AI tools transform how developers write, debug, and manage code. Three leading models—o3, Sonnet 3.7, and Gemini 2.5 Pro—stand out for their coding capabilities. This technical blog post compares these AI models across key areas: code generation, debugging, large project handling, API integration, and cost-effectiveness. Each model offers unique strengths, and understanding them helps developers pick the right tool for their needs.
Introduction: The Evolution of AI in Coding
The journey of AI in coding began with rudimentary tools offering basic code completion and syntax suggestions. Over the years, these tools evolved dramatically, leveraging advancements in natural language processing (NLP) and machine learning to tackle more sophisticated tasks. Today, AI models like o3, Sonnet 3.7, and Gemini 2.5 Pro represent the pinnacle of this evolution, capable of generating entire applications, debugging complex errors, and managing sprawling codebases.
- o3: Developed by OpenAI, o3 builds on the legacy of its predecessors with unmatched reasoning prowess. It excels in solving intricate algorithmic problems and delivering optimized solutions, making it a favorite among developers tackling high-complexity tasks.
- Sonnet 3.7: Anthropic’s Sonnet 3.7 is engineered for scale and clarity. With an impressive 200,000-token context window, it produces clean, maintainable code, ideal for large projects and collaborative environments.
- Gemini 2.5 Pro: Google’s Gemini 2.5 Pro pushes boundaries with its multimodal capabilities, processing text, images, and potentially other data types. Its massive 1-million-token context (expandable to 2 million) and speed make it a versatile choice for diverse development needs.
These models cater to different aspects of coding, from algorithmic precision to UI integration. In the sections below, we’ll dissect their performance across key areas, starting with code generation.
Code Generation: Precision, Readability, and Speed
Code generation is a litmus test for AI coding tools. Developers demand speed, accuracy, and versatility. Let’s evaluate each model with specific examples to highlight their strengths.
o3: Master of Complex Algorithms
o3 shines when generating code for intricate tasks. Its advanced reasoning ensures precise, optimized outputs. Consider a classic problem: implementing a binary search algorithm in Python. Here’s how o3 handles it:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = left + (right - left) // 2 # Avoids integer overflow
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
This implementation is efficient (O(log n) time complexity) and includes a subtle optimization to prevent integer overflow—a detail o3 often highlights in its explanations. It also excels in languages like C++ or Java, producing similarly robust solutions. However, for simpler tasks, such as generating a basic HTML form, o3 might over-engineer the output, adding unnecessary complexity that could overwhelm beginners.
Sonnet 3.7: Champion of Clarity
Sonnet 3.7 prioritizes readability and maintainability, making it ideal for team projects or educational purposes. When tasked with creating a REST API in Node.js, it delivers well-structured, commented code:
// Import Express framework
const express = require('express');
const app = express();
const port = 3000;
// Middleware to parse JSON bodies
app.use(express.json());
// In-memory data store
let items = [
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' }
];
// GET endpoint to retrieve all items
app.get('/items', (req, res) => {
res.json(items);
});
// POST endpoint to add a new item
app.post('/items', (req, res) => {
const newItem = { id: items.length + 1, name: req.body.name };
items.push(newItem);
res.status(201).json(newItem);
});
// DELETE endpoint to remove an item by ID
app.delete('/items/:id', (req, res) => {
const id = parseInt(req.params.id);
items = items.filter(item => item.id !== id);
res.status(204).send();
});
// Start the server
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}`);
});
This code is not only functional but also easy to follow, thanks to its detailed comments. Sonnet 3.7 scores 70.3% on SWE-Bench Verified with extended thinking mode, reflecting its reliability across Python, JavaScript, and more. Its downside? It can be slower than o3 for highly complex tasks, as it emphasizes thoroughness over raw speed.
Gemini 2.5 Pro: Multimodal Versatility
Gemini 2.5 Pro leverages its multimodal capabilities to generate code that integrates with visual elements, excelling in front-end development. For a Next.js page with a dynamic component, it might produce:
import React, { useState } from 'react';
export default function Home() {
const [count, setCount] = useState(0);
return (
<div style={{ textAlign: 'center', padding: '20px' }}>
<h1>Welcome to My Next.js App</h1>
<p>Counter: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
This snippet is fast to generate and aligns with UI requirements, such as styling or interactivity. Gemini 2.5 Pro’s speed suits tight deadlines, but its outputs occasionally contain errors—like misplaced semicolons or unclosed tags—requiring manual review.
Comparison
- o3: Best for complex, optimized algorithms.
- Sonnet 3.7: Ideal for clean, maintainable code in collaborative settings.
- Gemini 2.5 Pro: Excels in front-end and multimodal tasks with rapid output.
Next, let’s examine their debugging prowess.
Debugging and Error Handling: Precision Meets Context
Debugging is a critical skill for any coding tool. Each model tackles bugs differently, from syntax errors to performance bottlenecks.
o3: Logical Precision
o3 thrives on logical errors. Its reasoning capabilities unravel even the trickiest bugs. Take this buggy Python sorting function:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i): # Bug: Off-by-one error
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
o3’s Fix:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1): # Fixed range to prevent index error
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
o3 identifies the off-by-one error and explains the fix, ensuring the loop stays within bounds. It also handles performance issues, like optimizing a slow database query, but may overcomplicate simpler syntax fixes.
Sonnet 3.7: Contextual Mastery
Sonnet 3.7 leverages its large context window to debug across files. For a Flask app with a routing bug:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('index.html') # Bug: Template not found
Sonnet 3.7 traces the issue to a missing templates
folder, suggesting a fix and folder structure. Its detailed breakdowns are beginner-friendly, though it may over-engineer minor fixes.
Gemini 2.5 Pro: UI Debugging
Gemini 2.5 Pro excels at UI-related bugs. For a React component not rendering:
import React from 'react';
function Card() {
return (
<div>
<h2>Card Title</h2>
<p>Content</p>
</div> // Bug: Missing closing tag
);
}
Gemini 2.5 Pro spots the error and corrects it, aligning the code with the intended UI. Its multimodal skills shine here, but minor errors in fixes—like incorrect prop names—may slip through.
Comparison
- o3: Top for logical and performance bugs.
- Sonnet 3.7: Best for contextual, multi-file debugging.
- Gemini 2.5 Pro: Ideal for UI and front-end issues.
Now, let’s tackle large projects.
Handling Large and Complex Projects: Scale and Coherence
Large codebases demand robust context management. Here’s how each model performs, with real-world examples.
Sonnet 3.7: Scalable Clarity
With its 200,000-token context, Sonnet 3.7 excels in mid-to-large projects. In a real-world case, it refactored a Django app, adding user authentication across models, views, and templates. Its output is consistent and well-documented, though it may over-detail minor changes.
Gemini 2.5 Pro: Massive Scope
Gemini 2.5 Pro’s 1-million-token context handles massive systems. It was used to optimize a React-based e-commerce platform, reducing load times by refactoring components and API calls. Its multimodal skills also allow UI tweaks based on design inputs, making it a powerhouse for full-stack development.
o3: Focused Expertise
o3’s smaller context requires chunking large projects, but its reasoning shines within those limits. It optimized a microservices module, cutting latency by 30%, though it needs careful prompting for system-wide tasks.
Comparison
- Gemini 2.5 Pro: Best for massive, multimodal projects.
- Sonnet 3.7: Ideal for mid-to-large, maintainable codebases.
- o3: Suited for focused, complex segments.
Let’s explore API integration next.
API Integration: Streamlining Development
APIs connect AI tools to workflows, enhancing efficiency. Here’s how each model pairs with Apidog.
o3: Flexible Integration
o3’s OpenAI API integrates into IDEs or pipelines, generating and testing code. With Apidog, developers can create endpoints with o3 and validate them instantly, ensuring robust APIs.
Sonnet 3.7: Large-Scale API Work
Sonnet 3.7’s API handles extensive contexts, perfect for generating and testing complex APIs. Paired with Apidog, it automates documentation and testing, streamlining development.
Gemini 2.5 Pro: Dynamic APIs
Gemini 2.5 Pro’s API supports multimodal inputs, generating code from specs or designs. Using Apidog, developers can test and document these APIs, ensuring alignment with requirements.
Comparison
- Gemini 2.5 Pro: Best for dynamic, multimodal APIs.
- Sonnet 3.7: Great for large-scale API tasks.
- o3: Versatile for various API needs.
Now, onto cost-effectiveness.
Cost-Effectiveness: Balancing Price and Performance
Cost influences adoption. Here’s a breakdown:
Pricing Table
Model | Input Tokens Cost | Output Tokens Cost | Notes |
---|---|---|---|
o3 | $10/million | $30/million | High cost for premium features |
Sonnet 3.7 | $3/million | $15/million | Affordable for large contexts |
Gemini 2.5 Pro | $1.25/million (up to 128k) | $2.50/million (up to 128k) | Scales up for larger contexts |
Analysis
- o3: Expensive but worth it for complex tasks.
- Sonnet 3.7: Balanced cost for large projects.
- Gemini 2.5 Pro: Cheapest, with strong value for scale.
Let’s add community support.
Community Support: Resources and Assistance
Support is vital for adoption. Here’s the rundown:
o3: Robust Ecosystem
OpenAI’s documentation, forums, and tutorials are top-notch, though o3’s complexity may challenge newbies.
Sonnet 3.7: Growing Resources
Anthropic offers detailed guides, with an engaged community sharing insights for large projects.
Gemini 2.5 Pro: Google’s Backing
Google provides extensive resources, especially for multimodal tasks, with a vibrant developer network.
Comparison
- o3: Best for extensive support.
- Sonnet 3.7: Strong for large-project help.
- Gemini 2.5 Pro: Rich for multimodal needs.
Finally, the conclusion.
Conclusion: Choosing Your AI Coding Partner
- o3: Pick for complex algorithms and reasoning.
- Sonnet 3.7: Choose for large, maintainable projects.
- Gemini 2.5 Pro: Opt for scalable, multimodal tasks.
Enhance any choice with Apidog—download it free—to streamline API workflows. Your ideal AI depends on project scope, budget, and needs.
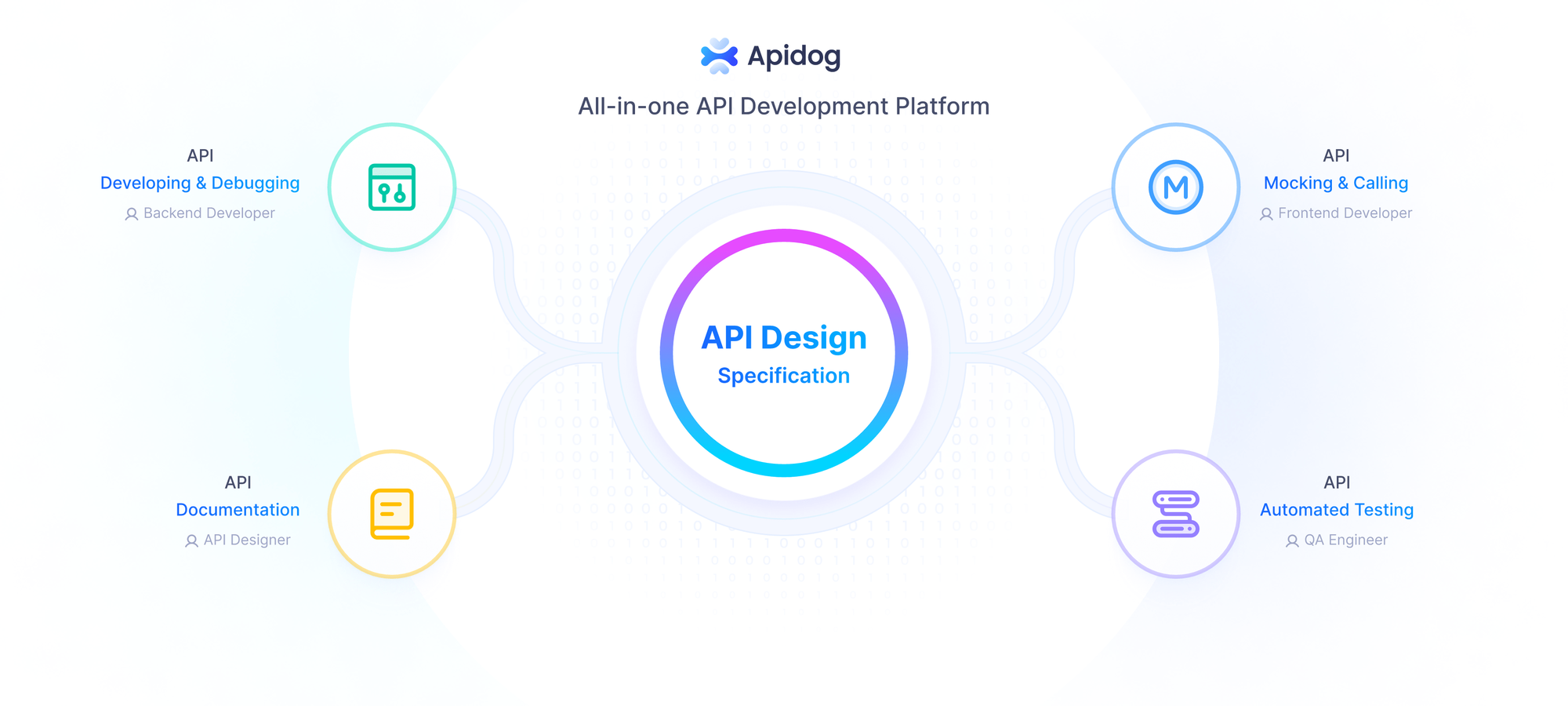