The phrase "SOAP cURLs" comprises two popular technologies you may have heard before. Before anything else, this article discusses the technologies and their capabilities.
If you need an API platform to build, mock, debug, or document APIs, Apidog is an optimal choice. All you have to do is click the button below. 👇 👇 👇
Basic Terminologies on SOAP and cURLs
The terms "SOAP" and "cURL" each refer to a unique technology.
- SOAP: SOAP (Simple Object Access Protocol) is a messaging protocol used primarily for exchanging structured information between two applications or systems.
It serves an important role in the web services industry due to its ability to facilitate data exchange and communication between different software components.
SOAP is a highly structured protocol that utilizes the XML format, mainly used for enterprise applications or legacy systems. With this mentioned, you may have observed other protocols becoming more popular, such as the heavily discussed REST architectural style.
SOAP web services also usually come in the form of WSDL files. - cURL: cURL stands for "Client for URL". cURL is a free, open-source software that provides two main functionalities, a command-line tool
curl
and a librarylibcurl
.
The command-line toolcurl
allows data transfers by using various network protocols like HTTP, HTTPS, and many other types directly from your terminal. There are many usages of curl. To name a few, you can download files, send data, and retrieve the content of a web page or server response.
On the other hand, the librarylibcurl
acts as the core functionality for data transfers.libcrul
can be integrated into various popular programming languages, such as C, C++, Python, and PHP. Withlibcrul
, developers can leverage a cURL's performance with applications for tasks like automating file downloads and uploads, as well as building custom tools or applications for interacting with web services.
When the terms are combined, "SOAP cURLs" refers to using a cURL's functionalities to specifically interact with SOAP-based web services.
Breakdown of How SOAP cURLs Work
1. Define the SOAP message:
- Firstly, a specific SOAP request message is required. This involves determining the operation you want to call on the web service and the data you want to send, all in XML format.
- You can find various tools and resources available to help you define the SOAP message, such as the WSDL (Web Services Description Language) file provided by the web service or online tools for generating SOAP messages.
2. Construct the cURL command:
- Next, construct a cURL command specifying the details of the HTTP request carrying the SOAP message. This involves using various cURL options to define:
URL: The address of the web service endpoint you want to reach.
Method: The HTTP method is typically set to "POST" as SOAP messages are usually sent using the POST method.
Headers: Specific headers for SOAP communication, such as "Content-Type: text/xml; charset=utf-8" are required to indicate the message format and character encoding. - Data: The actual SOAP message content will be included in the request body using the "-d" option with the message string or a file containing the message.
3. Sending the request and receiving a response:
- Once you have the complete cURL command, you can execute it in your terminal. This will send the crafted HTTP request containing the SOAP message to the web service.
- The web service will process the request and return a response, typically in XML format.
4. Further processing of the response:
- You can parse the XML response to extract the relevant information returned by the web service. This might involve using tools or libraries depending on the programming language used.
How to Call SOAP API Using cURL
Step 1: First, you will need to prepare your SOAP message. In case this is your first time creating a SOAP message, you can refer to the code sample below - however, you will need to modify it to ensure it satisfies your needs.
Sample SOAP message:
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:ws="http://www.example.com/webservice">
<soapenv:Header/>
<soapenv:Body>
<ws:getGreeting>
<ws:name>John Doe</ws:name>
</ws:getGreeting>
</soapenv:Body>
</soapenv:Envelope>
Step 2: Prepare your cURL command on your terminal. Similar to the step above, you can take a look at the code sample.
Sample cURL command:
curl -X POST \
-H "Content-Type: text/xml; charset=utf-8" \
-H "SOAPAction: http://www.example.com/webservice/getGreeting" \
-d @soap_request.xml "https://dummy.webservice.com/path/to/service"
cURL command explanation:
-X POST
: Specifies the HTTP request method as POST.-H "Content-Type: text/xml; charset=utf-8"
sets the content-type header by indicating XML data with UTF-8 encoding.-H "SOAPAction: http://www.example.com/webservice/getGreeting"
sets the SOAPAction header by specifying the targeted operation.-d @soap_request.xml
reads the SOAP message content from a file named "soap_request.xml"."https://dummy.webservice.com/path/to/service"
is replaced with the actual URL of the web service endpoint.
Real-Life Applications of SOAP cURLs
1. Legacy system integration: SOAP was often used in older enterprise applications. If you need to interact with such legacy systems that haven't been migrated to newer protocols, cURL can provide a way to manually craft and send SOAP requests for data exchange.
2. Testing and debugging SOAP web services: Developers can use cURL to directly send specific SOAP requests and analyze the responses to troubleshoot issues with SOAP web services. This allows for granular control over the request and helps isolate potential problems.
3. Custom scripts and automation: cURL can be integrated into scripts and programs to automate tasks involving SOAP communication. This could be useful for scenarios like periodically fetching data from a SOAP service or triggering specific actions based on received SOAP messages.
Apidog - Makes SOAP Testing Easier
Apidog is an API development platform that provides numerous functionalities to personalize APIs to your liking. Whether you are planning to create a new API from scratch or import a personal API you have been working on for some time, Apidog supports either choice.
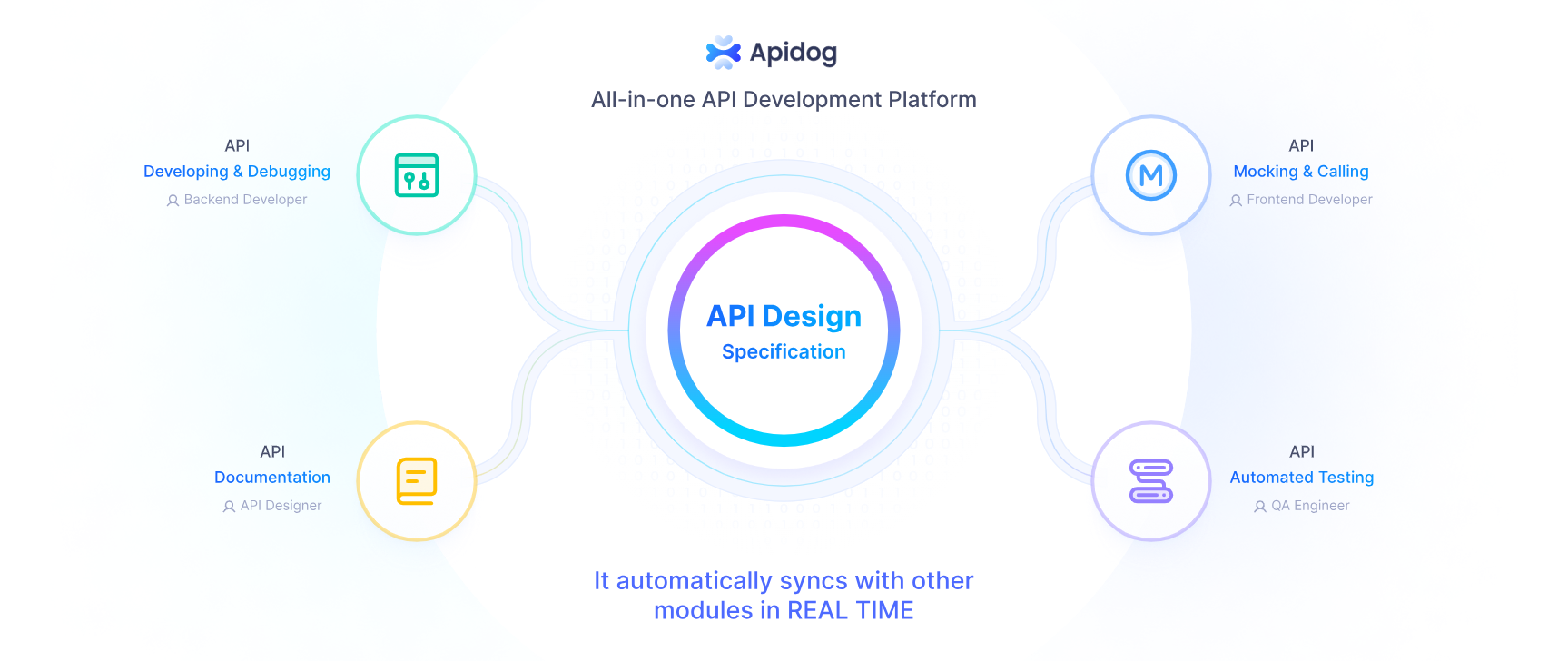
Apidog supports the imports of WSDL files so that you can view SOAP-based web services. On another note, you can also generate cURL commands using Apidog's client language code generation, so you do not have to second guess whether you are right or wrong!
Importing WSDL Files to View SOAP-based Web Services on Apidog
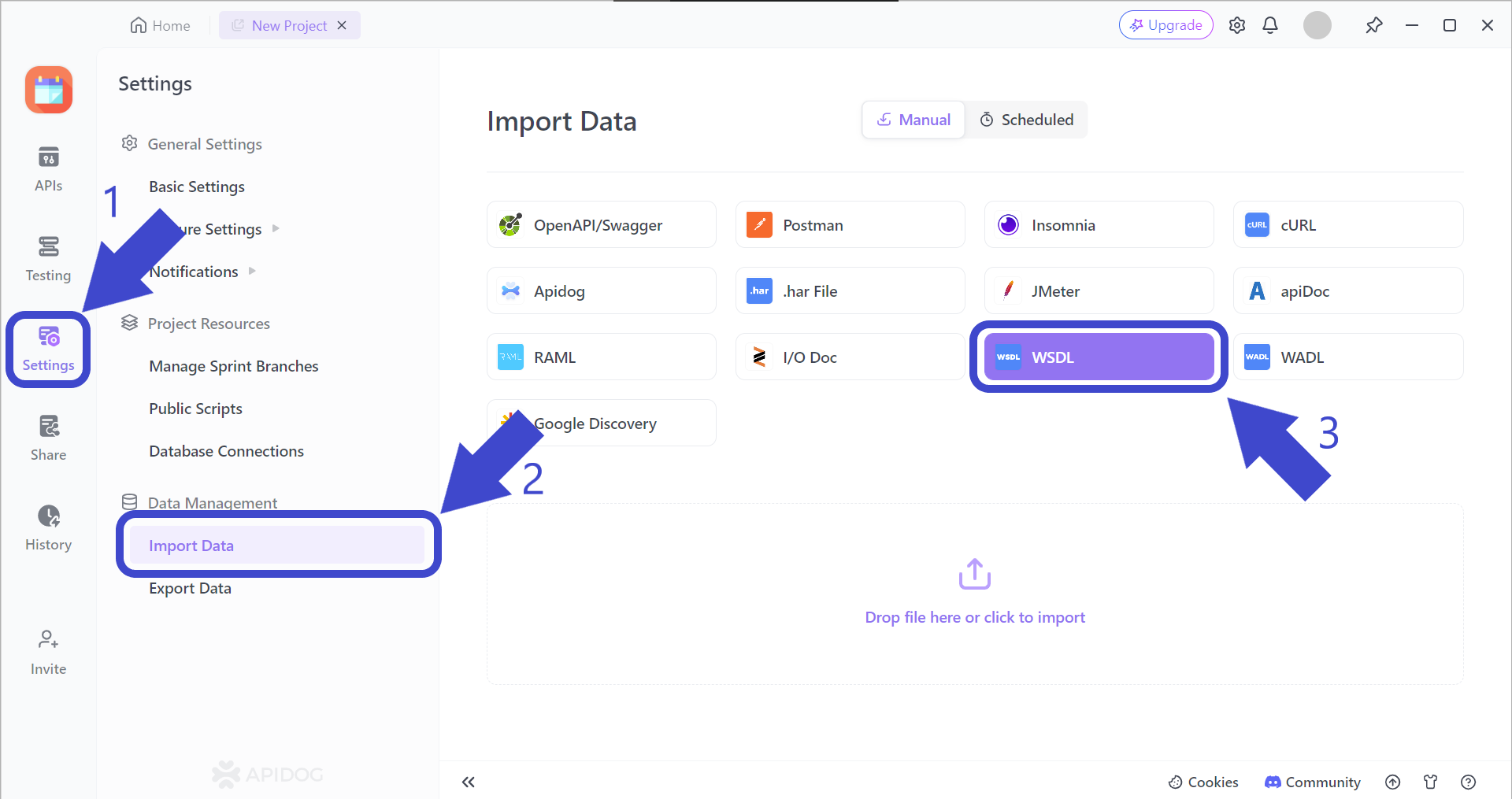
Firstly, press the Settings
button found on the left side of the vertical toolbar. Then, locate Import Data
under the Data Management
section. Lastly, choose WSDL
to import WSDL files onto Apidog.
Editing WSDL Files on Apidog
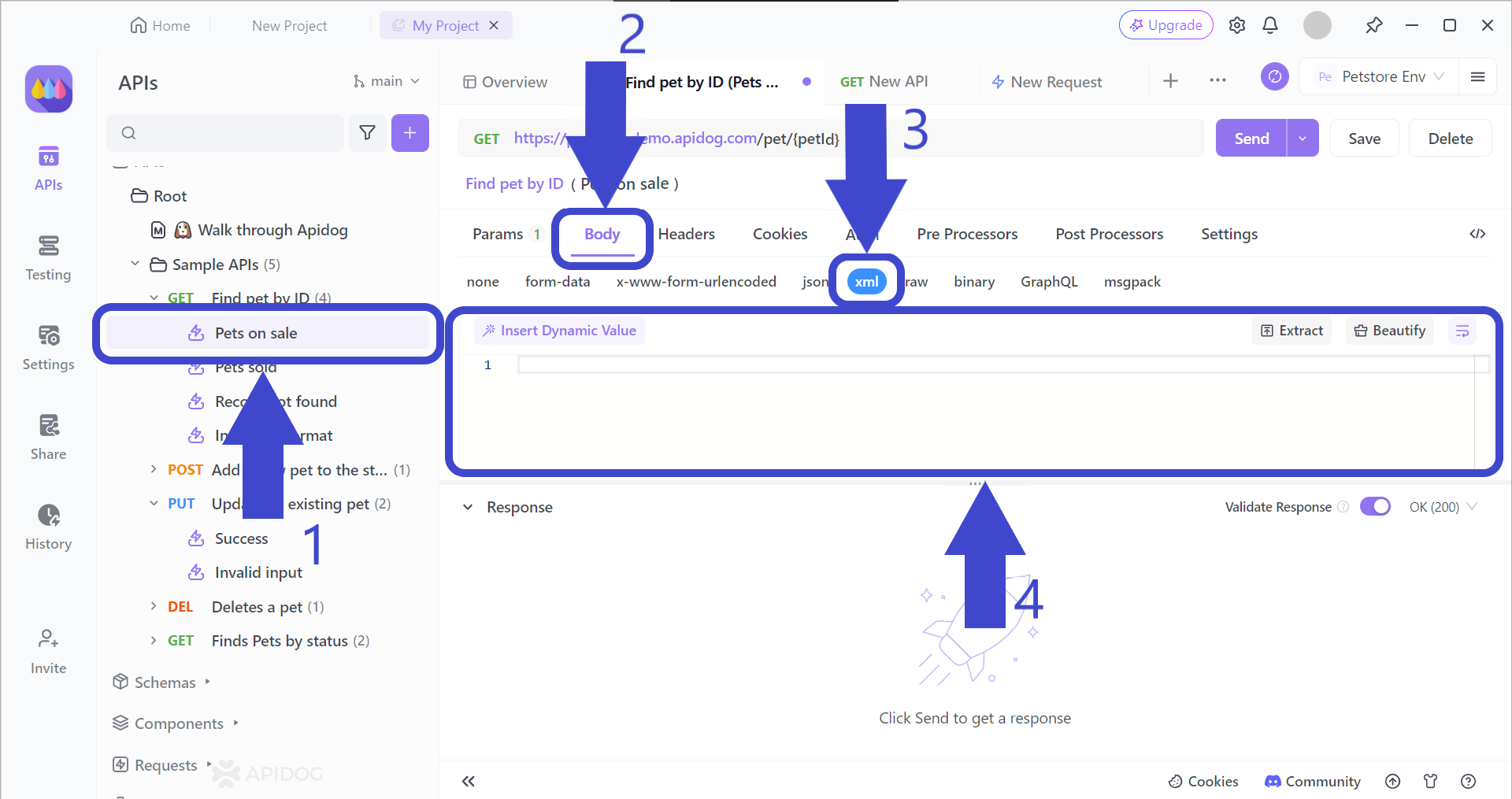
If the WSDL file has been successfully imported, you will find your WSDL file on the left, as shown in the image pointed out by Arrow 1. You can then click the numbered arrows to start editing.
Once you have your WSDL file imported and debugged, you can start testing it on Apidog for any more errors. If you are still unsure whether your WSDL file is right or wrong, check out WSDL file examples for more details.
Importing cURL to Apidog
Apidog supports importing cURL command line codes for those who have it ready.
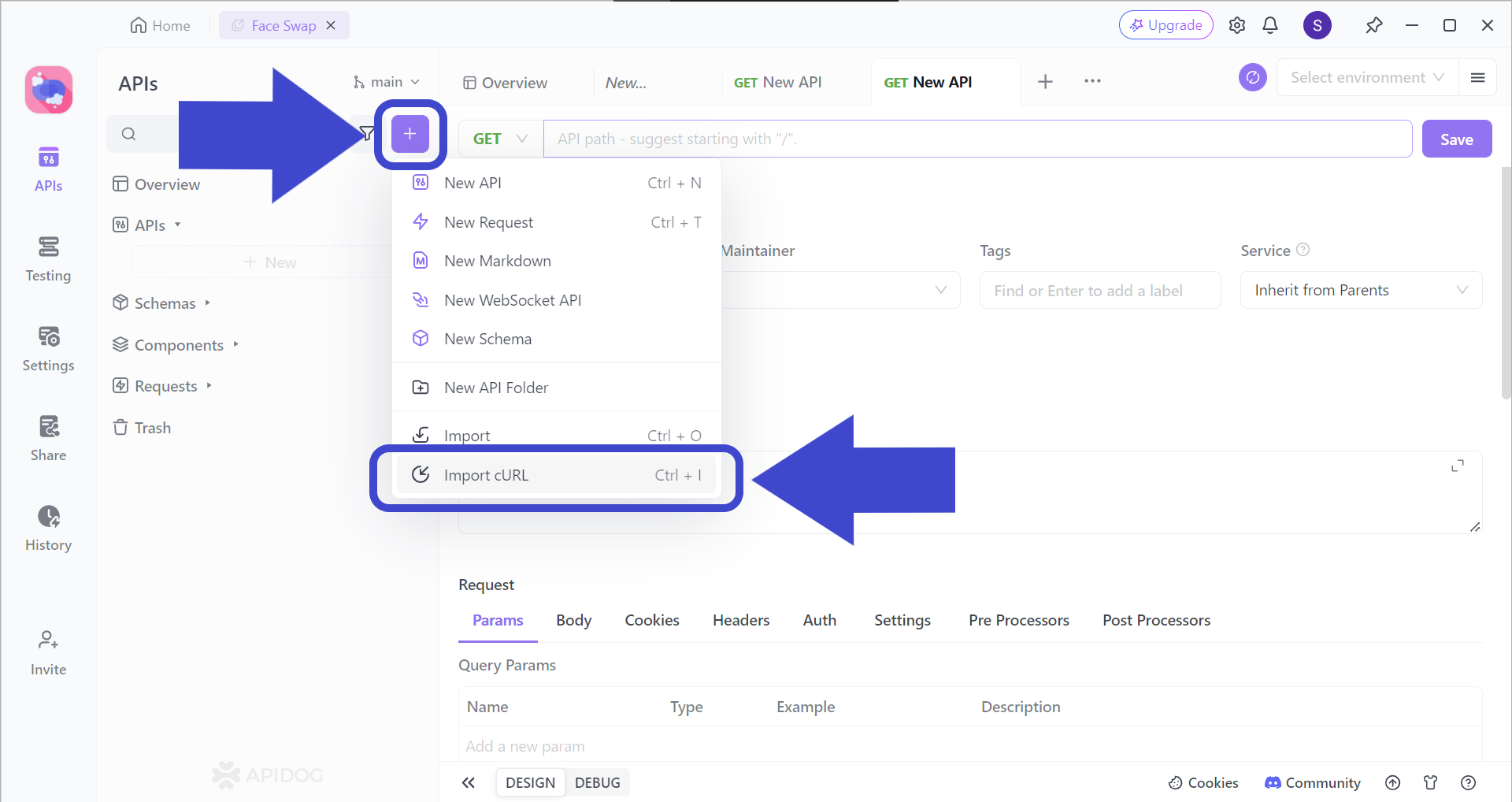
On the APIs
section, find a purple +
button found around the top left corner of the Apidog window. Then select Import cURL
. If you cannot find the mentioned button, you can use the shortcut, Ctrl + I
.
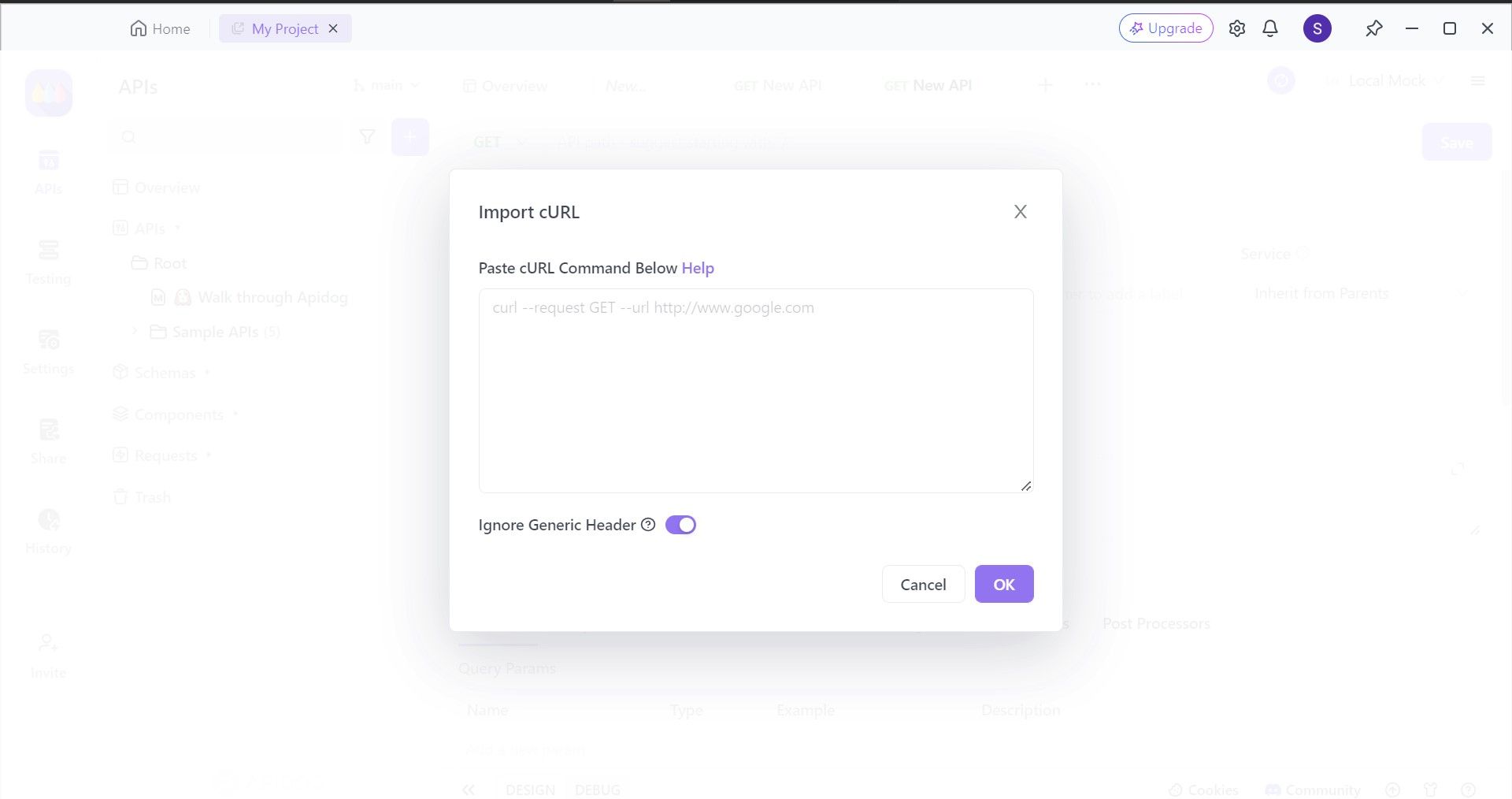
Here, you can paste your cURL command. Once you have, press Enter
on your keyboard or the OK
button to view your cURL command.
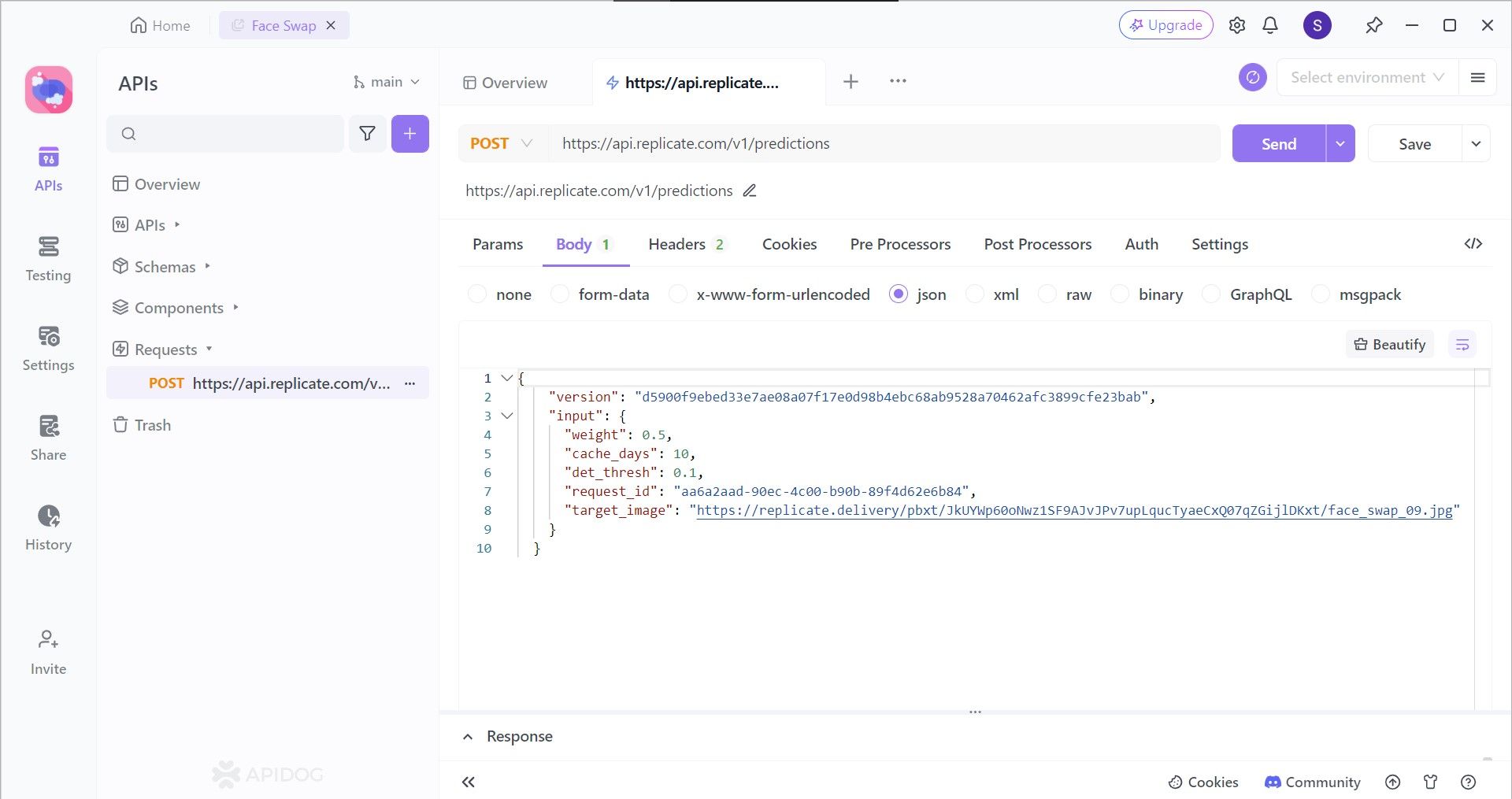
if successful, you can view the cURL command as part of the JSON body, as shown in the picture above. Here, you can do tweaks or changes, wherever and whenever required.
cURL Command Code Generation Using Apidog
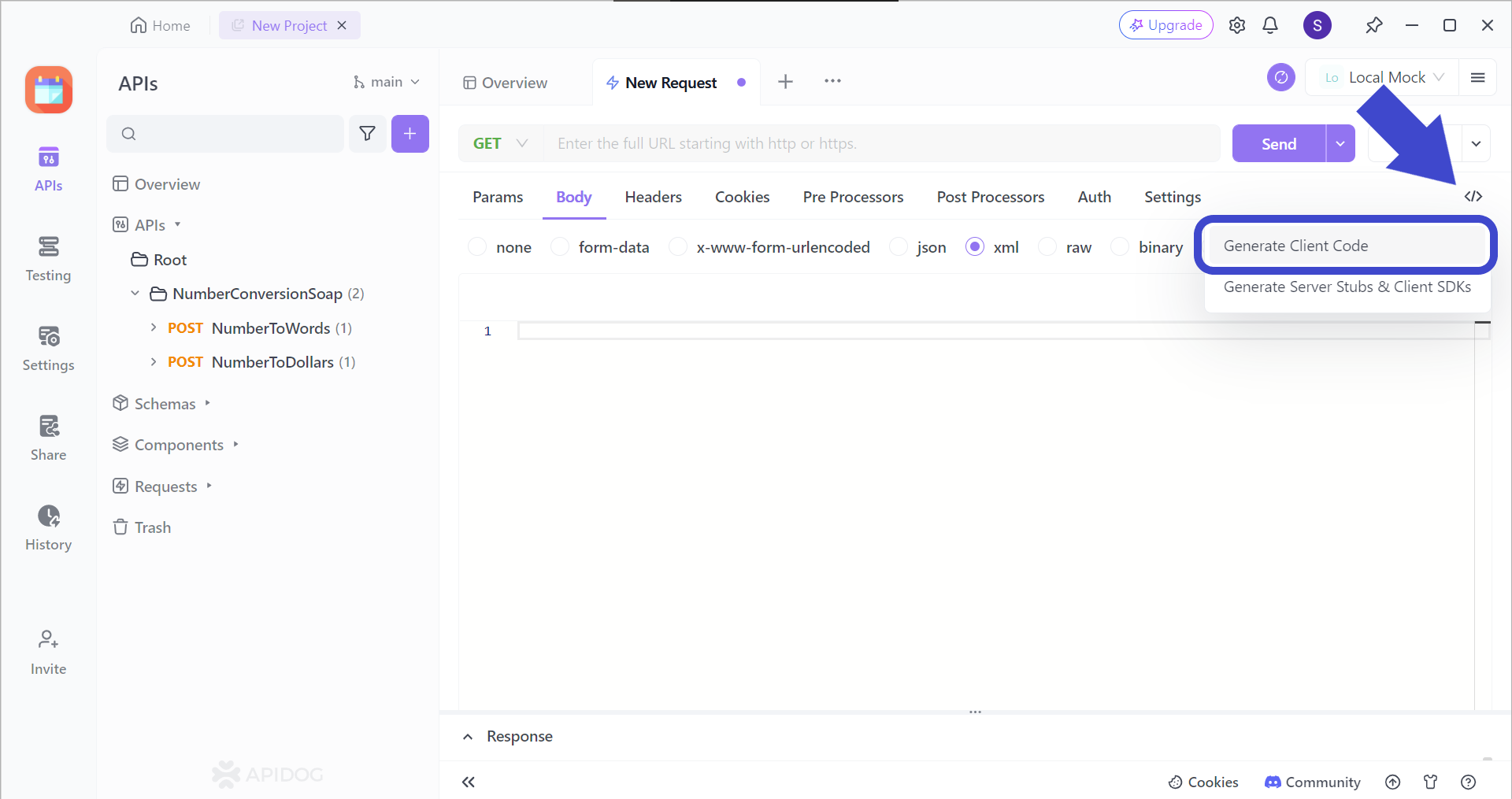
To begin cURL code generation, locate and press the </>
button found in the top right corner of the Apidog window. Then, select Generate Client Code
.
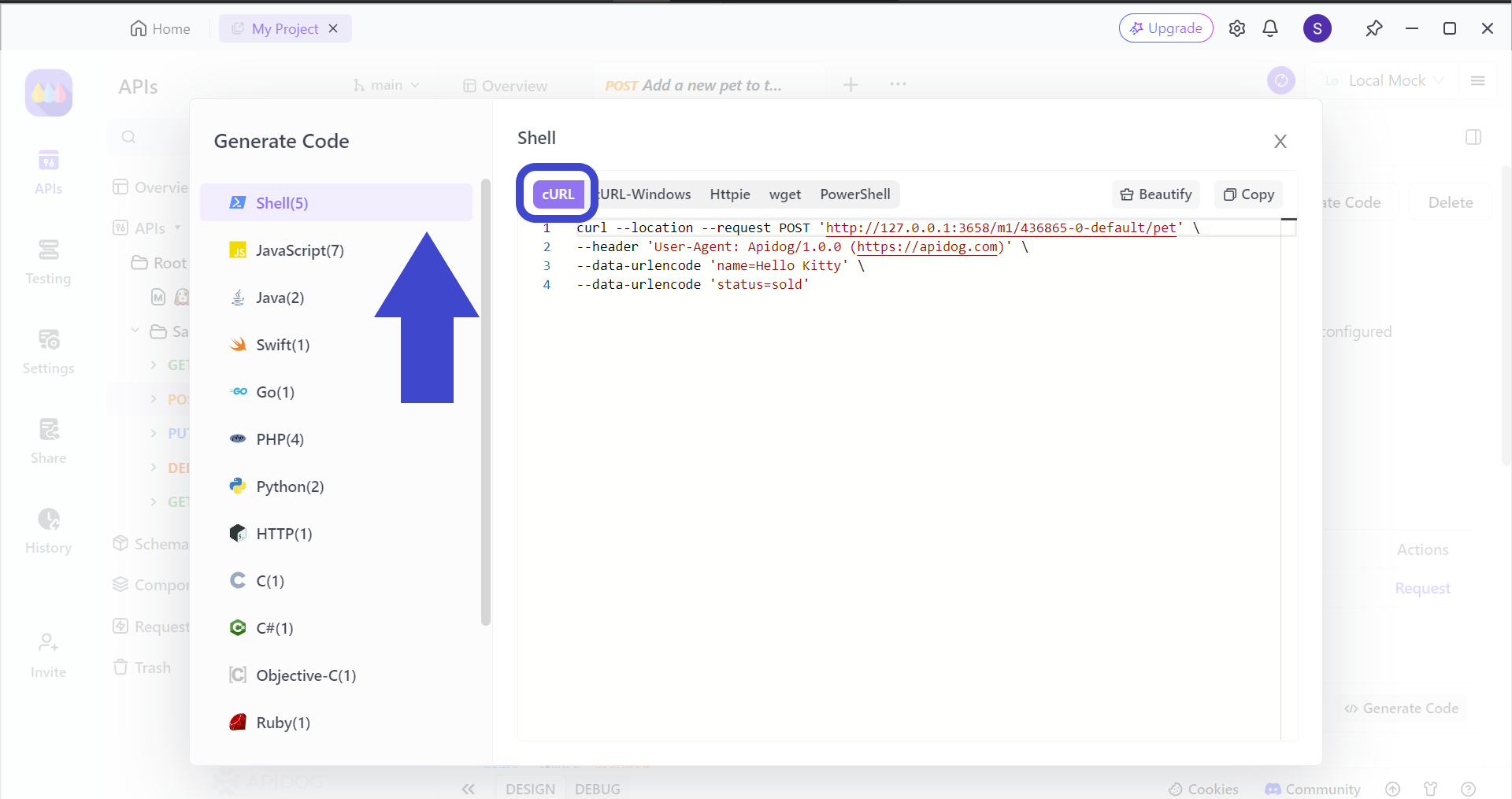
A pop-up window will prompt you to select which client code to generate. Here, select Shell
and cURL
. once you have, you can copy the code and paste it into your terminal. However, ensure that you modify it to fit your needs.
Conclusion
SOAP cURLs are two technologies that can work hand in hand, where cURL commands can be used for SOAP-based web service communication. However, do note that their use is declining due to more popular architectural styles like REST.
Apidog is an all-in-one API development platform that promotes a design-first development for APIs. With Apidog, you can easily modify and design APIs visually thanks to its simple and intuitive UI. Moreover, it is a solid choice for any developers who are interested in trying a new platform as Apidog supports various file type imports, with Postman, WSDL, and many more.