Building modern and sleek user interfaces is one of the main goals for front-end developers, and with the rise of component libraries, that task has become even easier. Today, I’m going to introduce you to Shadcn/UI, a powerful and customizable component library for React.js. Whether you’re new to React.js or a seasoned developer, Shadcn/UI can enhance your app’s design without the usual bloat of larger frameworks. Plus, I’ll walk you through the setup step-by-step, including how to leverage APIs and tools like Apidog to make development smoother.
By the end of this post, you’ll have a clear understanding of how to use Shadcn/UI in your React.js project. And, if you’re building out your own API or working with one, don’t forget to download Apidog for free—it’s a lifesaver when testing and developing APIs.
Let’s get started!
What is Shadcn/UI?
Before diving into the setup, let’s first define what Shadcn/UI is and why it’s a solid choice for your React.js project.
Shadcn/UI is a customizable component library built specifically for React.js. Unlike larger frameworks like Material UI or Bootstrap, Shadcn/UI focuses on giving you more control over the look and feel of your components. It provides you with basic building blocks, allowing you to create a unique interface without being tied to pre-defined themes.
Why Shadcn/UI?
- Lightweight: Unlike heavy libraries that bundle tons of components you’ll never use, Shadcn/UI provides only what you need.
- Customizable: You can style and structure the components according to your project’s unique requirements.
- Optimized for React.js: Seamless integration with React.js means you can focus on writing code rather than tweaking configurations.
- APIs Ready: The library is compatible with API tools like Apidog, making it easier to manage and test API endpoints within your React app.
Step-by-Step Guide: How to Use Shadcn/UI in Your React.js Project
Now that you know what Shadcn/UI is, let’s go through the step-by-step process of integrating it into a React.js project. This guide assumes you already have a basic understanding of React and that you have Node.js installed on your machine.
1. Create a New React.js Project
If you already have a React.js project set up, you can skip this step. Otherwise, you can create a new project using the following commands:
npx create-react-app my-shadcn-ui-app
cd my-shadcn-ui-app
npm start
This will create a new React.js project named my-shadcn-ui-app
and start the development server. Now, you should see the default React app up and running.
2. Install Shadcn/UI
Add dependencies to your project manually.
Add Tailwind CSS
Components are styled using Tailwind CSS. You need to install Tailwind CSS in your project.
Follow the Tailwind CSS installation instructions to get started.
Add dependencies
Add the following dependencies to your project:
npm install tailwindcss-animate class-variance-authority clsx tailwind-merge
Add icon library
If you're using the default
style, install lucide-react
:
npm install lucide-react
If you're using the new-york
style, install @radix-ui/react-icons
:
npm install @radix-ui/react-icons
Configure path aliases
I use the @
alias. This is how I configure it in tsconfig.json:tsconfig.json
{
"compilerOptions": {
"baseUrl": ".",
"paths": {
"@/*": ["./*"]
}
}
}
The @
alias is a preference. You can use other aliases if you want.
If you use a different alias such as ~, you'll need to update import statements when adding components.
You can now start adding components to your project.
3. Import and Use Shadcn/UI Components
Let’s jump into the fun part—adding some Shadcn/UI components to your React.js app. In your src/App.js
file, you can start by importing and using one of Shadcn/UI’s components, such as the button component.
Here’s an example:
import React from 'react';
import { Button } from 'shadcn-ui';
function App() {
return (
<div className="App">
<header className="App-header">
<h1>Welcome to My Shadcn/UI App</h1>
<Button variant="primary">Click Me!</Button>
</header>
</div>
);
}
export default App;
The Button
component from Shadcn/UI is imported and used in the App
component. You can use various props to customize it. In this case, we’re using the variant="primary"
prop to give the button a primary styling.
4. Customize the Shadcn/UI Theme
One of the best parts of Shadcn/UI is its customizability. You can tweak the components to match your app’s unique design language.
Shadcn/UI allows you to define a custom theme that can be applied globally. Here’s how to do that:
a) Create a Custom Theme File
First, create a new file called theme.js
in your src
directory. In this file, you’ll define your custom theme as follows:
const theme = {
colors: {
primary: '#ff6347', // Tomato color
secondary: '#4caf50', // Green color
},
fonts: {
body: 'Arial, sans-serif',
heading: 'Georgia, serif',
},
};
export default theme;
b) Apply the Theme to Your Components
Now that you have your custom theme defined, you can apply it to your components using the ThemeProvider component. Update your src/App.js
file as follows:
import React from 'react';
import { Button, ThemeProvider } from 'shadcn-ui';
import theme from './theme';
function App() {
return (
<ThemeProvider theme={theme}>
<div className="App">
<header className="App-header">
<h1>Welcome to My Shadcn/UI App</h1>
<Button variant="primary">Click Me!</Button>
</header>
</div>
</ThemeProvider>
);
}
export default App;
In this updated code, we’ve imported ThemeProvider
from Shadcn/UI and wrapped our app inside it, passing the custom theme
as a prop.
5. Managing APIs with Apidog in Your Shadcn/UI + React Project
Now that your front-end is looking great with Shadcn/UI, it’s time to make it functional by connecting it to an API. This is where Apidog comes in handy. If you haven’t heard of Apidog before, it’s a robust API management tool that simplifies API testing, documentation, and collaboration between developers.
Why Use Apidog with Your React.js App?
- API testing made easy: You can test your API endpoints right inside your React project.
- Seamless collaboration: Apidog lets you generate and share API documentation with your team.
- Speed up development: Easily mock API responses to speed up the development process.
Using Apidog for API Calls
Let’s say you’re building a React.js app that fetches data from a weather API. Here’s how you could use Apidog to manage the API calls:
Step 1: Open Apidog and create a new request.
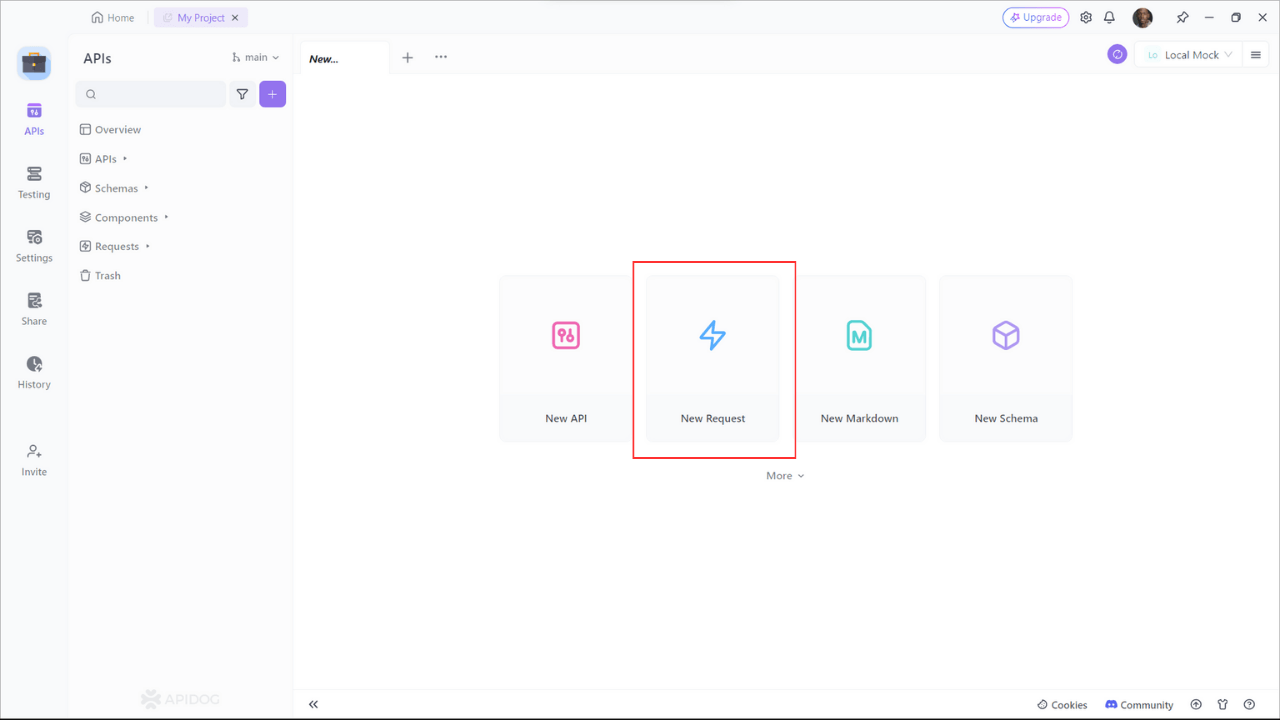
Step 2: In the test editor, enter the URL of your API endpoint, select the HTTP method, and add any headers, parameters, or body data that you need. For example, you can test the route that returns a simple message that we created earlier:
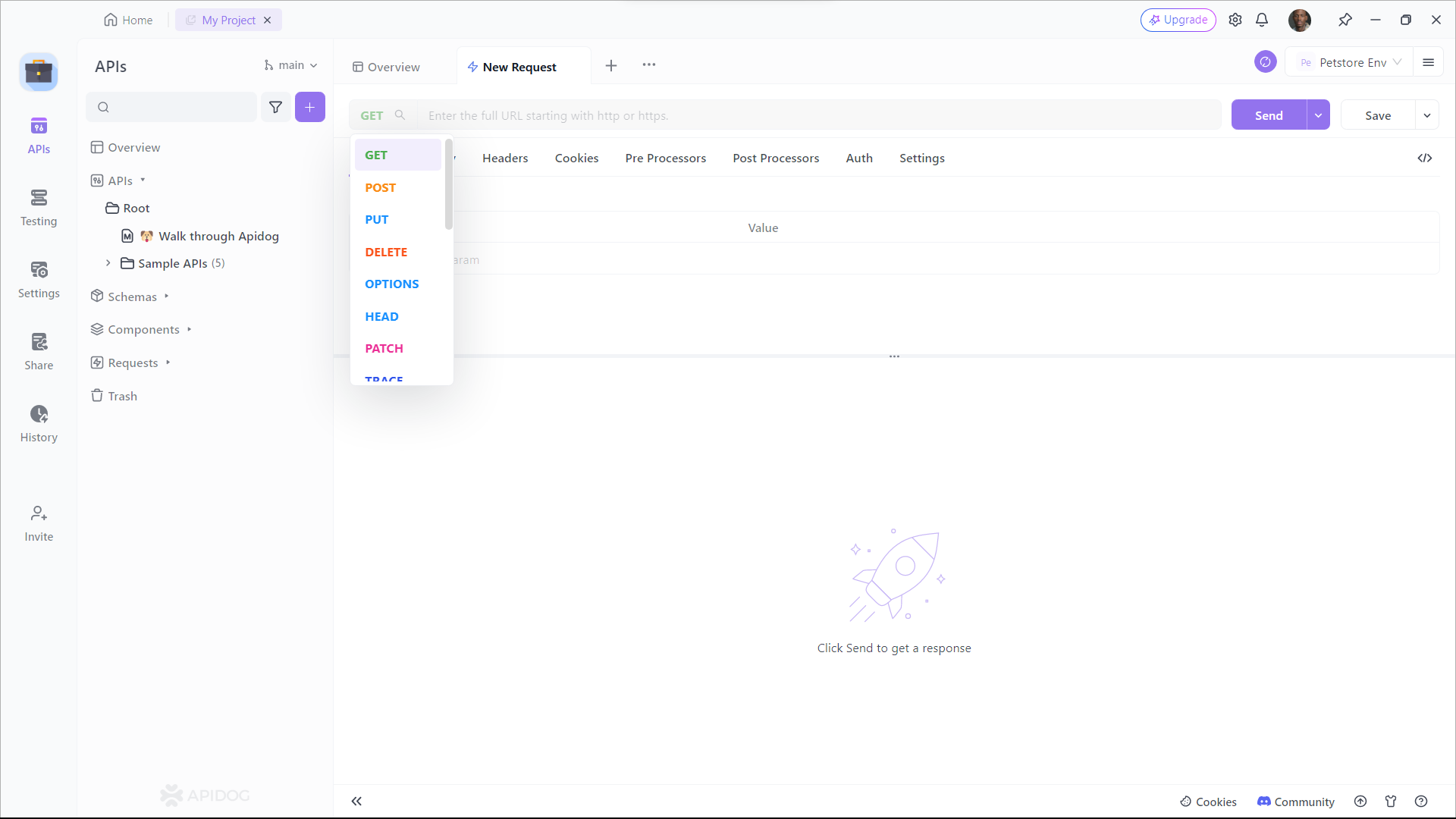
Step 3: Click on the Send button and see the result of your test. You should see the status code, the response time, and the response body of your API. For example, you should see something like this:
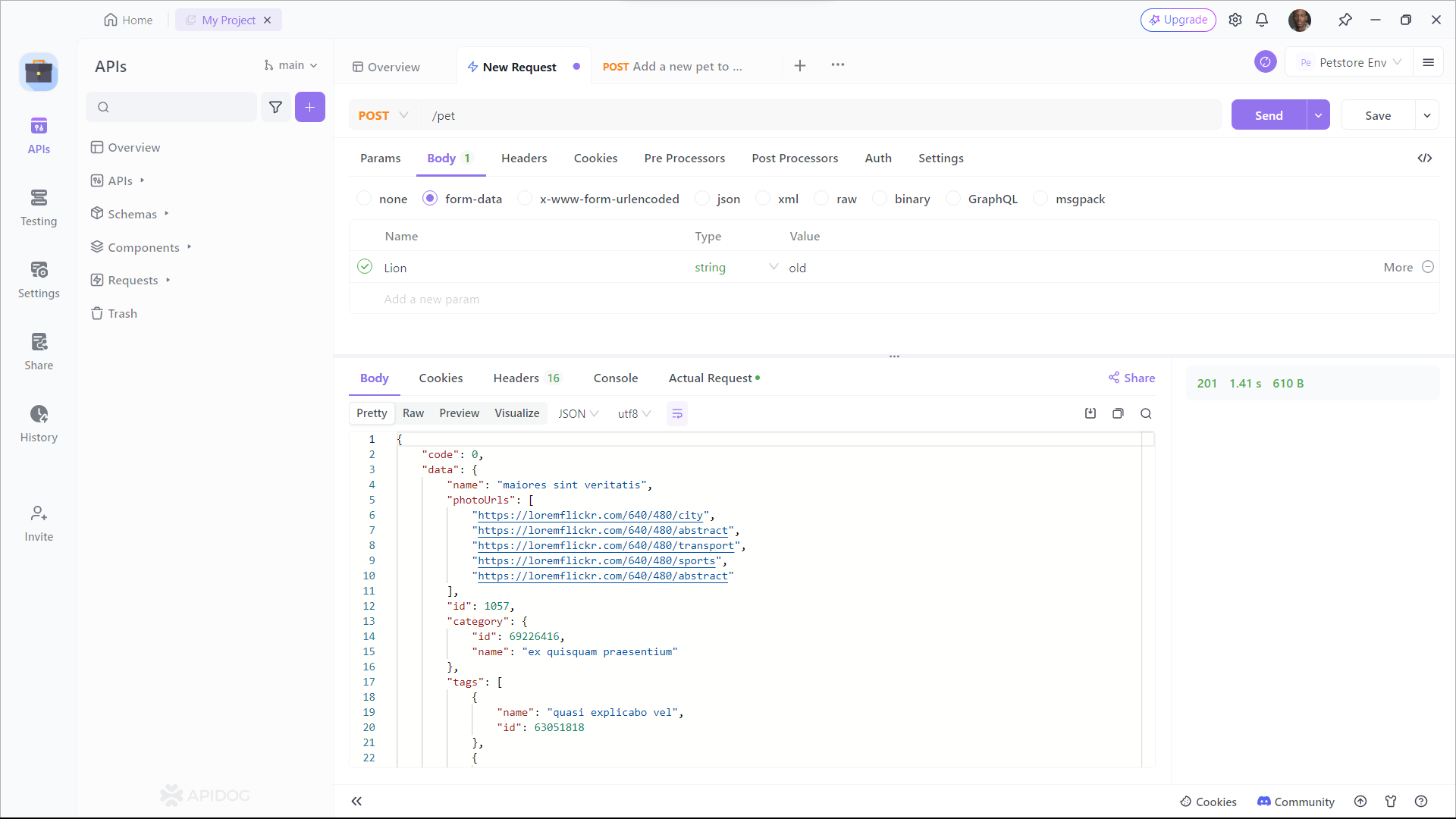
Apidog is a great tool for testing your APIs, as it helps you ensure the quality, reliability, and performance of your web services. It also saves you time and effort, as you don’t need to write any code or install any software to test your APIs. You can just use your browser and enjoy the user-friendly interface and features of Apidog.
6. Best Practices for Using Shadcn/UI and Apidog in React.js
Here are some best practices you should follow to get the most out of Shadcn/UI and Apidog:
- Optimize performance: Use only the Shadcn/UI components you need to keep your bundle size small.
- Modularize your components: Break down your UI into small, reusable components.
- Test your APIs: Use Apidog to thoroughly test your API endpoints and ensure they are working as expected.
- Use version control: Regularly commit your changes to avoid losing progress and to collaborate effectively with your team.
Conclusion: Building React.js Apps with Shadcn/UI and Apidog
Congratulations! You now have all the knowledge you need to use Shadcn/UI in your React.js projects. From setting up the library to customizing the components, you’ve seen how easy it is to create beautiful UIs. And with Apidog, managing your API calls becomes a breeze.
Remember, whether you’re building an internal tool or a customer-facing application, Shadcn/UI gives you the flexibility to create something unique, while Apidog helps streamline your API workflow.
P.S. If you're working on APIs, don't forget to download Apidog for free—it will save you hours of development and testing time!