Python API calls are powerful tools responsible for interactions between online services and applications. Essentially, they provide a set of instructions and rules for how you can request information or perform actions on the web service.
This article will let you in on why Python API calls are so important, and provide you with some code snippets for reference.
If you are interested in learning how to craft Python API calls, you can download Apidog first by pressing the button below! 👇 👇 👇
Before going straight into Python API calls, let's reinforce our knowledge of API calls.
Recapping What API Calls Are
There are slight technical differences between an API call and an API request that do not make them 100% the same.
API Call Attributes:
- Refers to the entire interaction between two programs using an API.
- Consistent elements such as a request being sent to an API, its processing on the server, and the response received.
- In a way, API calls are the full package - they are the entire conversations we can observe between programs.
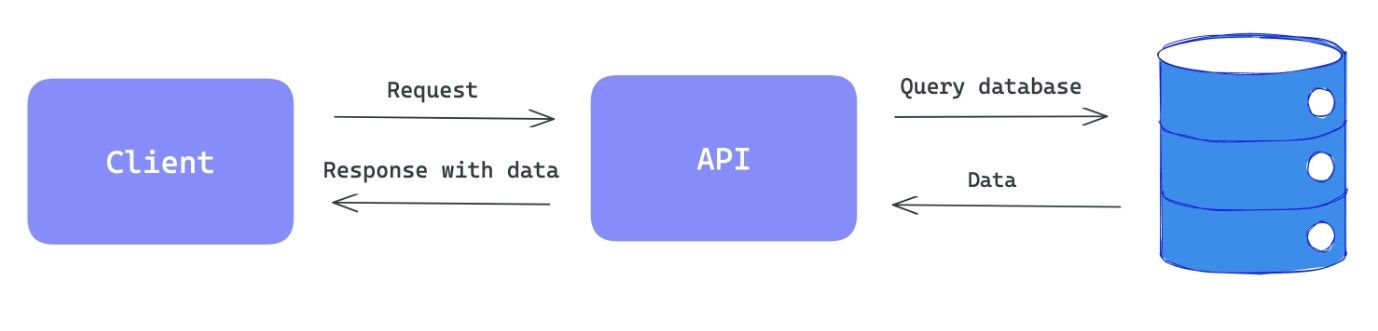
API Request Attributes:
- Refers to the initial message sent from the client program to the API server.
- API requests contain information such as HTTP method, data, and authentication details.
- You can think of API requests as being the questions or instructions sent to the server to start the conversation.
Now that we have discussed what API calls are, let's go into detail regarding Python API Calls
Python API Calls - An Interaction Process
Python API calls refer to the process of your Python code interacting with an API for exchanging information and performing actions.
Python API Call's Detailed Step-by-Step Process
- Code Initiation: Firstly, prepare the Python code to send a request to a specific API endpoint using libraries (such as
requests
andurllib
). - Sending the Request: This request specifies what you want from the API, for example:
HTTP Method:GET
methods can retrieve data, andPOST
methods can create something new.
Data: Additional information that is required for an action, like searching for a specific record, or filtering a database. Examples of additional information are search terms and user details. - Receive a Response: The API server receives and processes your request, then sends a response back to your code. This response typically includes:
Data: The requested information, like search results or a confirmation message.
Status code: Indicates whether the request was successful (e.g., 200 for OK) or encountered an error (e.g., 404 for Not Found). - Processing: Your Python code receives the response, and can use the data within your program.
Benefits of using Python API Calls
- Data Accessing and Functionality: APIs provide easy access to data and functionality from other programs, saving development time and effort. The possibilities of the functions that your Python app can do become quite virtually limitless.
- Combination of Services: Like the point above, you can connect different APIs by using Python API calls. You can create powerful Python applications with features beyond what a single program could offer.
- Automated tasks: Python API calls can automate repetitive tasks within your Python app, improving efficiency and reducing manual work.
Examples of Where Python API Calls Play a Big Role
- Weather App: Python API calls are required for retrieving real-time weather data from APIs such as OpenWeatherMap.
- Social Media Apps: You can rely on Python API calls to connect your app with other APIs like Twitter API to access data. You can therefore analyze what is trending, and create effective marketing campaigns.
- Online Science Databases: Creating a rich library full of information may rely on Python API calls to connect your app to numerous relevant scientific APIs. This may include the NASA API collection for providing genuine and reputable scientific information.
Code Sample of a Python REST API Call
Here is a code snippet of making a call to a Python REST API (Please do note that the code here should not directly be copy-pasted, as it is a structure to be followed and modified):
import requests
# Replace with your actual API endpoint URL, token, and data
api_url = "https://your-api.com/users"
api_token = "YOUR_BEARER_TOKEN"
user_data = {
"name": "John Doe",
"email": "johndoe@example.com",
"password": "strongpassword123"
}
# Set headers with bearer token
headers = {"Authorization": f"Bearer {api_token}"}
# Send POST request with JSON data
response = requests.post(api_url, headers=headers, json=user_data)
# Check for successful response
if response.status_code == 201:
# User created successfully, access response data
new_user = response.json()
print(f"User created: {new_user['name']} (ID: {new_user['id']})")
else:
# Handle error
print(f"Error creating user: {response.status_code} - {response.text}")
Code Explanation:
- Firstly, the code imports the
requests
library. - It then defines API details: Replace placeholders with your actual values.
- Next, it initializes the headers, which include the bearer token in the Authorization header.
- It sends over the POST request with
requests.post()
, along with the URL, headers, and JSON data. - It then checks for the response it receives. If successful (201 Created), access and print response data (new user details).
- In case it does not receive the right status code, it handles errors and proceeds to print the error status code and message.
Apidog - Making Python API Calls Was Never Easier!
Apidog is a design-oriented API development platform that allows users to generate various client languages within a few presses of buttons! Not only does it save precious time and effort, but also allows developers to worry no more about whether the code they use can function or not!
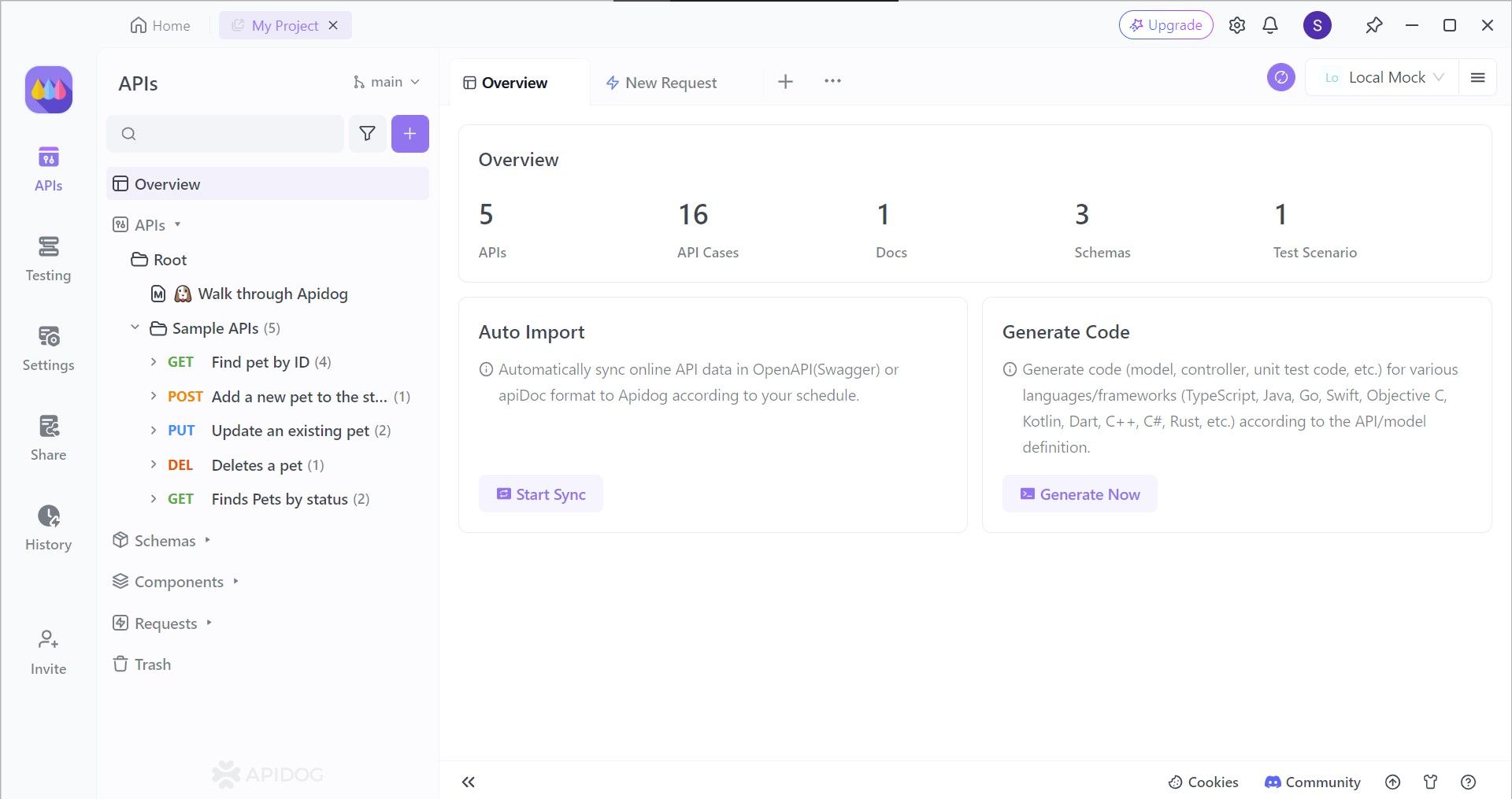
For you to connect your Python app, let's start by generating Python code to make a Python request, so you can initiate a Python API call.
Generating Python Code for a Request Using Apidog
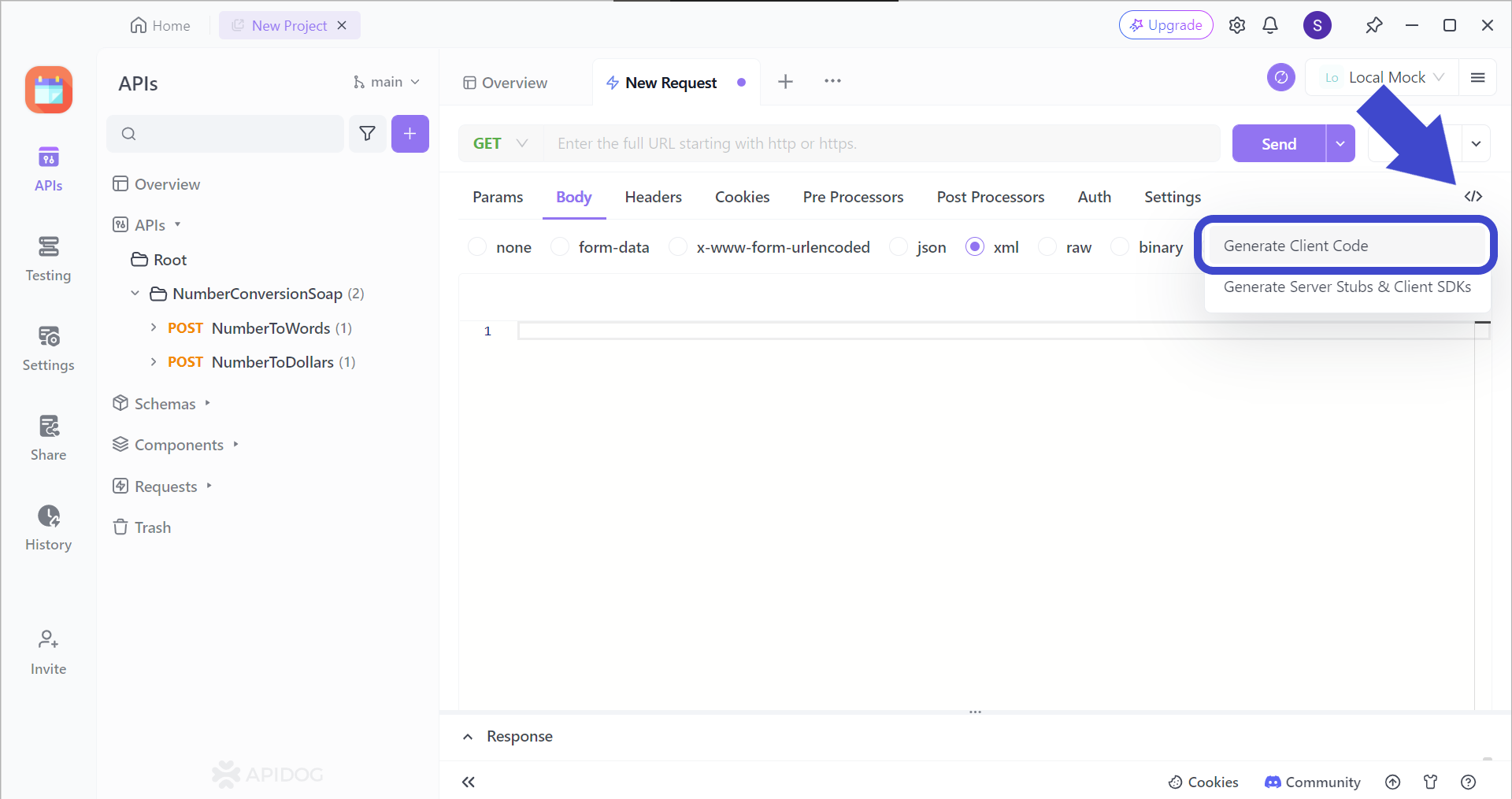
Firstly, locate this small </>
button found around the top right corner of the Apidog window.
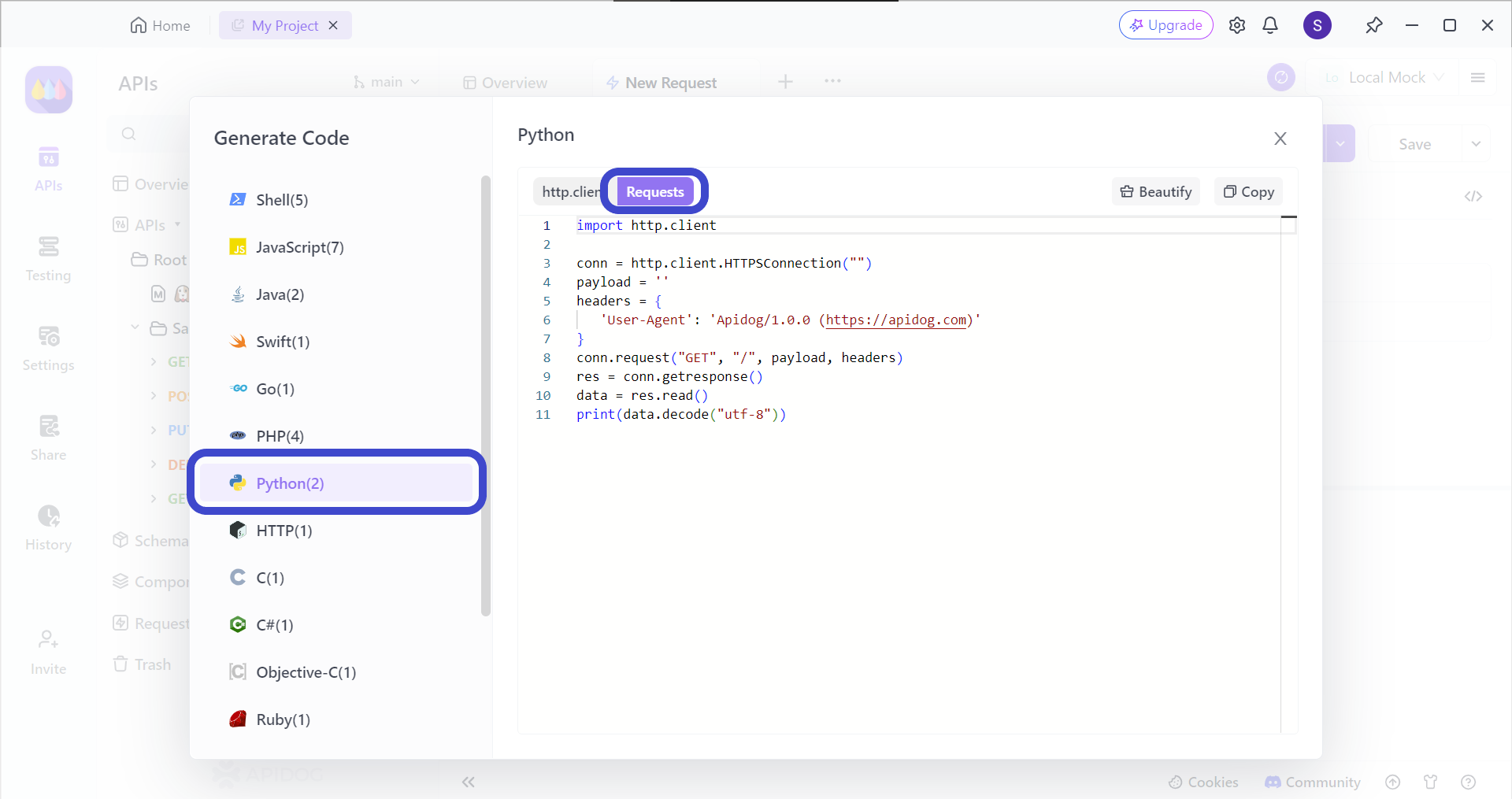
And there you have it! All you have to do is to add the code into your Python file, and add any customizations needed so that it can run!
Conclusion
To start learning how to make Python API calls, ensure that you have the necessary software and libraries downloaded on your device. Python API calls are very helpful assets to application developers, as they are enabled to connect to various sorts of services and databases. With Python API calls, you can create anything with your imagination!
Aside from just client code generation, Apidog can also create Python POST requests. Since Apidog is an all-in-one API development tool, you can build, test, mock, and debug your API, or even modify other developers' APIs that you think are interesting or cool. Quickly try out Apidog today - it is free of charge!