In the realm of API development, thorough testing is crucial for ensuring functionality and reliability. Postman, a popular API client, offers robust testing capabilities through its scripting feature.
Apidog is an all-in-one API development tool that provides developers with all the necessary tools for the entire API lifecycle. This removes the need for other applications.
To learn more about Apidog, make sure to click the button below! 👇
This article delves into practical examples of Postman test scripts, providing developers with a clear understanding of how to leverage this functionality for effective API validation.
Postman Test Scripts Roles
Validating API Responses
- Status Codes: Â Test scripts verify if the API responds with the expected status code. For instance, ensuring a successful request returns a 200 OK or handling error codes like 404 Not Found.
- Response Body: Scripts can delve deeper into the response body to ensure it contains the desired data. Â This could involve checking for the presence of specific fields in JSON responses, validating the data format (e.g., numbers, strings), or verifying the structure of the response object.
- Data Integrity: Scripts can confirm the accuracy of response data by comparing it with expected values. This can involve comparing against hardcoded values, environment variables, or calculations based on request parameters.
Handling Errors and Edge Cases
- Error Responses: Scripts can handle error scenarios by checking for specific error codes in the response and verifying if the error message aligns with expectations.
- Edge Cases:  Tests can be designed to simulate unusual conditions and ensure the API behaves as intended—for example, testing behavior with empty requests, invalid data types, or exceeding defined limits.
Automating Repetitive Tasks
- Streamlining Workflows: Scripts can automate repetitive testing tasks, saving developers valuable time and effort. These might include sending multiple requests with different parameters or repeatedly checking for specific data points in responses.
- Increasing Consistency: Scripts guarantee consistent testing procedures throughout development, reducing the risk of human error and ensuring comprehensive coverage.
Enhancing Maintainability and Collaboration
- Reusable Scripts: Once written, scripts can be reused across different API requests and collections, promoting code reuse and reducing maintenance overhead.
- Improved Collaboration: Scripts can be shared and documented within Postman workspaces, enabling teams to collaborate on API testing efforts.
Simplifying Complex Validations
- Leveraging Assertions: Postman scripting utilizes libraries like Chai for powerful assertions. Â These allow for complex data comparisons, logical checks, and string manipulations within the scripts.
- Custom Logic: Scripts can be tailored to implement specific validation rules or calculations based on the API's functionality. This adds a layer of flexibility and control to the testing process.
Benefits of Using Postman Test Scripts
Increased Efficiency and Automation
- Reduced Manual Testing: Â Scripts automate repetitive testing tasks, freeing developers to focus on more complex aspects of API development. This translates to faster development cycles and improved resource allocation.
- Improved Test Coverage: Scripts can be designed to test a wider range of scenarios compared to manual testing, leading to more comprehensive API validation.
- Reduced Time Investment: Automating tests saves developers significant time previously spent on manual execution and verification.
Enhanced API Reliability and Maintainability
- Early Detection of Issues: Scripts catch errors and inconsistencies in the API's behavior early in the development lifecycle, allowing for prompt fixes and preventing issues from reaching production.
- Consistent Testing: Scripts ensure consistent test procedures across development stages, reducing the risk of regressions and ensuring the API functions as intended throughout its lifecycle.
- Improved Code Quality: Automated tests promote a focus on writing clean and well-structured API code, leading to a more reliable and maintainable codebase.
Increased Developer Confidence
- Reliable API Behavior: Â Automated validation ensures the API behaves consistently and adheres to expectations, boosting developer confidence in its functionality.
- Reduced Risks and Errors: Proactive testing with scripts minimizes the chance of bugs and unexpected behavior slipping into production, reducing stress and improving the overall development experience.
- Improved Test Coverage Visibility: Scripts provide a clear picture of which aspects of the API have been tested, giving developers a sense of progress and confidence in the overall API quality.
Streamlined Collaboration and Documentation
- Reusable Scripts: Â Shared and well-documented scripts within Postman workspaces enable efficient collaboration among development teams, promoting knowledge sharing and reducing duplication of effort.
- Improved Communication: Scripts act as a clear communication tool, documenting API test cases and expected behavior for all team members to reference.
- Consistent Testing Practices: Team-shared scripts ensure everyone utilizes the same testing procedures, fostering a unified approach to API validation.
Postman Test Scripts Examples
Here are four code examples showcasing Postman test scripts with increasing complexity.
Example 1 - Verifying Status Code
pm.test("Successful GET Request", () => {
pm.response.to.have.status(200);
});
Code explanation:
This script checks if the API responds with a 200 (OK) status code, indicating a successful GET request.
Example 2 - Validating JSON Response Structure
pm.test("Response has expected properties", () => {
pm.expect(pm.response.json()).to.have.property("name");
pm.expect(pm.response.json()).to.have.property("id").to.be.a("number");
});
Code explanation:
This script ensures that the JSON response contains specific properties ( name
and id
) and verifies that the id
property is a number.
Example 3 - Using Environment Variables
const baseUrl = pm.environment.get("apiUrl");
const expectedUserId = pm.environment.get("userId");
pm.test("GET User by ID", () => {
pm.expect(pm.response.json().id).to.equal(expectedUserId);
});
pm.sendRequest(baseUrl + "/users/" + expectedUserId);
Code explanation:
This example utilizes environment variables for the base URL and expected user ID. The script then validates if the response data's id
matches the expected user ID stored in the environment.
Example 4 - Handling Errors with Chai Assertions
pm.test("Error handling for non-existent user", () => {
pm.expect(pm.response.code).to.equal(404);
pm.expect(pm.response.json().error).to.equal("User not found");
// Using Chai assertions for complex validations
pm.expect(pm.response.json().message).to.contain("The requested user ID does not exist");
});
pm.sendRequest("http://localhost:3000/users/12345"); // Assuming a non-existent user ID
Code explanation:
This script checks for a 404 error code and validates the presence of specific error messages in the response. Additionally, it leverages Chai assertions to verify if the error message contains a specific string, demonstrating more complex validation capabilities.
Comfortably Build APIs with Apidog
If you are looking for a Postman alternative, make sure to consider trying Apidog, a comprehensive API development tool that provides more luxurious functionalities.
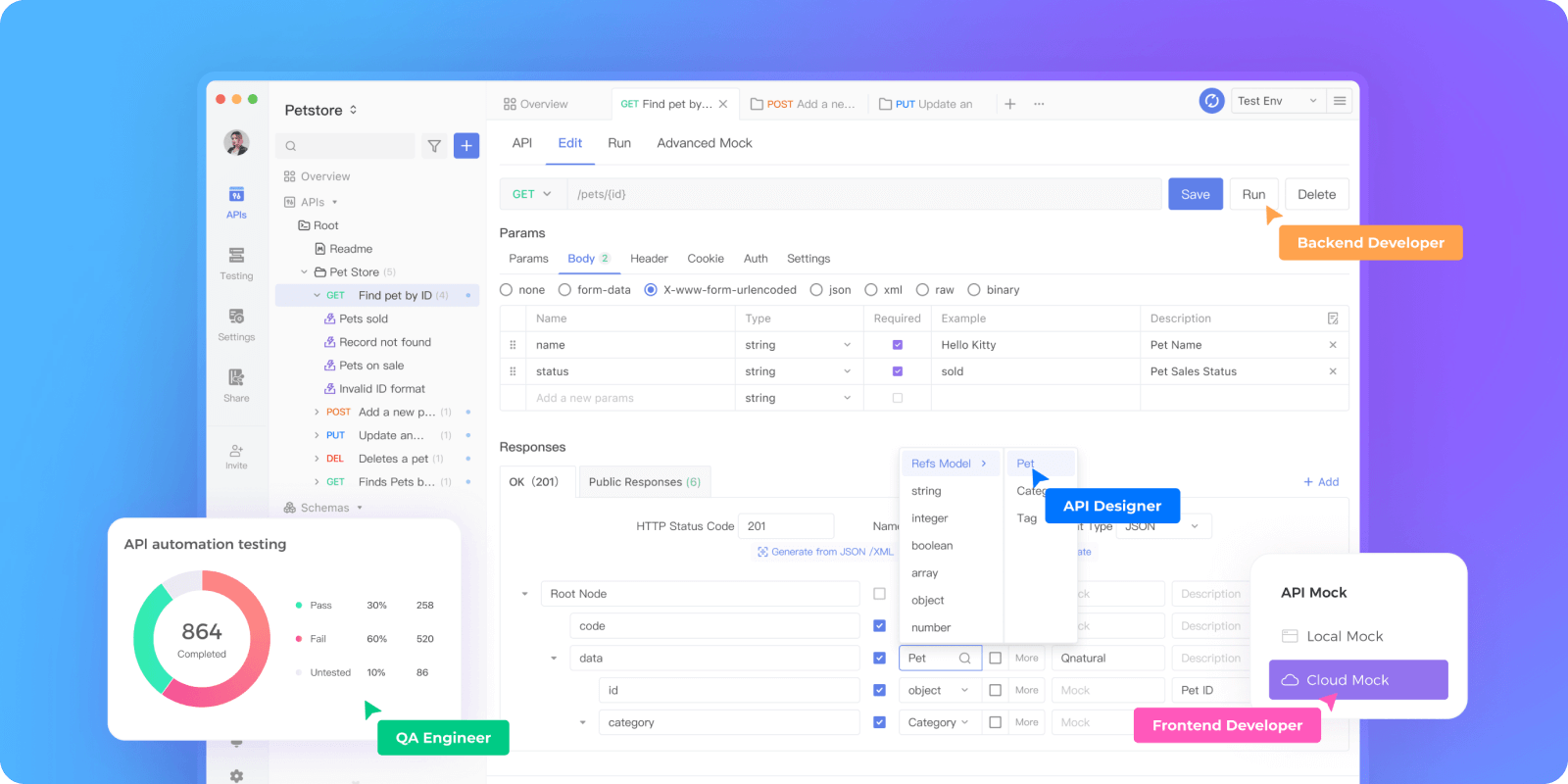
Import APIs to Apidog for Modificiation
Take your first step to perfecting your API by importing them onto Apidog. Apidog supports various API file types, including OpenAPI (or Swagger), Postman, and Insomnia.
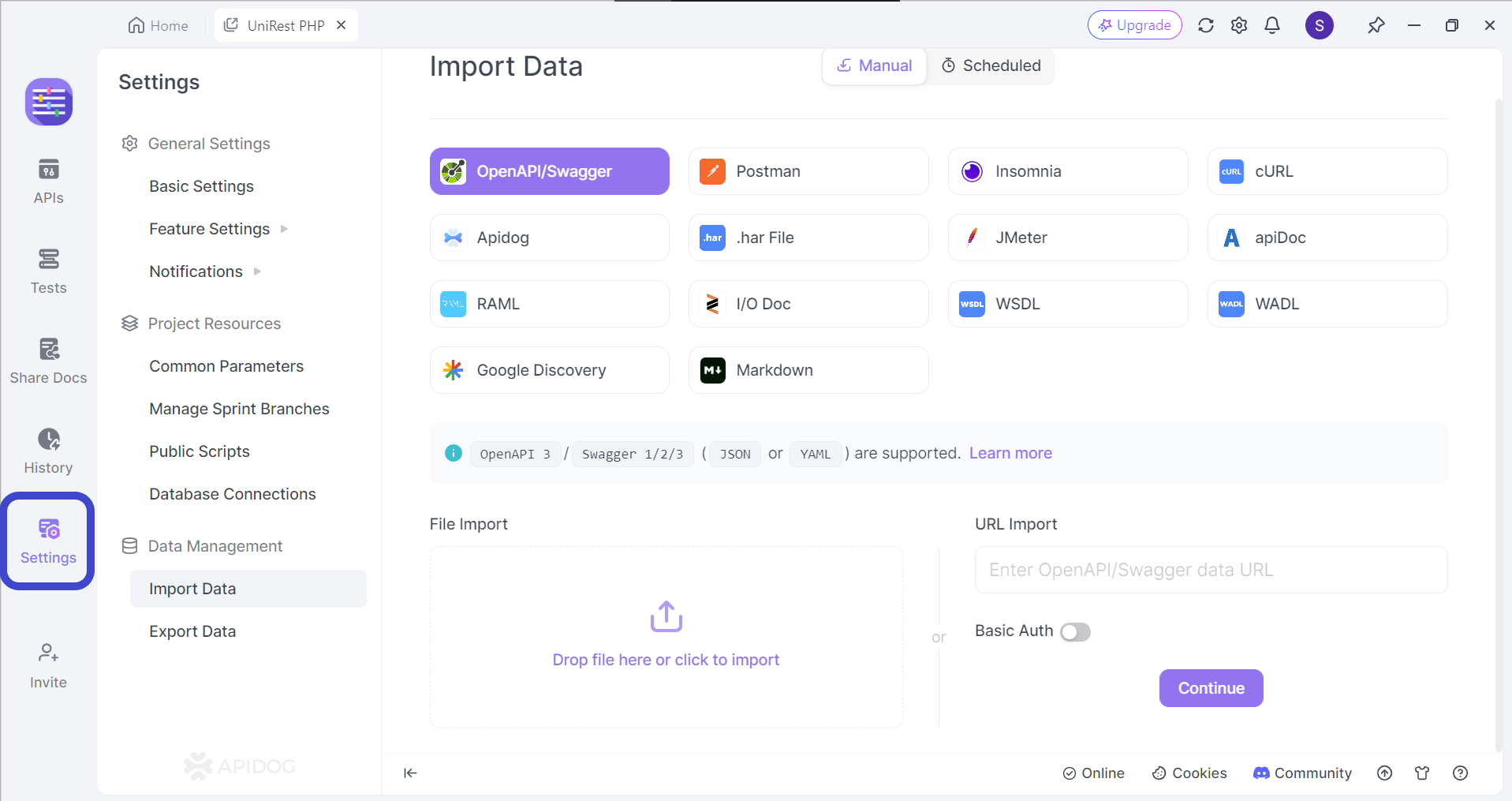
First, open the Settings section in your project, and locate the Import Data
button found under Data Management. If you cannot find the file type you wish to import, do not worry! Simply drag and drop the file to the bottom portion of the screen.
Adding Testing Scripts in Apidog
Once you have finished importing your API or creating a new project on Apidog, you can proceed with adding testing scripts.
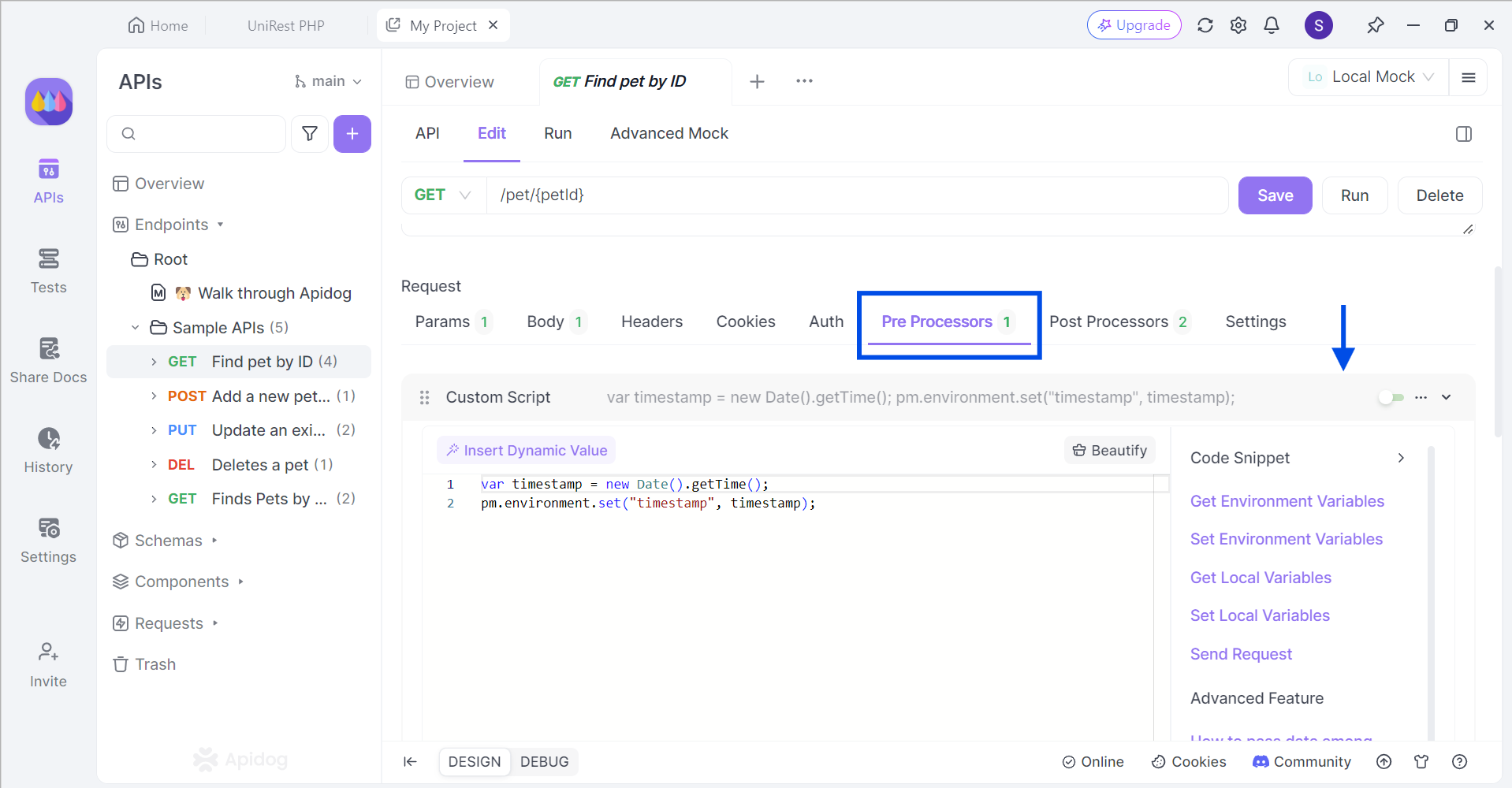
Under the Edit section of your API, locate the Pre Processors
heading. You should find a bar that is labeled Custom Script
. This is where you can implement custom scripts for your API requests. If you are struggling with figuring out what to write, you can also select the Code Snippet options found on the right of the codespace.
Conclusion
Postman test scripts empower developers to elevate their API testing practices. By automating repetitive tasks and enabling comprehensive validations, scripts streamline the development process and enhance the overall quality and reliability of APIs.
From basic status code checks to complex data verifications with error handling, the scripting capabilities within Postman provide a powerful and flexible solution for API testing. Â We encourage you to explore the vast potential of Postman test scripts and leverage them to ensure your APIs function flawlessly and deliver exceptional experiences.