How to Pass x-API-key in Header?
Unlock the secrets of effective API security by mastering how to pass x-API-key in headers. This comprehensive guide will reveal the importance of this process and how tools like Apidog can streamline your efforts. Read on to ensure your API interactions remain secure!
API security is paramount in today’s digital landscape, where unauthorized access can lead to significant consequences. Among the various methods to secure API interactions, the x-API-key serves as a critical component. This key is essential for verifying the identity of the client making the request and ensuring that only authorized users access specific resources.
In essence, an x-API-key is a unique identifier associated with an API client. By passing this key in the HTTP headers, developers can control access to their services. In this article, we’ll delve into how to pass x-API-key in headers effectively, focusing on practical examples and the role of tools like Apidog to facilitate the process.
What is x-API-key and Why is it Important?
An x-API-key is a custom HTTP header specifically used for API authentication and authorization. This header can forgo the necessity of complex OAuth flows, making it simpler for developers aiming to secure their endpoints. When a request is sent to an API, it can include the x-API-key to identify the user or application making the call.
Importance of x-API-key in API security:
- Access Control: By requiring an x-API-key, developers can control who can access their APIs, thus minimizing the risk of unauthorized usage.
- Unique Identification: Each key is unique to the user or application, ensuring every interaction is traceable.
- Reduced Load: Unlike more complicated authentication methods, using an x-API-key is quick, minimizing the number of steps needed for access.
Incorporating an x-API-key into your API design is a best practice that enhances security while simplifying user experience.
How to Pass x-API-key in Headers
Passing the x-API-key in headers can be done using various programming languages or tools. Here’s a simple procedure to follow:
- Identify the API Endpoint: Start by determining the API endpoint you plan to access.
- Generate an x-API-key: Ensure you have a valid x-API-key generated for your application or user account.
- Choose Your Tool/Method: Depending on the language or tool you are using (like Apidog), you can begin the process.
- Set Up Your Request: Prepare your HTTP request, including the necessary parameters and the x-API-key.
x-API-key Example in cURL:
A popular way to pass x-API-key is by using cURL. Below is a simple example:
curl -X GET "https://api.example.com/resource" \
-H "x-API-key: your_api_key_here"
x-API-key Example in JavaScript:
When working within a JavaScript environment, such as with fetch
:
fetch('https://api.example.com/resource', {
method: 'GET',
headers: {
'x-API-key': 'your_api_key_here'
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
x-API-key Example in Python:
import requests
headers = {
'x-API-key': 'your-api-key-here'
}
response = requests.get('https://api.example.com/endpoint', headers=headers)
if response.status_code == 200:
print('Success:', response.json())
else:
print('Error:', response.status_code, response.text)
x-API-key Example in HttpClient:
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class Main {
public static void main(String[] args) throws Exception {
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://api.example.com/endpoint"))
.header("x-API-key", "your-api-key-here")
.build();
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
if (response.statusCode() == 200) {
System.out.println("Success: " + response.body());
} else {
System.out.println("Error: " + response.statusCode() + " " + response.body());
}
}
}
x-API-key Example in Go:
package main
import (
"fmt"
"net/http"
"io/ioutil"
)
func main() {
client := &http.Client{}
req, err := http.NewRequest("GET", "https://api.example.com/endpoint", nil)
if err != nil {
panic(err)
}
req.Header.Add("x-API-key", "your-api-key-here")
resp, err := client.Do(req)
if err != nil {
panic(err)
}
defer resp.Body.Close()
if resp.StatusCode == http.StatusOK {
body, _ := ioutil.ReadAll(resp.Body)
fmt.Println("Success:", string(body))
} else {
fmt.Println("Error:", resp.Status)
}
}
Using Apidog simplifies this process further by allowing users to manage their APIs visually without manual coding.
Using Apidog to Pass x-API-key in Headers
Apidog simplifies the process of passing the x-API-key in headers, making it an ideal tool for developers and security professionals. Whether you're working on a small project or managing large-scale API deployments, Apidog provides the tools you need to ensure that your API keys are handled securely.
What is Apidog?
Apidog is an innovative API development and testing tool that enables users to define, document, and test their APIs with efficiency. One of its key features is the ability to easily configure headers, including the x-API-key.
Benefits of Using Apidog:
- Easy Configuration: Apidog’s intuitive interface allows for straightforward header configuration without deep technical knowledge.
- Team Collaboration: Multiple users can work together in Apidog, sharing API keys and configurations seamlessly.
- Comprehensive Testing: Users can execute requests directly within Apidog and immediately verify responses.
Passing x-API-key in Headers Using Apidog?
To pass the x-API-key in the header using Apidog, follow these steps:
Step 1: Launch Apidog and open the desired project.
Step 2: Create a new API endpoint or select the desired endpoint at Apidog.
Step 3: Within the API endpoint request section, navigate to Headers
section.
Step 4: On the header parameters, enter "x-API-key" as the name. In the value field, input your specific API key.
Step 5: Test the request by clicking on "Send" to ensure successful authentication.
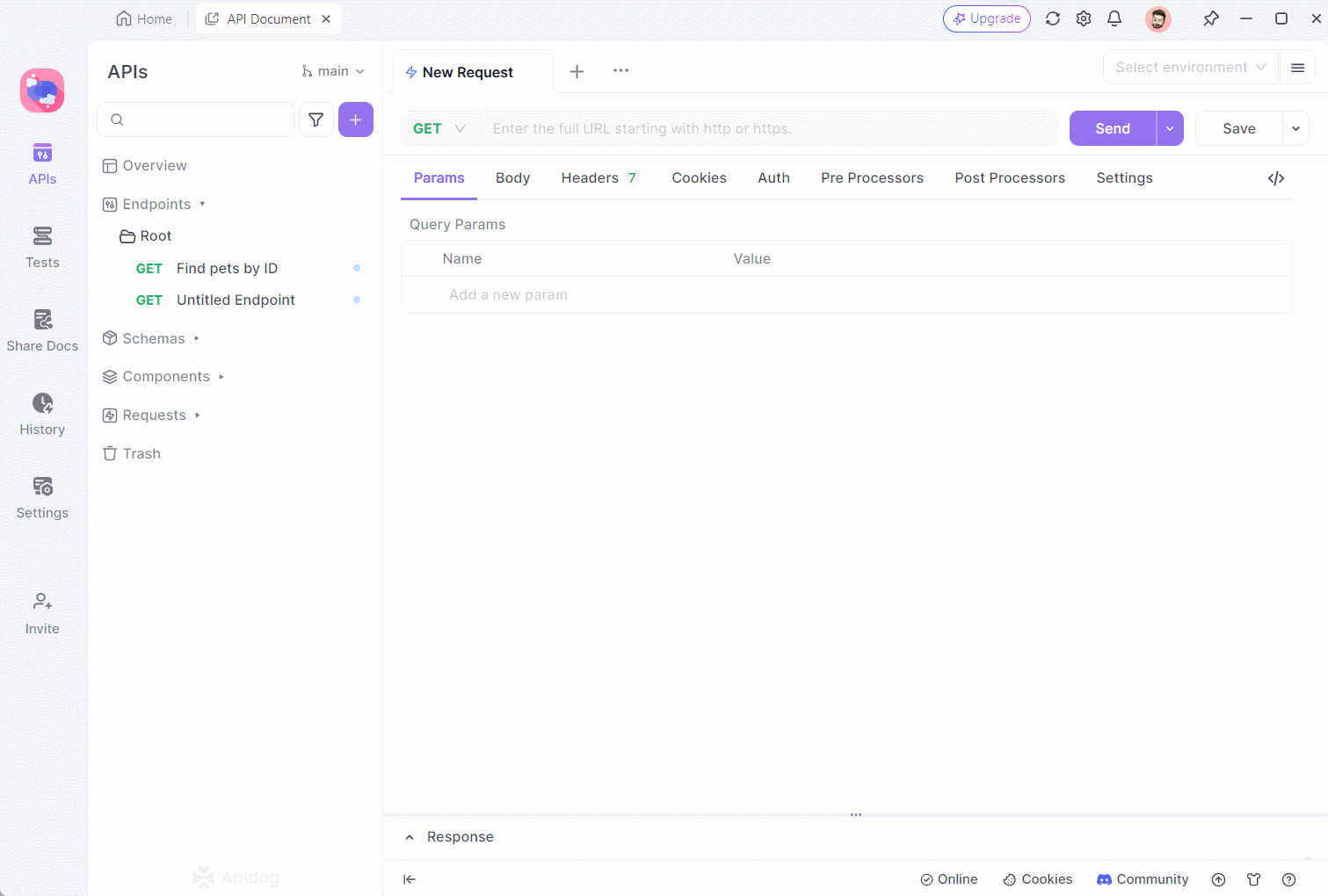
With Apidog, passing an x-API-key in headers is not only simplified but also convenient for testing various scenarios.
Common Mistakes to Avoid When Passing x-API-key in Headers
While passing x-API-key in headers may seem straightforward, several common pitfalls can impact API security and functionality. Awareness of these mistakes can save developers time and effort.
Frequent Mistakes:
1. Forgetting to Include the Header:
- This leads to unauthorized access errors, as the API will reject the request without the key.
2. Using an Expired or Revoked Key:
- Regularly check API keys for validity. Using a dead key can render your requests useless.
3. Hardcoding Keys in the Source Code:
- This poses a significant security risk. Instead, consider using environment variables.
4. Neglecting API Rate Limits:
- Be mindful of how often requests are made. Exceeding limits can lead to throttling or bans.
Tips to Prevent Mistakes:
- Always double-check API documentation for required headers.
- Use version control and environment variables to manage configurations securely.
- Regularly monitor and rotate keys to maintain security.
By avoiding these common mistakes, developers can ensure their APIs remain secure and functional.
Conclusion
Understanding how to pass x-API-key in headers is essential for developers looking to bolster their API security. By effectively implementing this practice, in conjunction with tools such as Apidog, teams can ensure seamless and secure interactions between applications.
From recognizing the significance of x-API-key to employing best practices in key management, mastering this skill is crucial. Developers should continually update their knowledge and practices in API security to stay ahead in an ever-evolving digital landscape.