Regular expressions, often abbreviated as regex or regexp, are powerful tools for working with text patterns in Ruby. They allow you to search, match, extract, and manipulate strings based on specific patterns. Whether you're validating user input, parsing data, or performing complex text processing, Ruby regular expressions provide a concise and flexible solution.
This tutorial will guide you through the fundamentals of regular expressions in Ruby, from basic syntax to advanced techniques.
For Ruby developers looking to streamline their API testing workflow should consider Apidog, a powerful alternative that outshines Postman in several key aspects.
Apidog combines API documentation, debugging, automated testing, and mocking in one cohesive platform, offering a more integrated experience than Postman's fragmented approach.
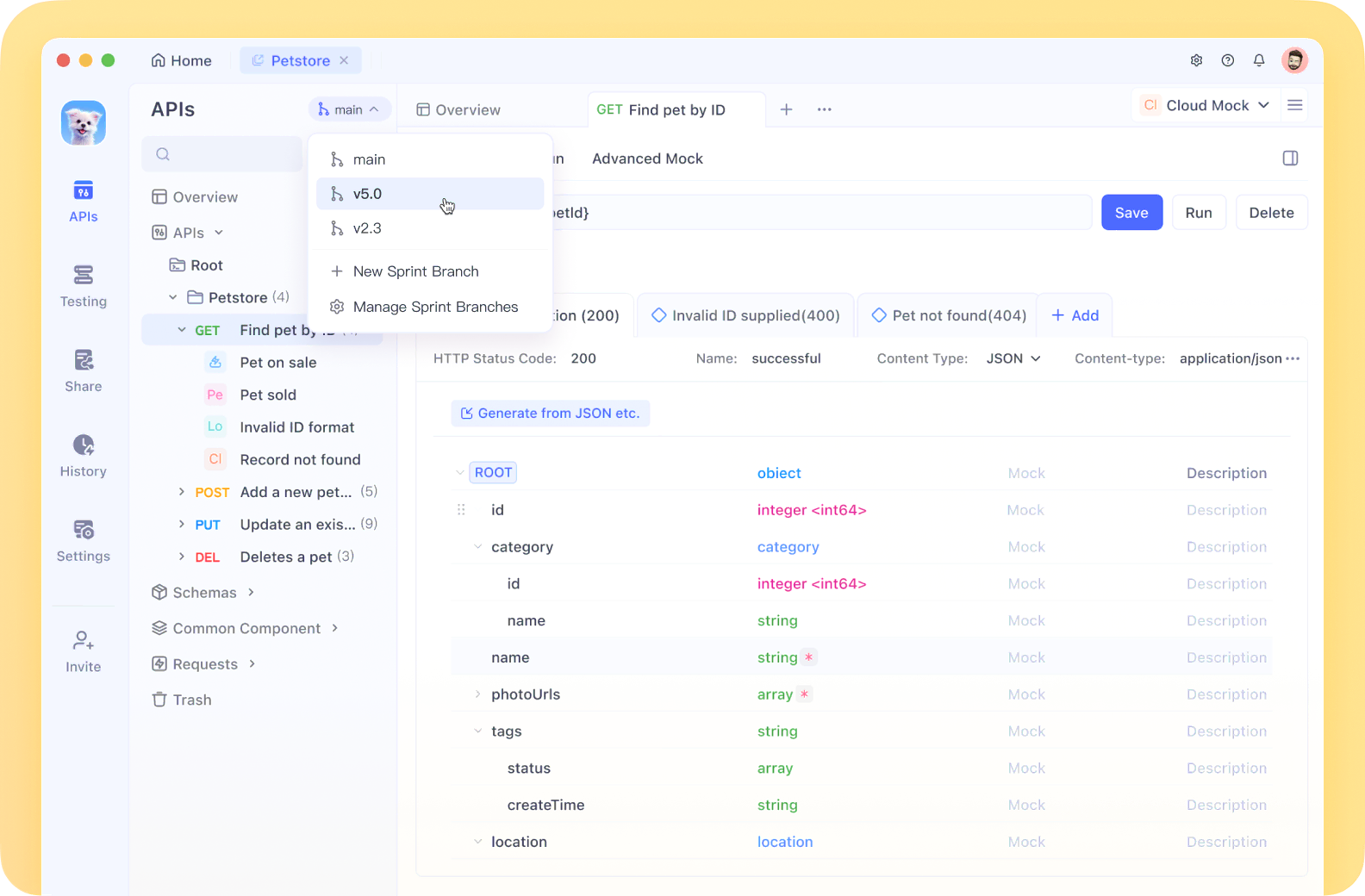
With its intuitive interface, built-in schema validation, and comprehensive team collaboration features, Apidog makes managing complex API projects significantly easier.
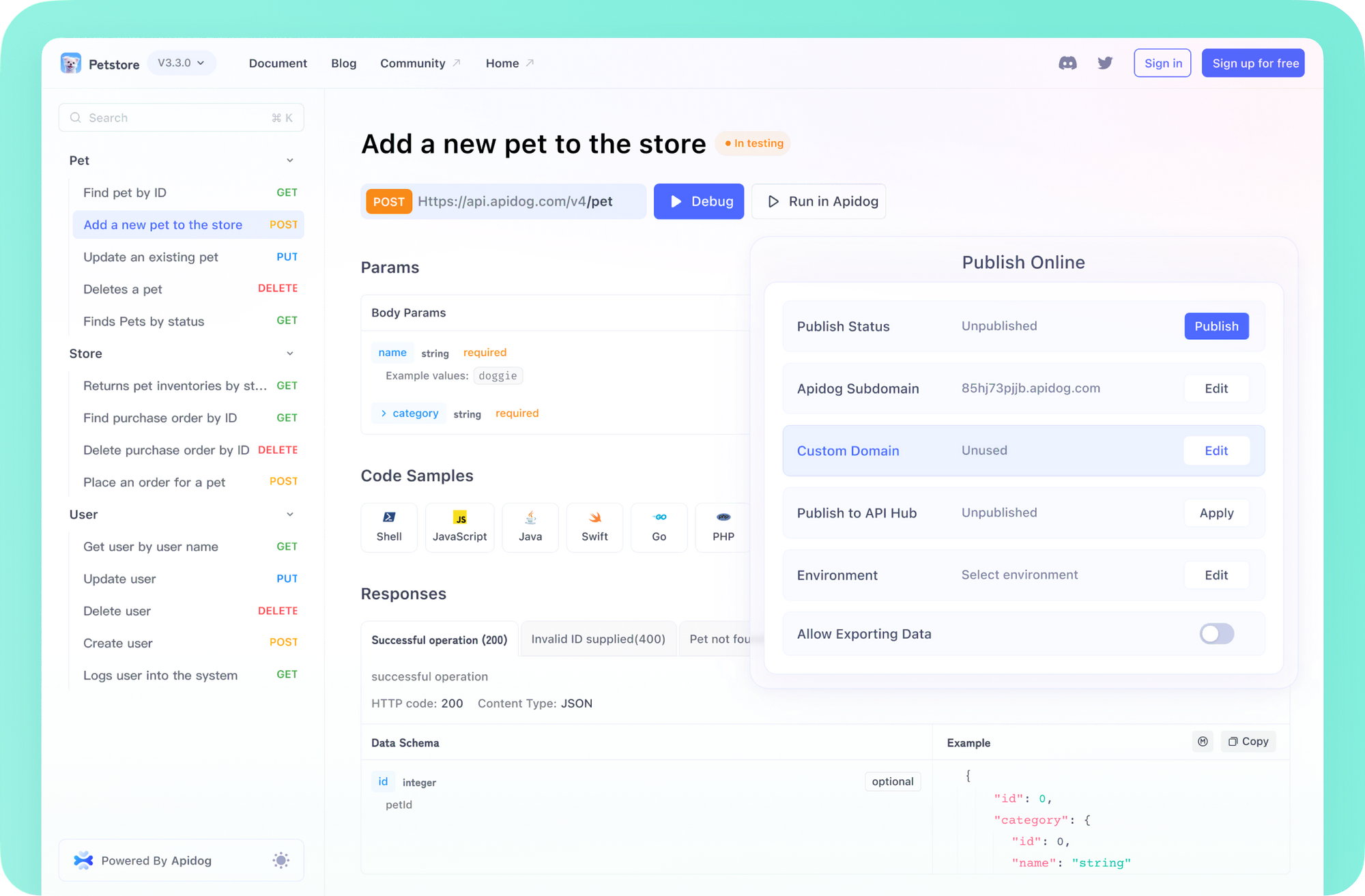
Ruby developers will appreciate Apidog's robust support for automation through its command-line interface, seamless Git integration for version control, and superior OpenAPI/Swagger compatibility—essential for maintaining accurate documentation alongside your Ruby applications.
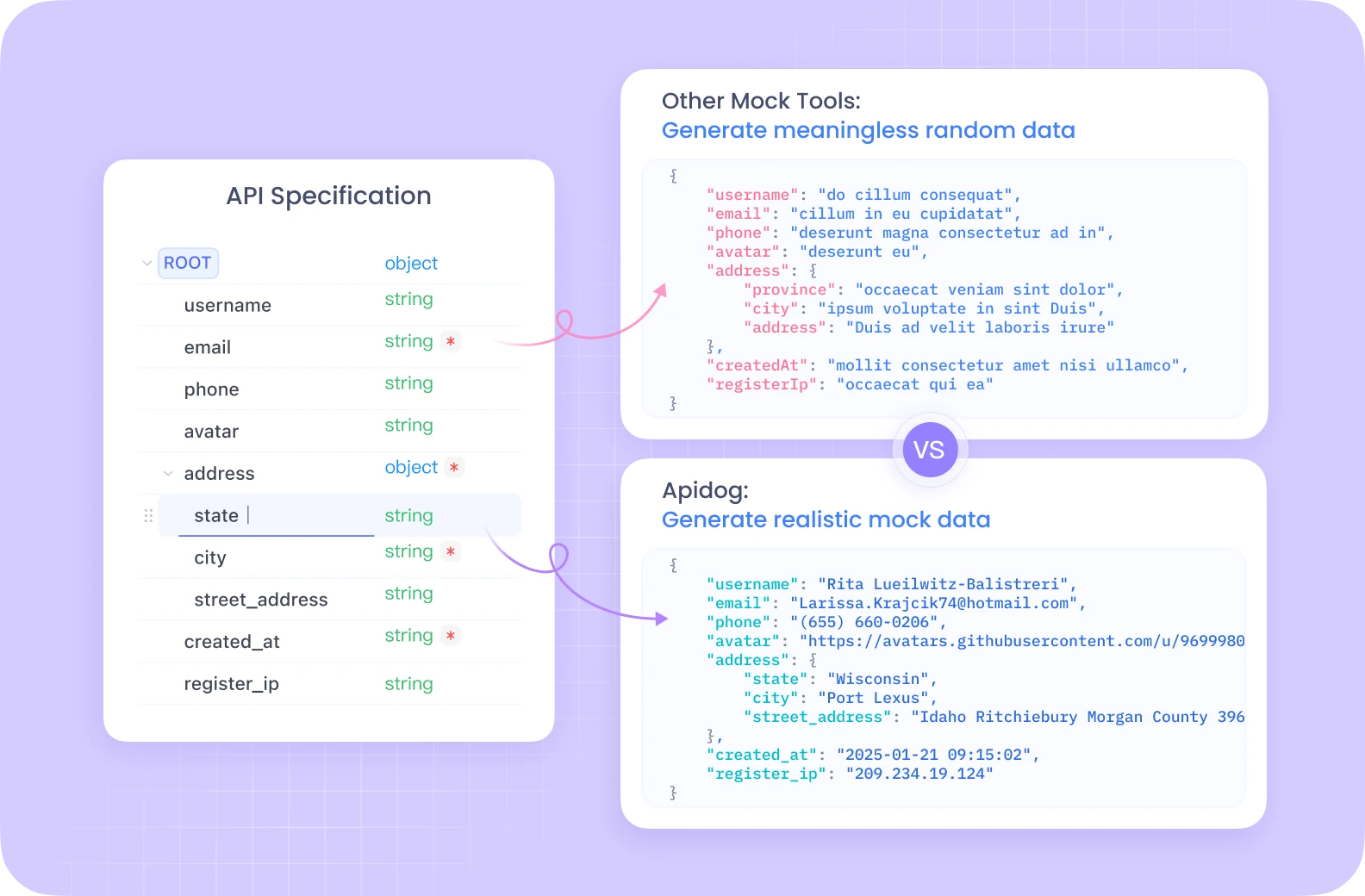
Whether you're building RESTful services with Rails or creating microservices with Sinatra, Apidog's all-in-one toolkit eliminates the context-switching that plagues Postman users, allowing you to focus on what matters most: writing great Ruby code.
Creating Ruby Regular Expressions
In Ruby, there are several ways to create regular expressions:
Method 1: Using Slashes in Ruby Regular Expressions
The most common way to create a regular expression in Ruby is by enclosing the pattern between forward slashes:
/Ruby/ # Creates a regular expression that matches "Ruby"
Method 2: Using Percent Notation for Ruby Regular Expressions
You can also use the percent notation with the %r
indicator:
%r(Ruby) # Same as /Ruby/
This is particularly useful when your pattern contains many forward slashes, as it helps avoid excessive escaping.
Method 3: Using the Regexp Class for Ruby Regular Expressions
Another approach is to use the Regexp.new
constructor:
Regexp.new("Ruby") # Same as /Ruby/
All these methods create instances of the Regexp
class.
Basic Ruby Regular Expressions Symbols
Ruby regular expressions use special symbols to represent different patterns. Here are the fundamental ones:
Symbol | Description | Example | Matches |
---|---|---|---|
. |
Matches any single character | /a.b/ |
"axb", "a2b", "a b" |
* |
Matches 0 or more of the preceding element | /ab*c/ |
"ac", "abc", "abbc" |
+ |
Matches 1 or more of the preceding element | /ab+c/ |
"abc", "abbc", "abbbc" |
? |
Matches 0 or 1 of the preceding element | /ab?c/ |
"ac", "abc" |
\| |
Alternation (OR) | /cat\|dog/ |
"cat", "dog" |
[] |
Character class - matches any one character listed | /[aeiou]/ |
"a", "e", "i", "o", "u" |
() |
Creates a capture group | /(abc)+/ |
"abc", "abcabc" |
^ |
Matches the start of a string | /^start/ |
"start of string" |
$ |
Matches the end of a string | /end$/ |
"the end" |
\\\\ |
Escapes a special character | /\\\\\\\\/ |
"\" |
Special Characters in Ruby Regular Expressions
Ruby regular expressions include predefined character classes that make pattern matching more convenient:
Symbol | Description | Example | Matches |
---|---|---|---|
\\\\d |
Matches any digit (0-9) | /\\\\d+/ |
"123", "9" |
\\\\D |
Matches any non-digit | /\\\\D+/ |
"abc", "!@#" |
\\\\w |
Matches any word character (alphanumeric + underscore) | /\\\\w+/ |
"ruby123", "user_name" |
\\\\W |
Matches any non-word character | /\\\\W+/ |
"!@#", " " |
\\\\s |
Matches any whitespace character | /\\\\s+/ |
" ", "\t", "\n" |
\\\\S |
Matches any non-whitespace character | /\\\\S+/ |
"hello", "123" |
\\\\A |
Matches the beginning of a string | /\\\\AHello/ |
"Hello world" |
\\\\z |
Matches the end of a string | /world\\\\z/ |
"Hello world" |
\\\\Z |
Matches the end of a string or before final newline | /world\\\\Z/ |
"Hello world\n" |
Quantifiers in Ruby Regular Expressions
Quantifiers allow you to specify how many times a pattern should match:
Quantifier | Description | Example | Matches |
---|---|---|---|
* |
0 or more repetitions | /ab*c/ |
"ac", "abc", "abbc" |
+ |
1 or more repetitions | /ab+c/ |
"abc", "abbc" |
? |
0 or 1 repetition | /colou?r/ |
"color", "colour" |
{n} |
Exactly n repetitions | /a{3}/ |
"aaa" |
{n,} |
At least n repetitions | /a{2,}/ |
"aa", "aaa", "aaaa" |
{n,m} |
Between n and m repetitions | /a{2,4}/ |
"aa", "aaa", "aaaa" |
For example, to match a phone number format like "123-456-7890", you could use:
/\\\\d{3}-\\\\d{3}-\\\\d{4}/
Pattern Matching with Ruby Regular Expressions
Using the Match Operator in Ruby Regular Expressions
The most basic way to check if a pattern matches a string is using the =~
operator:
text = "The quick brown fox"
if /quick/ =~ text
puts "Match found at index #{$~.begin(0)}"
else
puts "No match found"
end
The =~
operator returns the index of the first match or nil
if no match is found. After a successful match, you can use Regexp.last_match
or the global variable $~
to access information about the match.
Capturing Groups in Ruby Regular Expressions
You can use parentheses to create capture groups and extract specific parts of a match:
text = "Name: John, Age: 30"
if match_data = /Name: (\\\\w+), Age: (\\\\d+)/.match(text)
name = match_data[1] # First capture group
age = match_data[2] # Second capture group
puts "Name: #{name}, Age: #{age}"
end
Extracting Matched Content in Ruby Regular Expressions
To extract all occurrences of a pattern, use the scan
method:
text = "Contact us at support@example.com or info@example.org"
emails = text.scan(/\\\\w+@\\\\w+\\\\.\\\\w+/)
puts emails.inspect # ["support@example.com", "info@example.org"]
Working with Ruby Regular Expressions in Practice
String Replacement with Ruby Regular Expressions
The gsub
method allows you to replace all occurrences of a pattern with a specified string:
text = "apple banana apple"
new_text = text.gsub(/apple/, "orange")
puts new_text # "orange banana orange"
You can also use a block for more complex replacements:
text = "The price is $10"
new_text = text.gsub(/\\\\$(\\\\d+)/) do |match|
"$#{$1.to_i * 1.1}" # Increase price by 10%
end
puts new_text # "The price is $11"
Alternation in Ruby Regular Expressions
The pipe symbol (|
) allows you to match one pattern or another:
/cat|dog/.match("I have a cat") # Matches "cat"
/cat|dog/.match("I have a dog") # Matches "dog"
You can use parentheses to group alternatives:
/(apple|banana) pie/.match("I love apple pie") # Matches "apple pie"
Character Classes in Ruby Regular Expressions
Character classes let you match any single character from a set:
/[aeiou]/.match("hello") # Matches "e"
/[0-9]/.match("agent007") # Matches "0"
You can also negate a character class:
/[^0-9]/.match("agent007") # Matches "a"
String Operations with Ruby Regular Expressions
Starting and Ending Matches in Ruby Regular Expressions
Ruby provides convenient methods to check if a string starts or ends with a specific pattern:
"Hello, World!".start_with?("Hello") # true
"Hello, World!".end_with?("World!") # true
While these methods are not regex-based, they're often used alongside regular expressions for string manipulation tasks.
Global Variables in Ruby Regular Expressions
After a successful match, Ruby sets several global variables:
$&
- The complete matched text- `$`` - The portion of string before the match
$'
- The portion of string after the match
/bb/ =~ "aabbcc"
puts $` # "aa"
puts $& # "bb"
puts $' # "cc"
Modifiers for Ruby Regular Expressions
Ruby regular expressions support various modifiers that change how patterns are interpreted:
Modifier | Description | Example |
---|---|---|
i |
Case-insensitive matching | /ruby/i matches "Ruby", "RUBY", "rUbY" |
m |
Multi-line mode (dot matches newline) | /./m matches any character including newline |
x |
Extended mode (allows comments and whitespace) | /pattern # comment/x |
o |
Perform #{} interpolations only once | /#{pattern}/o |
u |
UTF-8 encoding | /\\\\u{1F600}/u matches emoji 😀 |
Examples:
/ruby/ =~ "RUBY" # nil (no match)
/ruby/i =~ "RUBY" # 0 (match at the beginning)
# Without 'm' modifier, dot doesn't match newlines
/a.b/ =~ "a\\\\nb" # nil
# With 'm' modifier, dot matches newlines too
/a.b/m =~ "a\\\\nb" # 0
Advanced Ruby Regular Expressions Techniques
Non-Capturing Groups in Ruby Regular Expressions
Sometimes you need grouping without capturing the matched text:
/(?:ab)+c/.match("ababc") # Matches "ababc" without capturing "ab"
Lookahead and Lookbehind in Ruby Regular Expressions
Lookahead assertions let you match a pattern only if it's followed by another pattern:
# Match 'apple' only if followed by 'pie'
/apple(?= pie)/.match("apple pie") # Matches "apple"
/apple(?= pie)/.match("apple tart") # No match
# Match 'apple' only if NOT followed by 'pie'
/apple(?! pie)/.match("apple tart") # Matches "apple"
Lookbehind assertions work similarly but check what comes before:
# Match 'pie' only if preceded by 'apple'
/(?<=apple )pie/.match("apple pie") # Matches "pie"
/(?<=apple )pie/.match("cherry pie") # No match
Performance Tips for Ruby Regular Expressions
- Be specific: More specific patterns perform better than general ones
- Avoid excessive backtracking: Complex patterns with many quantifiers can lead to performance issues
- Use anchors: When appropriate, use
\\\\A
,\\\\z
,^
and$
to limit where matches can occur - Consider alternatives: Sometimes simple string methods like
include?
orstart_with?
are faster than regex for simple cases
Conclusion on Ruby Regular Expressions
Ruby regular expressions are a powerful tool in your programming toolkit. They allow you to perform complex text processing with concise syntax. While the syntax might seem intimidating at first, mastering regular expressions will significantly boost your productivity when working with text in Ruby.
Practice is key to becoming proficient with regular expressions. Start with simple patterns and gradually incorporate more complex features as you become comfortable with the basics. Many online tools like Rubular (https://rubular.com/) can help you test and debug your regular expressions interactively.
By understanding the concepts covered in this tutorial, you'll be well-equipped to tackle a wide range of string manipulation challenges in your Ruby projects.