Unlock the potential of RESTful API interaction through jQuery, an influential JavaScript library celebrated for its simplicity and effectiveness. This exploration delves into the realm of utilizing jQuery to call REST services, exploring its significance, practical implementation, and the instrumental role of Apidog in generating concise and efficient jQuery code for RESTful API requests.
Understanding RESTful APIs and jQuery:
REST (Representational State Transfer) is an architectural style for designing networked applications. jQuery, known for its swiftness and compact nature, extends its capabilities to simplify the process of making AJAX requests for RESTful API consumption. Our comprehensive article "What is RESTful API" provides in-depth information on RESTful API. If you're interested in learning more about it, be sure to give it a read.
Key features of jQuery for RESTful API calls include streamlined DOM operations, convenient Ajax communication access, easy integration of animations and effects, harmonization of browser differences, straightforward and comprehensible code, and a rich collection of plugins for high expandability.
The integration of jQuery facilitates the authoring of intricate JavaScript code, significantly boosting development efficiency. With its ability to reconcile browser differences and present clear code, jQuery stands as a valuable library extensively employed in web front-end development.
Why Choose jQuery for REST API Calls?
jQuery emerges as the preferred tool for calling RESTful APIs due to its concise and user-friendly syntax for making AJAX requests. This characteristic appeals to developers by simplifying the process of interacting with RESTful APIs. Additionally, jQuery's cross-browser compatibility ensures uniform behavior across various browsers, eliminating the need for developers to create separate code for each browser—a time-saving and compatibility-enhancing feature.
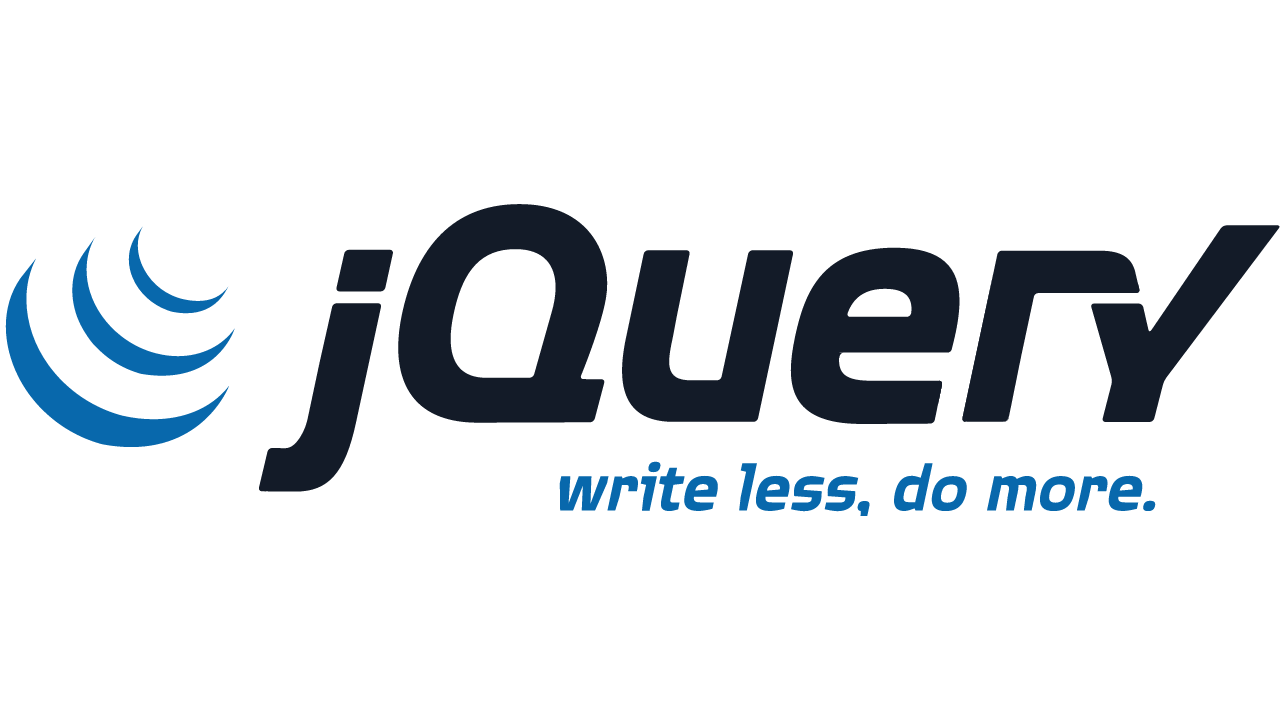
The simplicity of jQuery is a key advantage in RESTful API calls, reducing the amount of code required for such requests. This streamlined approach enhances development efficiency, enabling developers to achieve results with fewer lines of code. Moreover, jQuery abstracts away the complexities of AJAX, making it accessible to developers of all experience levels. This abstraction allows developers to focus on the core functionality of their applications without delving into the intricacies of AJAX implementation.If you want to learn more about How to Send API Requests Using jQuery, check out our detailed article.
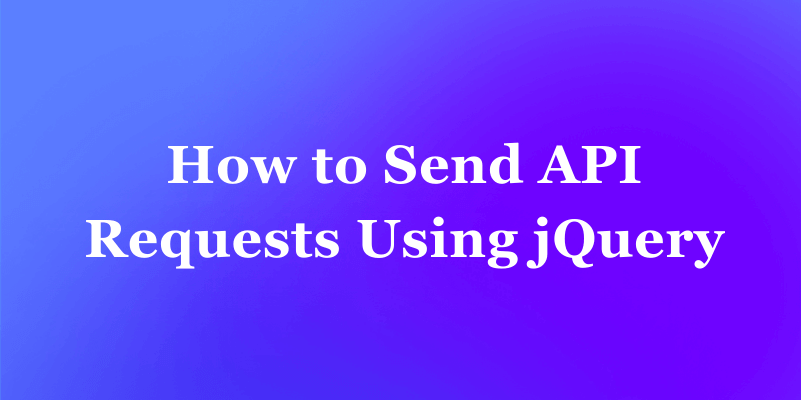
How to Employ jQuery for RESTful API Requests
jQuery offers a simple and efficient method for sending RESTful API requests from your web application. This tutorial guides you through the process of using jQuery to perform AJAX requests on a RESTful API endpoint. By the end of this tutorial, you will have a clear understanding of how to utilize jQuery for seamless interaction with RESTful APIs and handling responses.
Step 1: Include jQuery Library
Ensure the inclusion of the jQuery library in your HTML file by adding the following line within the  tags:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Step 2: Write the AJAX Request
Utilize jQuery's AJAX function to send RESTful API requests. The AJAX function enables asynchronous HTTP requests to the server. Here's an example of using the AJAX function to send a GET request to a RESTful API endpoint:
$.ajax({
url: 'https://api.example.com/data',
method: 'GET',
success: function(response) {
// Handle the API response here
console.log(response);
},
error: function(xhr, status, error) {
// Handle errors here
console.error(status, error);
}
});
Step 3: Customize the Request
Customize the AJAX request by specifying parameters such as the request method, data to be sent, headers, and more. For example, to send data with a POST request, include a data parameter in the AJAX call.
Step 4: Handle the API Response
Within the success function of the AJAX call, handle the API response. Process the data returned by the API and update your web page accordingly.
By following these steps, you can leverage jQuery to send RESTful API requests and manage responses in your web application. Replace 'https://api.example.com/data' with the actual URL of the RESTful API endpoint and customize the AJAX call according to your API's requirements.
Use apidog to generate jQuery code
Apidog proves to be a robust tool for automatically generating business code in various languages and frameworks, including jQuery, for RESTful API requests. This feature becomes especially handy when dealing with API interactions. Apidog simplifies the process by generating jQuery code snippets, saving time, and minimizing the risk of errors. This makes Apidog an indispensable asset for developers aiming to streamline their workflow and enhance efficiency.
Step 1: Open Apidog
To begin, launch Apidog and go to the "API Requests" section. From there, choose the API endpoint you wish to interact with and then click on the "Generate Code" button.
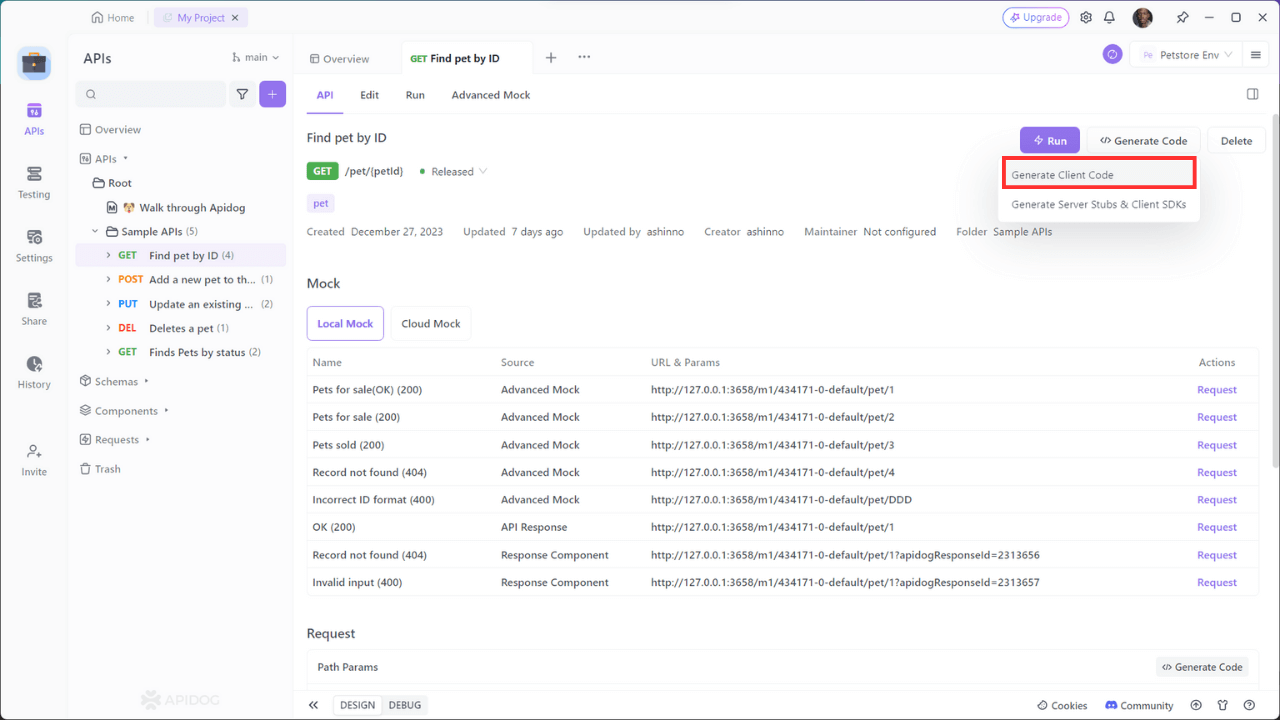
Step 2: Select jQuery as the desired programming language.
Once selected, Apidog will generate the corresponding jQuery code snippet for the API request you have chosen.
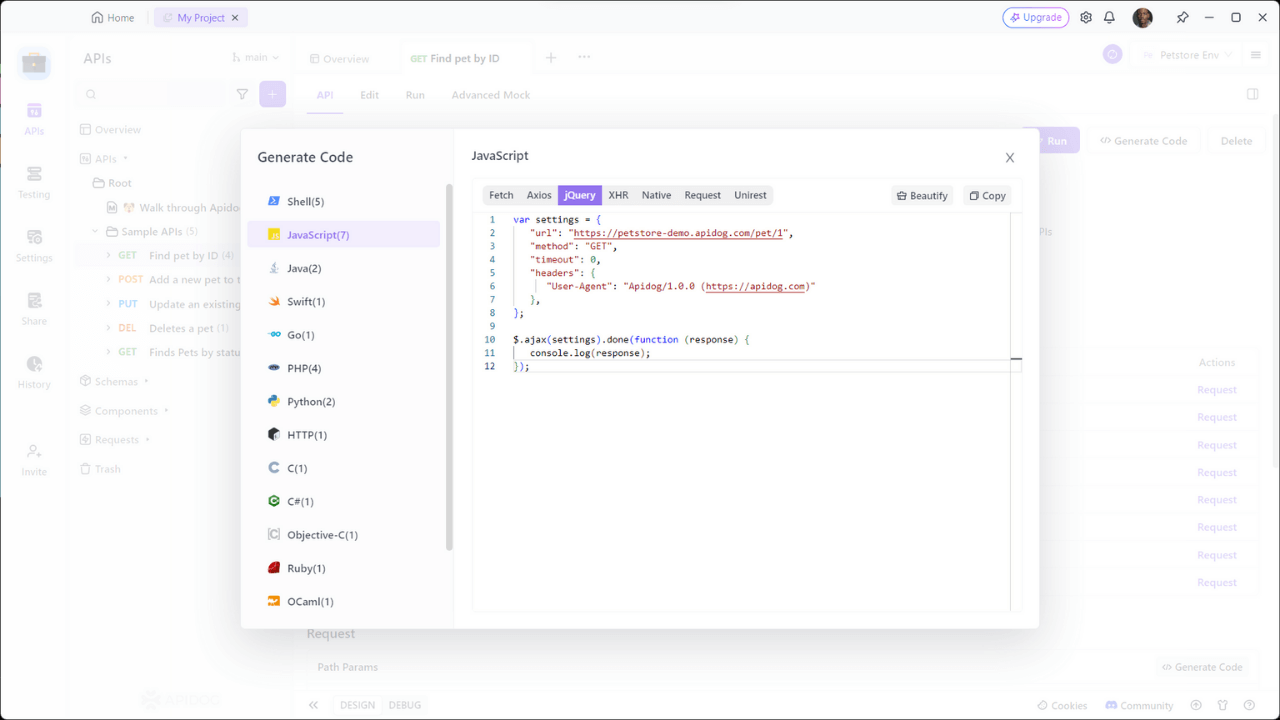
Making RESTful API Requests
In modern web development, interacting with RESTful APIs is a common task. One popular method for making API requests in JavaScript is using jQuery's AJAX function and the Fetch API. Â We'll explore how to make a simple GET request using jQuery's AJAX function.
var settings = {
"url": "https://petstore-demo.apidog.com/pet/1",
"method": "GET",
"timeout": 0,
"headers": {
"User-Agent": "Apidog/1.0.0 (https://apidog.com)"
},
};
$.ajax(settings).done(function (response) {
console.log(response);
});
Using jQuery AJAX simplifies the process of making RESTful API requests in JavaScript. The provided settings object allows customization of various aspects of the request, and the callback functions, such as .done()
, make it easy to handle responses.
Remember to adapt the code according to your specific API and project requirements. Test the code thoroughly and handle errors appropriately for a robust implementation. jQuery AJAX remains a reliable and widely used approach for making API requests in web development.
Conclusion
Mastery of RESTful API requests with jQuery opens doors to efficient and seamless communication with APIs. jQuery's user-friendly nature, broad cross-browser support, and ability to abstract complexities make it an ideal choice for developers at all levels of experience.
The integration of Apidog enhances the jQuery API request process, empowering developers to effortlessly generate code and maintain consistency across their projects. By harmonizing jQuery and Apidog, developers can elevate their capabilities in API communication, resulting in more efficient, sustainable, and error-resistant code within an intuitive interface.
Pre-testing APIs through Apidog and utilizing the code generation workflow enables the rapid construction of robust API call implementations. This approach enhances efficiency, facilitating the seamless integration of RESTful APIs into web applications and enabling developers to deliver stable and reliable solutions within a shortened timeframe.