In modern software development, applications rarely exist in isolation. They communicate, exchange data, and trigger actions in one another, forming vast, interconnected ecosystems. This communication is orchestrated by Application Programming Interfaces (APIs), which define the rules and protocols for how different software components interact. Over the decades, several architectural styles and protocols have emerged to facilitate this inter-service communication. Among the most prominent are Remote Procedure Calls (RPC), Representational State Transfer (REST), and GraphQL.
Understanding these three paradigms is crucial for any developer or architect designing distributed systems. Each comes with its own philosophy, strengths, weaknesses, and ideal use cases. This article aims to clearly explain RPC, REST, and GraphQL, delving into their core concepts, operational mechanics, benefits, drawbacks, and the scenarios where each shines.
Want an integrated, All-in-One platform for your Developer Team to work together with maximum productivity?
Apidog delivers all your demans, and replaces Postman at a much more affordable price!
The Foundation: Client-Server Communication
Before diving into the specifics, it's essential to grasp the fundamental model they all serve: client-server communication. In this model, a client (e.g., a web browser, a mobile app, another server) requires some data or wants to perform an action. Concurrently, a server (a remote machine or process) hosts the data or the logic to perform the action. The client sends a request to the server, and the server sends back a response. The mechanisms we're about to discuss – RPC, REST, and GraphQL – are different ways to structure these requests and responses.
RPC: Invoking Functions Across Networks
What is RPC?
Remote Procedure Call represents one of the earliest and most direct paradigms for inter-process communication. The foundational idea is to make a request to a remote server appear and operate much like a local function or procedure call. The client application invokes what seems to be a local function (the "procedure"), but this function's execution actually occurs on a remote server. The complexities of network communication are carefully abstracted away, lending distributed programming an air of simplicity akin to traditional, single-machine programming.
How RPC Works:
The RPC process unfolds through a sequence of coordinated steps, designed to make remote execution transparent. Initially, the client possesses a "stub" or "proxy" for the remote procedure. This stub mirrors the signature of the actual remote procedure. When the client application calls this stub, the logic isn't executed locally. Instead, the client stub takes the parameters passed to the function and "marshals" or "serializes" them. This critical step converts the parameters from their in-memory representation into a format suitable for network transmission, such as binary, XML, or JSON.
Following marshalling, these serialized parameters, accompanied by an identifier for the specific procedure to be invoked, are sent across the network to the server. On the server-side, a "skeleton" or server-side stub awaits and receives the incoming request. This server skeleton then undertakes the task of "unmarshalling" or "deserializing" the received data, transforming it back into the parameters that the actual server procedure expects.
With the parameters successfully reconstructed, the server skeleton calls the designated procedure on the server, passing it the unmarshalled parameters. Once the procedure completes its execution, its return value, along with any exceptions encountered, are marshalled by the server skeleton. This serialized response is then transmitted back across the network to the client stub. Upon receiving the response, the client stub unmarshals it, converting the data back into a return value that the client application can readily understand. Finally, the client stub returns this value to the original calling code, thereby completing the illusion that a local function call was performed.
Key Characteristics of RPC:
RPC APIs are typically action-oriented. They are designed around verbs or commands, such as addUser(userDetails)
or calculatePrice(itemId, quantity)
. The primary focus is on "what actions you can perform."
Traditionally, clients and servers in RPC systems exhibit tight coupling. The client often needs explicit knowledge of the specific function names and the precise parameter signatures available on the server. Consequently, modifications on the server-side frequently necessitate corresponding changes on the client-side.
RPC frameworks commonly provide tools for generating client stubs and server skeletons in various programming languages from a shared Interface Definition Language (IDL). Examples of IDLs include CORBA IDL, Protocol Buffers (.proto files), or Apache Thrift IDL. This code generation capability facilitates interoperability.
Regarding efficiency, many RPC protocols, particularly those employing binary formats, are engineered for optimal performance in terms of data size and processing speed.
Evolution: gRPC
While earlier RPC implementations like XML-RPC or Java RMI had certain limitations, the RPC paradigm has experienced a significant resurgence with the advent of modern frameworks such as gRPC (Google RPC). gRPC introduces substantial enhancements. It predominantly uses Protocol Buffers as its IDL and for message serialization. Protocol Buffers offer a language-agnostic, platform-neutral, extensible mechanism for serializing structured data, often described as a more compact, faster, and simpler alternative to XML.
Furthermore, gRPC operates over HTTP/2, which enables advanced features like multiplexing (allowing multiple requests and responses over a single connection), server push capabilities, and header compression. These features collectively contribute to improved performance and reduced latency.
A notable strength of gRPC is its support for various streaming modes. These include unary (a simple request-response pattern), server streaming (where the client sends a request, and the server responds with a stream of messages), client streaming (where the client sends a stream of messages, and the server issues a single response), and bidirectional streaming (where both client and server can send a stream of messages independently).
Finally, gRPC provides robust tooling for code generation, enabling the automatic creation of client and server code in a multitude of popular programming languages.
Pros of RPC (especially modern RPC like gRPC):
- Performance: It can be exceptionally performant, particularly when utilizing binary protocols like Protocol Buffers in conjunction with HTTP/2. Low latency is a hallmark benefit.
- Simplicity (for developers): The abstraction of a remote call as a local function can significantly simplify development efforts, especially for internal microservices.
- Strongly Typed Contracts: IDLs enforce a clear, unambiguous contract between the client and server, which helps in catching integration errors during compile time.
- Streaming Capabilities: It excels in scenarios that demand real-time data flow or the transfer of large datasets.
- Code Generation: The automatic generation of client libraries and server stubs reduces the amount of boilerplate code developers need to write.
Cons of RPC:
- Tight Coupling: Even with the use of IDLs, alterations to procedure signatures often necessitate the regeneration and redeployment of both client and server code.
- Discoverability: Unlike REST, there isn't a standardized method for discovering available procedures or their structures without prior access to the IDL or associated documentation.
- Less Browser-Friendly (Historically): Traditional RPC mechanisms were not as straightforward to integrate directly with web browsers when compared to REST. While gRPC-Web aims to bridge this gap, it typically requires a proxy layer.
- Firewall Traversal: Non-HTTP based RPC mechanisms could sometimes encounter difficulties with firewalls that are predominantly configured to permit HTTP traffic. gRPC, by using HTTP/2, largely mitigates this concern.
When to Use RPC:
Consider RPC for internal microservice communication where performance and low latency are critical design goals. It is also well-suited for applications requiring complex, high-performance streaming of data. If a clearly defined, strongly-typed contract between services is desired, RPC offers significant advantages. Polyglot environments, where code generation for multiple languages can streamline development, also benefit from RPC. Lastly, in network-constrained environments where the efficiency of message size is paramount, RPC, particularly gRPC with Protocol Buffers, is a strong candidate.
REST: Resources and Hypermedia
What is REST?
REST, or Representational State Transfer, is not a protocol or a rigid standard, but rather an architectural style for designing networked applications. It was meticulously defined by Roy Fielding in his 2000 doctoral dissertation. REST skillfully leverages the existing features and protocols of HTTP, placing emphasis on a stateless, client-server, cacheable mode of communication. The central concept revolves around resources (data entities) being uniquely identified by URLs, and interactions with these resources being performed using standard HTTP methods.
Core Principles of REST (Constraints):
The REST architectural style is defined by several guiding constraints:
A fundamental principle is the Client-Server Architecture. This mandates a clear separation of concerns. The client is responsible for the user interface and user experience aspects, while the server manages data storage, business logic, and the provision of the API itself.
Another crucial constraint is Statelessness. Every request sent from a client to the server must encapsulate all the information required for the server to understand and process that request. The server does not retain any client context (session state) between individual requests. Any state pertinent to a session is maintained on the client side.
Cacheability is also a key tenet. Responses generated by the server must explicitly define themselves as either cacheable or non-cacheable. This allows clients and intermediary systems (such as Content Delivery Networks or CDNs) to cache responses, which can significantly enhance performance and scalability.
REST systems are designed as a Layered System. This means a client typically cannot ascertain whether it is connected directly to the end server or to an intermediary (like a load balancer or proxy) along the communication path. Intermediary servers can bolster system scalability by facilitating load balancing and providing shared caches.
The Uniform Interface constraint is arguably what most distinguishes REST and serves to simplify and decouple the architecture. This constraint is further broken down into several sub-constraints.
First, Identification of Resources: All conceptual resources are identified within requests using URIs (Uniform Resource Identifiers), typically URLs. For example, /users/123 uniquely identifies a specific user resource.
Second, Manipulation of Resources Through Representations: Clients interact with resources not by directly invoking methods on them, but by exchanging representations of these resources. A representation can be in various formats, such as JSON, XML, or HTML. The client indicates its preferred format(s) using Accept headers, while the server specifies the format of the sent representation using the Content-Type header.
Third, Self-Descriptive Messages: Each message exchanged must contain sufficient information to describe how it should be processed. For instance, HTTP headers like Content-Type and Content-Length provide metadata about the message body, and status codes inform the client about the outcome of its request.
Fourth, Hypermedia as the Engine of Application State (HATEOAS): This principle, often considered the most sophisticated and sometimes the least implemented aspect of REST, dictates that server responses should include links (hypermedia). These links guide the client by indicating what actions it can take next or what related resources it can access. This allows clients to navigate the API dynamically, rather than relying on hardcoded URIs. For example, a response for a user resource might include links to view their orders or update their profile details.
An optional constraint is Code on Demand. This allows servers to temporarily extend or customize the functionality of a client by transferring executable code, such as JavaScript snippets.
How REST Works:
In a RESTful system, everything is conceptualized as a resource (e.g., a user, a product, an order). Each resource is uniquely identified by a URI. For instance, GET /users
might retrieve a list of users, while GET /users/123
retrieves the specific user with ID 123.
Standard HTTP Methods (Verbs) are employed to perform actions on these resources. GET
is used to retrieve a resource. POST
typically creates a new resource or can be used to trigger a process, with data for the new resource sent in the request body. PUT
is used to update an existing resource, usually requiring a full replacement of the resource's data, and is idempotent (multiple identical requests have the same effect as a single request). DELETE
removes a resource. PATCH
allows for partial updates to an existing resource. HEAD
retrieves metadata about a resource, similar to GET
but without the response body. OPTIONS
is used to get information about the communication options for the target resource.
Status Codes, which are part of the HTTP standard, are used to indicate the outcome of a request. Examples include 200 OK
, 201 Created
for successful creation, 400 Bad Request
for client errors, 404 Not Found
when a resource doesn't exist, and 500 Internal Server Error
for server-side issues.
Regarding Data Formats, JSON (JavaScript Object Notation) has become the most prevalent format for exchanging data in REST APIs due to its lightweight nature and ease of parsing. However, XML, HTML, or even plain text can also be utilized.
Pros of REST:
- Simplicity and Familiarity: It leverages well-understood HTTP standards, making it relatively easy to learn, implement, and consume.
- Statelessness: This simplifies server design and enhances scalability because servers do not need to maintain client session information between requests.
- Cacheability: HTTP caching mechanisms can be directly and effectively utilized to improve performance and reduce server load.
- Decoupling: The client and server are decoupled. As long as the resource URI and method contract remain consistent, the underlying implementations on either side can evolve independently.
- Discoverability (with HATEOAS): When HATEOAS is properly implemented, clients can dynamically discover available actions and navigate through resources, making the API more flexible and evolvable.
- Wide Adoption and Tooling: There is a vast ecosystem of tools, libraries, client SDKs, and gateways that support RESTful APIs. It is inherently browser-friendly.
- Human-Readable: URIs are often designed to be human-readable, and common data formats like JSON are easy for developers to inspect and debug.
Cons of REST:
- Over-fetching and Under-fetching: These are common issues.
- Over-fetching occurs when an endpoint returns more data than the client actually needs for a particular task. For example, to display a list of user names, a
/users
endpoint might return complete user objects including addresses, phone numbers, and other details for every user, most ofwhich might be unused. - Under-fetching happens when a client needs to make multiple requests to different endpoints to gather all the data it requires for a complete view. For instance, to get a user's details and their recent posts, a client might first need to call
/users/{id}
and then make a separate call to/users/{id}/posts
. - Multiple Round Trips: The problem of under-fetching often leads to multiple network round trips, which can increase latency and negatively impact user experience, especially on mobile or unreliable networks.
- Versioning Challenges: Evolving REST APIs without breaking existing clients can be challenging. Common strategies include URI versioning (e.g.,
/v1/users
), using custom request headers for versioning, or employing media type versioning (viaAccept
headers). Each approach has its own set of complexities and trade-offs. - HATEOAS Often Neglected: Despite being a core principle of REST, HATEOAS is frequently not fully implemented. This limits the true discoverability and dynamic evolvability that REST aims to provide.
- No Strong Typing Out-of-the-Box: Unlike RPC systems that use IDLs, REST relies on conventions and external documentation (such as OpenAPI/Swagger specifications) for defining API contracts. These are not always enforced at compile time, potentially leading to integration issues.
When to Use REST:
REST is an excellent choice for public-facing APIs where broad adoption, ease of integration, and interoperability are important. It is well-suited for resource-centric applications where standard CRUD (Create, Read, Update, Delete) operations on entities form the primary mode of interaction. If leveraging HTTP caching is crucial for performance and scalability, REST's alignment with HTTP standards is a significant advantage. Situations demanding statelessness and horizontal scalability also benefit greatly from the RESTful architectural style. Furthermore, when discoverability through hypermedia (HATEOAS) is desired to allow clients to dynamically navigate the API, REST provides the framework for it.
GraphQL: A Query Language for Your API
What is GraphQL?
GraphQL is a query language specifically designed for APIs, and it also encompasses a server-side runtime for executing these queries using a type system that you define for your data. Originally developed by Facebook and subsequently open-sourced in 2015, GraphQL was conceived to directly address some of the inherent limitations observed in RESTful architectures, most notably the twin problems of over-fetching and under-fetching data. It empowers clients to request precisely the data they need, no more and no less, typically within a single request-response cycle.
Core Concepts of GraphQL:
GraphQL's architecture is built upon several foundational concepts.
A cornerstone is the Schema Definition Language (SDL). GraphQL APIs are rigorously defined by a strong type system. The server publishes a schema that meticulously describes all the types of data a client is permitted to query, as well as the intricate relationships that exist between these data types. This schema serves as a binding contract between the client and the server. Within the SDL, you define various constructs:
- Types describe the objects you can fetch and their fields (e.g.,
type User { id: ID!, name: String, email: String, posts: [Post] }
). - Queries define the entry points for data fetching operations (e.g.,
type Query { user(id: ID!): User, posts: [Post] }
). - Mutations define the entry points for data modification operations—creating, updating, or deleting data (e.g.,
type Mutation { createUser(name: String!, email: String!): User }
). - Subscriptions define how clients can receive real-time updates when specific data changes on the server (e.g.,
type Subscription { newPost: Post }
).
Another distinguishing feature is its typical use of a Single Endpoint. Unlike REST, which commonly employs multiple URLs to represent different resources and operations, GraphQL APIs usually expose just one endpoint (e.g., /graphql
). All requests, whether they are queries for fetching data, mutations for modifying data, or subscriptions for real-time updates, are directed to this singular endpoint, generally via an HTTP POST request.
Central to GraphQL's power is the concept of Client-Specified Queries. The client application constructs a query string that explicitly specifies exactly what data it requires. This can include not only fields on a primary object but also fields on related objects, traversing complex data relationships. The server then processes this query and responds with a JSON object whose structure precisely mirrors the structure of the query submitted by the client.
How GraphQL Works:
The interaction in a GraphQL system follows a well-defined flow. It begins with Schema Definition, where the server defines its data capabilities using GraphQL's SDL.
Subsequently, the Client Query is constructed. The client formulates a GraphQL query string, detailing the specific data fields it needs. For example:GraphQL
query GetUserDetails {
user(id: "123") {
id
name
email
posts { # Fetch related posts
title
content
}
}
}
This query is then sent in a Request to Server, usually as a JSON payload within an HTTP POST request, targeting the single GraphQL endpoint.
Upon receiving the request, Server Processing commences. This involves several sub-steps. The server first Parses & Validates the incoming query, checking its syntax and ensuring it conforms to the defined schema. If valid, the query moves to Execution. The server executes the query by invoking "resolver" functions. Each field defined in the GraphQL schema is backed by a corresponding resolver function. A resolver is a piece of code responsible for fetching the data for its specific field. These resolvers can retrieve data from various sources, such as databases, other internal or external APIs, or even static data.
Finally, the server formulates and sends a Response to Client. This response is a JSON object whose structure mirrors the shape of the original query, containing only the data that was explicitly requested. For the example query above, the response might look like:JSON
{
"data": {
"user": {
"id": "123",
"name": "Alice Wonderland",
"email": "alice@example.com",
"posts": [
{
"title": "My First Post",
"content": "Hello world!"
},
{
"title": "GraphQL is Cool",
"content": "Learning about GraphQL..."
}
]
}
}
}
Pros of GraphQL:
- No Over-fetching or Under-fetching: Clients request precisely the data they require, leading to highly efficient data transfer and reducing wasted bandwidth.
- Single Request for Multiple Resources: Related data, even across different conceptual resources, can be fetched in a single query, significantly reducing the number of network round trips.
- Strongly Typed Schema: The schema serves as a clear and authoritative contract between client and server. This strong typing enables powerful developer tools, such as auto-completion, static analysis, and query validation, and it also acts as a form of self-documentation.
- Evolvability: It becomes easier to evolve APIs without resorting to versioning. Adding new fields or types to the schema does not break existing clients, as they only receive the data they explicitly request. Deprecating old fields is also more straightforward.
- Real-time Data with Subscriptions: GraphQL includes built-in support for real-time updates through subscriptions, allowing clients to listen for specific data changes on the server.
- Introspective: GraphQL APIs are introspective by nature. Clients can query the schema itself to discover the available types, fields, queries, mutations, and subscriptions, facilitating exploration and dynamic client generation.
Cons of GraphQL:
- Complexity: Setting up and managing a GraphQL server can be more complex compared to simple REST APIs, especially concerning the implementation of resolver logic, schema design, and performance optimization.
- Caching: HTTP caching mechanisms are less straightforward to apply directly in GraphQL compared to REST's resource-based caching. While sophisticated client-side caching solutions (like Apollo Client or Relay) exist, server-side and intermediary caching require different strategies, and field-level caching can be intricate.
- File Uploads: The GraphQL specification does not natively handle file uploads. This typically requires workarounds, such as using separate REST endpoints for file handling or implementing multipart request processing libraries alongside GraphQL.
- Rate Limiting: Implementing effective rate limiting can be more complex because the "cost" or resource intensity of a GraphQL query is not immediately obvious just by looking at it; a deeply nested or complex query can be very expensive to execute. This often necessitates query cost analysis mechanisms.
- Learning Curve: There is a learning curve associated with understanding GraphQL concepts, mastering schema design best practices, and becoming proficient in the query language itself.
- Performance Pitfalls: Poorly written resolvers or excessively complex, deeply nested queries can lead to performance issues, such as the N+1 problem (where fetching a list of items and their children results in one query for the list and N additional queries for the children of each item), if not handled carefully with techniques like data loaders.
When to Use GraphQL:
GraphQL is particularly well-suited for applications with diverse clients, such as web applications, mobile apps, and IoT devices, all of which may have varying data requirements. It shines in situations where minimizing data transfer and reducing the number of network round trips are critical, for example, in mobile applications operating on slow or unreliable networks. For complex systems where clients need to fetch data from multiple underlying sources or navigate deeply nested resource relationships, GraphQL offers an elegant solution. If a strongly typed API contract, robust self-documentation capabilities, and API evolvability are highly valued, GraphQL provides these benefits. Applications requiring real-time updates via subscriptions can leverage GraphQL's native support for this feature. Ultimately, GraphQL is an excellent choice when you want to give clients more power and flexibility in how they fetch data, tailoring requests to their precise needs.
RPC vs. REST vs. GraphQL: A Comparative Glance
Feature | RPC (e.g., gRPC) | REST | GraphQL |
Paradigm | Action/Function oriented | Resource oriented | Data query language |
Endpoints | Multiple endpoints (one per procedure) | Multiple endpoints (one per resource/collection) | Typically a single endpoint (/graphql ) |
Data Fetching | Server dictates data returned per procedure | Server dictates data returned per resource | Client dictates exactly what data is needed |
Over/Under Fetching | Prone to both, based on procedure design | Common problem (over-fetching/under-fetching) | Solves over/under-fetching |
HTTP Usage | Can use HTTP/2 (gRPC), or other transports | Heavily relies on HTTP methods, status codes | Typically uses HTTP POST, single endpoint |
Coupling | Tighter coupling (client needs to know methods) | Looser coupling (via hypermedia if used) | Decoupled (client queries schema) |
Schema/Contract | Defined by IDL (e.g., Protobufs), strong typing | Defined by convention/OpenAPI, less strict | Strongly typed Schema Definition Language (SDL) |
Caching | Custom, or via HTTP if on HTTP transport | Standard HTTP caching (GET requests) | More complex, often client-side or field-level |
Performance | High (esp. gRPC with Protobuf & HTTP/2) | Good, but can be affected by multiple calls | Good, efficient data transfer, complex queries |
Streaming | Strong support (gRPC) | Limited (chunked transfer, server-sent events) | Subscriptions for real-time updates |
Browser Support | gRPC-Web needs a proxy | Native, very browser-friendly | Good, libraries available |
Discoverability | Via IDL or documentation | HATEOAS (if implemented), OpenAPI | Introspection queries on the schema |
Evolution | Changes can break clients | Versioning strategies needed (URI, header) | Easier; additive changes don't break clients |
Choosing the Right Approach: It Depends
There is no universally "best" choice among RPC, REST, and GraphQL. The optimal solution depends heavily on the specific requirements of your project, your team's expertise, and the nature of the communication.
Choose RPC (especially gRPC) if:
- You are building internal microservices where high performance and low latency are paramount.
- You need efficient binary serialization and bidirectional streaming.
- A strongly typed contract and code generation across multiple languages are important.
- Network bandwidth is a significant constraint.
Choose REST if:
- You are building public APIs that need to be easily consumable by a wide range of clients.
- You want to leverage standard HTTP caching mechanisms effectively.
- Your application is primarily resource-centric with standard CRUD operations.
- Simplicity of the architecture and wide industry familiarity are priorities.
- Statelessness and scalability are key drivers.
Choose GraphQL if:
- Your clients (especially mobile or diverse frontends) have varying and specific data needs, and you want to avoid over/under-fetching.
- You need to fetch complex, nested data structures in a single request.
- A strongly typed schema, self-documentation, and API evolvability are crucial.
- Real-time updates with subscriptions are a requirement.
- You want to empower clients to request precisely what they need.
Hybrid Approaches:
It's also common to see hybrid approaches. For instance:
- A system might use REST for its public-facing API and gRPC for internal microservice communication.
- A GraphQL server might act as a facade, fetching data from various downstream REST or gRPC services.
- Some specific operations like file uploads might be handled by a REST endpoint even if the primary API is GraphQL.
The Future of API Design
The landscape of API design is continuously evolving. While REST remains a dominant force for public APIs, gRPC has gained significant traction for inter-service communication due to its performance benefits. GraphQL's rise has been propelled by its efficiency in data fetching and its developer-friendly approach to handling complex data requirements, especially for modern frontend applications.
We are also seeing the emergence of patterns and technologies that build upon or combine aspects of these paradigms. Technologies like AsyncAPI for event-driven architectures, or specification formats like OpenAPI for REST, are maturing and providing better tooling and governance.
The core principles of good API design – clarity, predictability, robustness, and maintainability – remain timeless. Understanding the strengths and weaknesses of RPC, REST, and GraphQL allows architects and developers to make informed decisions, selecting the right tool for the job to build efficient, scalable, and maintainable distributed systems. As applications become more complex and interconnected, the ability to design and consume APIs effectively will only grow in importance. Each paradigm offers a unique set of tools and philosophies to tackle the challenges of modern software communication, ensuring that the digital world remains seamlessly connected.
Want an integrated, All-in-One platform for your Developer Team to work together with maximum productivity?
Apidog delivers all your demans, and replaces Postman at a much more affordable price!
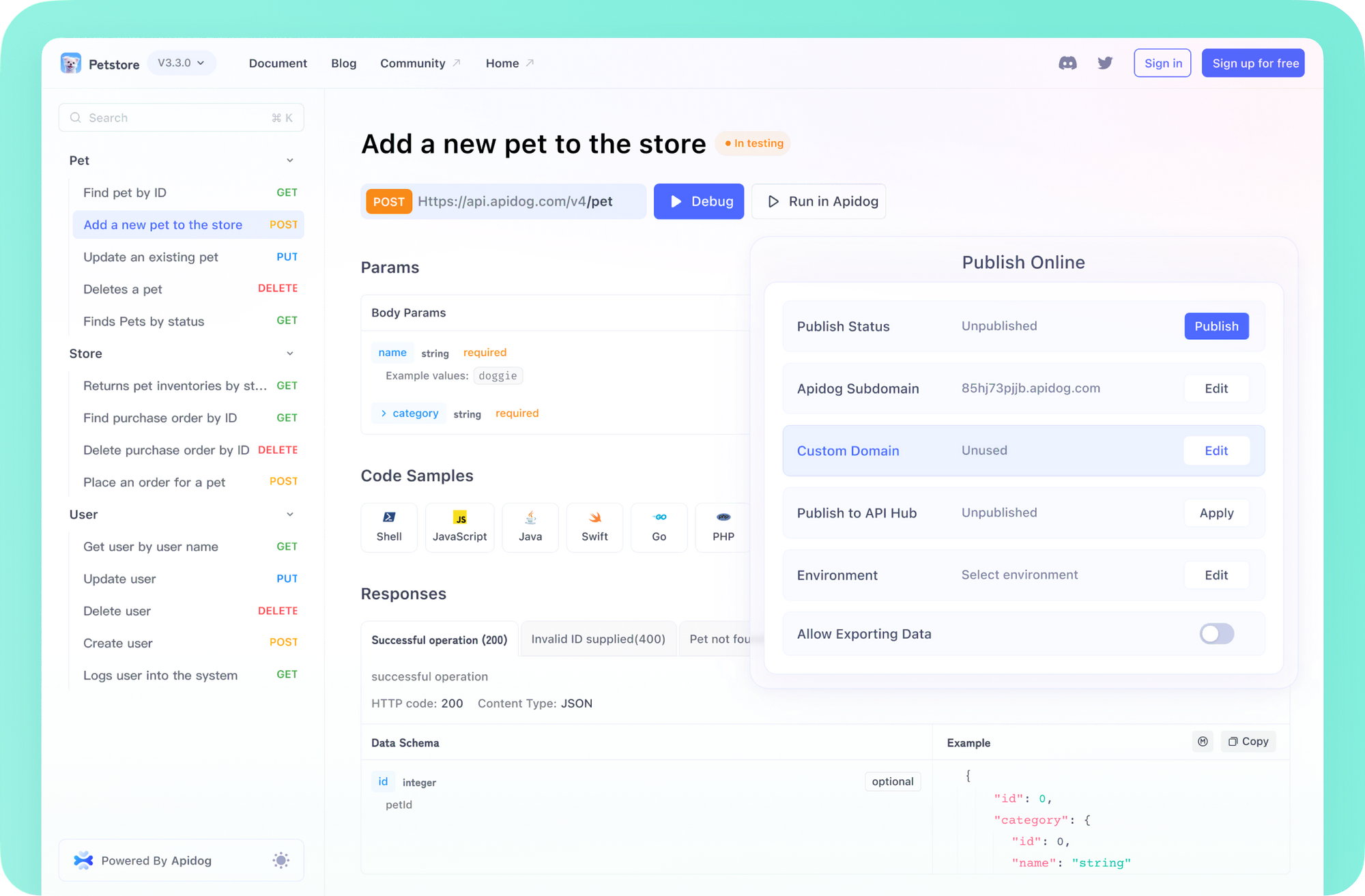