In today's fast-paced digital world, APIs (Application Programming Interfaces) are the unsung heroes, enabling seamless communication between software applications. Among the different types of APIs, REST APIs are particularly popular due to their simplicity and scalability. Whether you're a developer, a tech enthusiast, or someone curious about how the web works, understanding REST APIs is invaluable.
In this comprehensive guide, we'll explore the magic of REST APIs with practical examples, and introduce you to Apidog, a fantastic tool that simplifies working with APIs. So, buckle up, and let's dive into the world of REST APIs!
What is a REST API?
Before we delve into the examples, let's get our basics right. REST (Representational State Transfer) is an architectural style for designing networked applications. It relies on a stateless, client-server, cacheable communications protocol — the HTTP. Here’s why REST APIs are a big deal:
Stateless: Each request from a client to a server must contain all the information needed to understand and process the request. This means the server does not store any client context between requests, making REST APIs highly scalable.
Client-Server: The client and server are independent of each other, allowing them to evolve separately. This separation of concerns simplifies the development and deployment process.
Cacheable: Responses from the server can be cached to improve performance. REST APIs define themselves to be cacheable to avoid unnecessary load on the server.
Why Use REST APIs?
Now, you might wonder, "Why should I care about REST APIs?" Here’s why:
- Interoperability: REST APIs allow different systems to communicate with each other, regardless of their underlying architecture. This makes them perfect for integrating diverse applications.
- Scalability: Due to their stateless nature, REST APIs can handle multiple requests without storing the client state, making them suitable for large-scale applications.
- Flexibility: They can handle various types of calls, return different data formats, and even change structurally with the correct implementation of versioning.
A Gentle Introduction to Apidog
Before we jump into the nitty-gritty of REST API examples, let’s talk about Apidog. If you're new to APIs or even if you're an experienced developer, Apidog can be a game-changer. It’s a powerful tool that simplifies creating, testing, and managing APIs.
Getting Started with REST API Examples
To understand REST APIs better, let's go through some common examples. These will help you see how REST APIs work in real-world scenarios.
Example 1: Getting User Information
Let's start with a simple example – fetching user information. Imagine you have a user database, and you want to retrieve information about a specific user.
Request:
GET /users/{id}
Host: example.com
Response:
{
"id": 1,
"name": "John Doe",
"email": "john.doe@example.com"
}
In this example, the GET
method requests data from the server, and {id}
is a path parameter that specifies the user ID.
Example 2: Creating a New User
Next, let's see how you can create a new user.
Request:
POST /users
Host: example.com
Content-Type: application/json
{
"name": "Jane Doe",
"email": "jane.doe@example.com"
}
Response:
{
"id": 2,
"name": "Jane Doe",
"email": "jane.doe@example.com"
}
In this case, the POST
method sends data to the server to create a new resource.
Example 3: Updating User Information
Updating existing user information is another common use case.
Request:
PUT /users/{id}
Host: example.com
Content-Type: application/json
{
"name": "Jane Smith",
"email": "jane.smith@example.com"
}
Response:
{
"id": 2,
"name": "Jane Smith",
"email": "jane.smith@example.com"
}
The PUT
method updates the existing resource identified by {id}
with the new data.
Example 4: Deleting a User
Finally, let's look at how to delete a user.
Request:
DELETE /users/{id}
Host: example.com
Response:
{
"message": "User deleted successfully"
}
The DELETE
method removes the resource identified by {id}
.
Advanced REST API Examples
Now that we've covered the basics, let's explore some advanced examples.
Example 5: Filtering and Sorting Data
Often, you need to filter and sort data. Let's see how you can achieve this.
Request:
GET /users?age=25&sort=name
Host: example.com
Response:
[
{
"id": 3,
"name": "Alice",
"age": 25
},
{
"id": 4,
"name": "Bob",
"age": 25
}
]
In this example, the query parameters age=25
and sort=name
filter users aged 25 and sort them by name.
Example 6: Pagination
For large datasets, pagination is essential. Here's how you can implement it.
Request:
GET /users?page=2&limit=10
Host: example.com
Response:
{
"total": 50,
"page": 2,
"limit": 10,
"data": [
// Array of users
]
}
The query parameters page=2
and limit=10
specify the page number and the number of items per page.
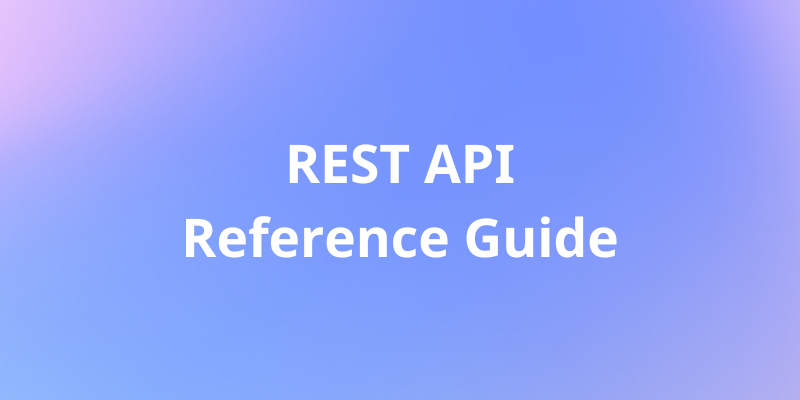
Best Practices for REST APIs
To make your REST APIs efficient and maintainable, follow these best practices:
- Use Proper HTTP Methods: Use
GET
for retrieving data,POST
for creating,PUT
for updating, andDELETE
for deleting. - Keep It Stateless: Ensure each request contains all the necessary information.
- Use Meaningful URIs: URIs should be intuitive and follow a consistent naming convention.
- Version Your APIs: Use versioning to manage changes and maintain backward compatibility.
- Implement Rate Limiting: Protect your APIs from abuse by limiting the number of requests.
- Provide Detailed Error Messages: Help users debug issues with clear and informative error messages.
Testing Your REST APIs with Apidog
Testing is a crucial part of API development. With Apidog, you can easily create, test, and debug your APIs. Here’s how:
How to Integrate REST API with Apidog
In the world of modern software engineering, API development has become a crucial aspect, and REST APIs are among the most commonly used types of web API. But, testing and documenting REST APIs can be a daunting and time-consuming task, especially when dealing with numerous endpoints.
This is where Apidog comes into play - an API testing and documentation tool that simplifies the process of testing and documenting REST APIs. With Apidog, developers can effortlessly test REST APIs, generate API documentation, and work together with team members on API development.
Integrating a REST API with Apidog involves a few basic steps. Here's a detailed step-by-step process to integrate REST API with Apidog:
The first step is navigating to the Apidog website and signing up for a free account.
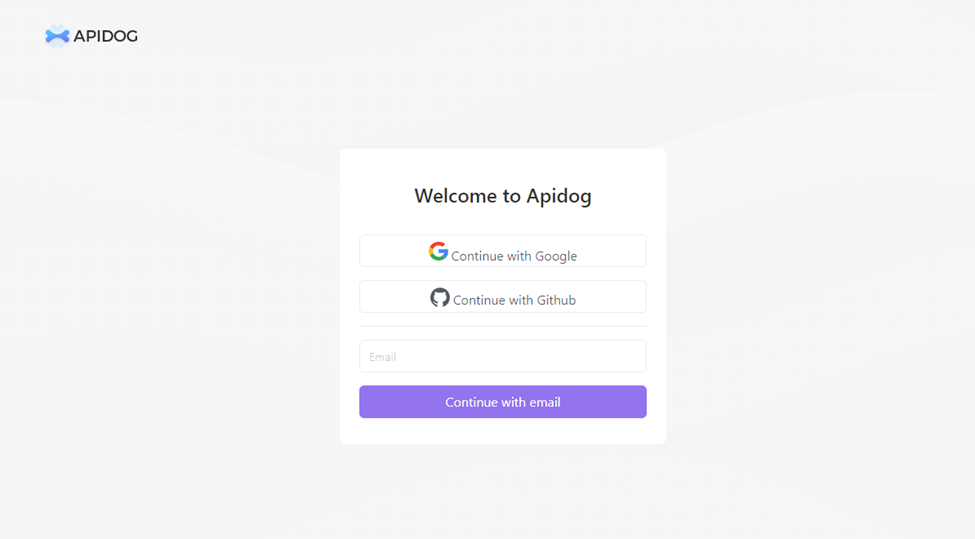
2. Click on "New Project" and give your project a name.
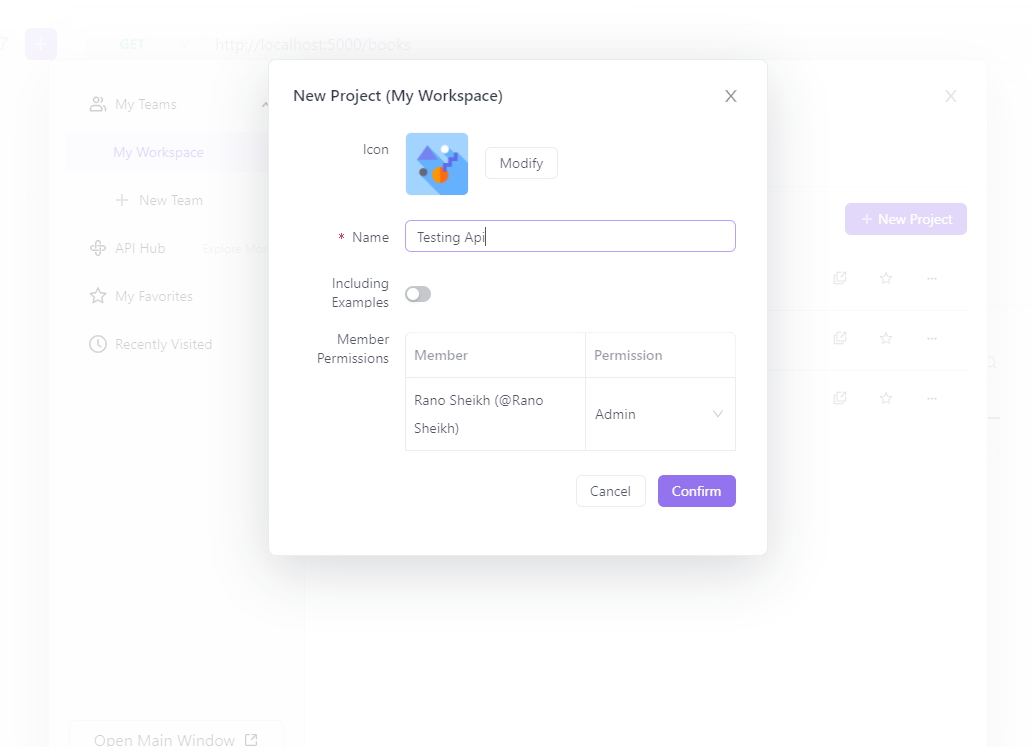
3. Create a New API.
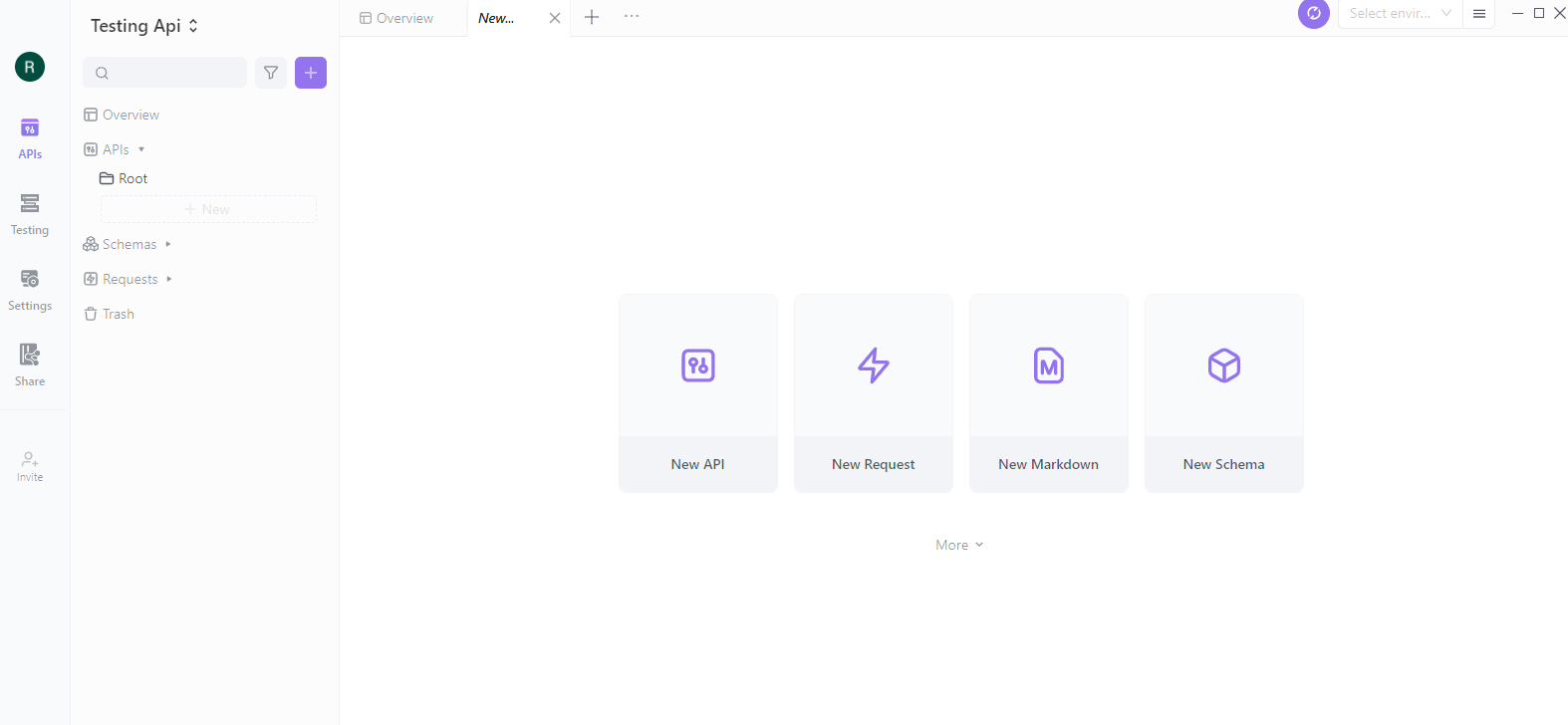
4. Now click on the "Add Endpoint" button and fill in the following details for the "Get all books" endpoint: In this case,
URL: http://localhost:5000/books
Method: GET
Endpoint name: Get all books
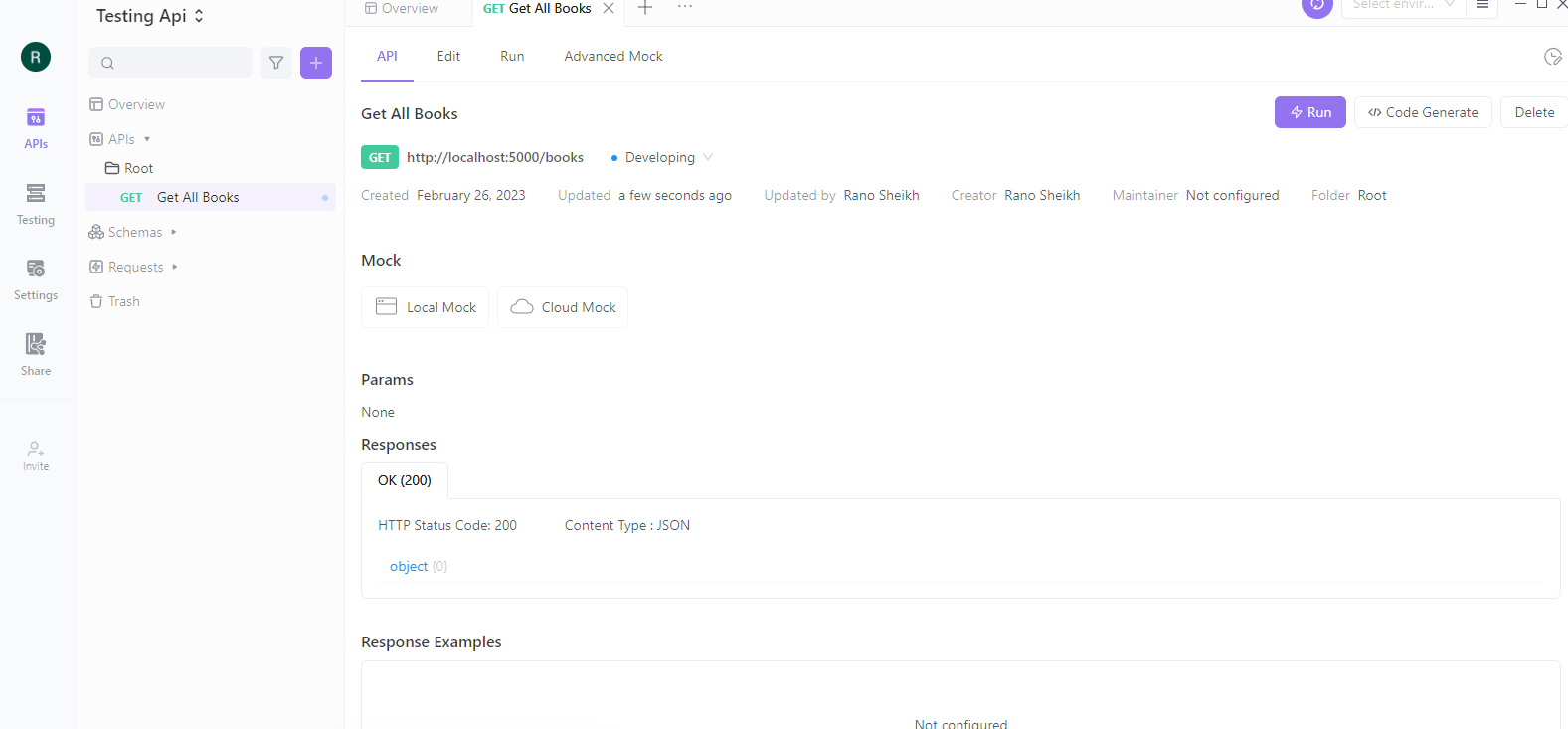
5. Specify any query parameters or headers that your endpoint may require by clicking on the "Add parameter" or "Add header" buttons.
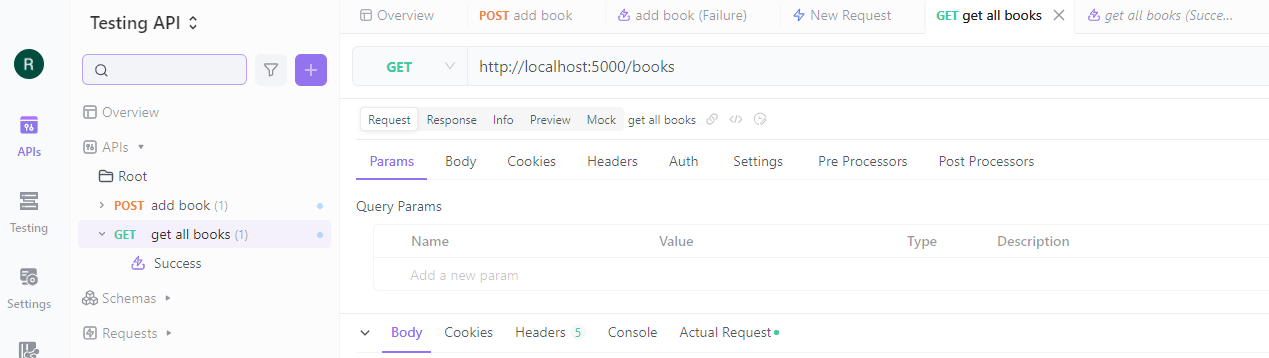
6. Click on the "Send" button to test your endpoint and ensure that it is working properly. Once your endpoint is working as expected, click on the "Save APICase" button to add it to your Apidog project.
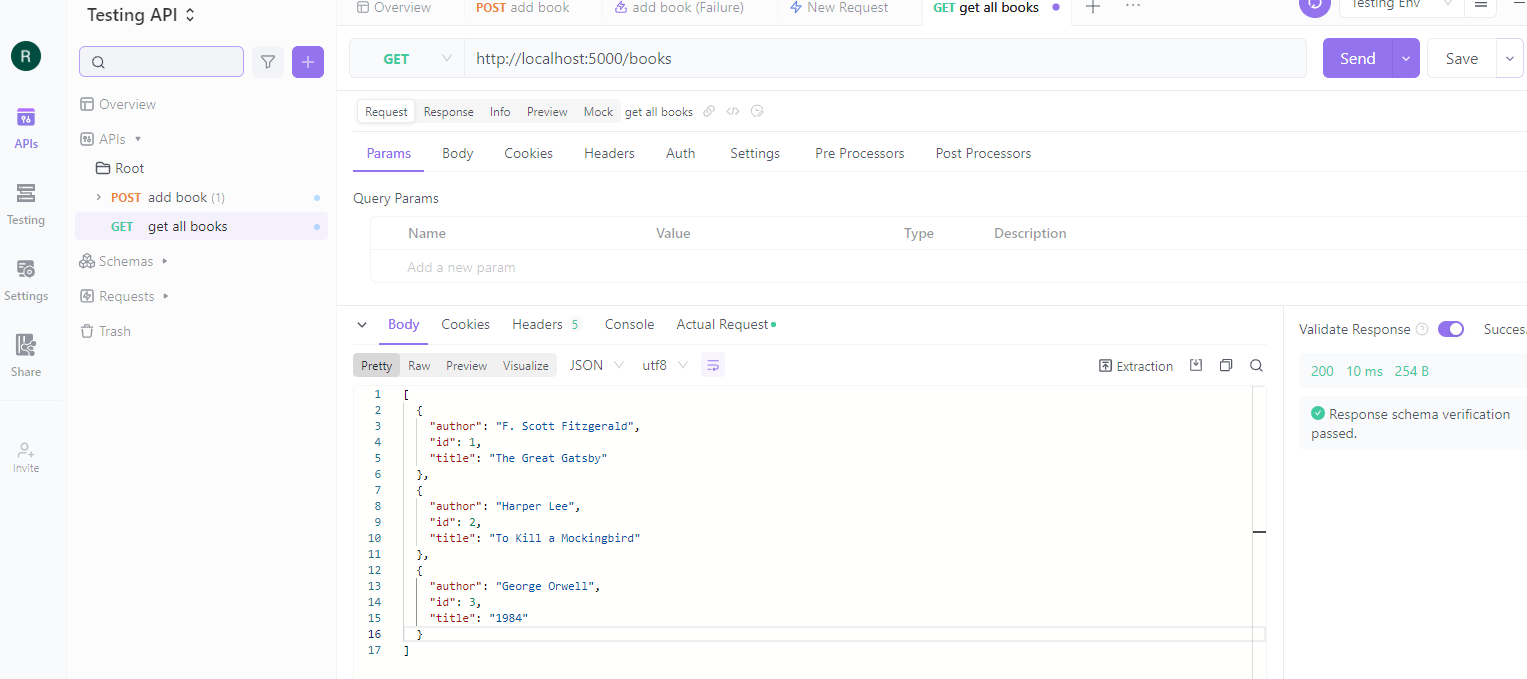
7. You can now use Apidog to test your endpoint and generate documentation for your Flask API.
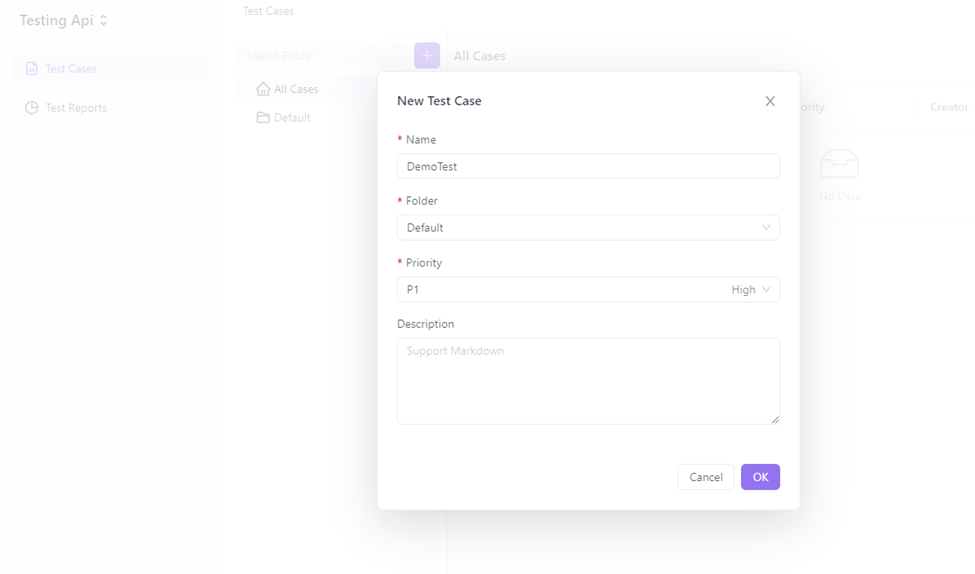
8. Define the test steps of your test case and select the endpoints you want to include in testing. Customize the Test Cases as per your needs.
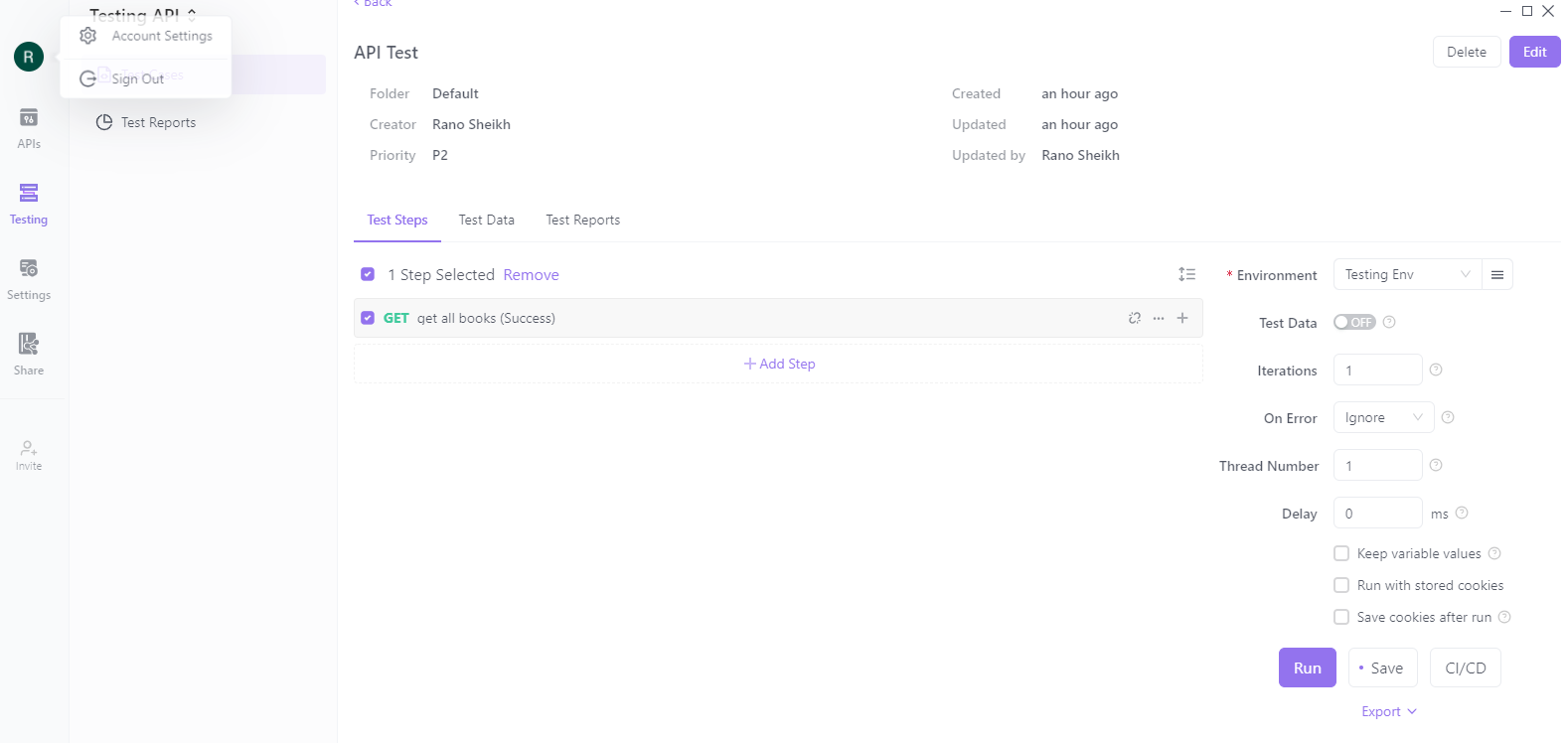
9. Once you test the cases, you can publish them on the web or export them to a PDF or Markdown file.
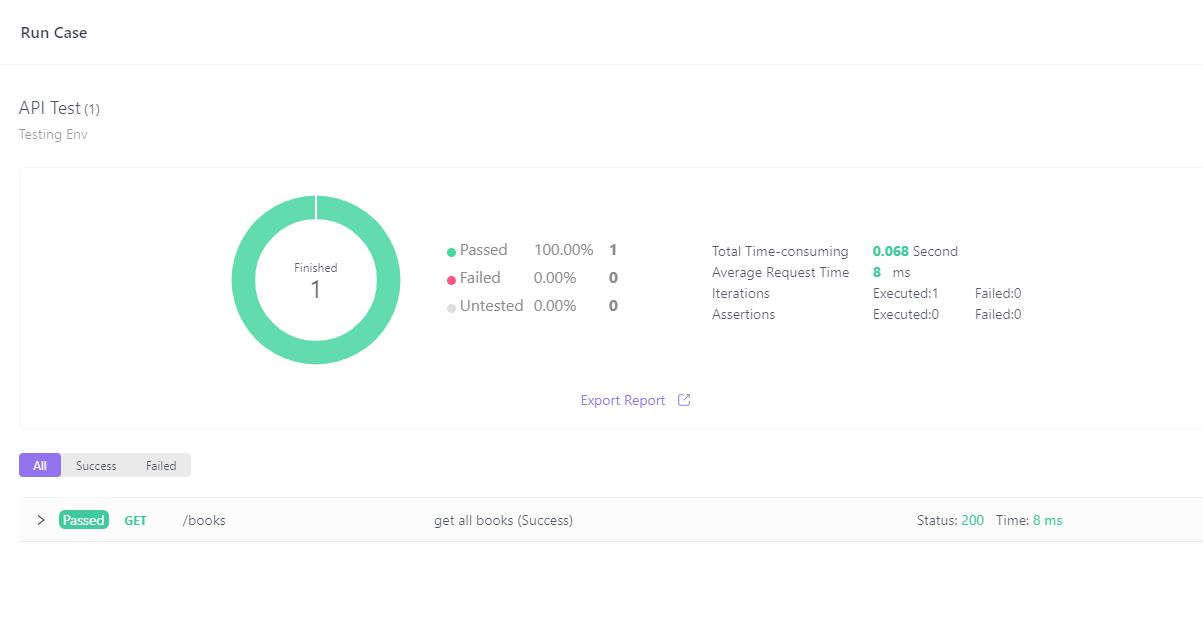
Apidog offers numerous customization options to assist users in utilizing and testing their APIs in accordance with their specific requirements.
API Challenges and Apidog Solutions
APIs (Application Programming Interfaces) are an essential part of modern software development, but they come with their own set of challenges. Here are some common API challenges and how API Dog covers them:
API Documentation: One of the biggest challenges in working with APIs is documentation. API documentation is critical for developers who want to integrate with an API, but it can be time-consuming to create and maintain. API Dog provides an easy way to create and maintain API documentation by automatically generating it based on your API's code. This means that developers can always have up-to-date documentation without having to spend time writing and updating it manually.
API Testing: Another major challenge in working with APIs is testing. Testing an API can be complex, as there are often multiple endpoints and parameters to test. API Dog makes it easy to test your API by providing a built-in testing tool. This tool lets you quickly and easily test your endpoints and view the response. You can also use the testing tool to simulate different scenarios and test edge cases.
API Security: API security is a critical consideration for any API. APIs can be vulnerable to attacks, such as SQL injection and cross-site scripting (XSS). API Dog provides various security features to help you secure your API, such as rate limiting, access control, and API keys. With API Dog, you can easily add security measures to your API to protect it from malicious attacks.
API Versioning: As APIs evolve, it's essential to have a versioning strategy in place to ensure backward compatibility. API Dog allows you to version your API easily by providing a versioning system that lets you define different versions of your API and manage them separately. This makes it easy to introduce new features without breaking existing integrations.
API Analytics: Analyzing API usage and performance is essential for improving the user experience and identifying areas for optimization. API Dog provides built-in analytics that allows you to monitor API usage and performance. With API Dog, you can see how many requests your API is receiving, which endpoints are the most popular, and how long each request takes to process.
Conclusion
REST APIs are the backbone of modern web applications, enabling seamless interaction between different systems. By understanding and implementing REST APIs effectively, you can build robust, scalable, and efficient applications.
Don't forget to check out Apidog to streamline your API development process. It’s an invaluable tool that can save you time and effort.