Reverse engineering APIs is the process of analyzing an API’s behavior, endpoints, and data structures without relying on official documentation. It involves capturing and inspecting network traffic, decoding data formats, and replicating API calls to understand how the API works.
In the world of software development, reverse engineering becomes crucial in several scenarios:
- Legacy Systems: When dealing with old, undocumented APIs that haven't been maintained.
- Third-Party Integrations: When integrating with third-party services that offer limited or no documentation.
- Unexplored APIs: Sometimes, APIs are created without providing adequate documentation or public-facing details.
Reverse engineering enables developers to understand these APIs, enabling smoother integration and more efficient troubleshooting.
Why is Reverse Engineering API Important?
Reverse engineering API offers significant benefits, especially when it comes to handling complex integrations or working with systems that do not offer public-facing documentation. Here’s why reverse engineering can be a game-changer:
- Understand Hidden Functionalities: You get to unveil undocumented features or endpoints, enabling better integration with third-party services.
- Accelerate Development Time: Understanding an API's inner workings can help speed up development by avoiding guesswork.
- Create Custom Integrations: Reverse engineering allows you to create tailored integrations with systems that you might not have full access to.
Benefits of Reverse Engineering API
- Increased Efficiency: Helps developers identify bugs faster and troubleshoot issues effectively.
- Improved API Interactions: By analyzing how an API responds, developers can optimize their interactions with the system.
- Better Documentation: As you reverse-engineer the API, you can document the discovered endpoints and parameters for your own use or share them with others. (Pro tip: Save time and effort by using Apidog's automated API documentation feature, which generates comprehensive documentation without the need for manual writing.)
Challenges of Reverse Engineering APIs
Although reverse engineering an API can be a powerful for development, it also comes with its own set of challenges and disadvantages that developers need to consider.
- Legal and Ethical Concerns: Some APIs are protected under terms of service agreements that prohibit reverse engineering. It’s essential to review any legal restrictions before diving into the process.
- Time-Consuming: Reverse engineering can be a complex and time-consuming process, requiring developers to manually test various endpoints and analyze responses. (Pro tip: You can use Apidog to easily send request and get API response report.)
- Risk of Errors: Without access to the source code or detailed documentation, there’s always a risk of misunderstanding how the API works, leading to integration errors.
Disadvantages
- Limited Visibility: Reverse engineering doesn’t give you the full picture; you only see what’s accessible through requests and responses.
- Inconsistencies: Some APIs may change their behavior over time, making it difficult to maintain consistency when reverse engineering over long periods.
General Steps to Reverse Engineering APIs
Here’s a general approach to reverse engineering APIs:
- Capture Network Traffic: Use tools like Charles or Proxyman to intercept HTTP/HTTPS requests and responses.
- Analyze Requests and Responses: Identify endpoints, headers, parameters, and data formats.
- Replicate API Calls: Use tools like Apidog or cURL to recreate and test API requests.
- Decode Data Formats: Convert encrypted or obfuscated data into readable formats.
- Document Findings: Record API behavior, endpoints, and data structures for future reference.
Best Tools for Reverse Engineering APIs
The right tools are essential for efficiently reverse engineering APIs. Here are some of the best tools to help you in the process:
Tool | Purpose | Why It's Useful |
---|---|---|
Apidog | API Design, Testing, Debugging, Mocking | Apidog is an all-in-one API development tool that simplifies debugging and testing. It helps developers test requests, mock API responses, and document API endpoints efficiently. |
Burp Suite | Web Application Security Testing | Great for inspecting traffic between a client and an API, helping identify hidden endpoints and data flows. |
Wireshark | Network Protocol Analyzer | Captures network traffic to analyze API requests and responses directly from HTTP(S) calls. |
Fiddler | HTTP Debugging Proxy | Useful for capturing and analyzing HTTP(s) traffic between a client and a server. |
Charles | HTTP Proxy and Monitoring Tool | Charles is a popular tool for inspecting and analyzing the HTTP and SSL traffic between clients and servers. It’s great for understanding and debugging the interactions with APIs in real-time. |
Proxyman | API Debugging Proxy | Similar to Charles, Proxyman is another powerful tool designed to intercept and analyze HTTP(s) traffic, providing an intuitive UI to inspect requests, responses, and API behaviors easily. |
Why Apidog Stands Out:
- API Debugging: Apidog’s built-in debugging tools allow you to troubleshoot API responses in real-time.
- Mock API Responses: You can mock API responses to simulate different scenarios.
- Client Code Generation: Apidog allows you to automatically generate client code based on the reverse-engineered API.
Best Practices for Reverse Engineering APIs
To ensure ethical and effective reverse engineering, follow these best practices:
- Respect Legal Boundaries: Avoid violating terms of service or intellectual property rights.
- Prioritize Security: Do not expose sensitive data or bypass security mechanisms.
- Document Everything: Keep detailed records of your findings and modifications.
- Use the Right Tools: Leverage tools like Charles and Proxyman for reverse engineering APIs.
- Collaborate with Experts: Seek guidance from professionals to avoid pitfalls.
Practical Examples of API Reverse Engineering
To demonstrate the value of API reverse engineering, let's consider two practical scenarios:
Scenario 1: Integrating with Websites Without a Public API
Some platforms do not offer a public API but still provide valuable data. By reverse engineering the traffic between a web application and the server, developers can replicate the API's functionality. This involves capturing network traffic, analyzing authentication mechanisms, and replicating requests programmatically.
Scenario 2: Replacing a Real API with a Stub API
If your application is integrated with an API that frequently changes, has rate limits, or needs isolated testing, you can reverse engineer the real API to create a stub API. This allows for testing and auditing without affecting the live system.
Real-World Example: Capturing HTTPS Traffic from a Flutter iOS App with Proxyman
When developing or debugging a Flutter app, understanding the network traffic between your app and external APIs is crucial. However, capturing HTTPS traffic, especially on iOS devices, can be tricky. In this example, we’ll walk through how to use Proxyman, a powerful debugging proxy tool, to capture HTTPS traffic from a Flutter iOS app, whether you’re using an iOS Simulator or a physical device.
Step 1: Configuring HTTP Proxy in Flutter
By default, Flutter doesn’t use an HTTP proxy, so you’ll need to manually configure it in your project. The setup depends on the HTTP library you’re using. Below are examples for two popular libraries: the http
package and the Dio
library.
Using the http
Package:
import 'dart:io';
import 'package:http/io_client.dart';
// Replace <YOUR_LOCAL_IP> with your computer's IP address for Android.
// For iOS, use 'localhost:9090'.
String proxy = Platform.isAndroid ? '<YOUR_LOCAL_IP>:9090' : 'localhost:9090';
// Create a new HttpClient instance.
HttpClient httpClient = HttpClient();
// Configure the proxy.
httpClient.findProxy = (uri) {
return "PROXY $proxy;";
};
// Allow Proxyman to capture SSL traffic on Android.
httpClient.badCertificateCallback =
((X509Certificate cert, String host, int port) => true);
// Pass the configured HttpClient to the IOClient.
IOClient myClient = IOClient(httpClient);
// Make your network request as usual.
var response = await myClient.get(Uri.parse('https://example.com/my-url'));
Using the Dio
Library:
import 'package:dio/dio.dart';
// Replace <YOUR_LOCAL_IP> with your computer's IP address for Android.
// For iOS, use 'localhost:9090'.
String proxy = Platform.isAndroid ? '<YOUR_LOCAL_IP>:9090' : 'localhost:9090';
// Create a new Dio instance.
Dio dio = Dio();
// Configure the proxy and SSL settings.
(dio.httpClientAdapter as DefaultHttpClientAdapter).onHttpClientCreate = (client) {
client.findProxy = (url) {
return 'PROXY $proxy';
};
client.badCertificateCallback = (X509Certificate cert, String host, int port) => true;
};
// Make your network request as usual.
var response = await dio.get('https://example.com/my-url');
Step 2: Capturing Traffic on an iOS Simulator
1. Open the iOS Simulator: Ensure the simulator is running and set as the target for your Flutter app.
2. Install the Proxyman Certificate:
- Open Proxyman and navigate to the Certificate menu.
- Select Install for iOS -> Simulator.
- Follow the on-screen instructions. Proxyman will automatically configure the proxy and install the certificate.
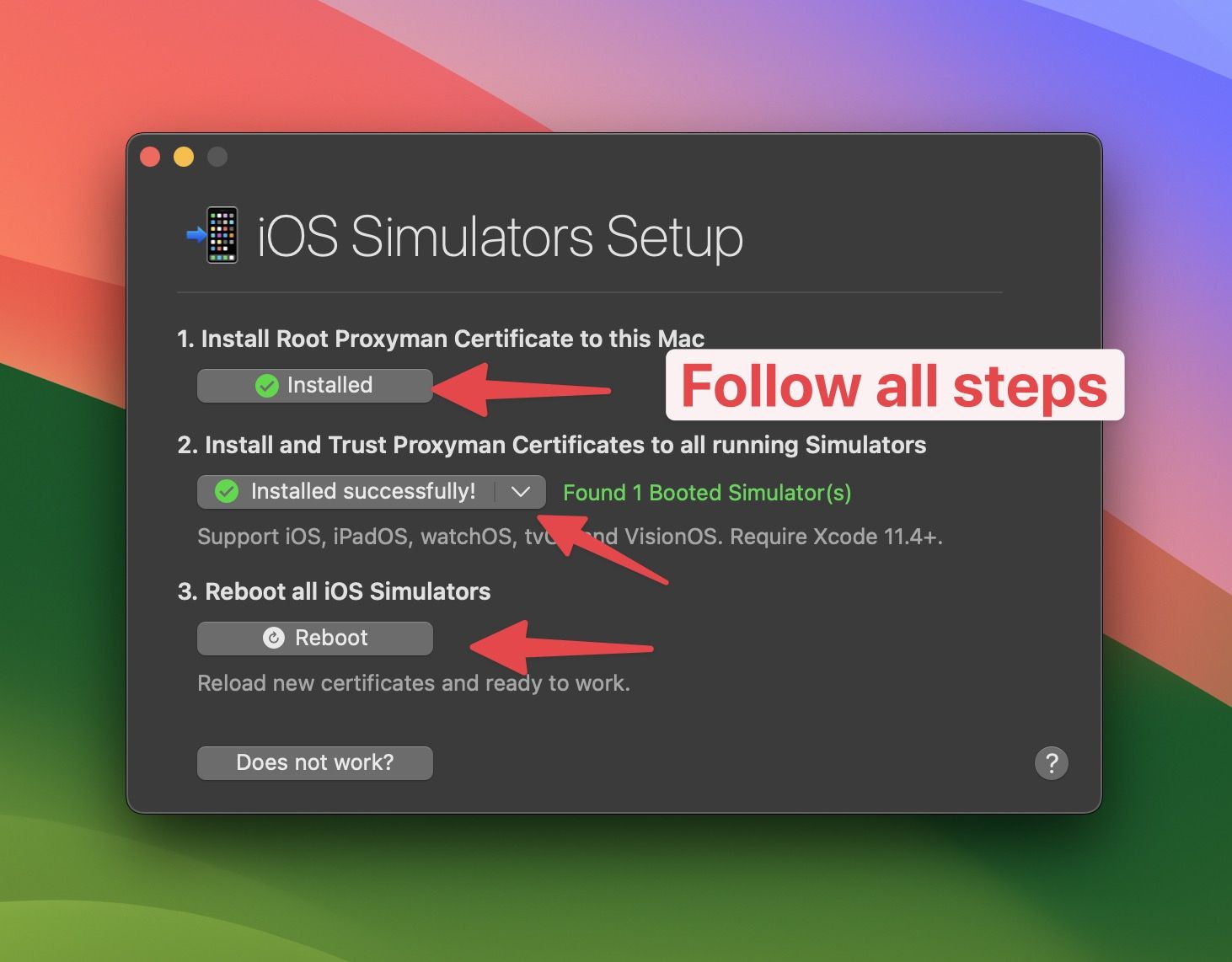
3. Run Your Flutter App: Launch your app on the simulator.
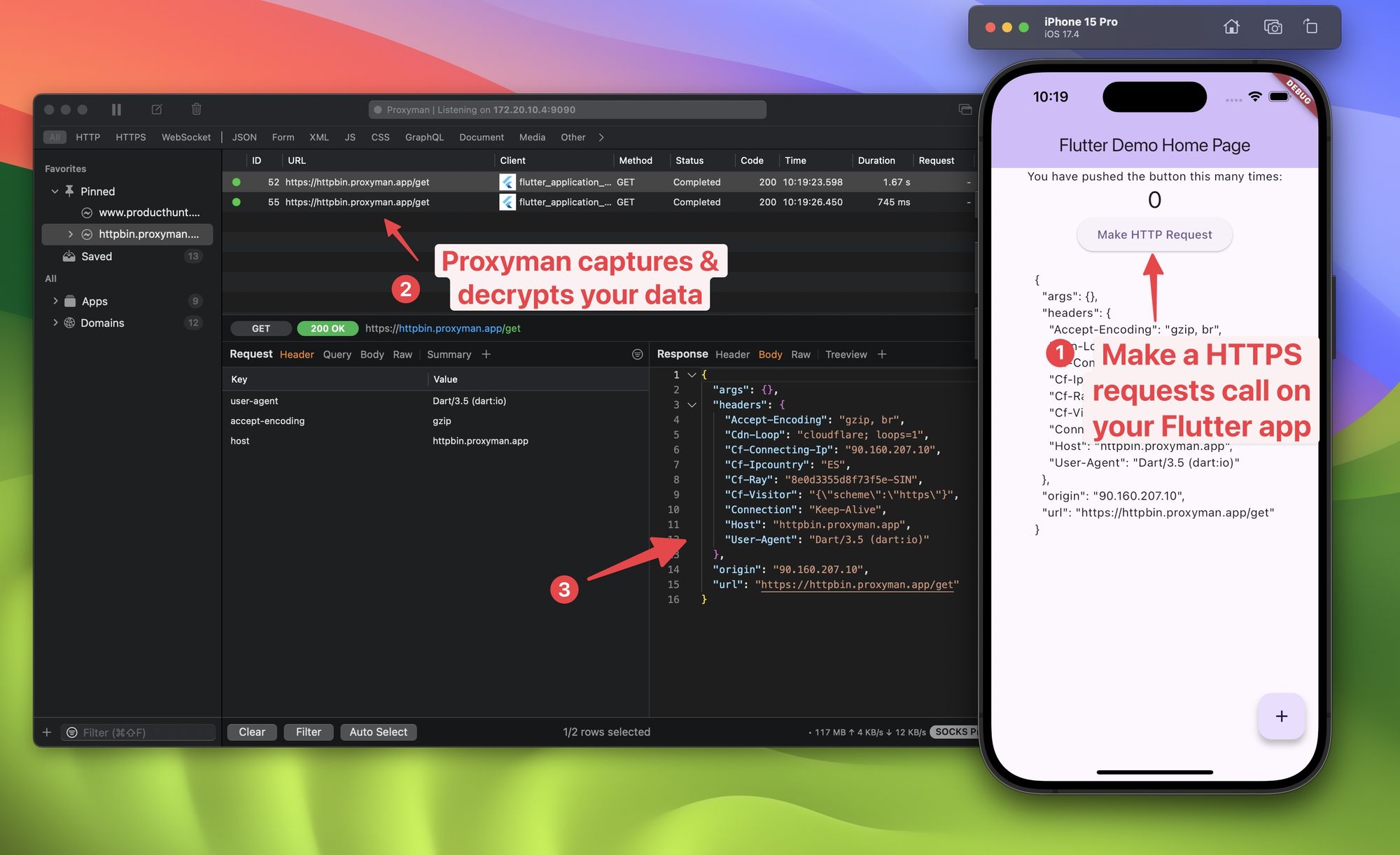
4. Capture Traffic: Proxyman will automatically start capturing all HTTP/HTTPS traffic from your app.
Step 3: Capturing Traffic on a Physical iOS Device
1. Install the Proxyman Certificate:
- Open Proxyman and go to the Certificate menu.
- Select Install for iOS -> Physical Device. Follow the steps to install.
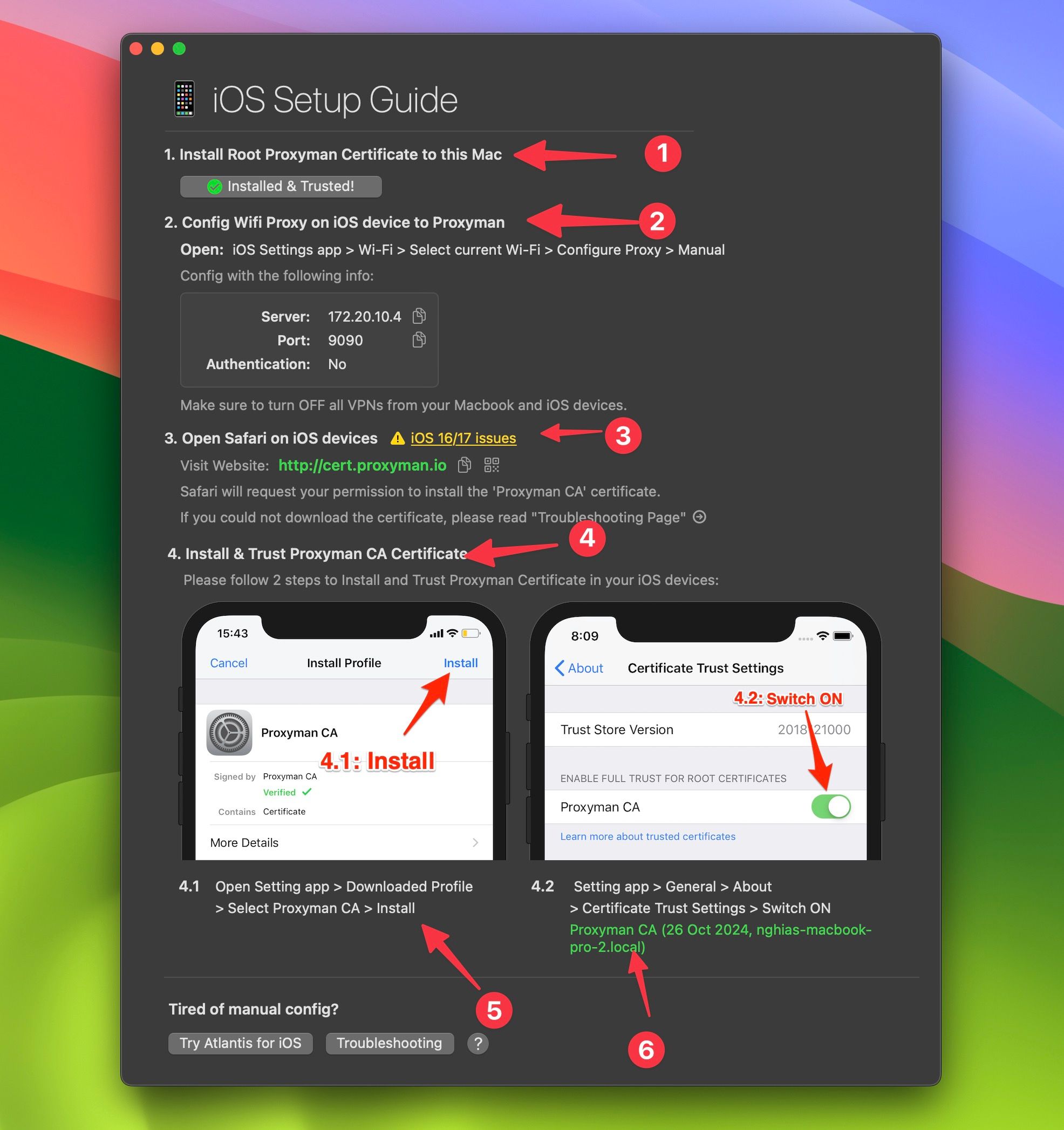
- Trust the certificate on your device.
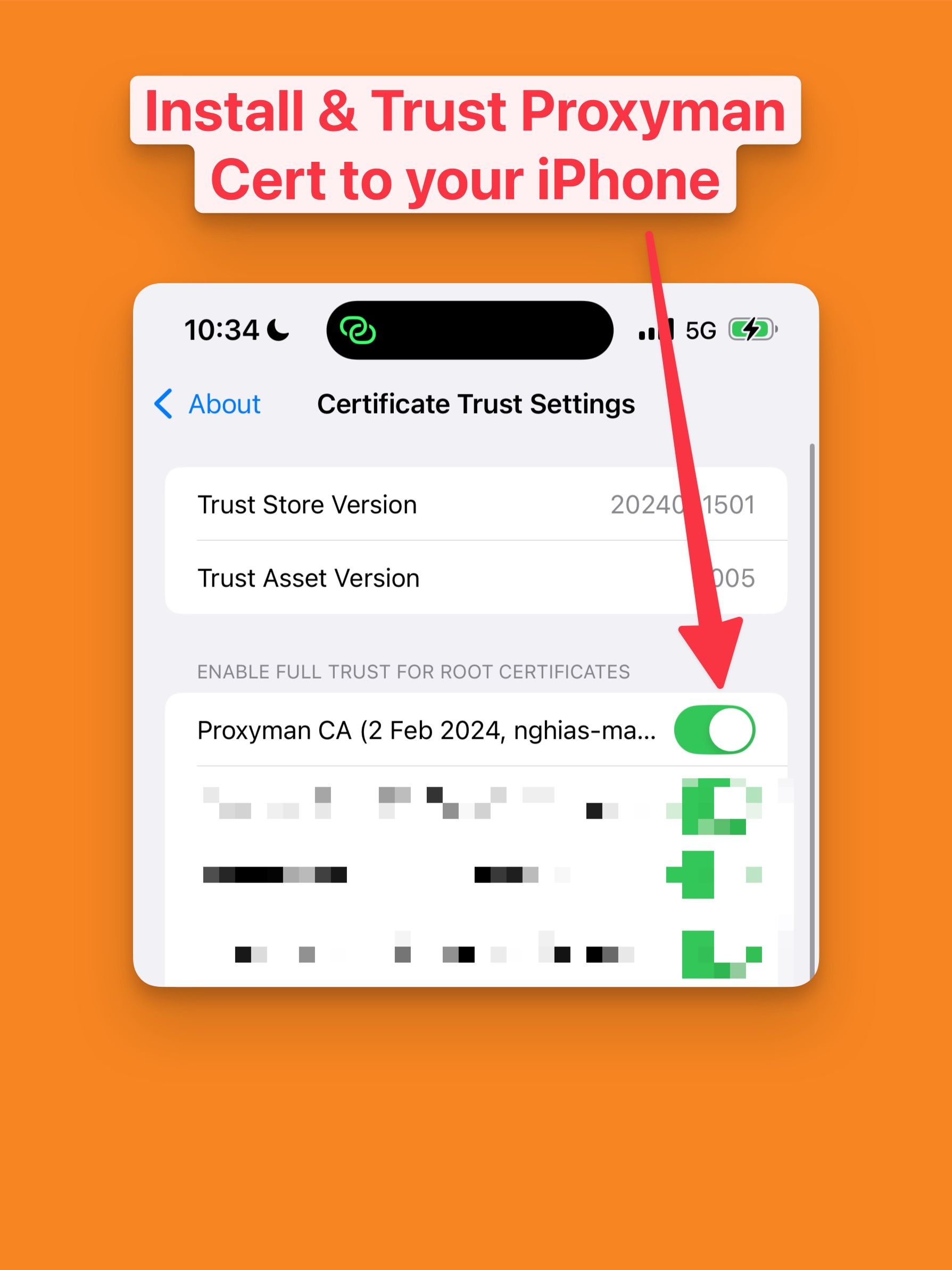
2. Run Your Flutter App: Launch your app on the physical device.
3. Capture Traffic: Proxyman will automatically capture all HTTP/HTTPS traffic from your app.
Capturing HTTPS traffic is essential for debugging API calls, inspecting request/response payloads, and ensuring your app communicates securely with backend services. Tools like Proxyman simplify this process, even for Flutter apps running on iOS, where HTTPS traffic is encrypted by default.
By following these steps, you can gain deep insights into your app’s network behavior, identify potential issues, and ensure seamless integration with external APIs. Whether you’re working with an iOS Simulator or a physical device, Proxyman makes it easy to capture and analyze HTTPS traffic, saving you time and effort during development.
Conclusion
Reverse engineering API is a powerful technique for understanding undocumented systems, optimizing integrations, and enhancing security. With tools like Charles and Proxyman, together with Apidog, developers can streamline the process, debug API requests, and ensure seamless communication between systems.