When using Apidog for API testing, there may be times when you need to extract the request parameters of the current test API for other purposes. For example, consider an order creation API for an e-commerce platform with the following request body:
{"id": 12345,"products": [
{"id": 1, "quantity": 2},
{"id": 3, "quantity": 1}
],"shippingAddress": "xx Province xx City xx District","paymentMethod": "credit_card"
}
In this scenario, you might want to:
- Extract and Encrypt Request Parameters: To protect privacy, you may need to encrypt the value of the
shippingAddress
parameter before sending it. - Store Request Parameters as Variables: You might want to set the
id
parameter as a "temporary variable" for use in subsequent API tests (like checking order status). - Validate Request Parameters: Before sending the request, you may need to check whether the
paymentMethod
parameter has a valid value.
To handle this, you can extract the request parameters from the API using the pm.request
method. You can create a custom script that prints pm.request
to the console, allowing you to view various parameter information. For example:
// Current api's request parameters informationconsole.log(pm.request);
The request
in this method refers to the request object of the API. In "Pre-request" scripts, it represents the "pending request," while in "Post-request" scripts, it refers to the "sent request."
You can decide whether to retrieve the current request parameters in the pre-request or post-request script based on your requirements:
- For parameters before sending the request: Use pre-request scripts.
- For parameters after sending the request: Use post-request scripts.
Note that only modifications made in the "Pre-request" scripts are effective; changes made in the "Post-request" scripts will not take effect.
This article mainly introduces how to obtain request parameters of the current API through scripts in Apidog's "Pre-request Script" and "Post-request Script" features.
To obtain request parameters from other APIs, you can store the parameters in environment variables and then read them, or use the "Tests" feature. With "Dynamic Values", you can directly read the request parameters or response data from previous steps (i.e., other APIs).
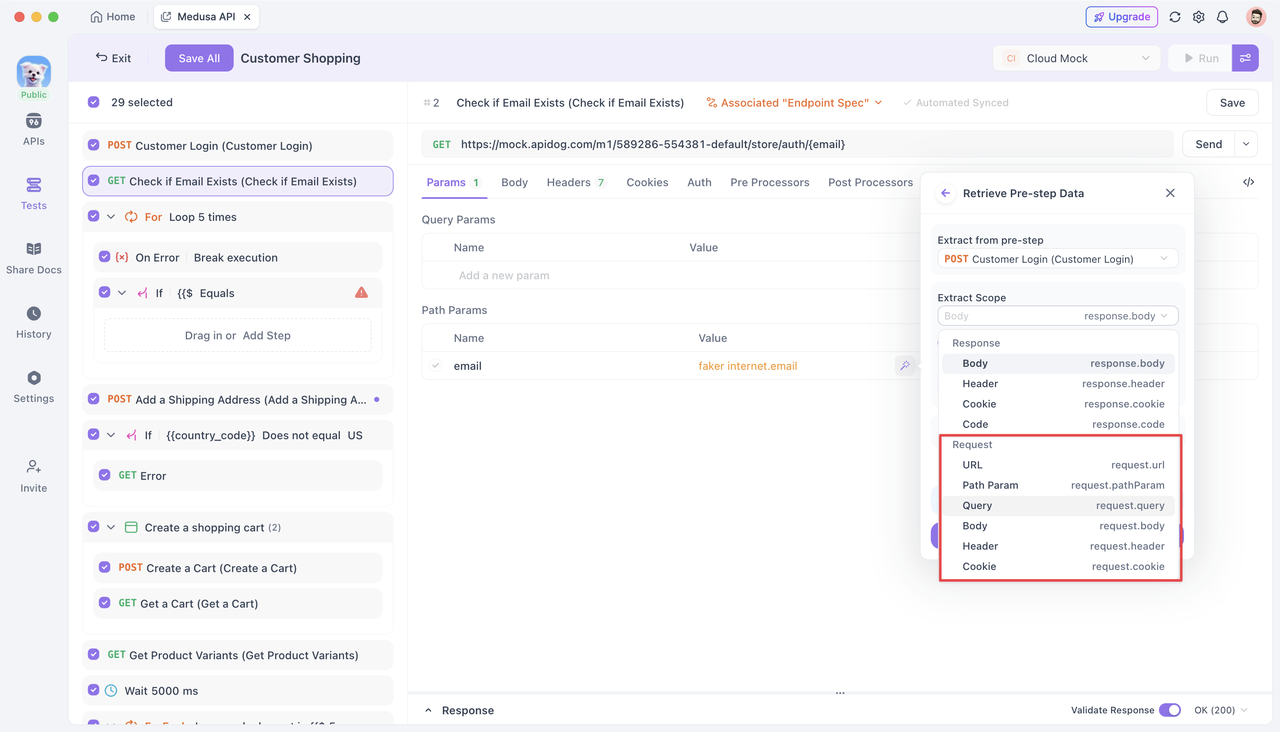
Step-by-step Guide to Extract Request Parameters?
Retrieve the Pre-Request URL
The pre-request URL is the service address configured in "Environment Management."
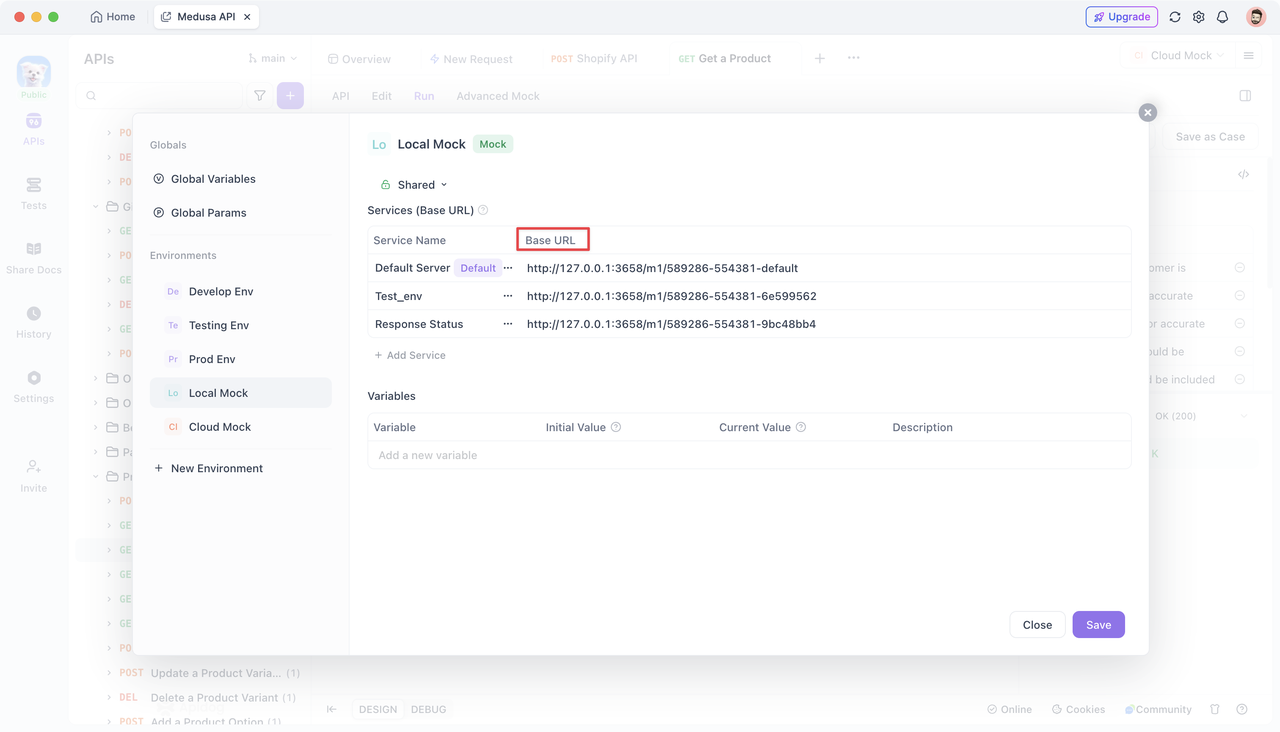
To get the current API's pre-request URL, you can use the pm.request.getBaseUrl()
or pm.request.baseUrl
methods. For example:
// Get the pre-request URL
let preUrl = pm.request.getBaseUrl();
console.log(preUrl);
// Or
let baseUrl = pm.request.baseUrl;
console.log(baseUrl);
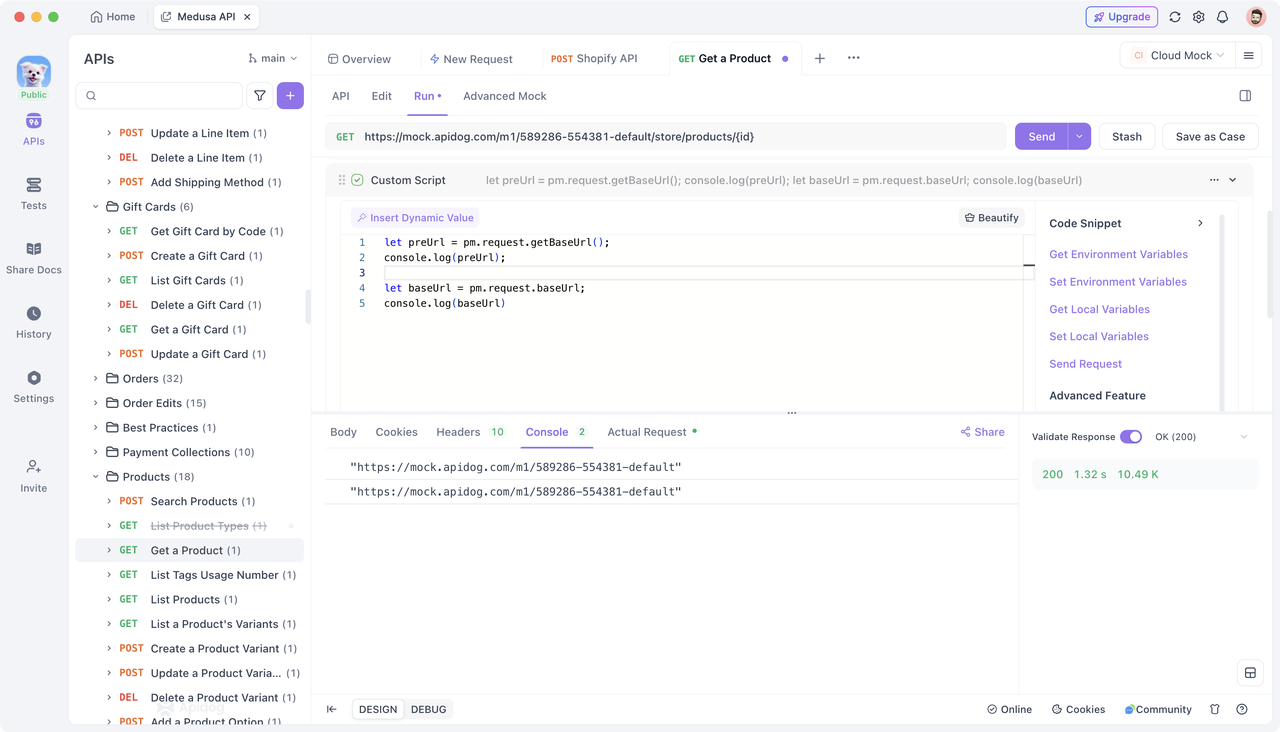
If multiple pre-request URLs are configured, the script will obtain the URL corresponding to the specified service. You can review and manage these configurations through "Edit-> Service (Pre-request URL)."
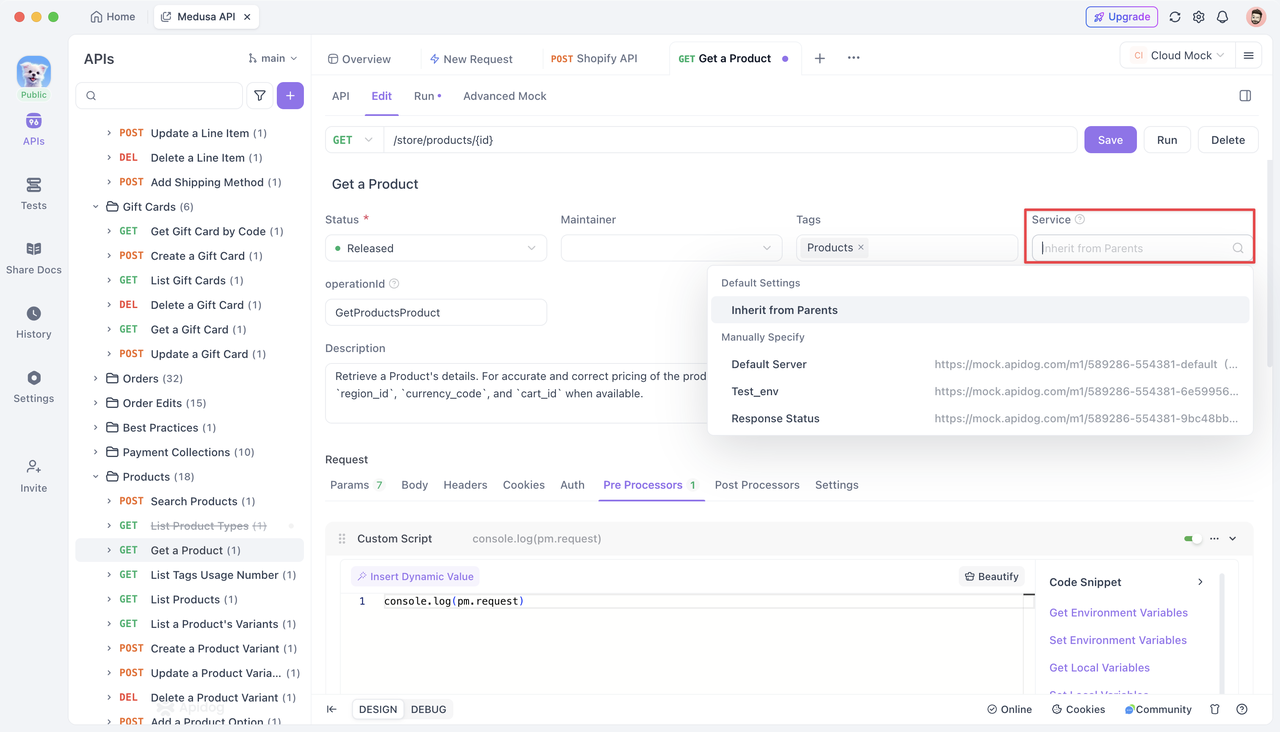
Retrieve Path Parameters
Path parameters are part of the URL used to specify the resource path, often identifying specific resources or collections. In Apidog, path parameters are represented using curly braces {}
, directly included in the URL path. For instance:
https://Apidogmock.com/xxx/xxx/store/products/{id}
In this example, {id}
is a path parameter that can be dynamically replaced. You can extract path parameters using pm.request.url.path
, which returns an array containing all path names, allowing precise access via array indexing:
// Get path parameters from pm.request.url.path
let getPathUrl = pm.request.url.path;
// Print data to consoleconsole.log(getPathUrl);
console.log(getPathUrl[4]);
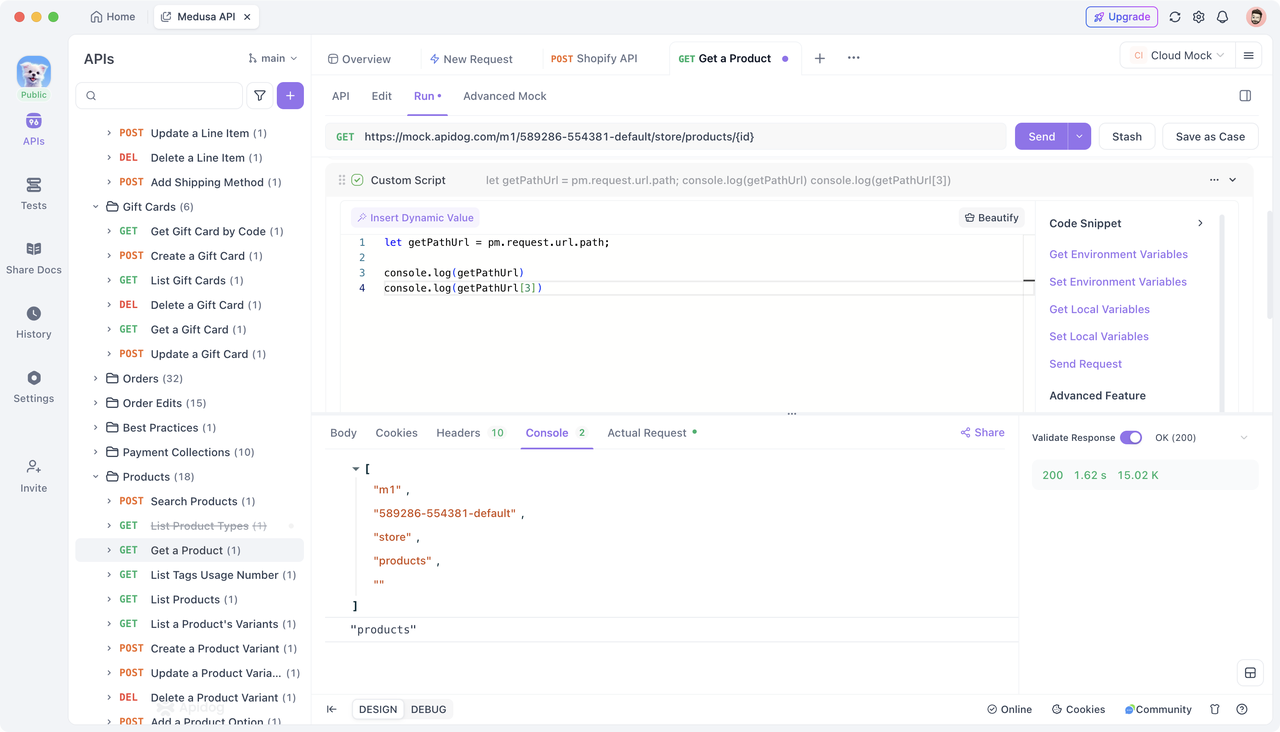
Retrieve Query Parameters
Query parameters are additional information attached to the URL, typically following the question mark ?
and formatted as key-value pairs, separated by &
. For example:
https://Apidogmock.com/xxx/xxx/store/products/{id}?cart_id=42®ion_id=43
To extract query parameters, use pm.request.url.query
, which retrieves all key-value pairs. You can then use the get()
method to specifically fetch a parameter. Here’s how to extract the cart_id
parameter:
// Get query parameters from pm.request.url.query
let getQueryUrl = pm.request.url.query;
// Print data to consoleconsole.log(getQueryUrl);
console.log(getQueryUrl.get("cart_id"));
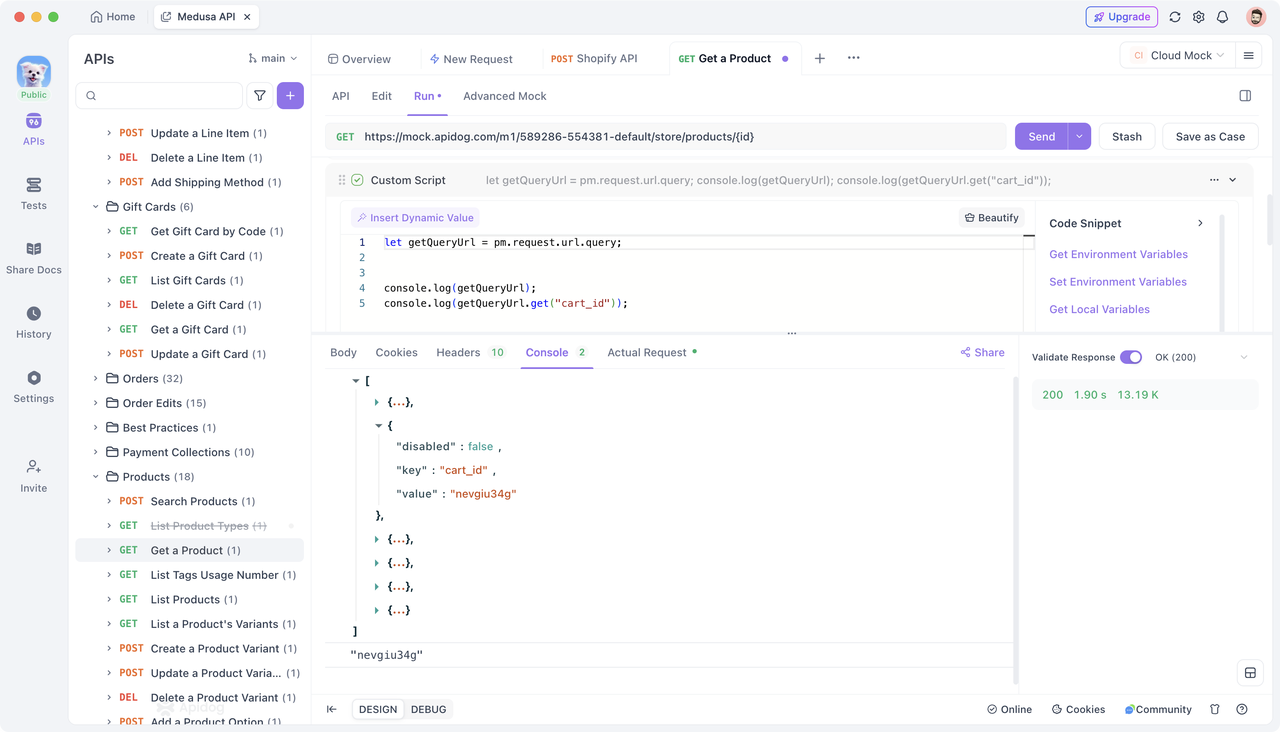
Get the Complete URL
To obtain the complete URL of the API request, including the pre-request URL, path parameters, and query parameters, use the pm.request.url.toString()
method:
// Print complete URL to consoleconsole.log(pm.request.url.toString());
This method returns a string containing all URL information, with dynamic replacements for path parameters and all query parameters.
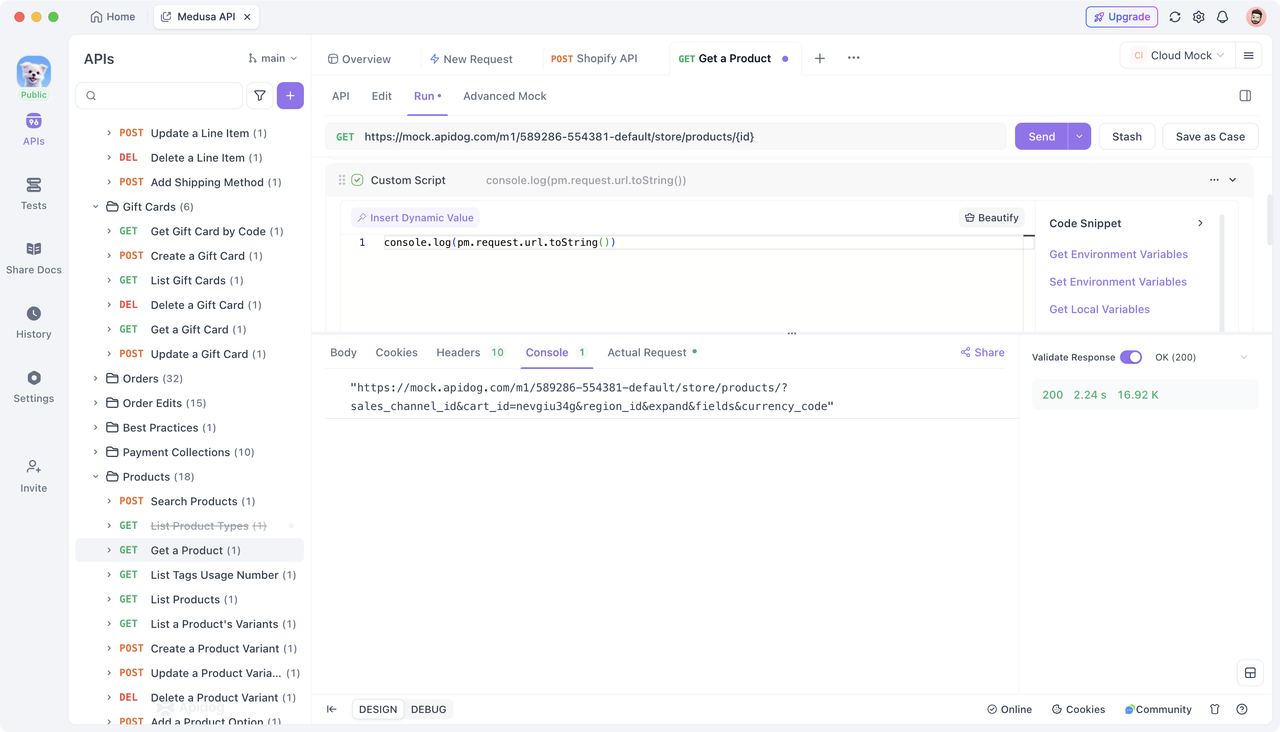
Retrieve Body Parameters
- For form-data format: Form-data organizes data in key-value pairs, often used for file uploads and complex form submissions. To obtain form-data body parameters, use
pm.request.body.formdata
, which returns an array object:
// When body type is form-data
let formData = pm.request.body.formdata;
// Print data to consoleconsole.log(formData);
console.log(formData.get("email"));
console.log(formData.get("password"));
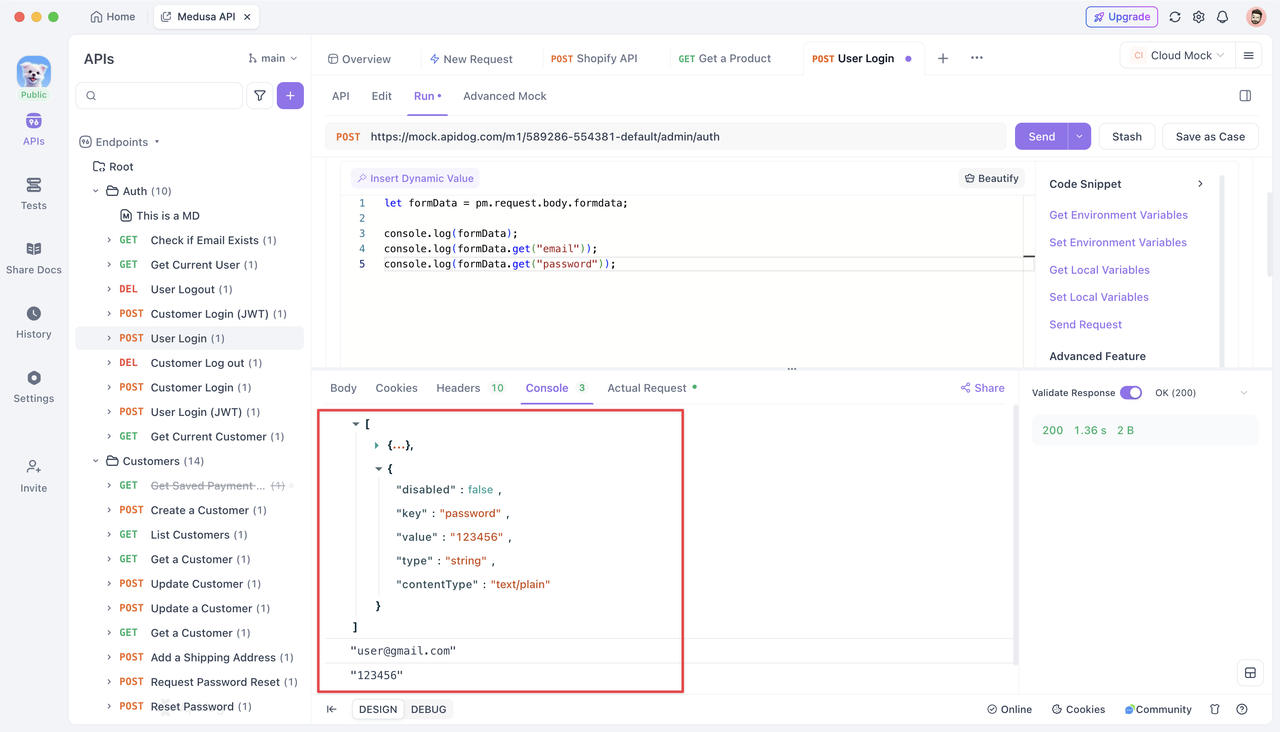
- For x-www-form-urlencoded format: Similar to form-data, x-www-form-urlencoded also uses key-value pairs. To obtain these parameters, use
pm.request.body.urlencoded
:
// When body type is x-www-form-urlencoded
let urlencoded = pm.request.body.urlencoded;
// Print data to consoleconsole.log(urlencoded);
console.log(urlencoded.get("email"));
console.log(urlencoded.get("password"));
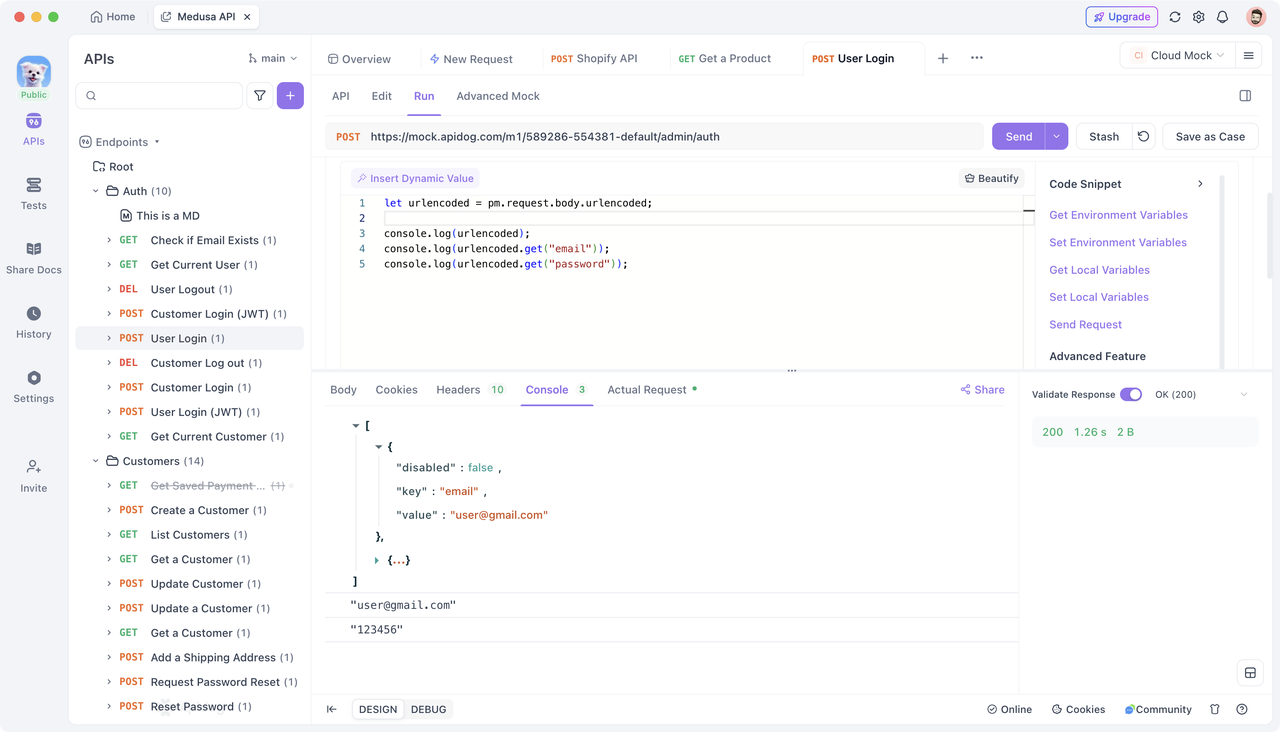
- For raw, JSON, or XML format: Use
pm.request.body.raw
to get the raw body content, returning a string. You’ll typically need to parse it to access specific parameters:
// For raw, JSON, or XML format
let formData = pm.request.body.raw;
// Parse to JSON object
let jsonData = JSON.parse(formData);
// Print data to console
console.log(jsonData);
console.log(jsonData.email);
console.log(jsonData.password);
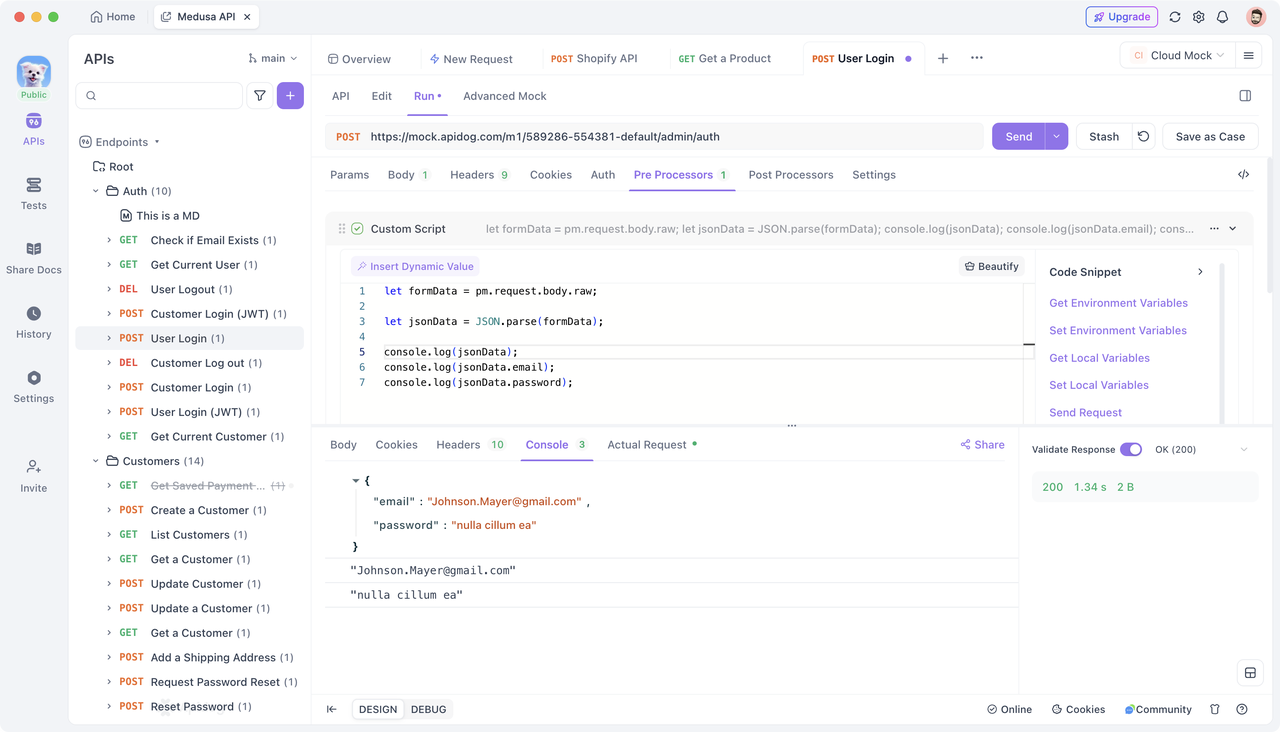
Retrieve Header Parameters
To retrieve the request's header parameters, use pm.request.headers
, which returns an array object containing all request header information. You can precisely locate and extract specific parameter values using the get()
function:
// Get request header parameters
let headers = pm.request.headers;
// Print data to consoleconsole.log(headers);
console.log(headers.get("Accept"));
FAQs of Retrieving Request Parameters
Why are referenced variables not being replaced?
If your API request parameters are referencing environment variable values but are not being replaced with specific values in the console, ensure that you move the "Variable Replacement & Inherit Parent" to the very start of your script in either the Pre-request or Post-request section. This ensures that all referenced variables (including dynamic values) are replaced with the actual contents.
Appendix
Methods and Descriptions
Method | Description | Purpose |
---|---|---|
pm.request | Get current interface's request parameter information | Access request object |
pm.request.getBaseUrl() | Get pre-request URL | Retrieve service address |
pm.request.baseUrl | Get pre-request URL (alternative method) | Retrieve service address |
pm.request.url.path | Get path parameters | Extract URL path parameters |
pm.request.url.query | Get query parameters | Extract URL query parameters |
pm.request.url.toString() | Get complete URL | Retrieve all parameters in complete URL |
pm.request.body.formdata | Get form-data body parameters | Extract form data |
pm.request.body.urlencoded | Get x-www-form-urlencoded body parameters | Extract URL-encoded form data |
pm.request.body.raw | Get raw, JSON, or XML body parameters | Extract raw request body content |
pm.request.headers | Get header parameters | Extract request header information |
JSON.parse() | Parse JSON string | Convert JSON string to JavaScript object |
Conclusion
This article has described how to extract request parameters in Apidog, including pre-request URLs, path parameters, query parameters, body parameters, and header parameters. By using the pm.request
object and its related methods, you can easily obtain and manipulate various request data.