In today's data-driven world, efficiently transferring large datasets between systems is crucial. While JSON has become the standard format for data exchange, it faces limitations when handling large volumes of data or streaming scenarios. This is where NDJSON (Newline Delimited JSON) comes in – a simple yet powerful format that's revolutionizing how we stream data over HTTP connections. In this article, we'll explore what NDJSON is, how it differs from standard JSON, and how Apidog can help you work with NDJSON streaming endpoints.
What is NDJSON?
NDJSON (Newline Delimited JSON) is a format that stores structured data as a sequence of JSON objects, with each object on its own line, separated by a newline character (\n
). It's also known as JSON Lines (JSONL) in some contexts.
Here's a simple example of NDJSON:
{"id": 1, "name": "Alice", "score": 95}
{"id": 2, "name": "Bob", "score": 87}
{"id": 3, "name": "Charlie", "score": 92}
Each line is a valid, complete JSON object, making it easy to process one record at a time without loading the entire dataset into memory.
NDJSON vs. Traditional JSON: Key Differences
Traditional JSON and NDJSON serve different purposes and have distinct characteristics:
Feature | Traditional JSON | NDJSON |
---|---|---|
Structure | Single, complete document | Multiple independent JSON objects |
Parsing | Must load entire document | Can process line by line |
Streaming | Not designed for streaming | Ideal for streaming |
Memory usage | Requires full document in memory | Processes one line at a time |
File size | Limited by available memory | Virtually unlimited |
The primary advantage of NDJSON is its ability to be processed incrementally. With traditional JSON, you need to parse the entire file before accessing any data. With NDJSON, you can read and process one line at a time, making it perfect for:
- Log files
- Data streaming
- Large datasets
- Real-time data processing
Why Use NDJSON for HTTP Streaming?
HTTP streaming allows servers to send data to clients incrementally rather than waiting for the entire response to be ready. NDJSON is particularly well-suited for HTTP streaming because:
- Simplicity: Each line is a complete, valid JSON object
- Compatibility: Works with existing JSON parsers
- Incremental Processing: Clients can process data as it arrives
- Memory Efficiency: No need to buffer the entire response
- Human Readability: Easy to inspect and debug
How to Stream NDJSON Over HTTP Endpoints
Implementing NDJSON streaming over HTTP is straightforward. Here's how it works on both the server and client sides:
Server-Side Implementation
To create an HTTP endpoint that streams NDJSON:
Set the appropriate headers:
Content-Type: application/x-ndjson
Transfer-Encoding: chunked
Write each JSON object to the response stream, followed by a newline character:
// Node.js example
app.get('/api/data/stream', (req, res) => {
res.setHeader('Content-Type', 'application/x-ndjson');
res.setHeader('Transfer-Encoding', 'chunked');
// Stream data as it becomes available
dataSource.on('data', (item) => {
res.write(JSON.stringify(item) + '\n');
});
dataSource.on('end', () => {
res.end();
});
});
Client-Side Processing
On the client side, you need to:
- Make an HTTP request to the streaming endpoint
- Process the response incrementally, line by line
- Parse each line as a JSON object
Here's a simple JavaScript example:
async function consumeNdjsonStream(url) {
const response = await fetch(url);
const reader = response.body.getReader();
const decoder = new TextDecoder();
let buffer = '';
while (true) {
const { value, done } = await reader.read();
if (done) break;
buffer += decoder.decode(value, { stream: true });
const lines = buffer.split('\n');
buffer = lines.pop(); // Keep the last incomplete line
for (const line of lines) {
if (line.trim() === '') continue;
const jsonObject = JSON.parse(line);
processData(jsonObject); // Do something with the data
}
}
}
Common Use Cases for NDJSON Streaming
NDJSON streaming is particularly valuable in scenarios such as:
- Real-time analytics: Stream event data for immediate processing
- Log aggregation: Continuously send application logs to monitoring systems
- Data exports: Transfer large datasets without memory constraints
- IoT applications: Process sensor data as it's generated
- Activity feeds: Deliver social media or application events in real-time
Testing NDJSON Endpoints with Apidog
Apidog, the comprehensive API development platform, offers built-in support for testing and debugging NDJSON streaming endpoints. Here's how Apidog simplifies working with NDJSON:
1. Real-Time Stream Visualization
Apidog's user interface displays NDJSON responses in real-time, showing each JSON object as it arrives from the server. This makes it easy to monitor streaming data without writing custom code.
2. Automatic Parsing and Formatting
Apidog automatically parses each line of NDJSON as it arrives, formatting it for readability while preserving the streaming nature of the response.
3. Request Configuration
Apidog makes it simple to configure the necessary headers and parameters for NDJSON streaming requests, ensuring proper communication with your streaming endpoints.
Step-by-Step Guide to Streaming HTTP Endpoints That Return NDJSON
To test an NDJSON streaming endpoint in Apidog:
Step 1: Create a new HTTP request
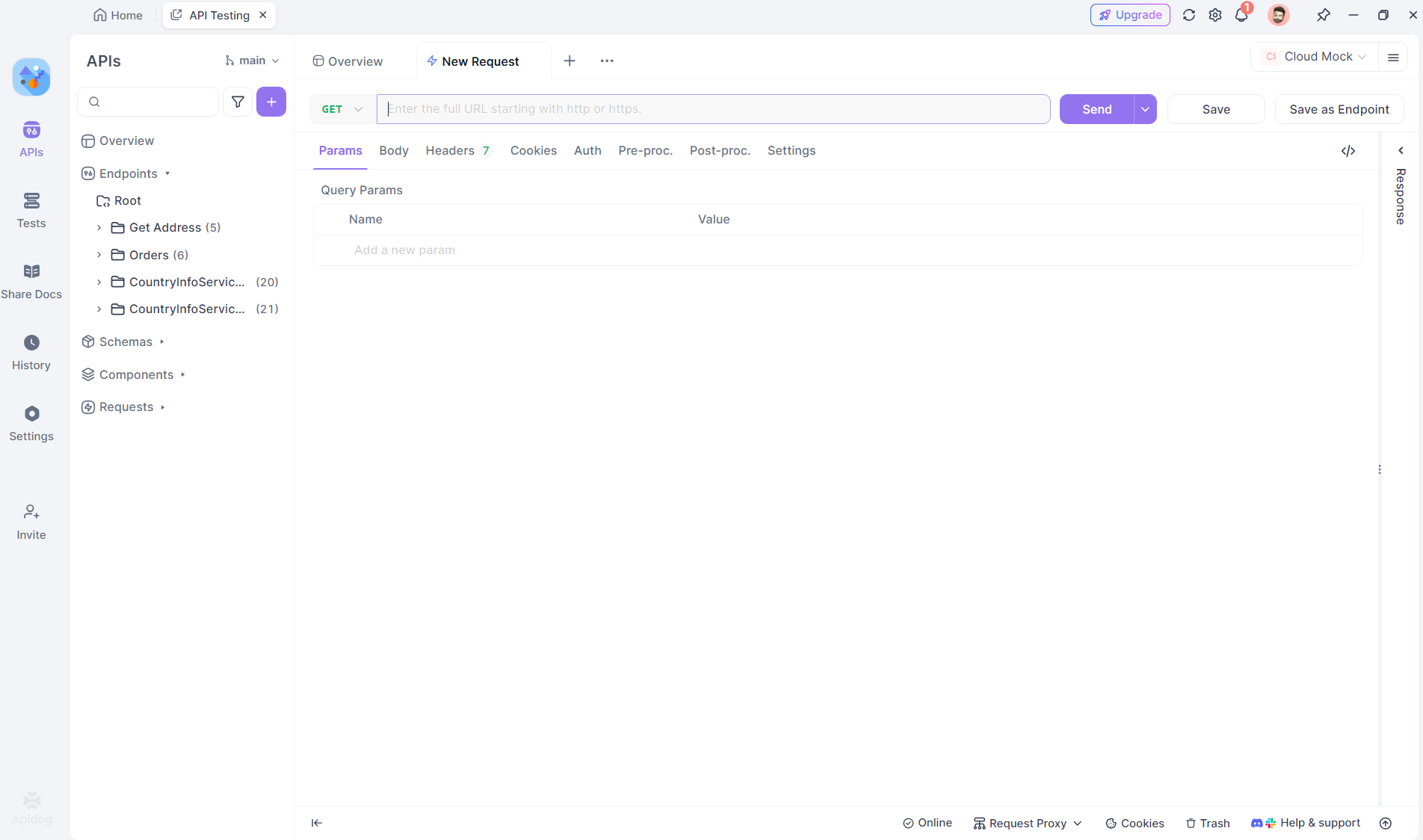
Step 2: Set the request method
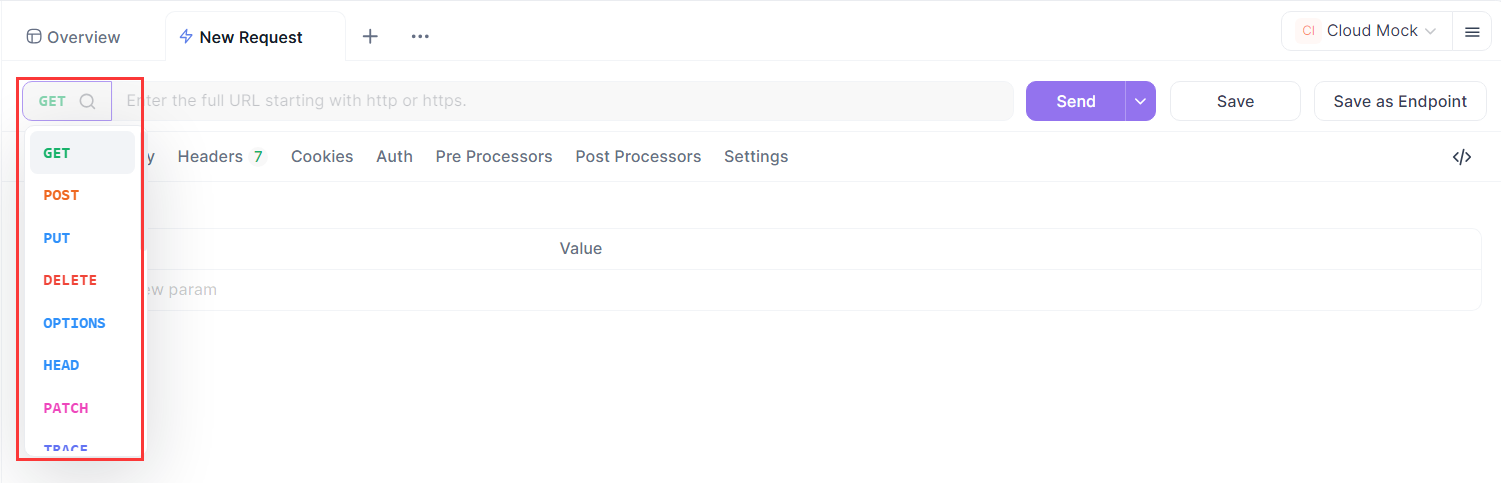
Step 3: Enter your streaming endpoint URL
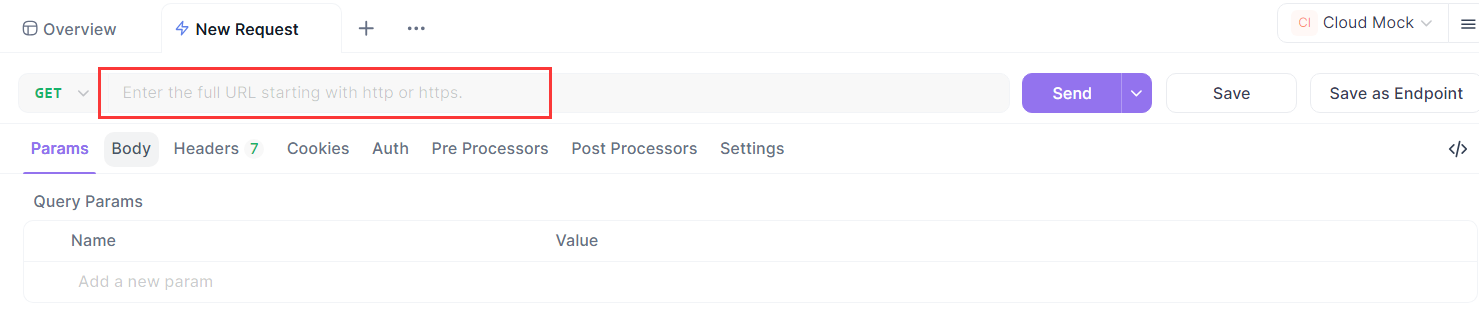
Step 4: Send the request
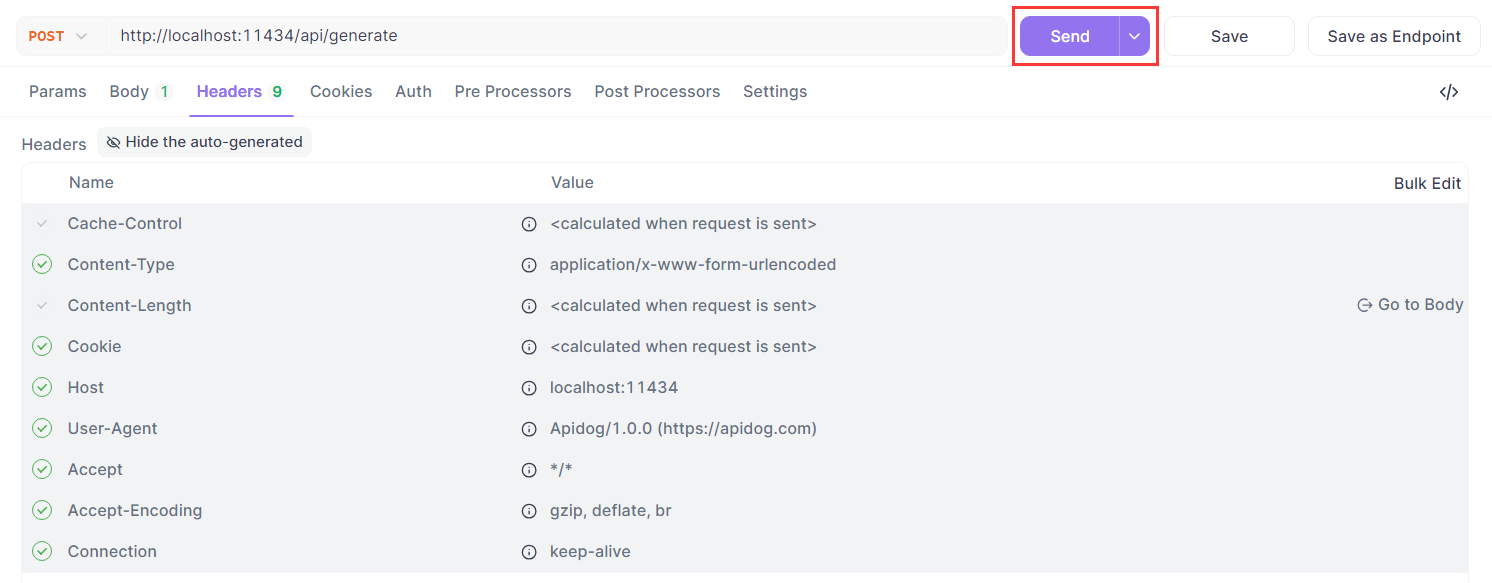
Step 5: View the test result
Watch as Apidog displays the streaming NDJSON response in real-time
You can choose to merge the returned NDJSON messages into a complete reply. Learn more here.
Conclusion
NDJSON provides an elegant solution for streaming structured data over HTTP, combining the flexibility of JSON with the efficiency of line-by-line processing. Whether you're building real-time analytics, log processing systems, or data export functionality, NDJSON offers a simple yet powerful approach to handling streaming data.
With Apidog's support for NDJSON streaming, testing and debugging these endpoints becomes significantly easier. You can visualize, inspect, and validate your streaming data without writing custom code, accelerating your development process and ensuring your streaming APIs work as expected.
Start exploring NDJSON streaming with Apidog today and experience the power of real-time data processing in your applications!