Large language models (LLMs) like Claude by Anthropic are becoming increasingly capable, but to fully unlock their potential, they need access to rich, external context. This is where the Model Context Protocol (MCP) comes in—a standardized way for tools, apps, and services to communicate context to LLMs. In this article, we’ll walk through building an MCP server in TypeScript, explore how it interacts with clients, and demonstrate how to connect it to Claude Desktop.
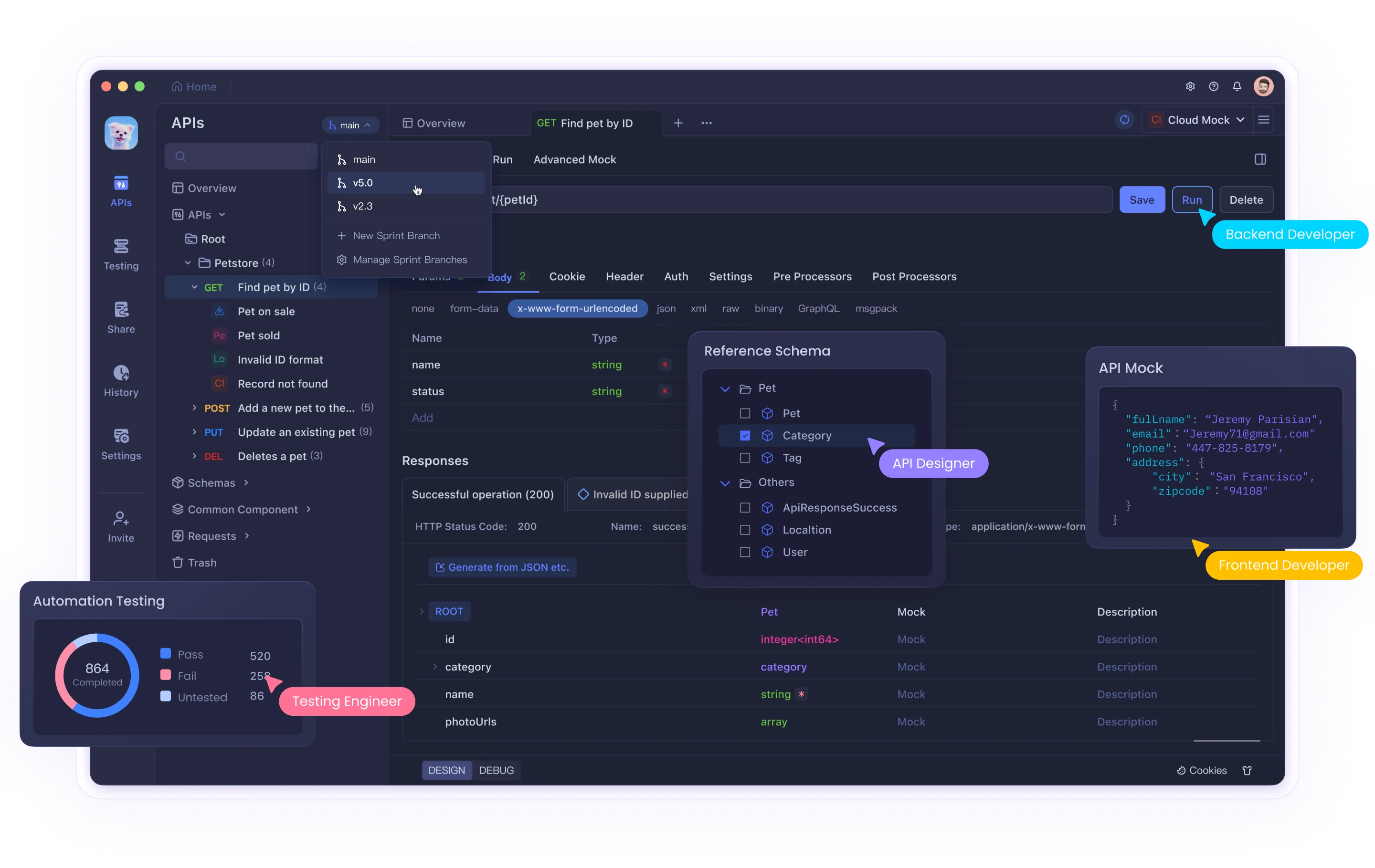
What Is the Model Context Protocol (MCP)?
The Model Context Protocol is a universal protocol that defines how LLM applications (hosts), servers, and clients exchange data. MCP enables modular, tool-based integration with local or online data sources in a scalable and structured way. It’s especially useful in desktop-based LLM environments, such as Claude Desktop or Sourcegraph Cody.
At a high level, the protocol includes:
- Hosts: The LLM-based app (e.g., Claude Desktop)
- Clients: Embedded modules that manage connections to servers
- Servers: Providers of tools, data, or services
- Transports: The underlying mechanism for sending and receiving JSON-RPC messages
Why Use MCP?
Traditional methods like copy-pasting or hardcoding context are clunky and limited. MCP solves these issues by allowing:
- Tool discovery and capability negotiation
- JSON-RPC based message exchange
- A consistent interface across languages and platforms
- Clean separation between the LLM app and the external tools
This modularity enables developers to create powerful context-aware tools that can interact with LLMs in real time.
Prerequisites
To build a TypeScript MCP server and connect it to Claude Desktop, you’ll need:
- Node.js (v18+)
- TypeScript
- Basic knowledge of JSON-RPC
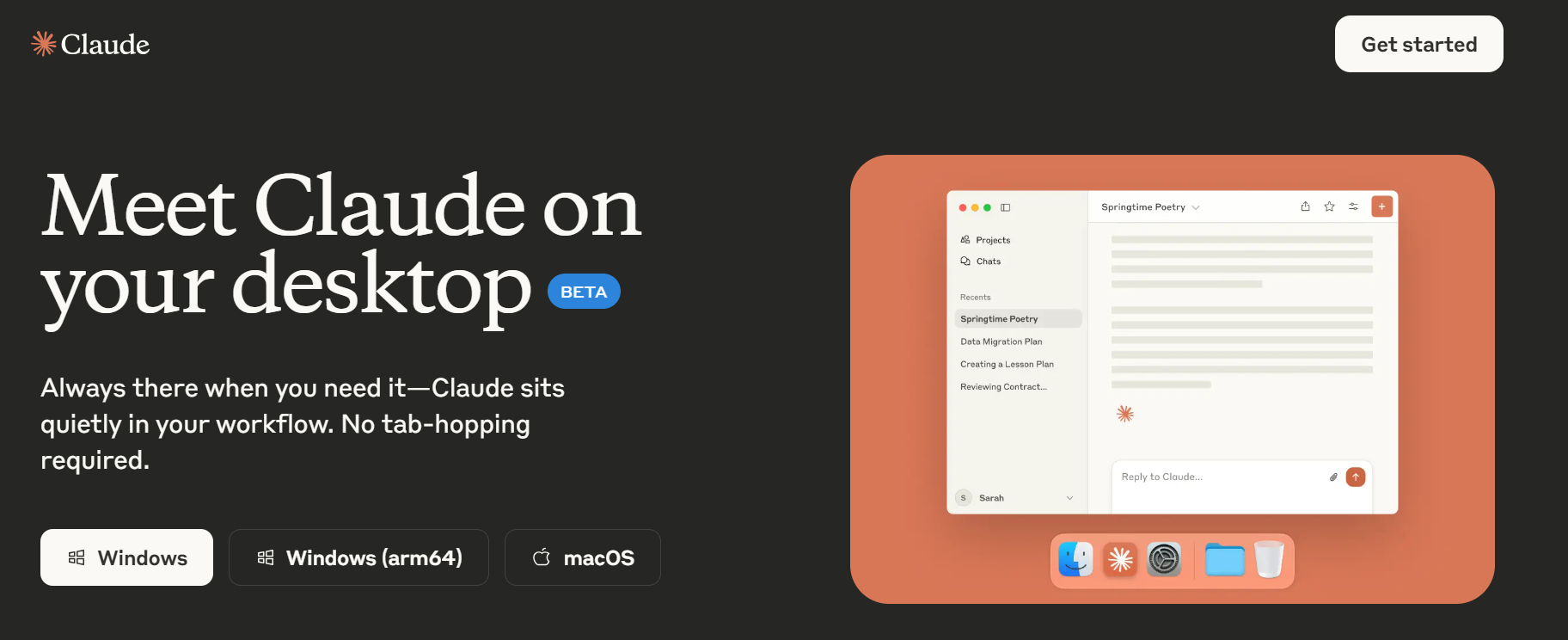
MCP TypeScript SDK
Step 1: Setting Up Your Project
Start by setting up a Node.js project:
mkdir mcp-ts-server
cd mcp-ts-server
npm init -y
npm install typescript ts-node @modelcontextprotocol/server-core
npx tsc --init
Create a src/
directory, and inside it, add your entry point file, e.g., index.ts
.
Step 2: Creating Your MCP Server in TypeScript
Let’s walk through creating a simple MCP server that provides a “tool” for greeting users.
Here’s the basic structure:
import { createServer, Server } from '@modelcontextprotocol/server-core';
const server: Server = createServer();
const GREETING_TOOL = {
name: 'greet_user',
description: 'Returns a friendly greeting message.',
inputSchema: {
type: 'object',
properties: {
name: { type: 'string', description: 'Name of the user' }
},
required: ['name']
}
};
// Register tool
server.setRequestHandler('ListTools', async () => {
return { tools: [GREETING_TOOL] };
});
// Handle tool call
server.setRequestHandler('CallTool', async (request) => {
const args = request.args;
const name = args.name || 'there';
return {
content: [{ type: 'text', text: `Hello, ${name}! Welcome to MCP.` }],
isError: false
};
});
// Start server
server.listen();
You can now compile and run this with:
npx ts-node src/index.ts
Step 3: Register Your Server with Claude Desktop
Each MCP server needs a unique URI. For example:
tool://greet_user/greet_user
When Claude Desktop initializes, it can discover the server through a Stdio
transport. This means your server communicates via standard input/output (stdin/stdout) rather than HTTP or sockets.
Step 4: Connecting MCP Server to Claude Desktop
In Claude Desktop:
- Go to Settings > Tool Providers
- Add a new tool provider using a Stdio transport
- Specify your tool URI and the command to run your server, e.g.:
npx ts-node src/index.ts
Claude Desktop will initiate communication using JSON-RPC 2.0 via stdin/stdout, and your server should respond with the list of tools it supports.
Certainly! Here's a rewritten version of the Claude Desktop configuration section that you can insert directly into your article:
Using Claude Desktop to Test Your MCP Server
The Claude Desktop app is one of the easiest environments to test MCP integrations locally.
To manually configure it to launch your MCP server, follow these steps:
Open or create the file at:
~/Library/Application Support/Claude/claude_desktop_config.json
⚠️ Note: This is not the same as config.json
in the same folder. Editing the wrong file won't have any effect.
Add the following JSON configuration, replacing the details as needed for your tool:
{
"mcpServers": {
"brave_search": {
"command": "npx",
"args": ["@modelcontextprotocol/server-brave-search"],
"env": {
"BRAVE_API_KEY": "your-api-key"
}
}
}
}
This tells Claude Desktop to:
- Recognize a tool named
brave_search
- Launch it via
npx @modelcontextprotocol/server-brave-search
- Inject environment variables, like your API key
- Save the file and restart Claude Desktop.
Once restarted, you can ask Claude Desktop to use your new tool. For example:
"Search the web for glama.ai"
If this is your first time using MCP, Claude will ask for permission via a pop-up dialog. Click "Allow for This Chat" to continue.
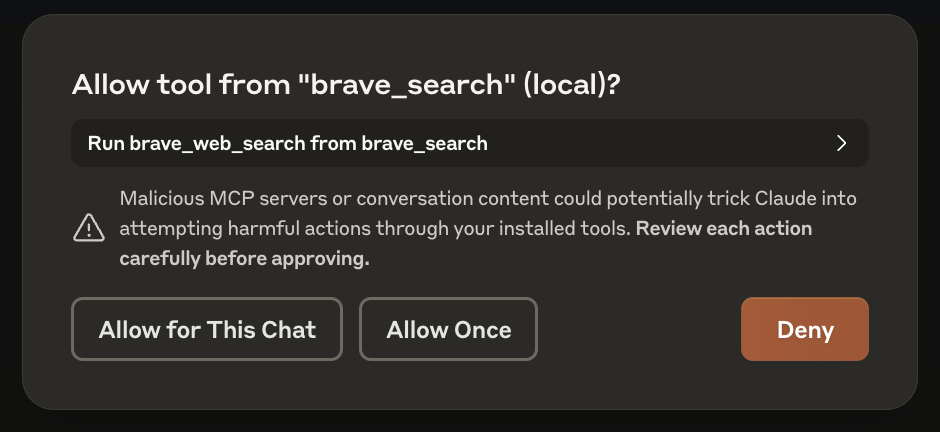
After that, Claude will trigger your MCP server and stream the results directly in the conversation.
Enhance Your AI Automation with Apidog MCP Server Integration
Take your AI-driven workflows even further by integrating with the Apidog MCP Server.
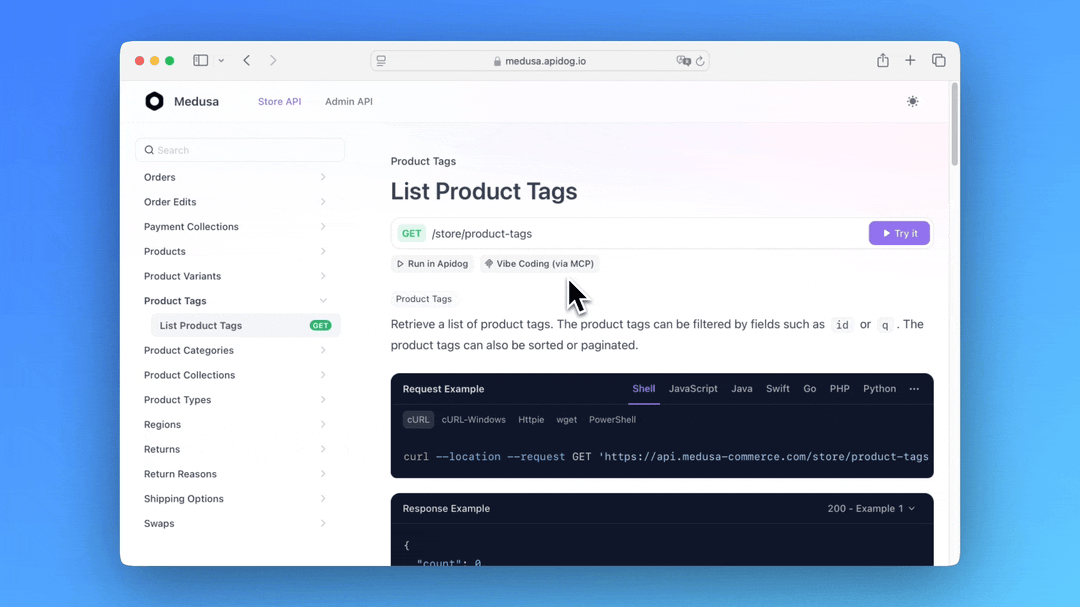
This powerful connection allows your AI assistant to interact directly with API specifications from Apidog projects, enabling seamless API exploration, code generation, and structured model creation.
Whether you're building a simple greeting tool or integrating advanced services like web search, creating your own MCP server in TypeScript gives you full control over how Claude accesses and processes external context—unlocking smarter, more interactive AI experiences..
Message Flow Overview
Here’s what happens under the hood:
1. Initialization
Claude (as host) sends an initialize request with supported protocol version and capabilities.
2. Capability Negotiation
Your server replies with its own capabilities, and the client confirms readiness with an initialized notification.
3. Tool Discovery
Claude sends a ListTools
request. Your server returns a list of tool definitions, including input schemas.
4. Tool Call
When the user triggers the tool (e.g., by typing “greet John”), Claude sends a CallTool
request with arguments.
Your server processes it and returns a result message with the response content.
Step 5: Expand Your Tooling Ecosystem
Once your server is working, you can expand its capabilities:
- Web Search: Integrate Brave Search API
- Filesystem: Secure file reads/writes
- Slack or GitHub Tools: Enable collaboration features
- Google Drive: Attachments and content context
For example, here’s a snippet from a Brave Search server integration:
const WEB_SEARCH_TOOL = {
name: 'brave_web_search',
description: 'Search the web using Brave.',
inputSchema: {
type: 'object',
properties: {
query: { type: 'string' },
count: { type: 'number', default: 10 }
},
required: ['query']
}
};
Transport Options
While Stdio is best for local testing, MCP also supports:
- HTTP + SSE: Good for web apps and remote services
- Custom Transports: Plug in your own transport adapter
All use JSON-RPC 2.0 for message encoding.
Debugging and Testing Tools
You can test your MCP server with the open-source Inspector tool:
git clone https://github.com/modelcontextprotocol/inspector
It allows for message tracing, request simulation, and debugging tool behaviours.
Real-World Use Cases
Some early adopters of MCP include:
- Sourcegraph Cody: Enhances dev context
- Zed Editor: IDE integrations
- Claude Desktop: Local and private LLM operations
These tools demonstrate the power of MCP in both offline and online settings, making it easier for developers to customize how AI understands their context.
Conclusion
MCP is a powerful step forward in bringing structure, scalability, and modularity to the way LLMs interact with tools and data. Whether you're building a personal assistant, an IDE helper, or a data pipeline, MCP offers a future-proof standard to plug in external capabilities.
By following this guide, you’ve learned how to:
- Build a simple MCP server using TypeScript
- Register it with Claude Desktop
- Understand how tool communication flows
- Extend the functionality using additional APIs and services
For developers building the next generation of AI-enhanced apps, mastering MCP is an essential step.
Further Reading and Resources
- Full QuickStart Guide: Model Context Protocol on Glama.ai
- GitHub Repos: MCP Tools