When you're diving into the world of web development and APIs, one essential tool in your toolkit is Python's requests library. In this post, we’re going to explore a powerful feature within this library: handling cookies. We’ll break down what cookies are, why they matter, and how to manage them effectively using Python's requests library.
What Are Cookies?
Cookies are small pieces of data stored on the client side that servers use to remember information about the user. They are crucial for maintaining sessions, storing user preferences, and tracking user behavior across sessions. When you interact with an API, cookies can help maintain the state between requests.
Why Cookies Matter in API Requests
Imagine you're building a web application that interacts with an API to fetch user data. When the user logs in, the server sets a cookie to keep the session active. Every subsequent request the user makes needs to include this cookie to authenticate the user. Without cookies, the user would need to log in with every request, which is far from ideal.
Getting Started with Python Requests
Python's requests
library is a powerful and user-friendly tool for making HTTP requests. It simplifies the process of sending HTTP requests and handling responses, making it an indispensable part of any Python developer’s toolkit.
Installing the Requests Library
First, ensure you have the requests
library installed. You can install it via pip:
pip install requests
Making a Basic Request
Let’s start with a simple GET request:
import requests
response = requests.get('https://api.example.com/data')
print(response.json())
This code sends a GET request to the specified URL and prints the JSON response.
Handling Cookies with Requests
Now, let's get into the nitty-gritty of handling cookies. The requests
library makes it straightforward to manage cookies.
Retrieving Cookies from a Response
When you make a request, the server might send back cookies. You can access these cookies using the cookies
attribute of the response object:
response = requests.get('https://api.example.com/login')
cookies = response.cookies
print(cookies)
Sending Cookies with a Request
To send cookies with your request, you can pass a cookies
parameter to the request methods. This parameter can be a dictionary or a RequestsCookieJar
object.
cookies = {'session_id': '123456789'}
response = requests.get('https://api.example.com/user', cookies=cookies)
print(response.json())
Maintaining Sessions with Session Objects
Managing cookies across multiple requests can be cumbersome. Thankfully, requests
provides a Session
object that handles cookies automatically.
session = requests.Session()
# Log in to the API and store the cookies
session.get('https://api.example.com/login', auth=('user', 'pass'))
# Make subsequent requests using the session
response = session.get('https://api.example.com/user')
print(response.json())
Using a Session
object, you don’t need to manually handle cookies between requests.
Advanced Cookie Handling
Custom Cookie Handling with RequestsCookieJar
If you need more control over your cookies, you can use the RequestsCookieJar
object. This allows you to manipulate cookies directly.
from requests.cookies import RequestsCookieJar
jar = RequestsCookieJar()
jar.set('session_id', '123456789', domain='api.example.com', path='/')
response = requests.get('https://api.example.com/user', cookies=jar)
print(response.json())
Deleting Cookies
Sometimes, you might need to delete cookies. You can do this by manipulating the RequestsCookieJar
object.
jar = response.cookies
jar.clear(domain='api.example.com', path='/', name='session_id')
response = requests.get('https://api.example.com/user', cookies=jar)
print(response.json())
Best Practices for Managing Cookies
- Security: Always ensure cookies containing sensitive information are handled securely. Use HTTPS to encrypt data in transit.
- Expiration: Be mindful of cookie expiration times. Implement proper handling for session expiration.
- Scope: Set appropriate domains and paths for your cookies to avoid unnecessary exposure.
Debugging Cookie Issues
When things don’t work as expected, debugging is crucial. Here are a few tips:
- Inspect Cookies: Print out cookie values and attributes to understand what’s being sent and received.
- Check Domains and Paths: Ensure cookies are being sent to the correct domain and path.
- Use Developer Tools: Browser developer tools can help you see what cookies are being set and sent during requests.
Streamlining API Development with Apidog
As you work with APIs and manage cookies, tools like Apidog can make your life significantly easier. Apidog is a powerful API development tool that simplifies the process of designing, testing, and documenting APIs.
Why Use Apidog?
- User-Friendly Interface: Apidog offers an intuitive interface for designing and testing APIs.
- Collaboration: Easily collaborate with team members on API development.
- Documentation: Automatically generate API documentation.
- Testing: Perform comprehensive API testing, including cookie management.
Using Apidog to Test Python Requests with Cookies
Apidog is a handy tool for testing and managing API requests, including those made with Axios.
Setup Your Apidog Environment
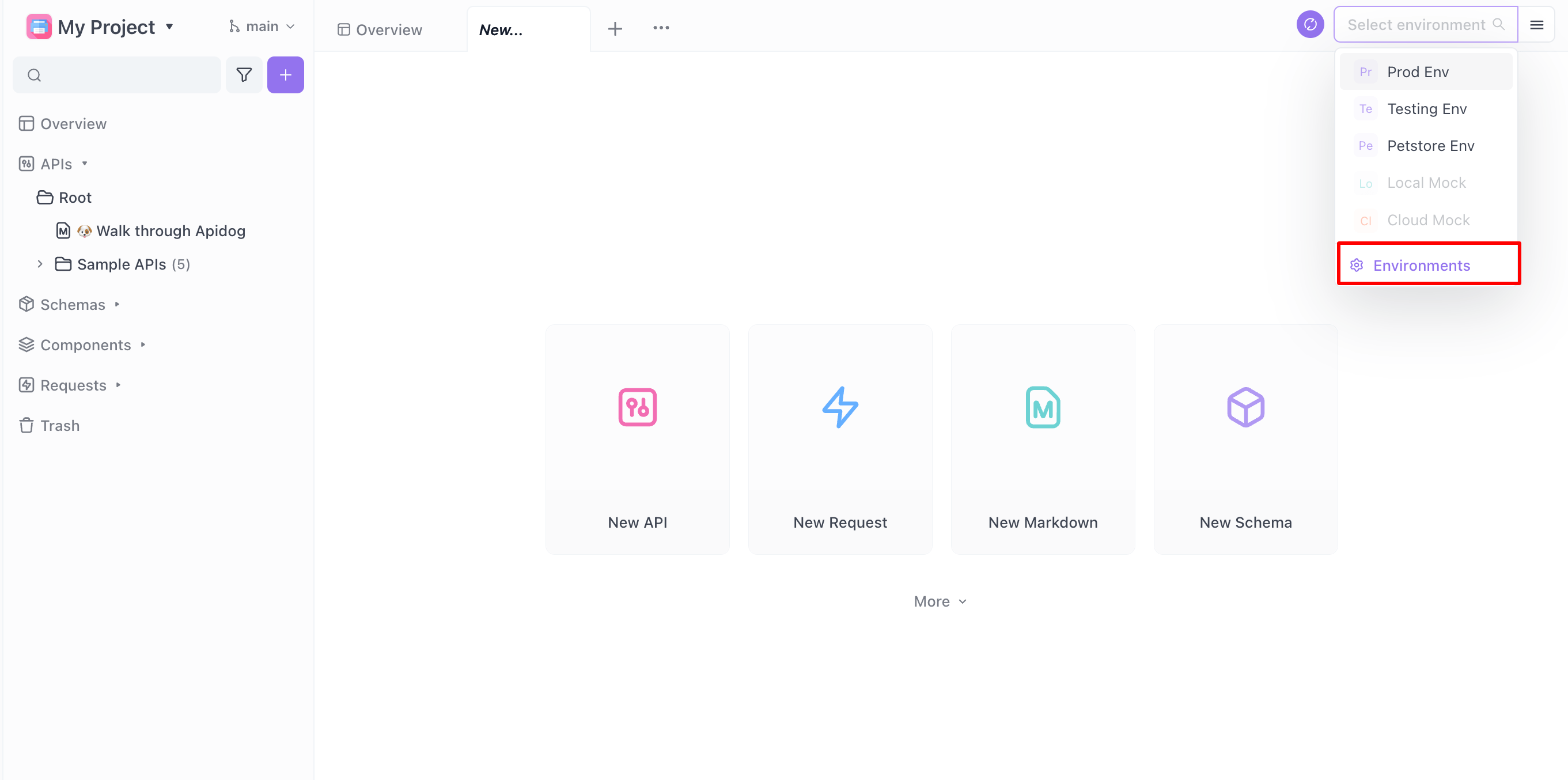
To initiate API testing using Axios and cookies with Apidog, setting up your testing environment is the first critical step. This setup will define the foundational parameters for your API interactions.
Initialize Apidog: Begin by launching Apidog and creating a new environment tailored to your project. This is your workspace for configuring all API testing settings.
Configure API Details: Input the base URL of your API in this environment. It serves as the root for all endpoint requests. Also, set any necessary headers or authentication tokens required by your API.
Creating a New Request
Creating a new request in Apidog involves specifying the type of request and the target API endpoint for testing.
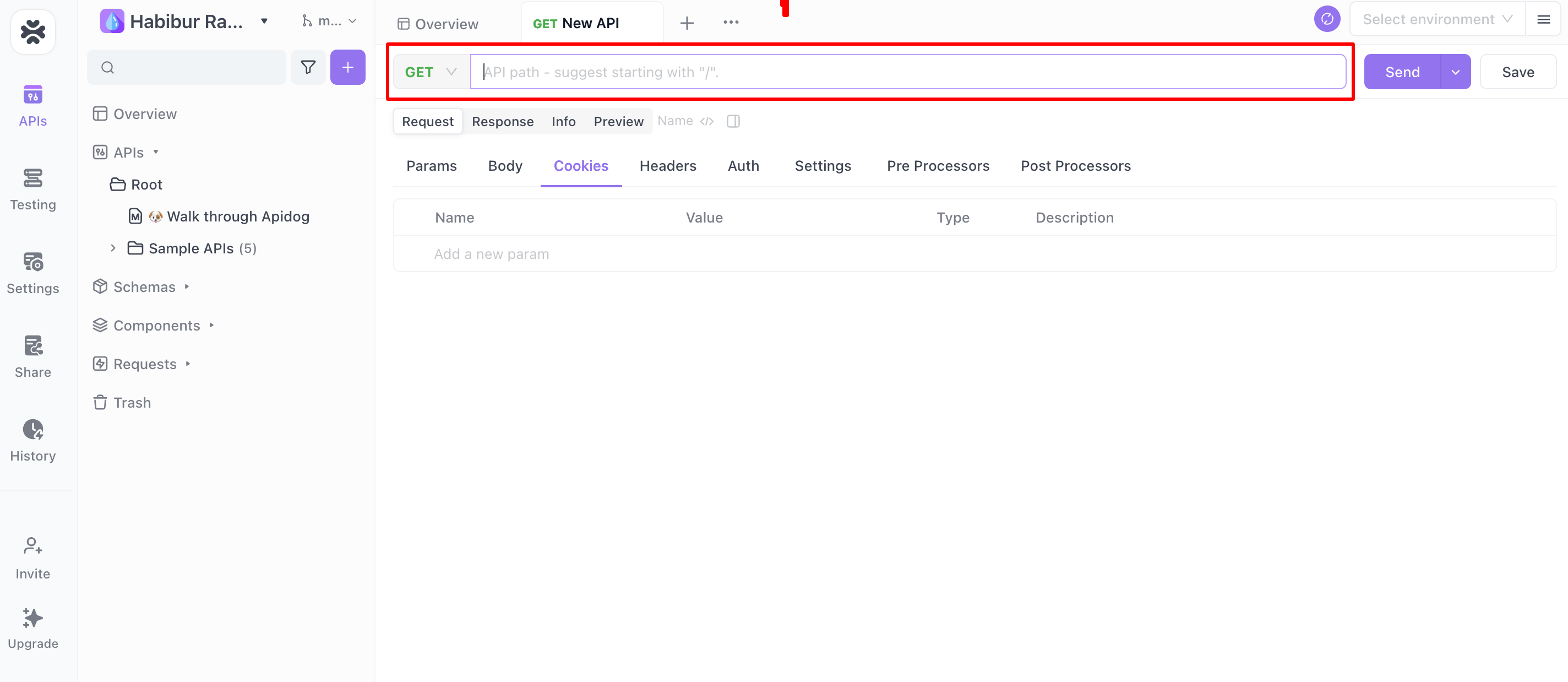
Create Request: In Apidog, establish a new API request, selecting 'GET' as the type if you’re starting with basic requests.
Input Endpoint: Enter the complete URL for the API endpoint you intend to test. This URL directs Apidog where to send your request.
Adding Cookies
Accurate cookie management is essential for effective API testing. In Apidog, the approach to handling cookies varies based on your testing environment.
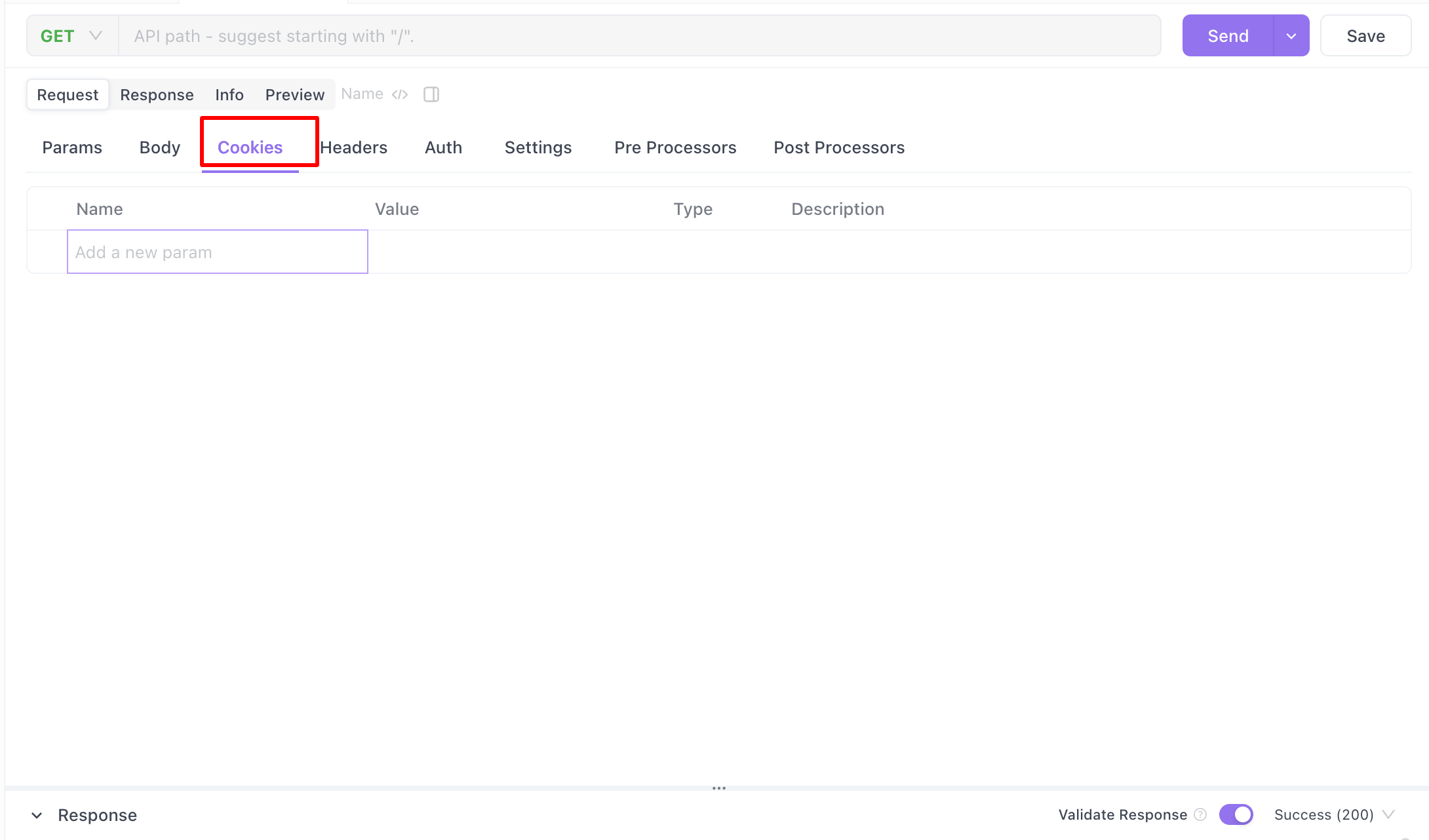
Browser Testing: When testing within a browser, Apidog automatically manages cookies, attaching them as necessary to your requests.
Best Practices for API Cookies
To ensure you're using API cookies effectively and securely, here are some best practices:
- Always Secure Sensitive Data: Avoid storing sensitive information like passwords in cookies. Use cookies to store tokens or session identifiers instead.
- Regularly Rotate Tokens: If you're using tokens in cookies, consider rotating them regularly for added security. This helps mitigate the risk of compromised tokens.
- Provide Clear Privacy Policies: Inform users about the use of cookies and their purpose on your website or application. This transparency is essential for compliance with data protection regulations.
- Regularly Audit Cookies: Periodically review and audit the cookies used in your applications to ensure they align with best practices and comply with regulations.
- Consider Privacy Regulations: Be aware of and adhere to privacy regulations such as the General Data Protection Regulation (GDPR) and the California Consumer Privacy Act (CCPA) when dealing with user data and cookies.Best Practices for API Cookies
- To ensure you're using API cookies effectively and securely, here are some best practices:
- Always Secure Sensitive Data: Avoid storing sensitive information like passwords in cookies. Use cookies to store tokens or session identifiers instead.
- Regularly Rotate Tokens: If you're using tokens in cookies, consider rotating them regularly for added security. This helps mitigate the risk of compromised tokens.
- Provide Clear Privacy Policies: Inform users about the use of cookies and their purpose on your website or application. This transparency is essential for compliance with data protection regulations.
- Regularly Audit Cookies: Periodically review and audit the cookies used in your applications to ensure they align with best practices and comply with regulations.
- Consider Privacy Regulations: Be aware of and adhere to privacy regulations such as the General Data Protection Regulation (GDPR) and the California Consumer Privacy Act (CCPA) when dealing with user data and cookies.
Conclusion
Managing cookies in Python using the requests
library is a fundamental skill for any developer working with APIs. By understanding how to retrieve, send, and manage cookies, you can create more robust and user-friendly applications. Additionally, leveraging tools like Apidog can further enhance your development workflow, making it easier to design, test, and document your APIs.
Remember, cookies are essential for maintaining state and session management in web applications. Proper handling ensures a smooth user experience and secure communication between your application and the server.