[Tutorial] Testing SOAP APIs using Axios
Axios can be used to debug SOAP-based web services with the help of various APLI platforms and API testing tools.
"SOAP Axios" might be a phrase that you may read or heard before when talking about web development. Nonetheless, the phrase comprises two important concepts that you should know.
When placed together, "SOAP Axios" is regarded as developers using Axios to debug a SOAP API.
SOAP - Simple Object Access Protocol
SOAP, also known as Simple Object Access Protocol, is a protocol that defines the rules for structuring messages in web development. It ensures the exchange of structured information in web services and systems in the same network.
With the SOAP protocol, it is possible for programs and applications running on different operating systems to communicate together. Check out popular SOAP APIs to understand more about what they do.
Significant Characteristics Defining SOAP
Standardized Protocol: SOAP is a standardized protocol maintained by the World Wide Web Consortium (W3C). This standardization ensures consistency and interoperability across different systems and platforms.
XML-Based Messaging: SOAP messages are formatted using XML (eXtensible Markup Language), allowing it to be easily read by humans and machines. = XML also provides a well-defined structure for requests and responses.
Strict Specification: SOAP has a strict and well-defined specification for message structure that includes headers and body. This can be an advantage in scenarios where high levels of consistency and formality are required.
Built-In Error Handling: SOAP has built-in error handling through standardized fault elements. making detailed error reporting easier.
Protocol Independence: SOAP messages can be carried over various transport protocols, including HTTP, SMTP, and more. This flexibility allows SOAP to be used in a wide range of network environments.
WSDL File Documentation: WSDL, an XML-based language, usually comes with a SOAP API to help describe it. It describes the functionalities, parameters, and other details provided by the web service, helping the web service users or clients to understand and interact with the service.
What is Axios?
Axios is a popular JavaScript library used to make HTTP requests from a web browser or Node.js environment. Axios was made to be easy, supporting the Promise API. This makes Axios a developer's choice for handling asynchronous operations when working with APIs.
Axios' Defining Features
Promise-based: Axios is built on top of Promises. Simplifying the handling of asynchronous code allows developers to focus on cleaner and more readable syntax, making it easier to manage responses and errors.
Browser and Node.js support: Axios is applicable in web browsers and Node.js environments, making it a versatile choice for developers who are working on full-stack applications.
Request and response interception: Axios allows developers to intercept and modify requests or responses before they are handled. This feature is particularly useful for tasks such as adding headers to requests or handling authentication tokens.
Automatic JSON data transformation: Axios automatically parses JSON responses, simplifying the process of working with JSON data from APIs.
Concurrency control: Axios provides features like canceling requests, which can be useful in scenarios where a user navigates away from a page or cancels an action, preventing unnecessary network requests.
Example of Simple Axios HTTP Get Request
If you are wondering what an Axios HTTP request may look like, here is a simple GET request.
import axios from 'axios';
axios.get('https://api.example.com/data')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
Advantages of Using Axios to Debug SOAP APIs
HTTP Request Inspection: Axios allows you to inspect the details of the HTTP request being sent to the SOAP API. The request's details include headers, request payload, and any other configuration options. Allowing developers to see these details is crucial for identifying issues in the request structure or headers.
Response Handling: SOAP API responses received after sending a request are very easy to read and understand due to the readable programming language. Axios also provides access to response data, headers, and status codes. This helps in understanding the server's response and identifying potential issues.
Error Handling: Axios allows you to handle errors that may occur during the HTTP request. This is valuable for debugging SOAP API calls, as it enables you to catch and log errors, providing insights into what went wrong during the communication.
Request and Response Interception: Axios supports request and response interception, allowing you to modify the request or response before it is sent or processed. This can be useful for adding custom headers, logging, or making adjustments during debugging.
Using Axios to Debug SOAP APIs
Apidog is an API platform that supports web developers with a myriad of functions, from designing, all the way to testing APIs.
Let's check out how you can use Axios with Apidog to debug a SOAP API. But before beginning this process, make sure to install Axios on Bash by running either line of code
npm install axios
# or
yarn add axios
Create a Node.js Script and Run it
If you have not created a Node.js script file, you can refer to the example below, named debug-soap-api.js
. Make sure to change the details according to what your SOAP API requires.
// Import Axios
const axios = require('axios');
// Define SOAP endpoint and request payload
const soapEndpoint = 'https://example.com/soap-endpoint';
const soapRequest = `
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/"...>
<!-- Your SOAP request here -->
</soapenv:Envelope>
`;
// Make a POST request using Axios
axios.post(soapEndpoint, soapRequest, {
headers: { 'Content-Type': 'text/xml' }
})
.then(response => {
// Handle the SOAP API response
console.log('SOAP Response:', response.data);
})
.catch(error => {
// Handle errors during the SOAP API request
console.error('SOAP Error:', error);
});
Once you have finished configuring your Node.js Script, run this line of code on Bash. Make sure to replace the name of debug-soap-api.js
according to the actual file name of your Node.js script.
node debug-soap-api.js
Inspect and Copy the Output
If the step above has been executed correctly, you should be able to see an output on your computer's console. This said output includes SOAP API responses. with any errors that may have occurred during the request.
If you believe that this output matches your expectations, then your SOAP API is functioning correctly.
Using Apidog as Axios Alternative for SOAP Testing
With using Apidog, you have the ability to test any web service request. In order to do so, take a look at the steps below.
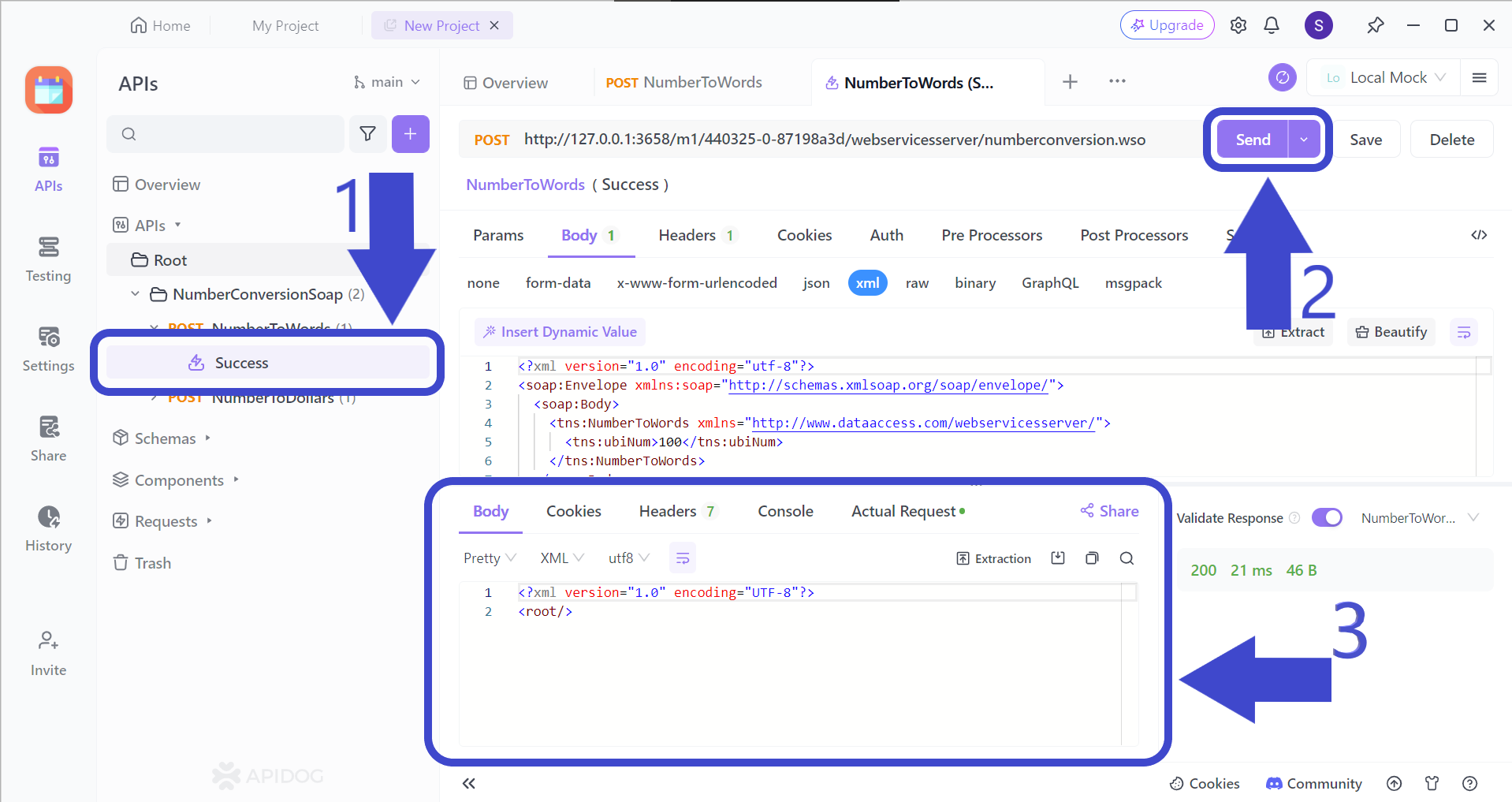
Arrow 1 - Select the specific request that needs to be selected. In this example, the selected request is called Success
.
Arrow 2 - Click the "Send" button once the request URL has been specified.
Arrow 3 - Observe the response received after the request has been sent.
Using Apidog to Generate Axios' Client Code
You can replicate the Axios HTTP Request for the SOAP API by using Apidog's code generation feature.
Follow the steps below to learn how to utilize it!
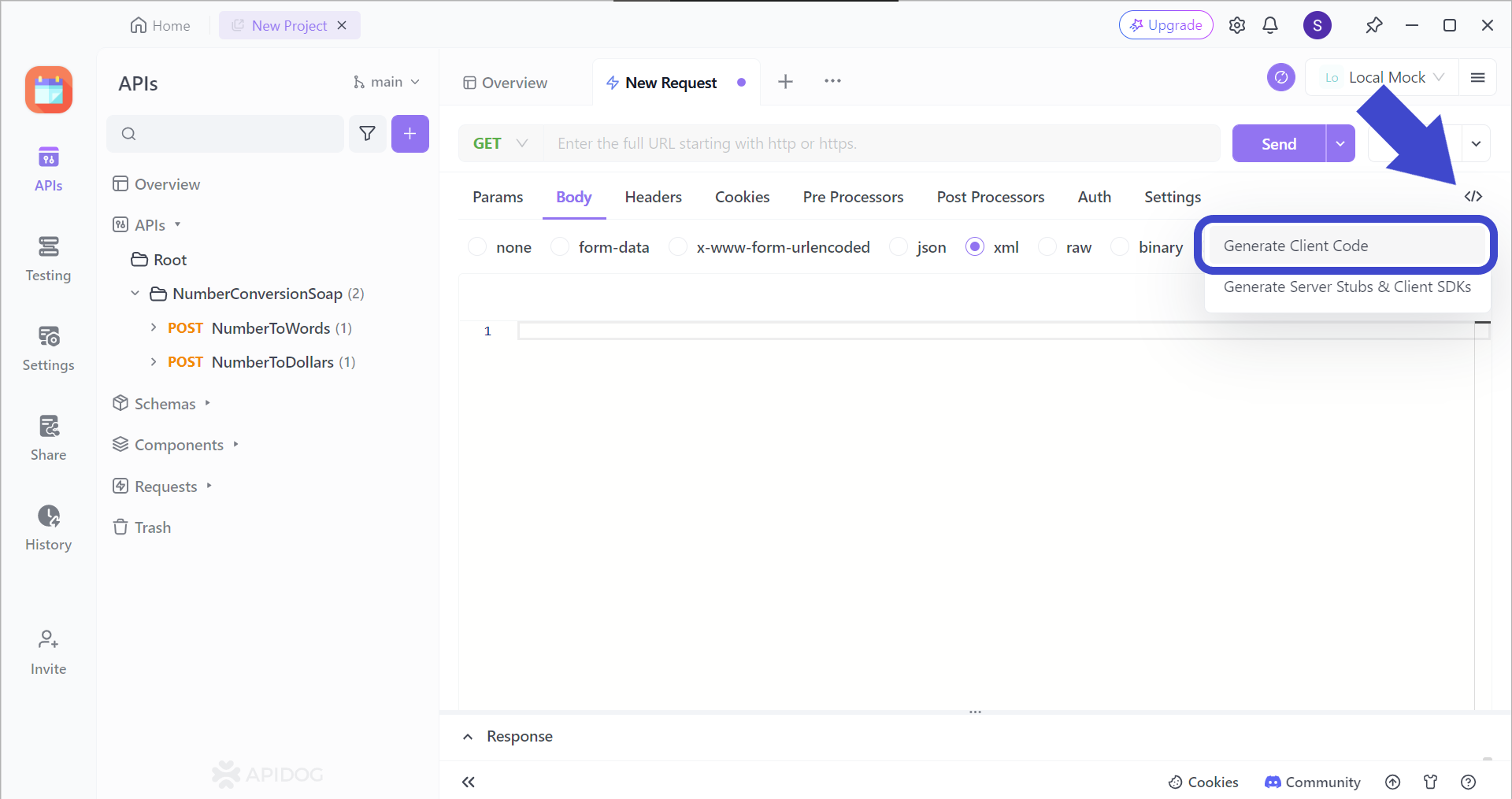
Firstly, start a new request and locate the </>
button that is found on the upper right-hand corner of the screen. If you have trouble finding it, refer to the picture, where it is pointed out by the arrow above.
Once you have located it, press it and select "Generate Client Code".
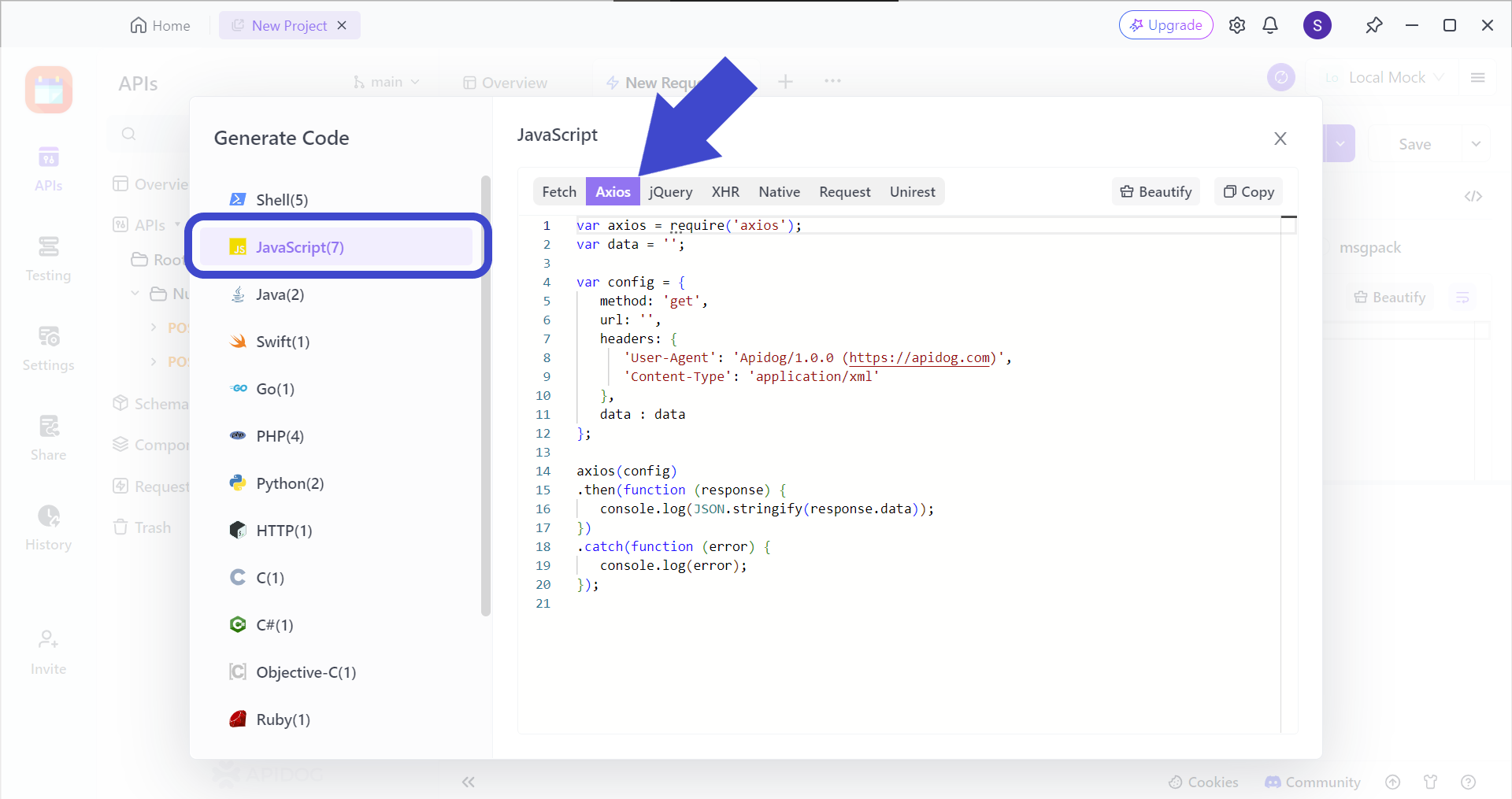
You should now have a pop-up window with the title "Generate Code". Here, you can select "JavaScript", and select "Axios" as shown in the image above.
Now, you can copy the code to your clipboard and paste it onto Axios as a skeleton structure to start creating the Axios HTTP request to debug your SOAP API!
Creating Documentation For Requests in Apidog
Apidog also allows users to seamlessly create API documentation for your SOAP API.
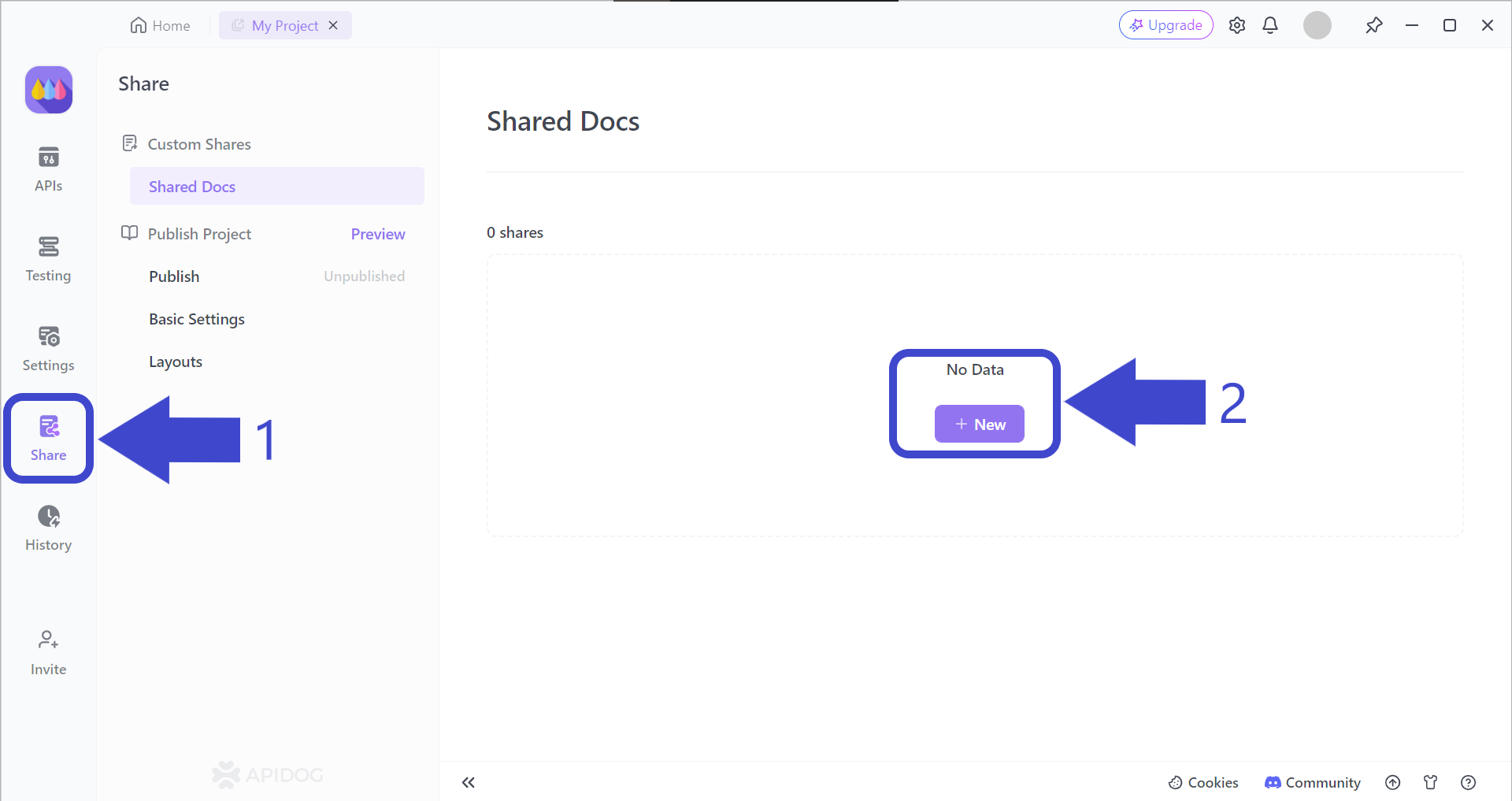
First, find the "Share" button as pointed out by Arrow 1, and press the "+ New" button as pointed out by Arrow 2
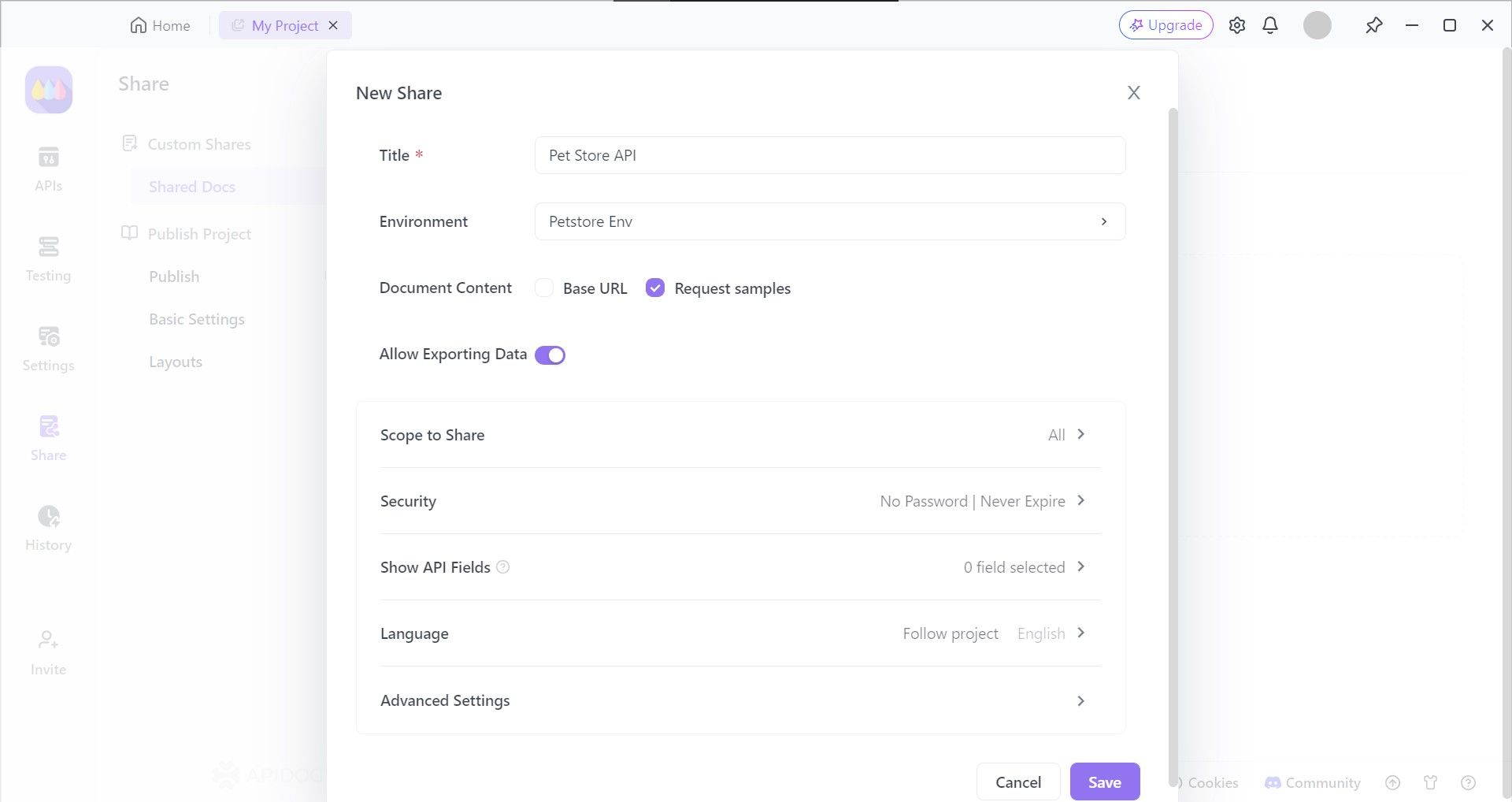
Apidog provides the option to choose who can view your API documentation as well as set a file password, so only chosen individuals or organizations can view it.
Once all required fields like API documentation name and language have been filled, hit Enter
on your keyboard or the Save
button.
View or Share Your REST API Documentation
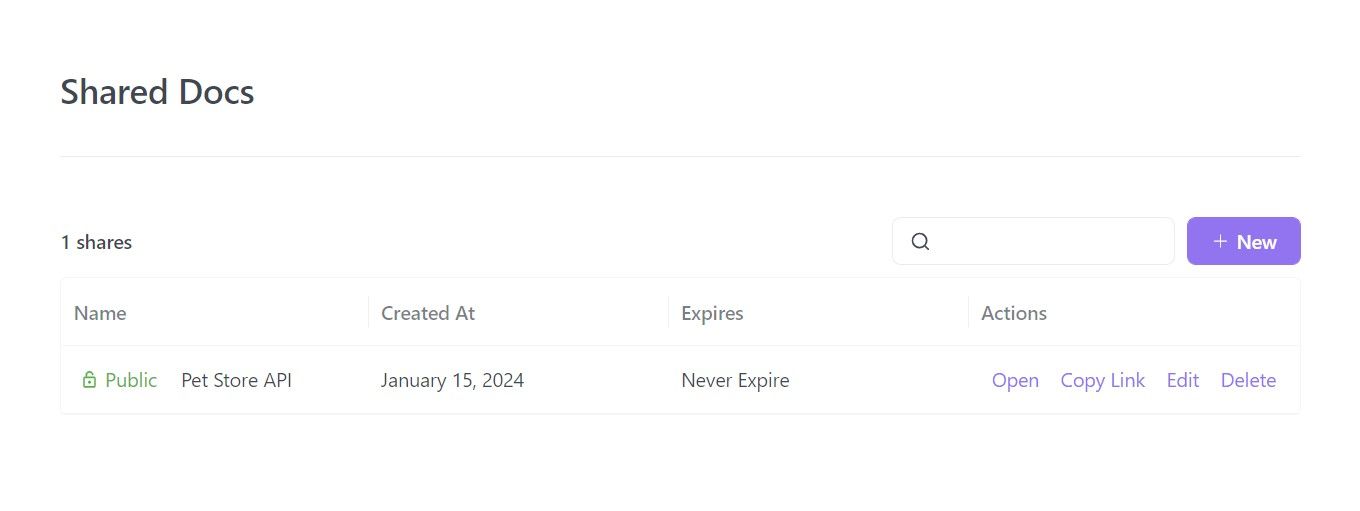
Apidog compiles your API project's details into an API documentation that is viewable through a website URL. All you have to do is click the "Copy Link" link under "Actions", and enter the URL on your favorite browser to view your API Documentation!
If you are interested, read this article on how to generate API documentation using Apidog.
Conclusion
While Axios provides these advantages for debugging, it's important to note that debugging SOAP APIs may also involve understanding the SOAP protocol, XML structure, and specific requirements of the SOAP service. Additionally, dedicated API testing tools such as Apidog may offer more specialized support for working with SOAP-specific features.