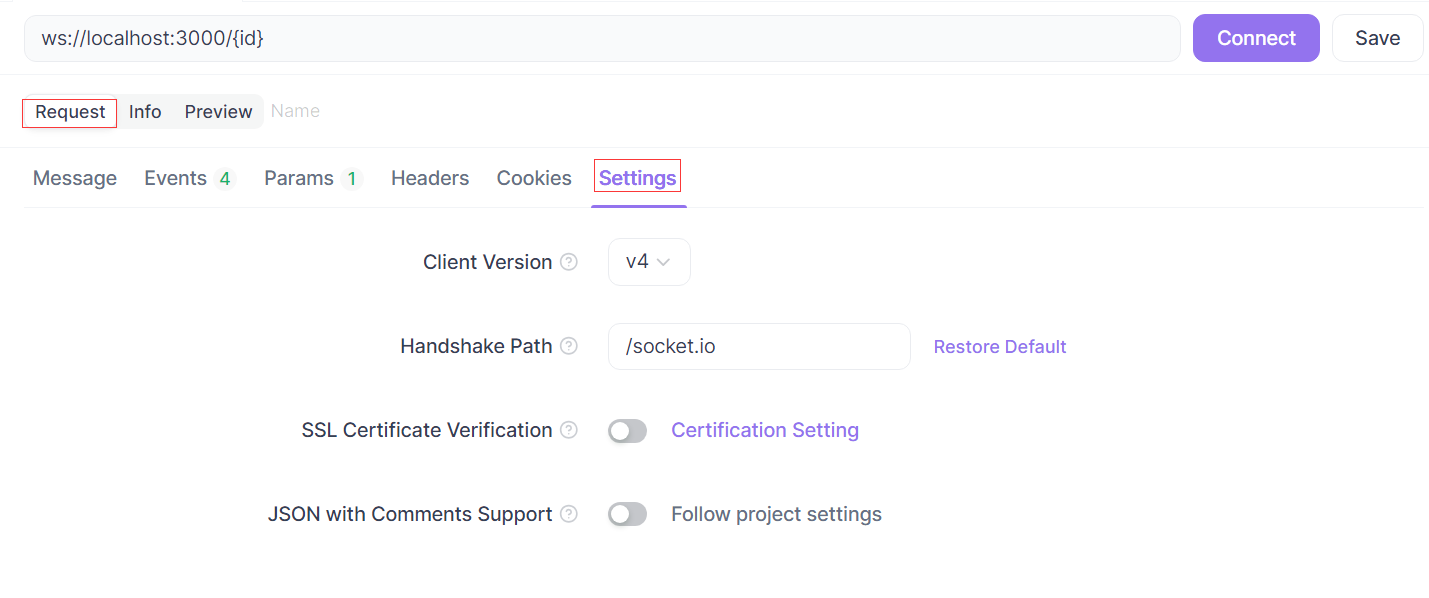
4. Establish the connection
- Click the "Connect" button to initiate the Socket.IO connection
- The connection status will update to indicate success or failure
- If the connection fails, check the error message for troubleshooting guidance
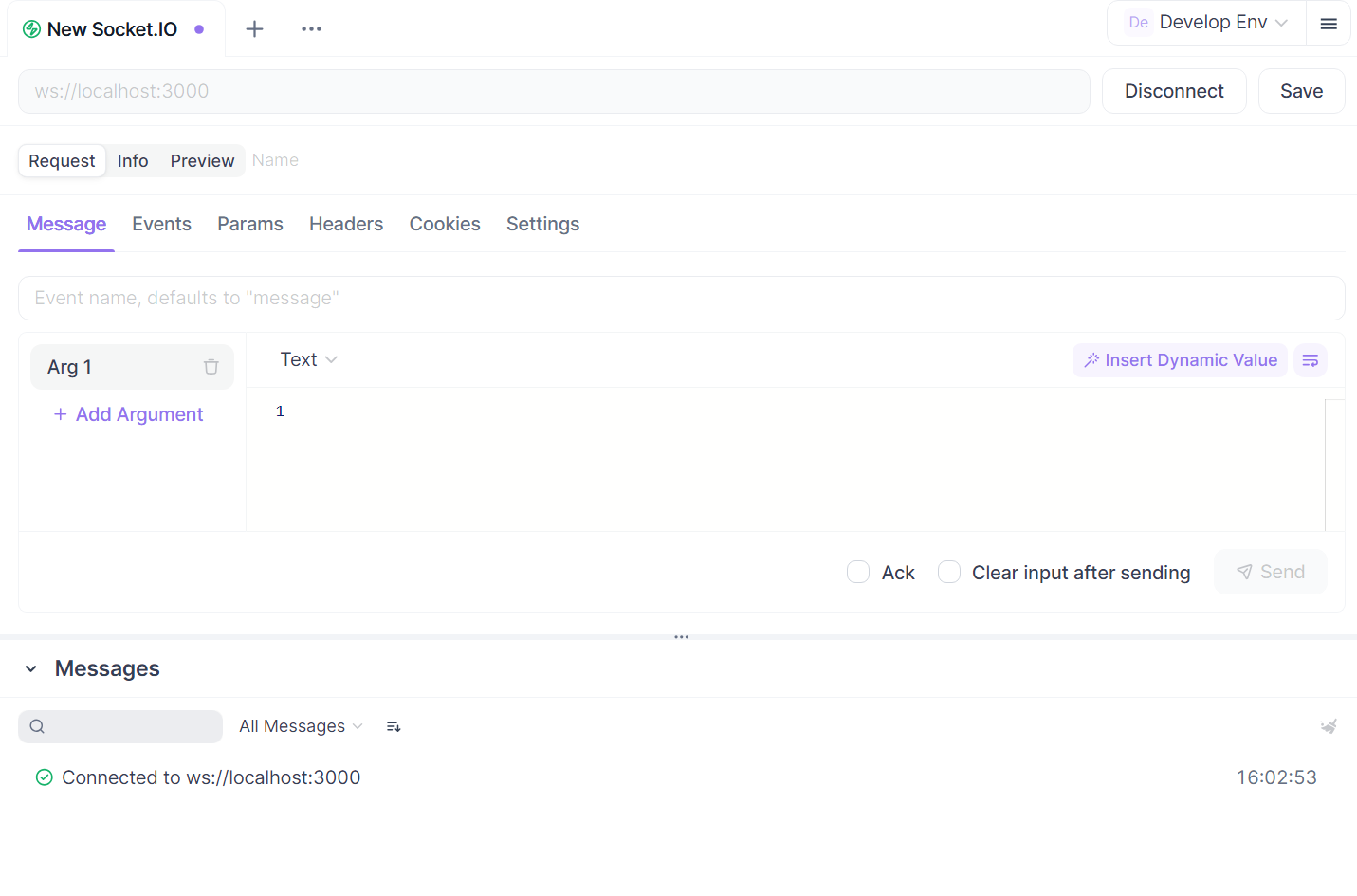
Monitoring and Interacting with Socket.IO Events
1. Listen for events
- Navigate to the "Events" tab
- The system listens for the
message
event by default - Add custom events by entering their names and enabling the "Listen" toggle
- Received events appear in the timeline with their payloads automatically decoded
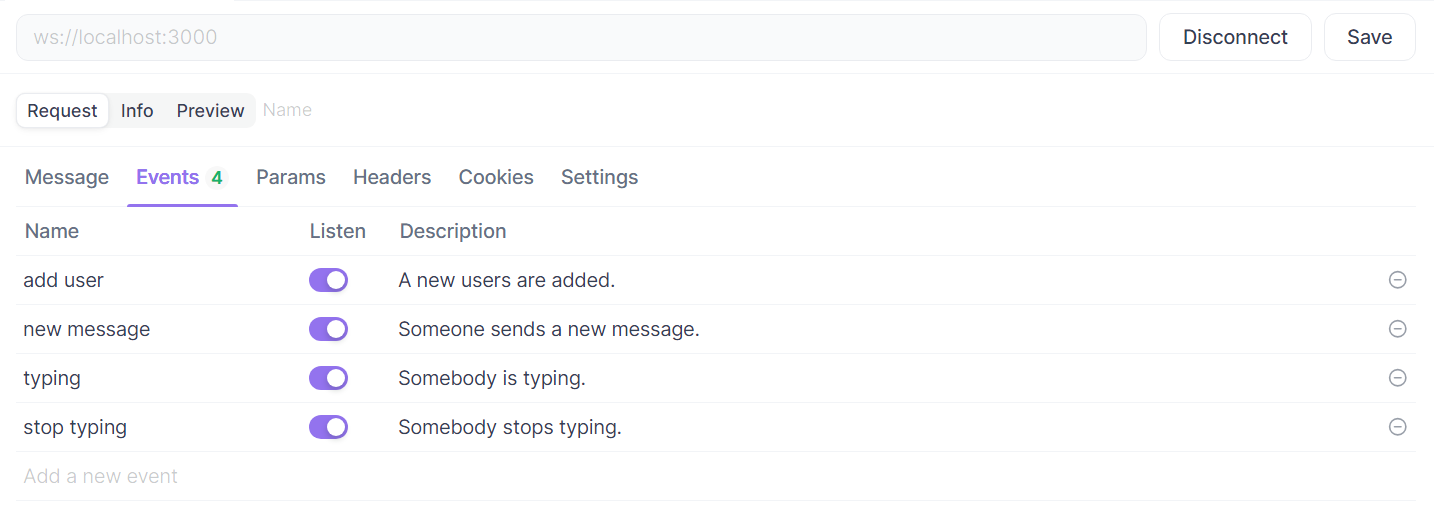
2. Send messages to the server
- Set the event name (defaults to
message
)
- Configure the argument(s):
- Select the appropriate format (JSON, text, or Binary)
- Enter the payload content
- Add multiple arguments if needed using the "+ Add Argument" button
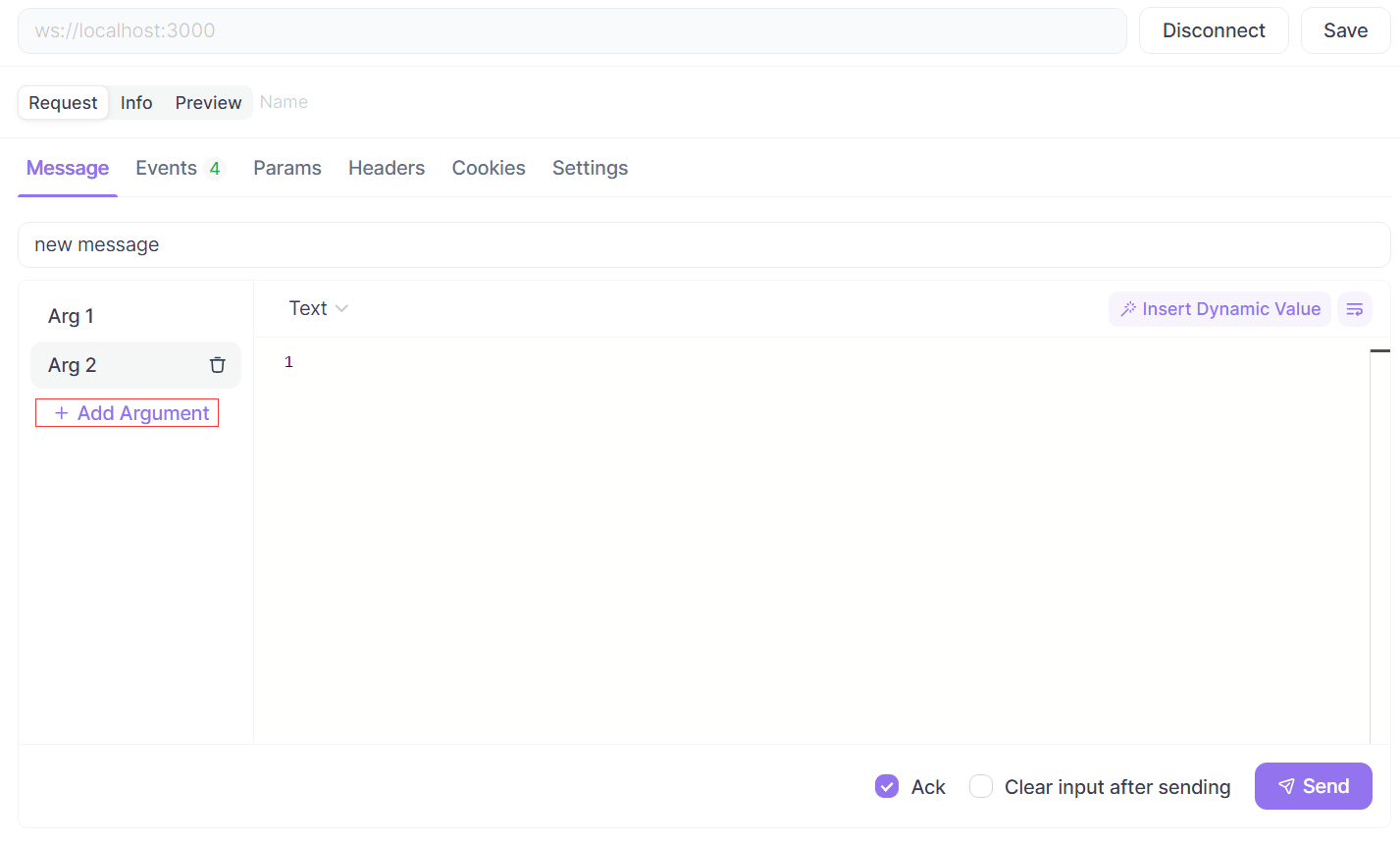
- Enable "Ack" if you expect a callback response
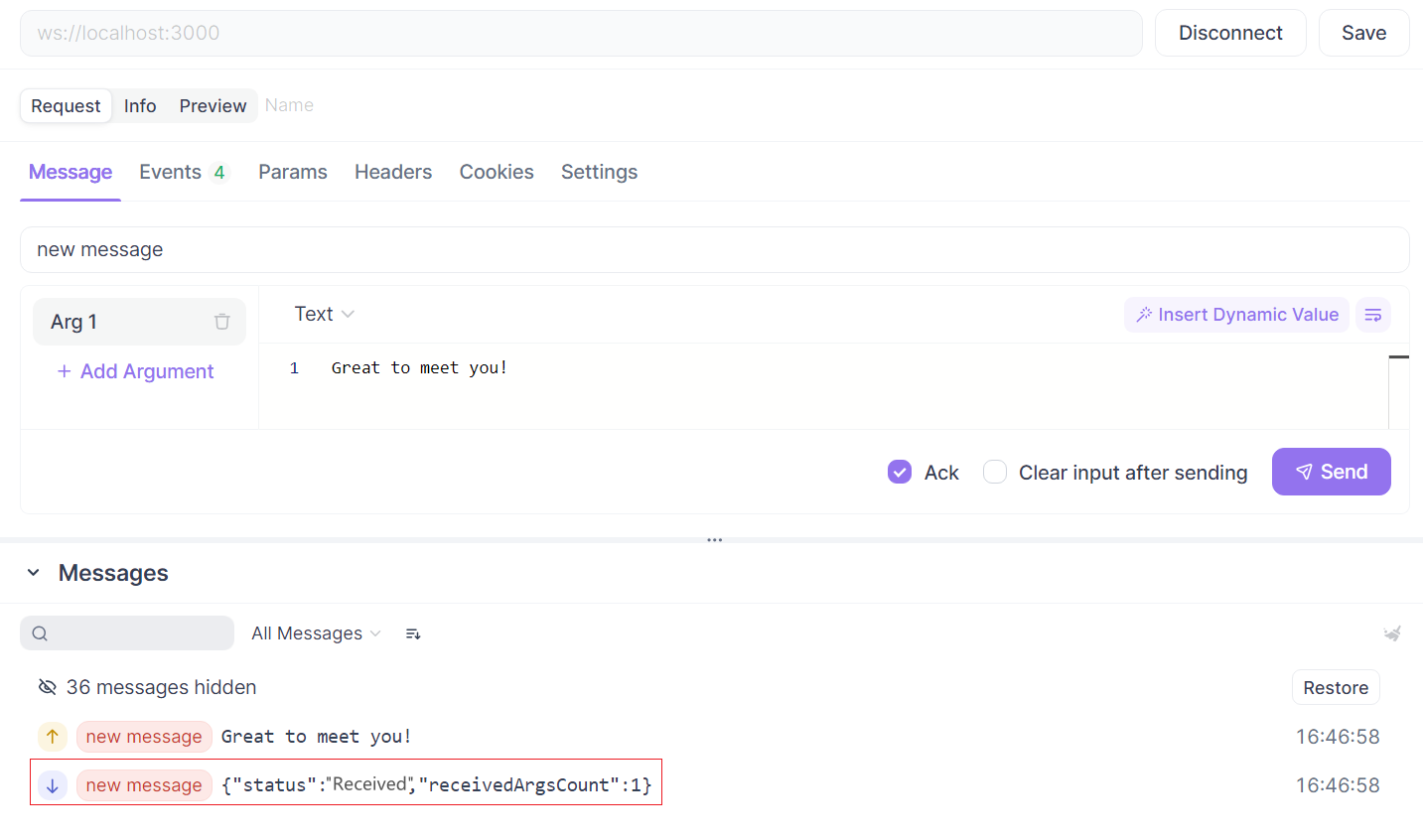
- Click "Send" to transmit the message
3. Analyze the communication timeline
- Review the chronological list of all sent and received events
- Events are tagged with their names for easy identification
- Click on any event to view its detailed payload
- For messages with multiple arguments, expand the "x Args" label to see all values
- Switch between tabs on the right panel for different perspectives on the data
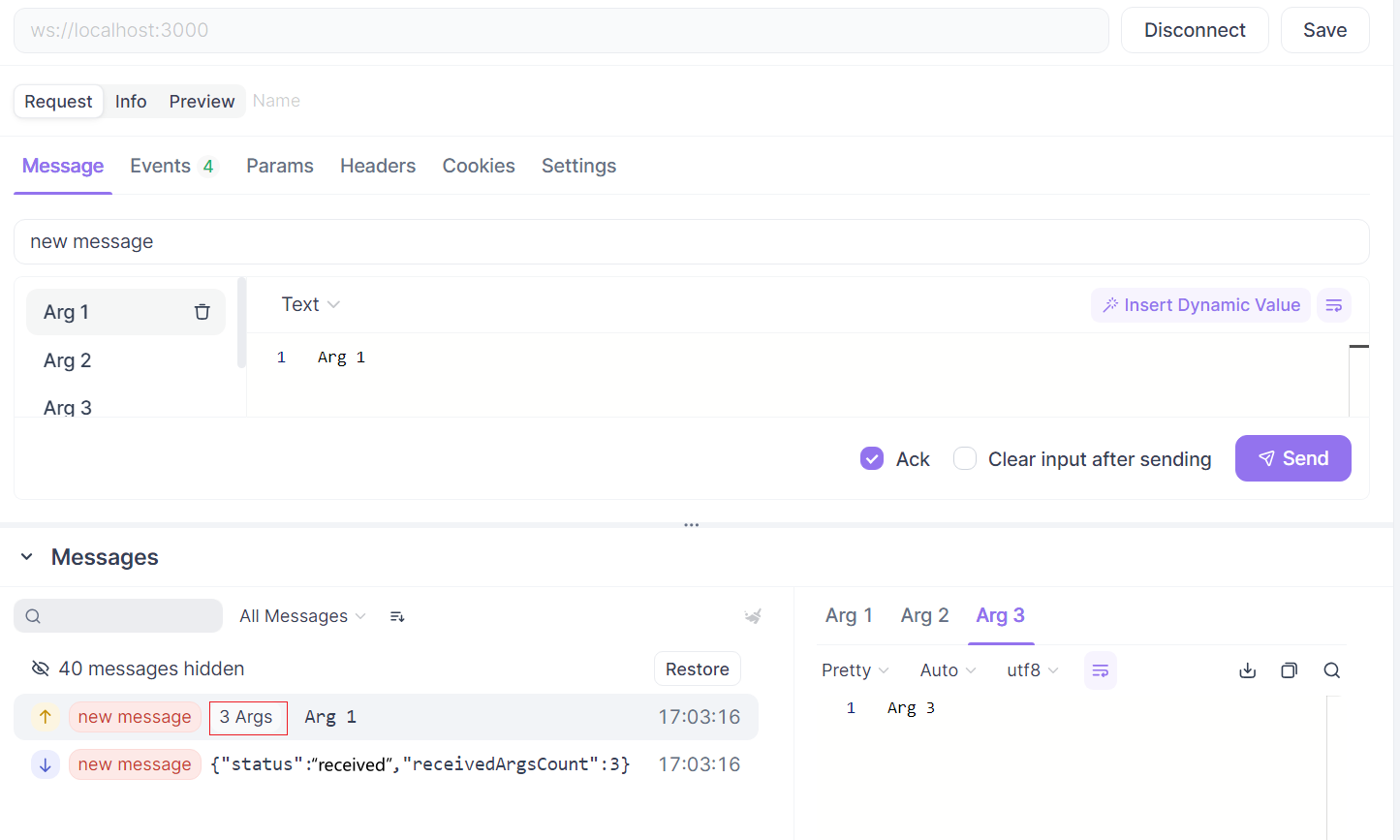
4. Utilize variables for dynamic testing
- Insert environment variables in your arguments using the
{{variable}}
syntax - These variables are automatically replaced with their actual values when sending
- This enables testing different scenarios without manually changing payloads
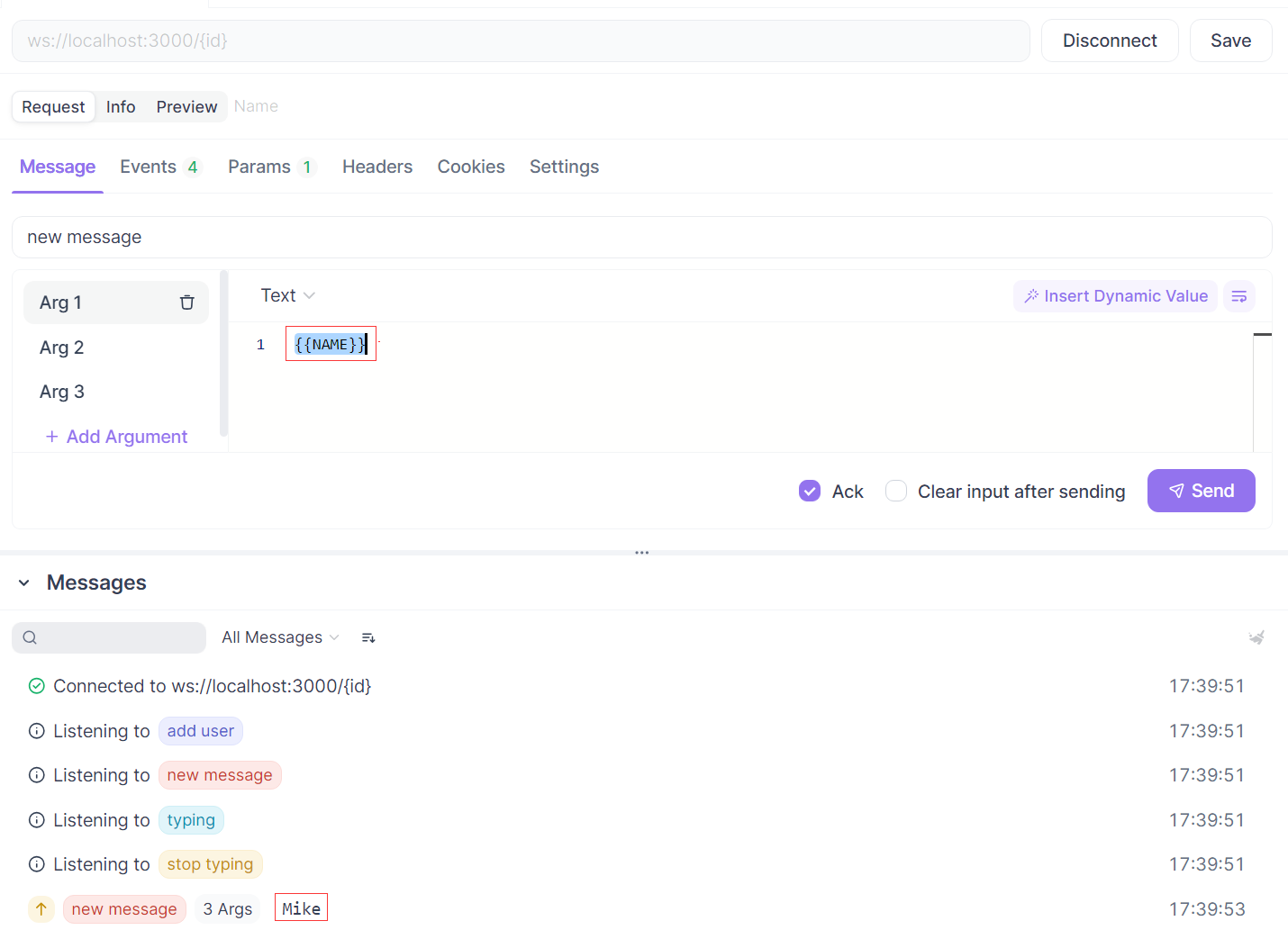
Troubleshooting Connection Issues
When facing connection problems, Apidog provides several diagnostic approaches:
1. Verify server availability
- Ensure the Socket.IO server is running and accessible
- Check network connectivity to the server address
2. Examine handshake parameters
- Review the handshake request in the timeline
- Verify that authentication tokens or cookies are correctly configured
- Check that the client version matches the server's expected version
3. Inspect transport selection
- Monitor the upgrade process in the timeline
- Confirm whether the connection successfully upgrades to WebSocket
- If using long-polling, investigate potential WebSocket blockers in your network
4. Test with different configurations
- Try alternative client versions
- Modify handshake paths if your server uses custom settings
- Adjust timeout values for problematic networks
Documenting and Sharing Socket.IO Endpoints
After successful debugging, preserve your configuration for future use:
1. Save the endpoint
- Click the "Save" button to store the Socket.IO endpoint
- Select a location in your project's folder structure
- Add a descriptive name and optional description
2. Enhance documentation
- Set the endpoint status (e.g., "Development", "Testing", "Production")
- Assign maintainers responsible for the endpoint
- Add relevant tags for categorization
- Write detailed descriptions using Markdown formatting
3. Share with team members
- Generate online API documentation including your Socket.IO endpoints
- Share the documentation URL with team members
- Collaborators can import the exact configuration for consistent testing
Benefits of Using Apidog for Socket.IO Debugging
The advantages of Apidog's approach to Socket.IO debugging extend throughout the development lifecycle:
- Comprehensive visibility: See both sides of the communication in a single interface
- Structured testing: Systematically verify event handlers with various payloads
- Efficient troubleshooting: Quickly identify and resolve connection issues
- Improved collaboration: Share configurations and findings with team members
- Better documentation: Automatically document Socket.IO endpoints alongside other APIs
- Streamlined workflow: Integrate Socket.IO testing into your broader API development process
By providing these capabilities in an intuitive interface, Apidog eliminates the need for custom debugging solutions or complex test setups. Developers can focus on building features rather than creating and maintaining debugging infrastructure.
Conclusion: Transforming Socket.IO Development with Specialized Tools
Socket.IO has revolutionized real-time web applications by providing a reliable, feature-rich solution for bidirectional communication. However, the power of Socket.IO comes with inherent complexity that traditional debugging tools struggle to address. Apidog's Socket.IO debugging tool fills this critical gap, offering developers a purpose-built solution that transforms the development experience.
By providing comprehensive visibility into Socket.IO communications, streamlining the testing process, and integrating with broader API development workflows, Apidog enables developers to build better real-time applications more efficiently. The tool's intuitive interface and powerful features address the unique challenges of Socket.IO development, from connection management to event debugging.
For development teams working with Socket.IO, Apidog's debugging tool represents a significant advancement in their toolkit. It reduces debugging time, improves collaboration, enhances documentation, and ultimately leads to higher-quality real-time applications. As real-time features become increasingly central to modern web applications, specialized tools like Apidog's Socket.IO debugger will play an essential role in the development process.
Developers interested in experiencing these benefits firsthand can download Apidog (version 2.7.0 or higher) and start using the Socket.IO debugging tool today. Whether you're building a chat application, collaborative editor, live dashboard, or any other real-time feature, Apidog provides the visibility and control needed to develop with confidence.